In C++, the colon is used in several contexts, including member initializer lists in constructors and in inheritance to define base classes.
Here's an example demonstrating the use of the colon in a constructor's member initializer list and inheritance:
#include <iostream>
class Base {
public:
Base() {
std::cout << "Base Constructor" << std::endl;
}
};
class Derived : public Base {
public:
Derived() : Base() {
std::cout << "Derived Constructor" << std::endl;
}
};
int main() {
Derived d; // Output: Base Constructor followed by Derived Constructor
return 0;
}
What is the Colon in C++?
Definition of the Colon Operator
In C++, the colon is a versatile symbol that serves multiple purposes, contributing to the language's expressive power. It primarily acts as an operator or separator that interacts with various constructs within the language, from class definitions to namespace resolution.
Historical Context
The colon has been a staple in programming languages for decades. Understanding its legacy can help programmers appreciate its significance and versatility in modern C++.
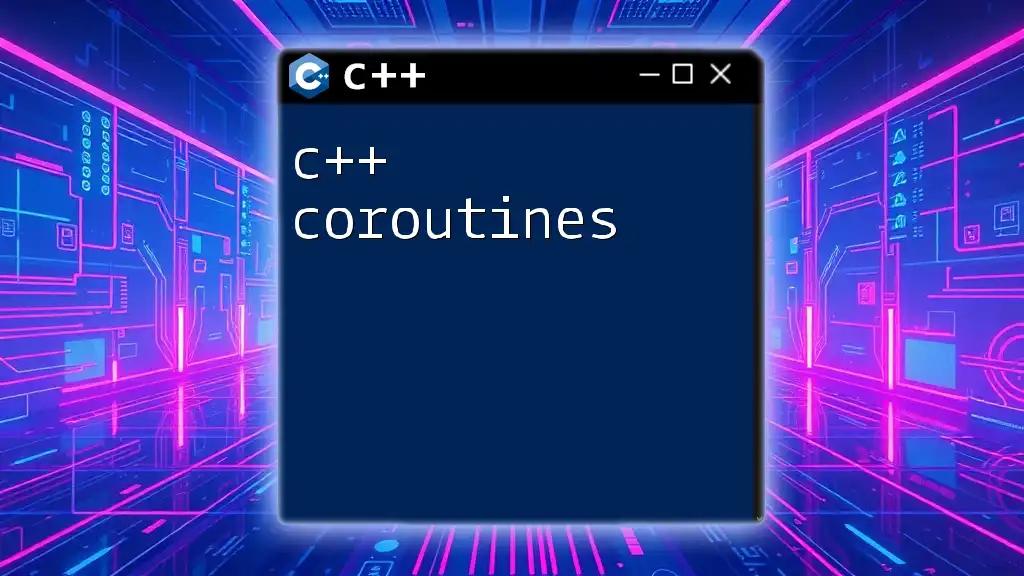
Uses of the Colon in C++
Namespace Resolution
One of the fundamental uses of the colon in C++ is for namespace resolution. This mechanism allows developers to define and use identifiers, like functions or variables, within specific namespaces to avoid naming conflicts.
Syntax: `namespace::functionName`
Example:
#include <iostream>
namespace MyNamespace {
void greet() {
std::cout << "Hello from MyNamespace!" << std::endl;
}
}
int main() {
MyNamespace::greet();
return 0;
}
In this example, the colon differentiates the `greet` function as part of `MyNamespace`, clarifying which function is being called and preventing any potential collisions with other identifiers in the global namespace.
Class Member Access
The colon is also crucial for accessing members of classes and structs. In C++, you use the colon to specify that you are referring to a member function or variable of a particular class.
Syntax: `ClassName::memberFunction`
Example:
class MyClass {
public:
void display() {
std::cout << "Display Function" << std::endl;
}
};
int main() {
MyClass obj;
obj.display(); // Correct usage: calling a member function
return 0;
}
Here, the colon would be used in a context requiring access to a specific member, allowing for clear association with the appropriate class.
Inheritance
In C++, the colon signifies inheritance, explicitly indicating the relationship between classes. This is vital for establishing the hierarchy of classes.
Syntax: `derivedClass : accessSpecifier baseClass`
Example:
class Base {
public:
void show() {
std::cout << "Base class function\n";
}
};
class Derived : public Base {
public:
void display() {
std::cout << "Derived class function\n";
}
};
int main() {
Derived obj;
obj.show();
obj.display();
return 0;
}
In this case, `Derived : public Base` shows that `Derived` inherits from `Base`, allowing it to access public members from the `Base` class.
Conditional Operator
It's easy to confuse the colon in a ternary operation with its different uses. Understanding this helps prevent mistakes in code evaluation.
Syntax: `condition ? expression1 : expression2`
Example:
int a = 10, b = 20;
int max = (a > b) ? a : b;
std::cout << "Maximum Value: " << max << std::endl;
In this instance, the colon is used in a conditional expression to determine which of `a` or `b` holds the maximum value, showcasing its role in decision-making processes.
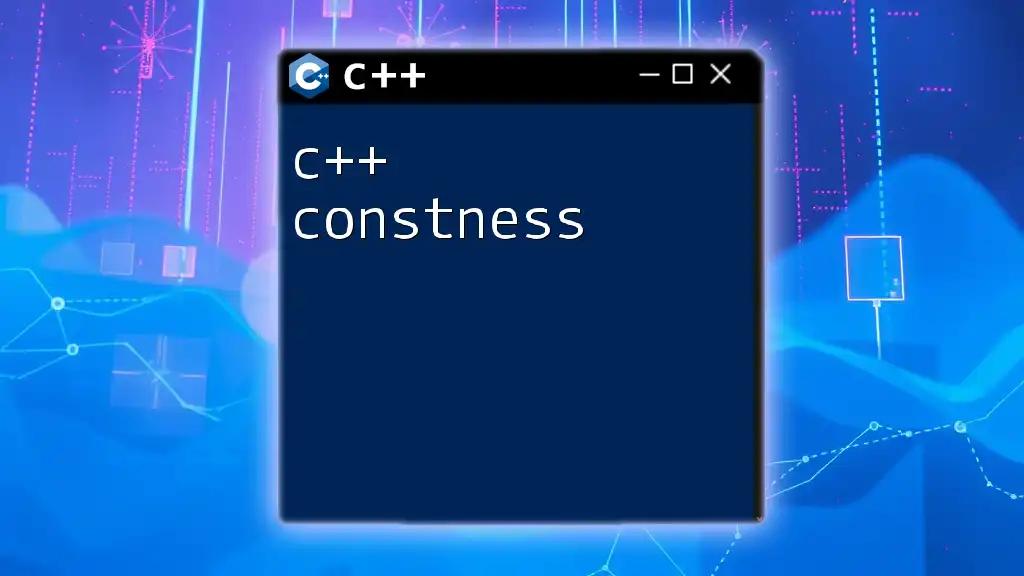
Special Cases of the Colon in C++
Member Initializer List
Another important use of the colon is in constructor definitions, specifically within member initializer lists. This syntactical structure allows for specific initialization of class member variables before the body of the constructor executes.
Syntax: `ConstructorName : member1(value1), member2(value2)`
Example:
class MyClass {
int x, y;
public:
MyClass(int a, int b) : x(a), y(b) {}
void display() {
std::cout << "X: " << x << ", Y: " << y << std::endl;
}
};
int main() {
MyClass obj(10, 20);
obj.display();
return 0;
}
Here, the colon acts as a bridge between constructor parameters and the member variables, allowing for efficient initialization without additional statements.
Using Colons in Enum Classes
In C++, the colon also appears in the context of scoped enumerations (enum classes). This feature enhances type safety and scope, preventing conflicts with identifiers.
Syntax: `enum class EnumName { value1, value2 };`
Example:
enum class Color { Red, Green, Blue };
Color color = Color::Red;
if (color == Color::Red) {
std::cout << "The color is Red." << std::endl;
}
In this case, the colon is used to access the enumerator `Red` within the scope of `Color`, helping ensure clarity and reducing errors from name clashes.
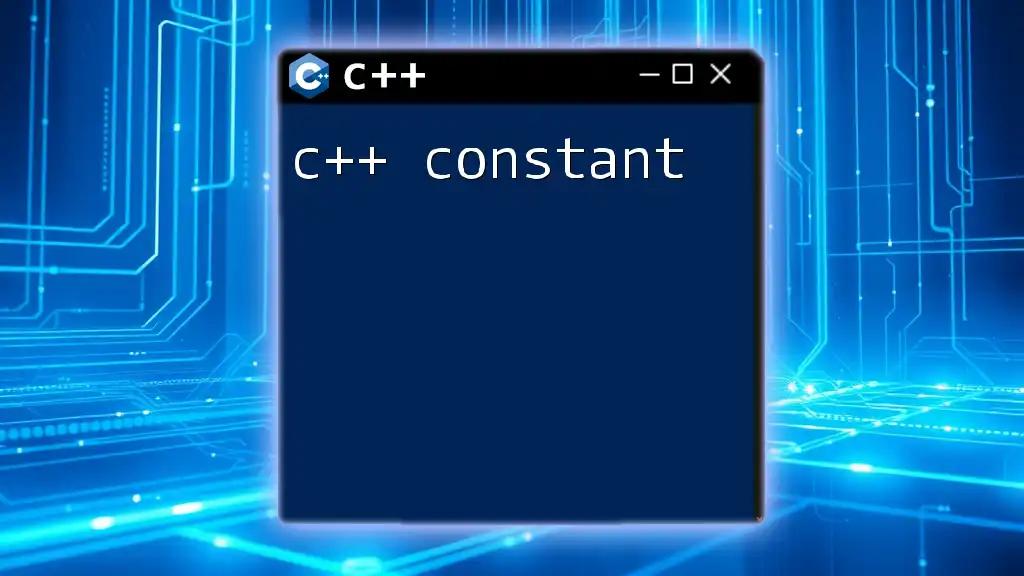
Common Mistakes with the C++ Colon
Despite its diverse uses, programmers often make mistakes when employing the colon, such as:
- Misusing scopes in class member access.
- Confusing the colon's purpose in different contexts, leading to runtime errors.
- Forgetting to define access specifiers during inheritance, which can cause unintended access issues.
By being mindful of these common pitfalls, programmers can enhance both the quality and functionality of their code.
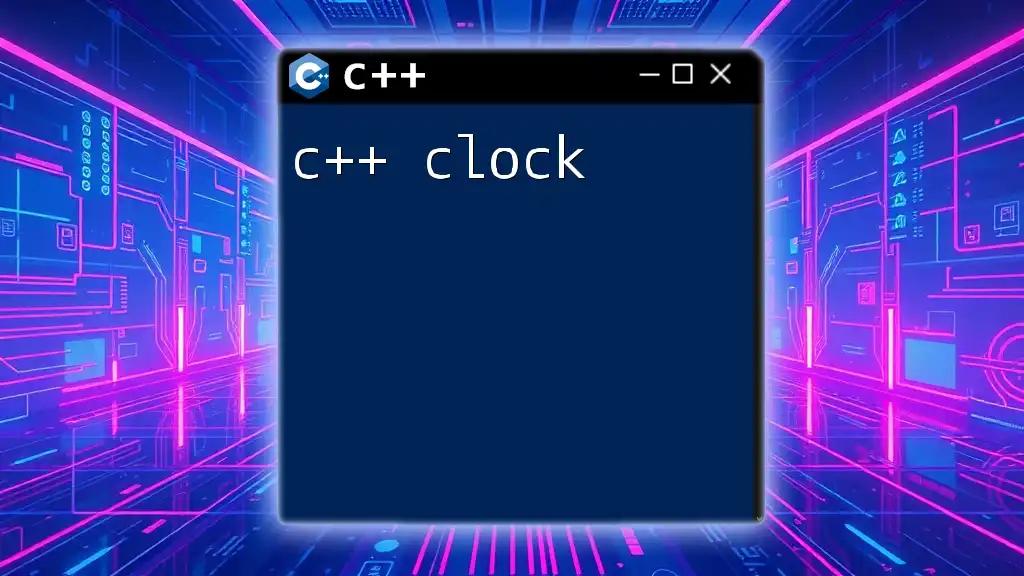
Best Practices for Using Colons in C++
To maximize the effectiveness of the colon in C++, consider the following best practices:
- Be clear and explicit: Always use the colon in context to clarify your intentions for member access or inheritance.
- Maintain readability: Utilize meaningful namespaces and class names to improve code comprehension.
- Consistency is key: Stick to standardized naming conventions to avoid confusion with similar identifiers.
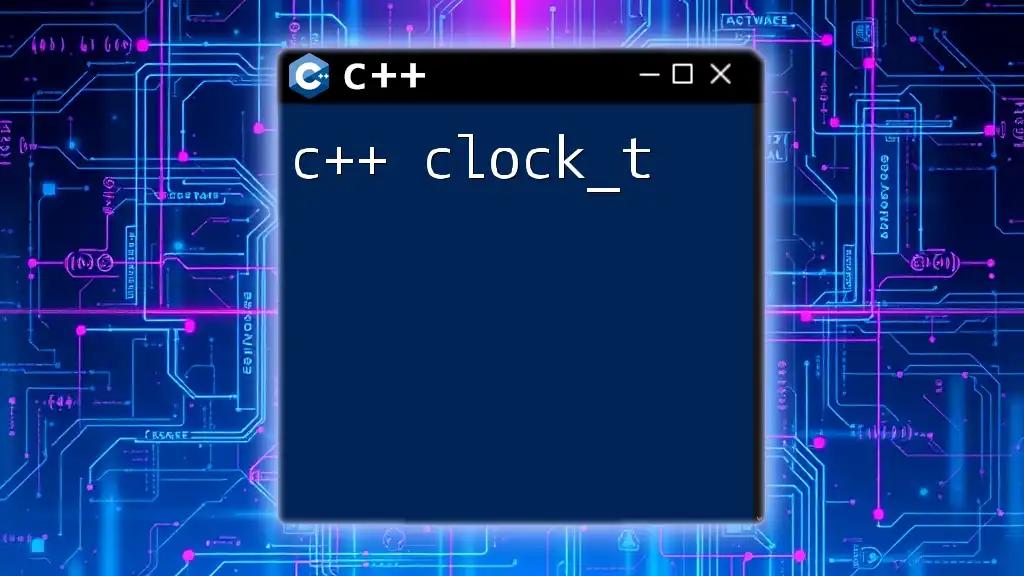
Summary
The C++ colon is a powerful tool embedded deeply within the syntax of the language, serving multiple roles from namespace management to controlling how classes interact. By mastering the proper usage of the colon, developers can write clearer, more efficient code.
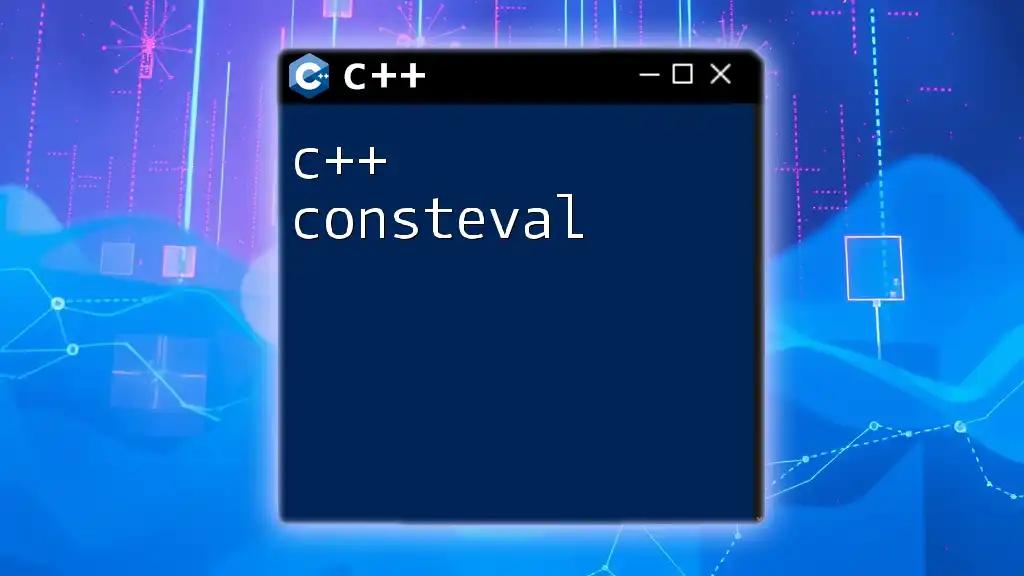
Frequently Asked Questions (FAQs)
-
What is the purpose of the colon in C++?
The colon has multiple purposes, such as indicating member access, namespace resolution, and controlling inheritance. -
Are there any alternatives to using colons?
While the colon is indispensable in certain contexts, alternatives like function pointers and typedefs can sometimes be used depending on the scenario. -
How can I troubleshoot issues related to colons in my code?
To troubleshoot, ensure correct syntax and check your access specifiers and context to avoid ambiguity or misdirected references.

Conclusion
Understanding and implementing the colon in C++ can drastically improve your programming efficiency and effectiveness. Practice using it in various contexts, and soon, it will become second nature. For more resources and more in-depth tutorials, stay engaged with our content!

Additional Resources
- [C++ Documentation](https://en.cppreference.com/w/)
- [C++ Programming Books](https://www.amazon.com/s?k=c%2B%2B+programming&ref=nb_sb_noss)
- [Online C++ Courses](https://www.coursera.org/courses?query=c%2B%2B)