String manipulation in C++ involves using various built-in functions to modify or interact with string data efficiently. Here's a simple example that demonstrates how to concatenate two strings:
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello, ";
std::string str2 = "World!";
std::string result = str1 + str2; // Concatenation
std::cout << result << std::endl; // Output: Hello, World!
return 0;
}
Understanding C++ Strings
What is a String in C++?
In C++, a string is a data type used for representing a sequence of characters. Unlike traditional character arrays, which require manual memory management and are susceptible to overflow errors, the C++ `std::string` class provides a safer, more flexible way to work with strings. The `std::string` class handles memory allocation automatically, allowing for dynamic resizing as needed. This makes string manipulation in C++ both easier and more efficient.
Instantiating Strings
Creating a string in C++ is straightforward. You can initialize a string using different methods, such as:
std::string str = "Hello, World!"; // Using string literals
std::string anotherStr("Hello!"); // Using constructor
char charArray[] = "Hello, World!";
std::string fromArray(charArray); // From a character array
This flexibility allows programmers to easily create strings in various contexts and formats.
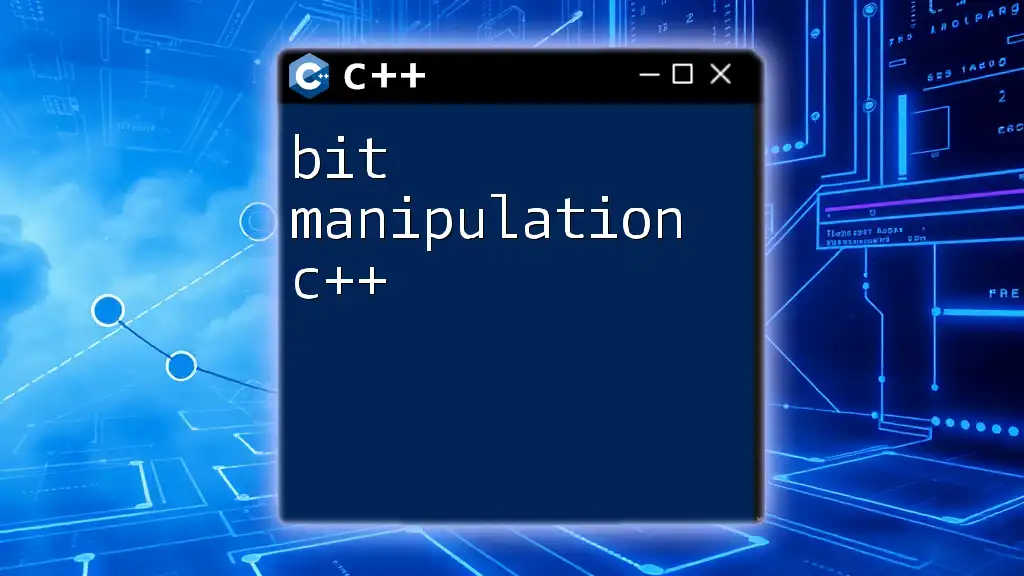
Basic String Manipulation Operations
Concatenation of Strings
Concatenation is the process of joining two or more strings. In C++, you can concatenate strings using the `+` operator or the `+=` operator. Here’s how it works:
std::string first = "Hello, ";
std::string second = "World!";
std::string result = first + second; // result is "Hello, World!"
You can also append strings using the `+=` operator:
first += second; // first now holds "Hello, World!"
Accessing Characters in a String
Accessing individual characters in a string can be performed using the `[]` operator or the `.at()` method. The `.at()` method provides bounds checking, making it safer to use:
char ch = str[0]; // Accesses the first character, 'H'
char ch2 = str.at(1); // Accesses the second character, 'e' (safer access)
Finding Substrings
Finding a substring within a string can be achieved using the `.find()` and `.rfind()` methods. These methods return the index of the first and last occurrence of a substring respectively:
std::string sentence = "Hello, welcome to C++ programming!";
size_t pos = sentence.find("C++"); // pos is the index of 'C'
If the substring is not found, `.find()` returns `std::string::npos`, which you can check against:
if (pos != std::string::npos) {
// Substring found, proceed with additional operations
}
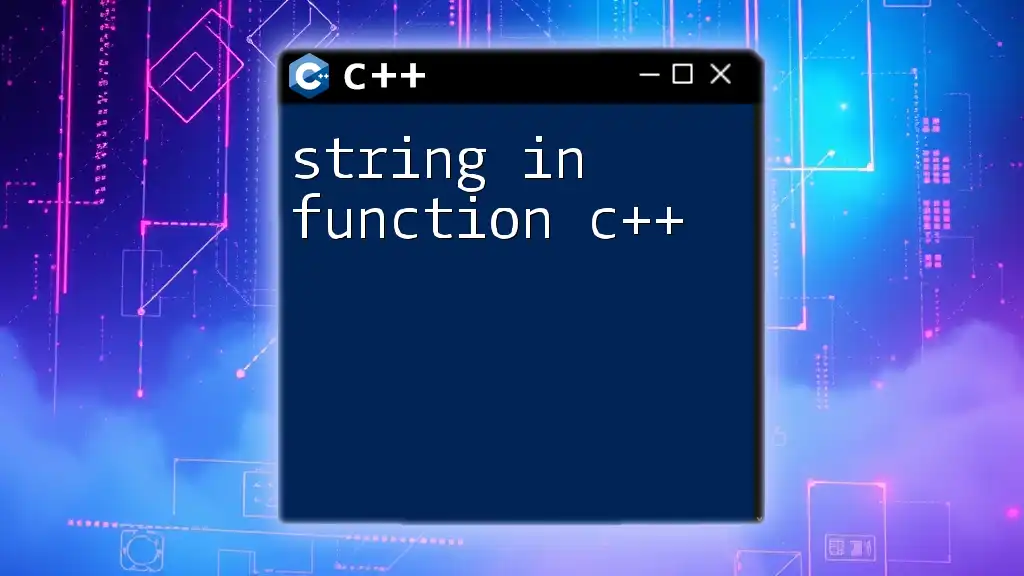
Advanced String Manipulation Functions
Substring Extraction
You can extract a substring from a string using the `.substr()` method. This method requires you to specify the starting index and the length:
std::string str = "Hello, World!";
std::string sub = str.substr(7, 5); // sub is "World"
String Replacement
If you need to replace a substring with another string, you can use the `.replace()` method. This function allows you to specify the position to start replacing, the number of characters to replace, and the new substring:
std::string str = "Hello, World!";
str.replace(7, 5, "C++"); // Changes "World" to "C++"
// Now str becomes "Hello, C++!"
String Splitting
Splitting a string into components based on a delimiter is no built-in feature in the standard string class, but you can create a utility function using a `stringstream`. Here is an example implementation:
#include <sstream>
#include <vector>
std::vector<std::string> split(const std::string &s, char delimiter) {
std::stringstream ss(s);
std::string item;
std::vector<std::string> tokens;
while (std::getline(ss, item, delimiter)) {
tokens.push_back(item);
}
return tokens;
}
You can use this function to split strings effectively based on specified delimiters, allowing for greater data manipulation.
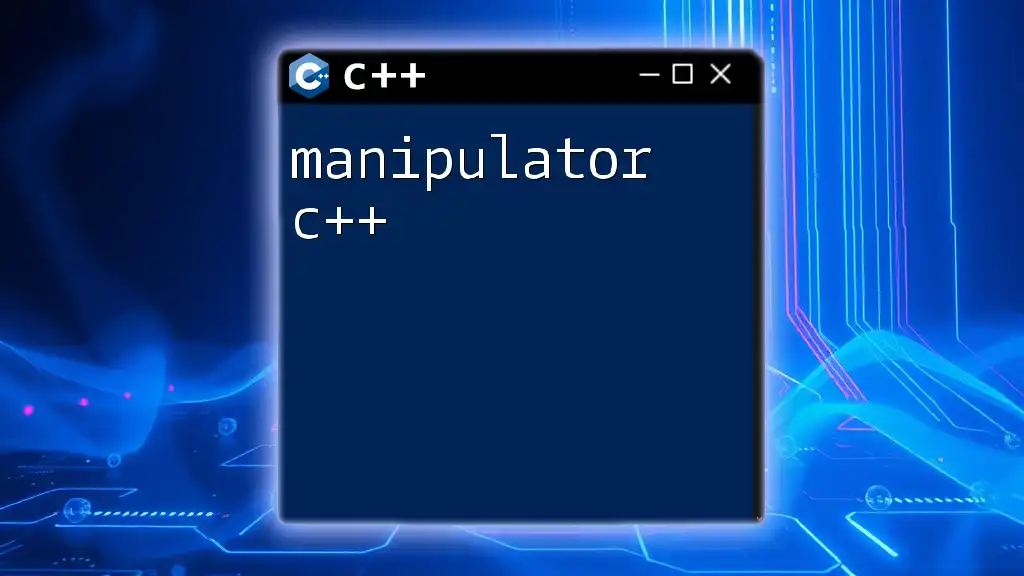
String Manipulation in C++: Useful Tips and Tricks
String Length and Capacity
You can determine the length of a string using either the `.length()` or `.size()` functions, both providing the same result:
std::string str = "Hello, World!";
size_t length = str.size(); // Length of the string is 13
Additionally, strings in C++ can reserve a specific amount of memory using the `.reserve()` method. This can improve performance when you know the final size of the string in advance.
Case Conversion
Transforming a string to upper or lower case is a common requirement in string manipulation. The C++ Standard Library provides the `std::transform` algorithm to facilitate this:
#include <algorithm>
std::string str = "Hello, World!";
std::transform(str.begin(), str.end(), str.begin(), ::tolower); // Convert to lowercase
This example demonstrates how to convert an entire string to lowercase, enhancing the versatility of your string manipulations.
String Comparison
Strings in C++ can be compared using relational operators or the `.compare()` method. The relational operators allow for a straightforward comparison:
std::string str1 = "apple";
std::string str2 = "banana";
if (str1 < str2) {
// str1 comes before str2 in lexicographic order
}
Alternatively, the `.compare()` method allows for more nuanced comparisons, returning values indicating the relationship between strings:
if (str1.compare(str2) < 0) {
// str1 comes before str2
}
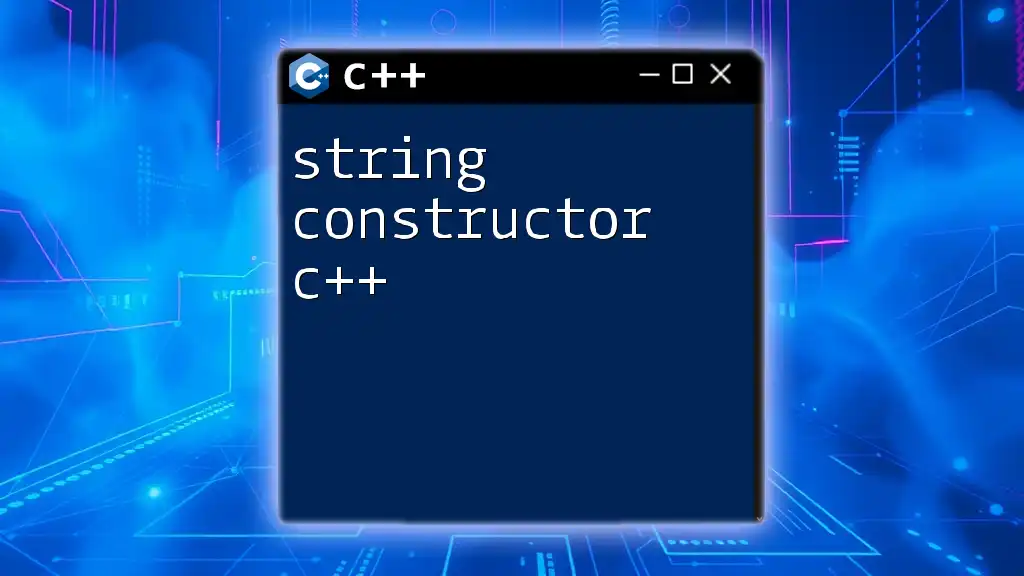
Best Practices for String Manipulation in C++
When to Use `std::string` vs. char arrays
Choosing between `std::string` and character arrays generally comes down to safety and ease of use. While character arrays offer simplicity, they are prone to overflow errors and require manual memory management. Utilizing `std::string` provides better safety, automatic memory management, and a richer set of functionalities for string manipulation in C++. Therefore, favor `std::string` unless absolutely necessary.
Common Pitfalls
It's important to be aware of potential pitfalls when working with strings. Using raw character arrays can lead to memory management issues, resulting in buffer overflows. Always ensure that your arrays are properly sized and null-terminated. When using `std::string`, such issues are inherently minimized, but programmers should still take care to manage string indices properly and avoid out-of-bounds access.
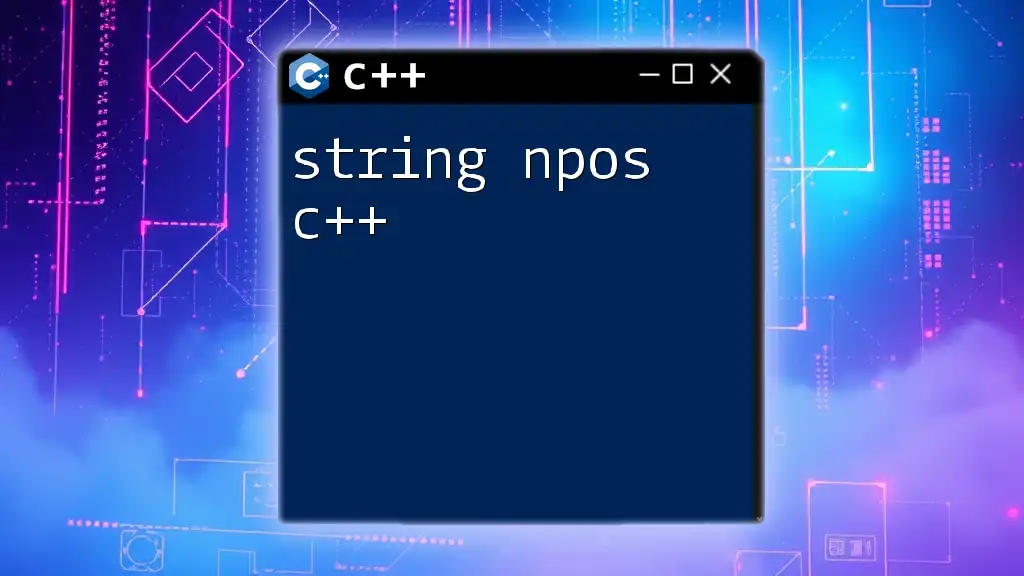
Conclusion
String manipulation in C++ is an essential skill for any programmer looking to write efficient and effective code. By mastering the methods and principles discussed in this article, you can significantly enhance your programming capabilities in C++. Whether you're working on data parsing, user input handling, or simply formatting strings, understanding how to manipulate strings effectively is key.
Challenge yourself to implement coding exercises that involve string manipulation to solidify your understanding and explore advanced techniques.