A matrix calculator in C++ allows you to perform various operations, such as addition and multiplication, on matrices with concise commands for improved efficiency in calculations.
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<vector<int>> matrixA = {{1, 2}, {3, 4}};
vector<vector<int>> matrixB = {{5, 6}, {7, 8}};
vector<vector<int>> result(2, vector<int>(2, 0));
// Matrix multiplication
for (int i = 0; i < 2; i++)
for (int j = 0; j < 2; j++)
for (int k = 0; k < 2; k++)
result[i][j] += matrixA[i][k] * matrixB[k][j];
// Display the result
for (auto row : result) {
for (auto val : row)
cout << val << " ";
cout << endl;
}
return 0;
}
Understanding Matrices
What is a Matrix?
A matrix is a rectangular arrangement of numbers, symbols, or expressions, organized in rows and columns. They're fundamental in various fields, particularly in programming and mathematics. For instance, a matrix can represent a system of linear equations and is extensively used in graphics transformations and machine learning.
The dimensions of a matrix refer to its size, denoted as rows x columns. For example, a matrix with 3 rows and 4 columns is referred to as a 3x4 matrix.
Basic Matrix Operations
To effectively develop a matrix calculator in C++, it is crucial to understand the primary operations you can perform on matrices:
Addition and Subtraction Matrices can only be added or subtracted if they have the same dimensions. The resulting matrix will have the same dimensions as the original matrices.
Here’s a code snippet demonstrating how to implement the addition of two matrices:
Matrix Matrix::add(const Matrix& other) {
if (rows != other.rows || cols != other.cols) {
throw std::invalid_argument("Matrices must have the same dimensions for addition.");
}
Matrix result(rows, cols);
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
result.data[i][j] = data[i][j] + other.data[i][j];
}
}
return result;
}
Scalar Multiplication Scalar multiplication involves multiplying each element of the matrix by a constant (scalar). This operation is straightforward and does not depend on the dimensions of the matrix.
Here’s how you could implement this:
Matrix Matrix::scalarMultiply(float scalar) {
Matrix result(rows, cols);
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
result.data[i][j] = data[i][j] * scalar;
}
}
return result;
}
Matrix Multiplication Unlike addition, matrix multiplication has specific rules: the number of columns in the first matrix must equal the number of rows in the second matrix. The resulting matrix will have dimensions equal to the number of rows from the first matrix and the number of columns from the second.
Here is an example of how to code matrix multiplication:
Matrix Matrix::multiply(const Matrix& other) {
if (cols != other.rows) {
throw std::invalid_argument("Matrix A's columns must match Matrix B's rows.");
}
Matrix result(rows, other.cols);
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < other.cols; ++j) {
result.data[i][j] = 0; // Initialize the result cell
for (int k = 0; k < cols; ++k) {
result.data[i][j] += data[i][k] * other.data[k][j];
}
}
}
return result;
}
Transposition The transpose of a matrix is produced by switching its rows and columns. The resulting transposed matrix has its first row equal to the first column of the original, and so on.
The implementation of the transpose function might look like this:
Matrix Matrix::transpose() {
Matrix result(cols, rows); // Notice the switched dimensions
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
result.data[j][i] = data[i][j];
}
}
return result;
}
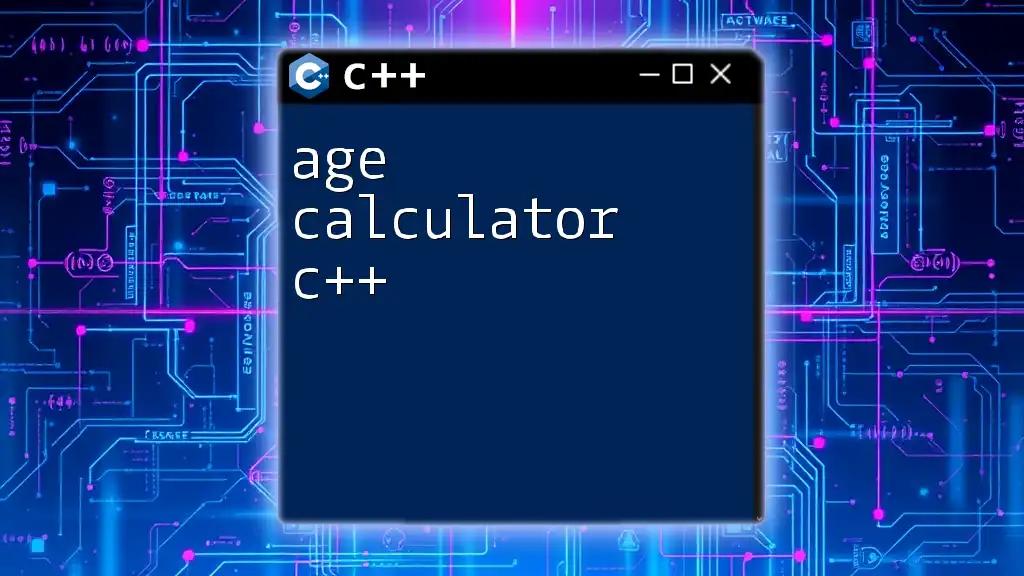
Setting Up the C++ Environment
Choosing a C++ Development Environment
Before diving into coding your matrix calculator in C++, select an integrated development environment (IDE) that suits your needs. Popular choices include Visual Studio, Code::Blocks, or CLion. Make sure you have a C++ compiler installed, such as GCC or MSVC.
Creating Your First C++ Matrix Class
A matrix calculator begins with the creation of a matrix class that encapsulates the properties and methods related to matrix operations. Here’s an example class structure definition:
class Matrix {
private:
int rows;
int cols;
float** data;
public:
Matrix(int r, int c);
~Matrix();
Matrix add(const Matrix& other);
Matrix subtract(const Matrix& other);
Matrix scalarMultiply(float scalar);
Matrix multiply(const Matrix& other);
Matrix transpose();
};
Constructor and Destructor
Managing memory manually is essential in C++, especially when dealing with dynamic arrays. The constructor initializes the matrix with user-defined dimensions, allocating the necessary memory:
Matrix::Matrix(int r, int c) : rows(r), cols(c) {
data = new float*[rows];
for (int i = 0; i < rows; ++i) {
data[i] = new float[cols];
}
}
Similarly, the destructor ensures there are no memory leaks by deallocating the memory allocated for the matrix:
Matrix::~Matrix() {
for (int i = 0; i < rows; ++i) {
delete[] data[i];
}
delete[] data;
}
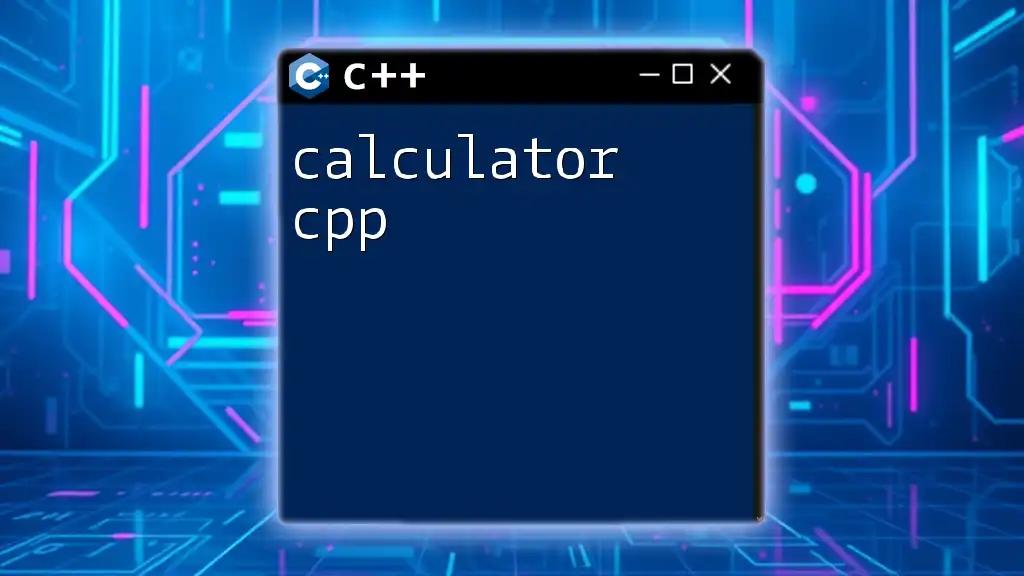
Moving Beyond Basics
Implementing Error Handling
To ensure that your matrix calculator handles edge cases, include error handling mechanisms. For instance, checking if matrices have compatible dimensions before performing operations can prevent runtime errors. Utilizing exceptions in C++ helps manage such inconsistencies gracefully.
Working with Dynamic Sizes
It's essential for your matrix calculator to support dynamic sizing. This allows users to create matrices of varying dimensions without limitations. Utilizing dynamic memory allocation principles ensures that your class remains flexible and robust.
Here’s an example of modifying the constructor to handle arbitrary sizes:
Matrix::Matrix(int r, int c) : rows(r), cols(c) {
// Memory allocation logic
}
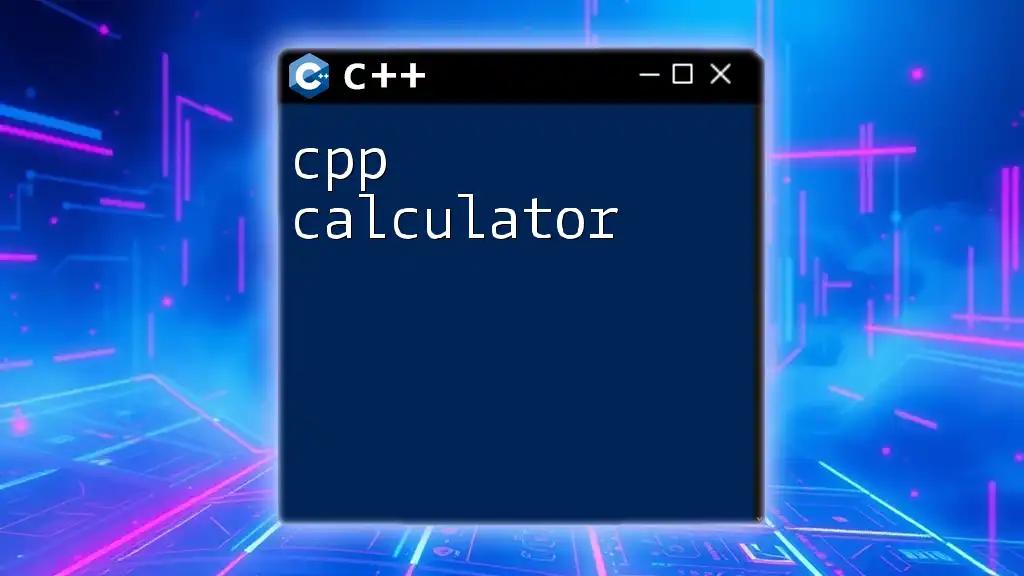
Example Use Cases
Building a Simple Interactive Matrix Calculator
As you build your matrix calculator, consider creating a simple console interface that allows users to interactively request matrix operations. Here’s how your main function could look:
int main() {
int r1, c1, r2, c2;
// Input dimensions and elements for matrix A and B
// Create matrices
Matrix A(r1, c1);
Matrix B(r2, c2);
// Perform operations based on user input
}
Real-World Applications
A matrix calculator in C++ has extensive applications in fields like computer graphics for transformations, machine learning for data manipulation, and even in scientific simulations. Understanding how to manipulate matrices effectively can significantly enhance productivity in these areas.
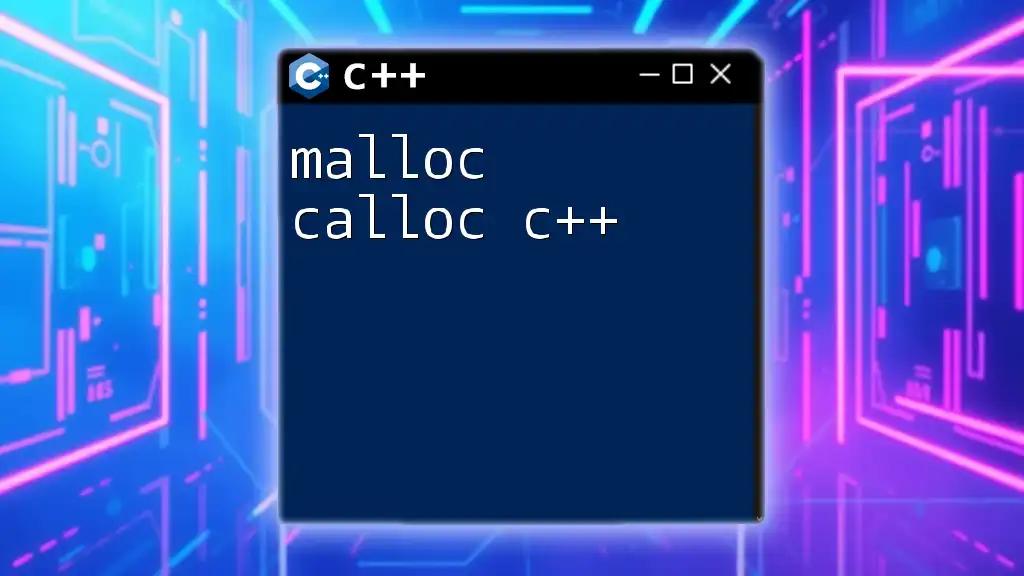
Conclusion
Throughout this guide, you have learned to build a matrix calculator in C++, exploring fundamental concepts, implementations, and how to extend functionality. This foundational understanding empowers you to create customized and complex matrix operations tailored to specific applications. As you continue your programming journey, experiment further with your matrix calculator, discovering new functionalities and applications in diverse fields.
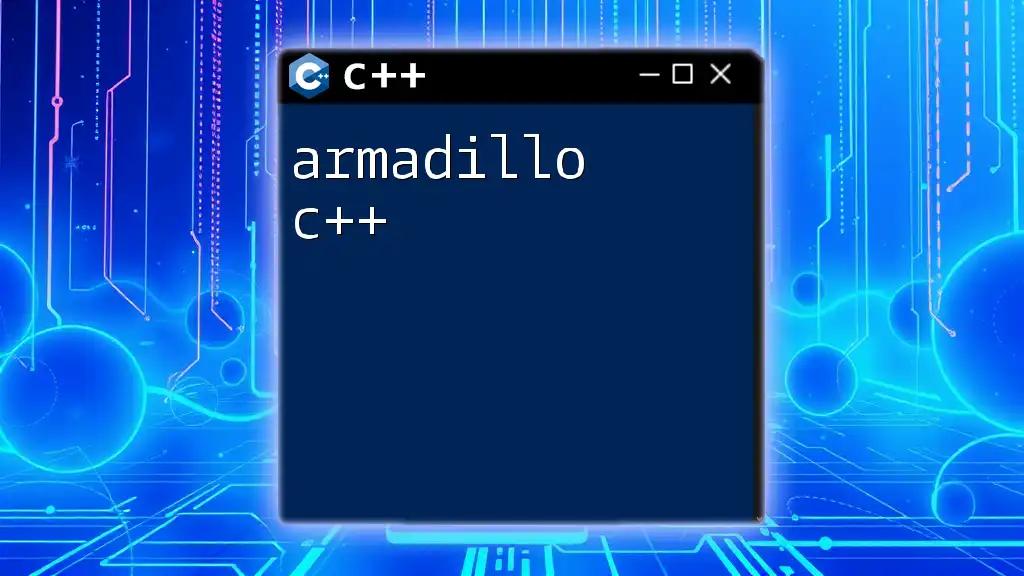
Additional Resources
To deepen your understanding, consider exploring recommended books on linear algebra and C++ programming. Online platforms such as documentation, coding forums, and instructional videos also serve as excellent resources. Stay curious and committed to continuous learning in your C++ programming journey!