To create a C++ project in Visual Studio, open the IDE, select "Create a new project," choose "Console App," and then write your code in the provided `main.cpp` file.
Here's a simple "Hello, World!" code snippet for your project:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Getting Started with Visual Studio
Downloading and Installing Visual Studio
To begin, you need to download and install Visual Studio, one of the most powerful Integrated Development Environments (IDEs) for C++ development. Visit the [Visual Studio download page](https://visualstudio.microsoft.com/downloads/) and choose the correct edition that suits your needs, such as Community, Professional, or Enterprise. The Community edition is free and suitable for individuals and small teams.
Once the installer is downloaded, follow these steps:
- Launch the installer.
- In the Workloads tab, select Desktop development with C++. This will install the necessary tools and libraries for C++ development.
- Click Install and wait for the installation to complete.
Visual Studio might require additional components or updates, so ensure you're connected to the internet during this process.
Launching Visual Studio
After installation, you can launch Visual Studio by searching for it in your operating system's menu. Familiarize yourself with the Visual Studio interface, which consists of several key components:
- Menu Bar: Contains options like File, Edit, Build, and Debug.
- Solution Explorer: Displays all your projects and files.
- Toolbox: Offers controls and components you can drag into your forms.
- Output Window: Displays build and debugging messages.
Understanding these components is vital for navigating the IDE effectively.
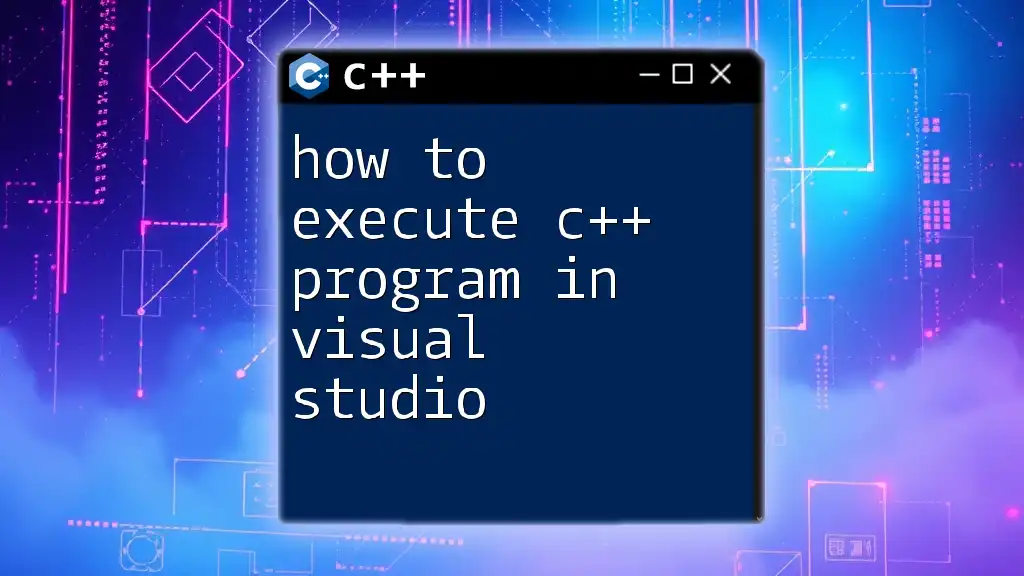
Creating a New C++ Project
Opening the New Project Dialog
To create a C++ project, navigate to the menu bar and select File > New > Project. This will open the New Project dialog box, where you can select the type of project you want to create.
Selecting the C++ Project Template
In the New Project dialog, look for C++ templates. Some common templates include:
- Console Application: Perfect for beginners, this template helps you learn the basics of C++.
- Windows Desktop Application: This template is ideal if you intend to develop GUI applications.
Choose the template that best fits your needs. Taking the time to select the correct template will ease your development experience.
Configuring Project Settings
Naming Your Project
Once you select the template, you'll need to name your project. Choose a descriptive name that adheres to common naming conventions, such as using CamelCase (e.g., `MyFirstCPPProject`). Set a location on your computer where you want to save the project files.
Choosing the Target Framework
The target framework defines the environment your application will run in. For most C++ applications, you might not need to change this, but it’s a good idea to be familiar with the different options available. Make sure you read up on the features and limitations of each to make an informed choice.
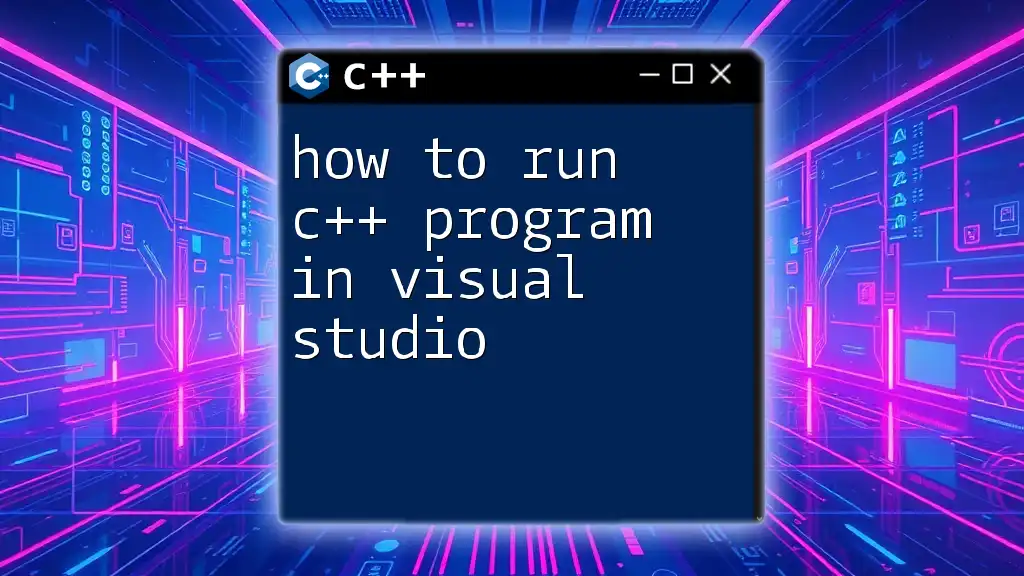
Writing Your First C++ Code
Setting Up the Source File
Once your project is created, navigate to the Solution Explorer on the right side. Here, you can view all project components. Right-click on the Source Files folder, select Add > New Item, and choose C++ File. Name it `main.cpp`—this is conventionally where the entry point of your C++ application will reside.
Writing a Simple C++ Program
Introduction to Main Function
Every C++ application must have a `main` function, which serves as the entry point for execution. Inside the function, you will write your code.
Here’s an example of a simple C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
- `#include <iostream>`: This line includes the Input Output Stream library, which allows you to use `std::cout` for console output.
Compiling the Program
Now that you’ve written your code, it’s time to compile it. Click on Build > Build Solution from the menu bar. This will compile your code and check for any syntax or build errors. Review any messages in the Output Window that may indicate what went wrong if there are errors.
Running Your C++ Application
To run your compiled C++ program, navigate to Debug > Start Without Debugging (or simply press `Ctrl + F5`). The console window should appear displaying "Hello, World!" If everything went well, congratulations! You’ve successfully created your first C++ project in Visual Studio.
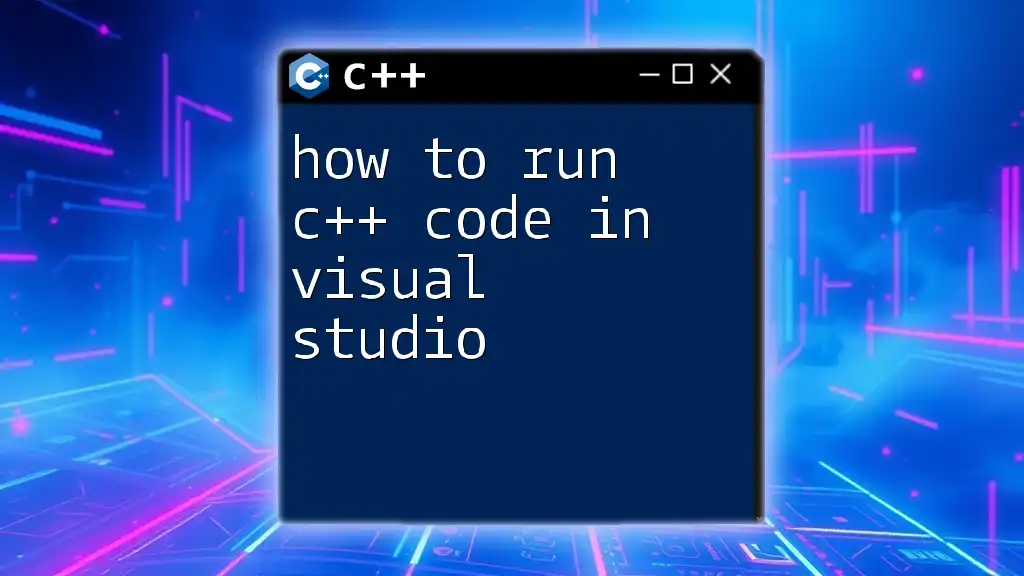
Debugging Your C++ Program
Setting Breakpoints
Debugging is a critical part of the development process. Breakpoints allow you to pause execution at specific lines of code, enabling you to inspect the values of variables and understand the flow of execution.
To set a breakpoint, click in the left margin next to the line you want to examine—this will place a red dot indicating where the program will pause during execution.
Using the Debugger
To begin debugging, navigate to the Debug menu and select Start Debugging (or simply press `F5`). When execution hits a breakpoint, Visual Studio will pause, allowing you to examine variables, line by line. Use the stepping tools such as Step Into (F11) to go into functions or Step Over (F10) to execute functions without going into them.
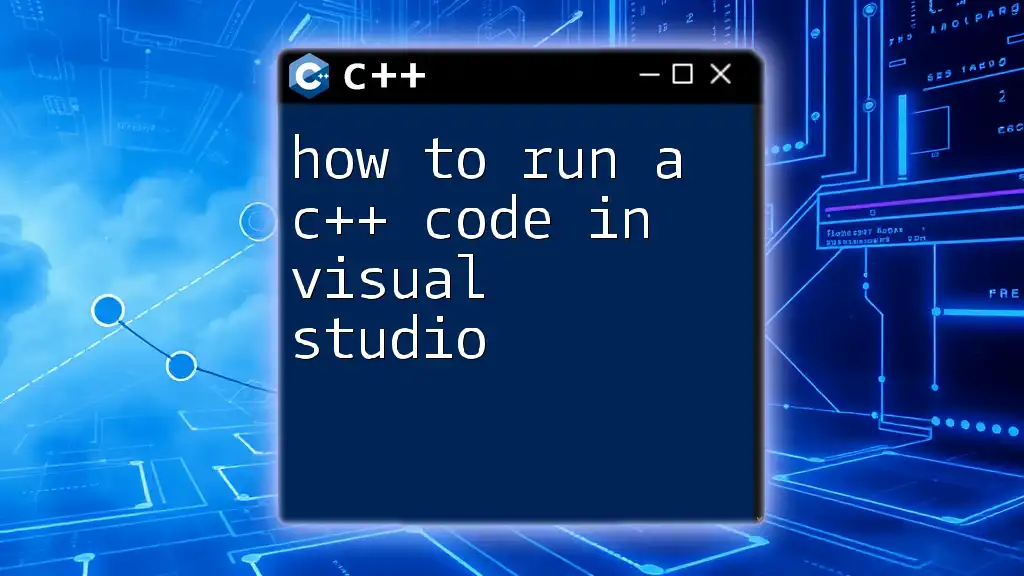
Building and Deploying Your Project
Creating an Executable
To create an executable that can be run outside Visual Studio, you need to build the project. Simply select Build > Build Solution again. Once this process is complete, your executable will be located in a subfolder of your project directory—typically under `Debug` or `Release`, depending on your build configuration.
Running Your Executable
To run your executable, open a command line or command prompt, navigate to the folder containing your `.exe` file, and type the filename to execute your program. For instance:
C:\path\to\your\project\Debug> MyFirstCPPProject.exe
After hitting Enter, you should see the output of your program.
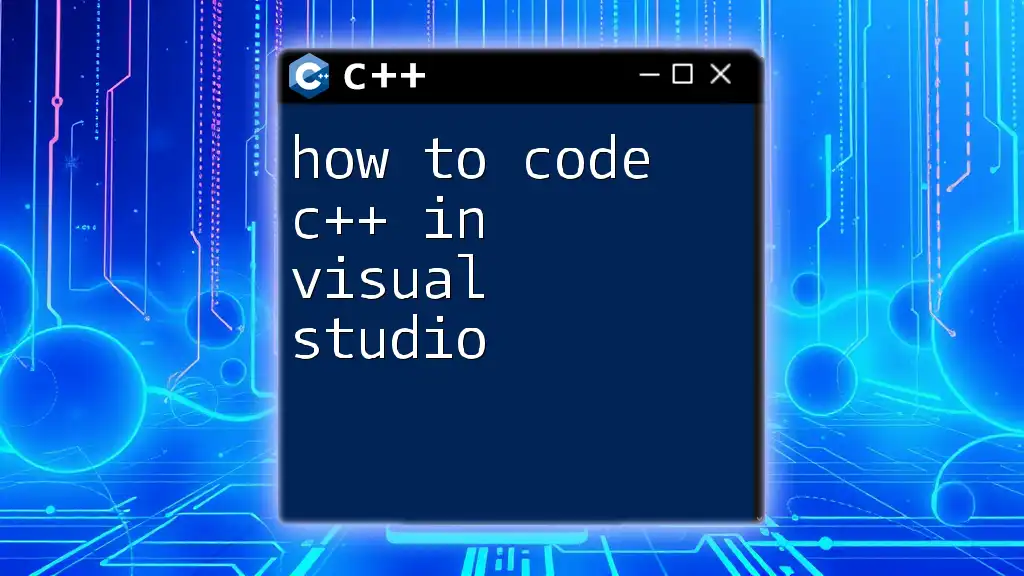
Additional Features in Visual Studio
Integrating with Git
Version control is essential for tracking changes and collaborating with others. Visual Studio integrates seamlessly with Git. To set up a Git repository:
- Navigate to View > Team Explorer.
- Select Manage Connections and click on Connect to a Project.
- You can either create a new repository or clone an existing one.
Customizing Visual Studio
Visual Studio is customizable to suit your workflow. Go to Tools > Options to change themes, fonts, and layouts. You can also install extensions from the Visual Studio Marketplace to enhance functionality—some popular ones include Visual Assist and C++ Code Coverage.
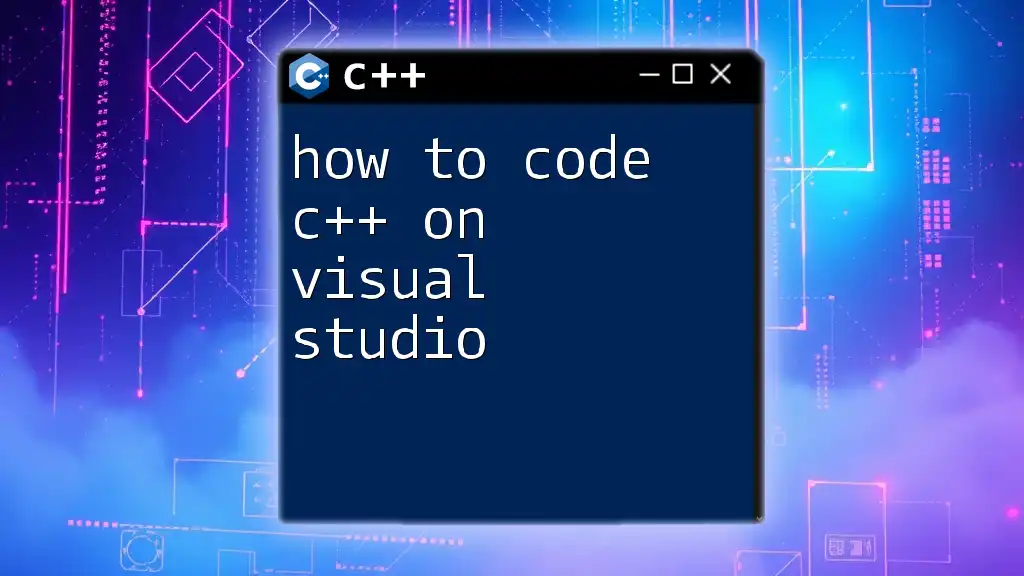
Conclusion
Creating a C++ project in Visual Studio can seem daunting at first, but with this guide, you should feel confident navigating the IDE and building your first application. Remember to explore further as you grow more familiar with C++. The resources available online are vast, and you can delve into specialized topics and advanced C++ concepts as you progress.
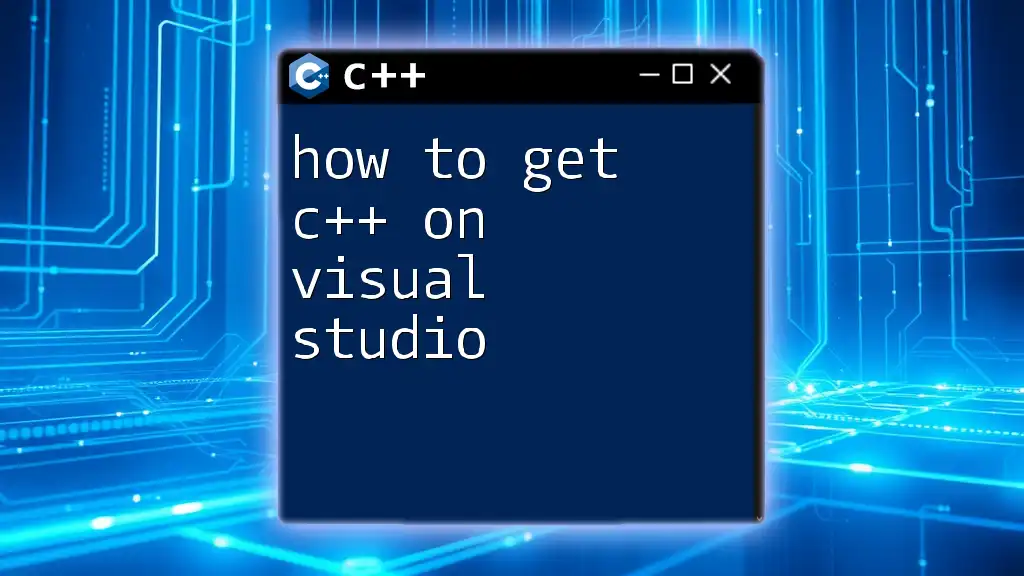
FAQs
Common Questions About Using Visual Studio for C++
Can I use Visual Studio for C++ development on Windows and Mac?
Yes, Visual Studio has a version available for Mac, but it is limited compared to Windows in terms of features. You can explore alternatives such as Visual Studio Code for a cross-platform experience.
How to fix common errors encountered during project creation?
Always check your project template selection and ensure all necessary workloads are installed. If you encounter syntax errors, the Output Window usually gives specific messages pointing to the problematic line of code.
What are some recommended resources for learning C++ after mastering Visual Studio?
Books such as "C++ Primer" by Lippman, LaJoie, and Moo, or online platforms like Codecademy, Udemy, or Coursera offer excellent C++ courses. Furthermore, engaging with communities such as Stack Overflow can greatly enhance your learning experience.
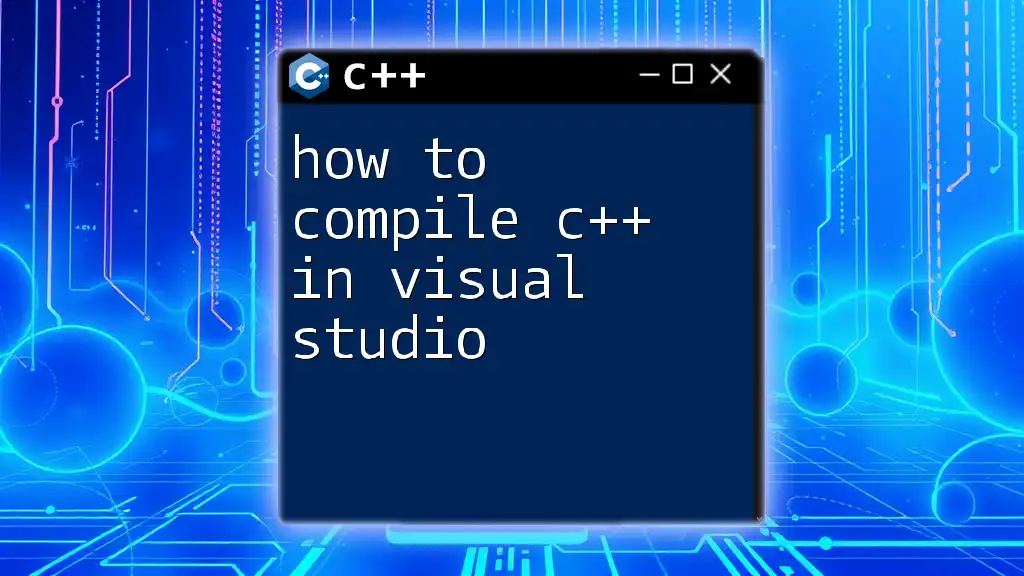
Call to Action
If you found this guide helpful, consider joining our community for more tutorials and resources, or enroll in one of our comprehensive C++ courses to further enhance your programming skills. Happy coding!