`malloc` and `calloc` are C-style memory allocation functions in C++ that allow dynamic memory allocation, where `malloc` allocates uninitialized memory, while `calloc` allocates memory and initializes it to zero.
#include <cstdlib> // For malloc and calloc
#include <iostream>
int main() {
int *arr1 = (int*)malloc(5 * sizeof(int)); // Allocate uninitialized memory for 5 integers
int *arr2 = (int*)calloc(5, sizeof(int)); // Allocate and initialize memory for 5 integers
// Example usage
for (int i = 0; i < 5; ++i) {
std::cout << "arr1[" << i << "] = " << arr1[i] << std::endl; // May contain garbage values
std::cout << "arr2[" << i << "] = " << arr2[i] << std::endl; // Will be 0
}
// Don't forget to free the allocated memory
free(arr1);
free(arr2);
return 0;
}
Understanding Memory Allocation in C++
Dynamic memory allocation is an essential aspect of programming in C++. It allows developers to allocate memory dynamically at runtime, facilitating more flexible and efficient memory management. Understanding how to use functions like `malloc` and `calloc` is crucial for any C++ programmer, as it directly impacts the performance and reliability of software applications.
What is Dynamic Memory Allocation?
Dynamic memory allocation enables programs to request memory during execution rather than at compile time. This is achieved mainly through the heap, a section of memory reserved for dynamic allocation. The key distinction here is between stack memory, which is fixed in size and automatically managed, and heap memory, which requires manual management.
The significance of dynamic memory allocation lies in its flexibility. For example, when the exact amount of memory needed is unknown at compile time or when the program handles large data sets that exceed stack limits, dynamic allocation becomes essential.
Why Use `malloc` and `calloc`?
In C++, effective memory management is vital for creating efficient and robust applications. The two primary functions for dynamic memory allocation are `malloc` (memory allocation) and `calloc` (contiguous allocation).
- Advantages of `malloc` and `calloc`:
- Both functions allow for allocating memory of various sizes without knowing their requirements beforehand.
- They enable handling complex data structures, such as linked lists and trees, by providing the necessary temporary storage.
- Scenarios for Manual Memory Management:
- Working with large arrays or matrices.
- Creating data structures that may grow or shrink in size.
- Situations where performance tuning and optimization are necessary.
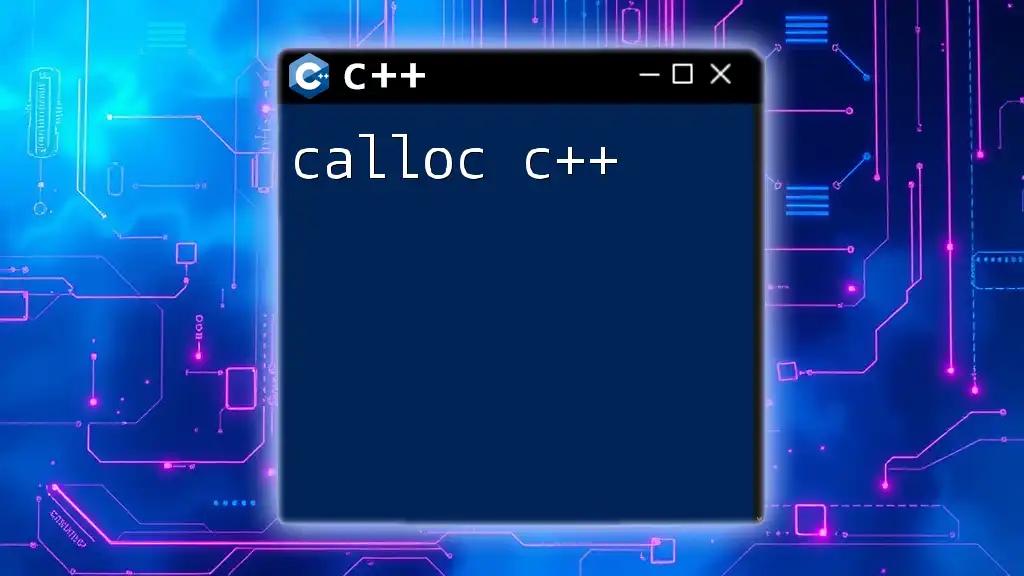
Introduction to `malloc`
What is `malloc`?
`malloc` stands for Memory Allocation. It is a function used to allocate a specified number of bytes of memory on the heap. The general syntax for `malloc` is as follows:
void* malloc(size_t size);
How `malloc` Works
When `malloc` is called, it requests a block of memory from the heap that is `size` bytes long. On success, it returns a pointer to the allocated memory block. If the allocation fails (due to insufficient memory), it returns `NULL`.
One important aspect of memory allocated using `malloc` is that it is uninitialized. This means the values in the allocated memory are indeterminate. Here’s an example:
int* arr = (int*) malloc(5 * sizeof(int));
if (arr == NULL) {
// Handle memory allocation error
}
In this example, an array of 5 integers is allocated. However, the values inside are uninitialized and may contain garbage values.
Common Use Cases of `malloc`
`malloc` is especially useful when dealing with arrays or data structures whose size isn’t known at compile time. For example:
- Dynamic Arrays: When the array size must be determined based on user input or runtime data.
- Linked Lists: As new nodes are added or removed, `malloc` can dynamically adjust memory usage.
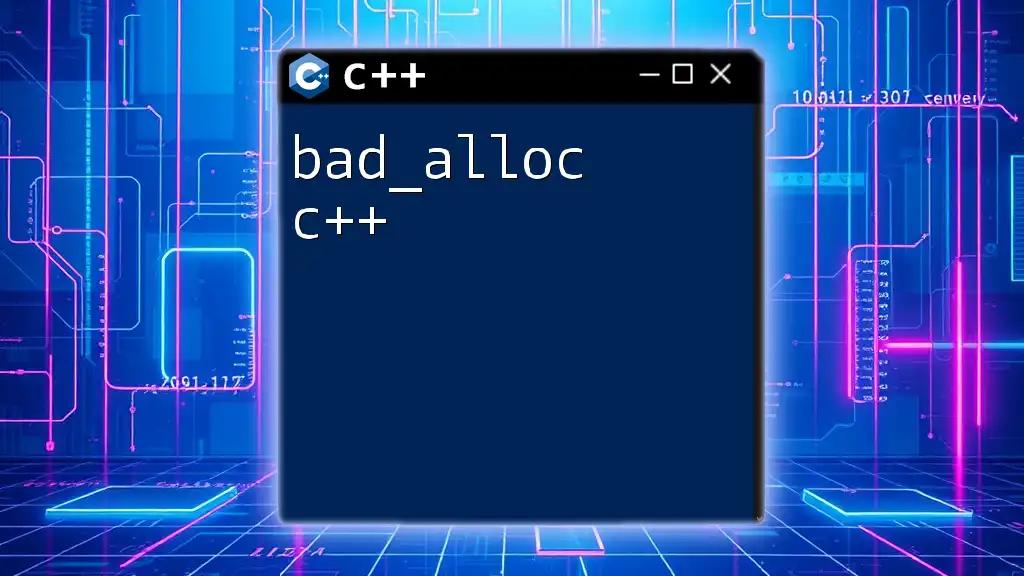
Introduction to `calloc`
What is `calloc`?
`calloc`, or Contiguous Allocation, is another function for memory allocation. Its syntax is as follows:
void* calloc(size_t num, size_t size);
How `calloc` Works
Unlike `malloc`, `calloc` not only allocates memory but also initializes all bits to zero. This can help prevent bugs related to uninitialized memory.
int* arr = (int*) calloc(5, sizeof(int));
if (arr == NULL) {
// Handle memory allocation error
}
In this example, an array of 5 integers is allocated, and all elements are initialized to zero.
Common Use Cases of `calloc`
`calloc` is beneficial when you want to ensure all values in a data structure start at a known state, particularly zero. Use cases include:
- Initializing Arrays: Guarantees zero-initialization for integer arrays.
- Data Structures: When creating nodes for linked lists or trees, ensuring that pointers start as `nullptr`.
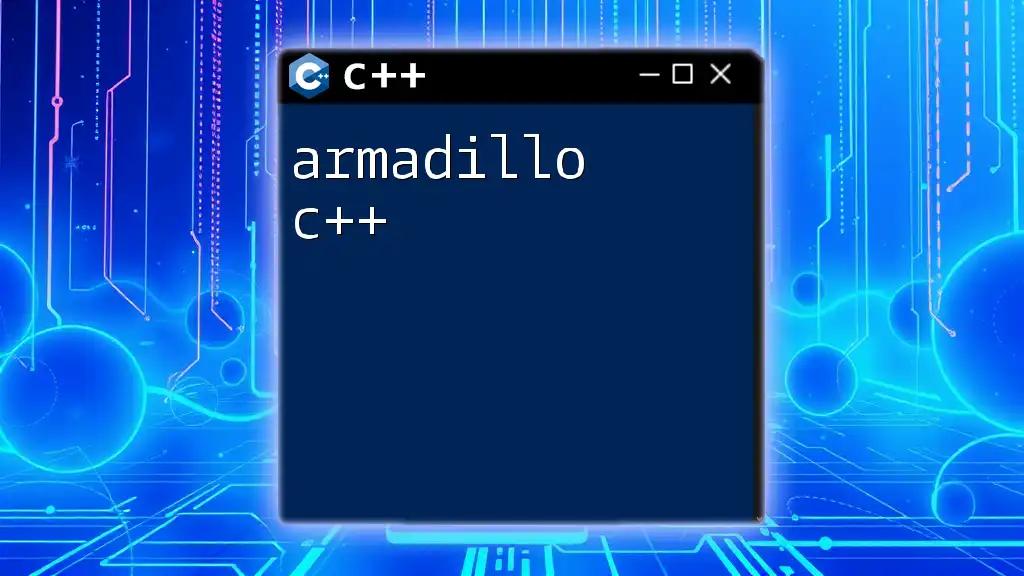
Key Differences Between `malloc` and `calloc`
Comparison of `malloc` and `calloc`
Feature | `malloc` | `calloc` |
---|---|---|
Initialization | Uninitialized | Zero-initialized |
Parameters | Single argument (size) | Two arguments (num, size) |
Return Type | void* pointer | void* pointer |
When to Use Each Function
Use `malloc` when:
- You need raw memory without initialization.
- Performance is critical, and you want to avoid the overhead of zero-initialization.
Use `calloc` when:
- You require memory initialized to zero, preventing garbage values.
- You need a dynamic multi-dimensional array and want to initialize it seamlessly.

Memory Management Best Practices in C++
Avoiding Memory Leaks
Memory leaks occur when allocated memory is not properly freed, leading to wasted memory resources over time. To avoid memory leaks, it’s crucial to ‘free’ the allocated memory when it’s no longer needed.
free(arr);
Proper Use of `free`
Whether using `malloc` or `calloc`, every allocation must be paired with a corresponding `free` call. Failing to do so can lead to memory fragmentation and leaks.
Here’s an example that includes both allocation and deallocation:
int* arr = (int*) malloc(5 * sizeof(int));
if (arr == NULL) {
// Handle memory allocation error
}
// Use the array
free(arr); // Free the allocated memory
arr = NULL; // Avoid dangling pointers
Common Mistakes to Avoid
- Forgetting to `free` allocated memory.
- Calling `free` on memory pointers that weren't allocated with `malloc` or `calloc`.
- Using pointers after they're freed, creating undefined behavior (dangling pointers).
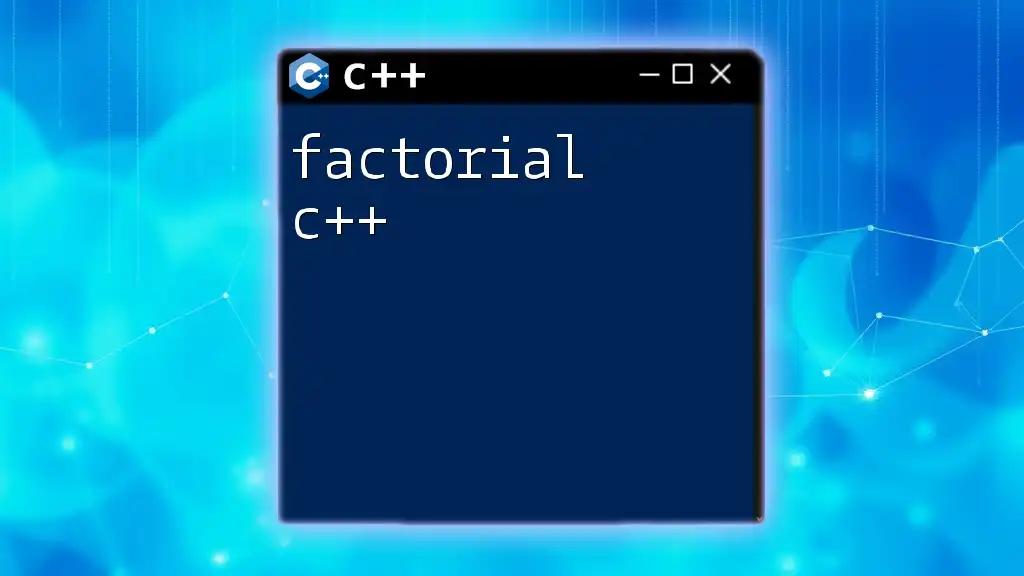
Alternatives to `malloc` and `calloc`
Using C++ STL Containers
In modern C++, it is often recommended to use STL containers such as `std::vector` and smart pointers like `std::unique_ptr` and `std::shared_ptr` for better memory management.
STL containers automatically manage memory, making them less prone to leaks and errors. For example:
std::vector<int> vec(5); // Automatically manages memory
Using these containers helps minimize memory management risks and simplifies the code.
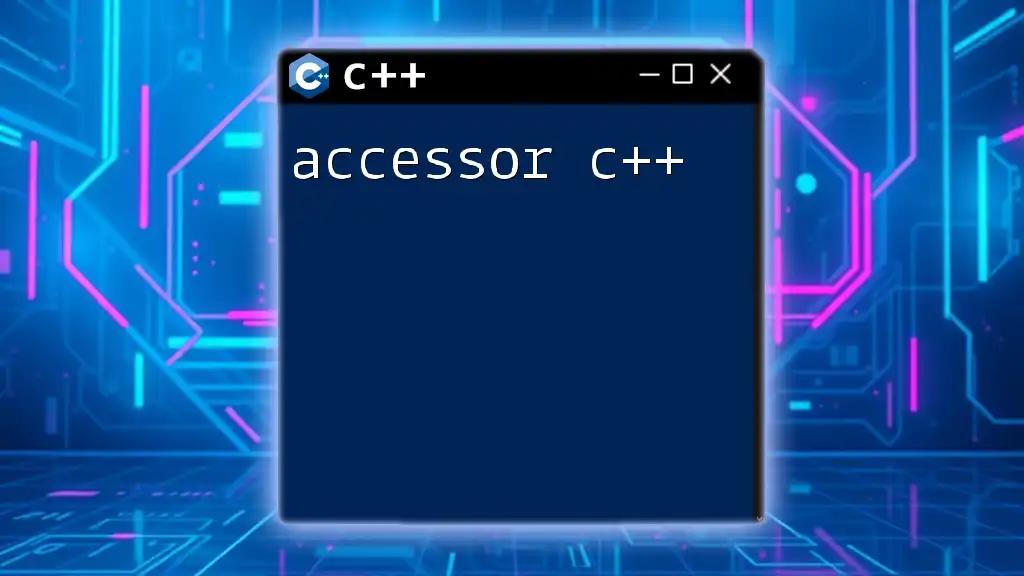
Conclusion
Understanding malloc, calloc, and dynamic memory allocation is fundamental for C++ programming. By utilizing these functions effectively, you can manage memory in a way that optimizes performance while mitigating memory-related issues such as leaks and segmentation faults.
As you refine your skills, remember to balance the power of manual memory management with modern alternatives that make code safer and more maintainable. Continuous practice and exploration will enhance your proficiency in mastering memory management in C++.
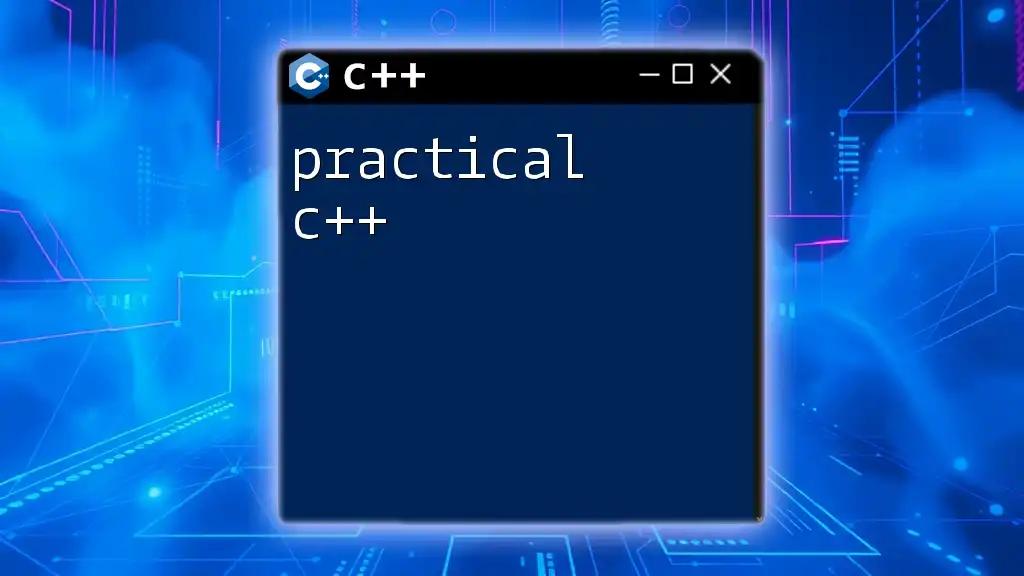
Additional Resources
For deeper learning, consider exploring the following:
- Recommended books on C++ memory management techniques.
- Online courses that include hands-on memory management practices.
- Official C++ documentation for `malloc`, `calloc`, and memory management practices.

FAQs
Common Questions Related to `malloc` and `calloc`
-
What is the primary difference between `malloc` and `calloc`?
- `malloc` does not initialize memory, while `calloc` initializes all bits to zero.
-
What happens if I forget to free allocated memory?
- You will encounter memory leaks, which can lead to increased memory consumption and eventual program failure.
Understanding the nuances of `malloc`, `calloc`, and memory management in general will substantially improve your C++ programming skills and provide you with the tools to write more efficient and reliable applications.