A C++ Modbus TCP library enables communication between a device and a server using the Modbus protocol over TCP/IP, allowing for efficient data exchange in industrial automation applications.
Here’s a simple code snippet demonstrating how to set up a Modbus TCP client using a hypothetical library:
#include <ModbusTCP.h>
ModbusTCPClient modbusClient;
void setup() {
modbusClient.begin("192.168.1.10", 502); // Set up the client with the server's IP and port
}
void loop() {
uint16_t value;
if (modbusClient.readHoldingRegisters(0, 1)) { // Read holding register at address 0
value = modbusClient.getResponseBuffer(0); // Get the value
Serial.println(value); // Print the value to the serial monitor
}
delay(1000); // Wait for a second before the next read
}
Introduction to Modbus and C++
What is Modbus?
Modbus is a communication protocol developed in the late 1970s for industrial applications. Originally created for connecting industrial electronic devices, it has since become a de facto standard in networking and data communications. The protocol facilitates the exchange of data in a master-slave architecture, where a master device requests data from one or multiple slave devices.
Modbus can function in three main modes: RTU, ASCII, and TCP. While RTU and ASCII are serial modes (utilizing RS-232 or RS-485 interfaces), Modbus TCP operates over Ethernet using the TCP/IP stack, allowing for faster and more robust communication suitable for modern networking environments.
Why Use C++ for Modbus Implementation?
C++ is a powerful programming language known for its performance and versatility. By utilizing C++ for implementing a Modbus TCP library, developers can harness both low-level hardware control and high-level abstraction. This combination is essential for real-time applications where performance is critical, such as industrial automation and control systems.
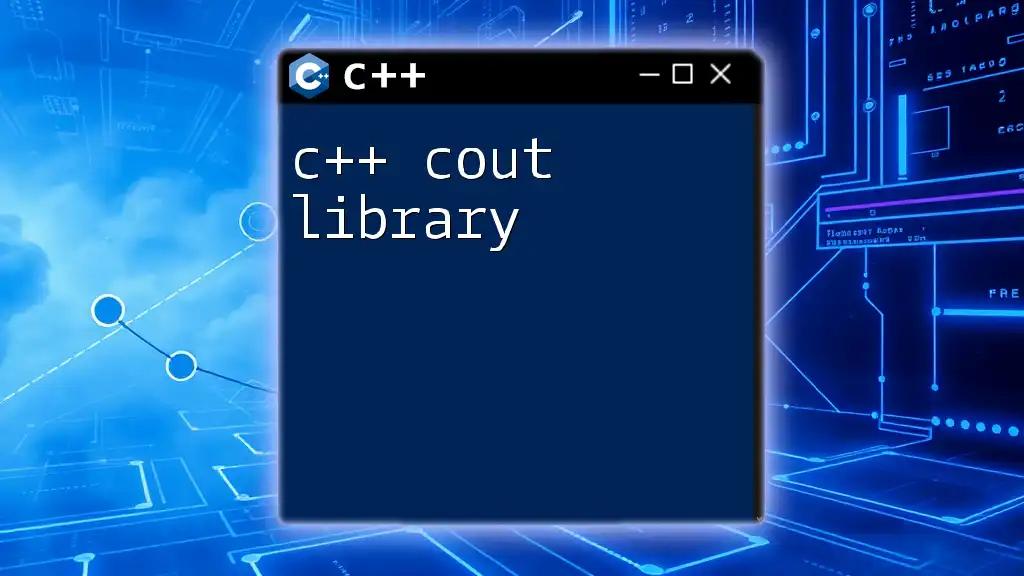
Setting Up Your C++ Development Environment
Required Tools and Libraries
Before diving into coding, ensure you have the right tools. You'll need a C++ compiler (like GCC or Clang), an Integrated Development Environment (IDE) such as Visual Studio, Code::Blocks, or CLion, and a Modbus TCP library. Popular choices include libmodbus, TinyModbus, and ModbusCPP.
Installing a Modbus TCP Library
Here’s how you can install libmodbus, a widely-used C++ Modbus TCP library:
-
Download the library: Clone the libmodbus repository from GitHub:
git clone https://github.com/stephane/libmodbus.git
-
Compile the library: Navigate to the downloaded directory and compile it using the following commands:
cd libmodbus ./autogen.sh ./configure make sudo make install
This step installs the library on your system, making it ready for your project.
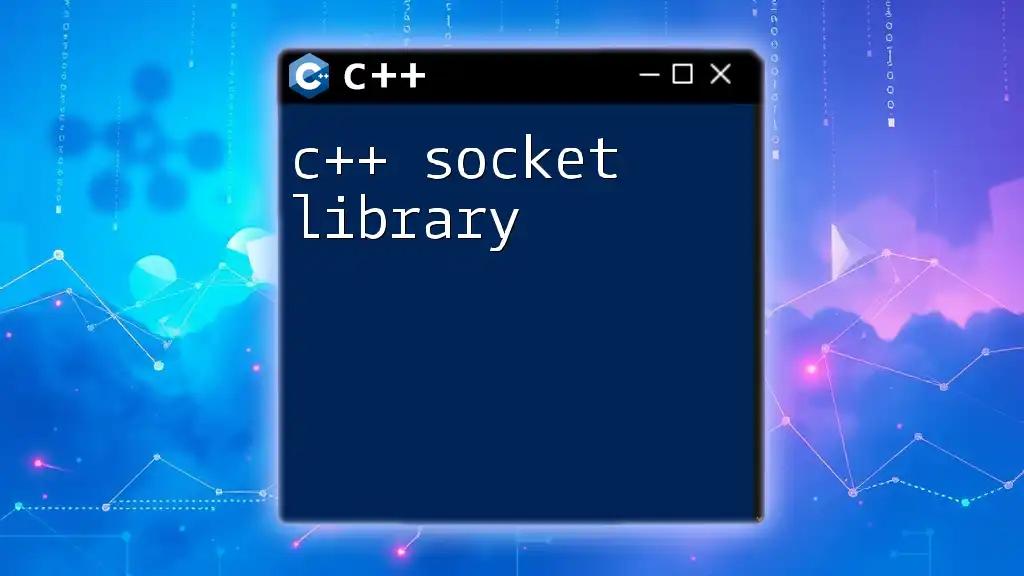
Understanding the Modbus TCP Protocol
Basics of Modbus TCP Communication
Modbus TCP introduces a straightforward method for devices to communicate over an Ethernet network. Unlike traditional Modbus protocols, which require serial communications, Modbus TCP simultaneously supports numerous devices, enhancing scalability.
Modbus TCP packets encapsulate requests and responses, enabling efficient data exchanges. Understanding how TCP/IP works (specifically, the client-server model) is crucial for implementing any Modbus TCP interactions properly.
Modbus Function Codes
Function codes are essential aspects of the Modbus protocol. They specify the type of action that a Modbus master wishes to perform. Some commonly used function codes include:
- Read Coils (0x01): Retrieves the status of a set of coils (digital signals).
- Read Holding Registers (0x03): Reads the contents of any specified holding registers.
- Write Multiple Registers (0x10): Allows writing multiple registers in a single operation.
These codes determine the operations performed during communication, making it imperative to grasp their syntax and application.
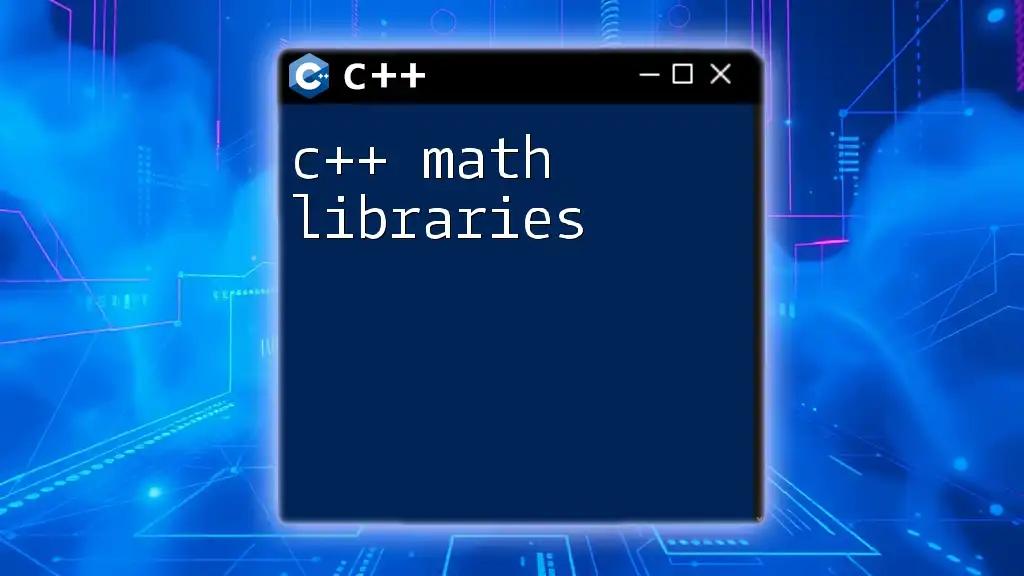
Using C++ Modbus TCP Library
Creating a Simple Modbus TCP Client
Creating a simple Modbus TCP client using `libmodbus` is straightforward. Below is a basic implementation:
#include <modbus.h>
#include <stdio.h>
#include <stdlib.h>
int main() {
modbus_t *ctx = modbus_new_tcp("192.168.1.100", 502);
if (modbus_connect(ctx) == -1) {
fprintf(stderr, "Connection failed: %s\n", modbus_strerror(errno));
return -1;
}
uint16_t tab_reg[32];
int rc = modbus_read_registers(ctx, 0, 32, tab_reg);
if (rc == -1) {
fprintf(stderr, "Read failed: %s\n", modbus_strerror(errno));
return -1;
}
for (int i=0; i < rc; i++) {
printf("Reg[%d]: %d\n", i, tab_reg[i]);
}
modbus_close(ctx);
modbus_free(ctx);
return 0;
}
In this code snippet, we create a Modbus TCP client that connects to a server and reads two holding registers. Each segment of the code is crucial for understanding how Modbus operates using C++. Always handle errors properly to ensure robust communication.
Communicating with a Modbus Server
Once your client is set up, establishing communication with a Modbus server allows you to perform operations. For example, consider reading from coils:
uint8_t tab_bits[32];
int rc = modbus_read_bits(ctx, 0, 32, tab_bits);
if (rc == -1) {
fprintf(stderr, "Read bits failed: %s\n", modbus_strerror(errno));
return -1;
}
for (int i = 0; i < rc; i++) {
printf("Bit[%d]: %d\n", i, tab_bits[i]);
}
This code reads the first 32 coils from the Modbus server and displays their status. As you create your applications, remember to adjust the starting addresses and counts based on the specific project requirements.
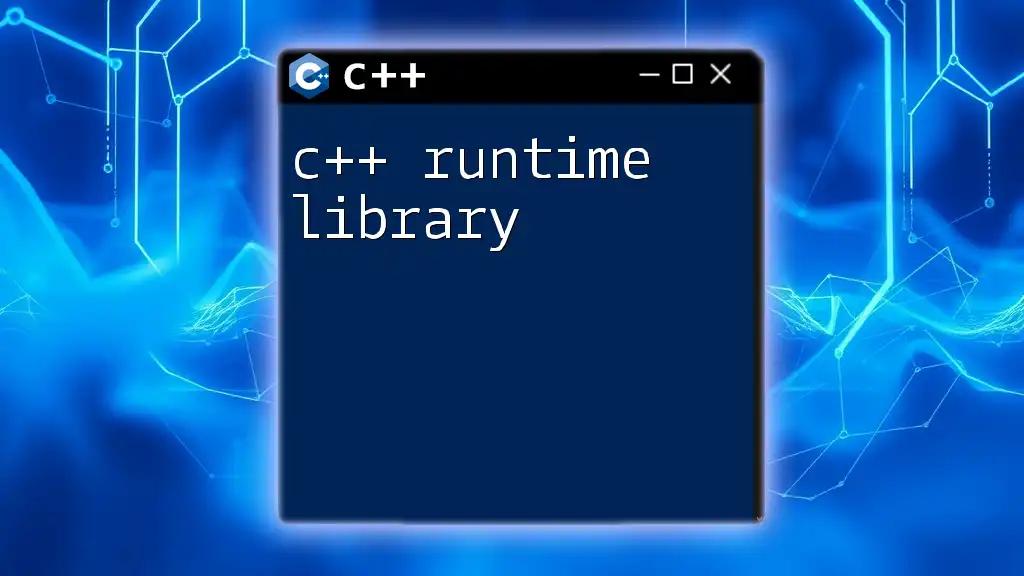
Advanced Functions and Features
Error Handling in Modbus TCP
Error handling is paramount in any communication protocol. The Modbus protocol defines a range of exceptions and errors that can be thrown. Implementing effective error checking enhances the reliability of your application. The following is an example of how to handle errors during communication:
if (modbus_connect(ctx) == -1) {
fprintf(stderr, "Connection error: %s\n", modbus_strerror(errno));
// Handle reconnection or fallback logic here
}
Whenever a communication error occurs, always log the error messages for debugging purposes.
Implementing Asynchronous Communication
While synchronous communication is common, implementing asynchronous methods can greatly enhance the performance of your application. Asynchronous communication frees your main thread for other tasks while awaiting responses. Such methods are essential for real-time applications needing fast response times.
Example of an asynchronous Modbus TCP client:
// Pseudo code for asynchronous behavior
void async_modbus_read() {
// Initiate Modbus read
// Non-blocking calls that handle responses as they arrive
// Use callback functions or event-loops to manage the workflow
}
By focusing on asynchronous methods, you can create responsive applications that interact with multiple devices concurrently.
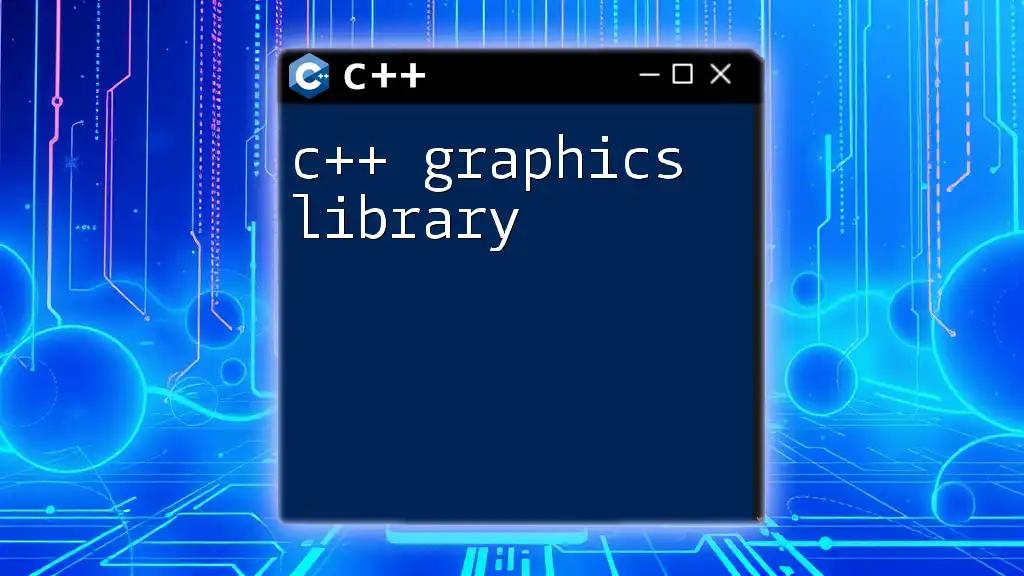
Real-World Applications of Modbus TCP and C++
Industries Utilizing Modbus TCP
Modbus TCP serves various industries including manufacturing, energy production, and transportation. Its robustness and reliability make it ideal for applications such as:
- Industrial Automation: Allows control and monitoring of machinery.
- Building Management Systems: Facilitates control over HVAC and lighting systems.
- IoT Applications: Connects industrial devices within Internet of Things frameworks for data exchange.
Case Study: Building a Smart Home System using C++ and Modbus TCP
Consider a project that aims to create a smart home system leverages the Modbus TCP library. This system could include connected light switches, thermostats, and security systems, all managed via a central server program developed in C++.
The overall architecture integrates Modbus TCP devices across a home network, allowing secure and efficient communication. By employing the strategies outlined in this guide, developers can implement a working prototype in a short time frame.
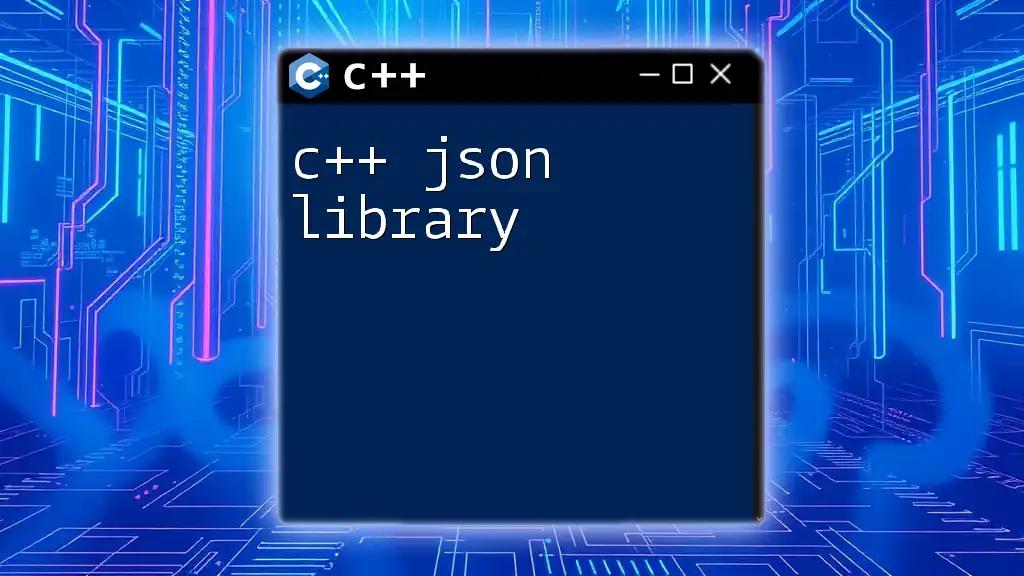
Testing and Debugging
Tools for Testing Modbus TCP Communication
To ensure that your Modbus TCP applications are robust, regular testing is essential. Tools such as Modbus Poll and QModMaster allow for simulating a Modbus server, making it easier to test and debug.
These tools help verify if your application can connect to and communicate with Modbus servers effectively, providing a user-friendly interface for testing function codes.
Debugging Common Issues
Common issues when developing with the C++ Modbus TCP library may include communication timeouts, incorrect function codes, or server response errors.
To effectively debug these issues:
- Log every request and response: This allows you to trace the exact sequence of events leading to an error.
- Use timing analysis: Evaluate response times, as excessive delays may indicate network issues.
Incorporating these debugging strategies will not only help identify bugs early but also improve the overall user experience.
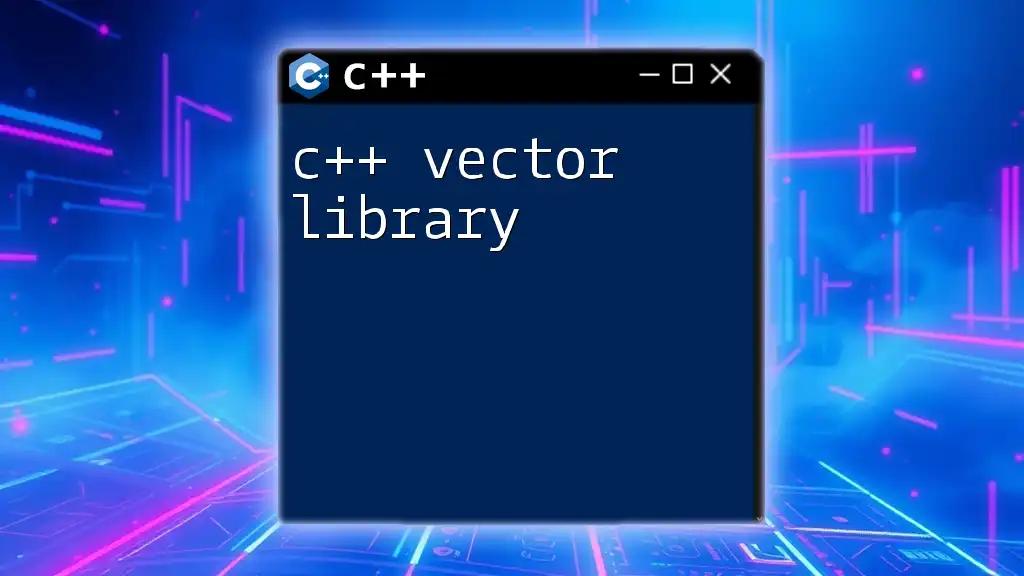
Conclusion
By mastering the intricacies of the C++ Modbus TCP library, developers can effectively communicate with various industrial devices, bringing their automation projects to life. From the setup of client-server architecture to advanced features like asynchronous communication, mastering this library opens a multitude of application possibilities in diverse industries.
Future Learning Paths
As you progress, consider delving deeper into advanced automation topics surrounding Modbus and C++. Engaging with online communities, reading the official documentation, and participating in relevant courses will enhance your skills and knowledge in this domain.
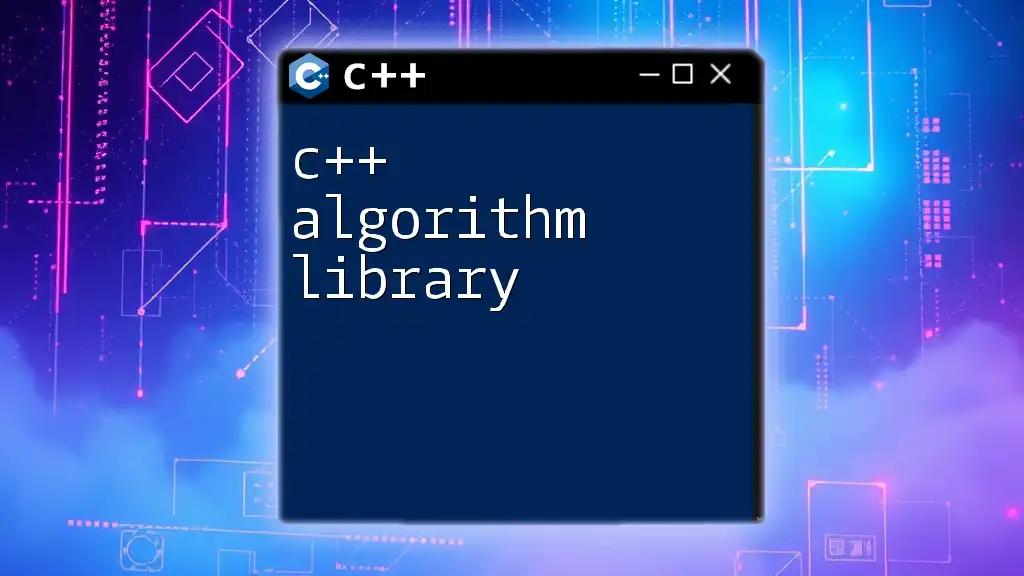
References
For further reading, check out:
- The official [Modbus protocol specification](https://modbus.org/specs.php)
- [libmodbus documentation](https://libmodbus.org/)
- Online forums and communities focused on C++ programming and industrial automation.
Call to Action
Now it’s your turn! Start implementing a project using the ideas outlined in this article, and don’t hesitate to reach out to our community for support and further learning. Whether you’re an expert or a beginner, the world of C++ and Modbus awaits you!