The Armadillo C++ library is an efficient and user-friendly linear algebra library that provides easy-to-use functionalities for matrix operations and scientific computing.
#include <iostream>
#include <armadillo>
int main() {
arma::mat A = arma::randu<arma::mat>(4, 4); // Create a 4x4 matrix with random values
arma::mat B = arma::randu<arma::mat>(4, 4); // Create another 4x4 matrix
arma::mat C = A * B; // Matrix multiplication
C.print("Result of A * B:"); // Print the result
return 0;
}
What is Armadillo?
The Armadillo C++ library is a powerful tool designed for linear algebra operations, facilitating matrix and vector math in a user-friendly manner. This library is particularly popular among scientists, engineers, and data analysts who require efficient and reliable computation for their applications. With its intuitive syntax, Armadillo provides a convenient interface to utilize complex mathematical operations without needing to delve into intricate details.
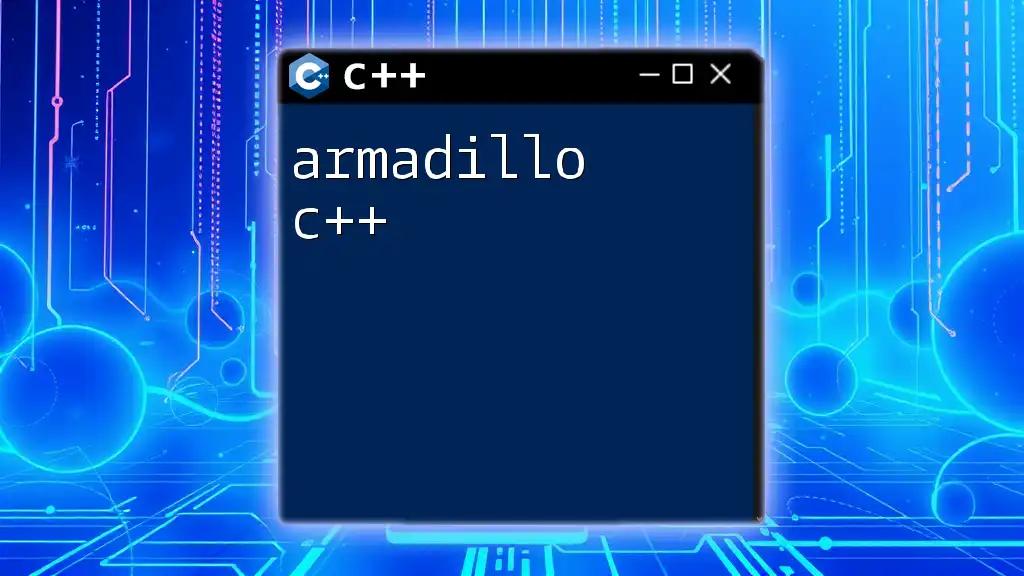
Key Features
Armadillo includes a range of essential features:
- Matrix and vector operations: Supports creating, manipulating, and performing operations on matrices and vectors.
- Built-in algorithms: Offers various algorithms for statistics, linear algebra, and optimization.
- Versatile data types: Supports integer, floating-point, and complex number types, accommodating a broad spectrum of applications.
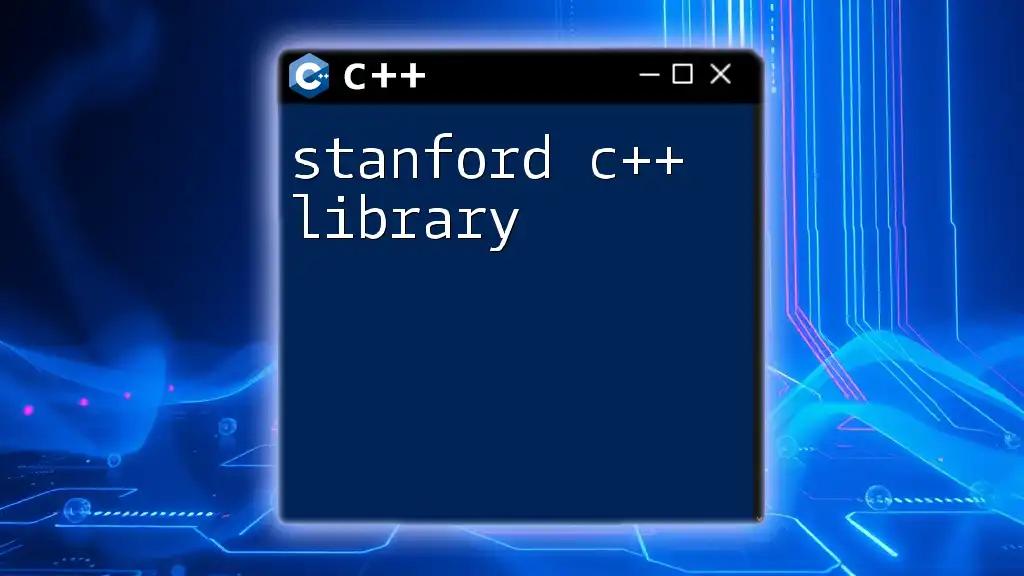
Getting Started with Armadillo
Installation Guide
To begin working with the Armadillo C++ library, you need to install it on your system. Here’s how:
-
Download options: You can obtain Armadillo from its [GitHub repository](http://arma.sourceforge.net/download.html) or use pre-compiled binaries depending on your operating system.
-
Required dependencies: Armadillo relies on LAPACK and BLAS for matrix computations. Make sure these libraries are installed as well.
-
Basic installation steps: Follow the specific instructions for Windows, macOS, or Linux, ensuring that the Armadillo library is properly linked with your project.
Setting Up Your Environment
To start using Armadillo in your C++ projects, you must include it in your source files. Begin by adding the following line at the beginning of your code:
#include <armadillo>
This statement allows you access to all functionality provided by the Armadillo C++ library.
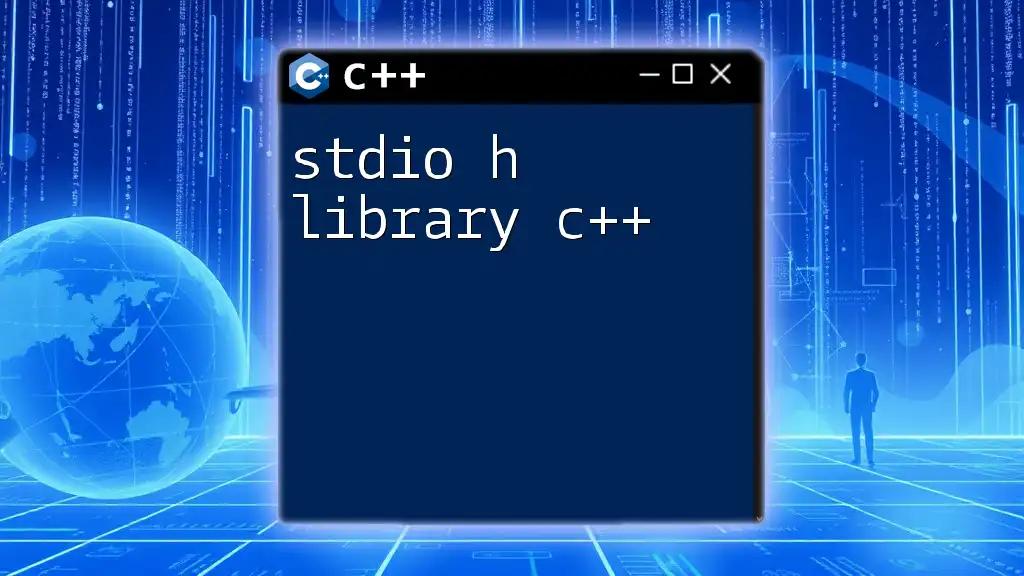
Core Functionality of Armadillo
Basic Data Structures
Creating Matrices and Vectors
The foundation of Armadillo lies in its matrix and vector classes. You can create matrices using the `mat` class and vectors using the `vec` class. Here’s how to create a random 3x3 matrix and a vector:
arma::mat A(3, 3, arma::fill::randu); // Random matrix with values between 0 and 1
arma::vec b = {1.0, 2.0, 3.0}; // Vector initialized with specific values
Matrix Initialization Options
Armadillo provides multiple ways to initialize matrices. You can fill matrices with zeros, ones, random values, or specific constructs like identity matrices. For instance:
arma::mat Z = arma::zeros<arma::mat>(3, 3); // 3x3 matrix of zeros
arma::mat I = arma::eye<arma::mat>(3, 3); // 3x3 identity matrix
Matrix Operations
Addition and Subtraction
Armadillo simplifies basic arithmetic operations on matrices and vectors. For addition and subtraction, you can do the following:
arma::mat C = A + A; // Matrix addition
arma::mat D = A - Z; // Matrix subtraction
Matrix Multiplication
Matrix multiplication is a core part of linear algebra, and Armadillo handles both dot product and element-wise multiplication:
arma::mat E = A * A; // Matrix multiplication
arma::mat F = A % A; // Element-wise multiplication
Transpose and Inversion
The library makes it straightforward to calculate the transpose and inverse of matrices:
arma::mat At = A.t(); // Transposing the matrix
arma::mat A_inv = inv(A); // Inversion of the matrix
Advanced Operations
Eigenvalues and Eigenvectors
Armadillo includes functionality for calculating eigenvalues and eigenvectors, essential in various applications like stability analysis and quantum mechanics:
arma::vec eigval;
arma::mat eigvec;
arma::eig_sym(eigval, eigvec, A); // Compute eigenvalues and eigenvectors
Solving Systems of Equations
An important application of the Armadillo C++ library is solving systems of linear equations, represented as Ax = b. You can use the `solve` function:
arma::vec solution = solve(A, b); // Solve Ax = b for vector x
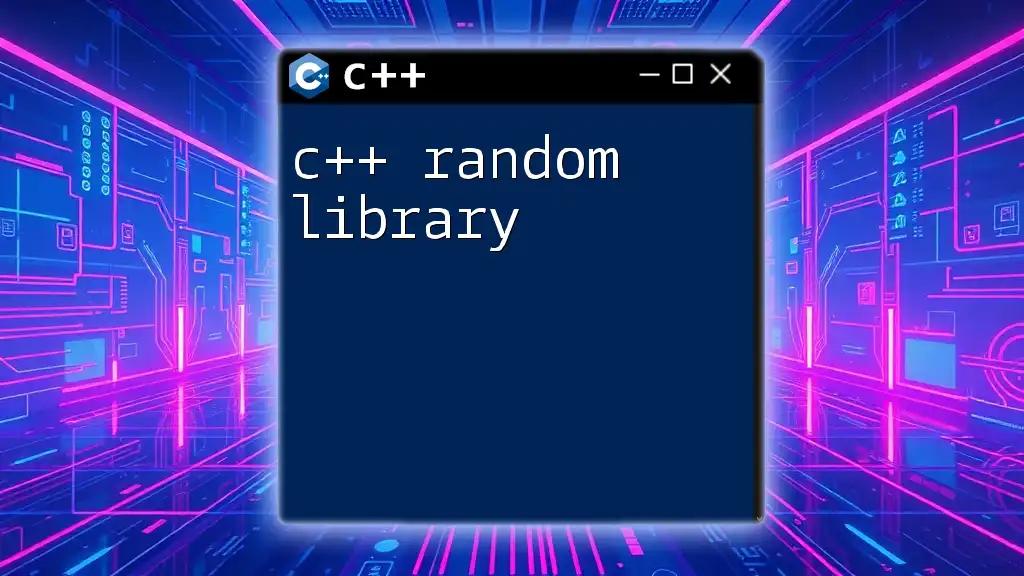
Armadillo's Built-in Functions and Algorithms
Statistical Functions
Armadillo offers various statistical functions, enabling easy computation of means, standard deviations, variances, etc. For instance, you can easily calculate a mean and standard deviation:
double mean_val = arma::mean(b); // Calculate the mean of the vector
double stddev_val = arma::stddev(b); // Calculate the standard deviation
Optimization
Armadillo provides routines for optimization tasks, enabling you to perform tasks such as curve fitting and parameter optimization efficiently.
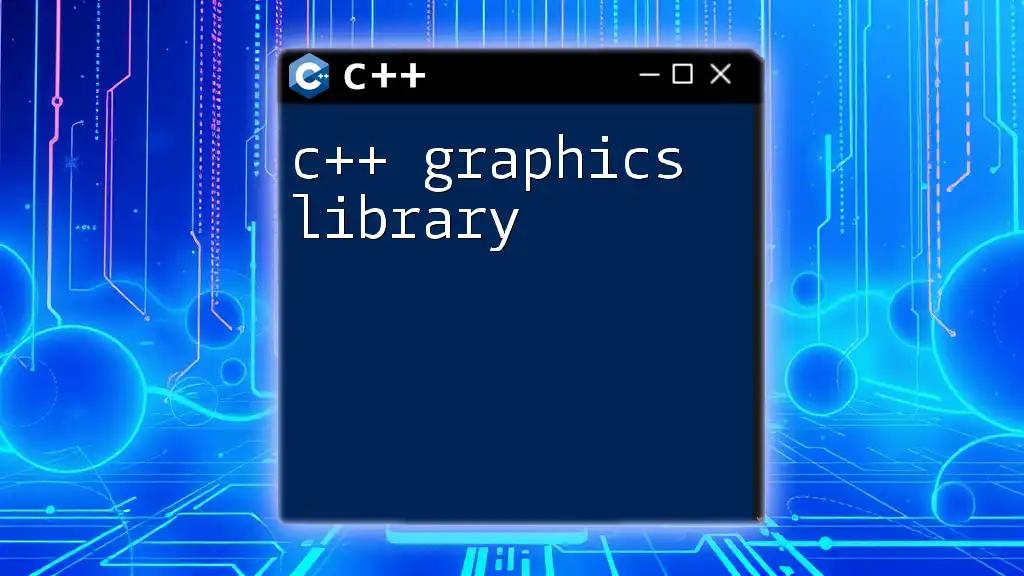
Integrating Armadillo with Other Libraries
Compatibility with NumPy and C
One of Armadillo's strengths is its ability to integrate seamlessly with Python through NumPy, allowing data scientists to leverage both languages' strengths. You can pass data between Armadillo and NumPy with ease, enhancing your computational capabilities.
Using Armadillo with Other C++ Libraries
Armadillo is often used alongside libraries such as OpenCV for image processing and Boost for additional C++ functionalities. This combination ensures that you have a robust toolkit for tackling complex problems.
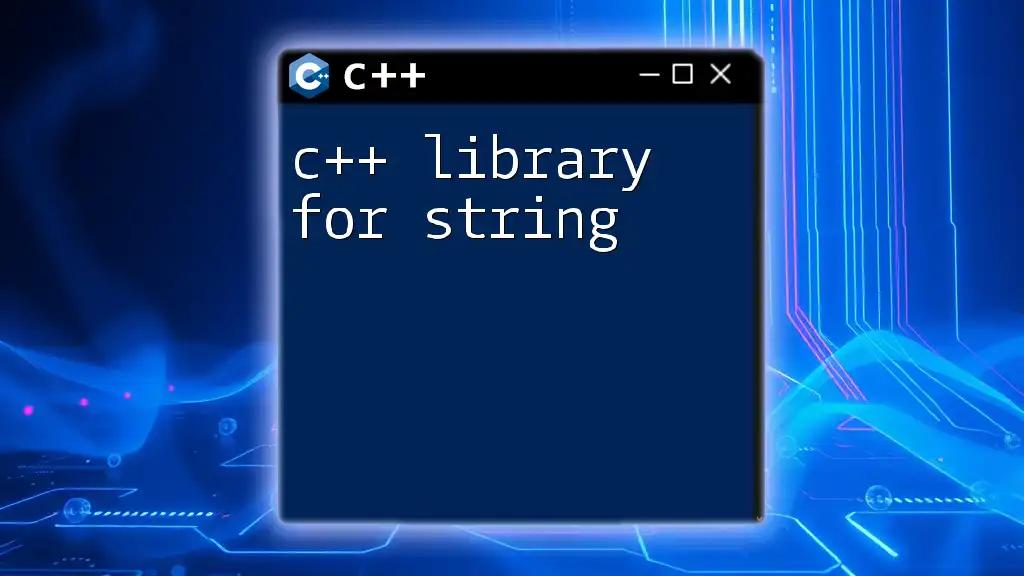
Common Use Cases for Armadillo
Machine Learning
In the realm of machine learning, the Armadillo C++ library proves invaluable for tasks requiring linear algebra operations. Whether implementing regression models or neural networks, Armadillo simplifies numerous algorithms.
Scientific Computing
Armadillo is widely utilized in scientific computing. Researchers in fields such as physics and engineering benefit from its efficiency, particularly in simulations and mathematical modeling.
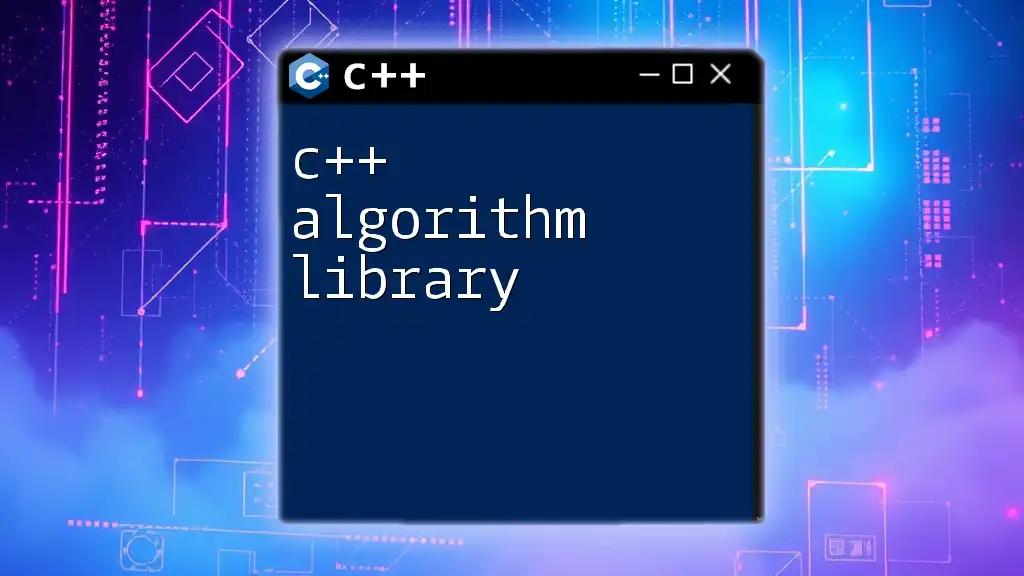
Best Practices
Optimization for Performance
To maximize performance when using Armadillo, ensure that your matrices and vectors are appropriately sized and avoid unnecessary copies where possible. Use Armadillo's built-in functions instead of writing custom code wherever feasible.
Debugging Techniques
When working with Armadillo, some common pitfalls include mismatched dimensions during operations. Ensure matrices and vectors are compatible before performing operations, and leverage Armadillo's error messages to troubleshoot issues effectively.
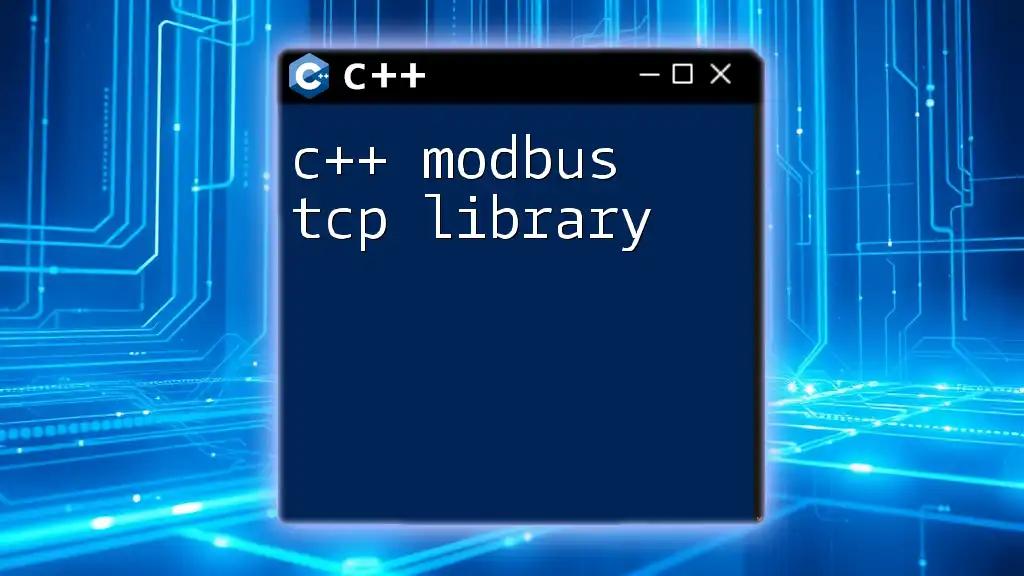
Conclusion
The Armadillo C++ library is a powerful ally for any programmer involved in mathematical computations. By streamlining complex operations into simple commands, it opens the door for efficient, readable, and maintainable code.
Get Involved
To deepen your knowledge of Armadillo, explore its comprehensive [official documentation](http://arma.sourceforge.net/docs.html) and consider joining community forums for collaboration and support.
Call to Action
Begin experimenting with the Armadillo C++ library in your projects today, and unlock the full potential of linear algebra in your C++ applications.
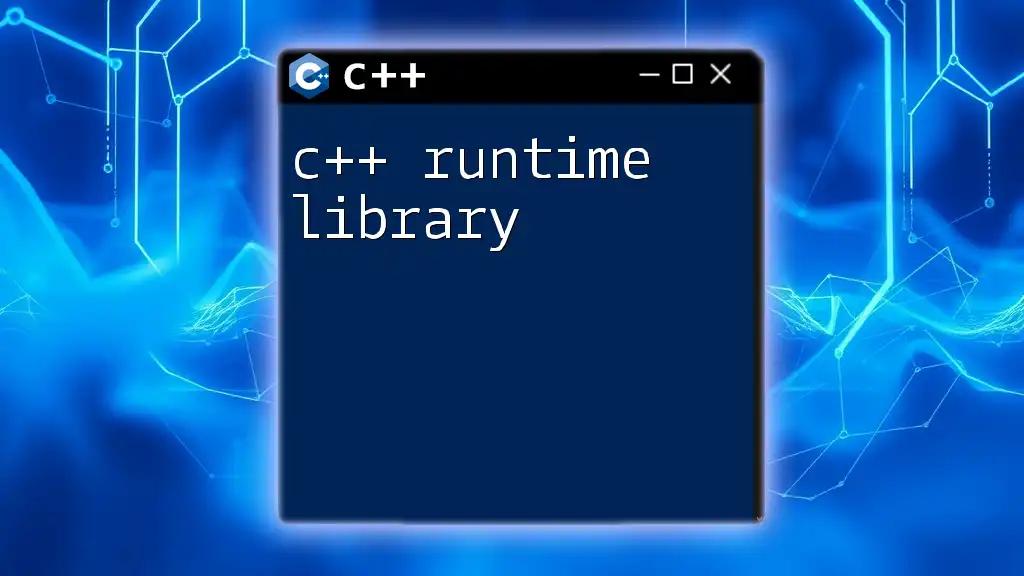
Additional Resources
Official Documentation
For detailed insights into the functions and features of Armadillo, visit the official documentation.
Community and Support
Engage with the community for support and tips to enhance your experience with Armadillo.