A "C++ concurrency book" provides essential knowledge on implementing simultaneous execution of threads, enabling developers to efficiently manage multiple tasks within their applications.
Here's a simple example of using `std::thread` in C++ for concurrency:
#include <iostream>
#include <thread>
void printMessage() {
std::cout << "Hello from a thread!" << std::endl;
}
int main() {
std::thread t(printMessage); // Start a new thread
t.join(); // Wait for the thread to finish
return 0;
}
Key Concepts of C++ Concurrency
Threads
Threads are the basic units of execution in a C++ program. Using multiple threads allows you to execute code concurrently, making better use of CPU resources. This is particularly beneficial in applications that perform heavy computations or deal with I/O operations.
Creating a simple thread in C++ can be done with the `<thread>` library as follows:
#include <iostream>
#include <thread>
void hello() {
std::cout << "Hello from thread!" << std::endl;
}
int main() {
std::thread t(hello);
t.join(); // Wait for the thread to finish
return 0;
}
In this example, a new thread runs the `hello()` function independently, demonstrating how threads can help in multitasking.
Mutexes and Locks
Concurrency introduces challenges like race conditions, where multiple threads attempt to modify shared data simultaneously. To address this issue, C++ provides the `std::mutex` class, allowing you to synchronously access shared resources.
Consider this example:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx; // Global mutex
void print_keyboard() {
mtx.lock(); // Lock the mutex
std::cout << "Keyboard Input" << std::endl;
mtx.unlock(); // Unlock the mutex
}
int main() {
std::thread t1(print_keyboard);
std::thread t2(print_keyboard);
t1.join();
t2.join();
return 0;
}
By locking the mutex before accessing shared resources, you ensure that only one thread can execute the critical section at a time, thus preventing data corruption.
Condition Variables
Condition variables allow threads to pause execution until a specific condition is met. They are particularly useful in a producer-consumer scenario, where one thread (the producer) produces data and another (the consumer) processes it.
Here’s a basic implementation:
#include <iostream>
#include <thread>
#include <queue>
#include <condition_variable>
std::queue<int> q;
std::mutex mtx;
std::condition_variable cv;
void producer() {
for (int i = 0; i < 10; i++) {
std::unique_lock<std::mutex> lock(mtx);
q.push(i);
cv.notify_one(); // Notify the consumer
}
}
void consumer() {
while (true) {
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, [] { return !q.empty(); }); // Wait until the queue is not empty
int value = q.front();
q.pop();
lock.unlock();
std::cout << "Consumed: " << value << std::endl;
if (value == 9) break; // Exit condition for consumer
}
}
int main() {
std::thread t1(producer);
std::thread t2(consumer);
t1.join();
t2.join();
return 0;
}
In this example, the consumer waits for the producer to produce items. Condition variables help synchronize the two threads effectively.
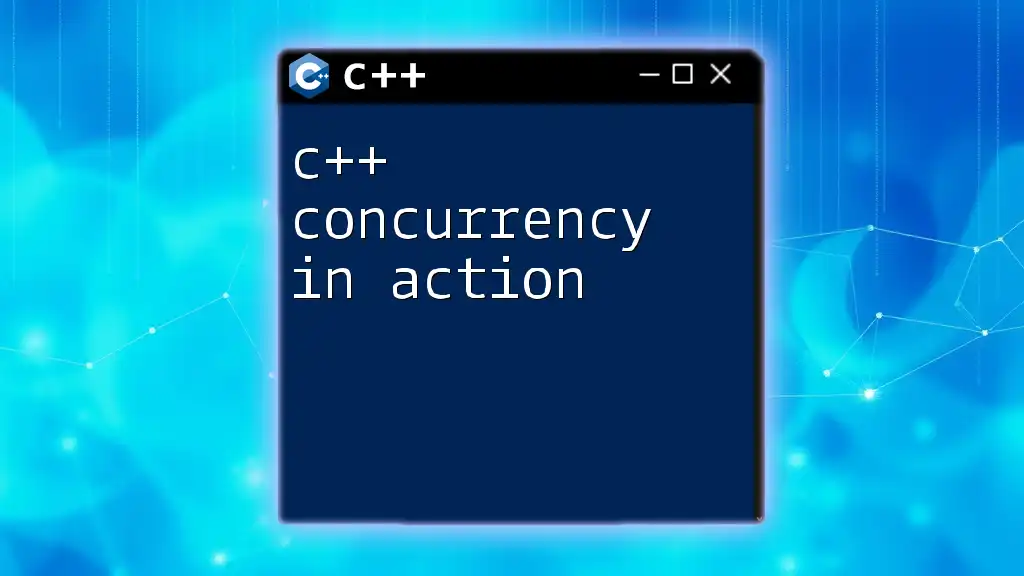
Advanced Topics in C++ Concurrency
Future and Promises
C++11 introduced `std::promise` and `std::future`, which enable asynchronous programming by allowing a thread to set a value that another thread will later retrieve. This model simplifies the handling of asynchronous tasks and improves code clarity.
Here’s an example illustrating their use:
#include <iostream>
#include <thread>
#include <future>
void calculate(std::promise<int> prom) {
int result = 10; // Some computation
prom.set_value(result); // Set the value for future
}
int main() {
std::promise<int> prom;
std::future<int> fut = prom.get_future();
std::thread t(calculate, std::move(prom)); // Pass promise to thread
std::cout << "Result: " << fut.get() << std::endl; // Get the value from future
t.join();
return 0;
}
In this case, the `calculate` function runs in a separate thread, setting the result for the main thread to use later. This keeps the threads decoupled, enhancing modularity.
Thread Pools
A thread pool is a collection of pre-initialized threads that can handle multiple tasks concurrently. This approach optimizes the system’s resources and reduces overhead due to frequent thread creation and destruction.
Here's a basic implementation of a thread pool:
#include <iostream>
#include <vector>
#include <thread>
#include <queue>
#include <functional>
class ThreadPool {
public:
ThreadPool(size_t threads);
void enqueue(std::function<void()> func);
~ThreadPool();
private:
std::vector<std::thread> workers;
std::queue<std::function<void()>> tasks;
std::mutex queue_mutex;
std::condition_variable condition;
bool stop;
};
ThreadPool::ThreadPool(size_t threads) : stop(false) {
for (size_t i = 0; i < threads; ++i)
workers.emplace_back([this] {
for (;;) {
std::function<void()> task;
{
std::unique_lock<std::mutex> lock(this->queue_mutex);
this->condition.wait(lock, [this] { return this->stop || !this->tasks.empty(); });
if (this->stop && this->tasks.empty()) return;
task = std::move(this->tasks.front());
this->tasks.pop();
}
task();
}
});
}
void ThreadPool::enqueue(std::function<void()> func) {
{
std::unique_lock<std::mutex> lock(queue_mutex);
tasks.emplace(func);
}
condition.notify_one();
}
ThreadPool::~ThreadPool() {
{
std::unique_lock<std::mutex> lock(queue_mutex);
stop = true;
}
condition.notify_all();
for (std::thread &worker: workers) worker.join();
}
In this implementation, the thread pool takes care of distributing the tasks among available threads, optimizing the handling of concurrent operations.
Atomic Types
The C++ Standard Library provides atomic types in the `<atomic>` header. These types are designed for safe access by multiple threads. Using `std::atomic<T>` ensures that operations on the object are indivisible, which helps prevent race conditions.
Here's a basic illustration:
#include <iostream>
#include <atomic>
#include <thread>
std::atomic<int> count(0);
void increment() {
for (int i = 0; i < 1000; ++i) {
count++;
}
}
int main() {
std::thread t1(increment);
std::thread t2(increment);
t1.join();
t2.join();
std::cout << "Final count: " << count.load() << std::endl; // Must be 2000
return 0;
}
This example shows how `std::atomic<int>` allows safe concurrent increments without the need for a mutex.
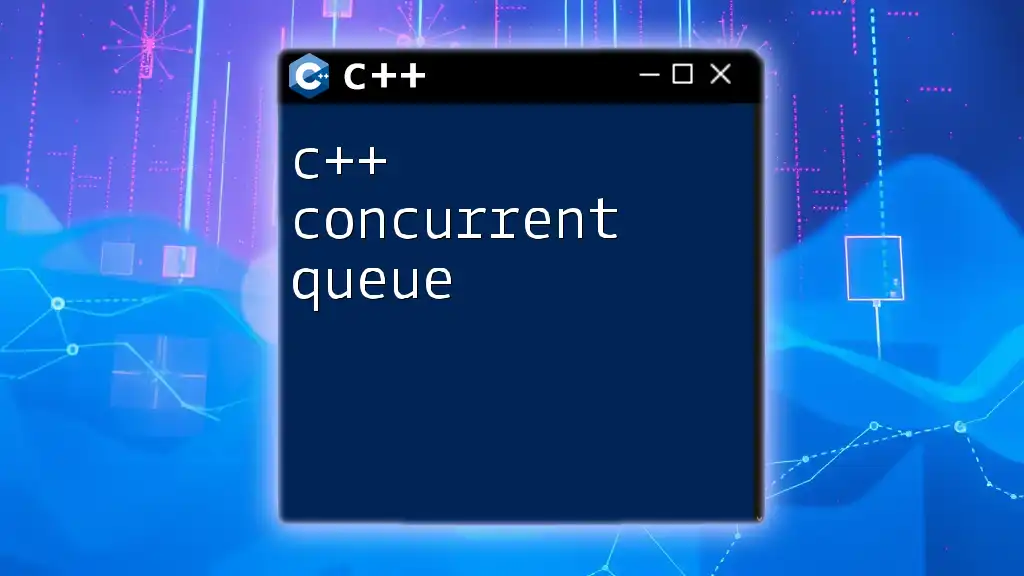
Tools and Libraries for C++ Concurrency
C++ Standard Library
The C++ Standard Library offers several components designed to simplify concurrent programming. Essential headers include `<thread>`, `<mutex>`, `<condition_variable>`, and `<atomic>`. These tools help developers create efficient and thread-safe applications.
Third-Party Libraries
In addition to the standard library, various third-party libraries like Boost and OpenMP enhance C++ concurrency capabilities. Boost provides additional high-level abstractions, while OpenMP simplifies parallel programming with directives.
When considering performance and ease of use, developers should weigh the trade-offs between using standard solutions versus leveraging robust third-party libraries.
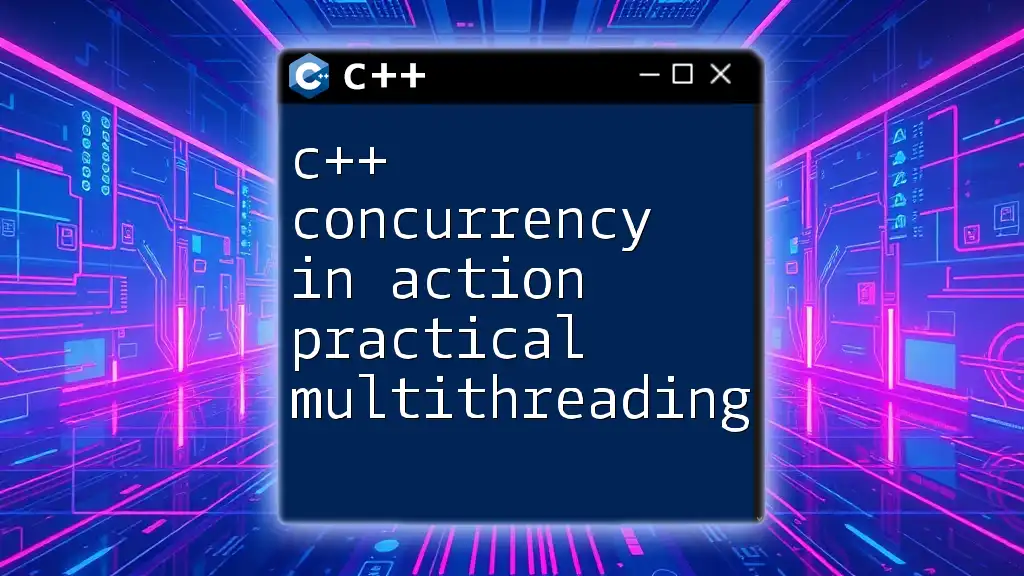
Best Practices for C++ Concurrency
Designing Concurrency with Safety in Mind
Always encapsulate shared resources to prevent unintended access. Using classes with well-defined interfaces can protect your data integrity and make the application easier to maintain.
Preventing Deadlocks
Deadlocks occur when two or more threads wait indefinitely for each other to release locks. To avoid deadlocks, establish a consistent locking order, avoid nested locks, and consider using timed locks or lock-free data structures.
Performance Considerations
Balancing the number of threads with system resources is crucial. The overhead of managing too many threads can negatively impact performance. Use profiling tools to measure performance and adjust thread usage accordingly.
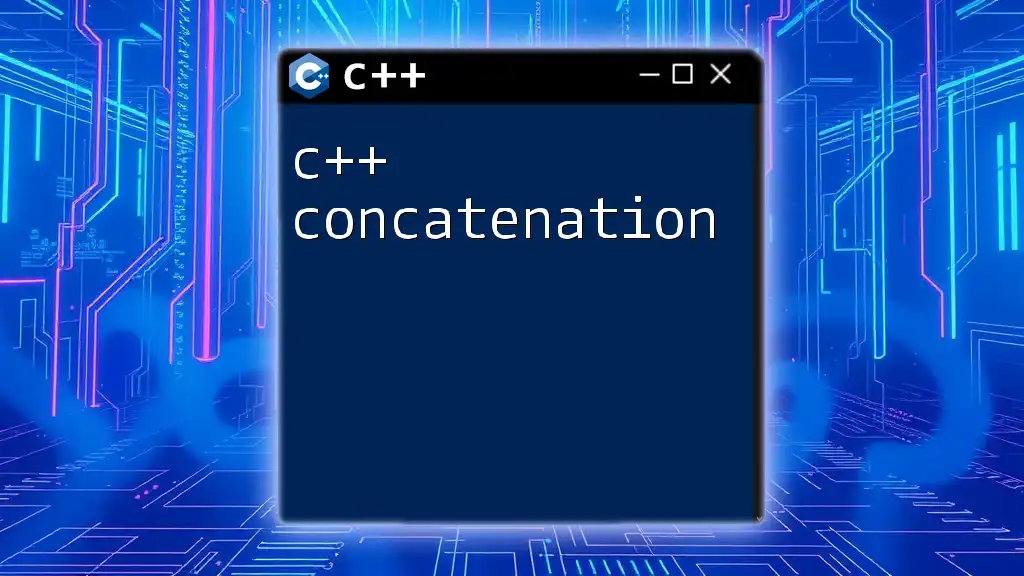
Conclusion
Understanding C++ concurrency is essential in today’s multi-core processing world. Leveraging threads, mutexes, condition variables, and atomic types effectively can lead to significant improvements in performance and responsiveness. By mastering these concepts, developers can build robust, efficient applications that fully utilize the capabilities of modern hardware.
Embarking on a journey with a "C++ concurrency book" can greatly enhance your skills in this area. Make use of the resources available, practice consistently, and engage with the community to further your understanding and experience.
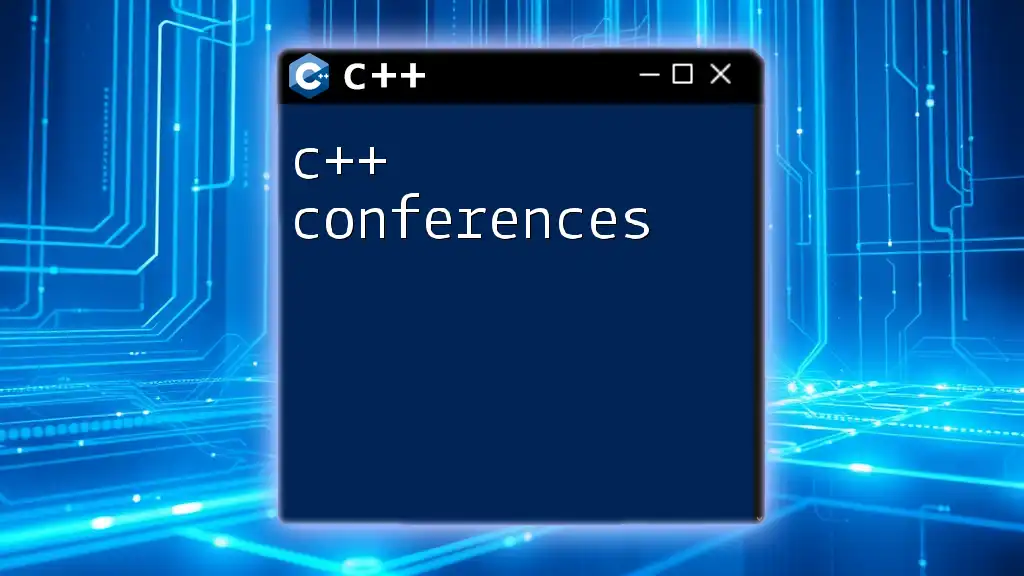
Call to Action
Join Our Community
If you found this guide helpful, consider subscribing for more exclusive insights and tips on C++.
Share Your Experience
We encourage you to share your own projects, challenges, or questions surrounding C++ concurrency. Engaging with like-minded individuals can lead to new insights and collaborations!