A C++ class catalog is a structured collection of class definitions that helps organize and manage various classes within a project, showcasing their attributes and methods for better code reusability and clarity.
class Car {
public:
string brand;
int year;
void displayInfo() {
cout << "Brand: " << brand << ", Year: " << year << endl;
}
};
Understanding C++ Classes
What is a Class?
In C++, a class is a blueprint for creating objects. It encapsulates data for the object and methods to manipulate that data. Classes are foundational to Object-Oriented Programming (OOP), emphasizing abstraction, encapsulation, inheritance, and polymorphism.
Class vs. Object
While a class defines the structure and behavior of objects, an object is an instance of a class. For example, if `Dog` is a class, then `myDog` can be an object of the `Dog` class.
Basic Structure of a Class
The syntax for declaring a class includes a header, body, and access specifiers. Here’s a basic structure:
class ClassName {
// member variables
// member functions
};
Understanding this structure is vital for defining new classes effectively in C++.
Access Specifiers
Access specifiers control the visibility of class members. The three types are:
- Public: Members accessible from outside the class.
- Private: Members not accessible from outside the class.
- Protected: Members accessible to derived classes but not outside the class hierarchy.
Here’s an example:
class Example {
private:
int privateData; // Accessible only within this class
public:
void setData(int value) {
privateData = value;
}
};
In this example, `privateData` can only be modified using the public method `setData()`.
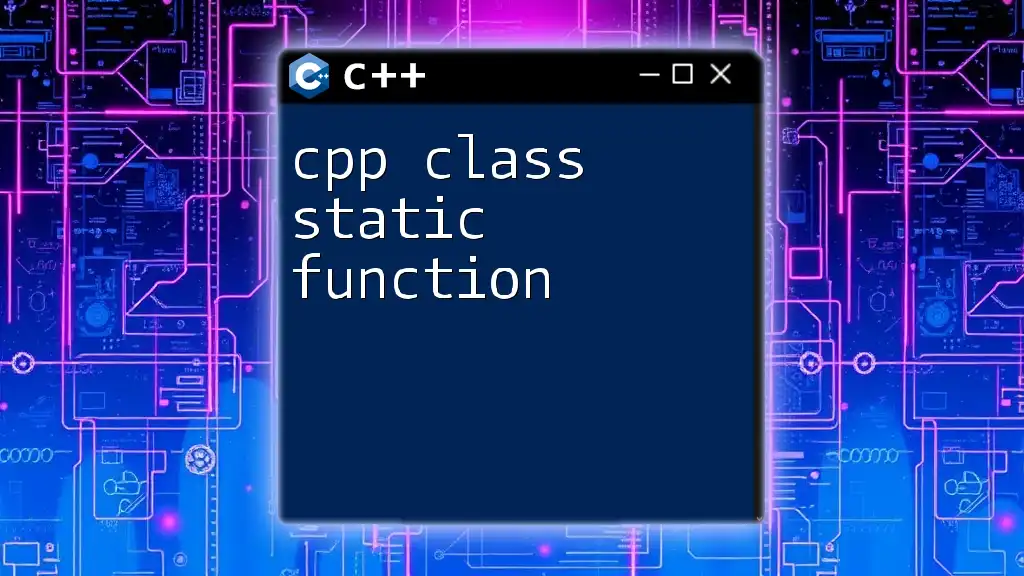
Types of C++ Classes
User-Defined Classes
User-defined classes allow programmers to create types tailored to their application's needs. A simple example of a user-defined class is a `Dog` class:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
This class encapsulates the behavior of a dog, showcasing the essence of C++ classes.
Abstract Classes
Abstract classes serve as base classes that can’t be instantiated. They contain at least one pure virtual function, indicating an incomplete class meant to be derived from. Here's an example:
class Animal {
public:
virtual void sound() = 0; // Pure virtual function
};
In this case, any class derived from `Animal` must provide an implementation for `sound()`.
Inheritance in Classes
Inheritance allows classes to derive properties and methods from other classes. C++ supports both single and multiple inheritance. Here’s an example illustrating single inheritance:
class Parent {
public:
void greet() {
std::cout << "Hello from Parent!" << std::endl;
}
};
class Child : public Parent {
};
Here, `Child` inherits the `greet` method from `Parent`, promoting code reuse and better organization.
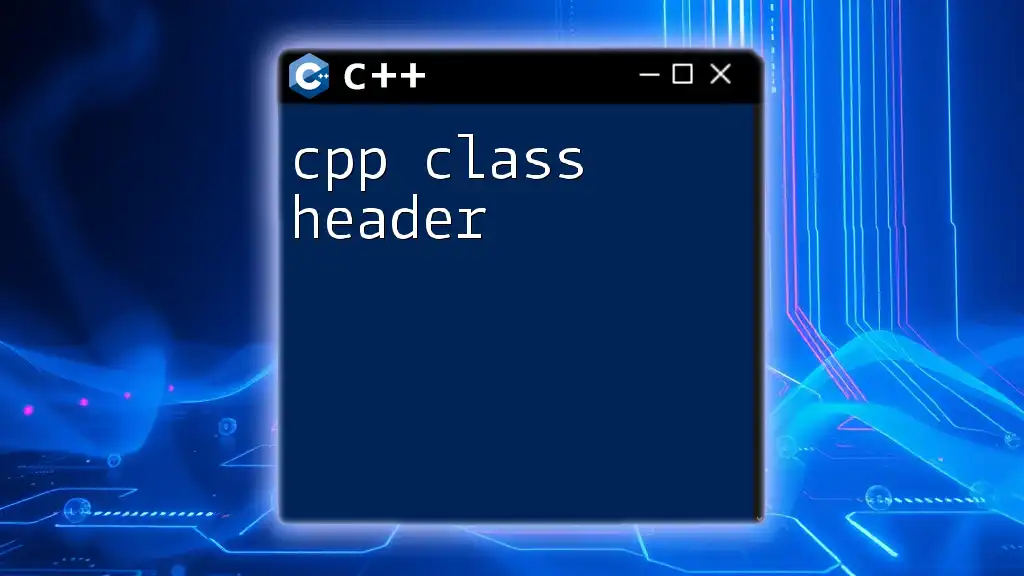
Class Components
Member Variables
Member variables are the data portions of a class. Each instance of the class has its own copy of the member variables, thus maintaining separate states for different objects.
class Car {
private:
std::string brand;
int speed;
public:
void setSpeed(int s) {
speed = s;
}
};
In this `Car` class, `brand` and `speed` serve as private member variables, ensuring encapsulation.
Member Functions
Member functions define the behavior of the class. They can modify the state of member variables or provide functionality to the objects.
class Calculator {
public:
int add(int a, int b) {
return a + b;
}
};
The `add` method illustrates how member functions can be used to perform operations relevant to the object’s functionality.
Constructors and Destructors
Constructors are special functions invoked when an object of a class is created. They initialize member variables. C++ supports default and parameterized constructors.
class Point {
public:
int x, y;
Point(int a, int b) : x(a), y(b) {}
};
In this example, when a `Point` object is instantiated, the coordinates are set to the values provided as arguments.
Destructors are invoked when an object is destroyed, performing necessary cleanup.
class MyClass {
public:
~MyClass() {
// Cleanup code
}
};
Proper use of destructors prevents memory leaks and ensures that resources are released when no longer needed.
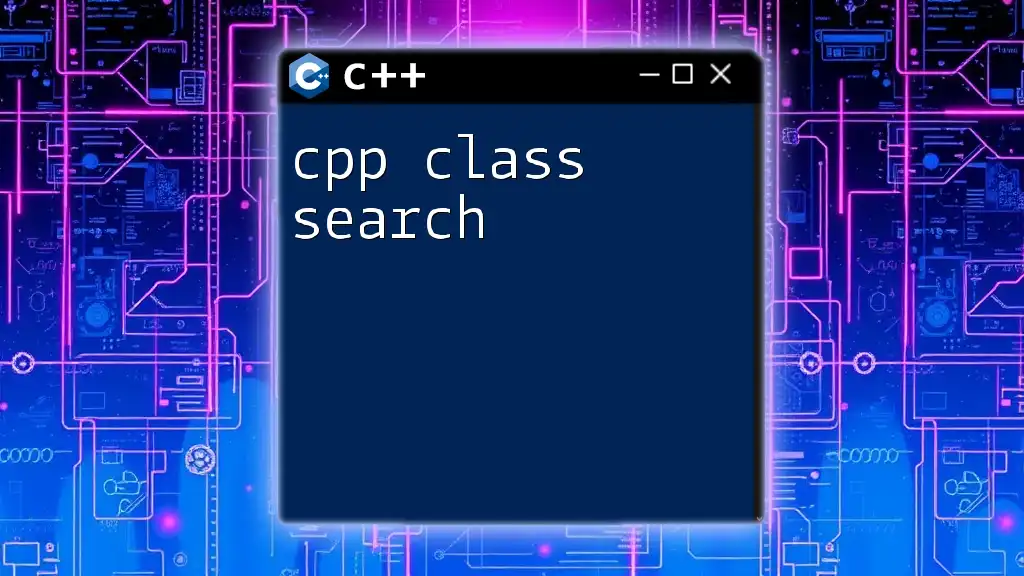
Advanced Class Concepts
Operator Overloading
C++ allows the overloading of operators, enabling operators to work with user-defined data types. This enhances code readability and provides intuitive syntax.
class Complex {
public:
int real, imag;
Complex operator+(const Complex& obj) {
Complex result;
result.real = this->real + obj.real;
result.imag = this->imag + obj.imag;
return result;
}
};
In this `Complex` class, the `+` operator is overloaded, allowing complex numbers to be added intuitively.
Friend Functions
Friend functions can access private and protected members of a class. This is beneficial when you want a non-member function to operate on class internals.
class Box {
friend void printVolume(Box b);
private:
int volume;
};
void printVolume(Box b) {
std::cout << "Volume: " << b.volume << std::endl;
}
In this case, `printVolume` can access the private `volume` attribute of `Box`, demonstrating its utility.
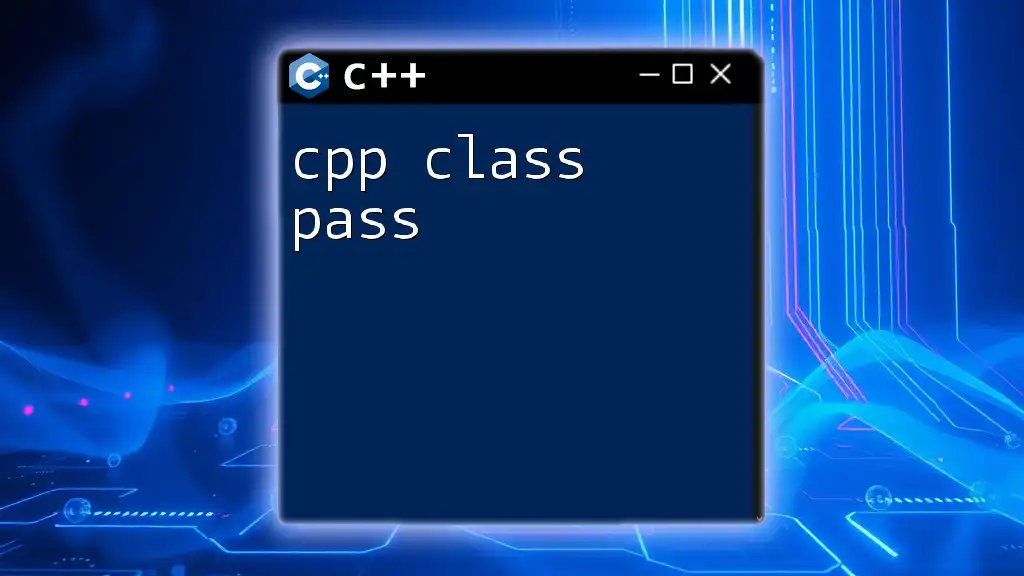
Working with Templates
Introduction to Class Templates
C++ supports class templates, allowing classes to operate with generic types. This results in reusable code that can handle various data types without modification.
template <typename T>
class Pair {
public:
T first, second;
Pair(T a, T b) : first(a), second(b) {}
};
This `Pair` class enables the creation of pairs of any type, enhancing flexibility in data structures.
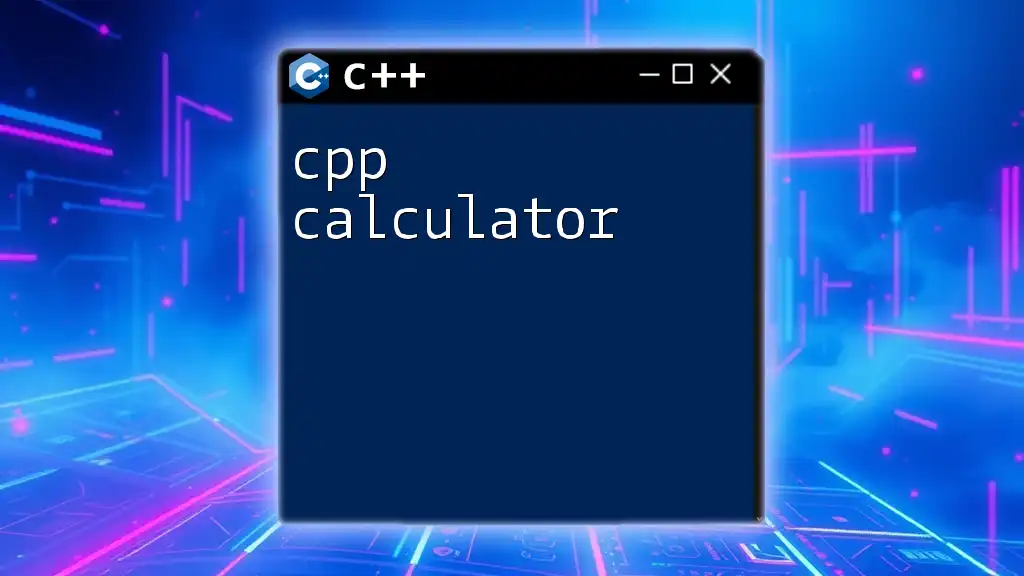
Best Practices for Designing Classes
Encapsulation and Information Hiding
Effective encapsulation focuses on keeping member variables private and exposing only necessary methods. This practice hides implementation details and protects the integrity of the data.
Keeping Classes Focused
Following the Single Responsibility Principle (SRP), each class should have one reason to change. This principle leads to cleaner, more maintainable code, making it easier to comprehend and debug.
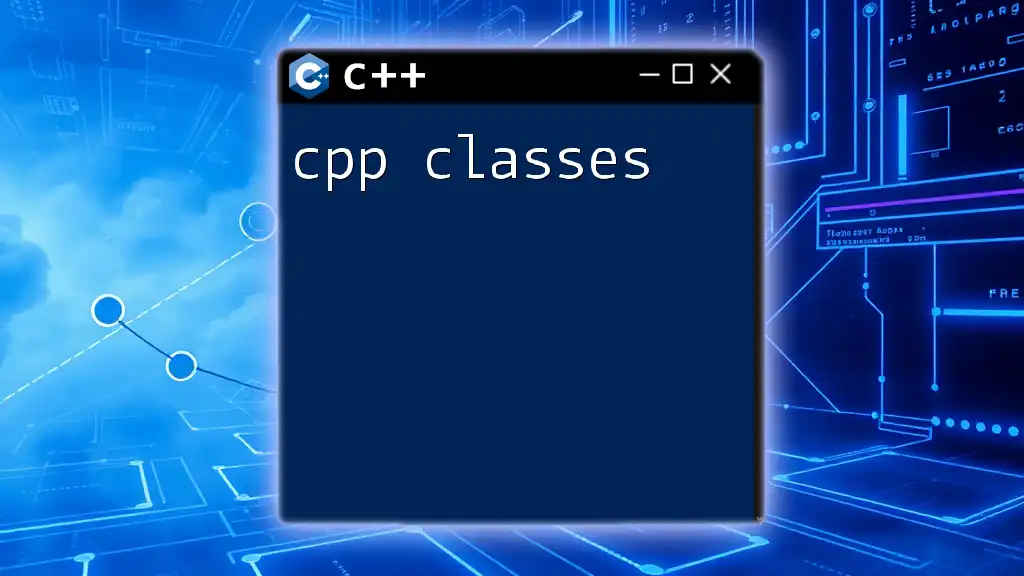
Conclusion
C++ classes are powerful tools for organizing and managing code. By mastering classes, programmers can embrace the principles of OOP, improving not only their coding practices but also the architecture of their applications.
Embrace the concepts within this cpp class catalog. Practice coding with classes and implementing these principles in real-world projects to enhance your understanding and proficiency in C++.
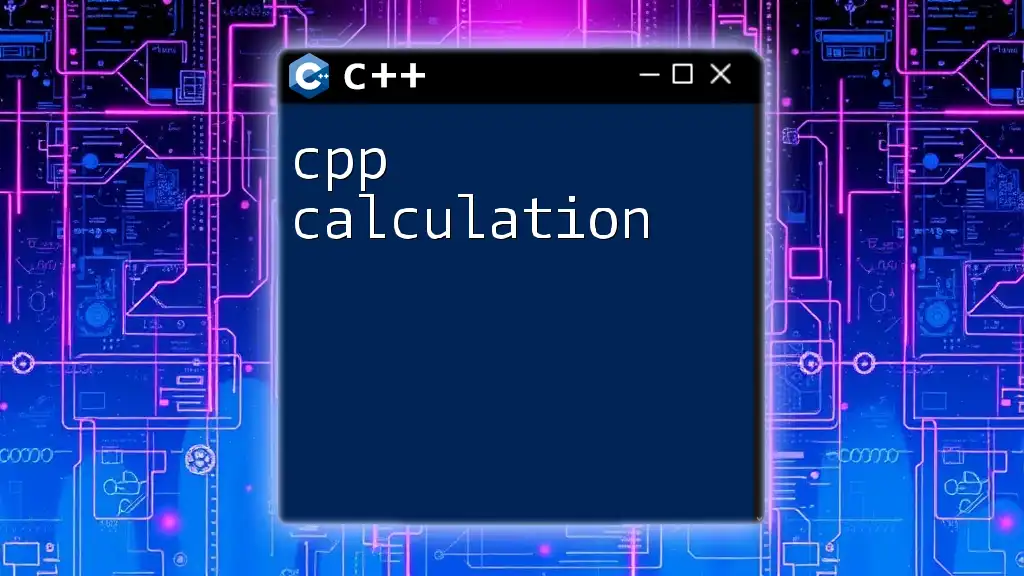
Additional Resources
Books and Online Tutorials
For continued learning, explore recommended books and online resources focusing on C++ programming and advanced OOP concepts.
Coding Challenges
Engage in coding exercises to solidify your understanding of class design and relationships. Challenge yourself with problems that require implementing unique classes and leveraging the features discussed in this guide.