In C++, a `map` is a versatile associative container that stores elements in key-value pairs, allowing for efficient retrieval based on unique keys.
Here’s a simple code snippet showcasing how to declare and use a `map` in C++:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
ageMap["Bob"] = 25;
for (const auto& pair : ageMap) {
std::cout << pair.first << ": " << pair.second << " years old" << std::endl;
}
return 0;
}
Understanding C++ Map Structure
A C++ map is a collection of key-value pairs where each key is unique and is used to access its corresponding value. This makes maps a powerful tool for storing and retrieving data based on specific identifiers.
C++ Map Declaration
To declare a map in C++, you use the `std::map` container from the Standard Template Library (STL). The general syntax for declaring a map is:
std::map<KeyType, ValueType> mapName;
For example, to create a map that associates names (of type `std::string`) with ages (of type `int`), you can write:
std::map<std::string, int> ageMap;
Associative Containers
Maps are part of a category known as associative containers, which allow for the rapid retrieval of information via keys. Unlike arrays, which are indexed by integers, maps link keys to values, providing a more flexible organization of data.
Creating a C++ Map
Basic Map Creation
To create a basic C++ map, first ensure you include the necessary header file:
#include <map>
#include <string>
Next, initialize the map as shown earlier. After initialization, the map is empty and ready for data insertion.
Inserting Elements into a Map
The primary methods to insert elements into a map are the `insert()` method and the `[]` operator.
-
Using the `insert()` method:
ageMap.insert(std::make_pair("Alice", 30)); // Adding "Alice" with age 30
-
Using the `[]` operator:
ageMap["Bob"] = 25; // Adding "Bob" with age 25
Both methods are essential for populating the map with data.
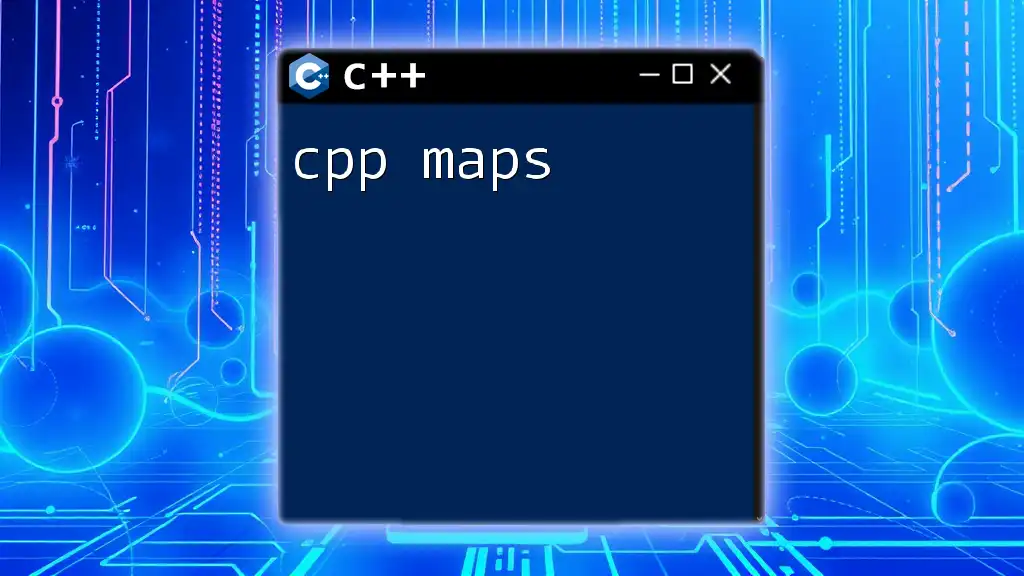
Accessing Elements in a Map
Once data is populated, accessing elements in a map can be performed in several ways.
Retrieving Values
You can retrieve values using the `[]` operator or the `at()` method. Both are concise and effective.
std::cout << "Alice's age: " << ageMap["Alice"] << std::endl; // Outputs: 30
std::cout << "Bob's age: " << ageMap.at("Bob") << std::endl; // Outputs: 25
Iterating Through a Map
To iterate through a C++ map and retrieve keys and their corresponding values, you can use a range-based for loop or iterators.
- Using Range-Based For Loop:
for (const auto& pair : ageMap) {
std::cout << pair.first << ": " << pair.second << std::endl; // Outputs key-value pairs
}
This loop is straightforward and enhances readability.
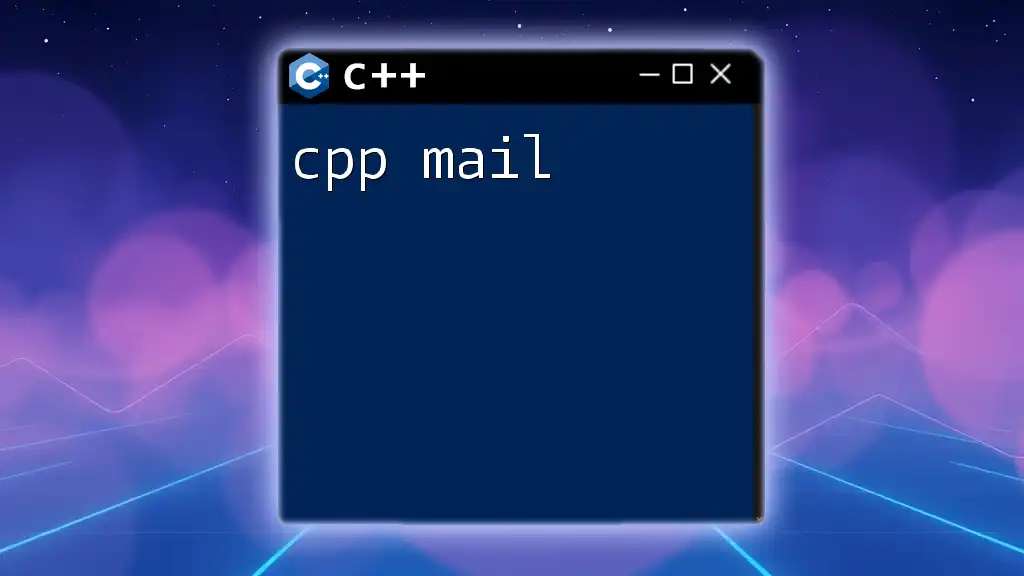
Modifying a C++ Map
Updating Values
Updating a value in a map is simple. You can directly access the element using its key and assign a new value:
ageMap["Alice"] = 31; // Updating Alice's age
Removing Elements
To remove elements from a map, use the `erase()` method. You can remove an element by specifying its key.
ageMap.erase("Bob"); // This removes Bob from the map
You can also clear the entire map using `ageMap.clear();`, which resets the map to its initial empty state.
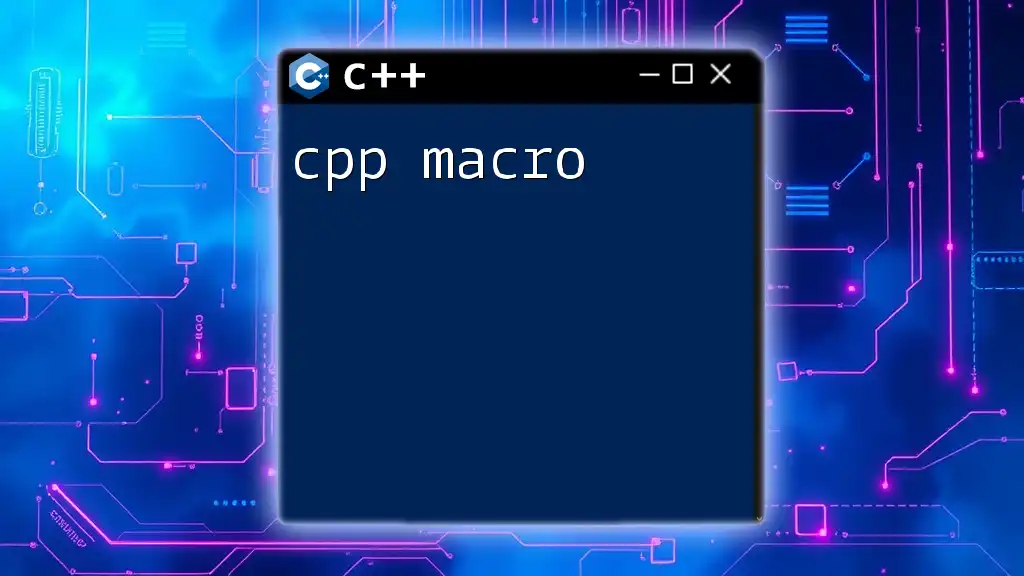
Advanced Features of C++ Maps
Sorting a Map
A C++ map automatically sorts its elements by keys in ascending order. However, if you need to sort by values, you'll need to use a custom comparator. This can be done with a combination of vectors and pairs.
#include <vector>
#include <algorithm>
std::vector<std::pair<std::string, int>> sortedMap(ageMap.begin(), ageMap.end());
// Custom sort based on value
std::sort(sortedMap.begin(), sortedMap.end(), [](const auto& a, const auto& b) {
return a.second < b.second; // Sorting by age
});
Using MultiMaps
Sometimes, it may be necessary to store multiple values for a single key, in which case the `std::multimap` is useful. A multimap allows duplicate keys.
std::multimap<std::string, int> scores;
scores.insert(std::make_pair("Alice", 90));
scores.insert(std::make_pair("Alice", 85)); // Multiple scores for Alice
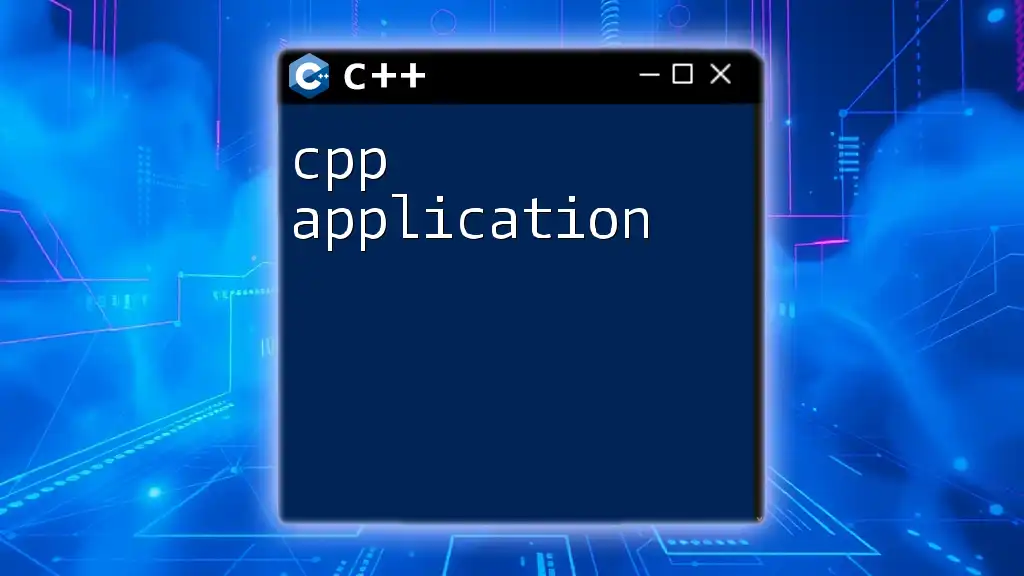
Performance Considerations
Time Complexity of Map Operations
Understanding the performance of map operations is crucial for optimizing your code. Most operations such as insertion, deletion, and access in a map have a logarithmic time complexity of O(log n). This efficiency originates from the underlying red-black tree implementation used by the `std::map`.
Memory Overhead of Maps
While maps are efficient, they do consume more memory compared to other linear data structures. If your data access is predictable and uniform, consider using arrays or vectors to conserve memory resources. You should evaluate whether a C++ map's functionalities justify the trade-off in memory usage.
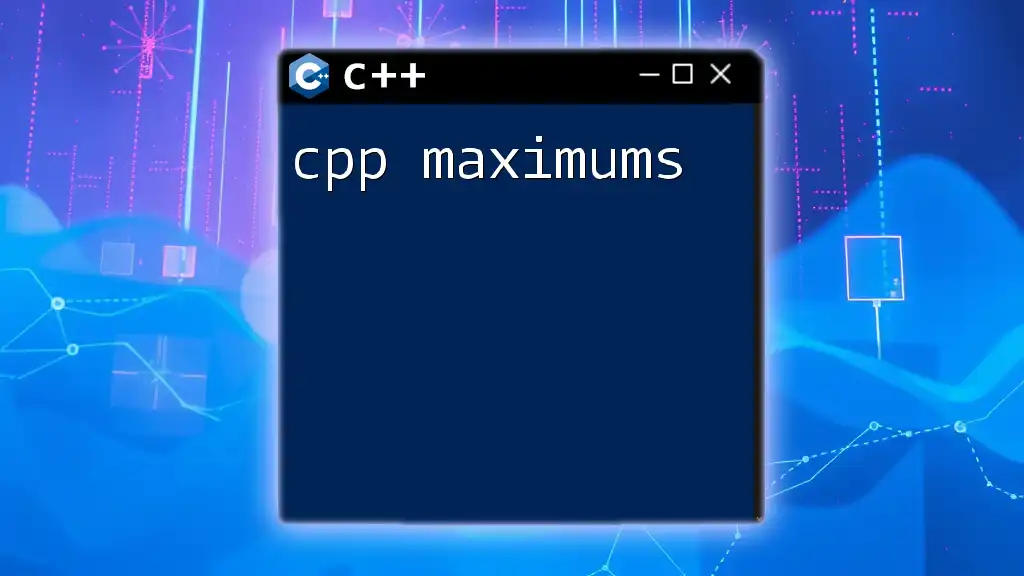
Common Pitfalls and Tips
Common Mistakes with C++ Maps
One common mistake involves misusing the `[]` operator. Unlike `at()`, using `[]` will automatically create a default entry if the key does not exist. This can lead to unexpected behavior:
std::cout << ageMap["Charlie"]; // This will create an entry for "Charlie" with age 0
Always ensure that the key exists before using `[]`. The `at()` method prevents this issue since it throws an exception.
Best Practices for Using Maps
Best practices for using maps include:
- Keep key types uniform to ensure consistency.
- Regularly evaluate whether the map's benefits compensate for its overhead and complexity.
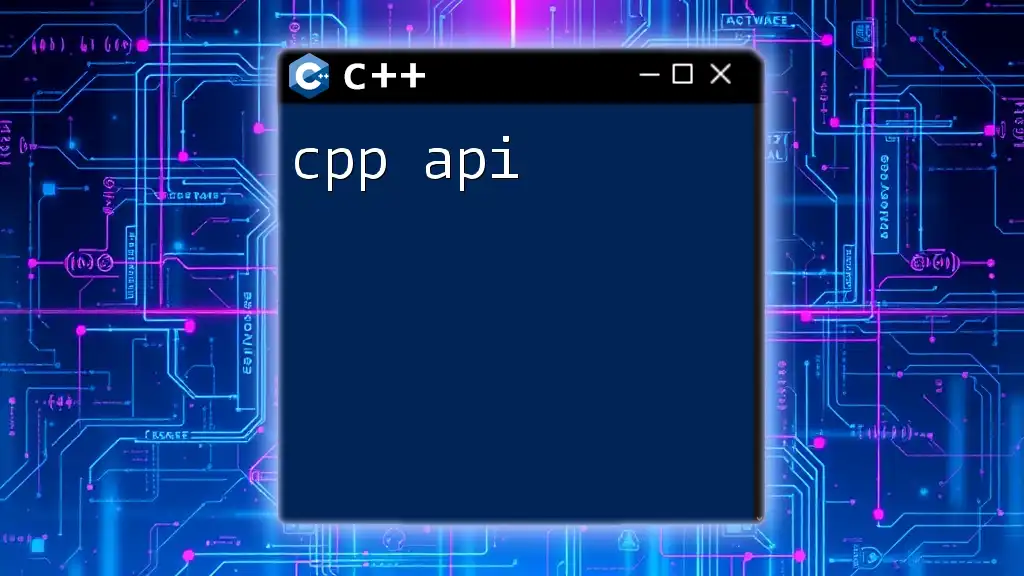
Conclusion
C++ maps are an indispensable tool for developers looking to efficiently store key-value pairs and access them with ease. By understanding their structure, advanced features, and potential pitfalls, you can leverage maps to enhance the performance and readability of your code. Whether you're working on small-scale applications or large projects, the C++ map provides a reliable solution for managing your data effectively.
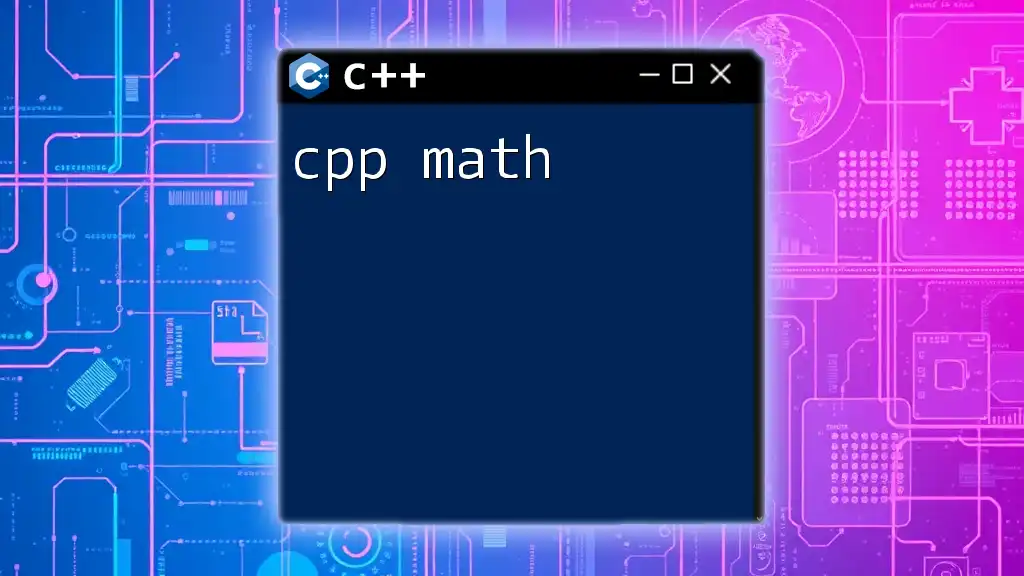
Additional Resources
For further exploration into C++ maps and related concepts, consider reviewing the following:
- Recommended books on C++ STL and data structures.
- Online courses focusing on C++ programming and best practices.
- Official C++ documentation for in-depth technical insights into standard libraries and containers.