Arduino programming primarily utilizes a language based on C++, but it includes simplified functions and libraries that make it easier for beginners, thus combining elements of both C and C++. Here’s a simple example of an Arduino C++ code snippet:
void setup() {
pinMode(LED_BUILTIN, OUTPUT); // Initialize the built-in LED pin as an output
}
void loop() {
digitalWrite(LED_BUILTIN, HIGH); // Turn the LED on
delay(1000); // Wait for a second
digitalWrite(LED_BUILTIN, LOW); // Turn the LED off
delay(1000); // Wait for a second
}
What is Arduino?
Arduino is a popular open-source electronics platform that combines hardware and software to allow users to create interactive projects. It has gained immense popularity among hobbyists, educators, and professionals alike due to its accessibility and versatility. The platform consists of various hardware boards (like the Arduino Uno, Mega, and Nano) and a simplified programming environment known as the Arduino IDE.
Understanding the programming language behind Arduino is essential for developers and beginners alike. This knowledge not only helps in writing efficient code but also enables deeper insights into how Arduino operates, allowing for more sophisticated project implementations.
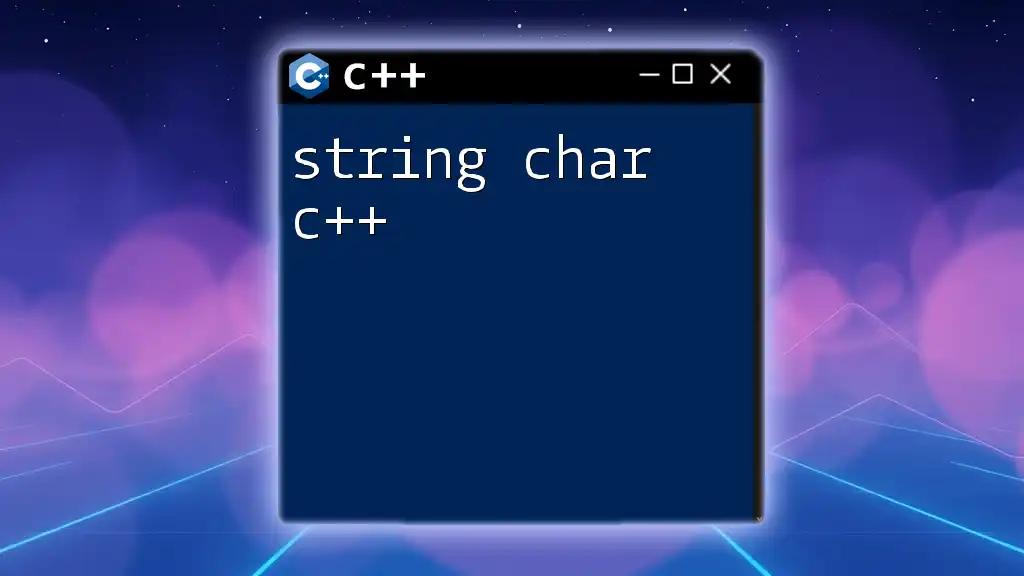
Is Arduino C or C++?
When discussing whether Arduino is C or C++, it's important to recognize that the Arduino programming language is fundamentally based on both. In fact, Arduino sketches are written in a dialect that incorporates elements of C and C++, enabling users to leverage the strengths of both languages while simplifying the programming experience.
Does Arduino Use C++?
Yes, Arduino does use C++. The Arduino IDE allows developers to write code that utilizes C++ features, such as classes and object-oriented programming (OOP). However, the environment is designed to abstract much of the complexity associated with C++. This approach ensures that even users with minimal programming experience can create functional projects with relative ease.
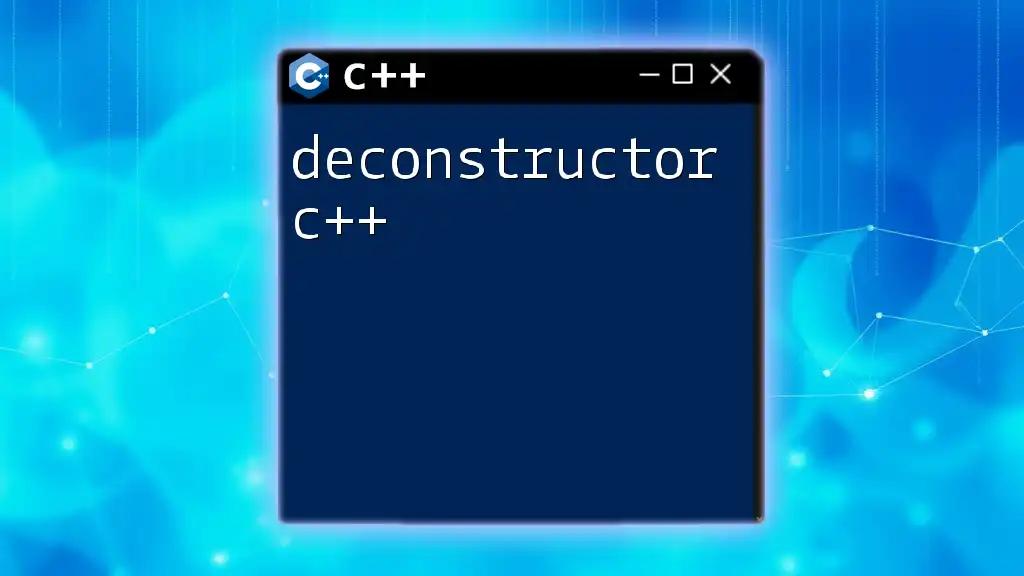
C vs C++: Key Differences
Overview of C Programming Language
C is a procedural programming language that focuses on functions and a straightforward linear flow of control. It is known for its efficiency and is widely used in system programming and embedded systems. The highlights of C include:
- Simplicity: C has a small set of keywords and straightforward syntax, making it easier to learn.
- Performance: As a low-level language, C offers fine control over system resources, making it ideal for performance-critical applications.
- Procedure-Oriented: C primarily emphasizes functions and procedures in its programming paradigm.
Overview of C++ Programming Language
C++, on the other hand, builds upon C by introducing object-oriented programming concepts, including classes, inheritance, and encapsulation. This evolution allows for more complex and structured programming, suitable for large-scale applications. Key features of C++ include:
- Object-Oriented: C++ encourages a design approach using objects, allowing for greater modularity and reusability of code.
- Extensive Standard Library: C++ provides a rich set of libraries, supporting various programming needs and algorithms.
- Performance: Like C, C++ is also performance-oriented but adds more abstraction layers through object-oriented features.
Comparing Syntax and Features
While C and C++ share a great deal of syntax, C++ introduces additional concepts that allow for more expressive and modular code. For example, consider the following points:
- Function Definitions: In C, functions are defined without the concept of classes, while in C++, functions can be part of a class.
- Data Encapsulation: C doesn’t support data encapsulation natively; C++ allows data to be bundled with methods that operate on it within classes.
- Polymorphism and Inheritance: These concepts are intrinsic to C++ but do not exist in C, enabling more advanced design patterns and code organization.
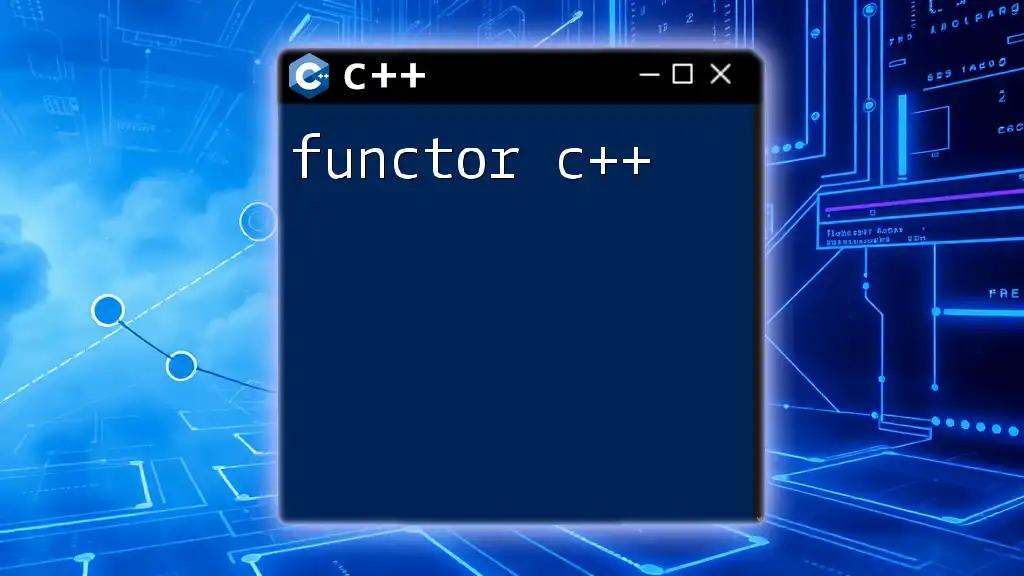
Key Features of Arduino’s Programming Language
Sketches
An Arduino program is referred to as a sketch. Each sketch consists of two primary functions: `setup()` and `loop()`. The `setup()` function runs once when the device starts and is typically used for initializing settings, such as pin modes. The `loop()` function continuously runs after `setup()` and is where the main code for your project resides.
Functions and Libraries
Arduino provides a rich set of built-in functions that allow for straightforward manipulation of hardware. Additionally, libraries extend the functionality, offering pre-written code for various devices and tasks. For instance, the `Wire` library makes it easier to communicate with I²C devices, while the `Servo` library simplifies the control of servo motors.
Object-Oriented Programming in Arduino
While many Arduino projects can be completed without OOP, developers can take advantage of C++ features to create more organized code. This approach can significantly improve maintainability and scalability. For example, you might define a class to represent a sensor, allowing you to encapsulate related properties and methods.
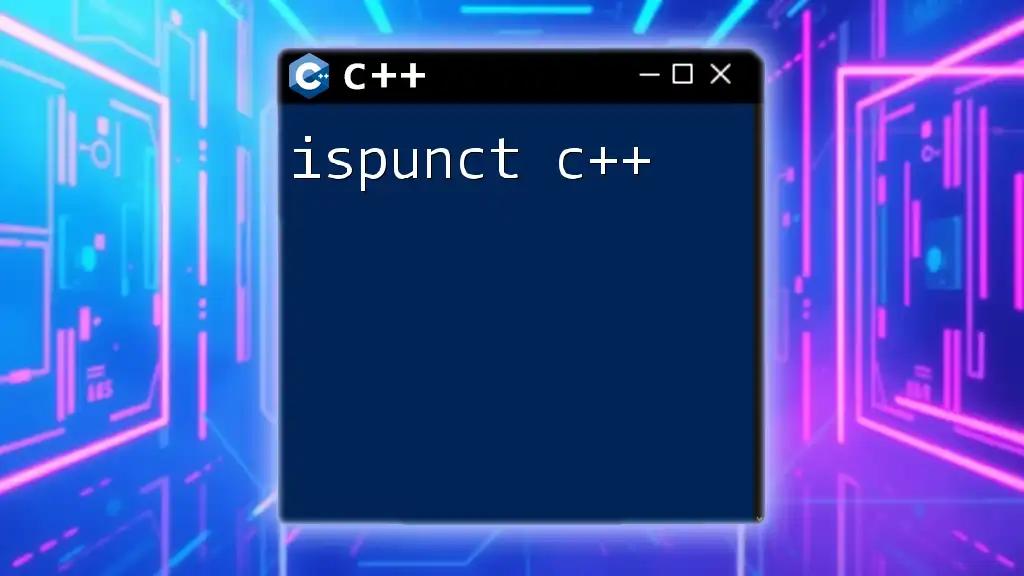
Examples of C and C++ in Arduino
Simple C-Style Code Example
Here's a simple example of C-style code that demonstrates procedural programming in Arduino:
void setup() {
pinMode(13, OUTPUT); // Set pin 13 as an output
}
void loop() {
digitalWrite(13, HIGH); // Turn on the LED
delay(1000); // Wait for a second
digitalWrite(13, LOW); // Turn off the LED
delay(1000); // Wait for a second
}
C++ Features in Arduino Code
Now, let’s look at an example that incorporates object-oriented features with a custom class:
class LED {
public:
LED(int pin) {
this->pin = pin; // Assign pin number to the member variable
pinMode(pin, OUTPUT); // Set the pin as an output
}
void on() {
digitalWrite(pin, HIGH); // Turn on the LED
}
void off() {
digitalWrite(pin, LOW); // Turn off the LED
}
private:
int pin; // Member variable to store the pin number
};
LED myLED(13); // Create an LED object for pin 13
void setup() {}
void loop() {
myLED.on(); // Turn on the LED
delay(1000); // Wait for a second
myLED.off(); // Turn off the LED
delay(1000); // Wait for a second
}
In this example, we define an `LED` class to encapsulate the behavior of an LED. It allows us to easily manage LED states by creating an instance of the class, promoting code reuse and clarity.
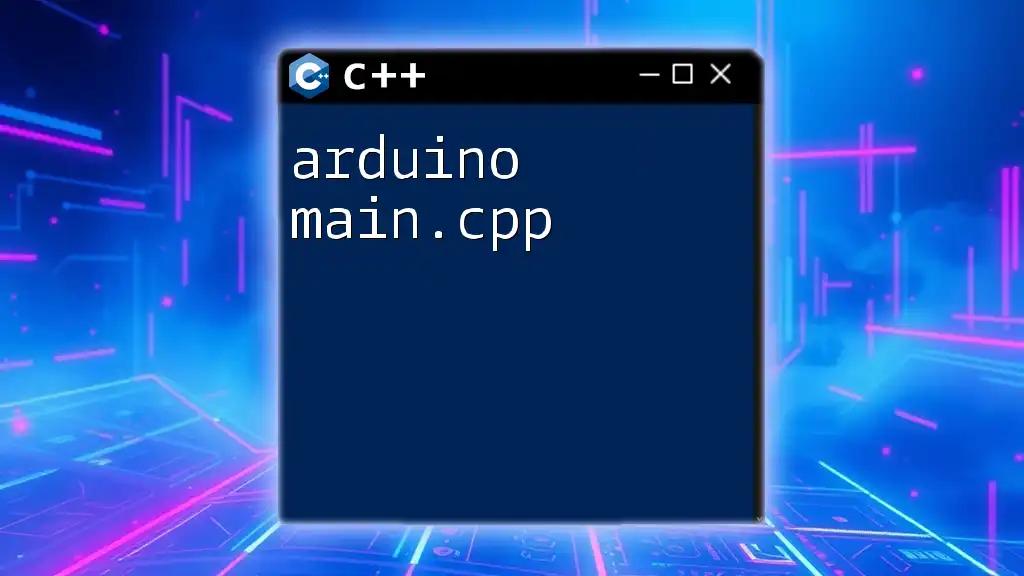
Common Misconceptions
Misconception: Arduino Only Uses C
One common misconception is that Arduino is limited to C programming. While it does provide C-style syntax, the underlying capabilities are rooted in C++, which means developers can write complex, object-oriented programs if they choose.
Misconception: C++ is Too Complex for Beginners
Another misconception is that C++ is too complex for beginners. The Arduino IDE abstracts many of the complex details and presents a user-friendly interface, allowing new users to focus on building projects without drowning in C++ complexities.
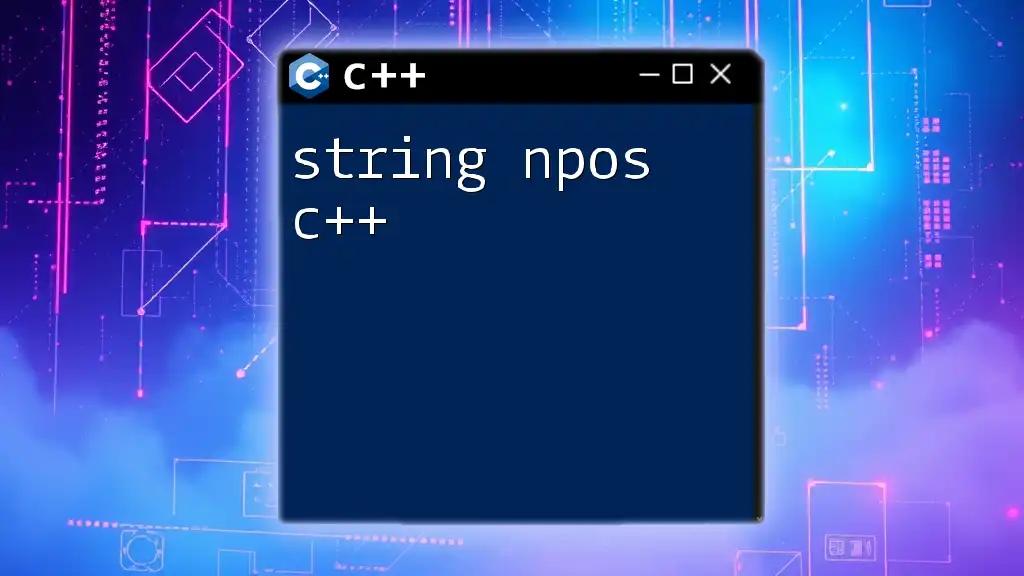
Final Thoughts
In conclusion, Arduino programming is built on the foundations of both C and C++, leveraging the strengths of each language. Familiarity with these languages can empower developers to use Arduino to its full potential, facilitating creative and sophisticated projects.
For those just starting, don’t be intimidated by the programming language. Arduino's simplicity and supportive community mean that programmers of all levels can learn and succeed.
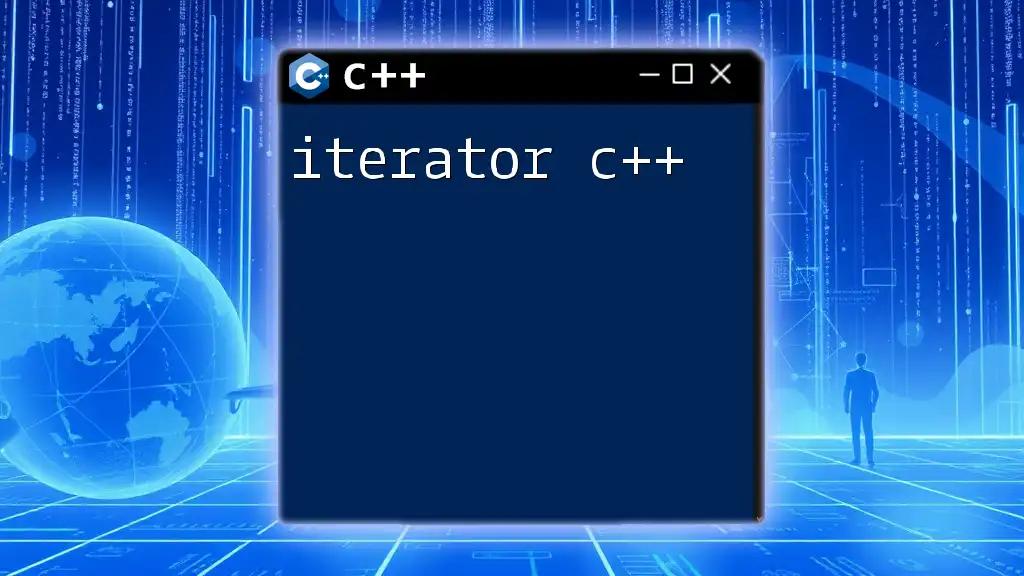
Additional Resources
To deepen your understanding of Arduino and its programming languages, consider exploring the official Arduino documentation, which offers extensive guidance on libraries, functions, and best practices. Additionally, a range of books and online tutorials are available that cater specifically to beginners and seasoned programmers alike.
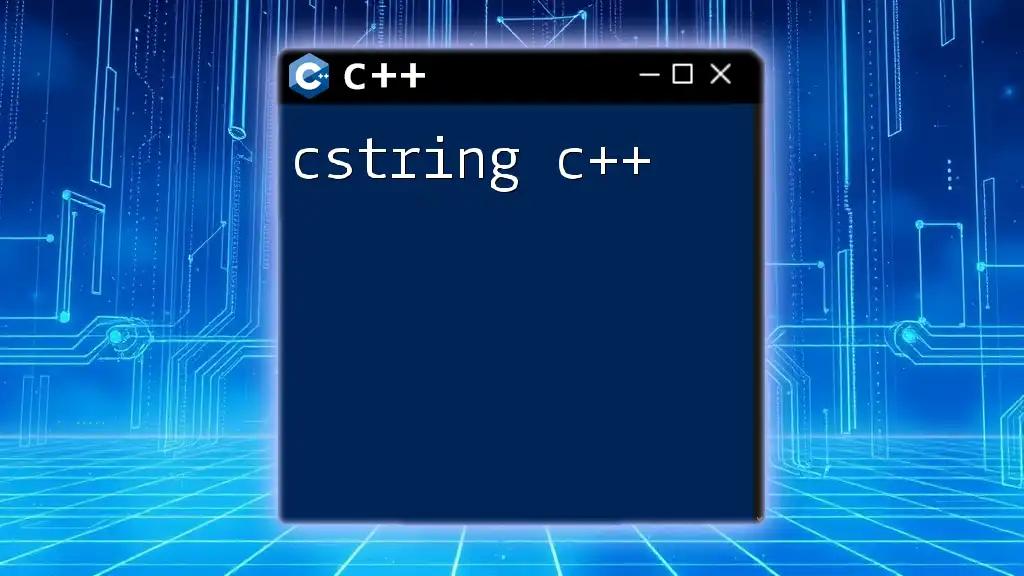
Call to Action
We’d love to hear about your experiences with Arduino programming! What challenges have you faced, and how have you overcome them? Your insights could inspire others in the Arduino community.