Dynamic casting in C++ is a type of casting used primarily to safely convert pointers or references of base class types to derived class types at runtime, ensuring that the conversion is valid and avoiding potential runtime errors.
Here's a code snippet to illustrate dynamic casting in C++:
#include <iostream>
class Base {
public:
virtual ~Base() {} // Base class must have a virtual destructor
};
class Derived : public Base {
public:
void show() { std::cout << "Derived class" << std::endl; }
};
int main() {
Base* basePtr = new Derived(); // Base pointer pointing to Derived object
Derived* derivedPtr = dynamic_cast<Derived*>(basePtr); // Safe downcasting
if (derivedPtr) {
derivedPtr->show(); // Valid cast
}
delete basePtr; // Clean up memory
return 0;
}
What is Dynamic Casting?
Dynamic casting in C++ is a type of casting operation that safely converts pointers or references from a base class to a derived class. It is primarily used in scenarios that involve polymorphism, allowing for the adjustment of references or pointers to the correct type at runtime. This unique feature provides type safety and prevents undefined behavior.
Importance of Dynamic Casting in Object-Oriented Programming
Dynamic casting facilitates seamless interaction within hierarchies of classes, particularly when a program deals with multiple derived types. This capability proves essential in frameworks and systems that rely on polymorphism to function efficiently.
Differences Between Static Casting and Dynamic Casting
While `static_cast` performs compile-time checks and conversions, dynamic casting utilizes runtime checks to ensure that the conversion is valid, thus promoting safety. With dynamic_cast, if the cast is not possible, it returns a `nullptr`, allowing developers to handle such situations gracefully. In contrast, `static_cast` can lead to undefined behavior if used incorrectly.
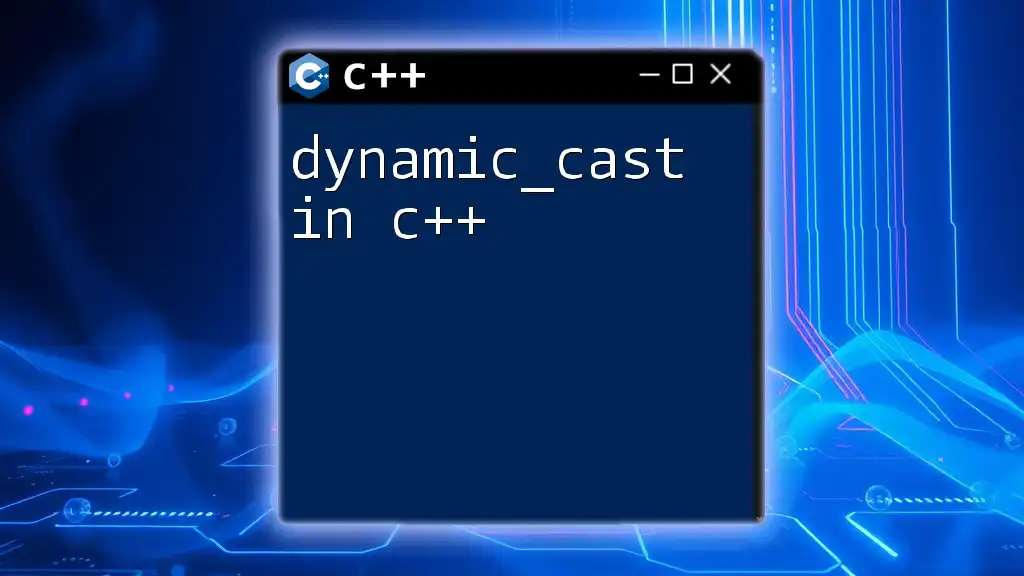
When to Use Dynamic Casting
Dynamic casting is particularly valuable in scenarios where you need to:
- Identify the exact type of an object in a polymorphic class hierarchy.
- Safely navigate complex class relationships reflecting an inheritance model.
- Implement dynamic behaviors where the type of an object determines how it should operate.
Scenarios Where Dynamic Casting is Beneficial
Consider a situation in a graphics application where various shapes inherit from a common base class. As you attempt to manipulate these shapes, dynamic casting can help determine the exact shape type that you are dealing with, enabling you to apply transformations specific to that shape.
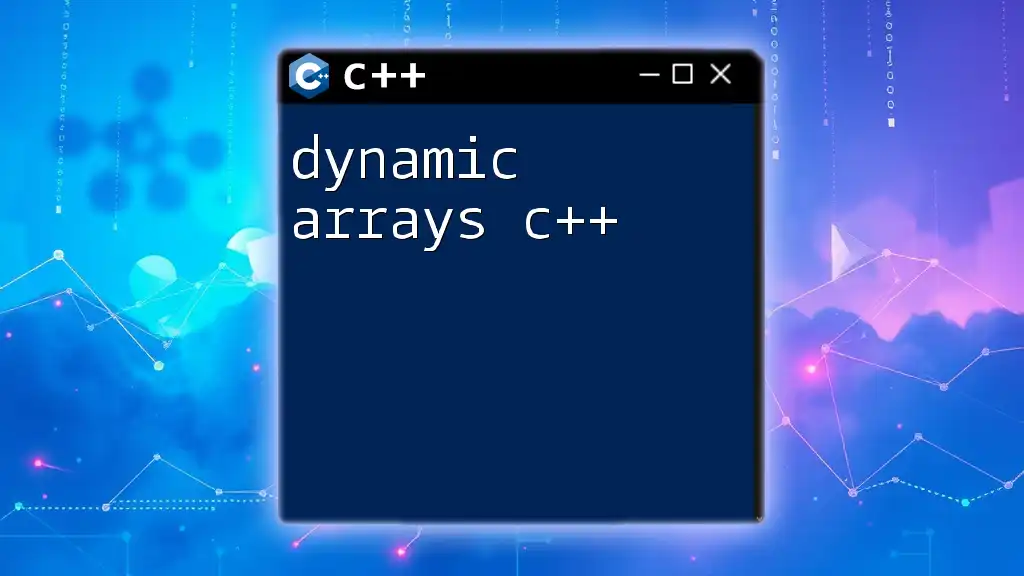
Types of Casting in C++
C++ offers various types of casting:
-
static_cast: Used for conversions that can be determined at compile time. This cast is faster but less safe, potentially leading to type errors at runtime.
-
reinterpret_cast: This cast provides low-level reinterpreting of types, allowing for converting any pointer type to another. It doesn't consider type safety and should be employed cautiously.
-
const_cast: Used to add or remove `const` qualifiers from a variable. It changes the constness but doesn't change the silicon-level representation of the object.
What Makes Dynamic Casting Unique
What sets dynamic_cast apart from the others is its reliance on runtime type checks, which makes it safer when working with polymorphic classes. This unique structural design of dynamic_cast ensures that incorrect casts do not lead to unexpected behavior, but are instead properly handled with a `nullptr` return. This can significantly reduce the chances of runtime errors caused by invalid casts.
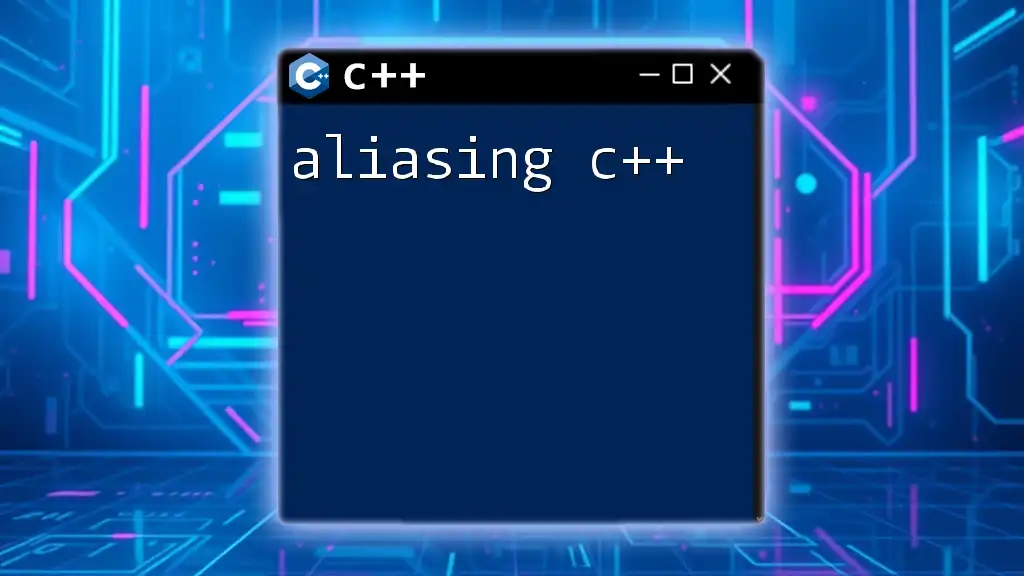
Basic Syntax Structure
The fundamental syntax for dynamic casting requires using the `dynamic_cast` operator along with a pointer or reference. The general syntax looks like this:
dynamic_cast<target_type>(expression)
Example of Its Usage in Simple Code
To illustrate, suppose we have a base class called `Base` and a derived class called `Derived`:
class Base {
public:
virtual ~Base() {}
};
class Derived : public Base {
public:
void derivedFunction() {}
};
// Example usage
Base* basePtr = new Derived();
Derived* derivedPtr = dynamic_cast<Derived*>(basePtr);
In the example above, `dynamic_cast` attempts to convert `basePtr` of the type `Base*` to `Derived*`. If `basePtr` indeed points to a `Derived` object, the cast is successful; otherwise, it returns `nullptr`.
Understanding the Result of Dynamic Casting
When using dynamic_cast, checking the return value is crucial. If the cast fails, the result will be `nullptr`, indicating that the conversion is invalid. Thus, a robust way to handle dynamic casting is as follows:
if (derivedPtr) {
derivedPtr->derivedFunction(); // Execute specific function if valid cast
} else {
// Handle failed casting appropriately
}
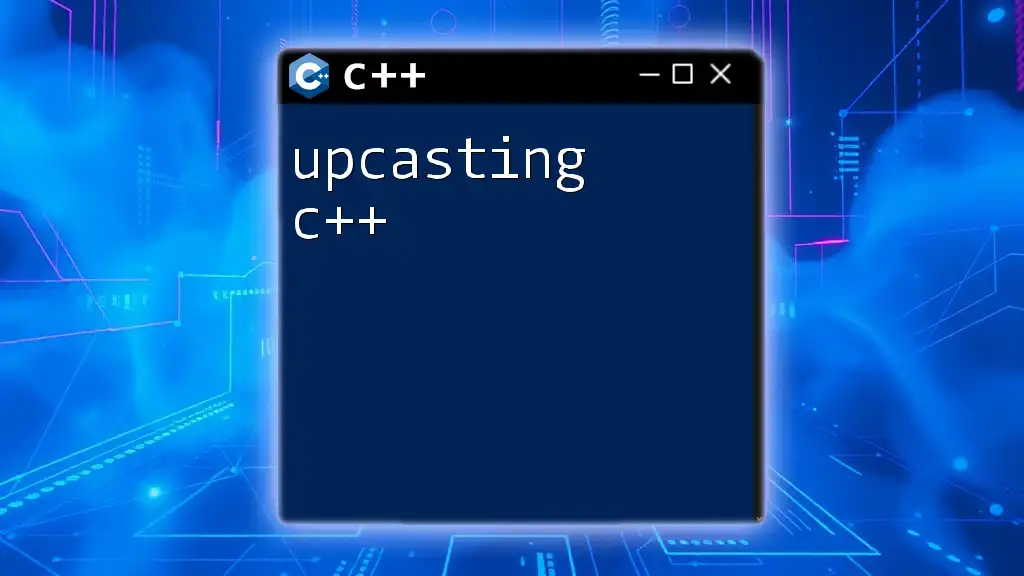
Using Dynamic Casting with Base and Derived Classes
To provide a more comprehensive understanding, let's implement a dynamic casting example incorporating both base `Base` and derived `Derived` classes.
class Base {
public:
virtual void display() { std::cout << "Base class\n"; }
virtual ~Base() {}
};
class Derived : public Base {
public:
void display() override { std::cout << "Derived class\n"; }
void derivedFunction() { std::cout << "Function specific to Derived class\n"; }
};
// Example usage
Base* basePtr = new Derived();
Derived* derivedPtr = dynamic_cast<Derived*>(basePtr);
if (derivedPtr) {
derivedPtr->derivedFunction(); // Executes successfully
} else {
std::cout << "Dynamic cast failed!\n";
}
delete basePtr; // Don't forget to free allocated memory
In this example, if `basePtr` points to a `Derived` instance, the `derivedFunction` will be executed successfully, demonstrating how dynamic casting efficiently identifies class types.
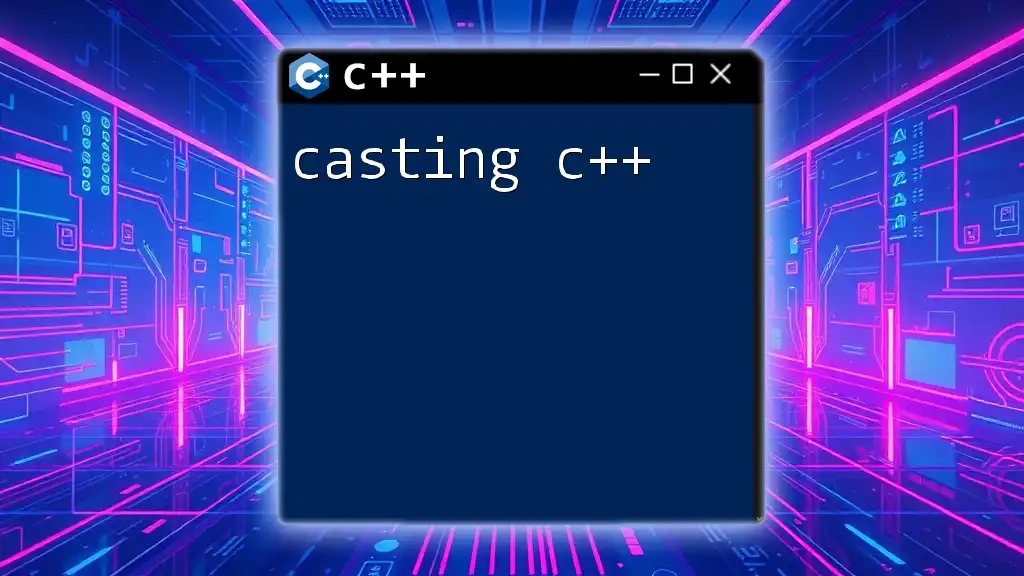
Real-life Examples of Dynamic Casting
Example 1: Game Development Scenario
In game development, an engine may consist of various entities like `Player`, `Enemy`, and `NPC` that inherit from a base class called `GameObject`. By using dynamic casting, developers can ensure that an event specific to player actions is triggered only when the entity is indeed a `Player`, allowing for smooth gameplay mechanics.
Example 2: GUI Frameworks
In a graphics user interface (GUI) framework, dynamic casting allows you to manage various types of widgets. For instance, if an event occurs on a UI element, you might need to cast the element to specific widget types like `Button`, `TextBox`, or `Label` for specialized event handling. This ensures that your event processing is type-safe and intuitive.
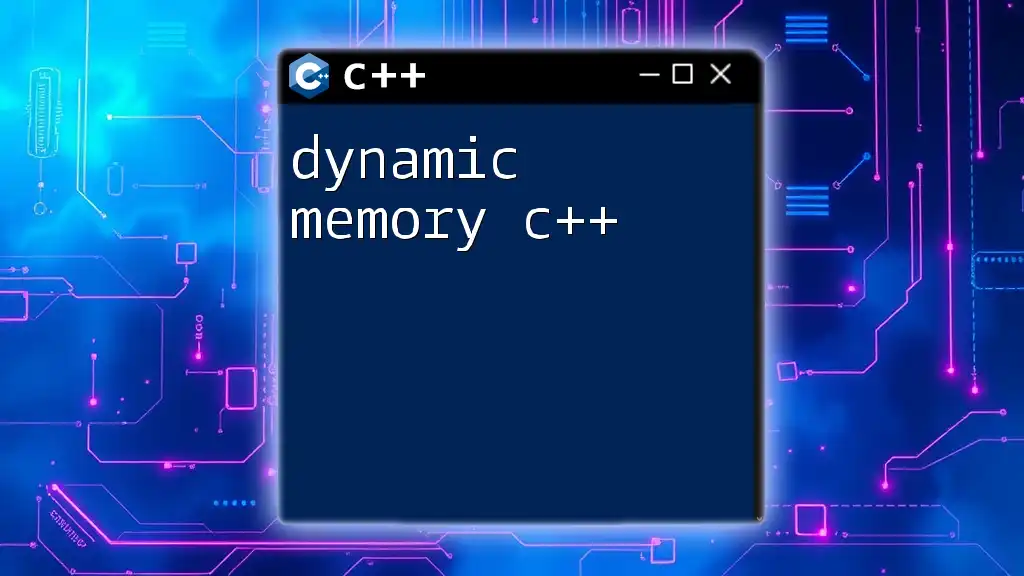
Common Mistakes with Dynamic Casting
Misunderstanding Polymorphism
One of the most common mistakes with dynamic casting is attempting to cast non-polymorphic types. Dynamic casting requires that the base class has at least one virtual function declared. If a base class does not declare any virtual functions, it will not participate in runtime type identification, leading to unintended behavior or crashes.
Handling nullptr Effectively
Always remember to check for a `nullptr` after dynamic casting. Failing to handle this can result in dereferencing a null pointer, which can cause your application to crash. Implementing smart error handling strategies is essential when working with dynamic_cast.
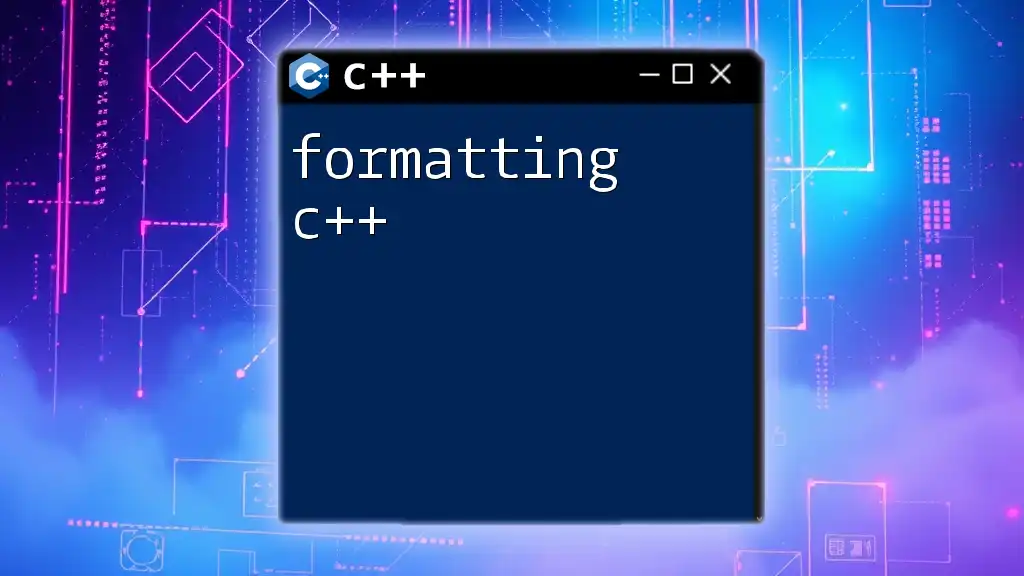
Performance Considerations
Impact of Using Dynamic Casting on Performance
One should be aware that dynamic casting incurs overhead due to the runtime checks performed during the cast. Although the performance impact is generally minimal for most applications, it can accumulate in performance-critical systems or when used excessively.
Alternatives to Dynamic Casting
In situations where dynamic casting introduces unacceptable overhead, consider alternatives like `static_cast` for simpler type conversions or utilizing templates where polymorphism can be achieved at compile time. These alternatives can reduce the runtime cost while maintaining type safety.
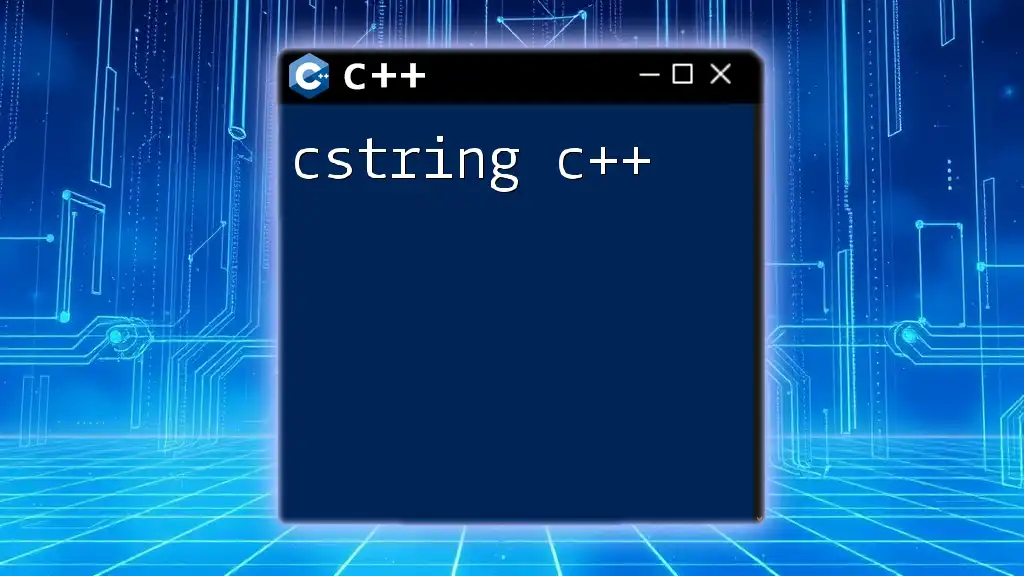
Conclusion
Dynamic casting in C++ is an invaluable tool within object-oriented programming, enabling safe and effective type conversion across class hierarchies. This capability is especially vital in dynamic and polymorphic scenarios, providing developers with a means to manage and manipulate complex relationships among objects with confidence.
By understanding the principles of dynamic casting and when to apply them, programmers can enhance the robustness of their applications while ensuring type safety and reducing runtime errors.
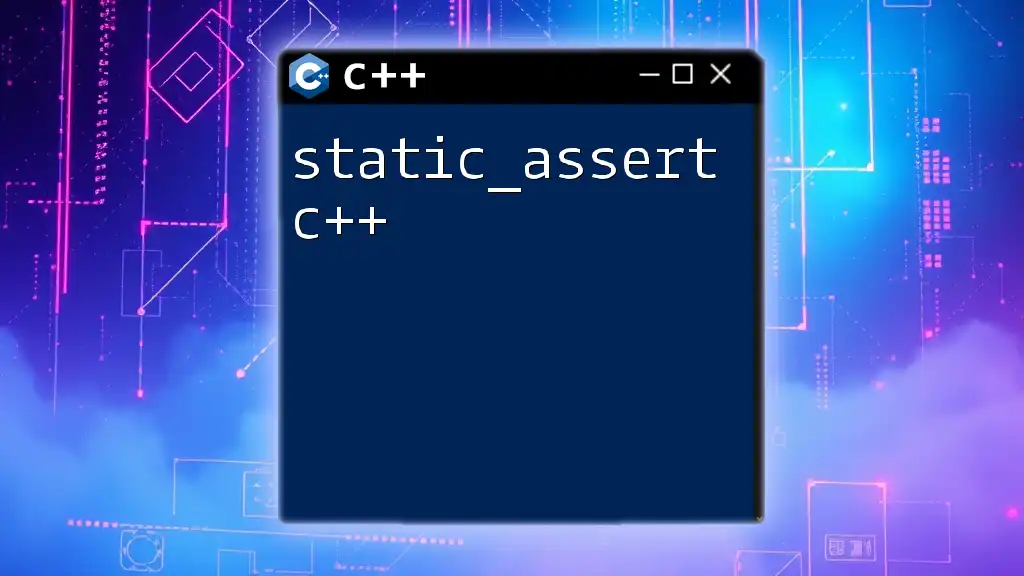
Additional Resources
For further learning about dynamic casting, consider exploring reputable books, online courses, or C++ documentation. Engaging in community forums and discussions can also provide valuable insights as you enhance your C++ programming skills.
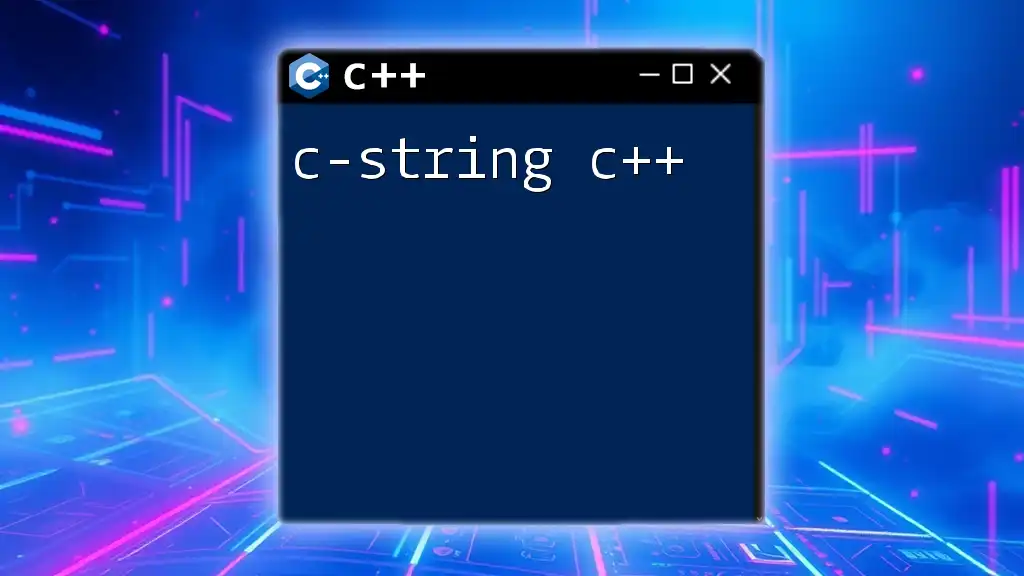
FAQs about Dynamic Casting in C++
What are some best practices when using dynamic_cast?
Best practices include checking the result of the cast for null pointers, ensuring your base class has virtual functions, and using dynamic casts only when necessary.
Can dynamic_cast be used with multiple inheritance?
Yes, dynamic_cast works with multiple inheritance but requires careful consideration of the virtual base classes to avoid ambiguity.
How do you handle failed dynamic_cast situations gracefully?
By always checking the result of dynamic_cast and implementing a fallback strategy, such as logging an error or raising an exception, developers can manage failed casts effectively.