In C++, a function is a block of code that performs a specific task and can be called independently, while a method is a function that is associated with an object and can access its data members.
Here's a code snippet illustrating both concepts:
#include <iostream>
using namespace std;
// Function
void displayMessage() {
cout << "Hello from a function!" << endl;
}
class MyClass {
public:
// Method
void showMessage() {
cout << "Hello from a method!" << endl;
}
};
int main() {
displayMessage(); // Calling the function
MyClass obj;
obj.showMessage(); // Calling the method
return 0;
}
Understanding Functions in C++
Definition of a Function
In C++, a function is a block of code designed to perform a specific task. Functions can operate independently and encapsulate a set of operations that can take inputs (parameters) and produce outputs (return values). This reusability makes them a fundamental component of C++ programming.
Structure of a Function
A function is defined with a specific syntax that includes various key components. Here's a breakdown of what a C++ function looks like:
returnType functionName(parameters) {
// function body
}
- returnType: Specifies what type of value the function will return (e.g., `int`, `void`, `double`).
- functionName: The identifier used to call the function.
- parameters: A list of variables that function as inputs. Parameters are optional.
- function body: Contains the executable code that defines the function’s behavior.
Example of a Function
Let’s consider a simple example where we define a function that adds two integers:
int add(int a, int b) {
return a + b;
}
This function `add` takes two integer parameters, `a` and `b`, and returns their sum.
Calling a Function
To utilize the function, you simply call it by its name and provide the required arguments:
int result = add(5, 3);
In this case, `result` will hold the value `8`, demonstrating the function’s capability to process input and return output.
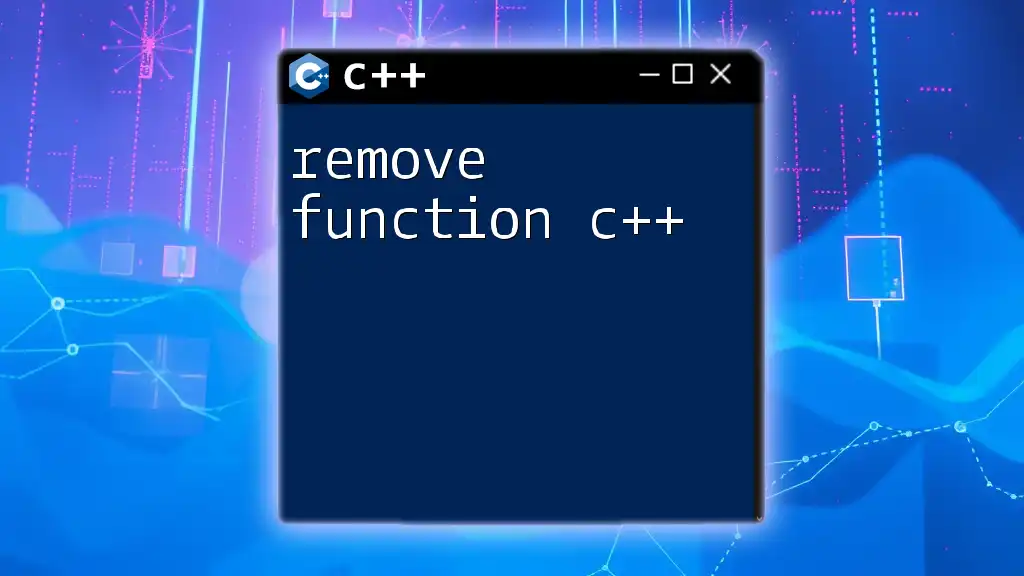
Understanding Methods in C++
Definition of a Method
A method is similar to a function, but it is associated with a class in C++ — a structure that encapsulates data and behavior. Methods are fundamentally linked with the concept of object-oriented programming (OOP), allowing for more organized and modular code.
Structure of a Method
A method is structured slightly differently than a function due to its association with a class. The syntax for defining a method is as follows:
returnType ClassName::methodName(parameters) {
// method body
}
- ClassName: Indicates which class the method belongs to.
- The rest of the structure is similar, including the return type, method name, parameters, and the method’s body.
Example of a Method
Here’s an example demonstrating a simple class with a method:
class Calculator {
public:
int add(int a, int b) {
return a + b;
}
};
In this example, `add` is a method of the `Calculator` class, and it operates similarly to the earlier function example.
Calling a Method
To call a method, you must first create an object of the class:
Calculator calc;
int result = calc.add(5, 3);
This time, `result` will also hold `8`, showing the importance of the object context in method invocation.
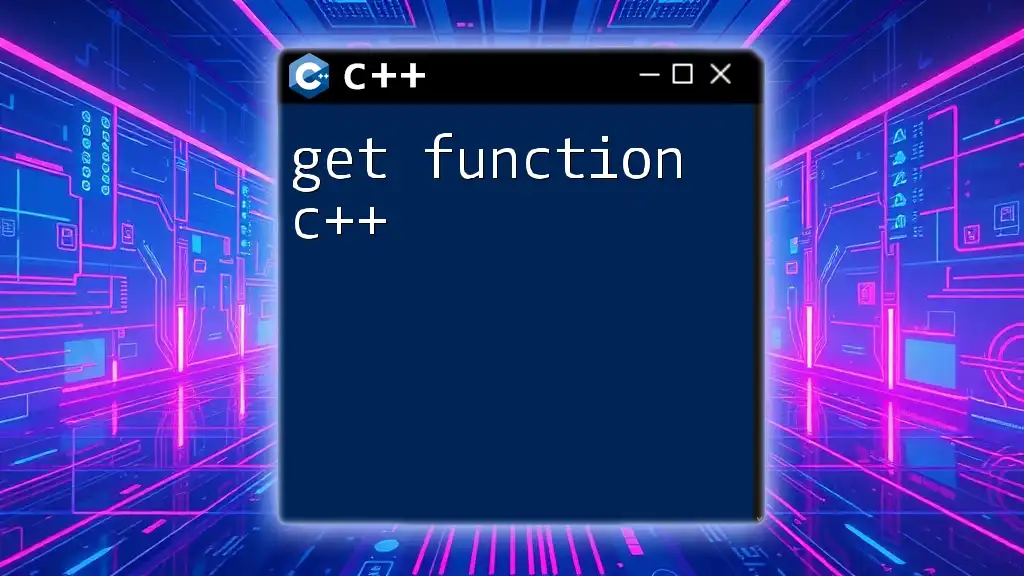
Key Differences Between Methods and Functions
Context Association
Functions are standalone entities that can exist independently, whereas methods are implicitly linked to the classes they belong to. This association with a class means methods operate within the context of an object.
Usage Context
When deciding between a function and a method, consider the program design:
- Use functions for utility tasks that do not require object interaction.
- Opt for methods when encapsulating behavior related to specific data models (i.e., classes).
Access Modifiers
Methods in C++ can have access specifiers (public, private, protected), which control their visibility and access level within the program. Functions, on the other hand, do not have such inherent access control unless defined within a namespace.
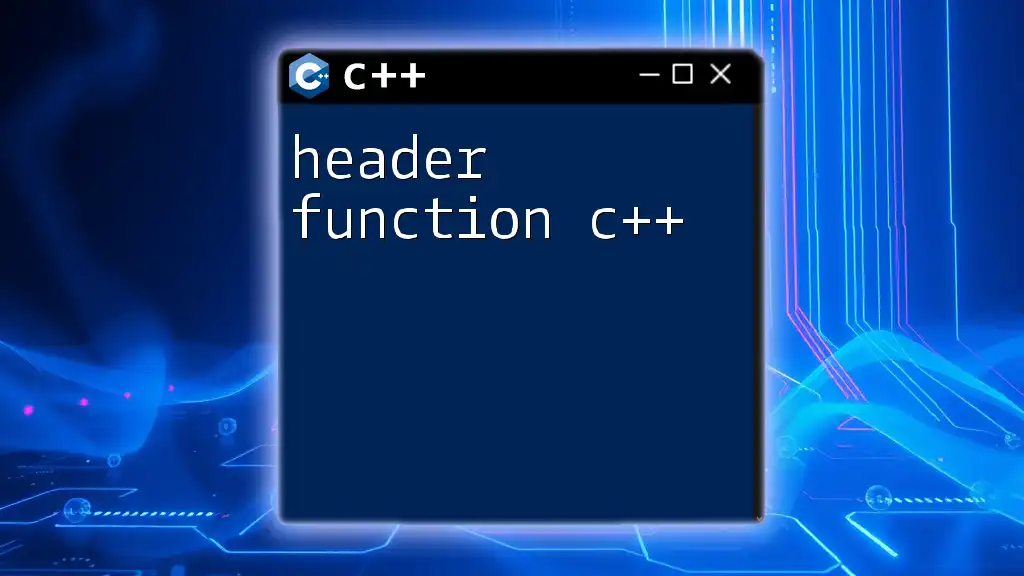
When to Use Methods vs Functions
Design Considerations
- If your design is function-oriented, favor using functions for tasks that are algorithmic in nature and do not require object states.
- Conversely, if you are following an object-oriented approach, methods are preferable to leverage encapsulation and data abstraction.
Performance Implications
Though generally minimal, the choice between methods and functions can have performance implications. Method calls involve an additional access context and can be slightly slower due to the overhead associated with object management. It's crucial to weigh these factors when designing more performance-sensitive applications.
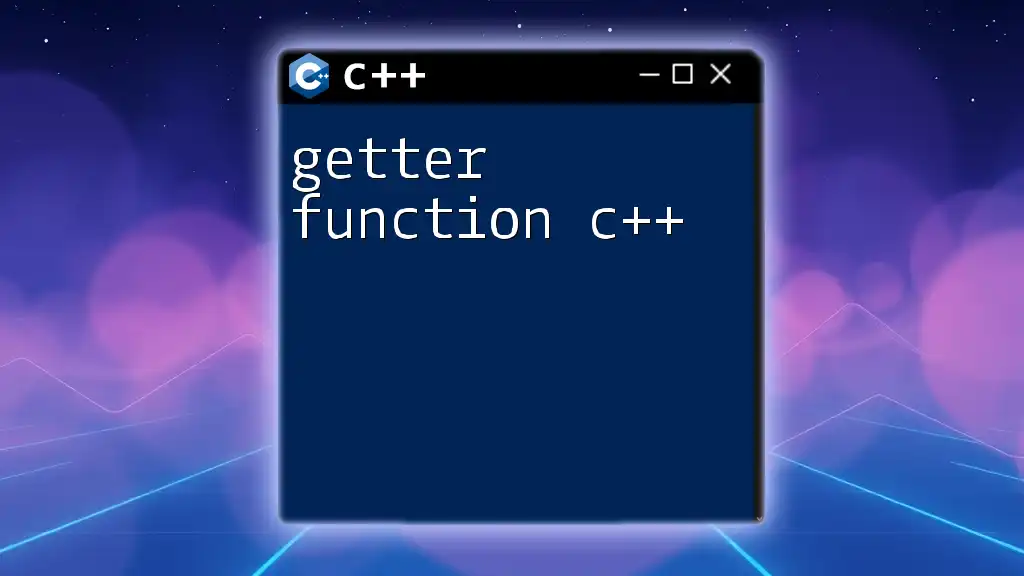
Common Use Cases
Functions in C++
Functions are typically utilized in scenarios where:
- You need reusable code (utility functions, mathematical calculations).
- The task does not require a specific object’s context, such as file handling or global computations.
Methods in C++
Methods are often deployed when:
- You want to implement behavior that corresponds directly to class attributes (e.g., setting values, manipulating data).
- Encapsulation of data and behavior is significant, allowing for better code organization.
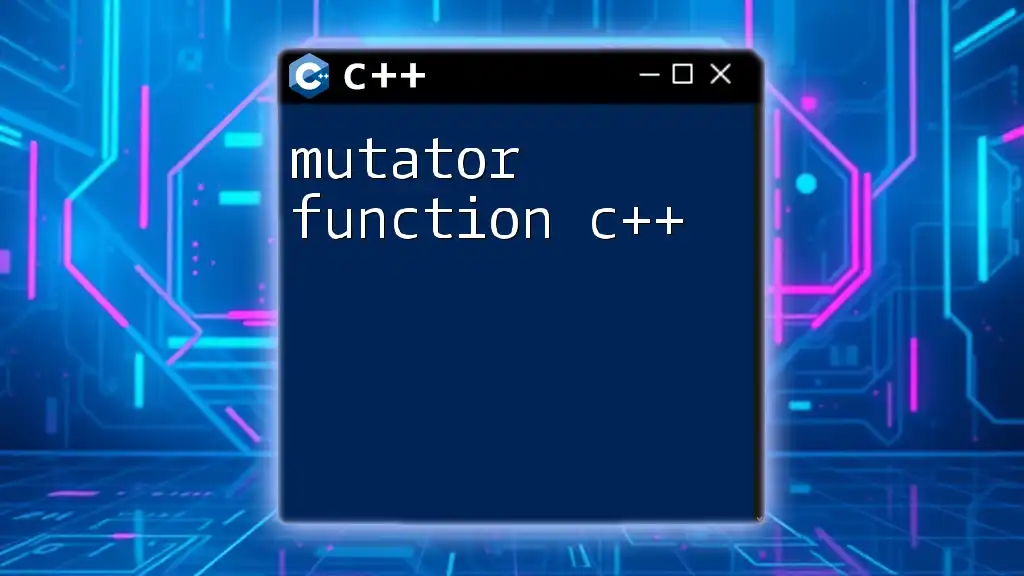
Conclusion
Understanding the difference between methods and functions in C++ is fundamental for effective programming. While both serve the purpose of organizing code and improving reusability, their contexts and applications differ significantly due to object-oriented principles. By recognizing when to use each form appropriately, developers can enhance their coding practices, leading to cleaner and more maintainable code.
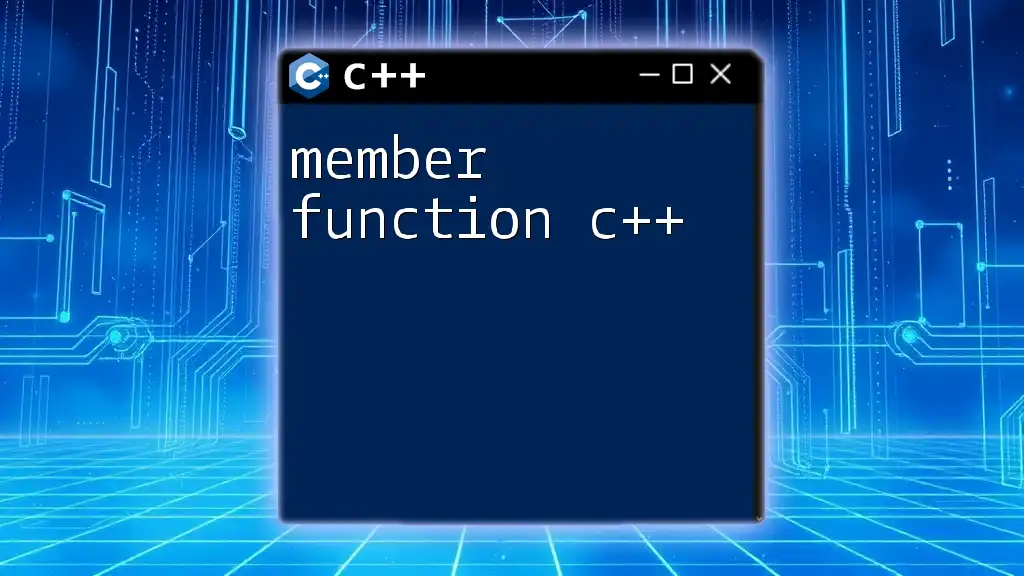
Additional Resources
For further exploration, consider diving into C++ documentation, online tutorials, and community forums that focus on methodologies to solidify your understanding of C++ programming concepts.
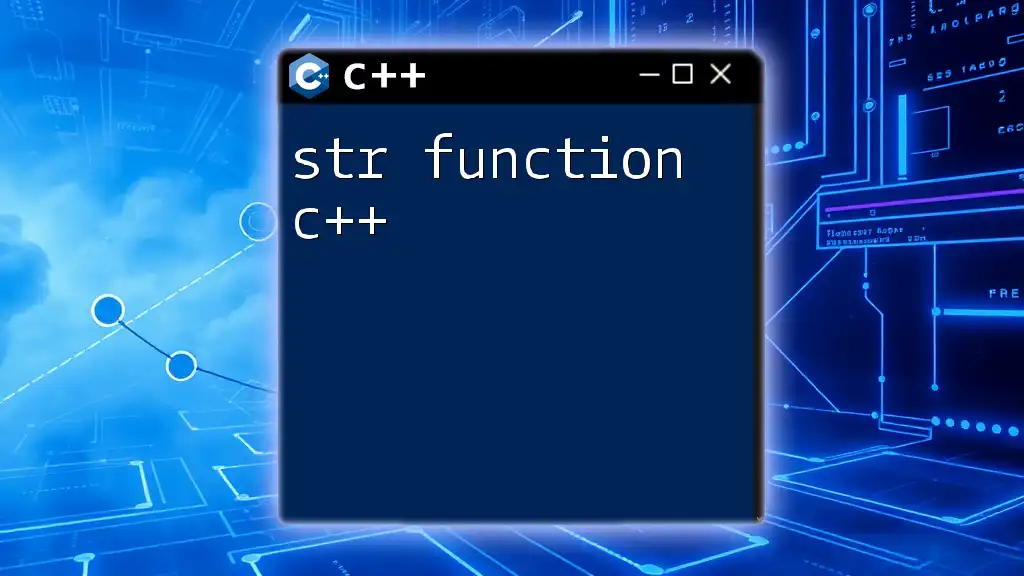
FAQs
Is a method a type of function?
Yes, a method can be viewed as a specialized type of function that belongs to a class and operates in conjunction with an object’s data.
Can functions exist within classes?
While functions traditionally exist outside classes, C++ allows for static functions within classes, which do not operate on instance data but can be called on the class itself.
Do I always need to use methods?
Not necessarily. If your operations do not need the power of OOP or encapsulation, using standalone functions can simplify your code and make it clearer.