A static member in a C++ class is a member variable or function that belongs to the class itself rather than any specific object, allowing it to be shared across all instances of the class.
Here’s a simple example:
#include <iostream>
class MyClass {
public:
static int staticMember; // Static member declaration
void increment() {
staticMember++; // Increment static member
}
static void display() { // Static member function
std::cout << "Static Member: " << staticMember << std::endl;
}
};
int MyClass::staticMember = 0; // Static member definition
int main() {
MyClass obj1, obj2;
obj1.increment();
obj2.increment();
MyClass::display(); // Display static member
return 0;
}
What Is a C++ Static Class Member?
A C++ class static member is a variable or function that is shared across all instances of a class. Unlike regular members, which each object instance owns, static members belong to the class itself, allowing all instances to access the same variable or method. This characteristic grants static members unique properties that are beneficial in various programming scenarios.
Characteristics of Static Members
Static members possess distinct characteristics:
-
Shared Among Instances: All instances of the class share the same static member. Thus, changing the value of a static member from one instance reflects that change across all instances.
-
Memory Allocation: Static members are allocated in a separate memory area (data segment) rather than on the stack like normal member variables, ensuring their persistence as long as the program runs.
-
Accessed Through Class Name: Static members can be accessed directly using the class name without needing to create an instance, reinforcing the idea that they are class-level attributes.

How to Declare a Static Member in C++
To declare a static member within a class, you can use the `static` keyword followed by the type, and the member name.
Syntax for Static Member Declaration
Here’s a simple example of declaring a static member:
class Sample {
public:
static int count; // Declaration of a static member
};
Static Member Initialization
While declaring static members is straightforward, initializing them requires a specific step. You must define and initialize static members outside of the class definition, typically in the source file (.cpp). Here's how to do this correctly:
int Sample::count = 0; // Defining and initializing the static member outside the class

Accessing C++ Static Class Members
Accessing static members can be performed both from within the class methods and outside the class.
Accessing in Class Methods
Static members can be accessed in both static and non-static methods. This is a powerful feature because it allows class-level functionality without relying on instance-specific data.
Example using a Static Method
class Sample {
public:
static void displayCount() {
std::cout << "Count: " << count << std::endl; // Accessing static member
}
};
Accessing Outside the Class
To access static members from outside the class, you do not need an object instance. You can call the static member directly using the class name, as shown below:
int main() {
Sample::count = 5; // Accessing static member directly
Sample::displayCount(); // Calling static method to display the count
}
This approach emphasizes the shared nature of static members and allows for clear code organization.

The Role of Static Members in Memory Management
Memory Allocation for Static Members
Static members are stored in the data segment of the memory, unlike instance members which reside on the stack. This allocation means that the memory for static members is reserved for the lifetime of the program, allowing values to persist beyond individual function calls or object scopes.
Life Cycle of Static Members
The lifetime of static members spans the entire runtime of the program. They are created when the program starts and are destroyed when the program terminates. This characteristic can be particularly useful for scenarios where you need to maintain an ongoing state, such as tracking counts or configuration settings across instances.

Use Cases for Static Members
Counting Instances of a Class
One of the most common use cases for static members is to keep track of the number of instances of a class. This is particularly useful for monitoring resource usage or implementing singleton patterns.
Code Snippet to Illustrate:
class Sample {
public:
static int instanceCount; // Static member to count instances
Sample() {
instanceCount++; // Increment the count when a new object is created
}
};
int Sample::instanceCount = 0; // Initialize static member
int main() {
Sample obj1, obj2; // Two instances created
std::cout << "Instances created: " << Sample::instanceCount << std::endl; // Output: 2
}
In this example, the static member `instanceCount` keeps track of how many `Sample` objects were instantiated, showcasing a practical application for static class members.

Limitations of C++ Static Members
Discouragement of Object-Oriented Principles
While static members can be very useful, over-relying on them can lead to designs that stray from core object-oriented principles. Using static members can limit the flexibility and modularity of your class since they tie the state to the class rather than individual instances.
Encapsulation Concerns
Static members can also raise concerns regarding encapsulation. Because they are shared across instances, you might expose class-level state inappropriately, leading to challenges in managing state and ensuring data integrity.
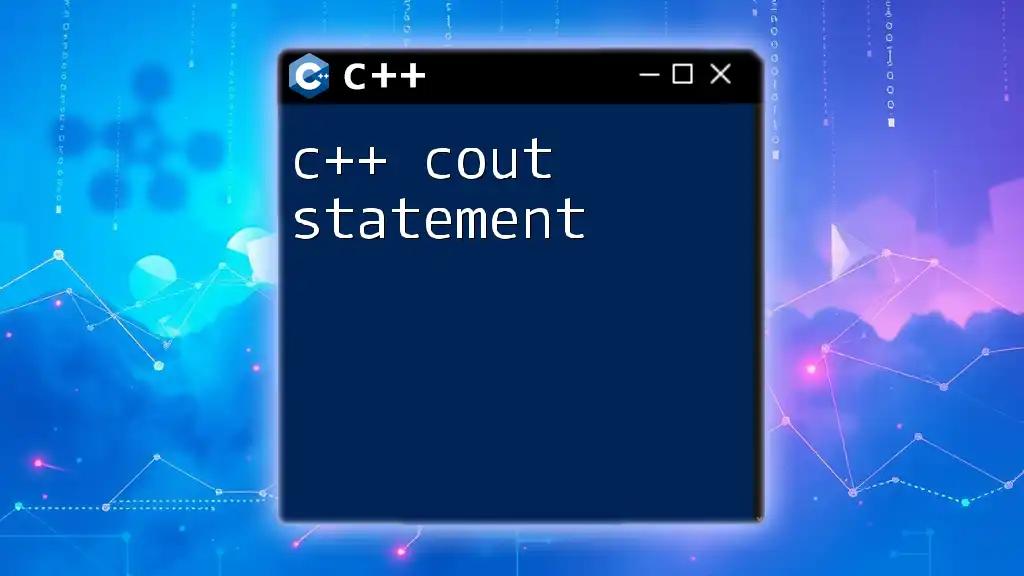
Conclusion on C++ Class Static Members
In summary, C++ class static members are a powerful feature that enhances the flexibility and capabilities of class design. Understanding how to declare, initialize, access, and use static members will empower you to write better code and leverage the full power of C++. As you continue your journey in C++, exploring static members opens up new avenues for creating efficient, maintainable software.
The interplay of static members in memory management and usage patterns provides rich learning opportunities for C++ developers at all levels.
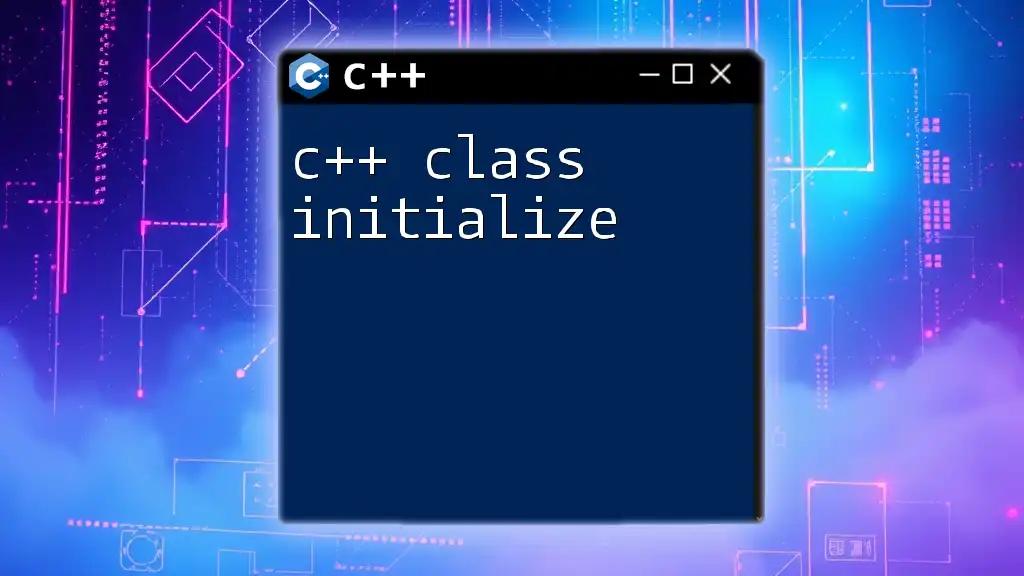
References and Further Reading
For deeper exploration of C++ topics and to enhance your understanding of static members and other advanced concepts, consider reading libraries, textbooks, or accessing reputable online resources that are geared towards C++ programming.