The `main.cpp` file in an Arduino project serves as the entry point for your program, where you define the setup and loop functions necessary for running your code on the microcontroller.
Here's a simple example of a basic Arduino `main.cpp` structure:
void setup() {
Serial.begin(9600); // Initialize serial communication
}
void loop() {
Serial.println("Hello, Arduino!"); // Print message to the serial monitor
delay(1000); // Wait for one second
}
Understanding the Basics of Arduino
What is Arduino?
Arduino is an open-source electronics platform based on easy-to-use hardware and software. It is designed for anyone, from beginners to advanced developers, to create interactive projects that can simulate real-world systems. Arduino boards can read inputs such as light or touch and turn them into output actions like turning on an LED or activating a motor. The beauty of Arduino lies in its simplicity and the robustness of its community, which continuously contributes tutorials, projects, and libraries.
Introduction to C++ and Arduino
The Arduino programming environment primarily utilizes C++, a powerful programming language known for its versatility and efficiency in embedded systems. C++ allows Arduino programmers to write code that can interact directly with hardware components. The ability to use C++ syntax enhances the performance and capabilities of Arduino sketches, making it an ideal choice for developing microcontroller-based projects.
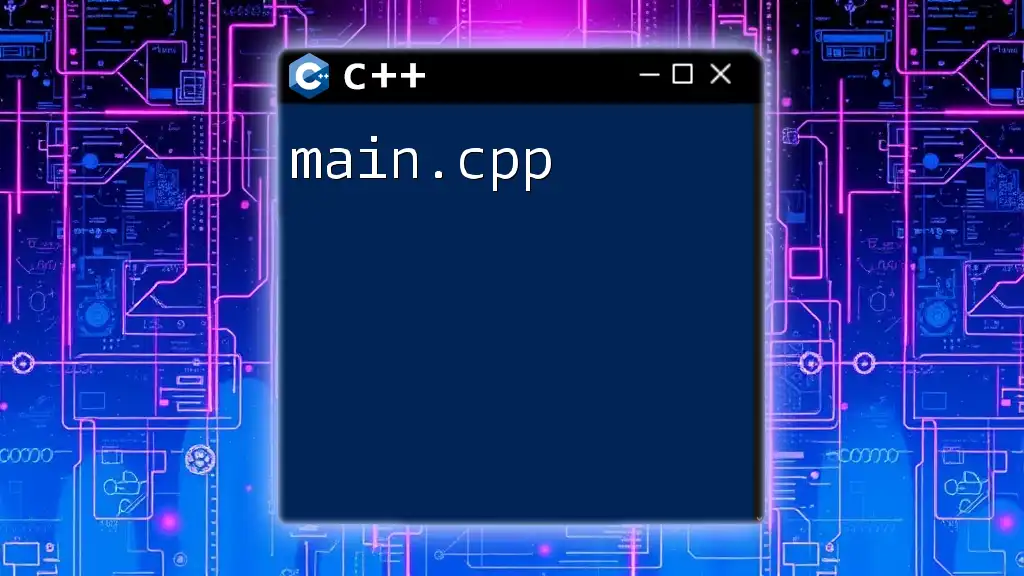
Setting Up Your Arduino Environment
Installing the Arduino IDE
To start programming with Arduino, the first step is to install the Arduino Integrated Development Environment (IDE). The IDE is the software where you’ll write, compile, and upload your code. Installation guides are available on the official Arduino website for various operating systems:
- Windows: Download the installer and follow the on-screen instructions.
- Mac: Open the disk image, drag the Arduino IDE into your Applications folder, and launch it.
- Linux: Use the package manager or download the tarball, extract it, and run the installation script.
Once the IDE is installed, you can create your first project by opening the application.
Connecting Your Arduino Board
Arduino boards come in various forms, such as Arduino Uno, Mega, and Nano. To start, carefully connect your selected Arduino board to your computer using a USB cable. Ensure you have the necessary drivers installed. You can choose the board type from the Tools menu in the IDE, ensuring that your selected board matches the physical device connected.
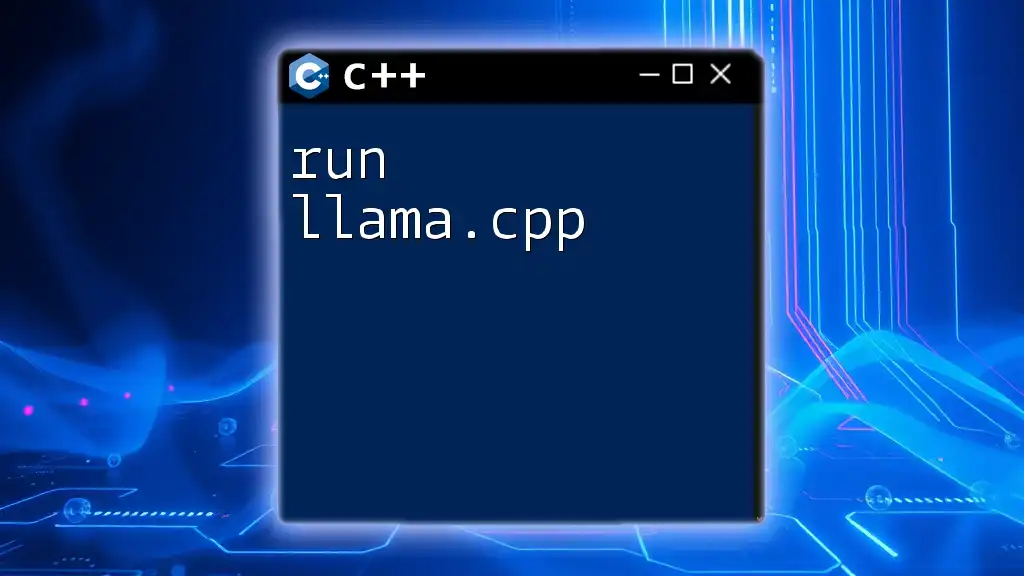
The Structure of main.cpp
Overview of main.cpp in Arduino
In Arduino programming, the actual sketch you write and upload consists of two crucial functions: `setup()` and `loop()`. Unlike standard C++ applications, where you may have a single `main()` function, in Arduino, the `main.cpp` file is structured to handle this specific event-driven architecture.
Explaining the Setup Function
The `setup()` function is executed only once when the program starts. It is used to initialize variables, pin modes, and start serial communication. Here’s an example of a simple `setup()` function:
void setup() {
// Initialize serial communication at 9600 bits per second
Serial.begin(9600);
// Set a pin as OUTPUT
pinMode(LED_BUILTIN, OUTPUT);
}
In this snippet, we begin by initializing serial communication, which is essential for debugging and data transmission. Additionally, we configure the built-in LED pin as an output so it can be controlled later.
Understanding the Loop Function
After the setup is complete, the program enters the `loop()` function, where all the action happens continuously. This function runs repeatedly, allowing your Arduino to respond to input and perform actions. Here’s a basic example of what the `loop()` function can look like:
void loop() {
// Turn the LED on (HIGH is the voltage level)
digitalWrite(LED_BUILTIN, HIGH);
// Wait for a second
delay(1000);
// Turn the LED off by making the voltage LOW
digitalWrite(LED_BUILTIN, LOW);
// Wait for a second
delay(1000);
}
In this loop, the built-in LED is turned on for one second and then turned off for one second, creating a blinking effect. This demonstrates how the loop allows for ongoing interaction with the hardware.
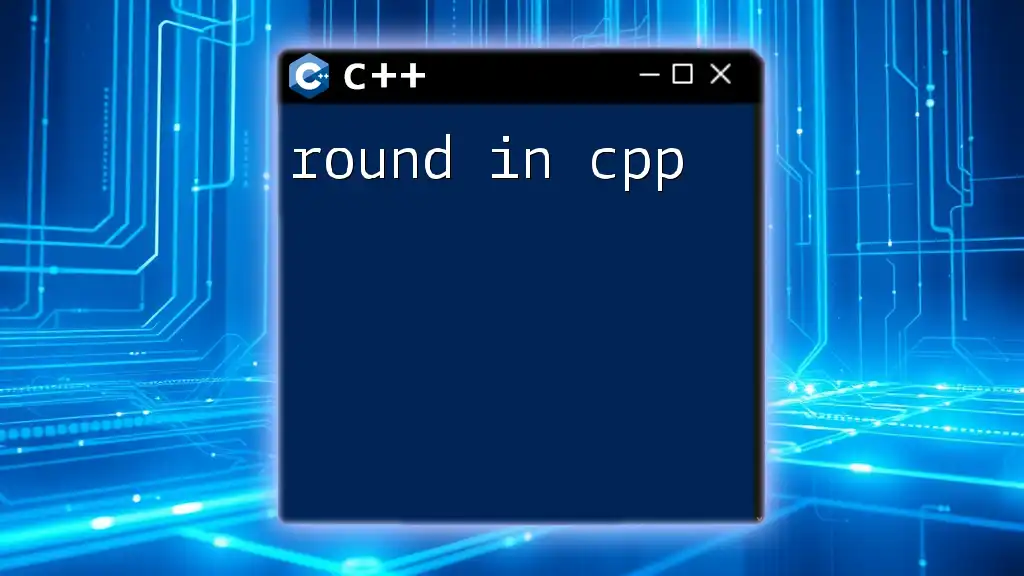
Deeper Dive into main.cpp Components
Variables and Data Types in Arduino
Understanding variables and their data types is critical in programming with Arduino. Common data types include:
- int for integers (e.g., `int ledPin = 9;`)
- float for decimal numbers
- bool for true/false values (e.g., `bool buttonState = false;`)
These data types allow you to store state information, interactive controls, and sensor data effectively.
Control Structures: If Statements and Loops
Control structures like if statements and loops are vital for executing conditional actions within your sketches. Here’s an example of an if statement used to read a button's state:
if (digitalRead(buttonPin) == HIGH) {
digitalWrite(ledPin, HIGH); // Turn on LED
} else {
digitalWrite(ledPin, LOW); // Turn off LED
}
This code checks the state of a button. If pressed, it activates the LED; if not, the LED is turned off.
Loops, such as `for` and `while`, help in repeating actions. For instance:
for (int i = 0; i < 5; i++) {
digitalWrite(ledPin, HIGH);
delay(500);
digitalWrite(ledPin, LOW);
delay(500);
}
This loop instructs the Arduino to blink an LED five times with a half-second delay between each blink.
Functions: Creating Your Own
Custom functions allow you to encapsulate code for reuse and improve readability. Creating a function can streamline your code as follows:
void blinkLed(int pin, int duration) {
digitalWrite(pin, HIGH);
delay(duration);
digitalWrite(pin, LOW);
}
You can call `blinkLed(ledPin, 1000);` within your loop to blink the LED for one second without writing all the code to manage the LED each time.
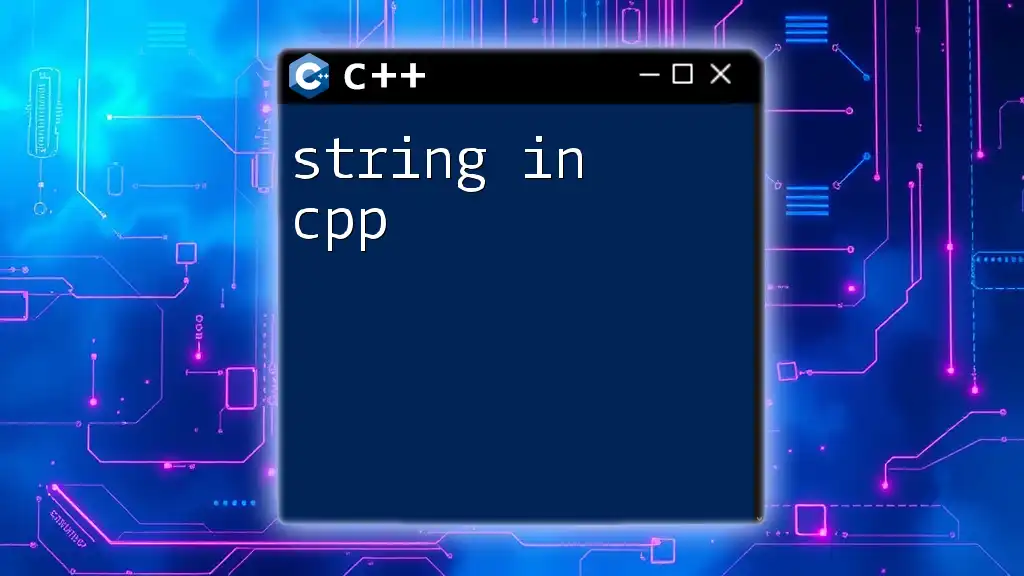
Uploading Your Code to the Arduino Board
Compiling Your Code
Before your code can run on the Arduino board, it first needs to be compiled. Compiling translates your human-readable code into machine code that the Arduino can understand. The Arduino IDE provides feedback on the process, and if any errors occur, it will highlight the lines needing attention.
Uploading and Running Your Code
After successfully compiling your code, it's time to upload it to the Arduino board. Click the Upload button in the IDE, which compiles the sketch again and transfers it to the connected board. Once uploaded, your sketch starts running immediately.
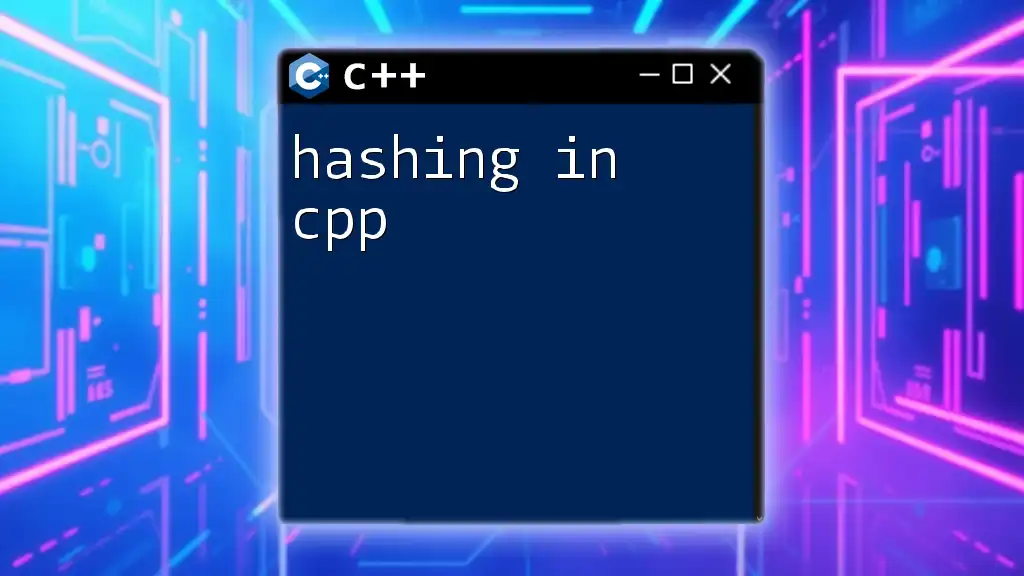
Debugging common issues with main.cpp
Troubleshooting Compilation Errors
Compilation errors can range from syntax mistakes to library issues. Common messages include "expected ';' before" and "undefined reference to". Carefully read the error messages provided, as they often point directly to the problematic line.
Runtime Issues on Arduino
Debugging runtime issues may require some additional strategies. Utilize the Serial Monitor to print out messages or variable states that can provide insights into what is happening in your sketch.
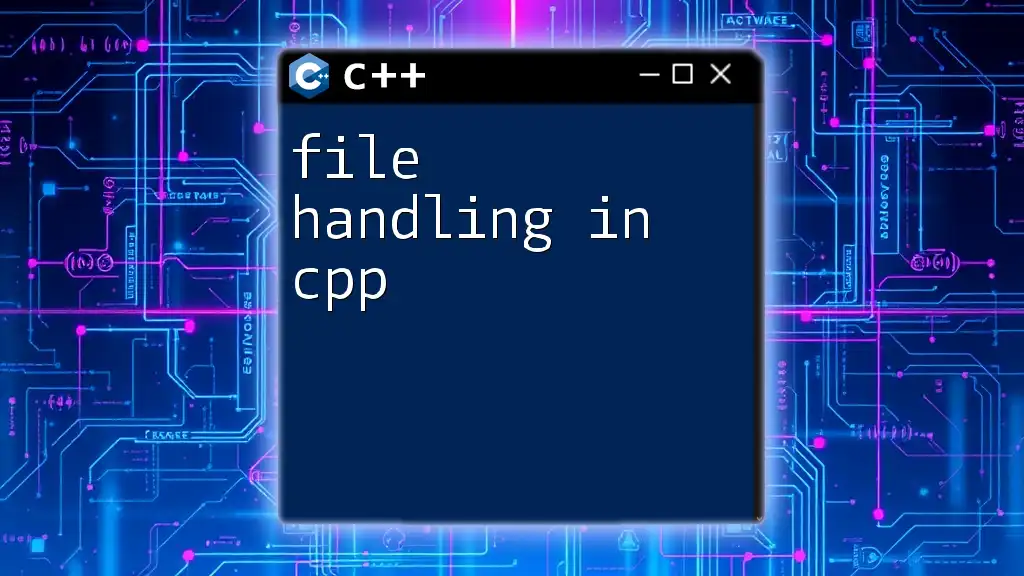
Best Practices for Writing Arduino Code
Code Organization and Readability
Writing clear, readable code is essential, especially in collaborative projects or when revisiting your work. Use consistent naming conventions for variables and functions, and include comments to explain complex sections of your code. For example:
// This function turns the LED on for a specific duration
This approach saves time for both you and others who may read or attempt to modify your code.
Using Libraries to Extend Functionality
Arduino boasts a vibrant ecosystem of libraries that can greatly enhance your projects. Libraries are pre-written code designed to manage complex hardware interactions. For instance, if you wanted to work with a liquid crystal display (LCD), you could include the LiquidCrystal library:
#include <LiquidCrystal.h>
Using libraries can significantly reduce development time and allow you to focus on more advanced topics without reinventing the wheel.
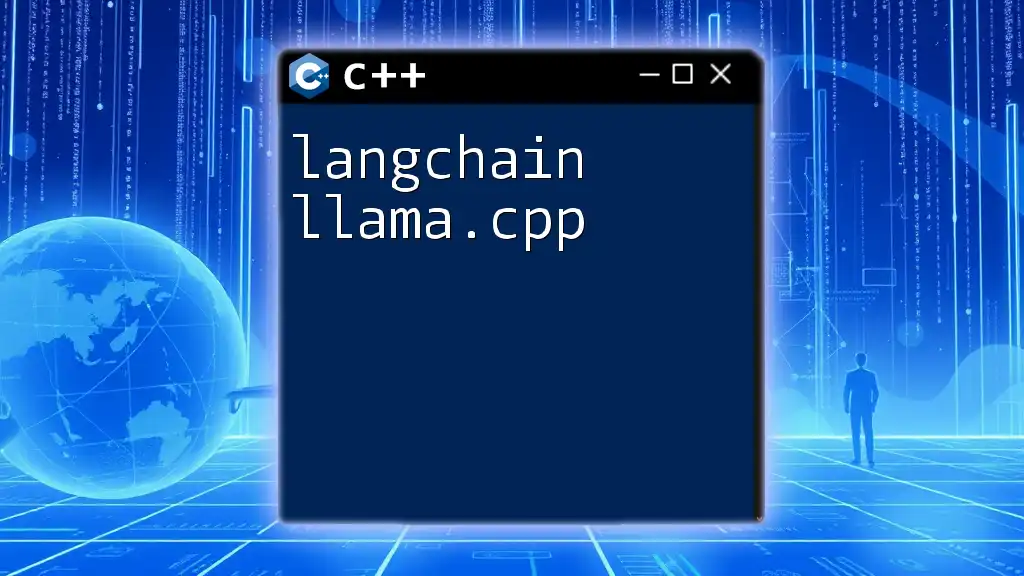
Conclusion
Recap of Important Points
In this exploration of arduino main.cpp, we covered the foundational concepts of Arduino and C++, examined the structure and key functions of main.cpp, and discussed practical coding strategies—from custom functions to debugging tips.
Next Steps for Arduino Enthusiasts
As you become more adept at using Arduino, consider exploring advanced libraries, participating in community forums, or starting your unique projects. The possibilities with Arduino are limitless, and every project is an opportunity to learn and grow in the exciting world of electronics and programming.