Concurrency in modern C++ allows developers to write programs that can perform multiple tasks simultaneously, leveraging features like threads and the standard library's `<thread>` header to improve performance and responsiveness.
Here's a simple example of creating a thread in C++:
#include <iostream>
#include <thread>
void task() {
std::cout << "Hello from the thread!" << std::endl;
}
int main() {
std::thread t(task);
t.join(); // Wait for the thread to finish
return 0;
}
Understanding Concurrency
What is Concurrency?
Concurrency refers to the ability of a program to manage multiple tasks or operations at the same time, improving the efficiency and responsiveness of applications. It’s essential to distinguish between concurrency and parallelism. Concurrency allows tasks to be in progress simultaneously, while parallelism refers to executing multiple tasks at exactly the same time, typically through multiple processors.
For example, in a restaurant, a waiter may take orders from multiple tables (concurrency) but can only serve one table at a time (parallelism). Understanding these concepts is vital because they influence how we design and implement software, especially in contexts where performance and responsiveness are critical.
Why Use Concurrency?
The benefits of using concurrency in programming are manifold:
- Improved Performance: By running tasks in parallel or overlapping their execution, you can significantly reduce total processing time.
- Responsiveness: In applications with user interfaces, concurrent processing ensures that the application remains responsive even when performing intensive computations.
- Resource Utilization: Properly implemented concurrent programs utilize system resources more effectively, particularly in multi-core systems where tasks can spread across multiple cores.
There are situations where concurrency can dramatically improve an application’s efficiency. For example, in web servers handling multiple client requests, each connection can be processed concurrently, allowing for better throughput and user experience.
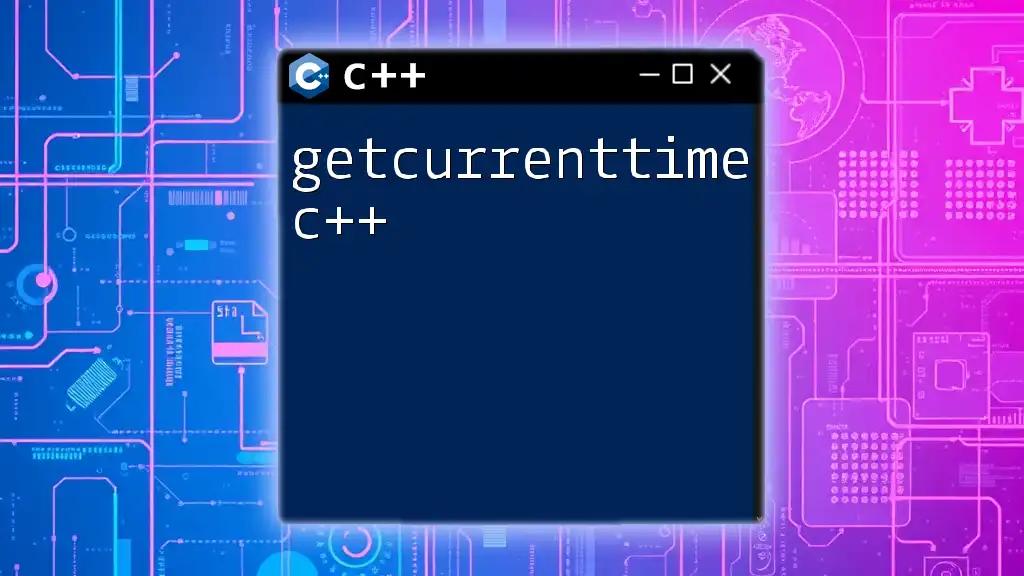
Modern C++ Concurrency Features
C++11: The Start of Modern Concurrency
The introduction of C++11 marked a significant advancement in how concurrency is handled in C++. The major features that were introduced included:
- `std::thread`: This allows the creation and management of threads.
- `std::async`: This simplifies asynchronous computation.
- `std::future`: A mechanism to retrieve the results of asynchronous operations.
These components empower developers to create concurrent programs with ease and flexibility.
C++14 and Beyond: Enhancements and Improvements
As C++ progressed, further enhancements were made to its concurrency model, notably in C++14 and C++17:
- C++14 introduced features like `std::shared_timed_mutex`, which provides enhanced capabilities for managing shared access across threads.
- C++17 brought `std::scoped_lock`, a convenient way to manage multiple locks in a deadlock-free manner.
These updates continue to build on the foundation set by C++11, making concurrent programming more robust and user-friendly.

Working with Threads
Creating and Managing Threads
Creating threads in C++ using `std::thread` is straightforward. Here’s a simple example:
#include <iostream>
#include <thread>
void threadFunction() {
std::cout << "Hello from thread!" << std::endl;
}
int main() {
std::thread t(threadFunction);
t.join();
return 0;
}
In this example, a new thread is created that runs `threadFunction`, which prints a message. The main program uses `t.join()` to wait for the thread to finish execution before proceeding, ensuring proper synchronization.
Thread Safety and Data Races
As concurrent operations increase, so does the risk of data races—situations where two or more threads read and write shared data at the same time without proper synchronization. To mitigate this, it's essential to implement thread safety mechanisms.
Mutexes and locks are critical tools in achieving thread safety. A mutex (short for mutual exclusion) is a locking mechanism that prevents multiple threads from accessing the same resource simultaneously.
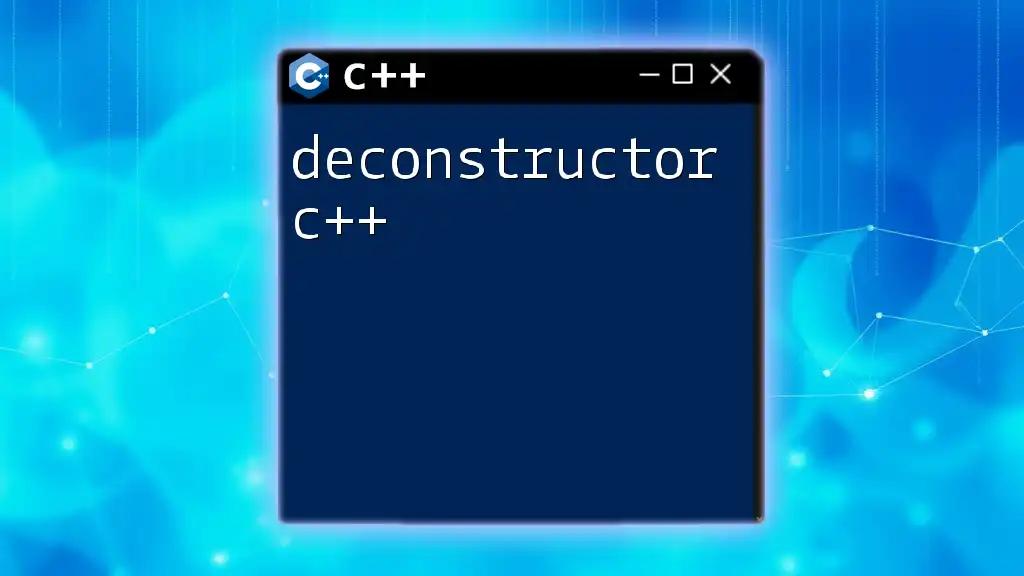
Synchronization Techniques
Understanding Locks
Locks are essential when working with shared resources.
Mutexes
Here's how you can implement thread safety using `std::mutex`:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx;
void safePrint() {
mtx.lock();
std::cout << "Thread-safe print!" << std::endl;
mtx.unlock();
}
In this example, the `mtx` mutex is used to ensure that only one thread can execute the `safePrint` function at a time, preventing data races.
Unique Locks
`std::unique_lock` offers more flexibility compared to raw mutexes, as it automatically manages the locking and unlocking:
void safePrintUnique() {
std::unique_lock<std::mutex> lock(mtx);
std::cout << "Thread-safe print with unique_lock!" << std::endl;
}
With `std::unique_lock`, you do not have to explicitly unlock; the lock is automatically released once the `unique_lock` object goes out of scope.
Shared Locks
In scenarios where multiple threads need to read data concurrently but write operations must be exclusive, `std::shared_mutex` is useful:
#include <shared_mutex>
std::shared_mutex sharedMtx;
void readFunction() {
std::shared_lock<std::shared_mutex> lock(sharedMtx);
// reading data
}
void writeFunction() {
std::unique_lock<std::shared_mutex> lock(sharedMtx);
// modifying data
}
In this example, `readFunction` allows multiple simultaneous read operations, while `writeFunction` ensures exclusive access during writes.
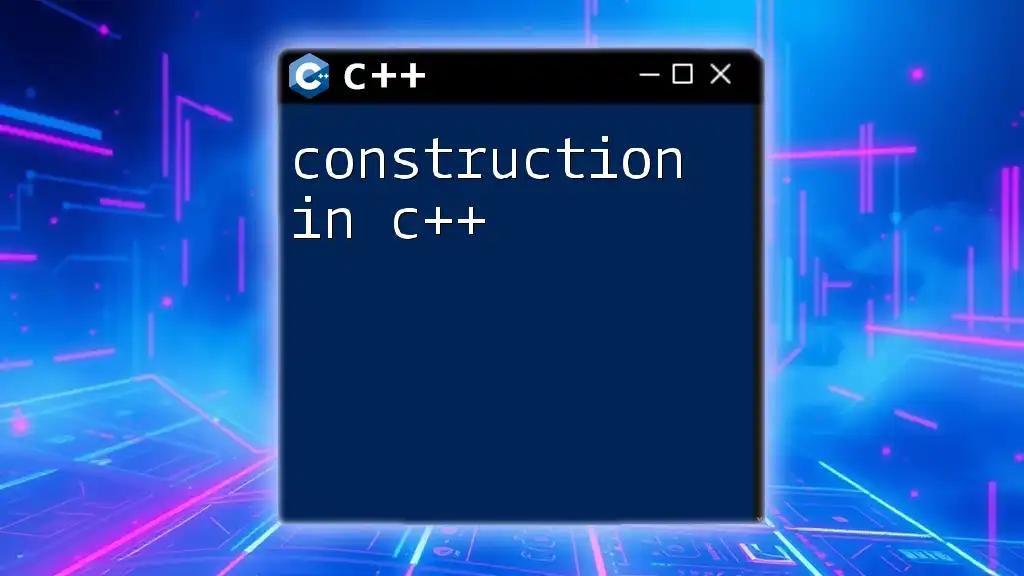
Using `std::async` for Simplicity
Introduction to `std::async`
`std::async` offers a high-level alternative for handling asynchronous operations. It allows you to express simple asynchronous tasks without direct thread management:
#include <iostream>
#include <future>
int compute() {
return 42;
}
int main() {
std::future<int> result = std::async(compute);
std::cout << "The answer is: " << result.get() << std::endl;
return 0;
}
This code demonstrates executing `compute` asynchronously, providing a `std::future` to retrieve results. The call to `.get()` will block until the result is ready, making it easy to synchronize with the computed value.

Advanced Concurrency with C++
Thread Pools
Thread pools keep a set of worker threads ready to execute tasks, optimizing resource use and reducing overhead from thread creation. Implementing a simple thread pool allows for efficient task management, especially when handling numerous tasks.
Condition Variables
Condition variables enable threads to communicate, allowing one thread to notify others that a specific condition has been met:
#include <condition_variable>
std::condition_variable cv;
std::mutex mtx;
bool ready = false;
void waitForSignal() {
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, [] { return ready; });
// proceed with work
}
void signal() {
std::lock_guard<std::mutex> lock(mtx);
ready = true;
cv.notify_all();
}
In this example, `waitForSignal` suspends execution until `signal` sets `ready` to true, demonstrating efficient synchronization between threads.
Atomic Operations
Atomic types provide a way to perform operations on shared variables without locks, maintaining consistency across threads:
#include <atomic>
std::atomic<int> counter(0);
void increment() {
counter++;
}
Using atomic operations like this prevents data races naturally, but they are only suitable for specific scenarios where operations can be performed as single, indivisible actions.
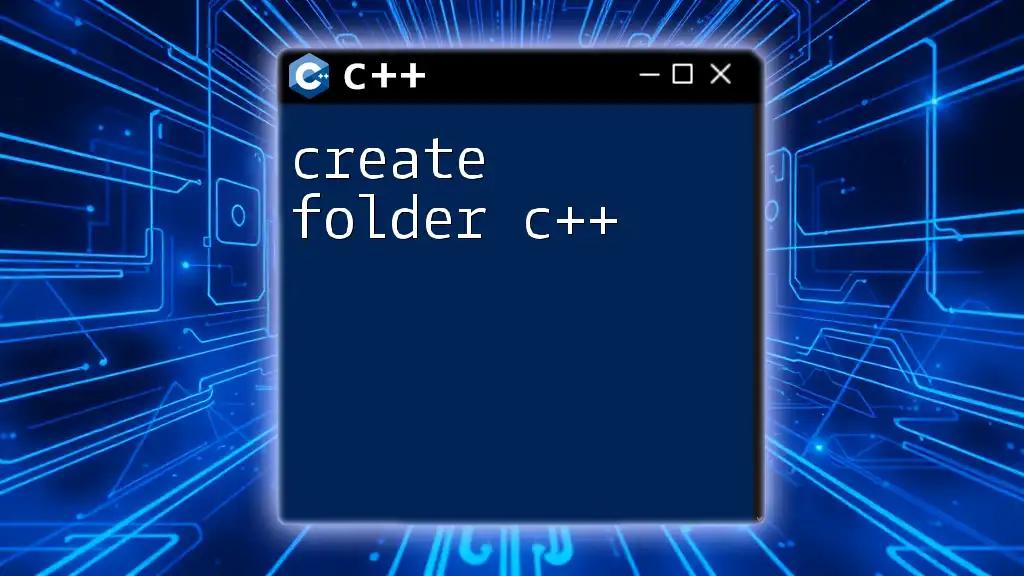
Best Practices for Concurrency
Concurrency can introduce complex behaviors and potential pitfalls. Here are some best practices to heed:
- Always prefer higher-level abstractions (like `std::async` or thread pools) where possible, as they reduce management complexity.
- Avoid global shared data when possible; encapsulate state within classes or objects.
- When using mutexes, prefer `std::unique_lock` for safer management of lock scope, reducing human error risk.
- Carefully design to avoid deadlocks by always acquiring locks in a consistent order.
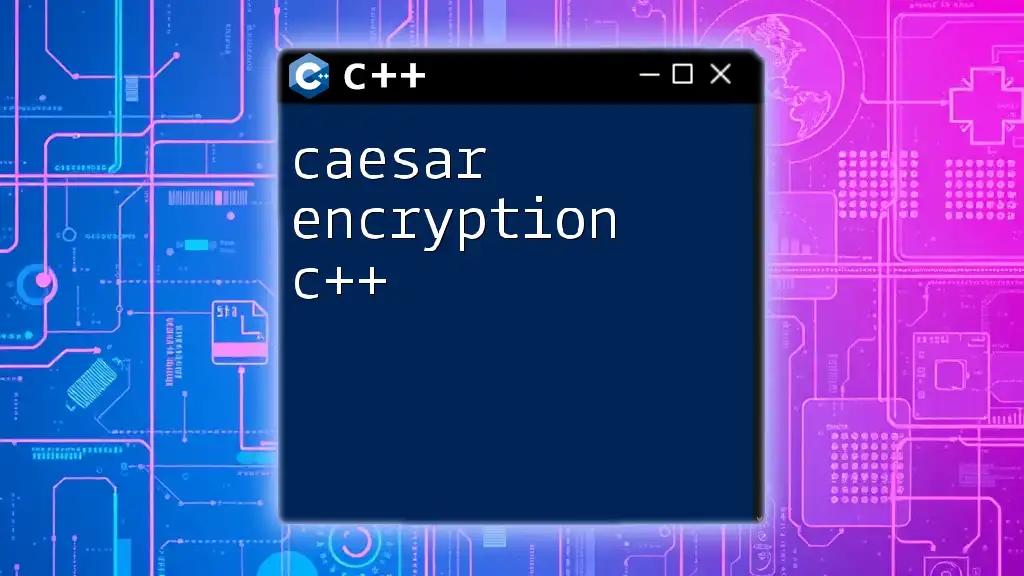
Conclusion
Concurrency is an essential feature of modern programming, particularly in C++. With its rich set of tools and features, C++ offers developers the ability to create highly efficient and responsive applications. Understanding and correctly applying concurrency with modern C++ will significantly enhance your programming capabilities. Practice the examples provided, and explore further to master concurrency in your applications.

Additional Resources
For further exploration of concurrency in C++, consider looking into the following resources:
- Books: The C++ Programming Language by Bjarne Stroustrup and C++ Concurrency in Action by Anthony Williams.
- Online Courses: Platforms like Coursera and Udacity often offer specific courses on C++ and concurrency.
- Documentation: Check the latest C++ standards and documentation on sites like cppreference.com.
Engaging with community forums like Stack Overflow can also help address queries and share insights with fellow C++ enthusiasts.