To download the C++ runtime library, you can typically install it through your package manager, but ensure to link the appropriate libraries in your project settings.
Here is an example command snippet to install the libstdc++ library on a Debian-based system:
sudo apt-get install libstdc++-dev
For Windows users, you can install it as part of the Visual Studio installation, or by downloading the appropriate packages from the Microsoft website.
Understanding C++ Runtime Libraries
What are C++ Runtime Libraries?
C++ runtime libraries are collections of pre-compiled code that help developers perform common tasks without needing to write everything from scratch. These libraries serve a vital role during the execution of C++ programs, allowing them to interact with various system resources efficiently.
The distinction between runtime and compilation is fundamental in C++. Compiled code is transformed into machine instructions before the program runs, while runtime libraries are actively utilized during program execution. There are two main types of C++ runtime libraries:
-
Static Libraries: These are linked into the program at compile-time, resulting in a single executable file. They increase the executable size but generally offer faster execution since they don't require external dependencies at runtime.
-
Dynamic Libraries: Also known as shared libraries, these are loaded during program execution. This allows multiple programs to share the same library code, significantly saving on memory usage and disk space.
Key Components of C++ Runtime Libraries
Several elements compose C++ runtime libraries, with the most common being:
-
Standard Template Library (STL): Offers a set of powerful data structures and algorithms, including vectors, lists, maps, and more.
-
Input/Output Libraries: Essential for handling data input and output operations, such as files and console.
-
System Libraries: Provide functions for system-level programming, including managing processes, memory, and more.
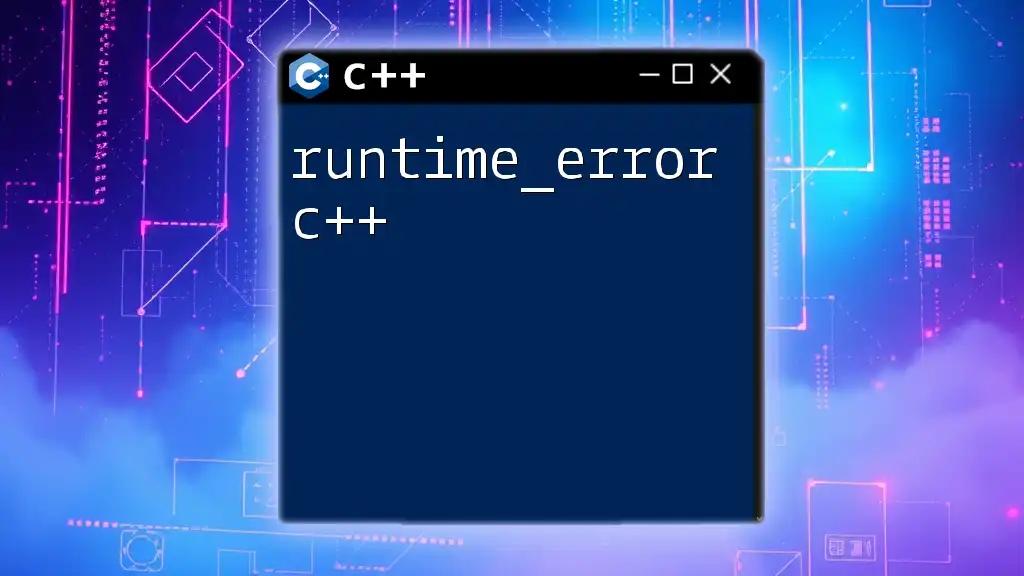
Finding the Right Runtime Library
Identifying Which Runtime Library You Need
Choosing the right runtime library often depends on the specific compiler you are using, as well as the requirements of your project. Various contexts necessitate different libraries. For instance, if you are developing games, you might prefer libraries optimized for performance, while scientific applications might leverage libraries that offer extensive mathematical functions.
Some popular C++ runtime libraries include:
-
Microsoft C++ Runtime Library: Widely used in Windows applications.
-
GNU C++ Standard Library: A standard library used for programs compiled with the GCC compiler.
-
Clang C++ Standard Library: Designed for use with the Clang compiler.
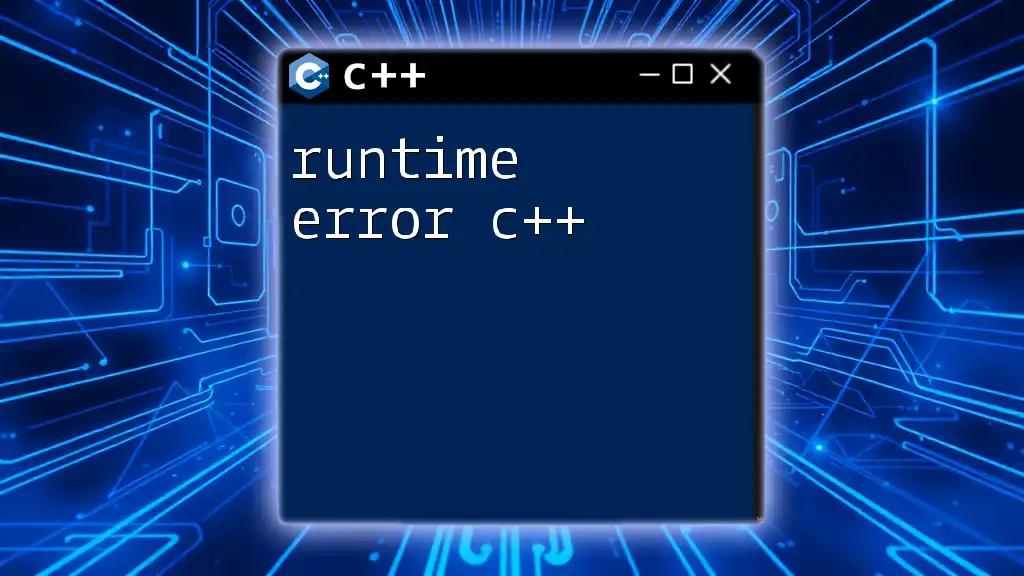
How to Download C++ Runtime Libraries
Official Sources for Runtime Library C++ Download
Accessing runtime libraries can be done from official sources directly linked to their respective compilers. Prominent sources include:
-
Microsoft Visual Studio: Use the Visual Studio Installer to install necessary runtime libraries.
-
GNU Compiler Collection (GCC): Available through various package management systems.
-
Clang: Downloadable from the official Clang website or through package managers.
Steps to Download and Install
Windows To download and install the Microsoft C++ Runtime Library, follow these quick steps:
- Open the Visual Studio Installer.
- Select “Modify” for your installed version of Visual Studio.
- Under the “Individual components” tab, locate the C++ runtime and select it.
- Complete the installation process.
Setting up the environment may require adjusting PATH variables in your system settings.
MacOS On MacOS, you can easily install the standard C++ libraries using Homebrew. Simply run the following command in your terminal:
brew install llvm
This command installs Clang and the associated standard library.
Linux For Linux users, installing the GNU C++ Libraries can typically be done with the command line. Depending on your distribution, you might use:
sudo apt-get install build-essential # For Debian/Ubuntu
sudo yum groupinstall 'Development Tools' # For Fedora/CentOS
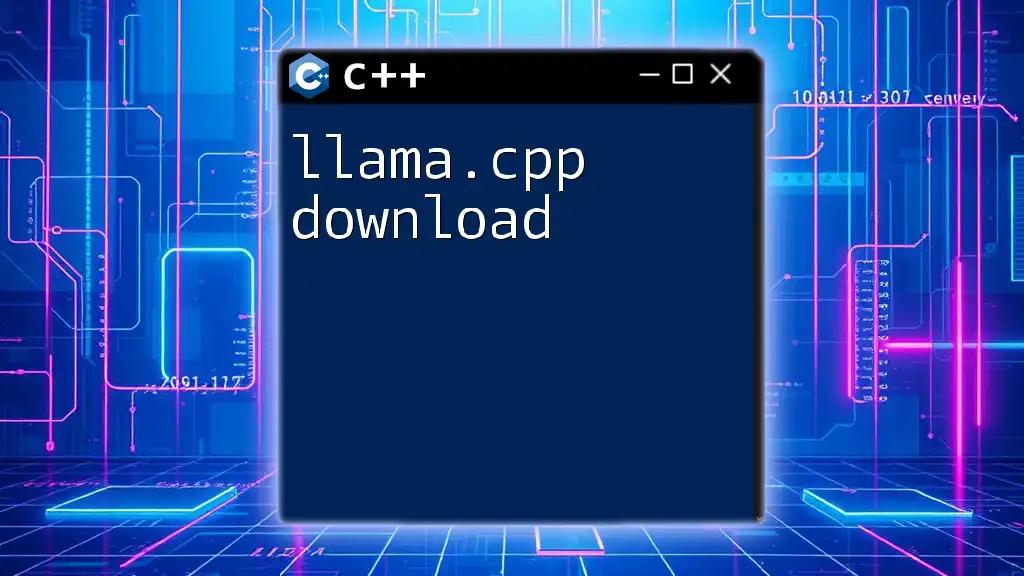
Installing and Configuring Runtime Libraries
Installation Process
Installation processes may vary depending on the operating system, but here are some general guidelines:
- Follow the specific steps provided by the documentation of your chosen compiler.
- If you encounter issues, ensuring that you have proper administrative permissions can be crucial.
Verifying Successful Installation
After following the installation instructions, it's important to verify that the library is installed correctly. You can check installed libraries using the command line. For example, you can list the installed packages on Linux with:
dpkg --get-selections | grep g++
Additionally, running a small test program can also confirm that everything is in place. Here is a simple test code:
#include <iostream>
int main() {
std::cout << "Runtime libraries installed successfully!" << std::endl;
return 0;
}
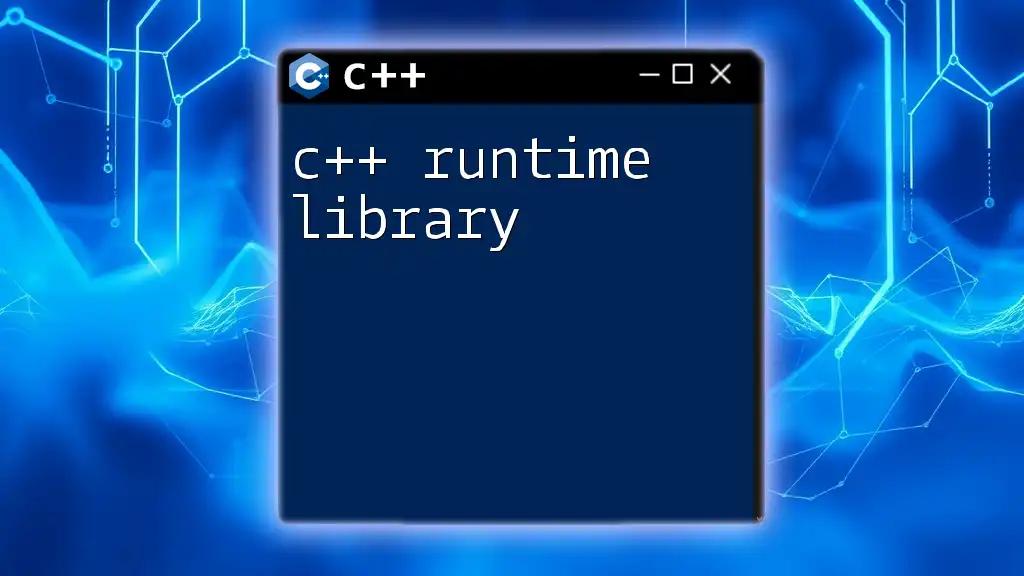
Examples of Using C++ Runtime Libraries
Basic Example of a C++ Program with Libraries
Here’s a straightforward example demonstrating the use of C++ Standard Library features such as vectors. This example also illustrates how to include libraries necessary for your program:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int number : numbers) {
std::cout << number << std::endl;
}
return 0;
}
In this snippet, the program includes `<vector>` to utilize the vector container effectively. The code iterates through the vector and prints each number to the console, showcasing how easily C++ libraries facilitate standard operations.
Advanced Example with Complex Data Structures
For more intricate operations, we can employ maps from the STL as shown below:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ageMap = {{"Alice", 30}, {"Bob", 25}};
for (const auto &pair : ageMap) {
std::cout << pair.first << " is " << pair.second << " years old." << std::endl;
}
return 0;
}
In this advanced example, we use a map to store names associated with ages. The code demonstrates the ease of storing and accessing data using C++ runtime libraries while also highlighting the library’s capability to minimize memory management concerns.

Common Issues and FAQs
Troubleshooting Common Issues
When working with runtime libraries, you may run into several common issues:
-
Dependency Errors: These often occur when the required library files are missing or not properly linked. Ensure you've downloaded all necessary components as prescribed by the documentation.
-
Runtime Errors: If a library is missing when a program is executed, it can lead to runtime errors. In such cases, you may need to check your installation and make sure all dependencies are satisfied.
Frequently Asked Questions
-
What to do if the library does not link properly? Ensure that your library paths are correctly set in the compiler/linker settings.
-
Best practices for managing multiple versions of libraries: Maintain clear documentation of the libraries you are using and their versions. Package managers can help keep them up to date.
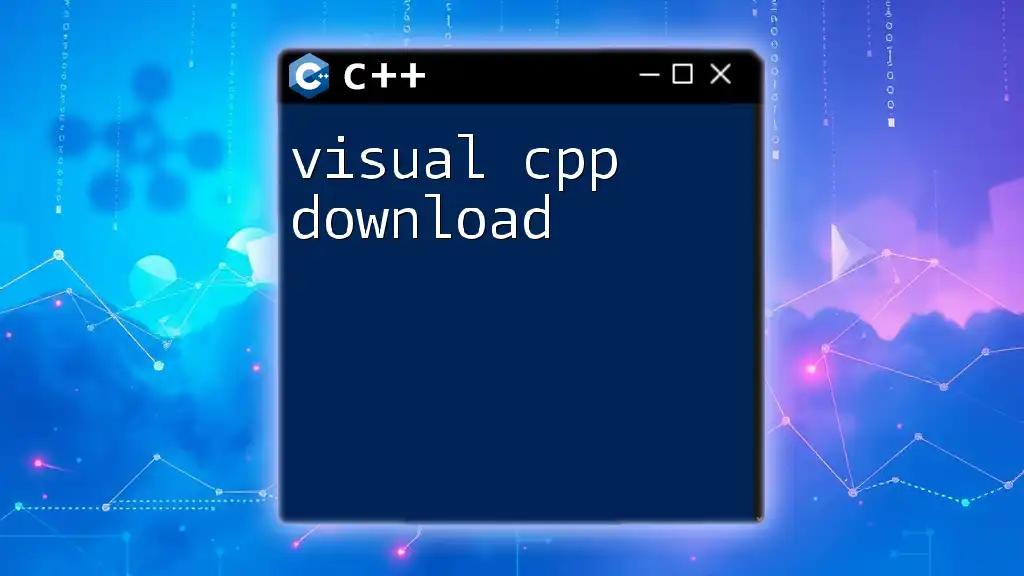
Conclusion
Understanding and using the correct runtime library C++ download is fundamental for developing efficient and reliable C++ applications. With the information provided, you should feel empowered to download, install, and utilize these libraries in your projects. Experimenting and practicing with different libraries will enhance your programming skills, pushing the boundaries of your C++ development capabilities.
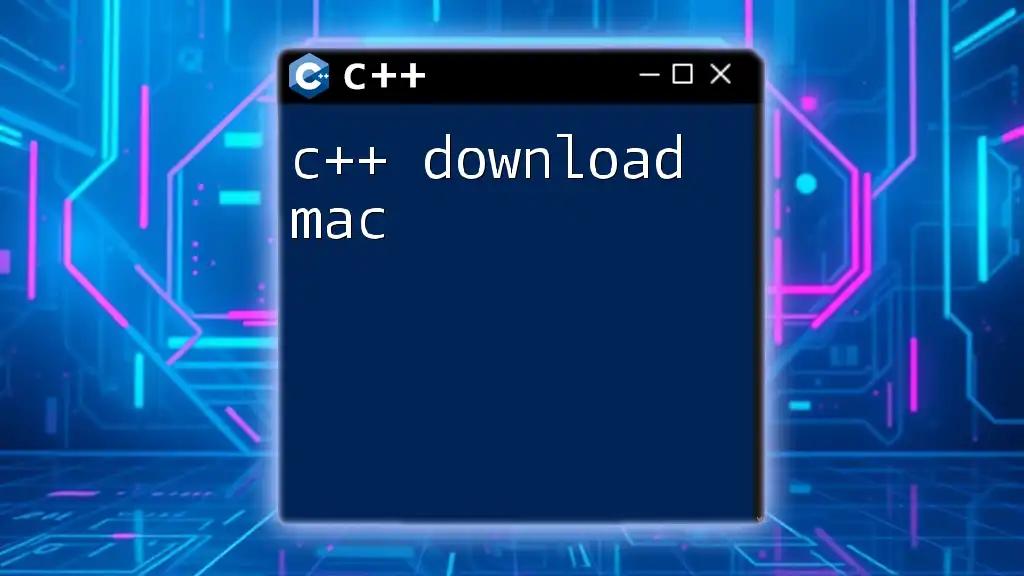
Call to Action
If you’re eager to further your knowledge and skills in C++, consider joining our course or community. We offer valuable resources and a platform for discussion where you can engage with fellow coders and enhance your learning experience.