To set up Visual Studio Code for C++, install the C++ extension, configure the build tasks, and create a basic `main.cpp` file for compiling and running your code seamlessly.
Here's a simple example of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Visual Studio Code?
Visual Studio Code (VS Code) is a lightweight yet powerful source code editor developed by Microsoft. It provides an excellent environment for coding, equipped with features that enhance productivity and streamline the software development process. Notable features of VS Code include:
- Extensions: A vast marketplace allows you to install plugins that enhance functionality.
- Debugging: Built-in debugging tools help identify and fix issues in your code quickly.
- Integrated Terminal: Run scripts and commands directly within the editor.
The versatility of VS Code makes it an ideal choice for C++ development due to its ease of use, extensive support, and customization options that cater specifically to C++ programming needs.
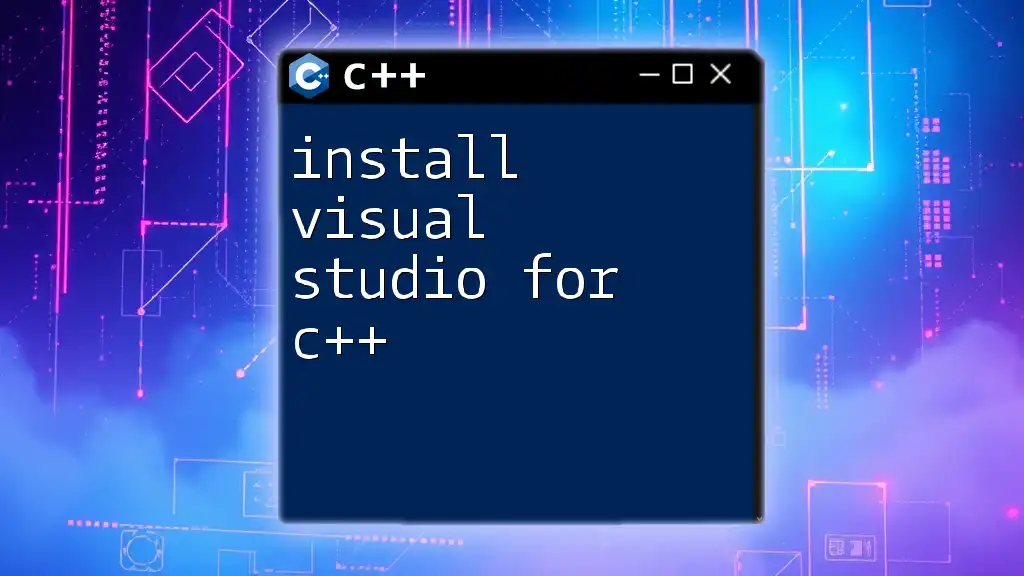
How to Install Visual Studio Code
To begin with, you must install Visual Studio Code. Here’s a straightforward guide on how to do this across several operating systems:
Step-by-Step Installation Guide
-
Download Visual Studio Code
- Visit the [official website](https://code.visualstudio.com/).
- Select the installer appropriate for your operating system (Windows, macOS, or Linux).
-
Installation Process
- On Windows, run the downloaded `.exe` file and follow the installation prompts.
- For macOS, drag the VS Code application to your Applications folder.
- On Linux, you may use a package manager (like APT for Ubuntu) to install VS Code, or you can download a `.deb` or `.rpm` package from the website.
Launching VS Code for the First Time
Upon launching VS Code for the first time, you will encounter a welcome page. Here, you can customize the editor's preferences according to your workflow. Familiarize yourself with the user interface, including the sidebar, the editor window, and the integrated terminal.
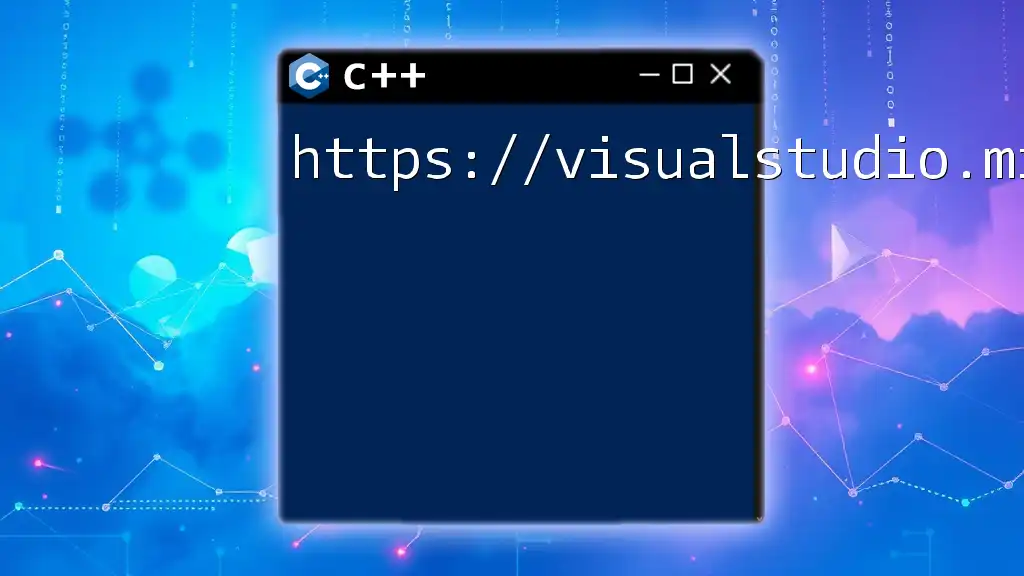
Setting Up Your C++ Environment
To effectively write C++ code in VS Code, you will need a C++ compiler.
Installing C++ Compiler
A C++ compiler converts your code into executable files. The most common options are:
-
Windows: Install MinGW, which includes the `g++` compiler.
-
macOS: Install Xcode command line tools by running `xcode-select --install` in your terminal.
-
Linux: Use your distribution's package manager to install `g++`. For example, on Ubuntu, run:
sudo apt install g++
Adding C++ Compiler to System PATH
Adding your compiler to the system PATH is crucial as it allows you to run C++ commands from any terminal or command prompt.
-
Windows:
- Right-click on "This PC" or "My Computer" and select "Properties."
- Click on "Advanced system settings," then "Environment Variables."
- In the "System variables" section, find the "Path" variable, and click "Edit."
- Add the path to your MinGW `bin` directory (commonly `C:\MinGW\bin`).
-
macOS/Linux:
- Open your terminal and run the following command to edit the profile file (e.g., `.bashrc`, `.zshrc`):
nano ~/.bashrc
- Add the following line:
export PATH="$PATH:/usr/local/bin/g++" # Adjust the path based on your setup
- Save the file (CTRL + X, then Y to confirm), and run `source ~/.bashrc` to apply the changes.
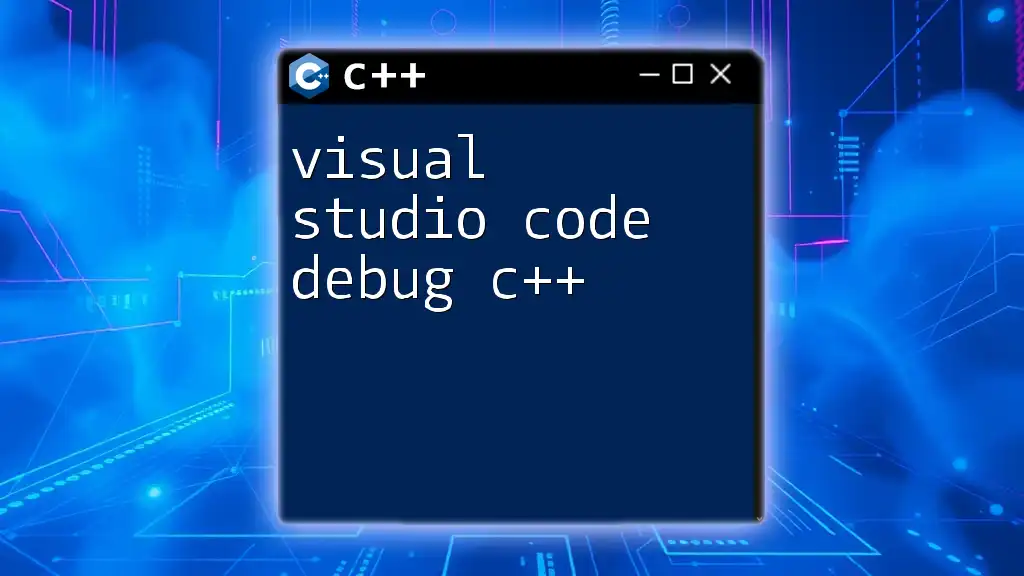
Configuring VS Code for C++
Installing Essential Extensions
To enhance your VS Code experience for C++, certain extensions must be installed:
C/C++ Extension by Microsoft
This vital extension offers a variety of features, including IntelliSense (code suggestions), debugging support, and file navigation.
- To install, go to the Extensions view by clicking the square icon in the sidebar or pressing `Ctrl + Shift + X`. Search for "C/C++" and click "Install."
CMake Tools Extension (Optional)
If you are working on larger C++ projects, CMake Tools can help you manage and build your projects more effectively.
- Install it similarly through the Extensions view.
Creating Your First C++ Project
Now that your environment is set up, creating a new C++ project is straightforward.
-
Create a New Project Directory
- Open your terminal and create a directory for your project. For example:
mkdir HelloWorld cd HelloWorld
-
Create a New C++ File
- Inside your project folder, create a new file named `main.cpp` and open it in VS Code:
code main.cpp
-
Write Your Program
- Enter a simple C++ code snippet:
#include <iostream> int main() { std::cout << "Hello, World!" << std::endl; return 0; }
-
Save the File
- Make sure to save the file (`Ctrl + S`).
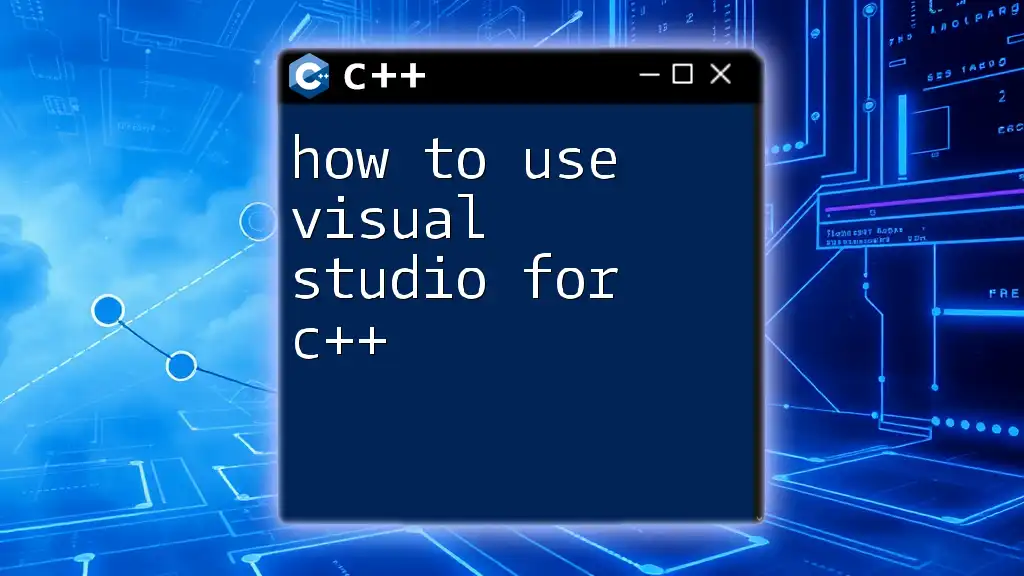
Configuring Build and Debugging
To compile and debug your C++ applications, you will need to set up tasks and configurations in VS Code.
Configuring Tasks in VS Code
Tasks in VS Code allow you to automate commands, including compiling your C++ code.
- Navigate to the Command Palette by pressing `Ctrl + Shift + P` and type “Tasks: Configure Task.”
- Select “Create tasks.json file from template” and then choose “Others.”
- Replace the generated JSON with the following configuration to create a build task:
{
"version": "2.0.0",
"tasks": [
{
"label": "build",
"type": "shell",
"command": "g++",
"args": ["-g", "*.cpp", "-o", "output"],
"group": {
"kind": "build",
"isDefault": true
}
}
]
}
Setting Up Debug Configuration
To debug your C++ programs, you need to create a launch configuration.
- Open the Command Palette again with `Ctrl + Shift + P` and type “Debug: Open launch.json”.
- Select the C++ (GDB/LLDB) option, which will create a default `launch.json` file. Modify it to match this configuration:
{
"version": "0.2.0",
"configurations": [
{
"name": "Debug C++",
"type": "cppdbg",
"request": "launch",
"program": "${workspaceFolder}/output",
"args": [],
"stopAtEntry": false,
"cwd": "${workspaceFolder}",
"environment": [],
"externalConsole": false,
"MIMode": "gdb",
"setupCommands": [
{
"description": "Enable pretty-printing for gdb",
"text": "-enable-pretty-printing",
"ignoreFailures": true
}
],
"preLaunchTask": "build",
"miDebuggerPath": "path/to/gdb" // Update with your gdb path
}
]
}
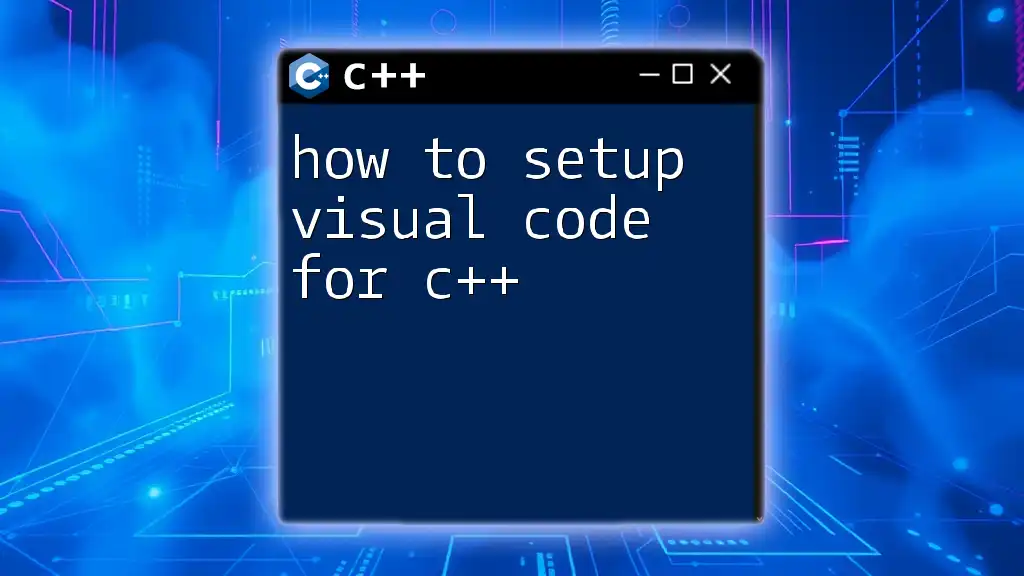
Additional Resources
Once you've set up your environment, it’s beneficial to further enhance your skills. Here are some recommended resources:
- Visit the [official VS Code documentation](https://code.visualstudio.com/docs) for in-depth guidance on using extensions and customizing your environment for C++.
- Explore free online tutorials and communities like Stack Overflow or dedicated C++ forums to ask questions and share knowledge.
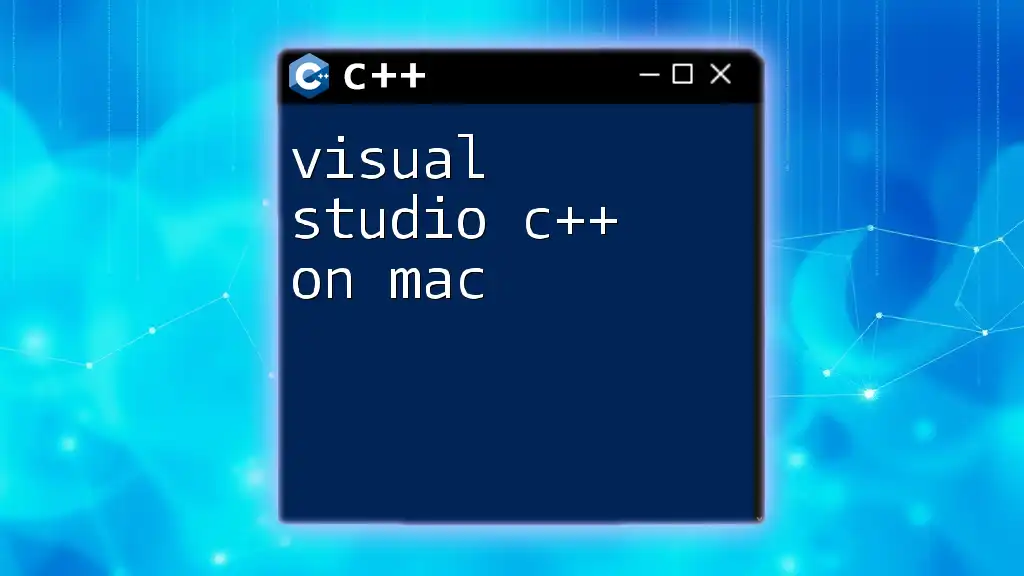
Conclusion
Setting up Visual Studio Code for C++ may seem challenging at first, but the effort pays off greatly as you unlock numerous features that facilitate efficient coding, testing, and debugging. With this guide, you should be well on your way to embarking on your C++ development journey. Don't hesitate to explore and experiment with different extensions and configurations to tailor your coding environment to your needs.
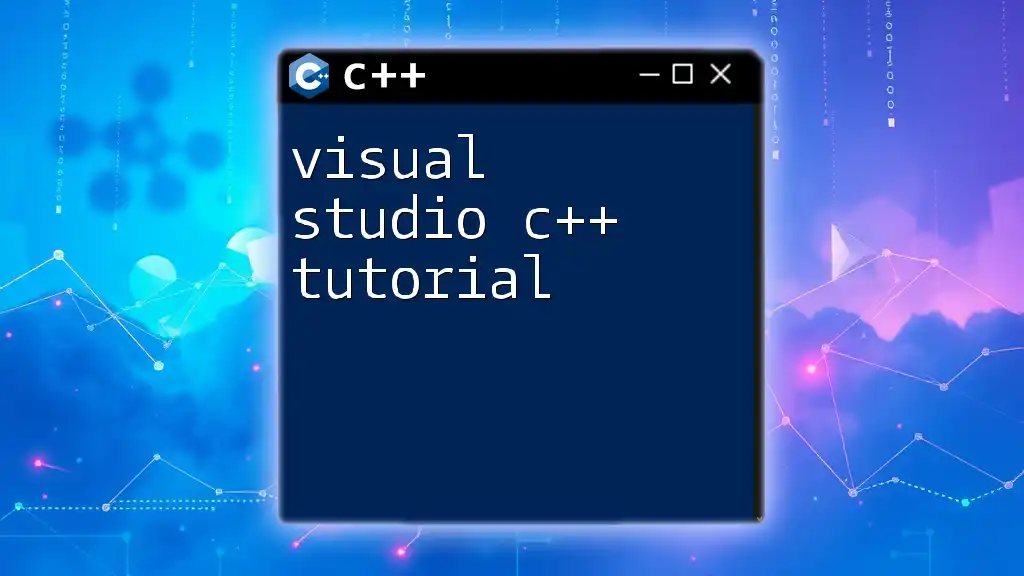
FAQs
What if I encounter issues with debugging?
Try verifying that your paths are correctly set up in the `launch.json` and that g++ is installed properly.
Can I use other compilers with VS Code?
Absolutely! VS Code supports various compilers, so you can use whichever one you prefer, provided it is set correctly in your task and launch configurations.