A Dynamic Link Library (DLL) in C++ is a collection of reusable code and resources that can be loaded and executed by applications at runtime, allowing for efficient memory use and modular programming.
Here's a simple example of how to load a DLL and use a function from it:
// LoadDLLExample.cpp
#include <iostream>
#include <windows.h>
typedef int (*AddFunction)(int, int);
int main() {
HMODULE hDll = LoadLibrary("MyLibrary.dll");
if (hDll) {
AddFunction add = (AddFunction)GetProcAddress(hDll, "Add");
if (add) {
int result = add(5, 3);
std::cout << "Result: " << result << std::endl;
}
FreeLibrary(hDll);
} else {
std::cerr << "Could not load the DLL." << std::endl;
}
return 0;
}
What is a Dynamic Link Library (DLL)?
A Dynamic Link Library (DLL) is a collection of small programs, or modules, that can be loaded and executed by applications. These libraries provide a way to modularize code so that multiple programs can share libraries for common functionalities without duplicating code. In contrast to static libraries, which are copied into the final executable at compile time, DLLs are executed at runtime, providing increased flexibility in memory management and resource allocation.
Benefits of Using DLLs
Using dynamic link libraries comes with several advantages:
- Code Reusability and Modularity: With DLLs, developers can create distinct modules for specific functionalities, allowing them to reuse code across multiple programs without modification.
- Memory Management Advantages: Since DLLs are loaded into memory only when required, they help conserve system resources, allowing more memory to be allocated elsewhere when needed.
- Patching and Versioning: Updating a DLL does not require recompiling dependent applications. This makes it easier to patch or update functionalities without requiring a change in the calling applications.
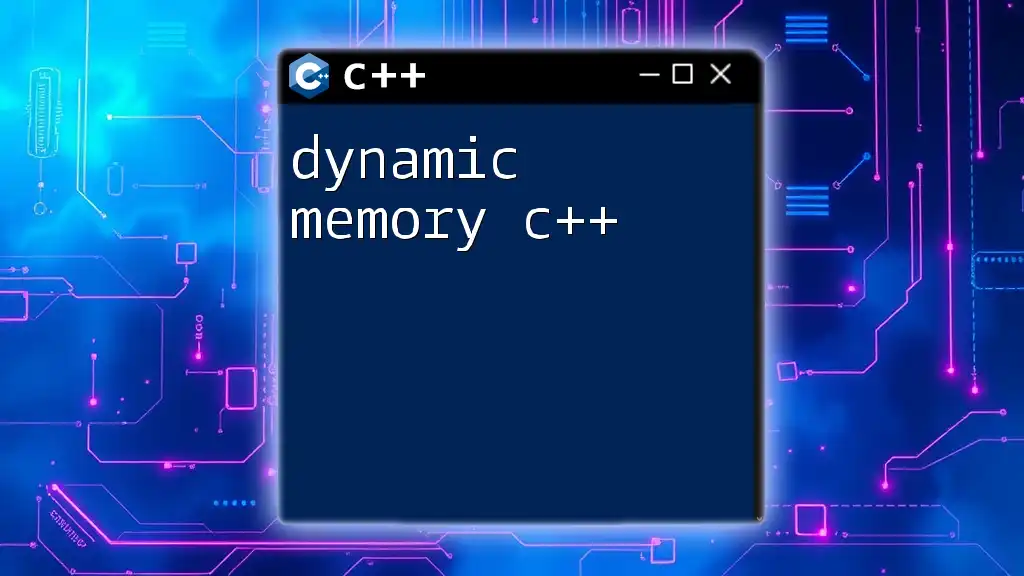
Understanding C++ Dynamic Link Libraries
How DLLs Work in C++
DLLs allow for dynamic linking, where a program does not have to be compiled and linked with the library. Instead, the linking occurs at runtime. This is achieved through the use of import and export tables that manage function and variable access between the application and the DLL.
Key Components of a DLL
A DLL comprises several key components:
- Header Files and Function Definitions: Header files declare the functions that will be available to applications using the DLL.
- Exporting Functions: Functions in the DLL must be designated for export using the `__declspec(dllexport)` keyword.
- Importing Functions: Applications that use the DLL need to set function declarations with `__declspec(dllimport)`.
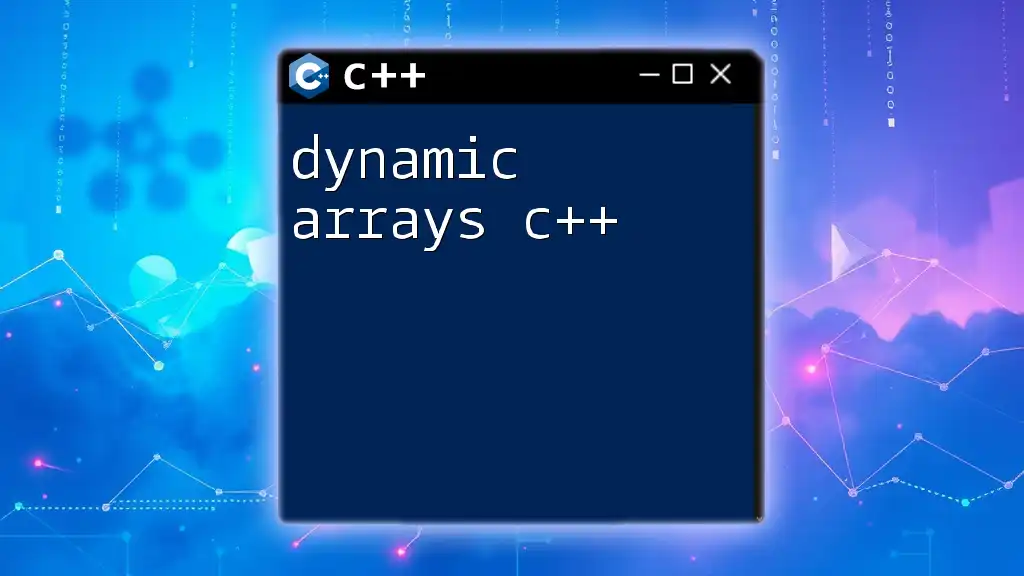
Creating a Simple Dynamic Link Library in C++
Setting Up Your Development Environment
When starting to work with a dynamic link library in C++, it is crucial to have an appropriate development environment. Visual Studio is recommended for its comprehensive features supporting project setup, compilation, and debugging.
Step-by-Step Guide to Creating a DLL
Creating the Project
To create a new C++ project for a DLL in Visual Studio, follow these steps:
- Open Visual Studio and select "Create a new project."
- Choose "Dynamic Link Library" as the project type and provide a name.
- Set your project properties according to your needs.
Writing Your First DLL
Below is a simple example of creating a DLL that includes basic mathematical operations.
- Creating the Header File (`SimpleMath.h`):
#ifdef SIMPLEMATH_EXPORTS
#define SIMPLEMATH_API __declspec(dllexport)
#else
#define SIMPLEMATH_API __declspec(dllimport)
#endif
extern "C" {
SIMPLEMATH_API int add(int a, int b);
SIMPLEMATH_API int subtract(int a, int b);
}
- Creating the Implementation File (`SimpleMath.cpp`):
#include "SimpleMath.h"
SIMPLEMATH_API int add(int a, int b) {
return a + b;
}
SIMPLEMATH_API int subtract(int a, int b) {
return a - b;
}
In this code, the functions are wrapped in an `extern "C"` block to prevent name mangling, making it easier for other applications to access them.
Compiling the DLL
After writing the code, the next step is to compile the DLL. This is done through the Build menu in Visual Studio, where you will select "Build Solution." If there are no errors, the output will be a `.dll` file located in the project’s output directory.
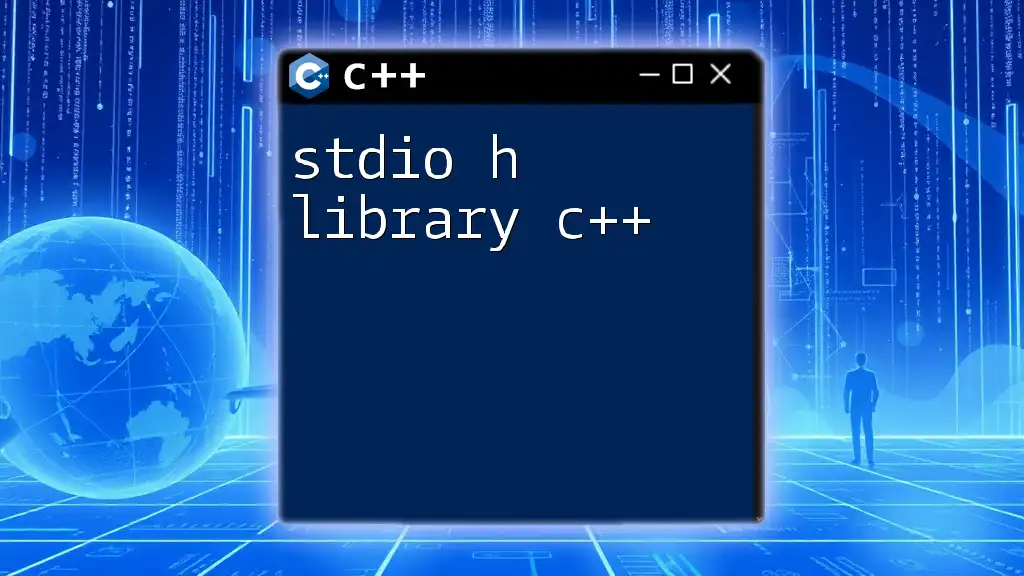
Using a Dynamic Link Library in C++
How to Link a DLL to Your Application
Linking a DLL to an application is straightforward. You need to ensure that your project settings include the directory where the DLL header files reside and link against the DLL when building the application.
Loading DLLs Dynamically at Runtime
To demonstrate how to use a DLL, consider the following example that employs the previously created `SimpleMath.dll`:
#include <windows.h>
#include <iostream>
#include "SimpleMath.h"
typedef int (*AddFunc)(int, int);
int main() {
HINSTANCE hDLL = LoadLibrary("SimpleMath.dll");
if (hDLL != NULL) {
AddFunc add = (AddFunc)GetProcAddress(hDLL, "add");
if (add != NULL) {
std::cout << "3 + 5 = " << add(3, 5) << std::endl;
}
FreeLibrary(hDLL);
}
return 0;
}
In this code, `LoadLibrary` loads the DLL at runtime, and `GetProcAddress` retrieves the address of the `add` function. Once invoked, it prints the result of adding two integers.
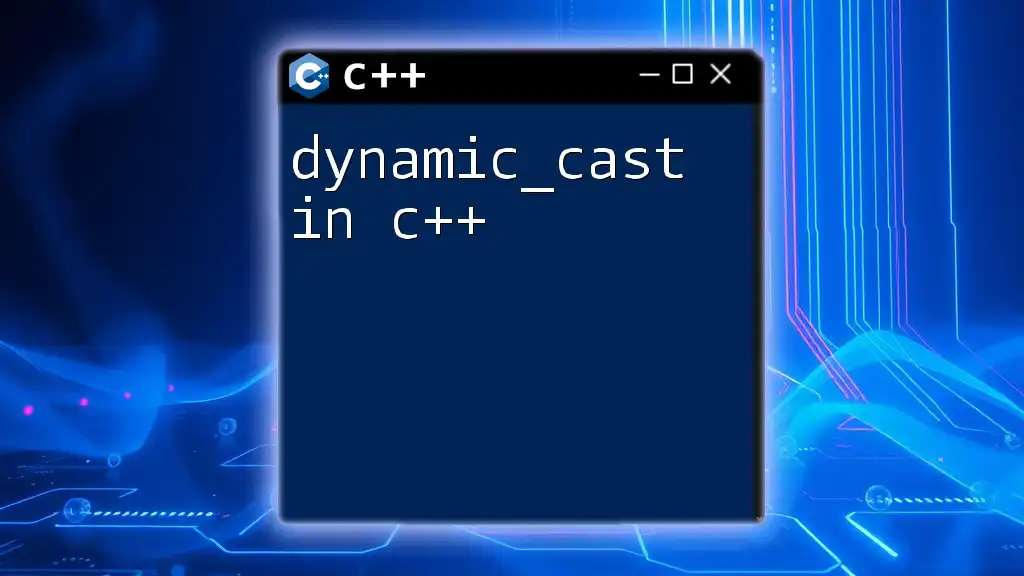
Debugging Issues with DLLs
Common Problems and Solutions
When working with dynamic link libraries in C++, you may encounter various issues:
- Unresolved External Symbols: This typically occurs if the function declarations are not correctly matched with those in the DLL. Double-check if you used the correct `dllexport` and `dllimport` keywords.
- Mismatched Calling Conventions: Ensure that the calling conventions are consistent between the DLL and the application.
- Path Issues: If the application cannot locate the DLL, verify that the DLL is in the application’s directory or included in the system PATH.
Debugging Techniques
Effective debugging techniques include:
- Logging entry and exit points for functions, along with parameters.
- Using breakpoints within the IDE to monitor function calls and data flow.
- Tools like Dependency Walker can help inspect DLL dependencies and ensure everything is linked correctly.
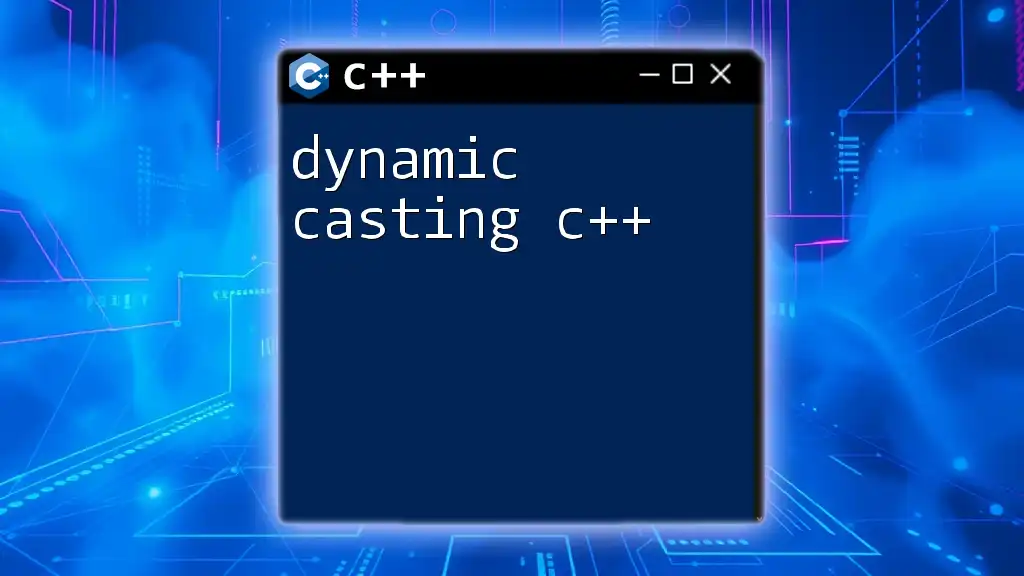
Best Practices for Developing DLLs in C++
Design Considerations
When developing DLLs, keep the following in mind:
- Managing Dependencies: Minimize the number of dependencies between DLLs to enhance modularity and ease version control.
- Versioning Your DLL: Implement a versioning system for your DLLs to facilitate easier updates and maintain compatibility with dependent applications.
Security Concerns
Protecting sensitive information is vital. Implement security measures to safeguard against vulnerabilities like DLL injection, which could allow malicious code execution.
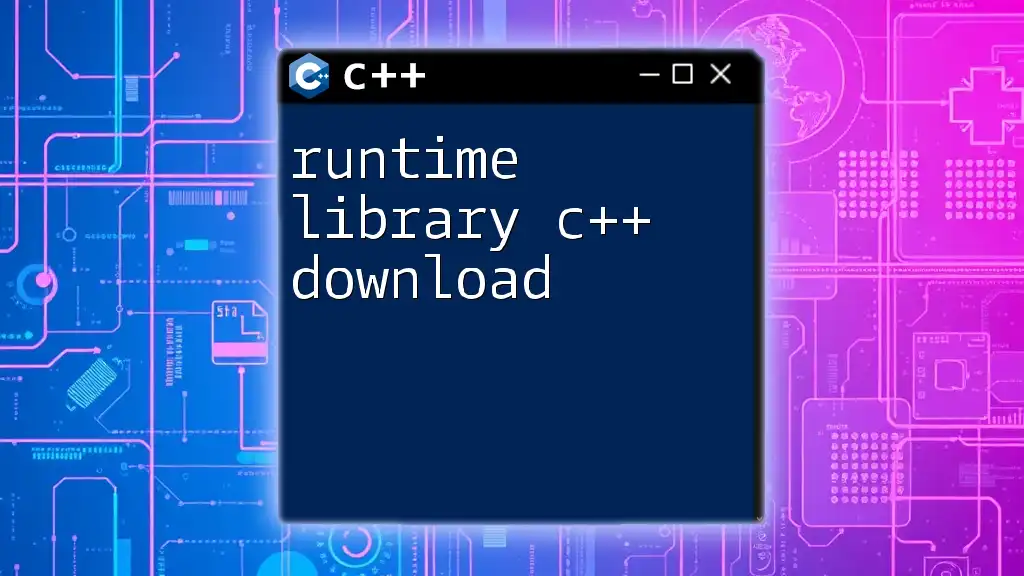
Conclusion
In summary, exploring dynamic link libraries in C++ opens the door to modular programming, enabling more efficient memory usage and facilitating code reuse across applications. As you delve deeper into this topic, consider advancing your skills with libraries, versioning, and safe coding practices to harness the power of DLLs fully.
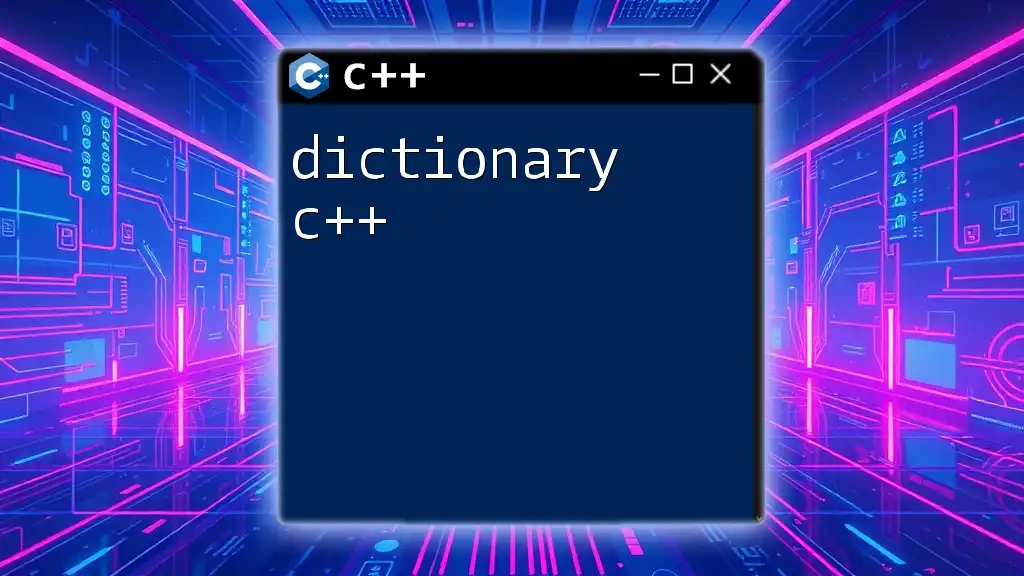
Additional Resources
For those looking to expand their knowledge further on dynamic link libraries, consider checking out dedicated books and online courses. Community forums can also be invaluable for troubleshooting and sharing insights with fellow developers.