The "cpp maximums" refers to the use of the `std::max` function in C++ to determine the maximum value between two or more given numbers.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <algorithm> // for std::max
int main() {
int a = 10;
int b = 20;
int max_value = std::max(a, b);
std::cout << "The maximum value is: " << max_value << std::endl;
return 0;
}
Understanding Maximum Values in C++
Maximum values are fundamental concepts in programming that refer to the highest value within a set of numbers. In C++, understanding cpp maximums is crucial, not only for manipulating data but also for optimizing algorithms, ensuring efficiency in code execution, and making informed decisions based on data analysis.
Maximums play a pivotal role in various situations, such as determining the best result from a dataset, enhancing search algorithms, or comparing performance metrics. For example, when analyzing a student’s grades, finding the maximum score can help determine academic success.
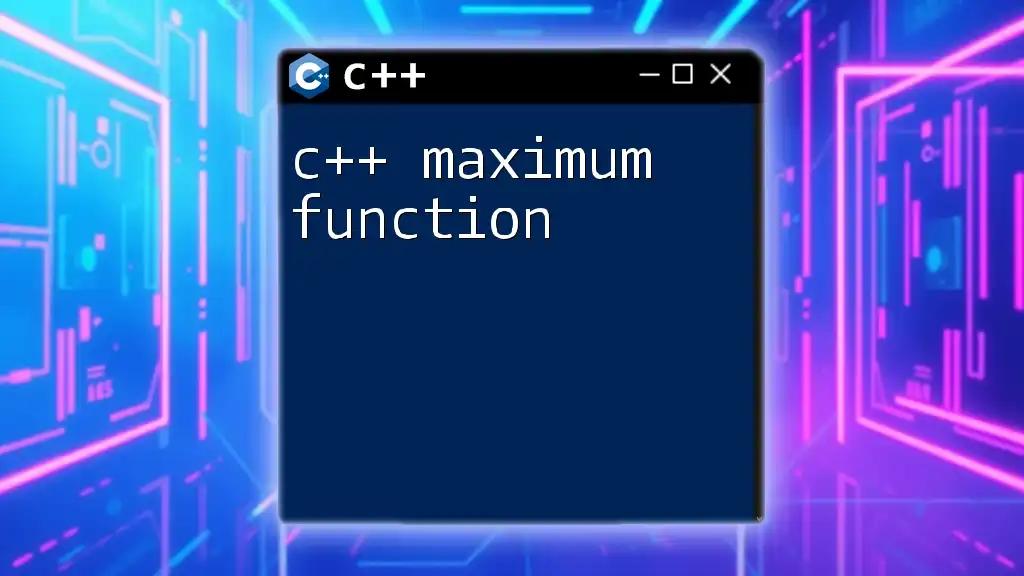
Getting Started with C++ Maximums
Basic Syntax and Functions
To work with maximums in C++, the standard library provides the `std::max` function, which simplifies the process of comparing two values. This function can be used to find the larger of two provided numbers.
For example, the following code demonstrates how to use `std::max`:
#include <iostream>
#include <algorithm>
int main() {
int a = 10, b = 20;
int max_value = std::max(a, b);
std::cout << "The maximum value is: " << max_value << std::endl;
return 0;
}
In this code, we declare two integers, `a` and `b`, and use `std::max` to find the larger value between them. The result is then printed to the console. Understanding this basic operation is fundamental in working with cpp maximums.
Example: Using std::max in Practice
In practice, the `std::max` function can be beneficial in various algorithms, especially within conditional statements. You can easily integrate it into loops and decision-making structures to efficiently determine maximum values.
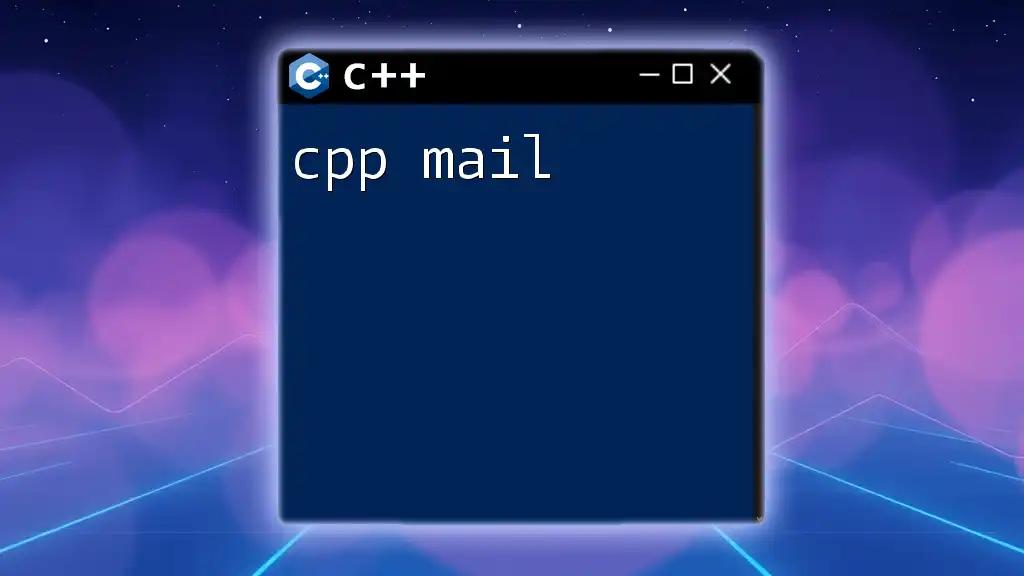
Working with Arrays and Vectors
Finding Maximum in Arrays
When it comes to working with arrays, finding the maximum value requires some iterative approach. Below is an example illustrating how to find the maximum in an array.
#include <iostream>
int findMaxInArray(int arr[], int size) {
int max_val = arr[0];
for (int i = 1; i < size; i++) {
max_val = std::max(max_val, arr[i]);
}
return max_val;
}
int main() {
int arr[] = {3, 5, 7, 2, 8};
std::cout << "Max value in array: " << findMaxInArray(arr, 5) << std::endl;
return 0;
}
In this code snippet, we start by assuming that the first element in the array is the maximum. As we loop through each element of the array, we compare it with the current maximum using `std::max`. If an element is larger, it replaces the current maximum value. At the end of the loop, we have the maximum value of the array displayed.
Finding Maximum in Vectors
Vectors in C++ are dynamic arrays that have certain advantages, such as automatic memory management and flexibility in size. To find the maximum value in a vector, you can utilize the `std::max_element` function, which scans through the vector and returns an iterator to the maximum element.
Here is an example:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 4, 6, 2, 9};
int max_value = *std::max_element(numbers.begin(), numbers.end());
std::cout << "Maximum value in vector: " << max_value << std::endl;
return 0;
}
In this code, we use `std::max_element` to find the maximum value within the specified vector range, effectively allowing us to retrieve the maximum without manually iterating through each element.
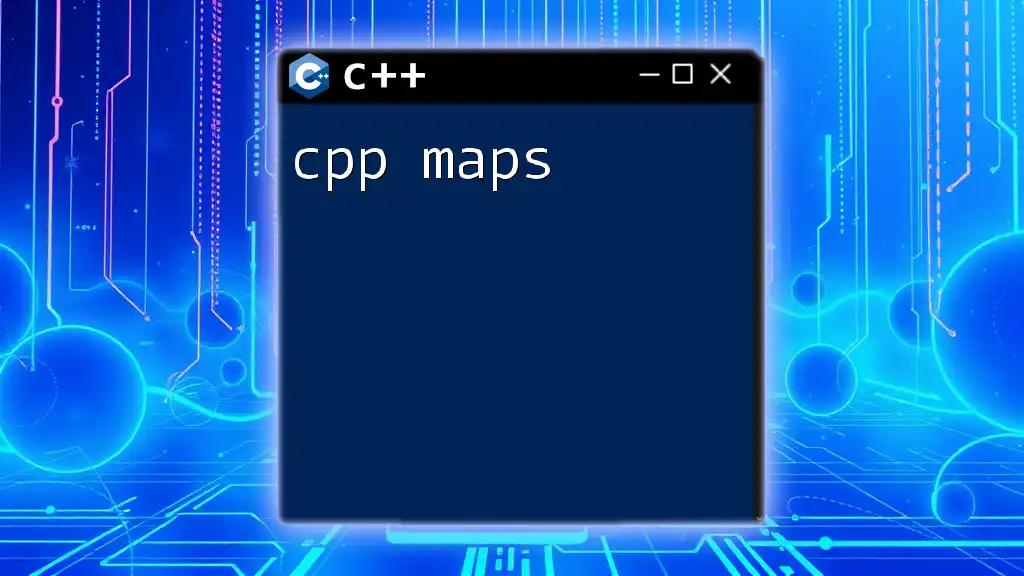
Advanced Techniques for Finding Maximums
Finding Maximum with Custom Comparison
In scenarios where data structures are more complex than simple integers, or where custom criteria is needed, we can employ function pointers or lambda functions for comparison. This allows for more flexibility, especially when working with structs or classes.
The following example demonstrates how to find the maximum using a custom comparison function based on age:
#include <iostream>
#include <vector>
#include <algorithm>
struct Person {
std::string name;
int age;
};
bool compareAge(const Person &a, const Person &b) {
return a.age < b.age;
}
int main() {
std::vector<Person> people = {{"John", 25}, {"Jane", 30}, {"Mark", 20}};
auto oldest = std::max_element(people.begin(), people.end(), compareAge);
std::cout << "Oldest person: " << oldest->name << " age " << oldest->age << std::endl;
return 0;
}
Here, the `compareAge` function determines who has the higher age. The `std::max_element` then utilizes this comparison to identify the oldest person in the vector.
Using Recursive Functions to Find Maximum
Recursion can also be employed when looking for a maximum. Although not always the most efficient method, recursion can simplify the logic in certain cases. Here’s how you can implement a recursive function to find the maximum value:
#include <iostream>
#include <vector>
int findMaxRecursive(const std::vector<int>& numbers, int index) {
if (index == 0) return numbers[0];
return std::max(numbers[index], findMaxRecursive(numbers, index - 1));
}
int main() {
std::vector<int> numbers = {10, 20, 30, 5, 15};
std::cout << "Maximum using recursion: " << findMaxRecursive(numbers, numbers.size() - 1) << std::endl;
return 0;
}
In this function, we recursively compare each number with the maximum found so far until we reach the base case.
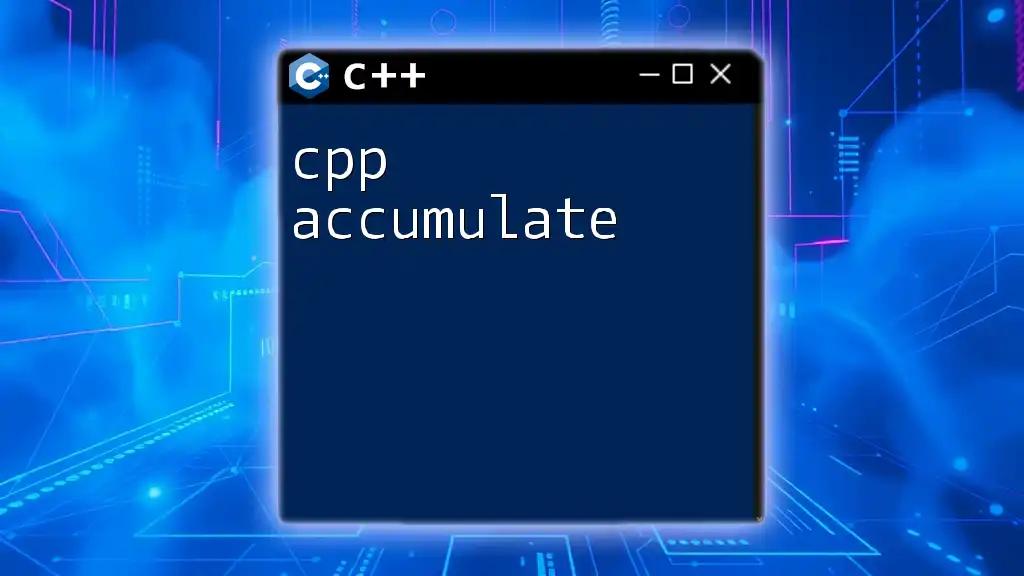
Best Practices When Working with C++ Maximums
Performance Considerations
When considering performance, the time complexity of finding maximums becomes essential. Both iterative and recursive approaches have their advantages, but iterative solutions often perform better in terms of speed and resource management. However, recursion can lead to cleaner code in some scenarios, though it may consume more stack space.
Code Readability and Maintainability
Writing clean and maintainable code when working with maximums is equally critical. This includes using descriptive variable names, consistent formatting, and clear logic. This clarity makes it easier for others (or even yourself) to understand the code when revisiting it in the future.

Common Mistakes and Troubleshooting
Misunderstanding std::max Behavior
A frequent mistake made by programmers is misunderstanding how `std::max` operates, particularly when multiple parameters are provided. It's crucial to know that `std::max` only evaluates two arguments at a time. Thus, when comparing more than two values, one must chain calls to `std::max` correctly.
Handling Edge Cases
When working with arrays or vectors, handling edge cases is vital. For instance, if the input vector is empty, accessing an element or performing a maximum calculation could lead to runtime errors.
Here's an example of handling an empty vector:
std::vector<int> numbers;
if (numbers.empty()) {
std::cout << "Vector is empty, no maximum." << std::endl;
}
This simple check ensures that you do not attempt to process an empty dataset, which might cause your program to crash.
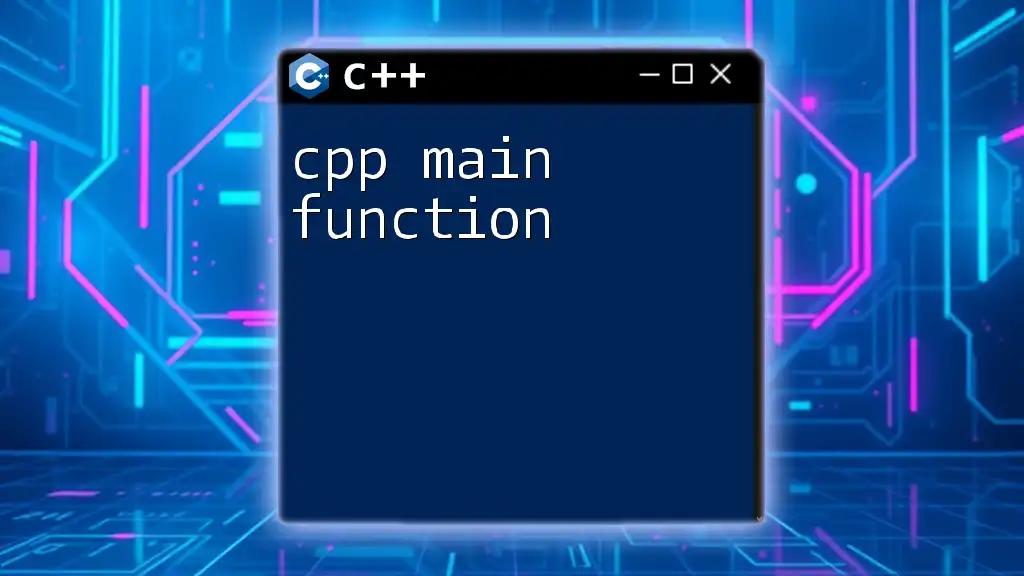
Conclusion
Understanding cpp maximums is fundamental for effective programming in C++. From basic usages of `std::max` to advanced techniques like recursive functions and custom comparisons, mastering these concepts can significantly enhance both performance and readability of your code. By implementing best practices and being mindful of potential pitfalls, you can efficiently handle maximum values in C++, paving the way for robust algorithm development and data management.