The "cpp calc" command allows users to perform quick arithmetic calculations within the C++ preprocessor by defining simple macros for mathematical operations.
Here's a code snippet demonstrating how to use it:
#define ADD(x, y) ((x) + (y))
#define SUBTRACT(x, y) ((x) - (y))
#define MULTIPLY(x, y) ((x) * (y))
#define DIVIDE(x, y) ((x) / (y))
// Usage examples
// int sum = ADD(5, 3); // sum will be 8
// int difference = SUBTRACT(5, 3); // difference will be 2
// int product = MULTIPLY(5, 3); // product will be 15
// int quotient = DIVIDE(6, 3); // quotient will be 2
What is `cpp calc`?
`cpp calc` refers to a set of commands and operations used in C++ programming to perform calculations. As a critical aspect of C++, these commands enable developers to efficiently handle mathematical operations, ranging from basic arithmetic to more sophisticated calculations involving advanced functions. The utility of `cpp calc` is evident in various applications, whether you're developing software, scripting, or solving mathematical problems.
Key Concepts
Understanding the foundational concepts behind `cpp calc` is crucial. You'll often encounter basic mathematical operations, which include:
- Addition
- Subtraction
- Multiplication
- Division
Additionally, being familiar with data types such as integers, floats, and doubles is vital. Each of these data types has specific use cases and limitations, influencing how you handle calculations within your code.
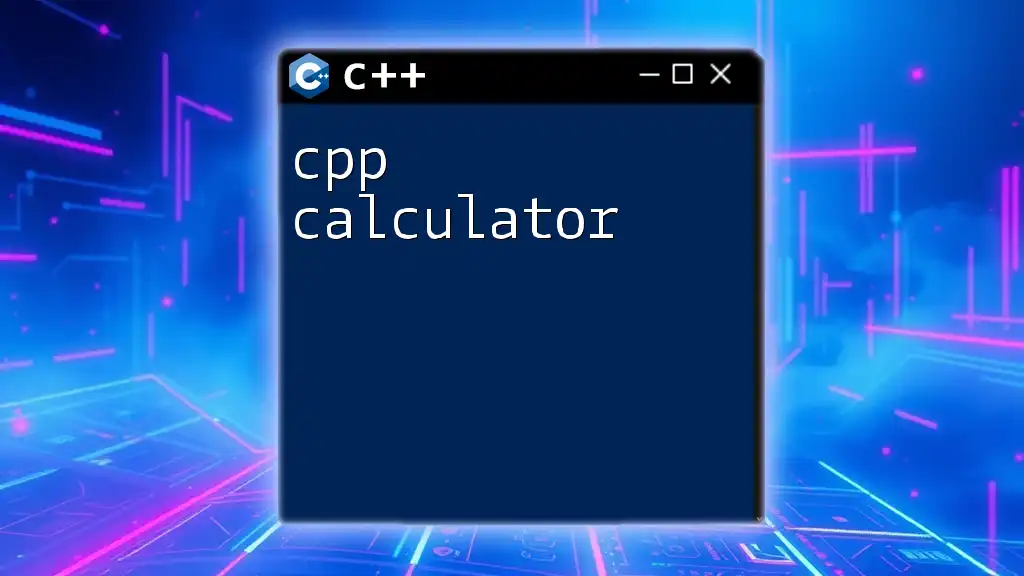
Setting Up Your C++ Environment
Necessary Tools
To work effectively with `cpp calc`, you'll need a proper development environment. Suitable Integrated Development Environments (IDEs) include:
- Visual Studio: Great for Windows users, offering extensive debugging and editing features.
- Code::Blocks: A free option that supports multiple compilers.
- CLion: A powerful IDE from JetBrains favored by many professional developers.
Setting up a C++ compiler, such as GCC or Clang, is also essential to compile and run your code effectively. Each compiler has its installation guide, which typically includes downloading and configuring the necessary binaries.
Example: Basic C++ Program Structure
Writing the simplest C++ program can familiarize you with the syntax. Here’s how a basic program structure looks:
#include <iostream> // Header file for input/output streams
using namespace std;
int main() {
// Your cpp calc code here
return 0;
}
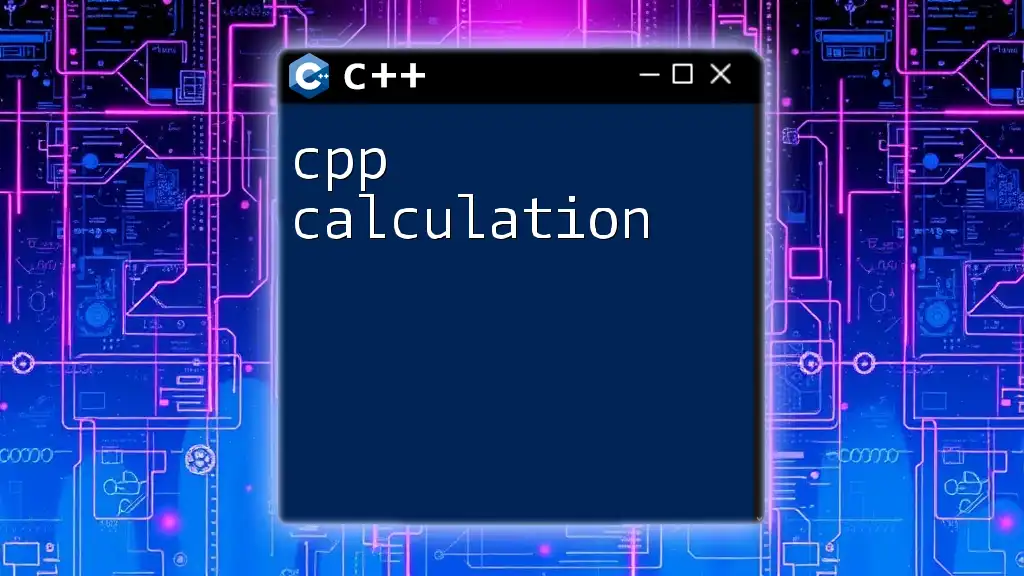
Essential `cpp calc` Commands
Basic Arithmetic Operations
At the core of `cpp calc` are the fundamental arithmetic operations. C++ provides intuitive symbols to perform calculations:
- Addition: `+`
- Subtraction: `-`
- Multiplication: `*`
- Division: `/`
Code Example: Basic Arithmetic
Here’s a simple demonstration of basic arithmetic operations in C++:
int a = 10;
int b = 5;
int sum = a + b; // Addition
int diff = a - b; // Subtraction
int product = a * b; // Multiplication
float quotient = static_cast<float>(a) / b; // Division
In this snippet, we've initiated two integers, `a` and `b`, then performed addition, subtraction, multiplication, and division. Note the use of `static_cast<float>(a)` to ensure the division results in a floating-point number instead of truncating to an integer.
Advanced Mathematical Functions
For more complex calculations, C++ offers an extensive mathematics library—`<cmath>`. By leveraging this library, you can access advanced mathematical functions that enhance your programming capabilities.
Key Functions
Some key functions you will frequently utilize include:
- Square Root: `sqrt()`
- Power: `pow(base, exponent)`
Code Example: Using Advanced Functions
Let’s look at how to implement these functions in a program:
#include <cmath>
#include <iostream>
using namespace std;
int main() {
int number = 16;
float root = sqrt(number); // Square root of 16
float power = pow(2, 3); // 2 to the power of 3
cout << "Square root of " << number << " is " << root << endl;
cout << "2 to the power of 3 is " << power << endl;
return 0;
}
In this example, we calculate the square root of 16 and \(2^3\), showcasing the usability of the `<cmath>` library for advanced mathematical operations.
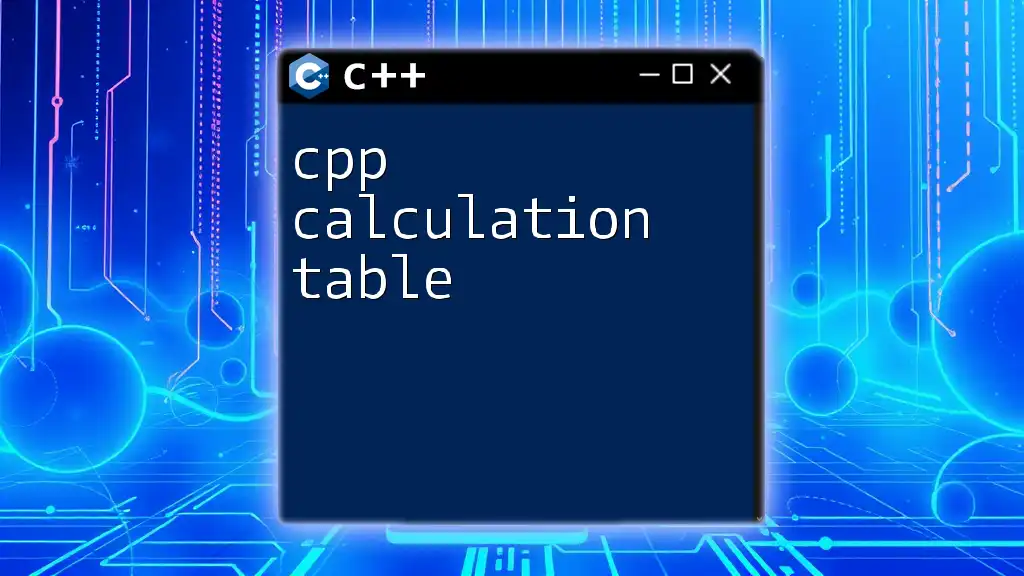
Handling User Input for Calculations
Taking Input from the User
An integral part of programming is interacting with users. In C++, you can use the `cin` command to take input. It's essential first to validate the data to avoid runtime errors.
Code Example: Interactive Calculator
Here’s a simple interactive calculator prompt:
#include <iostream>
using namespace std;
int main() {
float num1, num2;
char operation;
cout << "Enter first number: ";
cin >> num1;
cout << "Enter an operator (+, -, *, /): ";
cin >> operation;
cout << "Enter second number: ";
cin >> num2;
// Further calculation logic here
return 0;
}
In this code snippet, we prompt the user to enter two numbers and an operator. Note how we capture user input with `cin` to facilitate future calculations.
Outputting Results
Once you've captured user input and performed calculations, formatting the output appropriately is crucial for clarity. C++ offers the `cout` command for outputting results.
Code Snippet: Formatted Output Example
When displaying results, you might want to maintain fixed-point notation for better presentation. Here’s how:
cout << "Result: " << fixed << setprecision(2) << result << endl;
In this snippet, `setprecision(2)` limits the result display to two decimal places, enhancing readability.
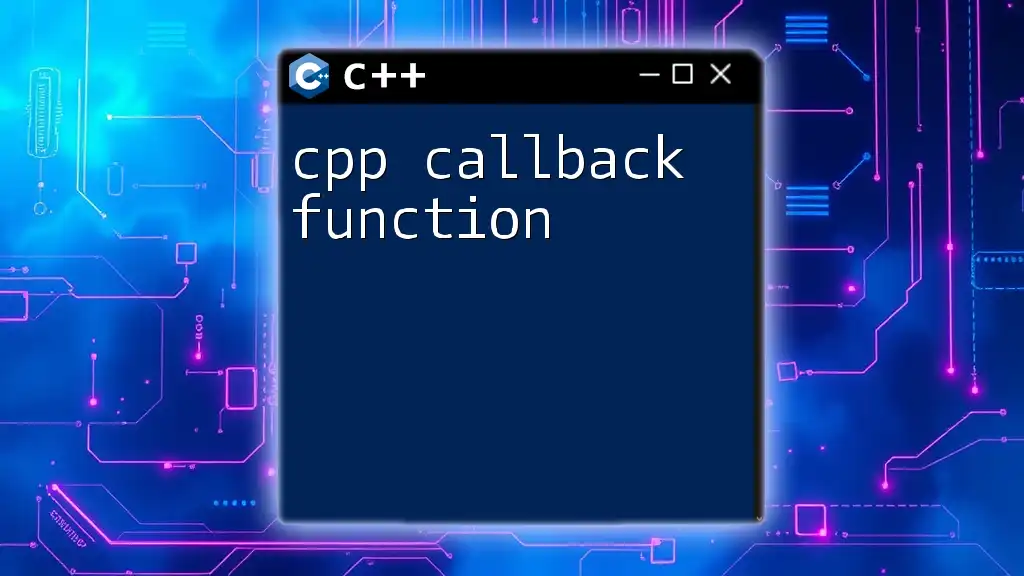
Error Handling in C++ Calculations
Common Errors
While working with calculations, you might encounter issues such as division by zero or data type mismatches. Recognizing and addressing these errors is pivotal in developing reliable software.
Implementing Error Handling
To prevent runtime errors, implement conditional checks. For example, before performing division, ensure that you're not dividing by zero.
Code Example: Error Handling
Here's how to handle division safely:
if (num2 == 0) {
cout << "Error: Division by zero is not allowed!" << endl;
} else {
float quotient = num1 / num2;
cout << "Quotient: " << quotient << endl;
}
This error-handling mechanism prompts an error message if the user attempts to divide by zero, thereby preventing the program from crashing.
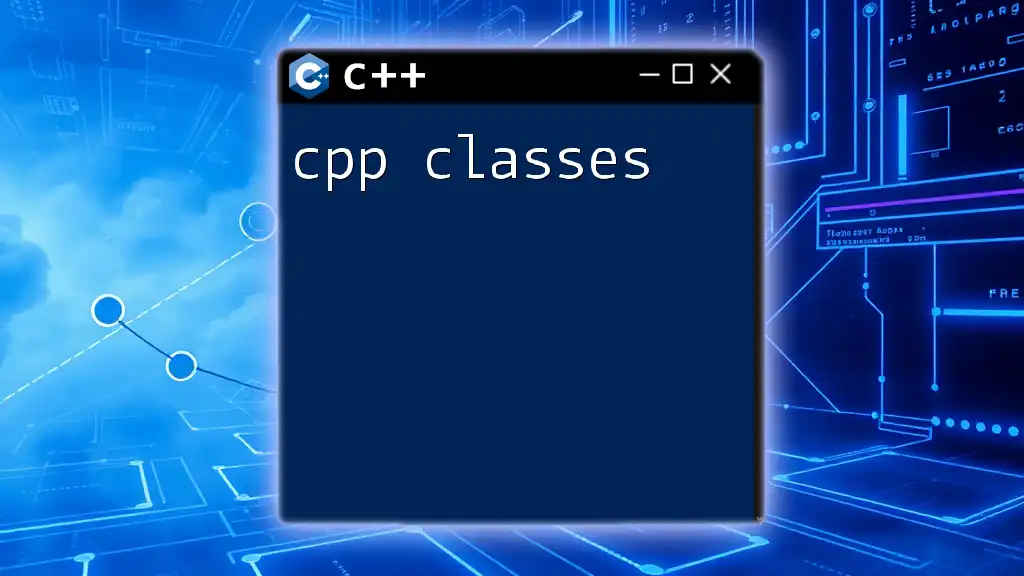
Creating Functions for Reusable Calculations
Introduction to Functions in C++
Functions are essential for simplifying repetitive tasks in your programs. Instead of rewriting the same code, functions allow you to encapsulate logic and reuse it whenever necessary.
Example Function: Simple Calculator
Creating a basic calculator function can significantly streamline your code. Here’s an example function that achieves this:
float calculate(float a, float b, char operation) {
switch(operation) {
case '+': return a + b;
case '-': return a - b;
case '*': return a * b;
case '/': return (b != 0) ? a / b : throw invalid_argument("Division by zero");
default: throw invalid_argument("Invalid operation");
}
}
This function takes two operands and an operator as arguments and returns the calculated result based on the specified operation. The use of `switch-case` statements enhances clarity and control over multiple operations.
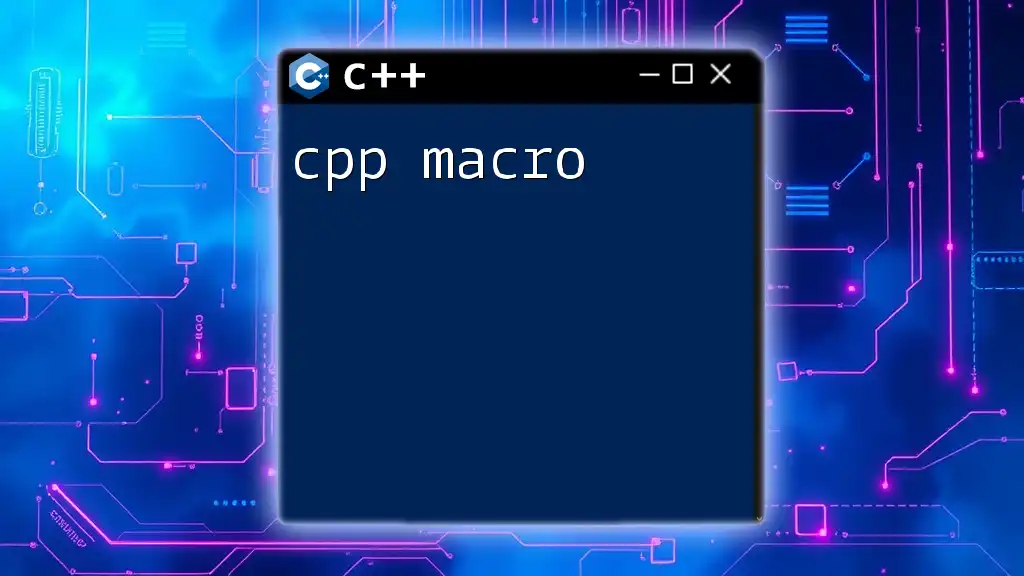
Conclusion
Mastering `cpp calc` commands equips you with the necessary skills to handle calculations within your C++ programs efficiently. By exploring basic and advanced mathematical functions, user input handling, and error management, you prepare yourself for a variety of programming challenges. Keep practicing with additional coding exercises and challenges to solidify your understanding, and don’t hesitate to seek further resources to deepen your knowledge of C++.