Caesar encryption is a simple substitution cipher where each letter in the plaintext is shifted a certain number of places down or up the alphabet, and here's an example C++ implementation:
#include <iostream>
#include <string>
std::string caesarEncrypt(const std::string& text, int shift) {
std::string result = "";
for (char c : text) {
if (isalpha(c)) {
char base = islower(c) ? 'a' : 'A';
result += char(int(base + (c - base + shift) % 26));
} else {
result += c;
}
}
return result;
}
int main() {
std::string plaintext = "Hello, World!";
int shift = 3;
std::string encrypted = caesarEncrypt(plaintext, shift);
std::cout << "Encrypted: " << encrypted << std::endl;
return 0;
}
What is Caesar Cipher?
The Caesar Cipher is a classic encryption technique that dates back to ancient Rome, named after Julius Caesar, who is said to have used it to communicate with his generals. This encryption method involves shifting the letters of the alphabet by a fixed number. For example, with a shift of 3, 'A' becomes 'D', 'B' becomes 'E', and so forth. This substitution cipher is one of the simplest forms of encryption, making it an excellent educational tool for introducing basic cryptographic concepts.
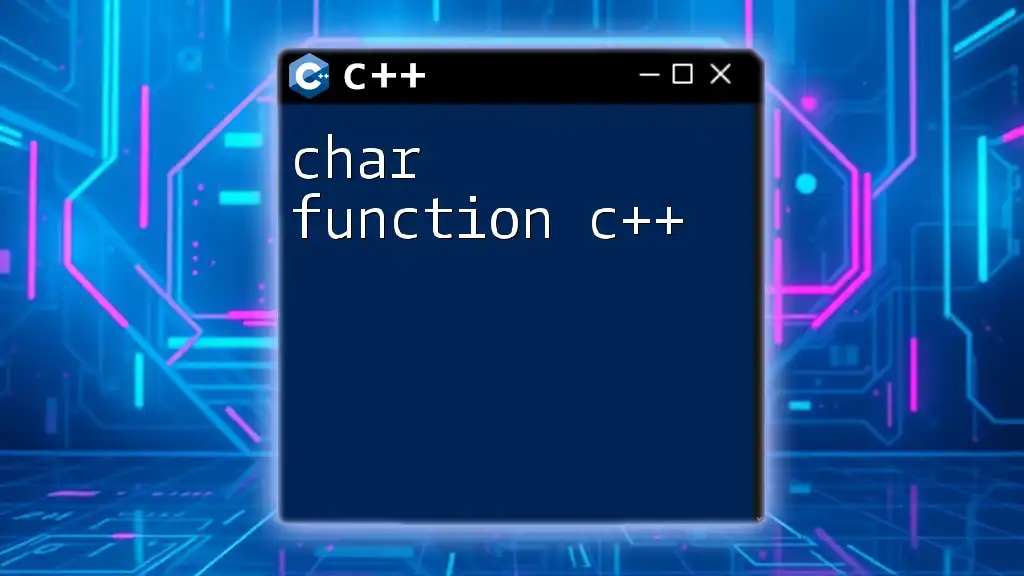
Why Use Caesar Cipher?
The Caesar Cipher is not just a historical curiosity; it serves as a fundamental example of how encryption works. Its simplicity allows learners to grasp the core principles of encryption, such as:
- Substitution: Each character is replaced with another character.
- Key: The number of positions each letter is shifted serves as the key for encryption.
- Reversibility: The encrypted text can be decrypted back to the original text using the same key.
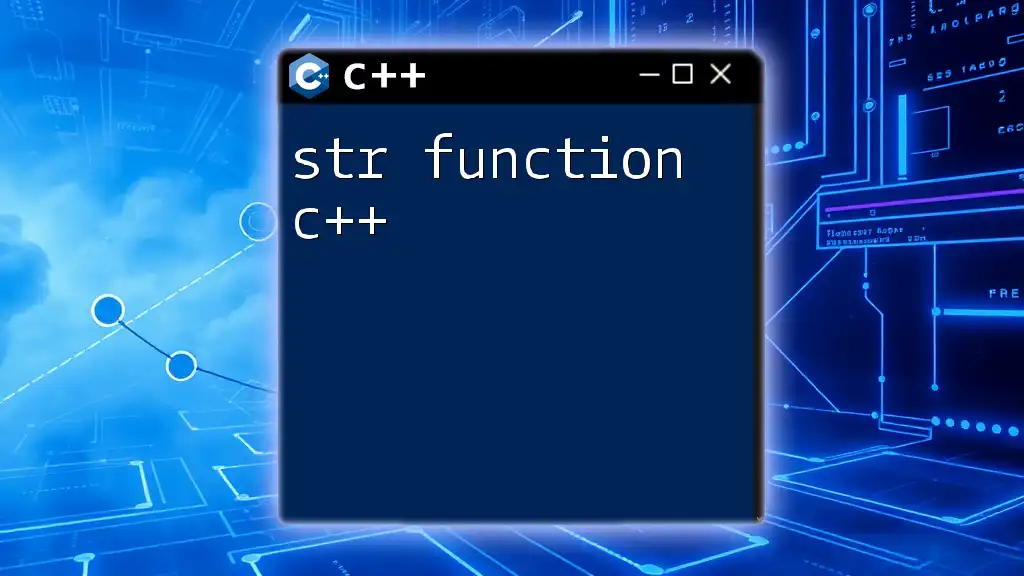
Core C++ Concepts Needed for Implementation
To implement a Caesar Cipher in C++, you need a good grasp of several core concepts:
- Variables and Data Types: Essential for storing the user input and the shift key.
- Functions in C++: Allows you to modularize your code, making it reusable and organized.
- Basic Input/Output Techniques: To read from the user and display the results.
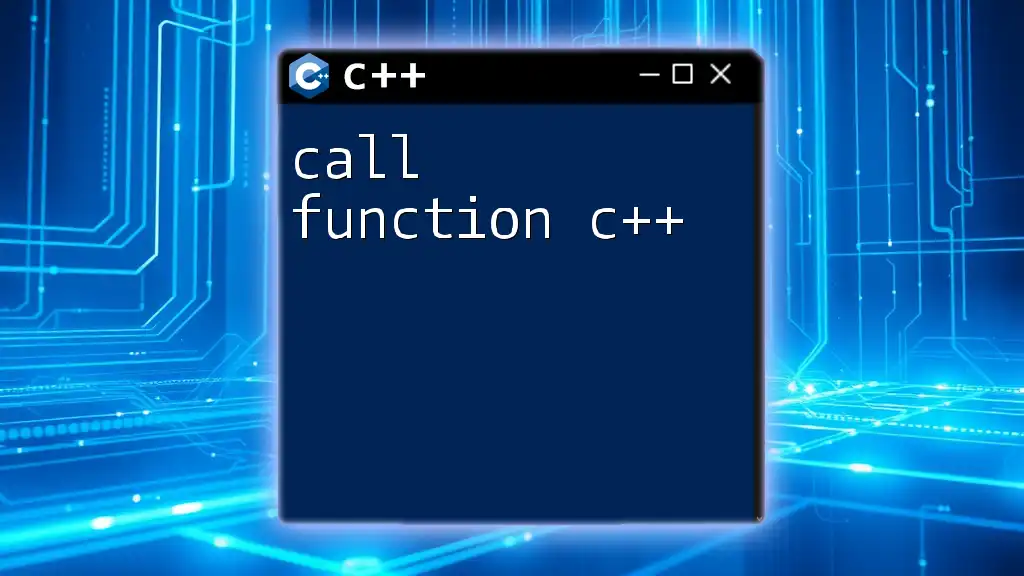
Overview of the Algorithm
How Caesar Cipher Works
The mechanics of a Caesar Cipher are quite straightforward. Each letter in the plaintext is replaced with another letter that is a fixed number of positions down or up the alphabet. The fixed number is known as the shift. For instance, with a shift of 3:
- Plaintext: A B C D E F G
- Ciphertext: D E F G H I J
Example of a Simple Caesar Cipher Program
Here's a basic skeleton of a C++ program for implementing Caesar Cipher.
#include <iostream>
#include <string>
void encrypt(std::string &text, int shift) {
// Implementation will go here.
}
void decrypt(std::string &text, int shift) {
// Implementation will go here.
}
int main() {
// Example usage.
return 0;
}
Explanation of Code Structure
In this code structure:
- encrypt() and decrypt() are the functions that will handle the encryption and decryption processes.
- main() serves as the entry point of the application, providing the user interface.
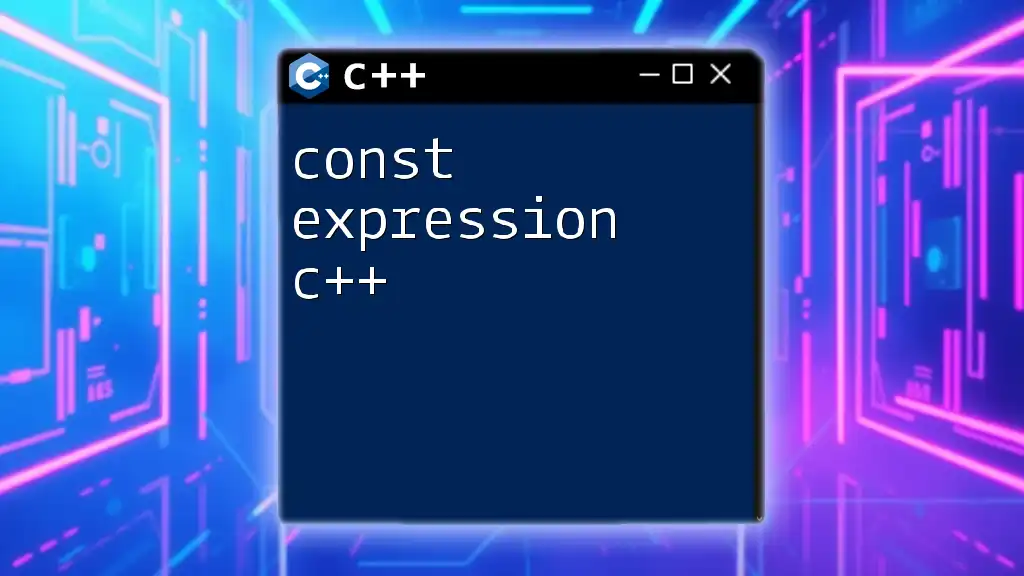
Creating the Encrypt Function
Code Snippet for Encrypt Function
The following function demonstrates how to encrypt a string using the Caesar Cipher method.
void encrypt(std::string &text, int shift) {
for (char &c : text) {
if (isalpha(c)) {
char offset = islower(c) ? 'a' : 'A';
c = (c - offset + shift) % 26 + offset;
}
}
}
Explanation of Encryption Logic
- Loop through each character: The function iterates over each character in the string.
- Character Check: It checks if the character is an alphabetic character using `isalpha(c)`.
- Handling Case Sensitivity: The `offset` variable determines whether the character is lowercase or uppercase. This ensures that shifts remain within the same case.
- Cyclic Behavior: The formula `(c - offset + shift) % 26 + offset` ensures that if the shift takes us past 'Z' or 'z', it wraps around to the beginning of the alphabet.
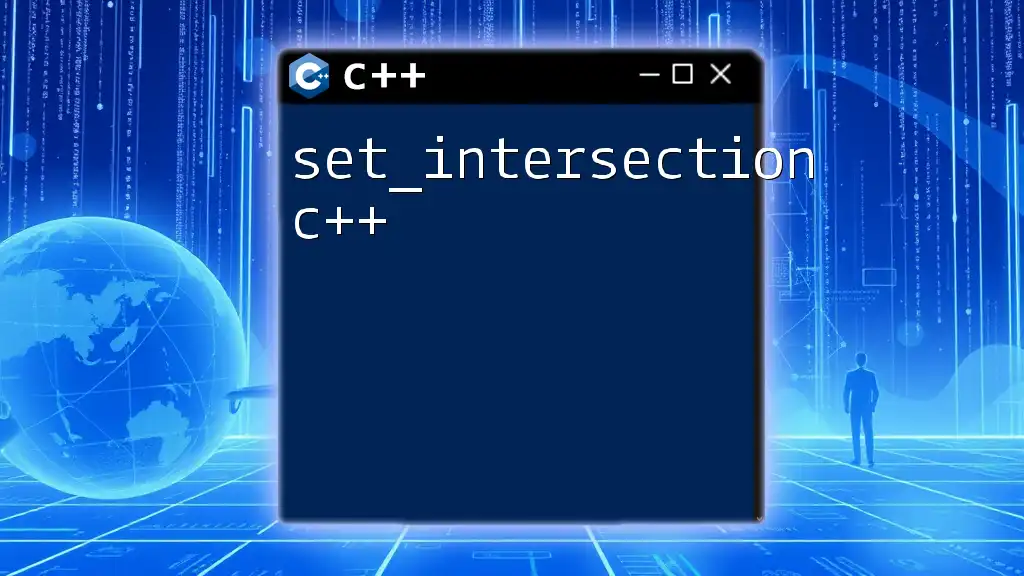
Creating the Decrypt Function
Code Snippet for Decrypt Function
You can easily create the decrypt function by modifying the encrypt function, as shown below.
void decrypt(std::string &text, int shift) {
encrypt(text, 26 - shift); // Reusing the encrypt function
}
Explanation of Decryption Logic
Decrypting the message involves a reversal of the encryption process. By passing `26 - shift` to the `encrypt` function, you effectively shift the letters back to their original positions. The use of modular arithmetic ensures that the text wraps around correctly even when `shift` is greater than 26.
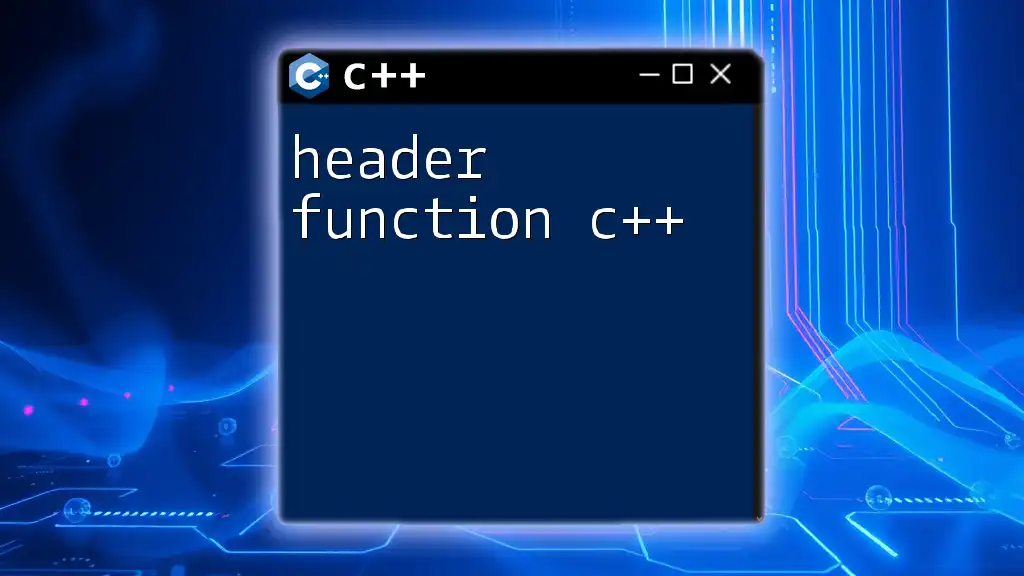
Example of a Fully Functional Caesar Cipher in C++
Below is a complete implementation of the Caesar Cipher program in C++.
#include <iostream>
#include <string>
// Function prototypes
void encrypt(std::string &text, int shift);
void decrypt(std::string &text, int shift);
int main() {
std::string text;
int shift;
std::cout << "Enter text to encrypt: ";
std::getline(std::cin, text);
std::cout << "Enter shift value: ";
std::cin >> shift;
encrypt(text, shift);
std::cout << "Encrypted Text: " << text << std::endl;
decrypt(text, shift);
std::cout << "Decrypted Text: " << text << std::endl;
return 0;
}
Detailed Explanation of the Complete Code
This code prompts the user for the text they wish to encrypt and the desired shift amount. The program then:
- Encrypts the text: Calls the `encrypt()` function and displays the encrypted text.
- Decrypts the text: Calls the `decrypt()` function to revert the text back to its original state.
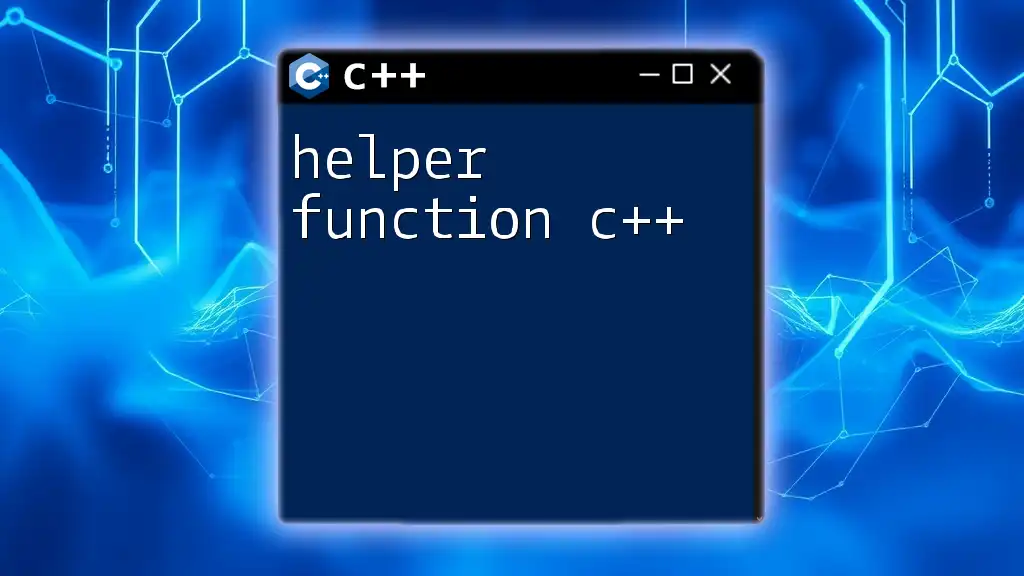
Common Improvements
Validating User Input
Adding input validation for the shift value can enhance user experience. Ensure that the shift is within the range of 0-25, as shifts beyond 25 simply loop back through the alphabet.
Handling Non-Alphabetic Characters
To improve the robustness of the cipher, consider how to handle spaces and other non-alphabetic characters gracefully, so they are preserved as they are within the output.
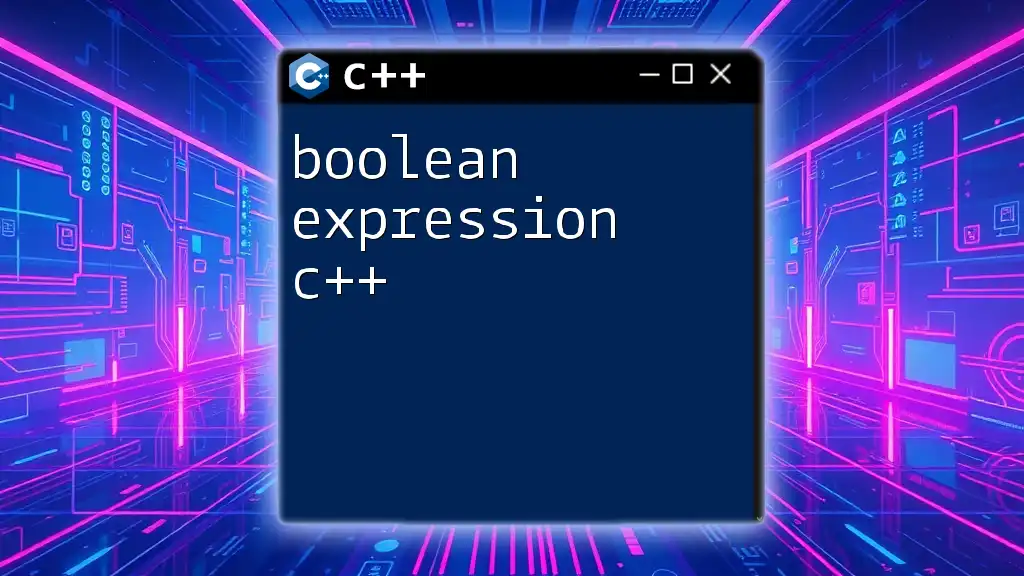
Performance Considerations
While the Caesar Cipher is simple and effective for small texts, it's essential to recognize that this method is not secure against modern cryptographic methods. Its time complexity is O(n), where n is the length of the text, making it efficient for educational use but not for secure communications.
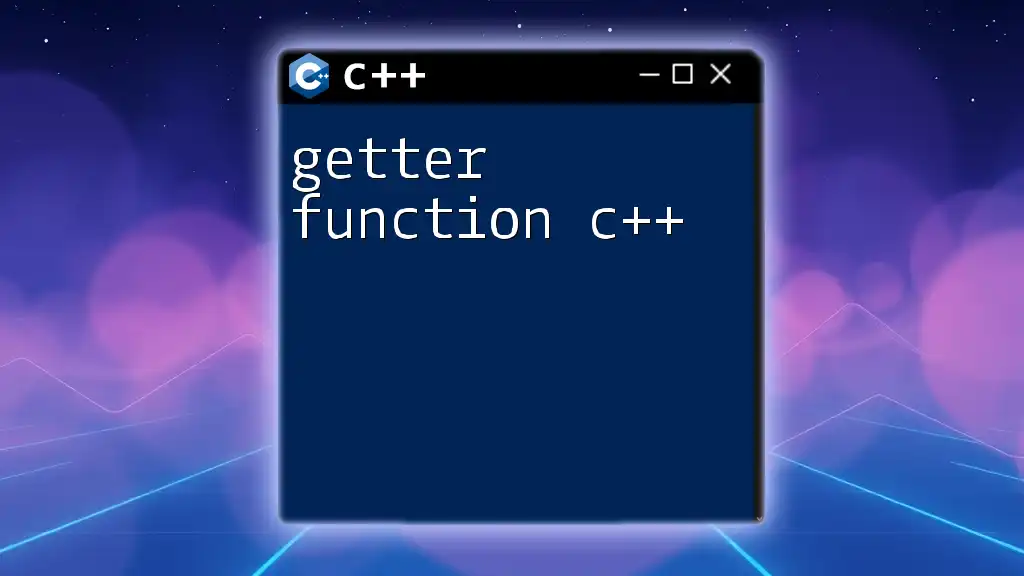
Where You Might Use Caesar Cipher
- Cryptography Courses: The Caesar Cipher is often used as an introductory example in both academic and practical cryptography courses.
- Programming Challenges: Simple encryption tasks often feature in coding competitions and exercises.
- Games: Some puzzle or adventure games may use variations of this cipher as part of their storyline.
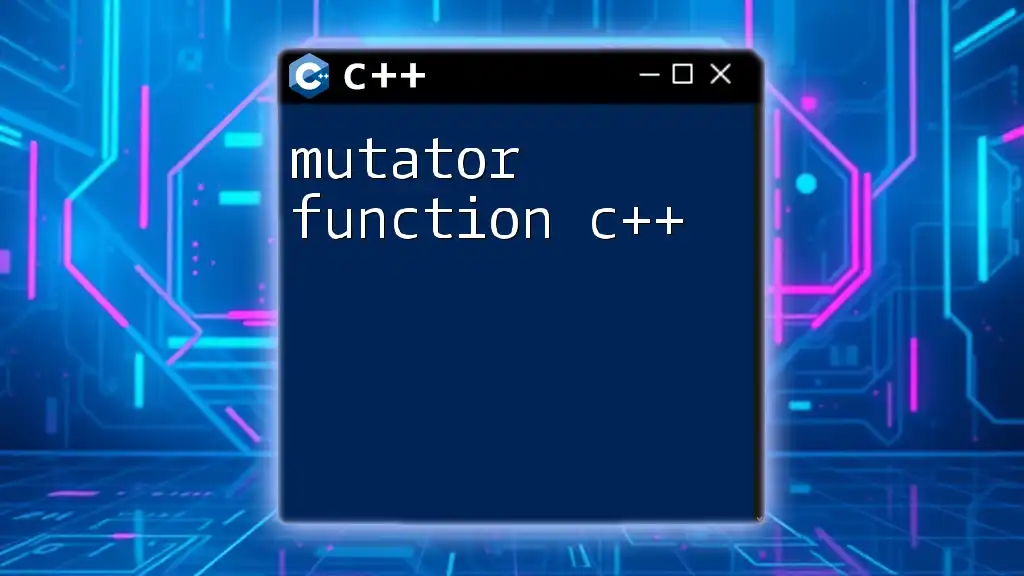
Final Thoughts on Caesar Cipher C++ Implementation
Implementing the Caesar Cipher in C++ serves as an invaluable exercise for budding programmers. This implementation not only illustrates core programming techniques but also offers a foundational understanding of how encryption methods work.
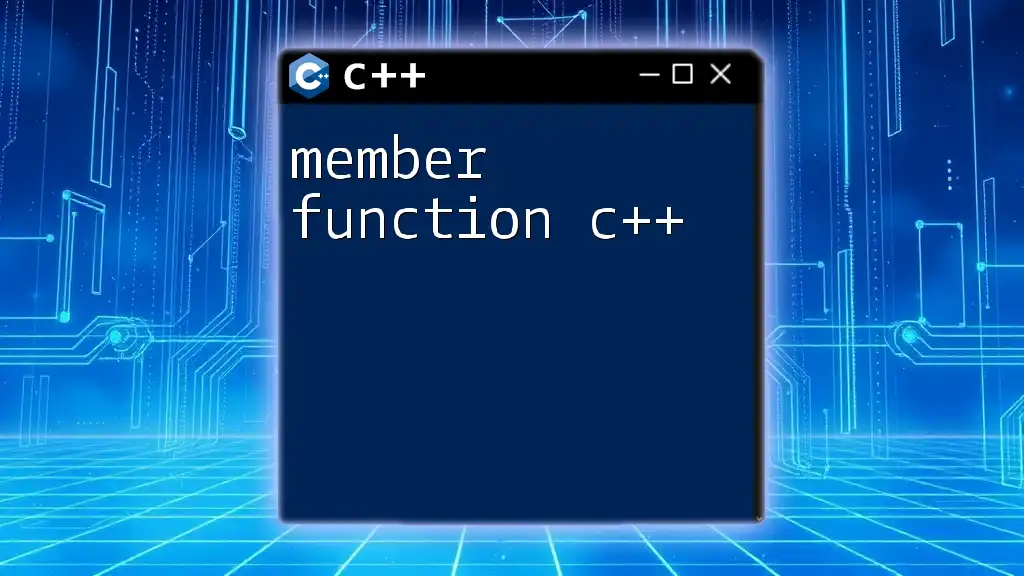
Further Learning and Resources
For those eager to delve deeper, consider exploring additional resources on cryptographic algorithms such as the Vigenère Cipher, Advanced Encryption Standard (AES), or other more complex cryptographic schemes. Online coding platforms can offer practice problems to enhance your skills further.
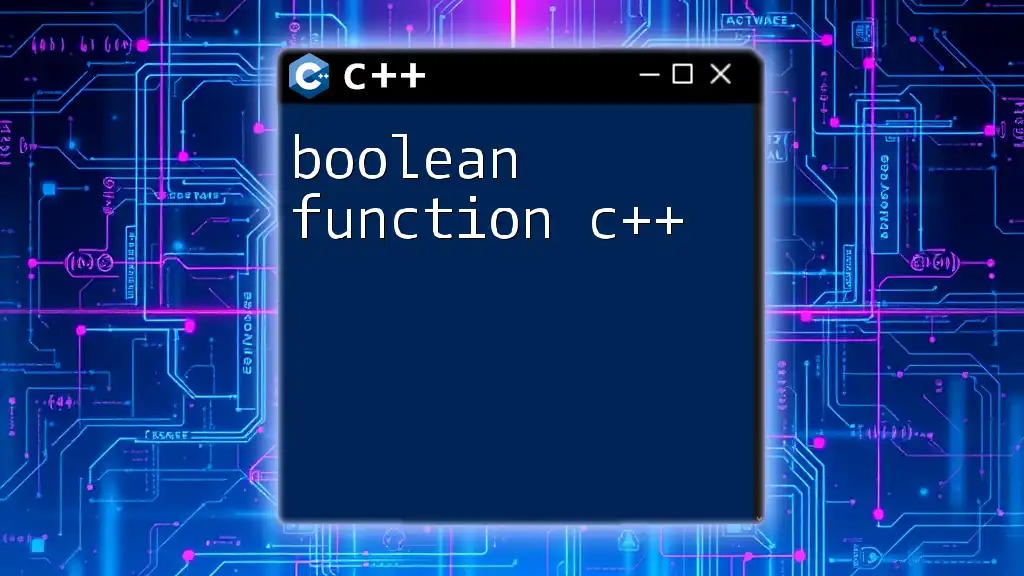
Encouragement to Experiment with the Code
The implementation of the Caesar Cipher is an excellent starting point for experimenting with cryptography. Feel free to modify the existing code, try different shift values, or even create a UI for the program. The more you play with it, the more you’ll learn!