In C++, a class is a user-defined data type that allows you to bundle data and functions together, providing a blueprint for creating objects.
class MyClass {
public:
int myNumber;
void display() {
std::cout << "Number: " << myNumber << std::endl;
}
};
Understanding C++ Classes
What is a Class in C++?
A class in C++ is essentially a blueprint for creating objects. It encapsulates data and functions that operate on that data, allowing programmers to model real-world entities programmatically. For example, consider a `Car` class that has properties like `make` and `model`, as well as methods to `start()` and `stop()`. In this analogy, the `Car` class represents all cars, while each specific car (like a Toyota Camry) is an object created from this class.
Purpose of Using Classes
Classes serve numerous purposes in C++ programming, with three of the most defining principles being encapsulation, inheritance, and polymorphism:
-
Encapsulation allows you to bundle the data (attributes) and methods (functions) that operate on the data into a single unit or class. This encapsulation protects the data from outside interference and misuse.
-
Inheritance enables new classes to adopt the properties and behaviors of existing classes, creating a hierarchical relationship that promotes code reuse and organization.
-
Polymorphism allows methods to do different things based on the object invoking them. This flexibility is essential for building scalable applications.
By understanding these principles, you can create more organized, efficient, and readable code.
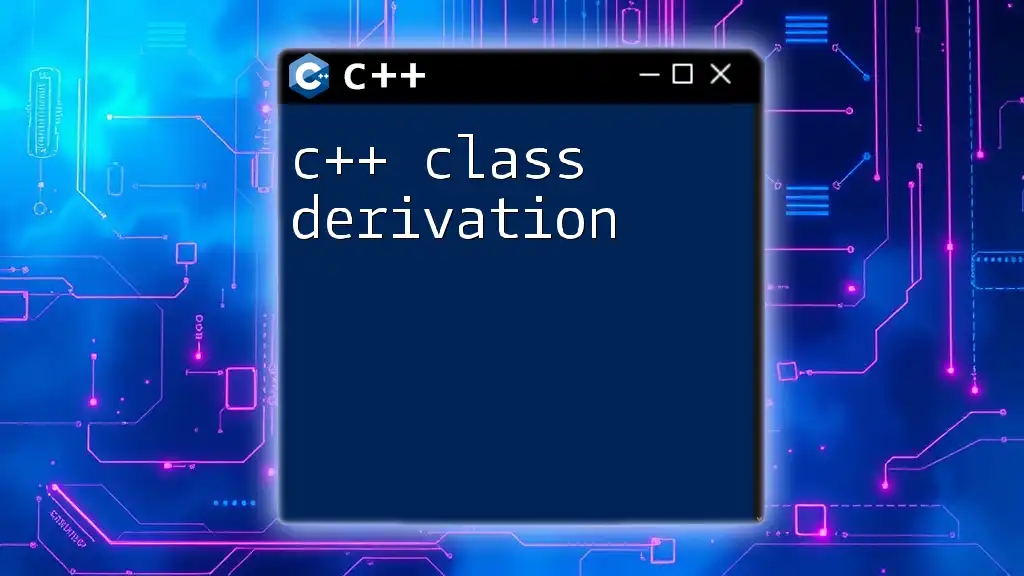
How to Create a Class in C++
Basic Structure of a Class
Creating a class in C++ involves defining a new type that can encapsulate data and functionality. The basic structure is as follows:
class ClassName {
public:
// Member variables
// Member functions
};
This structure consists of:
- The class keyword, which defines a class.
- The class name, which should be unique within its scope.
- Access specifiers (like `public`, `private`, etc.) that control the visibility of class members.
Step-by-Step Process to Create a Class
1. Defining the Class
To define a class, you start with the class keyword followed by the name of the class. Here's an example:
class Dog {
public:
// Members will be defined here
};
2. Adding Member Variables
Member variables represent the state or attributes of a class. Below, we extend the `Dog` class to include name and age as member variables:
class Dog {
public:
std::string name; // Member variable for dog name
int age; // Member variable for dog age
};
3. Creating Member Functions
Member functions define behaviors associated with a class. For example, let’s add a `bark` function to our `Dog` class:
class Dog {
public:
std::string name;
int age;
void bark() {
std::cout << "Woof! My name is " << name << std::endl;
}
};
This function can be called on any `Dog` object to produce a simple output.
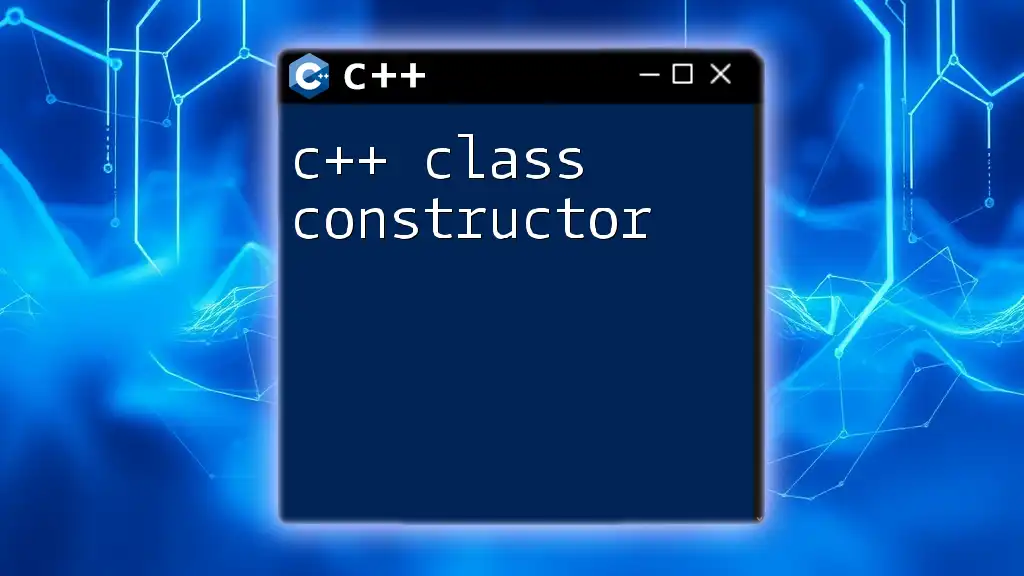
How to Make a Class in C++ with Constructors
What are Constructors?
Constructors are special member functions invoked when an object of the class is created. They are used to initialize member variables. Constructors can be overloaded, allowing multiple ways to create an instance of a class.
Creating Constructors
Here’s how you can include a constructor in your `Dog` class:
class Dog {
public:
std::string name;
int age;
Dog(std::string dogName, int dogAge) {
name = dogName; // Initialize name
age = dogAge; // Initialize age
}
};
Example Usage
Now, to instantiate the `Dog` class and utilize its constructor:
Dog myDog("Buddy", 3);
myDog.bark(); // Output: Woof! My name is Buddy
This example shows how we can create a `Dog` object named "Buddy" with the age of 3.
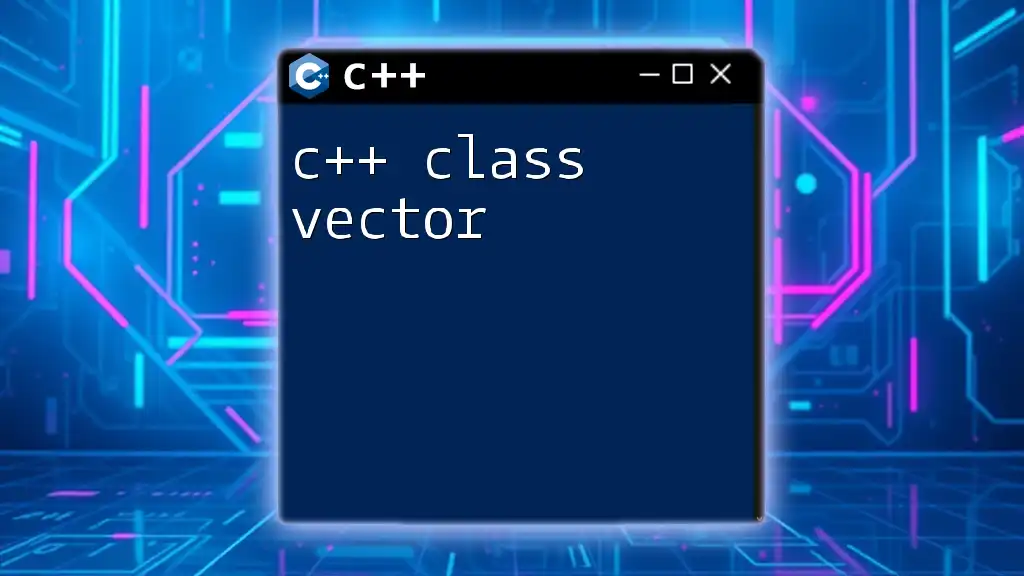
How to Make a Class in C++ with Destructors
Understanding Destructors
Destructors are invoked automatically when an object goes out of scope or is deleted. They are primarily used for cleanup duties, like releasing resources or memory that the object may have acquired during its lifetime.
Creating Destructors
Here’s how you can add a destructor to the `Dog` class:
class Dog {
public:
std::string name;
int age;
~Dog() { // Destructor
std::cout << "Destructor called for " << name << std::endl;
}
};
Example of Using Destructors
Simply declaring an object will invoke the destructor when it goes out of scope:
{
Dog myDog("Buddy", 3);
} // Destructor called for Buddy
In this situation, when the `myDog` object goes out of scope, the destructor message is printed automatically.
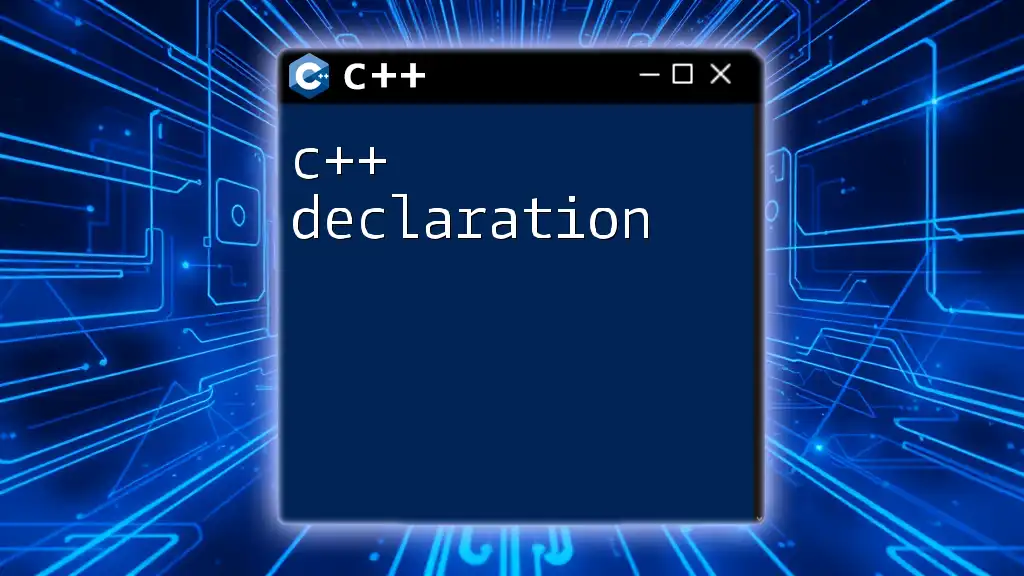
How to Create a Class in C++ with Inheritance
What is Inheritance?
Inheritance allows a new class, known as a derived class, to inherit properties and methods of an existing class, known as the base class. This mechanism fosters code reuse and enhances organization in complex systems.
Creating Base and Derived Classes
Let's create a base `Animal` class and derive a `Dog` class from it:
class Animal {
public:
void eat() {
std::cout << "Animal eats" << std::endl;
}
};
class Dog : public Animal {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
Example of Using Inheritance
With this setup, a `Dog` object can call methods from both `Dog` and `Animal`:
Dog myDog;
myDog.eat(); // Output: Animal eats
myDog.bark(); // Output: Woof!
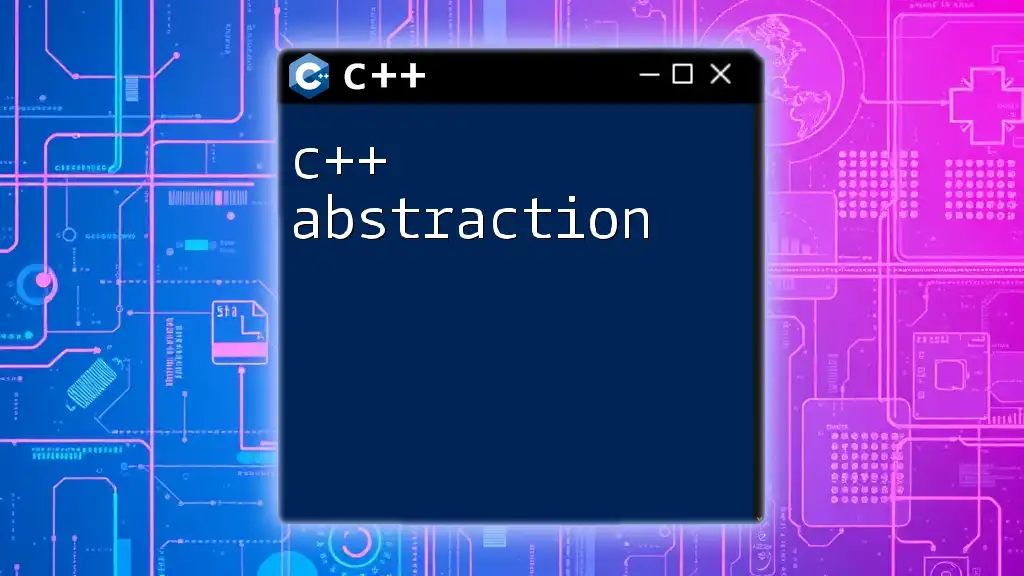
Conclusion
Mastering C++ class creation is fundamental for anyone wishing to delve into object-oriented programming with C++. By leveraging classes, you enable encapsulation, inheritance, and polymorphism, creating systems that are modular and maintainable. The examples provided illustrate how to define classes, constructors, destructors, and inheritance in a practical manner, setting you up for more complex programming challenges ahead.
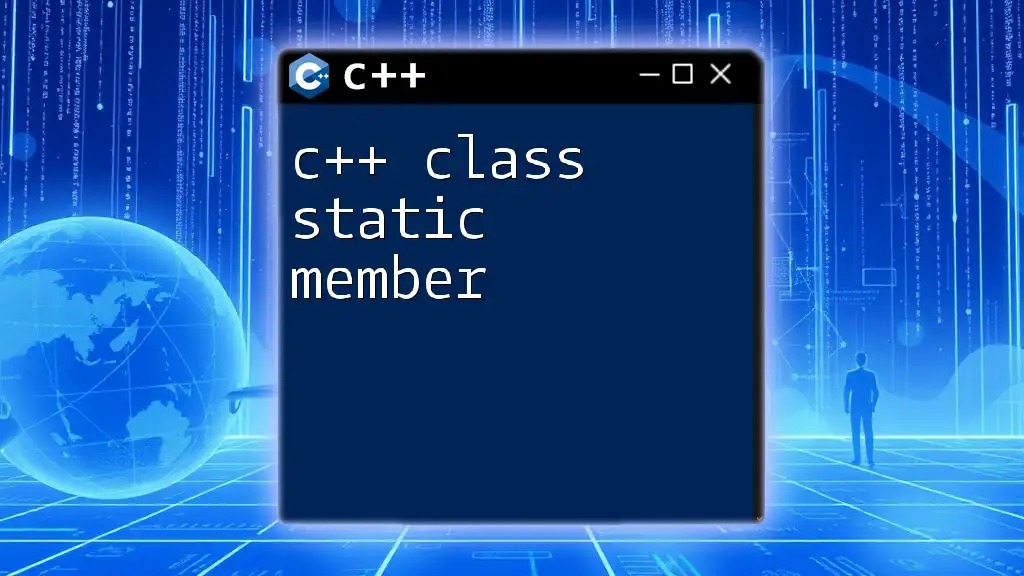
Additional Resources
For further enrichment, consider exploring C++ documentation, online tutorials, or joining programming communities where enthusiasts share insights and solutions.
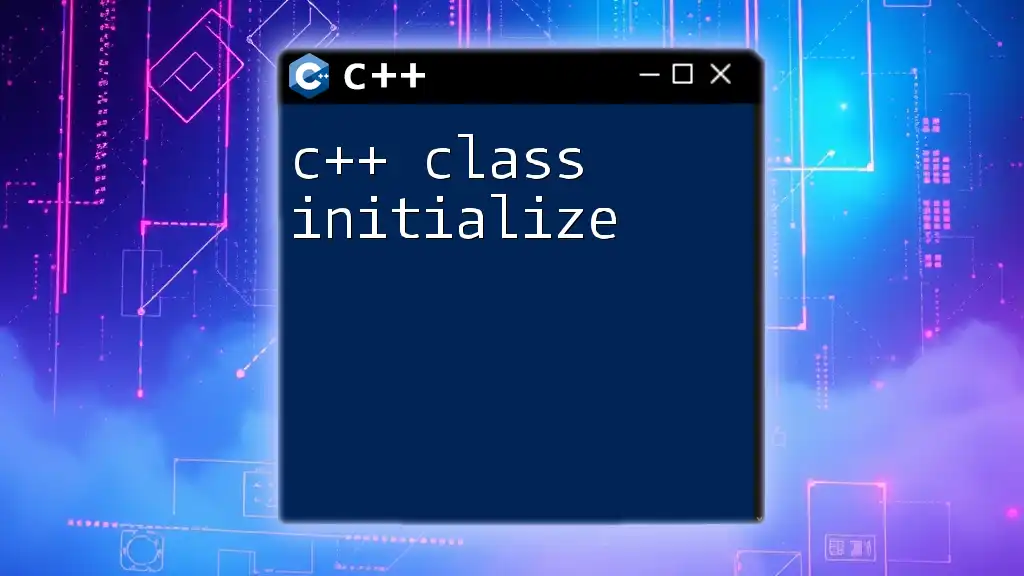
Final Thoughts
Practicing the creation of your own classes and experimenting with the principles outlined in this article will solidify your understanding. Share your experiences in the comments, and stay tuned for more advanced topics in C++ that can elevate your programming skills!