The `<stdio.h>` library in C++ is used for input and output operations, providing functionality for reading from and writing to standard input and output streams.
#include <cstdio>
int main() {
printf("Hello, World!\n");
return 0;
}
Overview of the `stdio.h` Library
What is `stdio.h`?
The `stdio.h` library, short for Standard Input Output, is a fundamental part of C and C++ programming. It provides a set of functions and macros that facilitate input and output operations. Originally developed for the C programming language, this library has been extended and utilized in C++ to enable efficient data transfer between programs and users or other systems. The historical significance of `stdio.h` lies in its role in shaping how we interact with input and output in these languages, making it indispensable for both beginners and experienced programmers.
Key Features of `stdio.h`
`stdio.h` offers a range of I/O operations that are crucial for effective program communication. Some of its features include:
- Formatted Input and Output: This library allows you to read and write data in various formats using a standardized approach, which is essential for processing user input correctly.
- File Handling: It provides functions to manage files, enabling programmers to read from and write to disk storage effortlessly.
- Portability: Code utilizing `stdio.h` is highly portable across different platforms, making it easier to share and execute without modification.
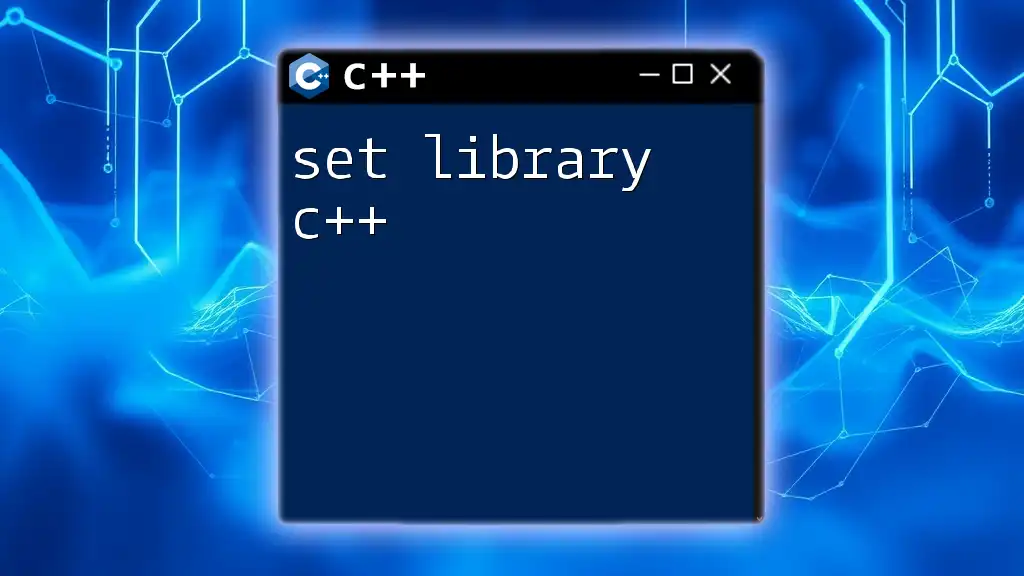
Core Functions of `stdio.h`
Standard Input Functions
One of the most commonly used functions in the `stdio.h` library is `scanf()`, which handles formatted input from standard input (usually the keyboard). It’s important to understand the syntax and usage of this function.
#include <stdio.h>
int main() {
int number;
printf("Enter a number: ");
scanf("%d", &number); // Reads an integer input from the user
printf("You entered: %d\n", number);
return 0;
}
In this example, `scanf()` takes a format specifier (`%d` for integers) along with the address of the variable to store the input. This function is crucial for gathering user input efficiently.
Standard Output Functions
Another vital function is `printf()`, which outputs formatted data to standard output (usually the console). Like `scanf()`, its syntax is straightforward and powerful.
#include <stdio.h>
int main() {
printf("Hello, World!\n"); // Outputs the message to the console
return 0;
}
In this case, `printf()` prints a simple message to the screen. The ability to format the output using specifiers (like `%d`, `%s`, etc.) makes it highly versatile for displaying various kinds of data elegantly.
File Handling Functions
The `stdio.h` library essentially simplifies file management in C++. Functions such as `fopen()` and `fclose()` are fundamental for opening and closing files during read/write operations.
#include <stdio.h>
int main() {
FILE *file;
file = fopen("example.txt", "r"); // Opens a file for reading
if (file == NULL) {
printf("Error opening file!\n");
return 1; // Returns an error code if file opening fails
}
fclose(file); // Closes the file
return 0;
}
In this snippet, `fopen()` attempts to open "example.txt" in read mode. If the file cannot be opened (e.g., it doesn't exist), an error message is displayed. Proper file closure with `fclose()` is critical to avoid resource leaks.
Additional I/O Functions
In addition to the essential input and output functions, `stdio.h` also provides capabilities for reading from and writing to files with functions like `fgets()` and `fputs()`.
#include <stdio.h>
int main() {
FILE *file;
char buffer[100];
file = fopen("example.txt", "r"); // Opens a file for reading
if (file) {
fgets(buffer, 100, file); // Reads a line from the file
printf("Read from file: %s\n", buffer); // Displays the read content
fclose(file);
}
return 0;
}
This example reads a line from "example.txt" and prints it to the console. The safety of operations is highlighted here, as careful checks ensure the file is opened before performing actions on it.
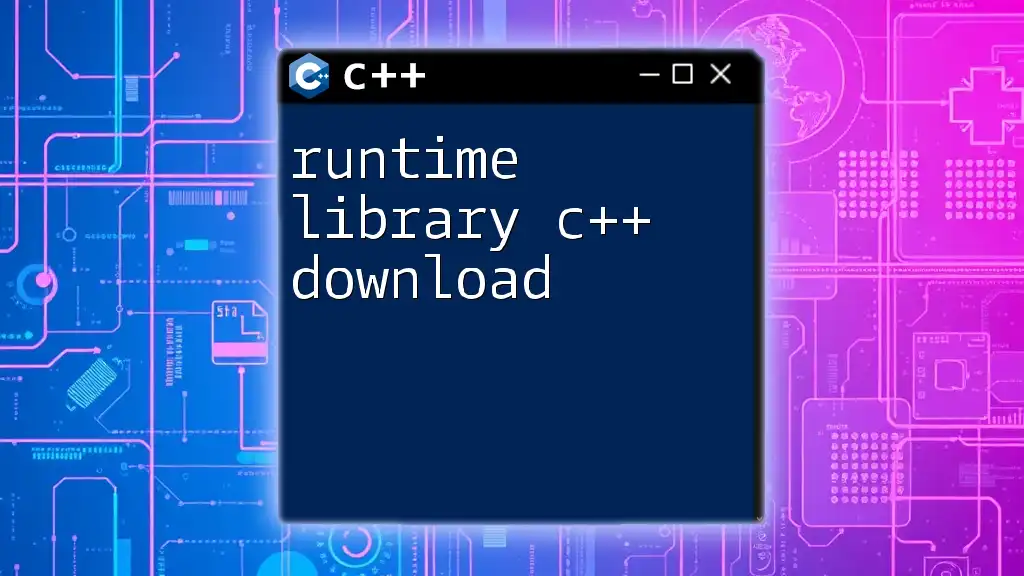
Error Handling in `stdio.h`
Understanding Error Codes
Effective error handling is critical when working with file and input/output operations. Functions in `stdio.h` often return specific error codes that programmers can check. It’s essential to understand these codes to handle potential issues gracefully.
Using `ferror()` and `perror()`
The `ferror()` function checks for errors in file operations, while `perror()` prints a human-readable string describing the last error encountered.
#include <stdio.h>
int main() {
FILE *file = fopen("nonexistent.txt", "r"); // Try opening a nonexistent file
if (!file) {
perror("Error opening file"); // Prints an error message
}
return 0;
}
In this case, if the file does not exist, `perror()` provides a clear message regarding the failure to open the file, making debugging much easier.
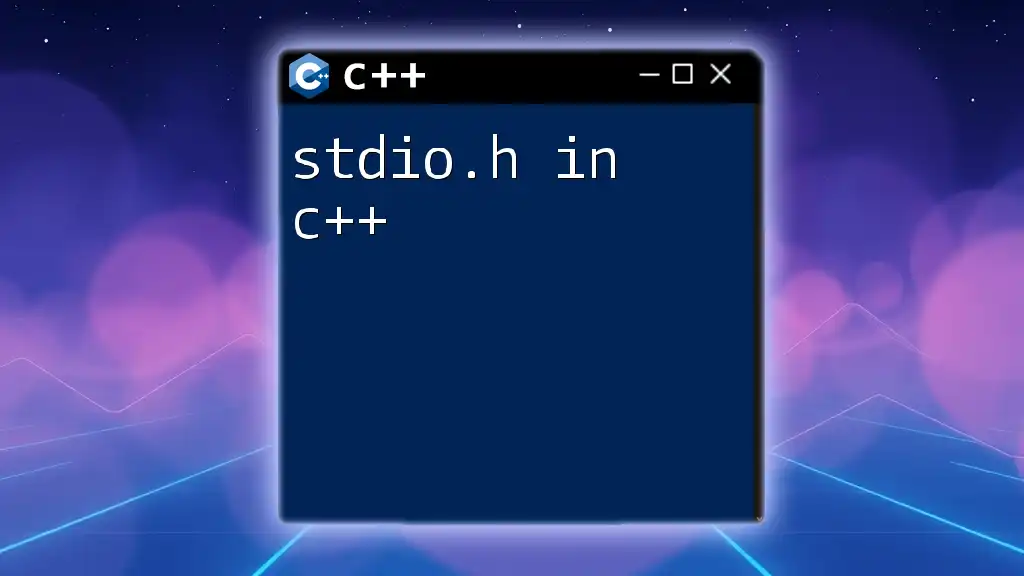
Best Practices When Using `stdio.h`
Memory Management
When working with file operations, it's crucial to allocate and deallocate resources effectively. Always ensure that every file opened with `fopen()` is closed with `fclose()` to prevent memory leaks and file corruption.
Code Readability and Maintainability
Maintainability of code is significantly enhanced by writing clear and organized snippets. Use meaningful variable names, add comments where necessary, and strive for code that tells a story. This practice becomes beneficial, especially when returning to code after extended periods or while collaborating with other developers.
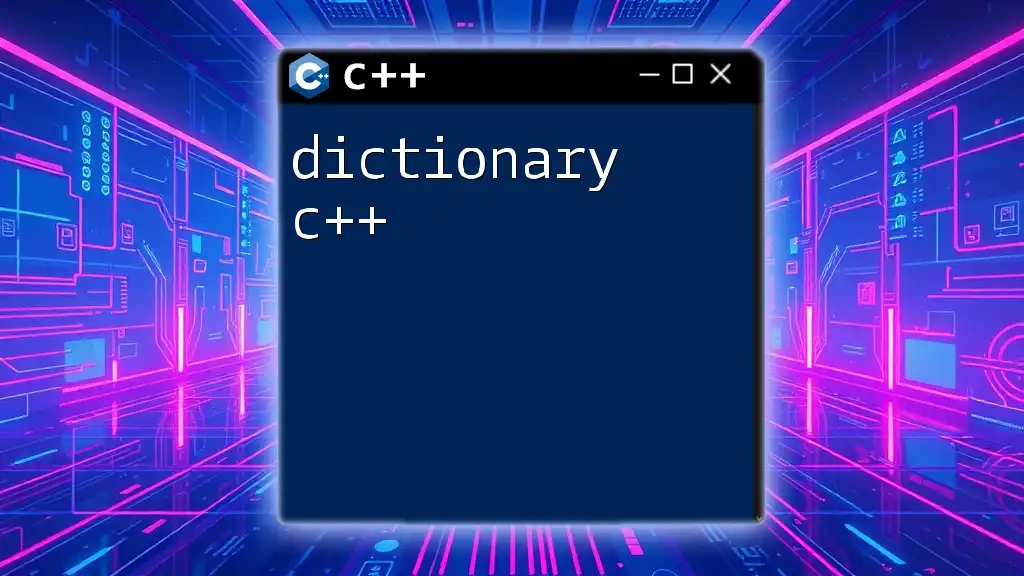
Alternatives to `stdio.h` in C++
While `stdio.h` is powerful, C++ also offers alternatives through the C++ I/O Streams with the `<iostream>` library. These streams provide features that align more closely with object-oriented concepts, such as method chaining and operator overloading, making them more intuitive for C++ developers.
Comparison between `stdio.h` and `iostream`
When comparing `stdio.h` with `iostream`, developers will notice differences in syntax and usage. The `<iostream>` library uses `cin` and `cout`, improving type safety and eliminating the need for format specifiers.
Advantages of using C++ I/O Streams
Using C++ I/O Streams allows for better type safety, easier debugging, and improved readability due to the use of overloadable operators, which many find more elegant than the traditional C-style functions.
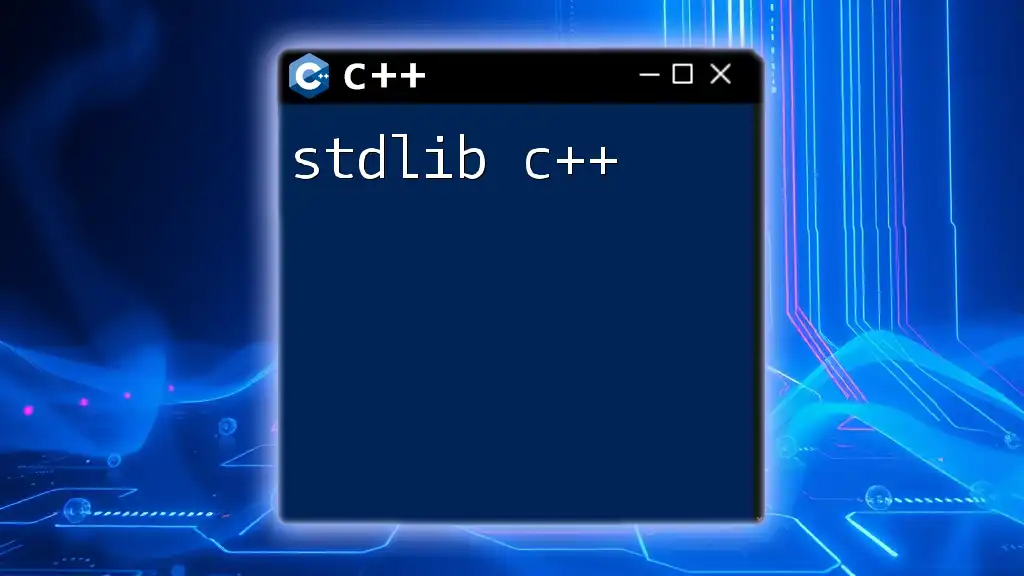
Conclusion
Recap of the Importance of `stdio.h`
In conclusion, the `stdio.h` library remains a cornerstone for handling input and output operations in C++. From formatted input and output to file handling, this library is versatile and widely used. Understanding its functions not only aids in developing efficient C++ code but also serves as a foundation for grasping more advanced concepts in programming.
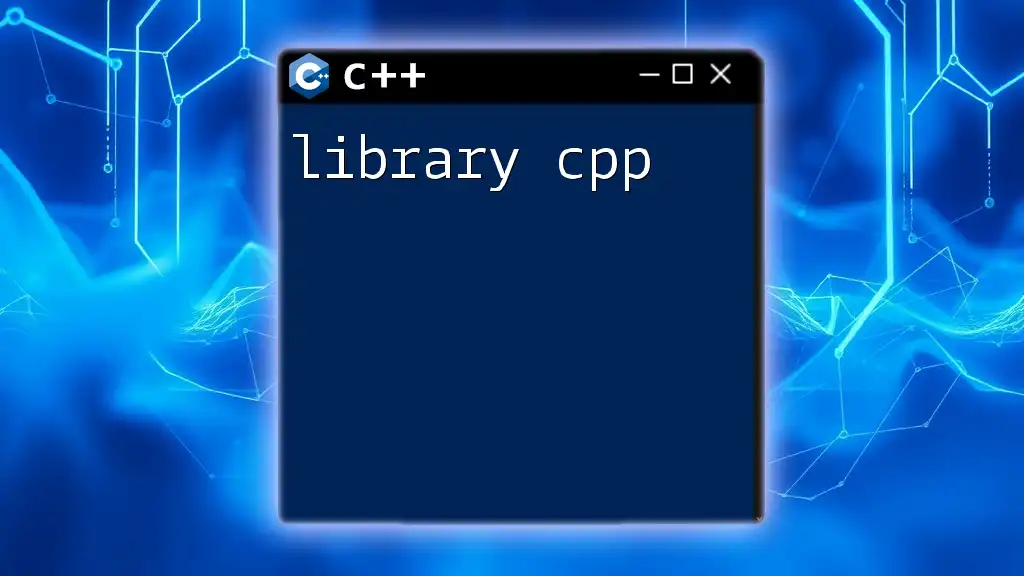
Additional Resources
To further enhance your understanding of the `stdio.h` library in C++, consider exploring additional resources, such as official documentation, coding tutorials, and textbooks that focus on C and C++ programming. Engaging with hands-on projects will also solidify your skills.
Call to Action
We encourage you to practice using `stdio.h` in various small projects. Experimenting with file handling and input/output will deepen your understanding and help sharpen your programming abilities. Share this article with peers who are interested in mastering C++ commands and libraries!