C++ class derivation allows a new class (derived class) to inherit properties and behaviors from an existing class (base class), enabling code reusability and hierarchy.
class Base {
public:
void display() {
std::cout << "This is the base class." << std::endl;
}
};
class Derived : public Base {
public:
void show() {
std::cout << "This is the derived class." << std::endl;
}
};
int main() {
Derived obj;
obj.display(); // Inherits from Base
obj.show(); // Unique to Derived
return 0;
}
Understanding Classes in C++
What is a Class?
A class in C++ serves as a blueprint for creating objects. It encapsulates data for the object and methods that operate on that data. In C++, a class consists of two primary components: attributes and methods. Attributes (or member variables) hold the state of an object, while methods (or member functions) define the behavior.
For example, consider a basic class structure for an `Animal`:
class Animal {
public:
void makeSound() {
cout << "Some sound..." << endl;
}
};
In this example, `Animal` is a class with a method `makeSound()`, illustrating a simple concept of encapsulation.
Properties of Classes
Classes have several key properties:
- Attributes: Variables defined within the class that represent the object's state.
- Methods: Functions defined within the class that represent the object's behavior.
For example, we can enhance the `Animal` class by adding an attribute to store the animal's name:
class Animal {
public:
string name;
void makeSound() {
cout << "Some sound..." << endl;
}
};
This addition allows each `Animal` object to have a unique name.
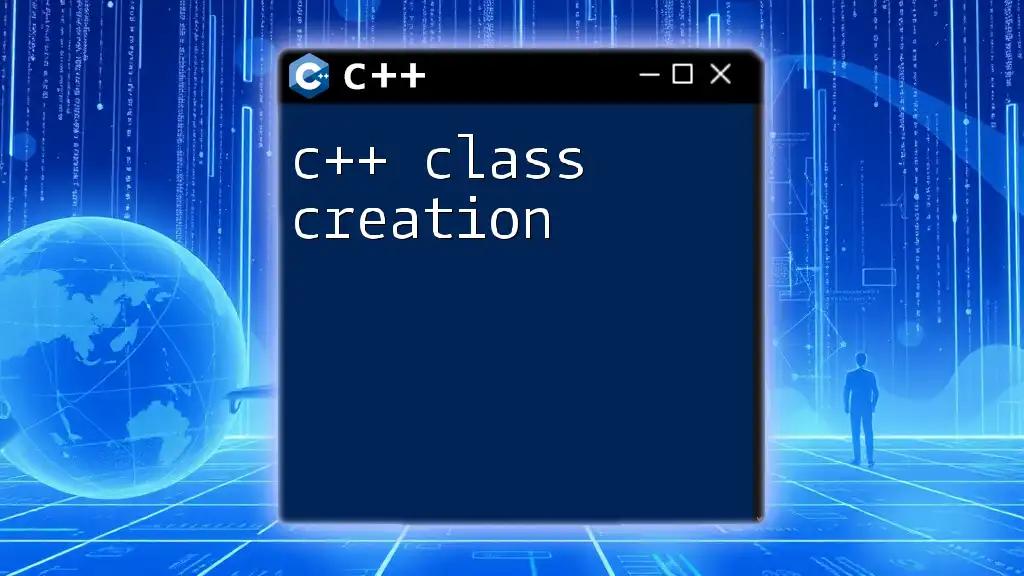
The Concept of Inheritance
What is Inheritance?
Inheritance is a fundamental concept in object-oriented programming (OOP) that allows a class (the derived class) to inherit properties and behaviors from another class (the base class). This establishes an "is-a" relationship and promotes code reusability and scalability.
Types of Inheritance
Single Inheritance
Single inheritance allows a derived class to inherit from a single base class. This is the simplest form of inheritance.
For example:
class Dog : public Animal {
public:
void makeSound() {
cout << "Bark!" << endl;
}
};
In this case, `Dog` derives from `Animal` and can use its method while also overriding the `makeSound` method to provide specific behavior for dogs.
Multiple Inheritance
Multiple inheritance enables a derived class to inherit from multiple base classes. While this increases flexibility, it can also lead to complexity and ambiguity—particularly the Diamond Problem.
Example of multiple inheritance:
class Canine {
// Canine specific properties
};
class Dog : public Animal, public Canine {
// Dog specific properties
};
It’s crucial to manage the complexities through appropriate design choices.
Multilevel Inheritance
In multilevel inheritance, a class derives from another derived class. This creates a chain of inheritance.
For example:
class Puppy : public Dog {
// Puppy specific properties
};
Here, `Puppy` inherits from `Dog`, which in turn inherits from `Animal`, forming a hierarchy.
Hierarchical Inheritance
Hierarchical inheritance occurs when multiple derived classes inherit from a single base class.
Example:
class Cat : public Animal {
// Cat specific properties
};
class Lion : public Animal {
// Lion specific properties
};
Both `Cat` and `Lion` inherit from the `Animal` class, showcasing the versatility of inheritance.
Hybrid Inheritance
Hybrid inheritance is a combination of two or more types of inheritance. This can be powerful but requires careful design to avoid complications.
Example illustrating hybrid inheritance can involve both multiple and multilevel inheritance scenarios.

Access Specifiers in Inheritance
Introduction to Access Specifiers
Access specifiers determine how the members of a class can be accessed. The three primary access specifiers in C++ are public, protected, and private.
How Access Specifiers Affect Inheritance
Public Inheritance
Public inheritance allows the public and protected members of the base class to remain accessible in the derived class.
class Base {
public:
int baseValue;
};
class Derived : public Base {
// baseValue can be accessed here
};
Here, `baseValue` can be accessed in `Derived` because of the public inheritance relationship.
Protected Inheritance
Protected inheritance restricts the access level of the base class members. The public members become protected in the derived class.
class Derived : protected Base {
// baseValue cannot be accessed directly
};
The members of `Base` are only accessible within `Derived` and classes derived from `Derived`.
Private Inheritance
Private inheritance means that all members of the base class become private in the derived class, and they cannot be accessed outside of the derived class.
class Derived : private Base {
// baseValue cannot be accessed outside Derived
};
This level of access restriction is typically used in implementation details.
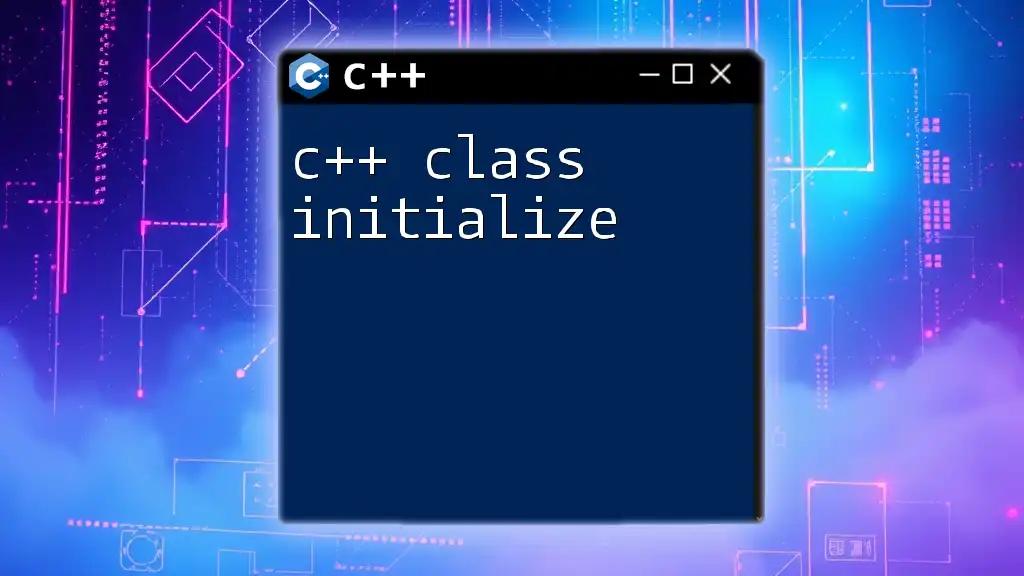
Function Overriding and Virtual Functions
What is Function Overriding?
Function overriding allows a derived class to provide a specific implementation of a function that is already defined in its base class. This flexibility is essential in allowing polymorphic behavior.
Using Virtual Functions
In order to perform function overriding, the method in the base class must be declared as `virtual`. This signals to the compiler that you intend to override the function in derived classes.
Here’s an example:
class Animal {
public:
virtual void makeSound() {
cout << "Some sound..." << endl;
}
};
class Dog : public Animal {
public:
void makeSound() override {
cout << "Bark!" << endl;
}
};
In this case, when `makeSound()` is called on a `Dog` object, it outputs "Bark!" instead of "Some sound...", demonstrating polymorphism.
The Role of the `override` Keyword
The `override` keyword in C++11 (and later) enhances code readability and safety. It indicates that a method is meant to override a base class method, providing a safeguard against accidental mistakes such as signature mismatches.
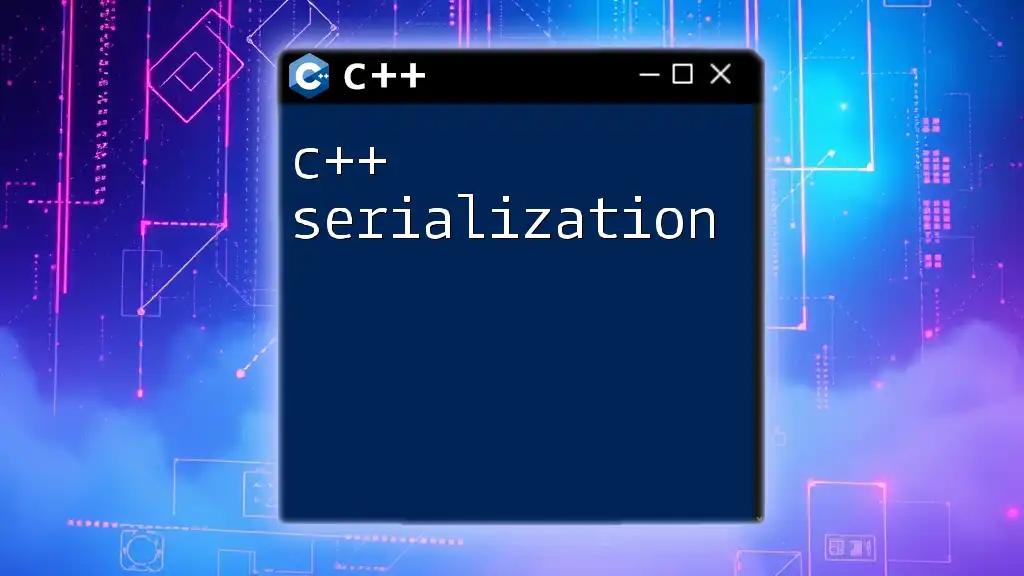
Abstract Classes and Interfaces
What are Abstract Classes?
An abstract class is a class that cannot be instantiated. Instead, it is used to define interfaces through pure virtual functions.
For example:
class Shape {
public:
virtual void draw() = 0; // Pure virtual function
};
In this case, `Shape` cannot be instantiated directly but must be derived from concrete classes that implement the `draw()` method.
Interfaces in C++
C++ does not have explicit interfaces but can achieve similar functionality by using abstract classes with only pure virtual functions. This allows you to define a contract that derived classes must implement.
Example:
class Drawable {
public:
virtual void draw() = 0; // Pure virtual function
};
class Circle : public Drawable {
public:
void draw() {
cout << "Drawing Circle." << endl;
}
};
In this code, `Circle` implements the `draw()` method, satisfying the contract laid out by the `Drawable` interface.
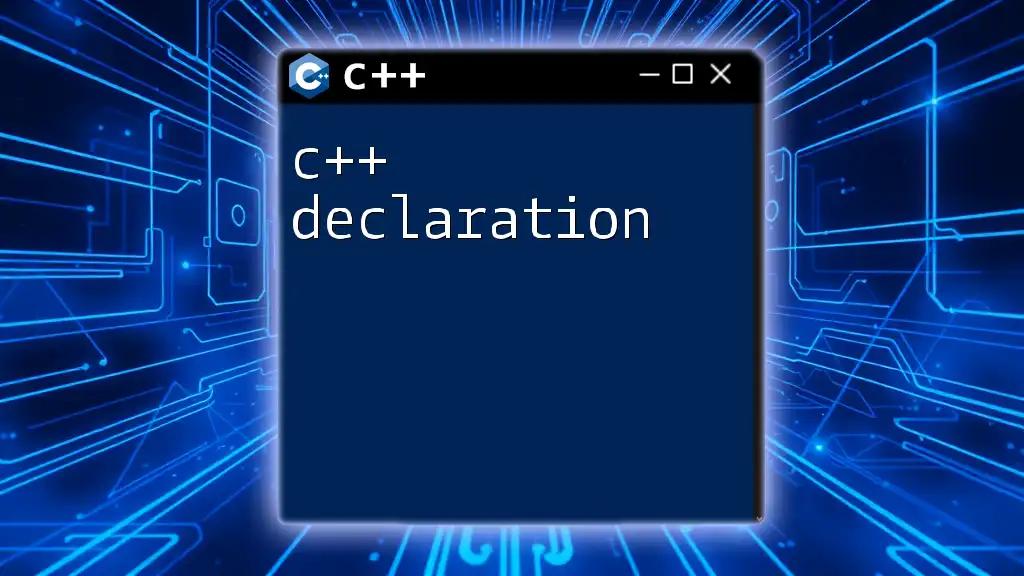
Conclusion
Understanding C++ class derivation is crucial for effective object-oriented programming. It provides powerful tools for modeling real-world relationships through inheritance, allows for code reusability, and promotes a more organized code structure. By mastering the concepts of class inheritance, access specifiers, function overriding, and abstract classes, programmers can unlock the full potential of C++ in their applications. With practice, these concepts will become second nature, enhancing the capability to design robust and scalable software solutions.