C++ collections refer to data structures such as arrays, vectors, lists, and maps that allow you to store and manage groups of related data efficiently.
#include <iostream>
#include <vector>
#include <map>
int main() {
// Using a vector to store a collection of integers
std::vector<int> numbers = {1, 2, 3, 4, 5};
// Using a map to associate string keys with integer values
std::map<std::string, int> ageMap = {{"Alice", 30}, {"Bob", 25}};
std::cout << "Numbers: ";
for (int num : numbers) {
std::cout << num << " ";
}
std::cout << "\nAges: ";
for (const auto& pair : ageMap) {
std::cout << pair.first << ": " << pair.second << " ";
}
return 0;
}
Understanding C++ STL Containers
C++ collections primarily revolve around STL containers, which are a fundamental aspect of C++ that allow programmers to handle and store data efficiently. Each container is suited for different scenarios and is defined by its own unique characteristics, performance implications, and functionalities.
Types of C++ STL Containers
Sequence Containers maintain the order of elements, allowing for dynamic resizing and element access.
Vectors
Vectors are dynamic arrays that can adjust their size automatically when elements are added or removed. They are stored in contiguous memory, which gives them an advantage in terms of pointer arithmetic and memory caching.
Code Example: Using Vectors
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
for (const auto& value : vec) {
std::cout << value << " ";
}
return 0;
}
Pros and Cons of Vectors
- Pros: Fast random access and efficient memory usage for small to medium-sized datasets.
- Cons: Slower when adding or removing elements at the beginning or in the middle due to shifting elements.
Lists
Lists are doubly linked lists, which means each element points to both the next and the previous element. This structure allows for efficient insertions and deletions from any part of the list.
Code Example: Using Lists
#include <iostream>
#include <list>
int main() {
std::list<std::string> lst = {"Apple", "Banana", "Cherry"};
for (const auto& fruit : lst) {
std::cout << fruit << " ";
}
return 0;
}
Pros and Cons of Lists
- Pros: Fast insertions and deletions at any position.
- Cons: No direct access to elements (i.e., accessing the nth element requires iterating through the list).
Deques
Deques, or double-ended queues, allow for insertions and deletions at both ends. They are similar to vectors but with additional functionality.
Code Example: Using Deques
#include <iostream>
#include <deque>
int main() {
std::deque<int> deq = {10, 20, 30};
deq.push_front(5); // Adding element at the front
for (const auto& value : deq) {
std::cout << value << " ";
}
return 0;
}
Pros and Cons of Deques
- Pros: Efficient manipulation of both ends.
- Cons: Random access is slightly slower than vectors due to elements not being contiguous in memory.
Associative Containers store elements in a manner that allows for fast retrieval based on keys.
Sets
Sets are a type of associative container that stores unique elements in a specific order (usually sorted). They are particularly useful for applications that require uniqueness and fast search operations.
Code Example: Using Sets
#include <iostream>
#include <set>
int main() {
std::set<int> s = {1, 2, 3, 4};
s.insert(5);
for (const auto& num : s) {
std::cout << num << " ";
}
return 0;
}
Pros and Cons of Sets
- Pros: Automatically handles duplicates, providing fast access for unordered operations.
- Cons: Slower insertions and deletions compared to vectors and lists.
Maps
Maps are another associative container that stores elements in key-value pairs. Each key must be unique, and values can be accessed via their corresponding keys.
Code Example: Using Maps
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> scoreMap;
scoreMap["Alice"] = 90;
scoreMap["Bob"] = 85;
for (const auto& pair : scoreMap) {
std::cout << pair.first << ": " << pair.second << "\n";
}
return 0;
}
Pros and Cons of Maps
- Pros: Efficient lookup, insertion, and deletion of elements based on keys.
- Cons: Requires more memory than simpler containers like arrays due to key storage.
Multisets and Multimaps
Multisets allow duplicate elements, while multimaps enable duplicates for keys but maintain unique values. They extend the capabilities of sets and maps for specific use cases.
Unordered Containers
Unordered Containers do not maintain any specific order for their elements. They use hashing to ensure that retrieval is fast, generally offering average constant-time complexity.
Unordered Sets
Unordered sets efficiently store unique elements without any specific ordering, which helps in faster lookups.
Unordered Maps
Similar to unordered sets, unordered maps provide quick access to key-value pairs without maintaining order.
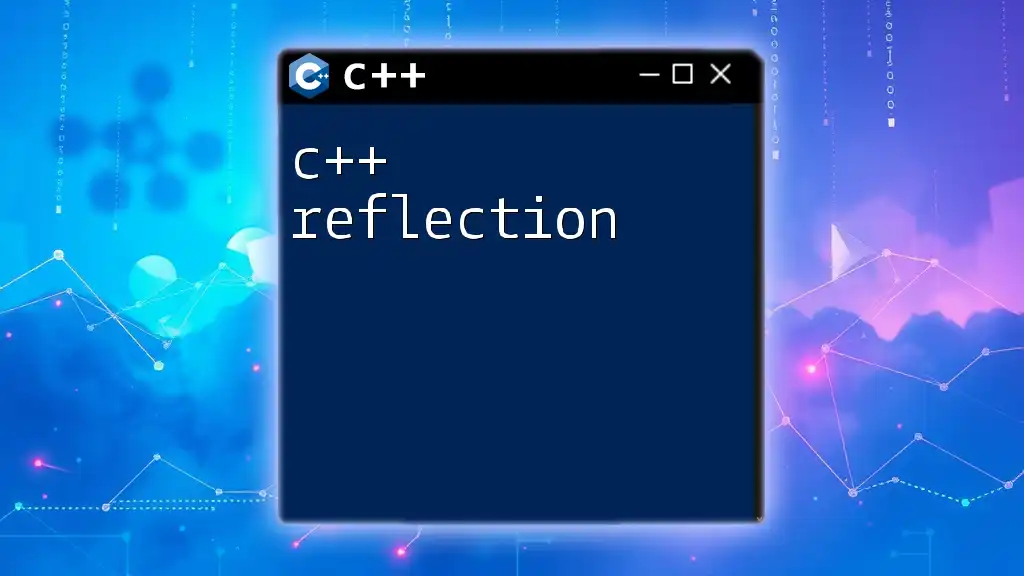
Choosing the Right Container
Selecting the appropriate container for your use case is crucial for optimizing performance and code efficiency. Key factors to consider include:
- Performance Implications: Evaluate how each container manages time complexity for insertions, deletions, and retrievals.
- Memory Usage: Different containers employ varying allocation strategies, impacting overall memory consumption.
- Algorithm Compatibility: Some algorithms may work more effectively with certain types of containers; understanding these relationships can save processing time.
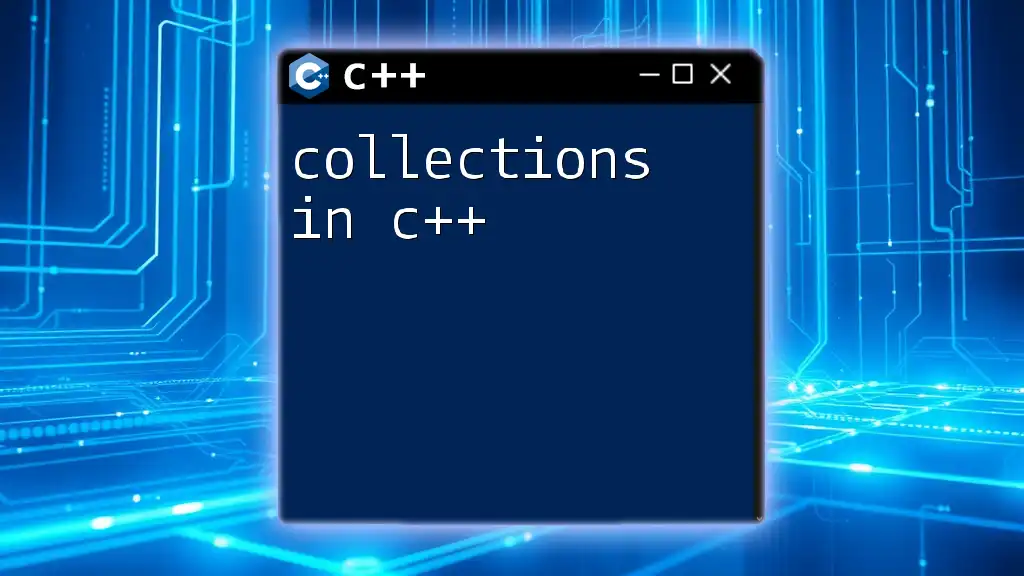
Best Practices for Using C++ STL Containers
To ensure maximum efficiency while working with C++ collections, consider the following best practices:
- Efficiency Tips: For vectors, utilize the `reserve()` method to allocate memory in advance, thus minimizing reallocations when the container grows.
- Code Readability and Maintainability: Use meaningful variable names, and keep your code clean and well-organized. Comments can greatly enhance clarity.
- Container-related Error Handling: Always check for boundary conditions and potential errors when manipulating containers, such as accessing elements out of range or modifying a container during iteration.
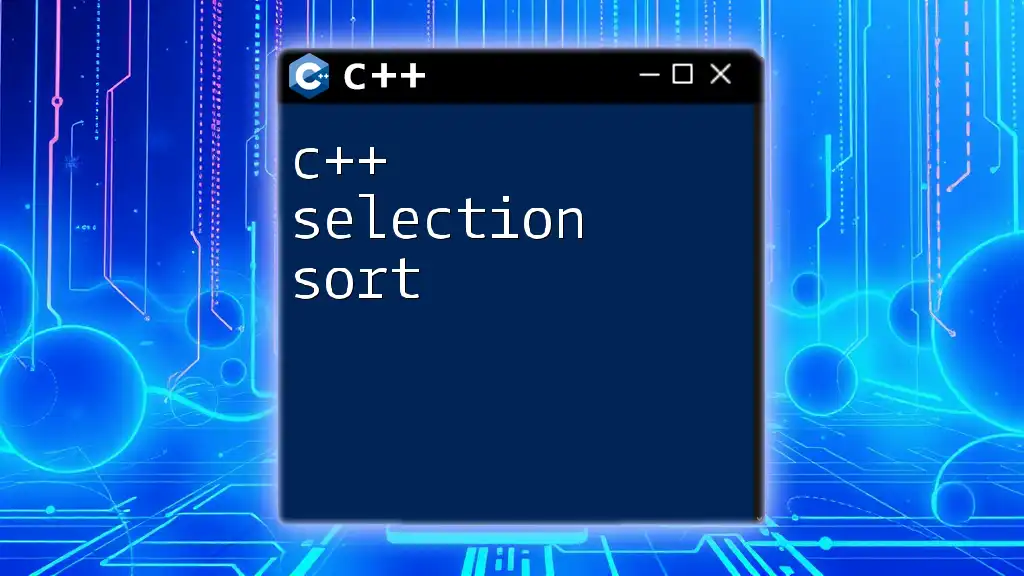
Conclusion
C++ collections, particularly through the use of STL containers, provide a robust framework for managing data in efficient and effective ways. By understanding the characteristics, advantages, and limitations of each container type, programmers can write optimized, maintainable code that meets the needs of their applications. Embrace the flexibility of these containers to enhance your programming endeavors and streamline data management in your C++ projects.
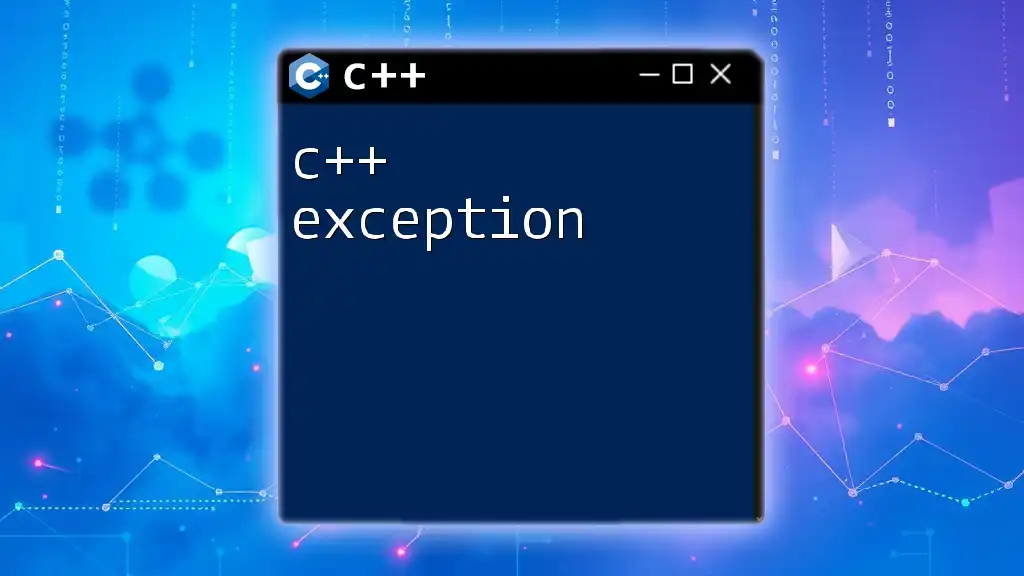
Additional Resources
- Look for books and online courses that delve deeper into C++ and STL containers.
- Refer to official documentation for up-to-date information and examples.
- Engage with community forums and Q&A sites where you can ask questions and share insights with fellow programmers.