C++ classes allow the creation of user-defined types that can encapsulate data and functions, while inheritance enables a new class to derive properties and behaviors from an existing class, promoting code reuse and a hierarchical class structure.
Here's a simple example demonstrating classes and inheritance:
class Animal {
public:
void speak() {
std::cout << "Animal speaks" << std::endl;
}
};
class Dog : public Animal {
public:
void speak() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.speak(); // Outputs: Woof!
return 0;
}
What is a C++ Class?
A C++ class is a blueprint for creating objects. It allows you to encapsulate data and functions that operate on that data, promoting modularity and reusability in your code. Classes help organize code into logical, manageable pieces, making it easier to maintain and scale. Here's why classes are essential in C++:
- Data Encapsulation: Classes allow for bundling of data and methods, controlling access via access modifiers.
- Abstraction: They enable a simplified interface while hiding complex implementation details.
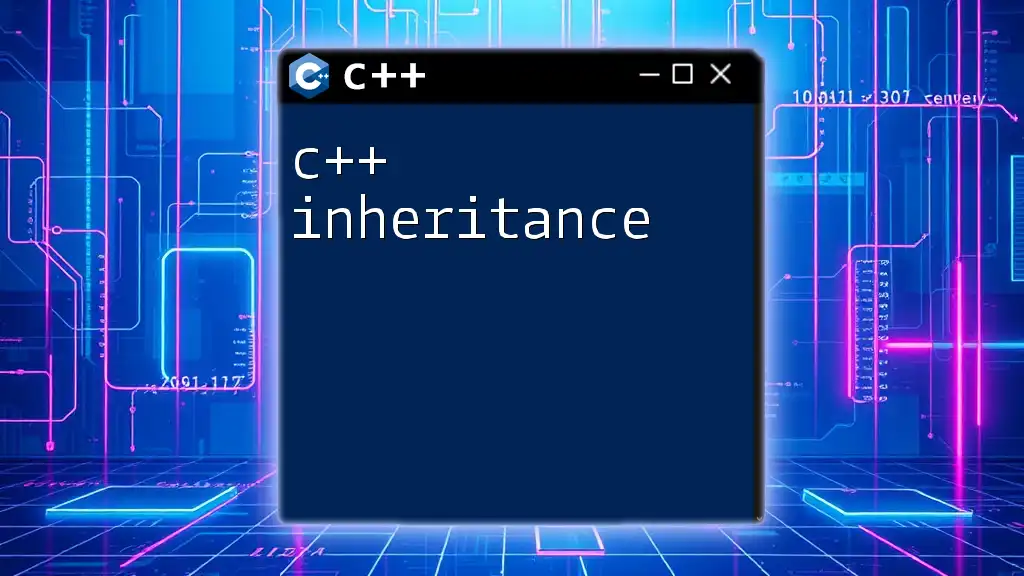
Understanding Inheritance in C++
Inheritance is a fundamental concept in C++ where one class shares the properties and behaviors (methods) of another. This mechanism allows for the creation of a new class that is based on an existing class, promoting code reusability and establishing a hierarchical relationship. Inheritance is particularly important for the following reasons:
- It reduces code duplication by allowing new classes to inherit common traits from existing classes.
- It enables the creation of a hierarchy that reflects real-world relationships and improves code organization.
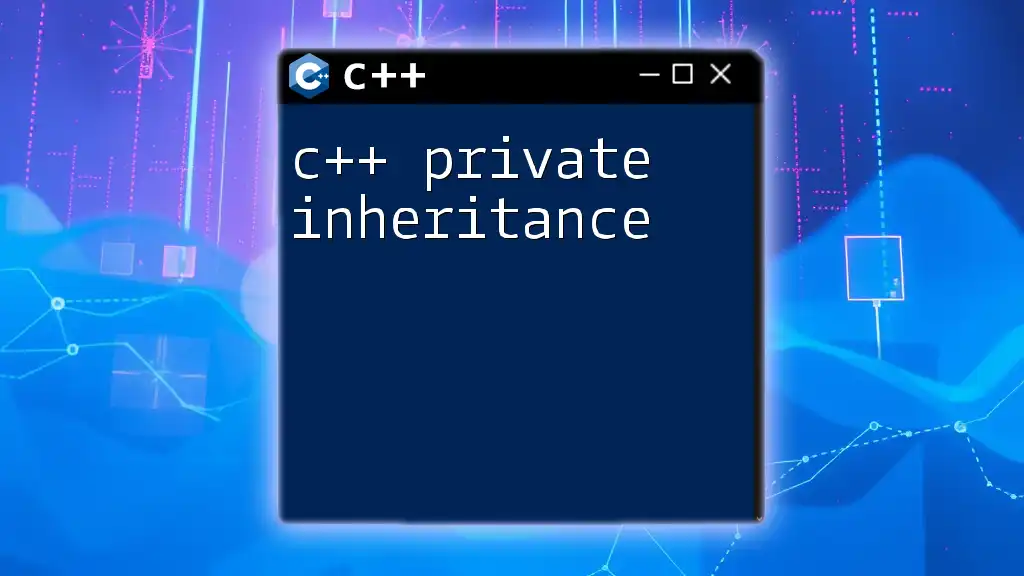
The Basics of C++ Class Inheritance
What is a C++ Inherited Class?
An inherited class (or derived class) is a class that derives properties and behaviors from another class (the base class). The relationship allows the derived class to access and use the attributes and methods defined in the base class.
Types of Inheritance
C++ supports various types of inheritance:
- Single Inheritance: A class inherits from one base class.
- Multiple Inheritance: A class inherits from more than one base class.
- Multilevel Inheritance: A derived class acts as a base class for another derived class.
- Hierarchical Inheritance: Multiple derived classes inherit from a single base class.
- Hybrid Inheritance: A combination of two or more types of inheritance.
Visual Representation of Inheritance
While diagrams will be added later, imagine a tree structure where the root is the base class, and the branches represent derived classes that inherit its attributes and methods.
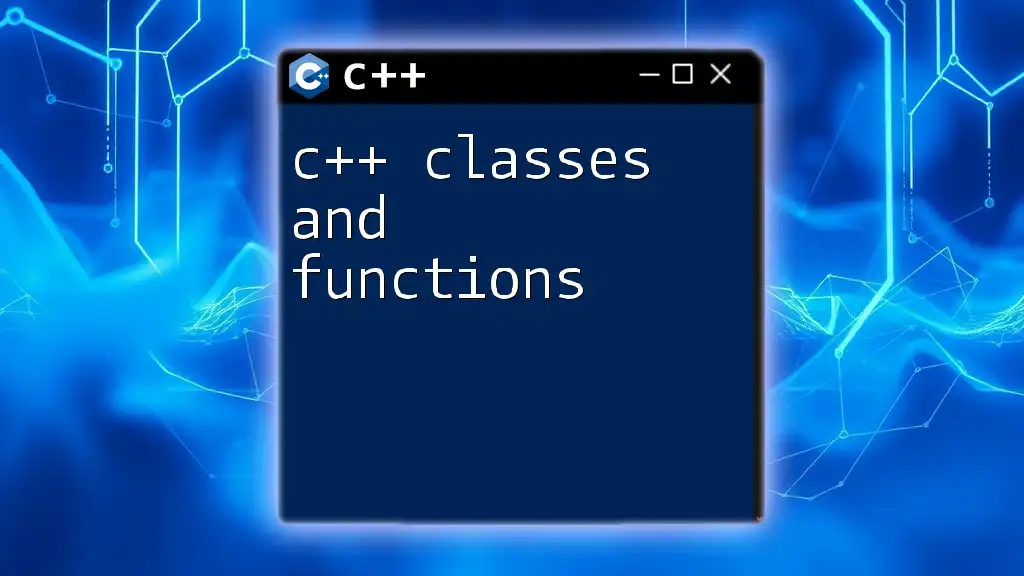
Creating C++ Classes
Defining a Basic Class
To create a C++ class, you need to define its properties and methods. Here’s a simple example of defining a `Car` class:
class Car {
public:
string brand;
void honk() {
cout << "Honk!" << endl;
}
};
In this snippet, the `Car` class encapsulates the property `brand` and the method `honk()`, which can be called to display a sound.
Encapsulation and Access Modifiers
C++ provides three access modifiers:
- Public: Members are accessible from outside the class.
- Protected: Members are accessible within the class and by derived classes.
- Private: Members are only accessible within the class itself, enforcing data hiding.
Using proper access modifiers is crucial for maintaining data integrity and encapsulation.
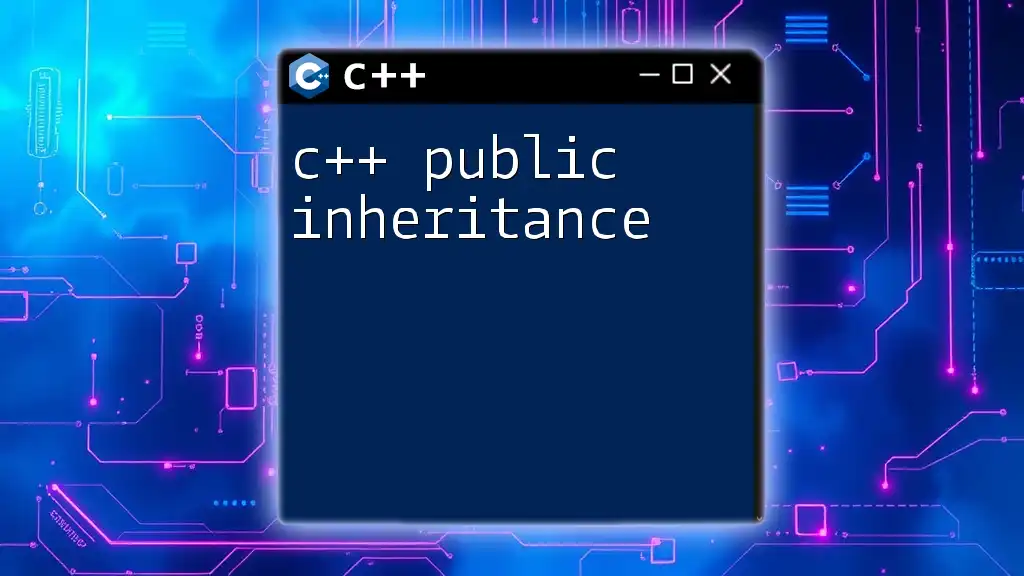
Implementing C++ Class Inheritance
Syntax for Inheriting a Class
To create an inherited class, you use a colon (:) followed by the access specifier and the name of the base class. Here’s the syntax illustrated:
class Vehicle {
public:
void start() {
cout << "Vehicle started" << endl;
}
};
class Car : public Vehicle {
public:
void honk() {
cout << "Car honks!" << endl;
}
};
In this example, the `Car` class inherits from the `Vehicle` class. This means `Car` now has access to the `start()` method defined in `Vehicle`.
Creating an Inherited Class Example
Let’s expand on the previous example by demonstrating how an inherited class can utilize the base class's methods.
int main() {
Car myCar;
myCar.start(); // Calls base class method
myCar.honk(); // Calls derived class method
return 0;
}
In this code, the `Car` object `myCar` can call both `start()` (from `Vehicle`) and `honk()` (from `Car`), demonstrating inheritance in action.
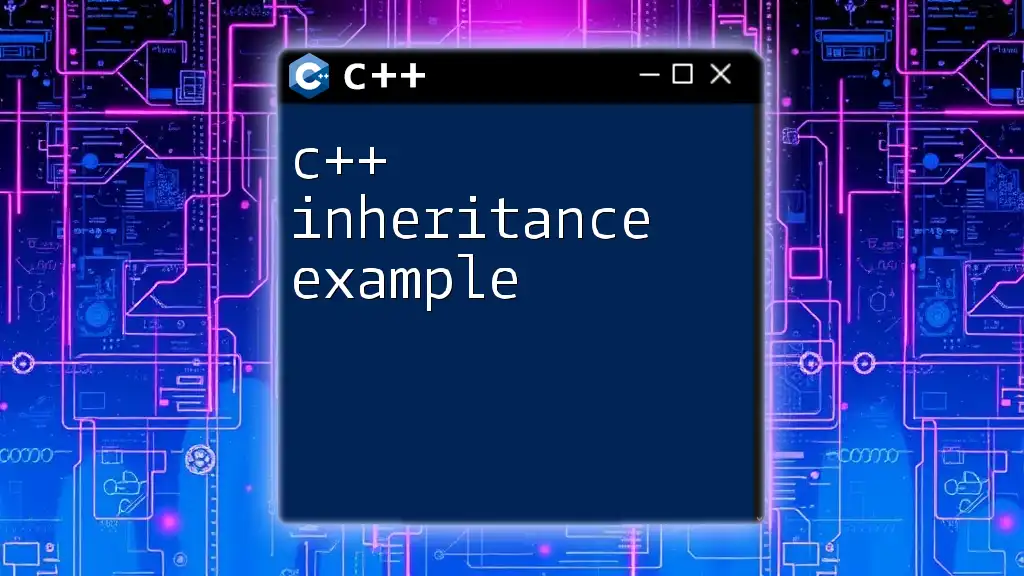
Advantages of C++ Class Inheritance
Code Reusability
Inheritance allows for the creation of new classes by reusing existing code. Instead of rewriting common functionality, developers can create subclasses that extend or modify behavior.
Logical Structure
By establishing a class hierarchy, inheritance promotes a clearer organization of code. This mirrors real-world relationships, making it easier for developers to manage and debug their code.
Polymorphism
Inheritance facilitates polymorphism, enabling the same function to behave differently based on the object that calls it. This is crucial for implementing flexible and reusable code structures.
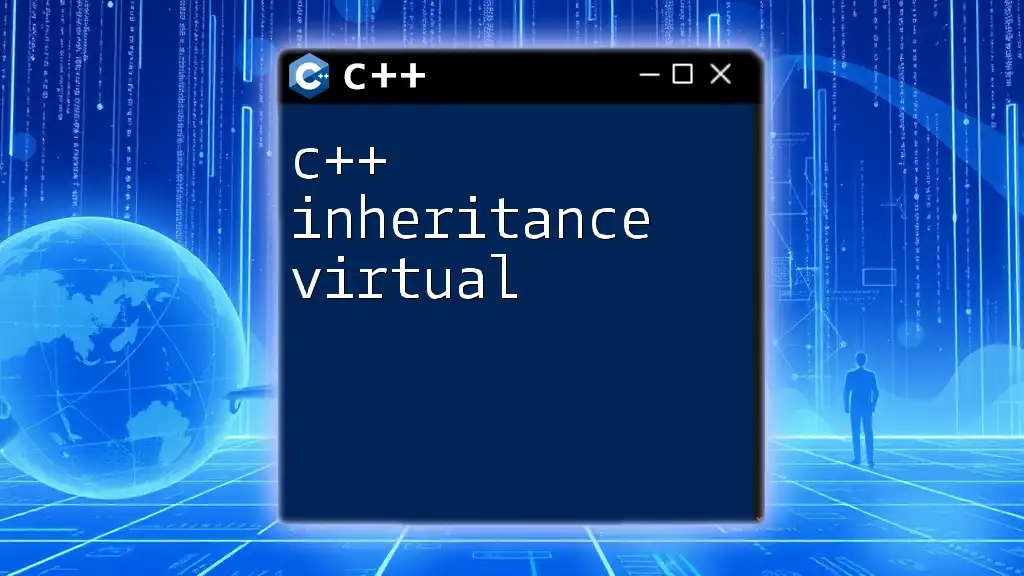
Virtual Functions and Polymorphism
Introduction to Virtual Functions
Virtual functions enable derived classes to override methods of base classes while preserving a consistent interface. This allows function calls to be resolved at runtime based on the object type.
Creating a Virtual Function Example
Here's how you can use virtual functions in a class hierarchy:
class Animal {
public:
virtual void sound() {
cout << "Animal sound" << endl;
}
};
class Dog : public Animal {
public:
void sound() override {
cout << "Bark!" << endl;
}
};
Using Base Class Pointer to Call Derived Class Method
When utilizing a base class pointer to refer to a derived class object, polymorphism shines:
int main() {
Animal* myDog = new Dog();
myDog->sound(); // Outputs: Bark!
delete myDog; // Clean up memory
return 0;
}
In this case, even though `myDog` is a pointer of type `Animal`, it calls the `sound()` method defined in the `Dog` class because of the virtual mechanism.
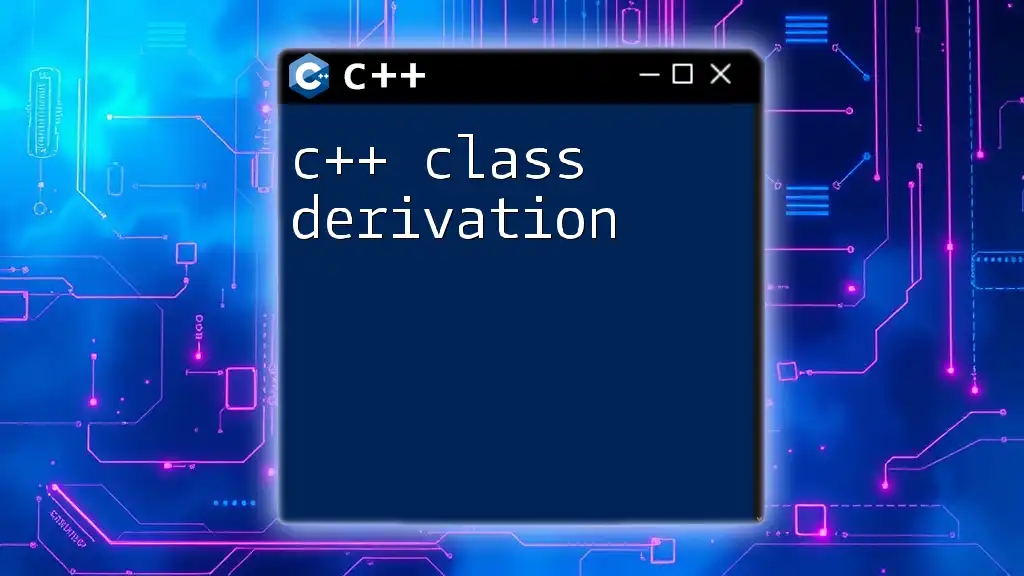
Common Mistakes to Avoid with C++ Class Inheritance
Careful with the Base Class Destructor
Always declare base class destructors as virtual. Failing to do so can lead to resource leaks and undefined behavior, especially when using pointers of the base class type.
Ambiguity in Multiple Inheritance
Be cautious of the Diamond Problem, where two base classes inherit from the same parent class. This can lead to ambiguity about which base class's method should be called. Use virtual inheritance to resolve such issues.
Overriding vs. Hiding Methods
Understand the difference between overwriting (where a derived class method replaces a base class method) and hiding (where a derived class declares a method with the same name as a base class method). Use the `override` keyword to clarify your intentions in your code.
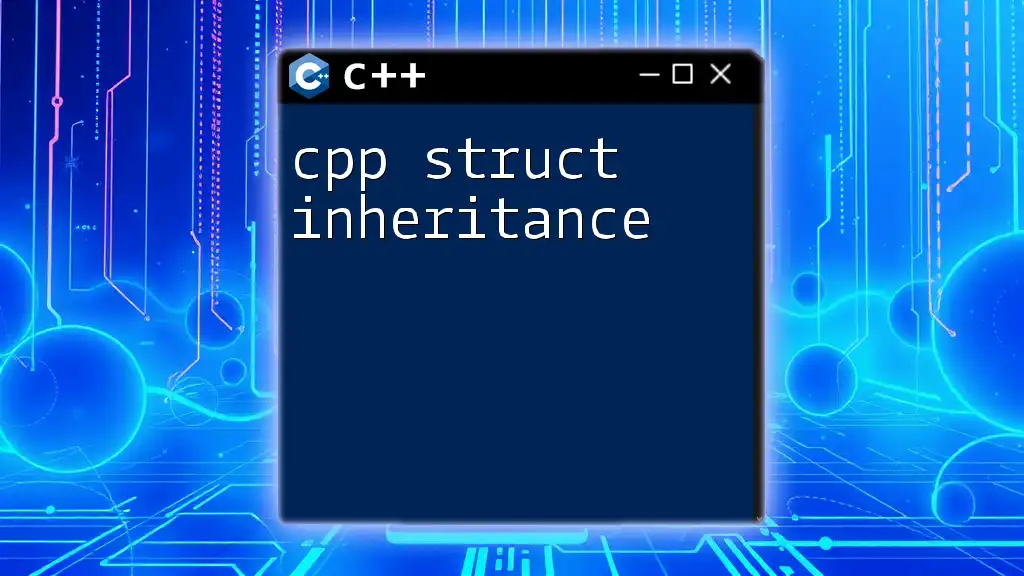
Conclusion
In this exploration of C++ classes and inheritance, we've unpacked the vital concepts of class definition, inheritance types, advantages, and best practices. Mastering these topics lays a solid foundation for effective and organized coding in C++.
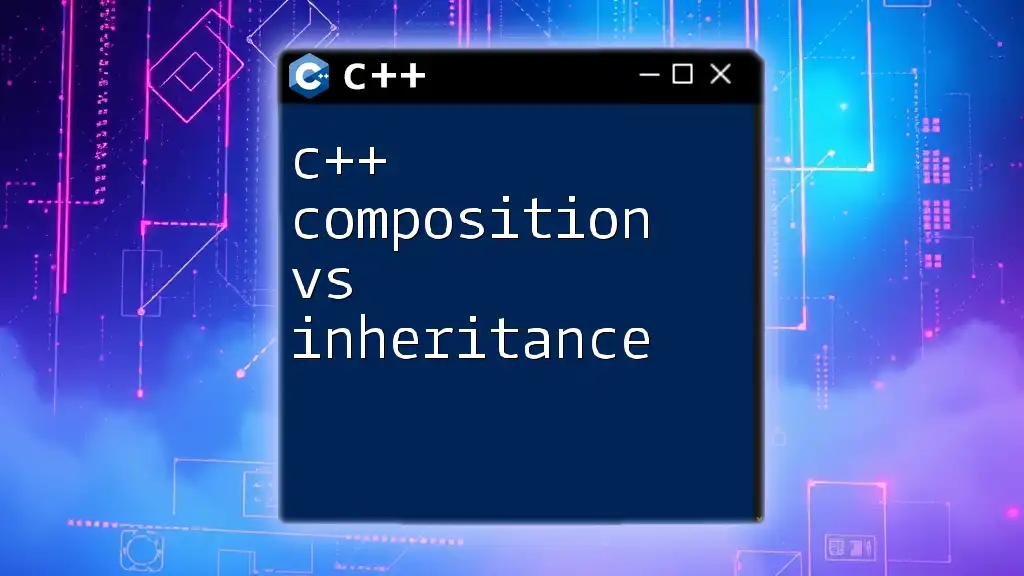
Additional Resources
For those eager to dive deeper, consider exploring books on object-oriented programming or online courses that provide practical exercises and coding challenges. Engaging with developer communities can also enhance your understanding and application of C++.
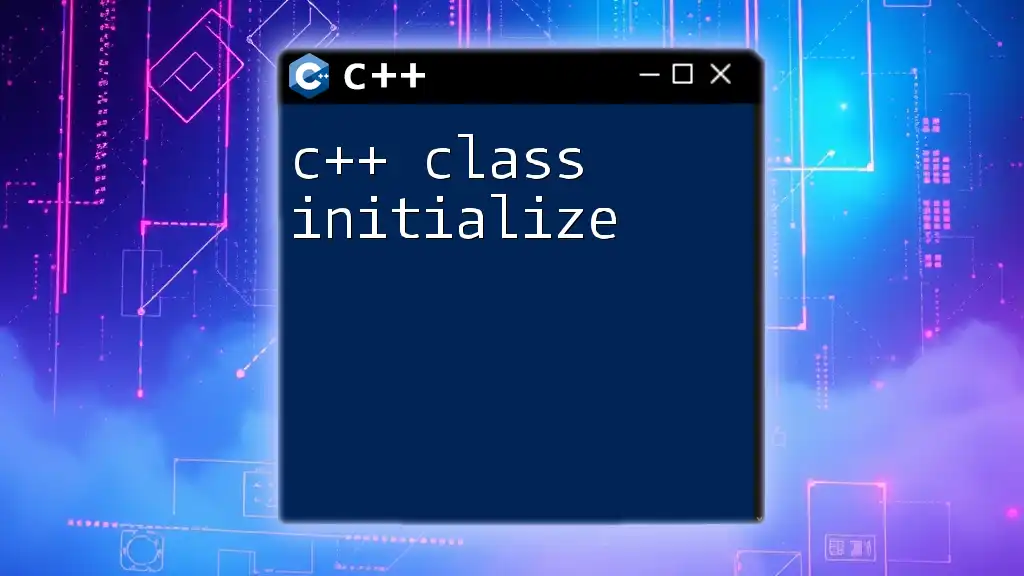
Call to Action
Join our community for more enlightening tutorials, practical examples, and continuous learning resources that will help elevate your programming skills to the next level!