To debug C++ in Visual Studio Code, set breakpoints in your code, utilize the integrated terminal to build your program, and launch the debugger to step through the execution.
Here’s a code snippet that illustrates a simple C++ program to debug:
#include <iostream>
int main() {
int a = 10;
int b = 0;
// Set a breakpoint on the line below to check the value of b
std::cout << "Enter a number: ";
std::cin >> b;
// Potential division by zero error
std::cout << "Result: " << a / b << std::endl;
return 0;
}
Setting Up Visual Studio Code for C++ Development
Installing Visual Studio Code
To get started with debugging C++ in Visual Studio Code, the first step is to install the editor itself. Visit the official Visual Studio Code website to download the appropriate version for your operating system. After installation, consider adding the following essential extensions to enhance your C++ experience:
- C/C++: This extension provides rich language support, including IntelliSense and debugging capabilities.
- CMake Tools: If you are using CMake for your projects, this extension simplifies the management of builds.
- Code Runner: A handy tool to execute code snippets quickly from the editor.
Installing C++ Compiler
A C++ compiler is crucial for compiling your code. The choice of compiler depends on your operating system:
-
Windows: You can use MinGW or Microsoft Visual C++ (MSVC). For MinGW, download the installer, and during installation, select the "C++ Compiler" option. If you prefer MSVC, install Visual Studio Community Edition, which includes the necessary components.
-
Mac: To install the C++ compiler, you can easily set it up by installing the Xcode Command Line Tools. You can initiate this via the terminal with the command `xcode-select --install`.
-
Linux: Most Linux distributions have the GNU Compiler Collection (GCC) readily available. You can install it using your package manager. For example, on Ubuntu, run `sudo apt install g++`.
Configuring Visual Studio Code for C++
Once your compiler is installed, it's time to configure Visual Studio Code:
-
Editing `settings.json`: Adjust the built-in settings to accommodate C++. Open the command palette (`Ctrl + Shift + P`), type in "Preferences: Open Settings (JSON)", and add compiler path configurations as required.
-
Creating tasks.json: This JSON file specifies how to build the project. Here’s a simple example:
{ "version": "2.0.0", "tasks": [ { "label": "build", "type": "shell", "command": "g++", "args": [ "-g", "${file}", "-o", "${fileDirname}/${fileBasenameNoExtension}" ], "group": { "kind": "build", "isDefault": true }, "problemMatcher": ["$gcc"] } ] }
-
Crafting launch.json: This file helps in configuring the debugger. You can create it by clicking on the Debug icon on the sidebar and selecting "create a launch.json file." Here's how it looks:
{ "version": "0.2.0", "configurations": [ { "name": "C++ Launch", "type": "cppdbg", "request": "launch", "program": "${fileDirname}/${fileBasenameNoExtension}", "args": [], "stopAtEntry": false, "cwd": "${workspaceFolder}", "environment": [], "externalConsole": false, "MIMode": "gdb", "setupCommands": [ { "description": "Enable pretty-printing for gdb", "text": "-enable-pretty-printing", "ignoreFailures": true } ], "preLaunchTask": "build", "miDebuggerPath": "/usr/bin/gdb", "miDebuggerArgs": "" } ] }
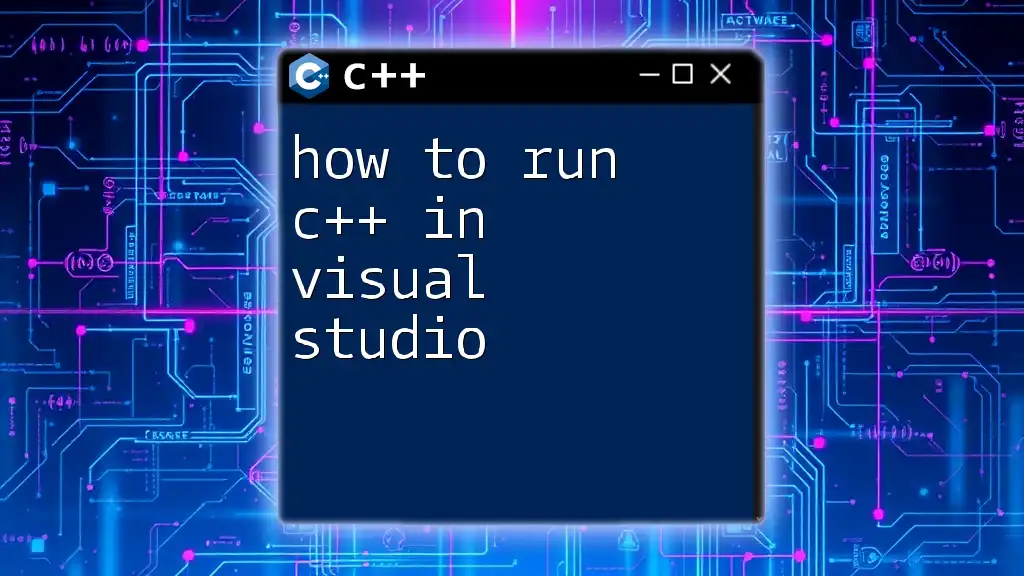
Understanding the Debugging Interface
Overview of the Debugger
The debugger in Visual Studio Code provides an intuitive interface for tracking down issues in your code. It comprises several panels, including:
- Variables: Displays the current values of all local variables.
- Watch: Allows you to monitor specific expressions or variables' values.
- Call Stack: Shows the sequence of function calls leading to a breakpoint.
- Debug Console: Where you can evaluate expressions and interact with the debugger.
Setting Breakpoints
Breakpoints are placeholders in your code where execution will pause, allowing you to inspect the current state. To set a breakpoint, click in the gutter next to the line numbers. For example, consider the following simple C++ program:
#include <iostream>
int main() {
int x = 10; // Set breakpoint here
std::cout << "Value of x: " << x << std::endl;
return 0;
}
Setting a breakpoint on the line `int x = 10;` allows you to monitor how the value of `x` changes.
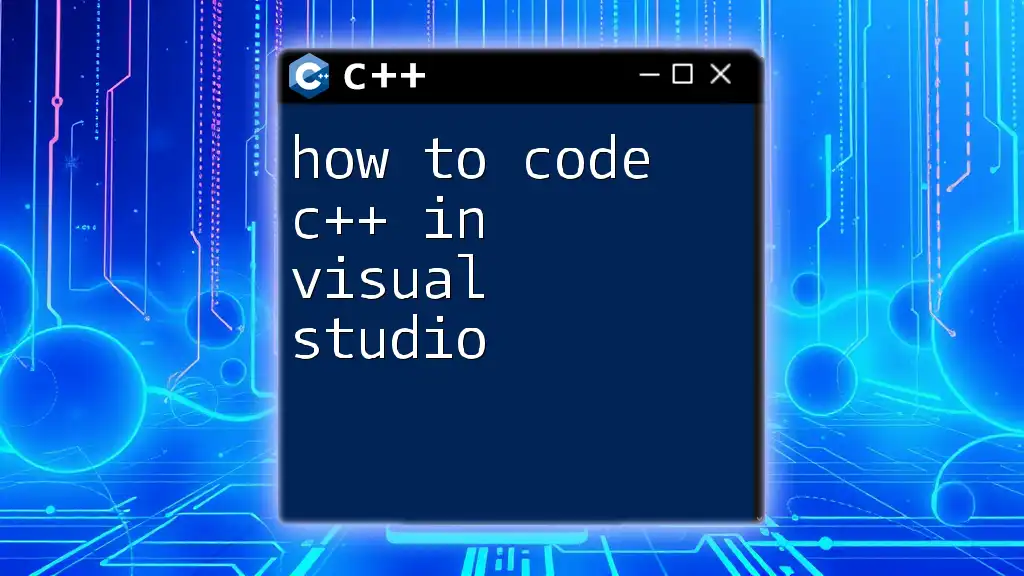
Starting a Debugging Session
Building the C++ Project
Before initiating the debugger, ensure your project is compiled without errors. Use the build task configured in `tasks.json` to compile the project. Verify for any compiler errors that might impede the debugging process.
Launching the Debugger
To begin debugging, click on the debug icon or use the shortcut (`F5`). Select your debug configuration if prompted. This will start the debugger and automatically pause at any defined breakpoints.
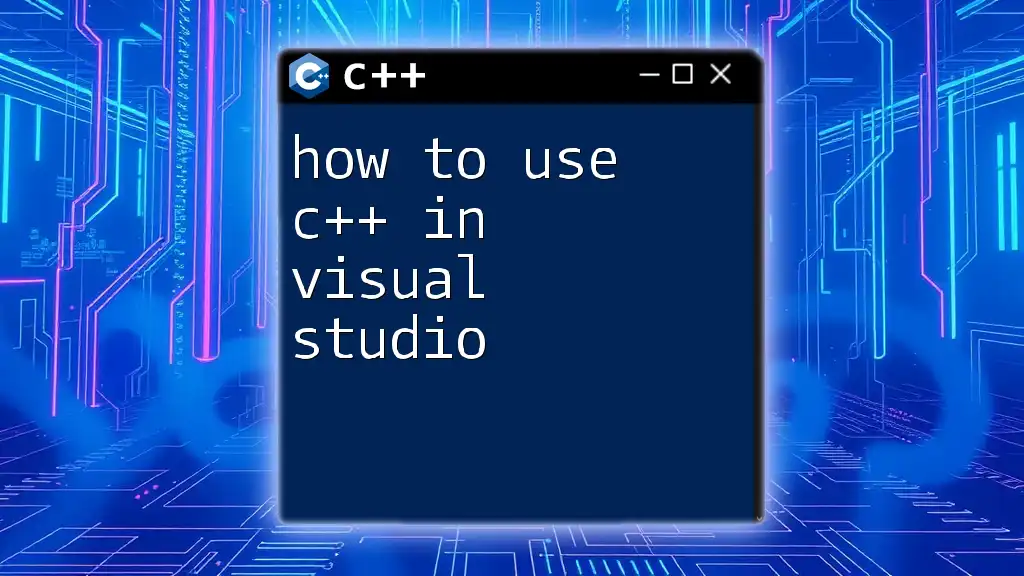
Navigating Through Your Code During Debugging
Using Step Over, Step Into, and Step Out
These commands are crucial for controlling program execution during debugging:
- Step Over: Executes the next line of code without entering any function calls.
- Step Into: If the next line is a function call, this command will take you into that function.
- Step Out: Used to finish the execution of the current function and return to its caller.
For instance, given this code snippet:
#include <iostream>
void myFunction(int y) {
std::cout << "The value is " << y << std::endl;
}
int main() {
int x = 10;
myFunction(x); // Step into here
return 0;
}
If you Step Into the `myFunction`, you can analyze how the function processes the variable `y`.
Monitoring Variables and Expressions
The variables panel displays the current values of your variables as you step through the code. You can also add specific variables to the watch list by right-clicking them in the editor and selecting Add to Watch. This is beneficial for keeping track of expressions that are critical to your debugging process.
Evaluating Expressions
The debug console allows you to evaluate expressions dynamically. You can check the value of a variable at any point during execution. For example, if you type `x` into the console during a breakpoint, you will immediately see its current value.
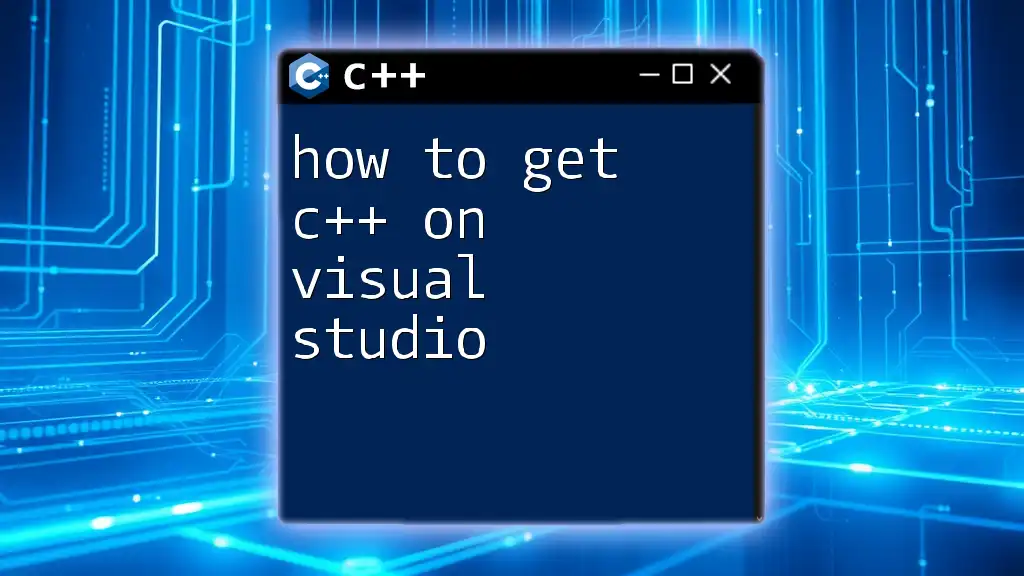
Advanced Debugging Techniques
Conditional Breakpoints
These breakpoints activate only when specified conditions are met, making them powerful for debugging loops or frequent function calls. Set a conditional breakpoint by right-clicking an existing breakpoint and selecting "Edit Breakpoint." An example:
#include <iostream>
int main() {
for (int i = 0; i < 100; i++) {
if (i == 50) { // Set conditional breakpoint here
std::cout << "Reached halfway!" << std::endl;
}
}
return 0;
}
The breakpoint will only trigger when the loop index `i` reaches 50.
Exception Breakpoints
Exception breakpoints allow you to pause execution when an exception is thrown. This is particularly useful for debugging code that might not handle errors gracefully. Configure this in the breakpoints panel by selecting "Add Exception Breakpoint."
Performance Profiling
While debugging focuses on code errors, performance profiling helps identify bottlenecks. Use tools and extensions such as the C/C++ Profiler to gain insights into execution time and resource usage. Regular profiling can significantly enhance application performance.
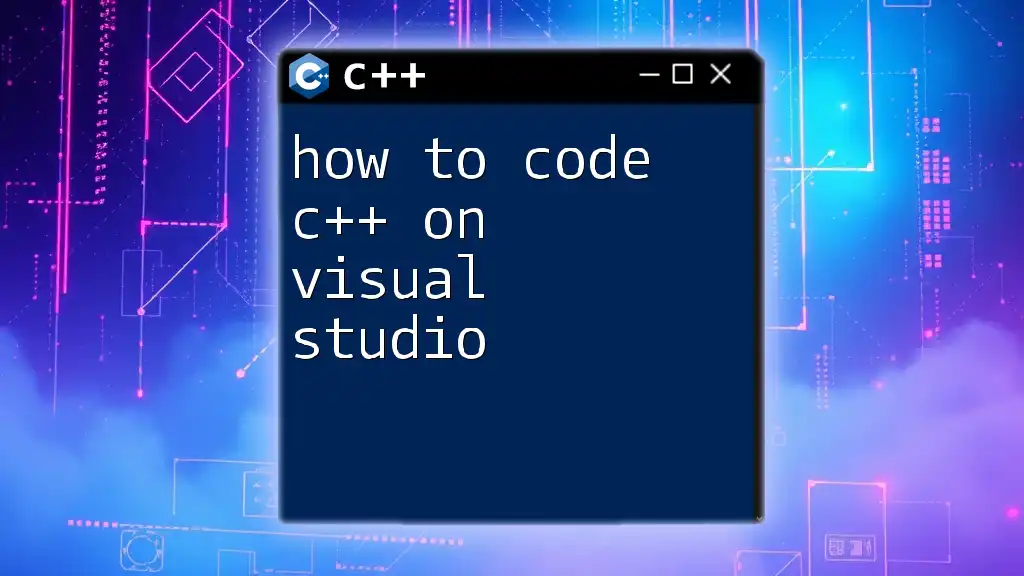
Common Debugging Mistakes and How to Avoid Them
Forgetting to Rebuild After Changes
After making any changes to your code, it's crucial to rebuild your project. Failing to do so results in running outdated binaries that may not accurately reflect the current code state.
Ignoring Compiler Warnings
Compiler warnings can provide valuable insights into potential issues. Configure Visual Studio Code to show compiler warnings prominently, and make sure to address them as part of your debugging routine.
Not Using the Debugger's Full Capabilities
Many developers overlook the powerful features of the debugger. Invest time in exploring various debugging tools within Visual Studio Code, including the call stack and watch expressions.
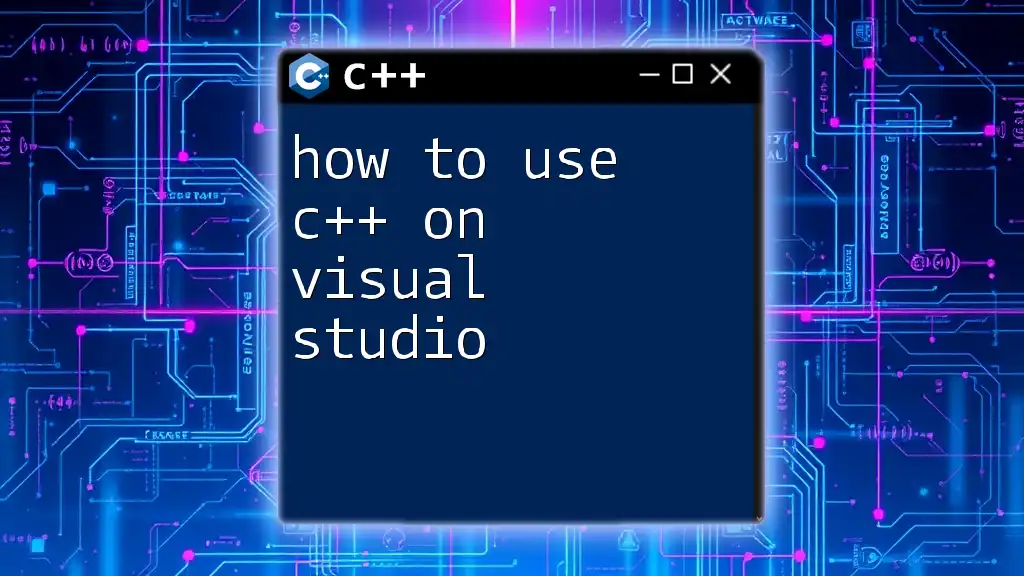
Conclusion
Effective debugging is an essential skill for any C++ developer, and Visual Studio Code provides a robust environment for mastering this process. By setting up your workspace correctly, leveraging the debugging tools, and following best practices, you can enhance your debugging efficiency dramatically. Explore your own workflow and keep experimenting with the features available in Visual Studio Code. Your debugging journey has just begun!
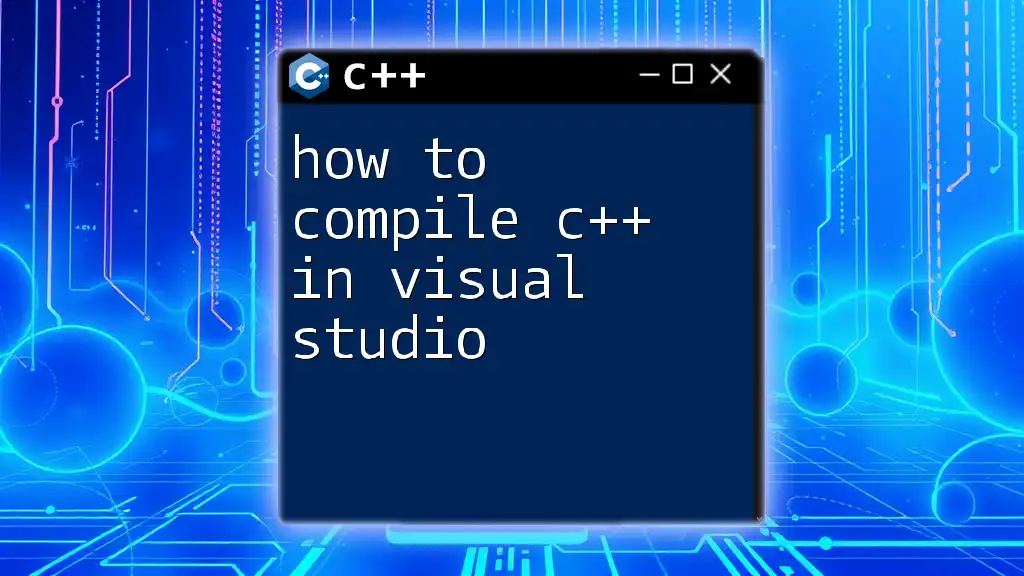
Additional Resources
For more in-depth knowledge, check out the [official Visual Studio Code documentation](https://code.visualstudio.com/docs). Engage with community forums and consider diving into recommended C++ debugging books to elevate your understanding further.