In C++, the `cpp` command is often used for processing a file and generating its output with macros and preprocessor directives, and an example of a simple use case for sending an email could look like this:
#include <iostream>
int main() {
std::cout << "Subject: Test Email\n";
std::cout << "To: example@example.com\n";
std::cout << "Hello, this is a test email!\n";
return 0;
}
(Note: The above code is a simplified example for educational purposes and does not actually send an email. To send an email in C++, you would typically use a library or an external service.)
Setting Up Your Environment
Requirements
To get started with cpp mail, you need to ensure that your environment is fully equipped with the necessary libraries and frameworks. The essential components include:
- libcurl: A free and easy-to-use client-side URL transfer library that supports various protocols, including SMTP.
- openssl: Required for secure communication over SSL/TLS.
Installing these packages is a straightforward process. For most Linux distributions, you can use the package manager:
sudo apt-get install libcurl4-openssl-dev libssl-dev
For Windows, you can download the binaries from their respective official websites or use package managers like vcpkg or nuget.
Configuring Your Development Environment
Once you have the necessary libraries installed, it’s important to configure your Integrated Development Environment (IDE).
For example, if you’re using Visual Studio:
- Create a new C++ project.
- Link libcurl and Openssl: Go to project properties and set the appropriate include directories and library paths.
To ensure everything is functioning correctly, you can create a simple cpp mail project that connects to a test SMTP server.
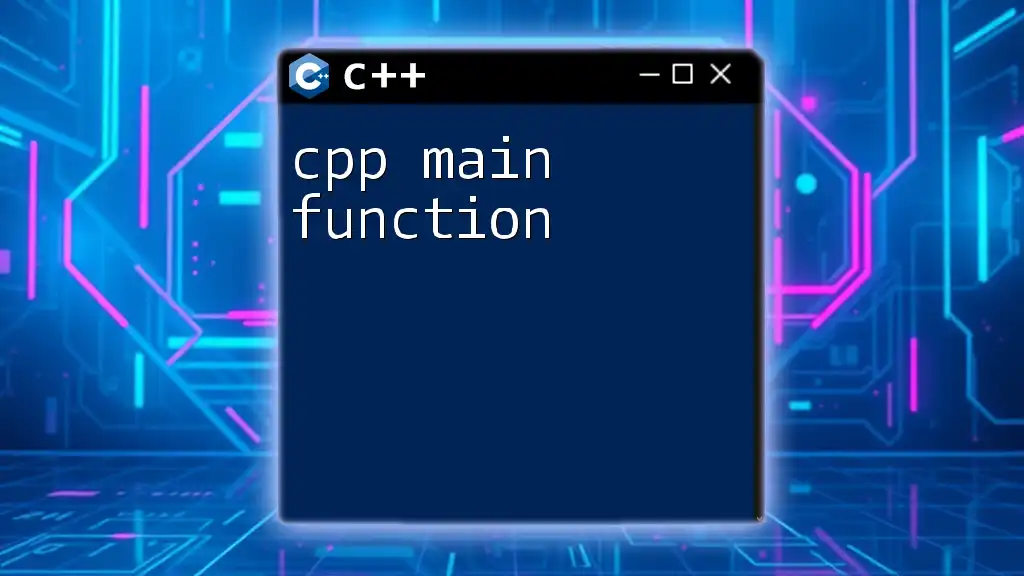
Basic Concepts of cpp mail
Understanding the Components of cpp mail
Before diving into coding, it’s crucial to understand the components involved in cpp mail. The key players are servers, clients, and protocols.
- SMTP Server: Responsible for sending emails.
- Email Client: The application that allows users to create, send, and manage their emails.
- Protocol Types: SMTP (Simple Mail Transfer Protocol) is mainly used for sending emails, whereas IMAP (Internet Message Access Protocol) and POP3 (Post Office Protocol) are often used to retrieve emails.
SMTP Protocol
Introduction to SMTP
The SMTP protocol is the backbone of email communication. It governs how emails are sent and routed to the recipient's mail server. Understanding SMTP is vital for implementing cpp mail effectively.
Creating a Basic SMTP Client
To send an email, you need to set up a connection with an SMTP server. Below is a code snippet demonstrating how to connect to an SMTP server using cpp:
#include <iostream>
#include <curl/curl.h>
int main() {
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "smtp://smtp.example.com:587");
// Add any necessary authentication
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
}
return 0;
}
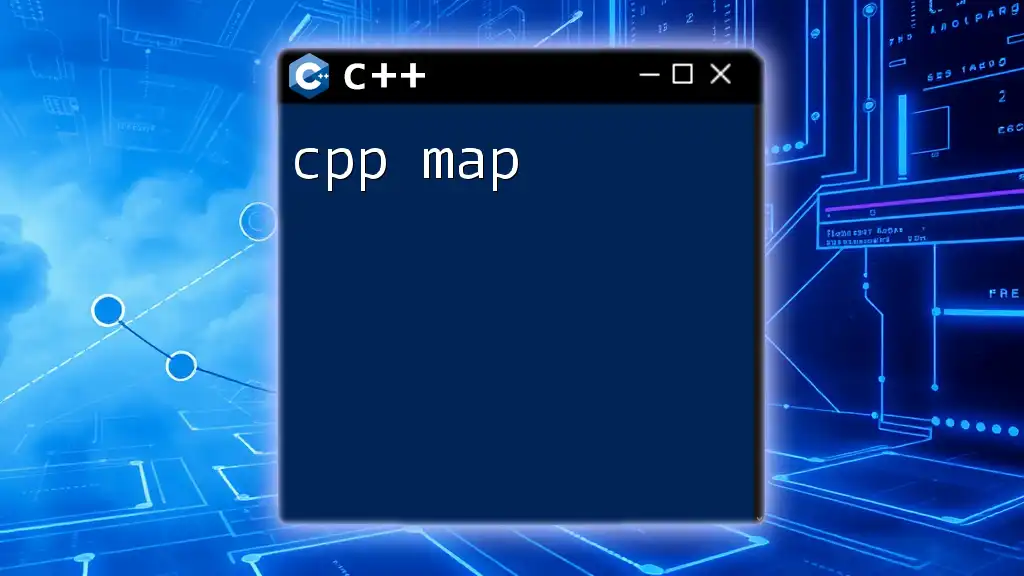
Sending Emails with cpp mail
Setting Up the Connection
Connecting to an SMTP server requires a few steps. You need to specify the server’s address, port, and account credentials. It is best to use secure connections whenever possible. The following code snippet demonstrates how to establish a secure connection using TLS:
curl_easy_setopt(curl, CURLOPT_USE_SSL, CURLUSESSL_ALL);
curl_easy_setopt(curl, CURLOPT_USERNAME, "your_email@example.com");
curl_easy_setopt(curl, CURLOPT_PASSWORD, "your_password");
Drafting Your Email
The next step involves composing your email. The email consists of several components, including headers and body text.
Here’s a code snippet that demonstrates how to create a well-formed email in cpp:
curl_easy_setopt(curl, CURLOPT_MAIL_FROM, "<your_email@example.com>");
struct curl_slist *recipients = NULL;
recipients = curl_slist_append(recipients, "<recipient@example.com>");
curl_easy_setopt(curl, CURLOPT_MAIL_RCPT, recipients);
curl_easy_setopt(curl, CURLOPT_READDATA, &data);
curl_easy_setopt(curl, CURLOPTupload, 1L);
Sending the Email
After configuring all the parameters, you are ready to send the email. The sending process is initiated by calling `curl_easy_perform()` again. Here’s how to actually send the email:
res = curl_easy_perform(curl);
if(res != CURLE_OK) {
fprintf(stderr, "curl_easy_perform() failed: %s\n", curl_easy_strerror(res));
}
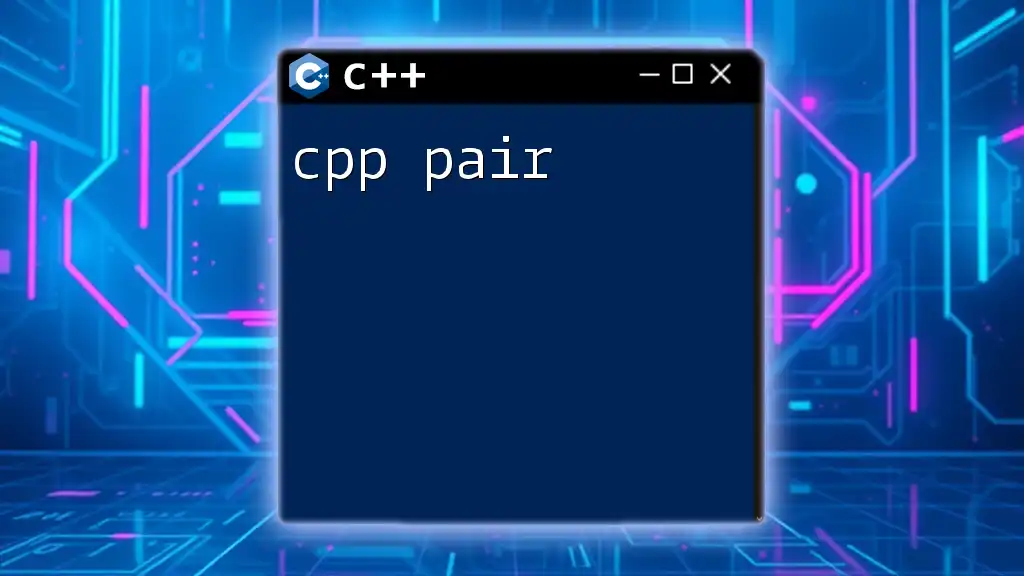
Handling Attachments
How to Attach Files to Your Email
Attachments are an essential feature of email communication. They allow users to send documents, images, and other file types along with their messages.
Code Example: Adding Attachments
To enable attachments, you first specify the file in your email client settings. Here’s a simplified code snippet to demonstrate how to attach a file:
curl_easy_setopt(curl, CURLOPT_MIMEPOST, mime);
Using the `libcurl` library allows you to implement complex attachments such as multiple files or MIME types efficiently.
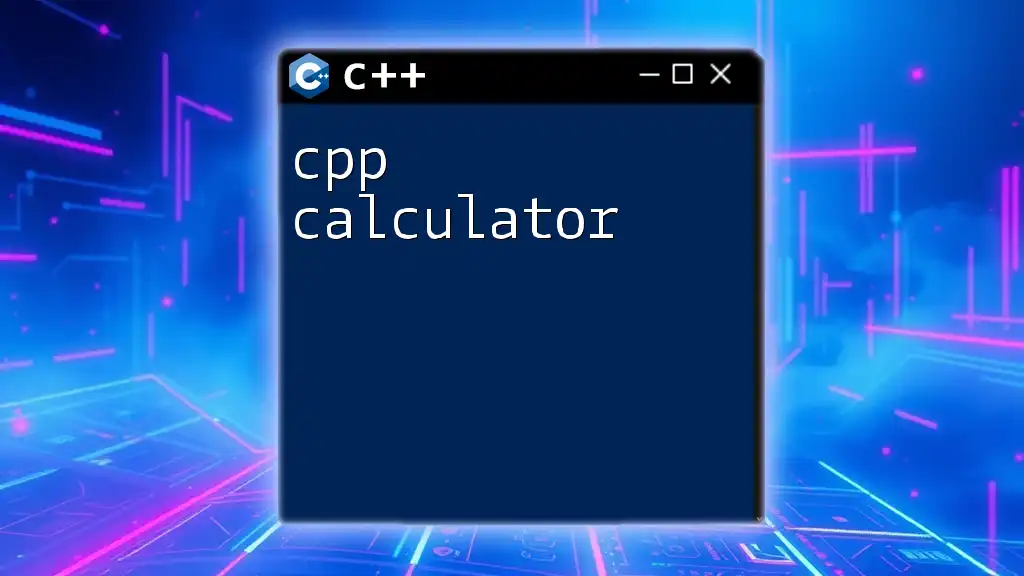
Error Handling and Debugging
Common Issues with cpp mail
When dealing with cpp mail, several issues may arise, including connection problems, authentication errors, or problems with the SMTP server.
Debugging Tips
Utilizing logging is an effective way to troubleshoot errors in your application. By implementing log statements, you can see where issues are occurring. Here’s a code snippet for setting up basic error logging:
if(res != CURLE_OK) {
std::cerr << "Error: " << curl_easy_strerror(res) << '\n';
}
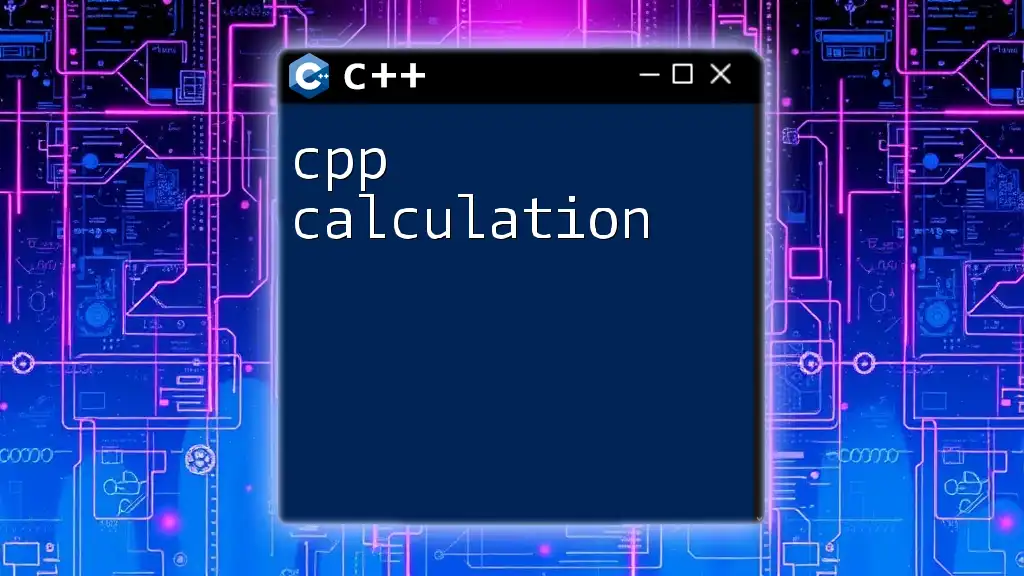
Best Practices for Using cpp mail
Security Considerations
When dealing with email communication, security must be a top priority. Always ensure that sensitive data such as user passwords are protected through SSL/TLS connections. Regularly update your libraries to patch any vulnerabilities.
Making Your Emails Stand Out
To improve engagement, invest time in crafting compelling subject lines, selecting appropriate sending times, and ensuring your email format is visually appealing. Personalized content tends to yield better responses.
Avoiding Spam Filters
Design your emails in a way that minimizes the risk of landing in spam folders. Use proper authentication techniques such as DKIM and SPF, and avoid spammy phrases that can trigger filters.
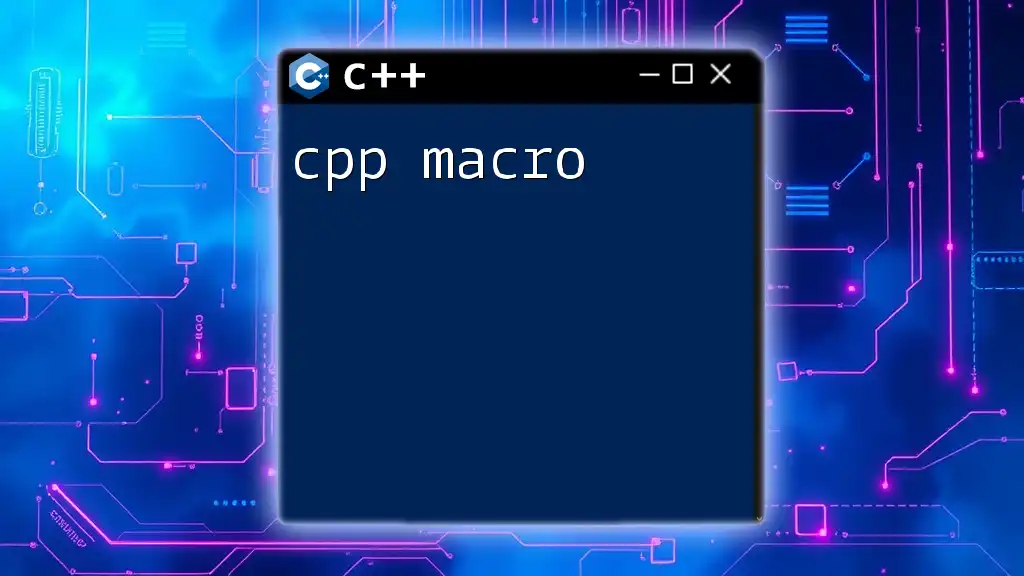
Advanced Features
Using Templates for Emails
Dynamic email templates allow for personalized user experiences. By integrating template engines, you can populate email content based on user data effectively.
Integrating with Other Services
Many applications benefit from integration with third-party services like Mailchimp or SendGrid. This can enhance your cpp mail feature by adding capabilities like tracking and analytics.
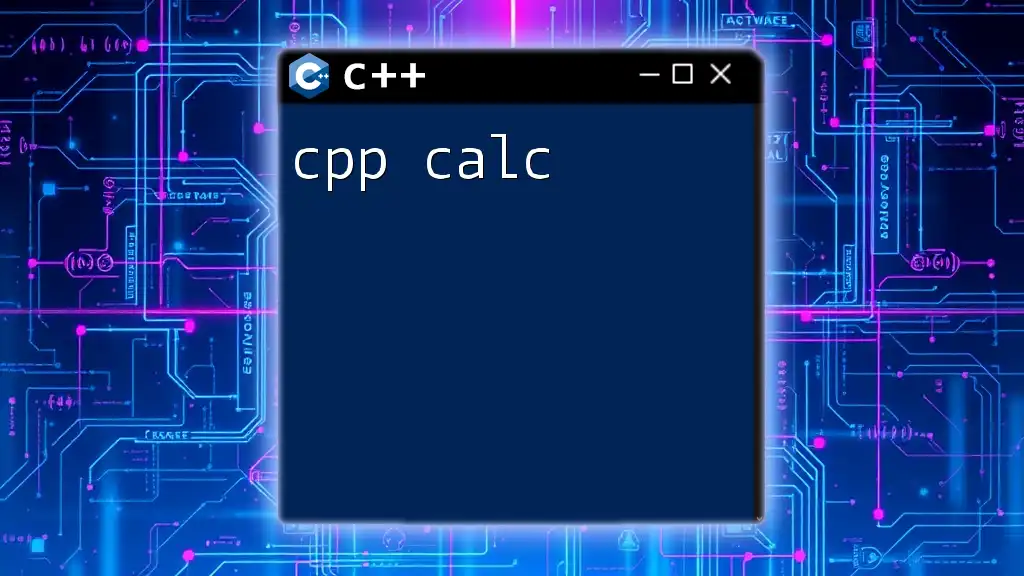
Conclusion
In this guide, we explored cpp mail, covering everything from setting up your development environment to sending emails with attachments. As you continue to experiment with these concepts and code examples, you’ll find that cpp mail is a powerful tool for enhancing communication in your applications.
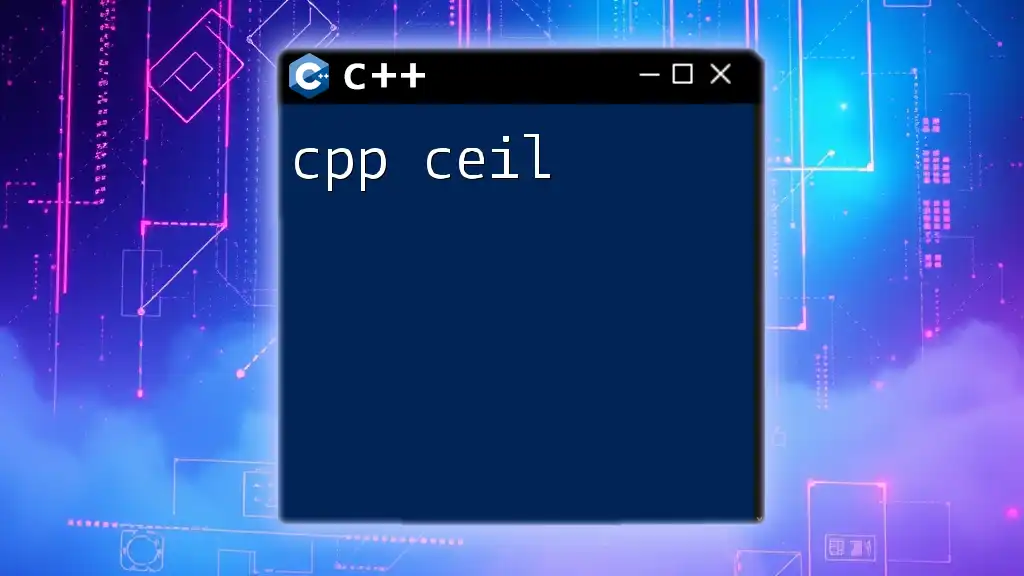
Additional Resources
To further your cpp mail journey, consider exploring libcurl and OpenSSL documentation for deeper insights. Additionally, online courses and tutorials are plentiful and can enhance your skillset significantly. Happy coding!