To run a C++ file in Visual Studio, simply open the file, select "Debug" from the menu, and click "Start Without Debugging" or press `Ctrl + F5`.
Here's a sample code snippet demonstrating a simple C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Visual Studio
Downloading and Installing Visual Studio
To get started, you’ll need to download and install Visual Studio. The Community version is free and suitable for individual developers and small teams.
- Visit the Visual Studio website: Navigate to the [Visual Studio Download Page](https://visualstudio.microsoft.com/downloads/).
- Select the Community Edition: Click on the Community edition option to begin your download.
- Run the Installer: Once the download is complete, run the installer.
Installing Necessary Components for C++
During the installation process, it’s essential to choose the right components for C++, particularly Visual C++ tooling. Here’s what you should do:
- Check the box for Desktop Development with C++: This option ensures that you have all essential tools and libraries for C++ programming.
- Optional Components: While the defaults are usually sufficient, you can customize your installation by selecting any additional components you may find useful.
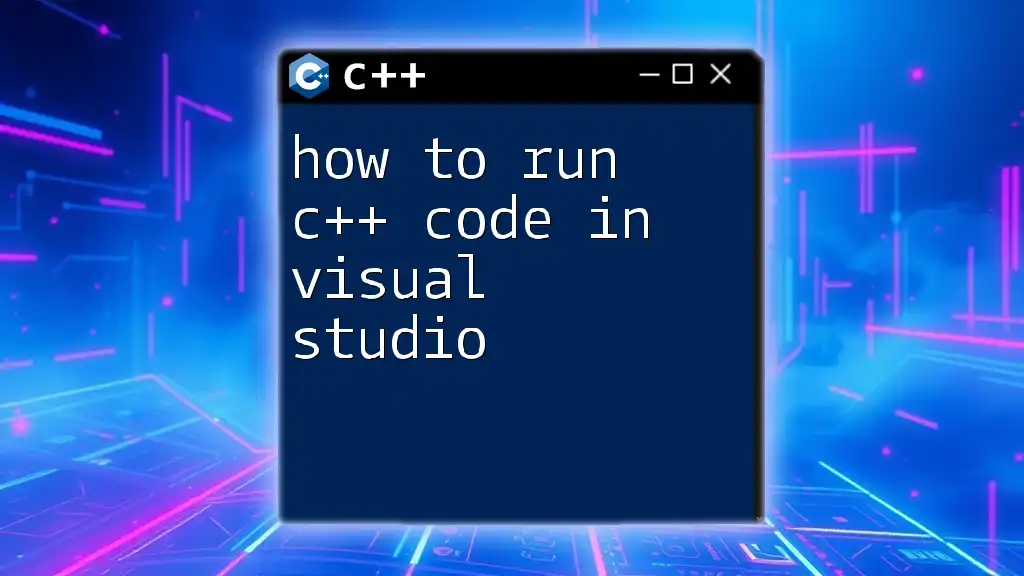
Creating a New C++ Project
Launching Visual Studio
After installation, open Visual Studio by finding it among your applications. The first window you'll encounter is often referred to as the Start Window.
Navigating to Create a New Project
In the Start Window, locate and click on the "Create a new project" button. This will take you to the project templates.
Choosing the Right Project Type
From the project templates, you will want to select a Console Application. This is typically the ideal choice for beginners working in C++.
- Search Filter: You can use the search bar to type "Console App" and quickly find the template you need.
- Make sure to select C++ as the language in the filter options.
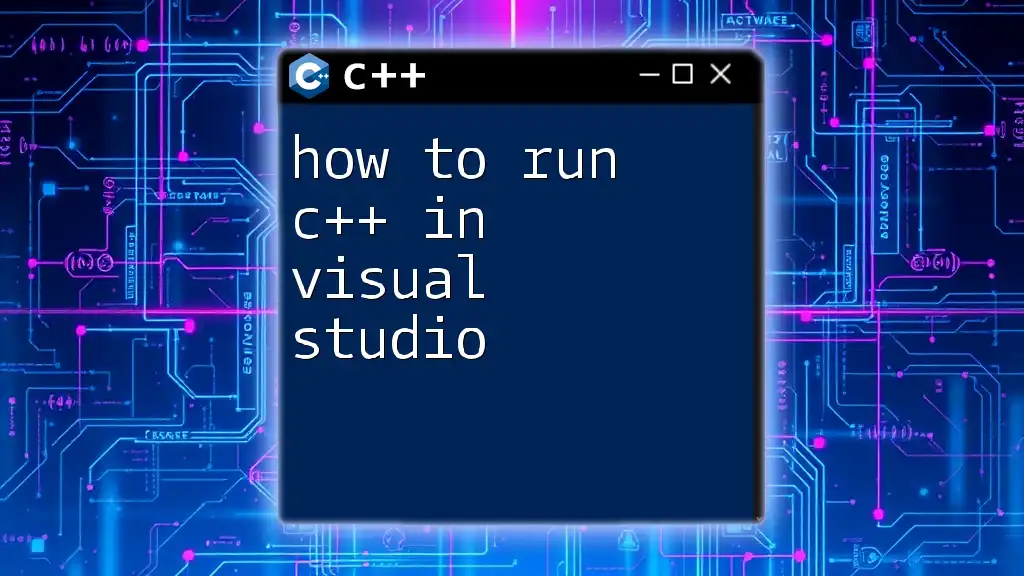
Writing Your First C++ Program
Opening the Source File
Once your project is created, Visual Studio will automatically generate a default source file, often named `main.cpp`. This is where you will write your C++ code.
Writing Simple Code
Now, let’s write a basic C++ program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
- In this example, `#include <iostream>` is a directive that includes the Input/Output stream library, allowing you to use the `cout` command to print text to the console.
- The `int main()` function serves as the entry point of any C++ application, and the `return 0;` statement signals that the program has executed successfully.
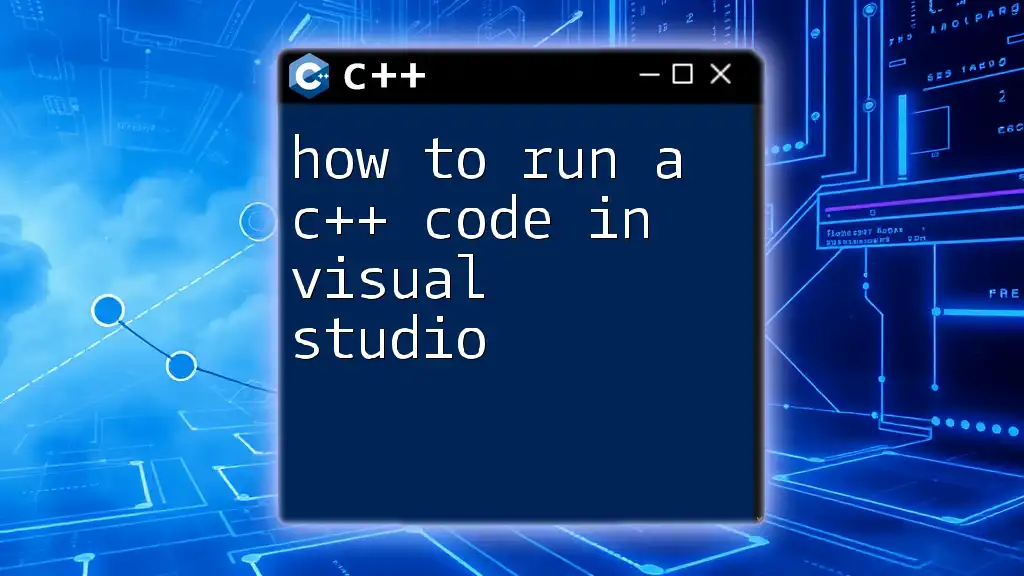
Building the Project
Understanding the Build Process
Building your project is crucial as it translates your C++ code into an executable program. When you build, the compiler checks your code for errors and prepares it for running.
How to Build Your Project in Visual Studio
To build your project in Visual Studio, you can either:
- Use the Shortcut: Press Ctrl + Shift + B to build the solution quickly.
- Go to the Menu: Click on Build in the top menu and then select Build Solution.
The output pane will show any errors encountered during the build process, which you will need to resolve before your program can run.
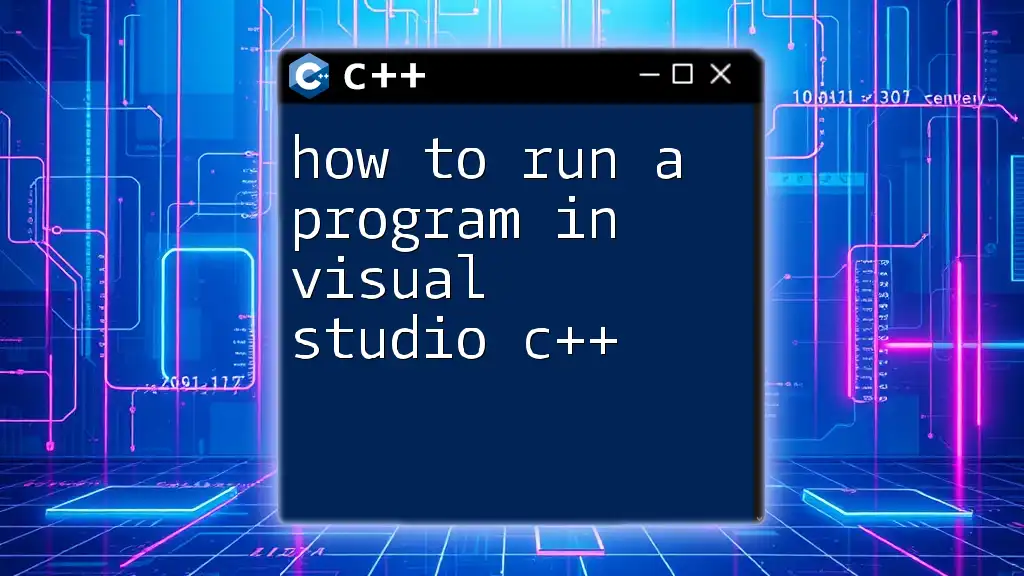
Running the C++ Program
Using the IDE Interface
Once your project is built successfully, you can run it easily.
- Navigate to Debug in the top menu and select Start Without Debugging. This runs your program without stepping through the code.
- Alternatively, you can use the keyboard shortcut Ctrl + F5, which serves the same purpose.
Checking Output
After executing the program, check the console window that opens. If you wrote the "Hello, World!" example, you will see this message printed out.
It’s important to note that once the program finishes running, the console window may disappear quickly. To keep it open, use the Start Debugging option instead (which runs with F5).
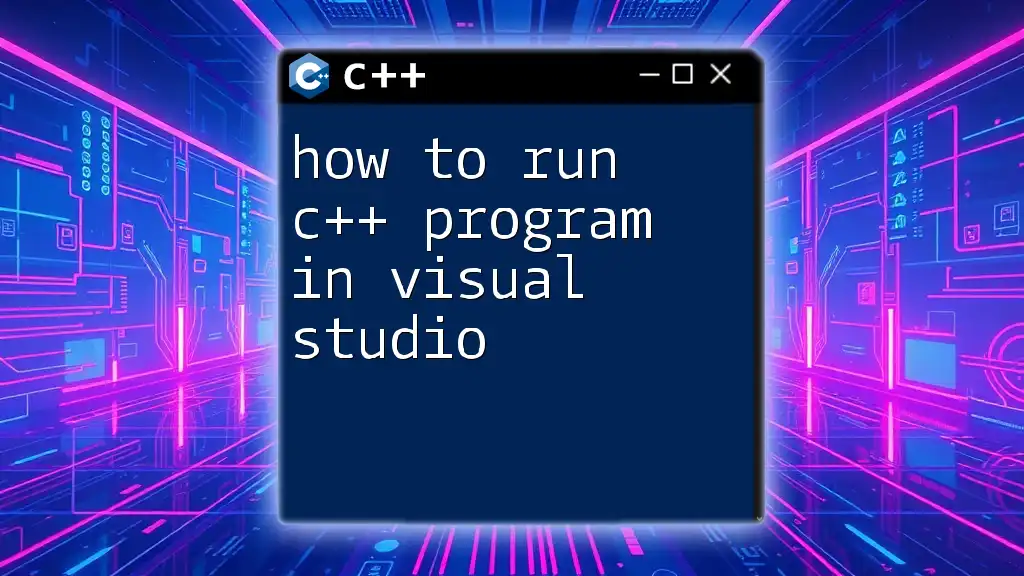
Debugging Your C++ Program
What is Debugging?
Debugging is the process of identifying and resolving bugs or issues within your code. It’s a vital skill that every programmer should develop.
Setting Up Debugging in Visual Studio
Visual Studio offers powerful debugging tools. To set up debugging:
- Set a Breakpoint: Click on the left margin next to the line number where you want to pause execution. A red dot will appear, indicating a breakpoint.
- Start Debugging: Begin debugging with F5. The program will halt at the breakpoint, allowing you to examine variables and step through your code line by line.
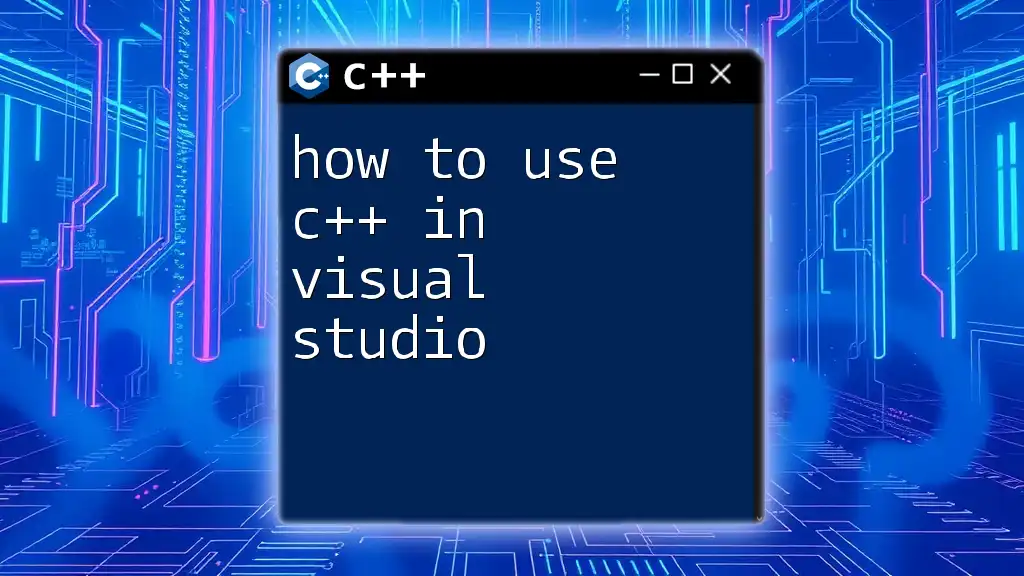
Common Issues and Troubleshooting
Compilation Errors
Errors may arise during the build process. Common compilation errors include:
- Syntax Errors: Misspelled keywords, missing semicolons, or unmatched braces.
- Undefined Variables: Using variables that have not been declared.
Read the error message carefully; it typically includes the file name and line number where the issue occurred.
Runtime Issues
Common runtime issues include accessing invalid memory or division by zero. To troubleshoot:
- Use debugging techniques such as breakpoints and variable watching to identify where your program behaves unexpectedly.
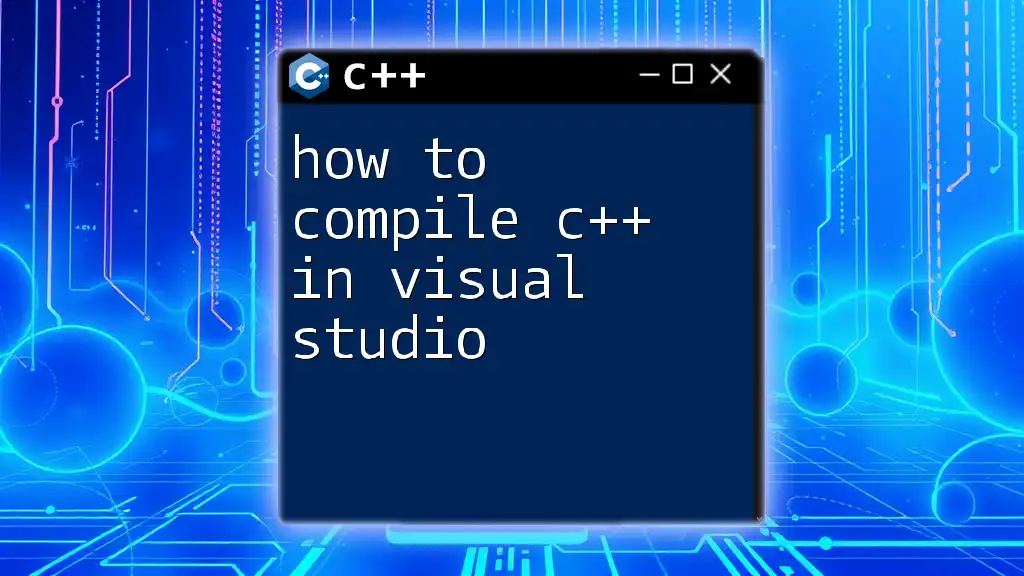
Conclusion
In this guide, we explored how to run a CPP file in Visual Studio, from downloading and installing the IDE to creating a project, writing code, and troubleshooting common issues.
The key takeaway is to practice regularly and explore the various features of Visual Studio as you become more comfortable with C++. With time and experience, you will become proficient in running C++ programs and developing complex applications.
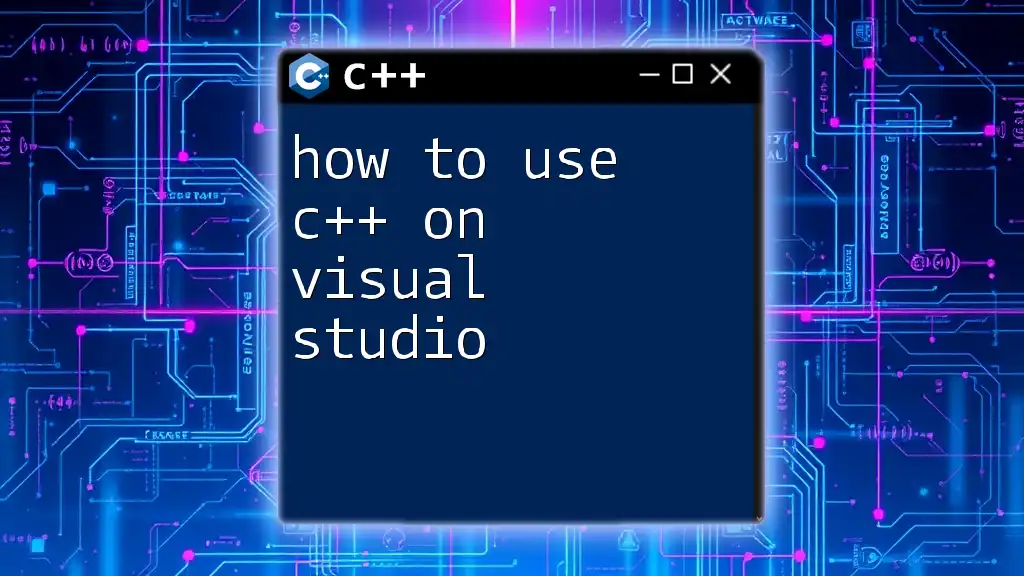
Additional Resources
For further reading, you can check out the official Visual Studio documentation and engage with online C++ community forums for more support and tips.
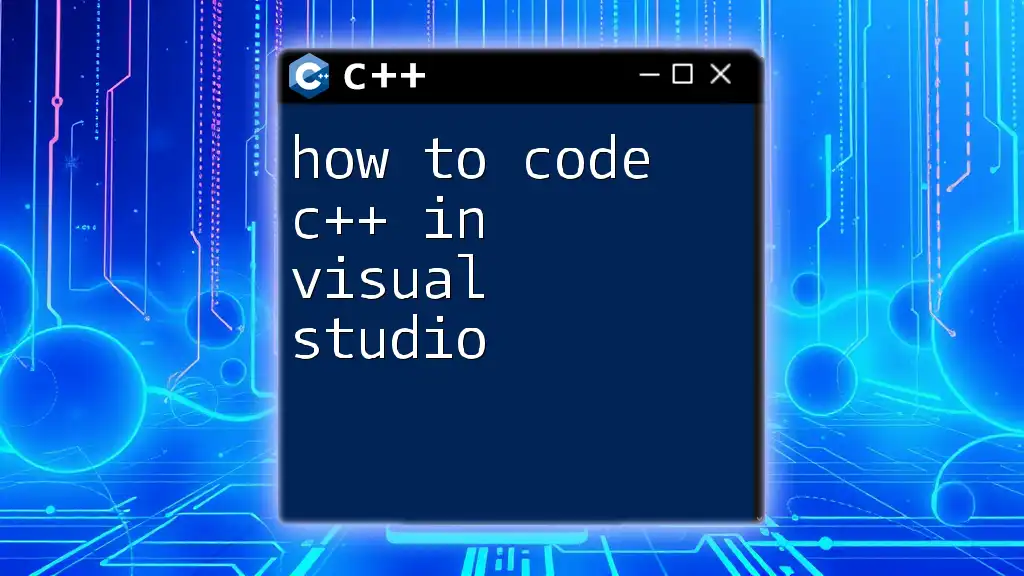
Call to Action
Now that you know how to run a CPP file in Visual Studio, it's time to dive into your own projects! Start coding today, experiment with different functionalities, and enjoy the process of learning C++. Happy coding!