A class declaration in C++ defines a blueprint for creating objects, encapsulating data and functions that operate on that data.
Here’s an example of a class declaration in C++:
class MyClass {
public:
int myNumber; // An integer variable
void display() {
std::cout << "The number is: " << myNumber << std::endl;
}
};
Understanding Class Declaration in C++
What is a Class in C++?
A class in C++ acts as a blueprint for creating objects, encapsulating both data and functions that operate on that data. It is a fundamental concept in Object-Oriented Programming (OOP), allowing developers to model real-world entities. By utilizing classes, programmers can create modular and reusable code, which enhances maintainability and scalability.
What is Class Declaration?
A class declaration in C++ defines the structure of a class but does not provide the complete implementation. It specifies what member variables and functions the class will have, while the actual implementation of those functions typically occurs after the declaration. Understanding the distinction between class declaration and class definition is crucial; while a declaration indicates the existence of a class, a definition provides the required details.
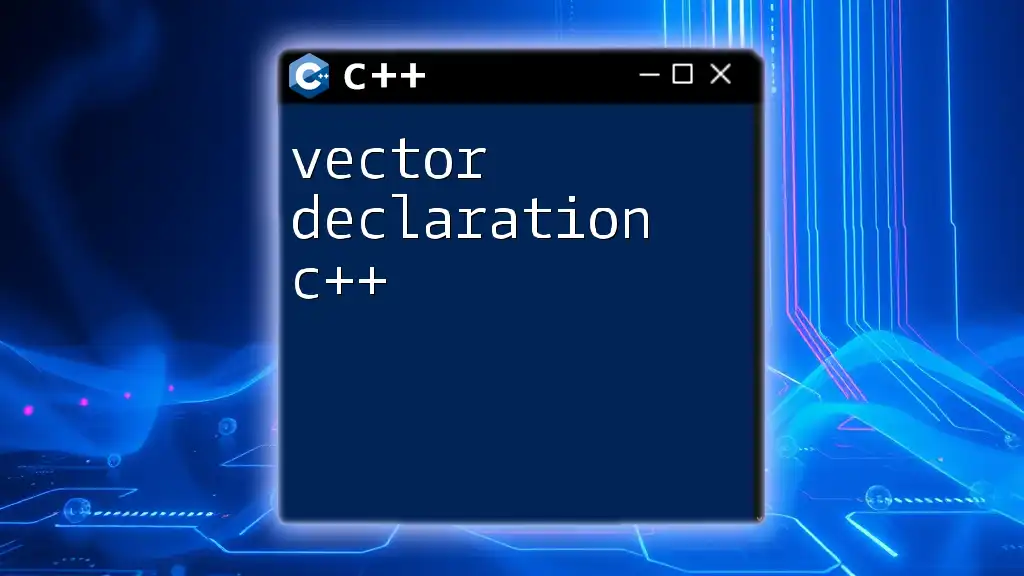
Syntax of Class Declaration
Basic Syntax
The syntax for a C++ class declaration is straightforward. It begins with the keyword `class`, followed by the class name, and a pair of curly braces to enclose its body. Here's the basic structure:
class ClassName {
public:
// Member variables and functions
};
Example of a simple class declaration:
class MyClass {
public:
int myVariable;
void myMethod();
};
This example outlines a class named `MyClass` that has one integer member variable `myVariable` and one member function `myMethod()`.
Access Specifiers
In C++, access specifiers play a critical role in determining the visibility of class members—whether they are accessible from outside the class. The three main specifiers are public, private, and protected.
- Public: Members declared under public can be accessed from anywhere in the program.
- Private: Members declared as private are accessible only within the class itself.
- Protected: Members declared protected can be accessed in the class and by derived classes.
Example of access specifiers in a class:
class MyClass {
private:
int myPrivateVariable;
public:
void myPublicMethod();
protected:
int myProtectedVariable;
};
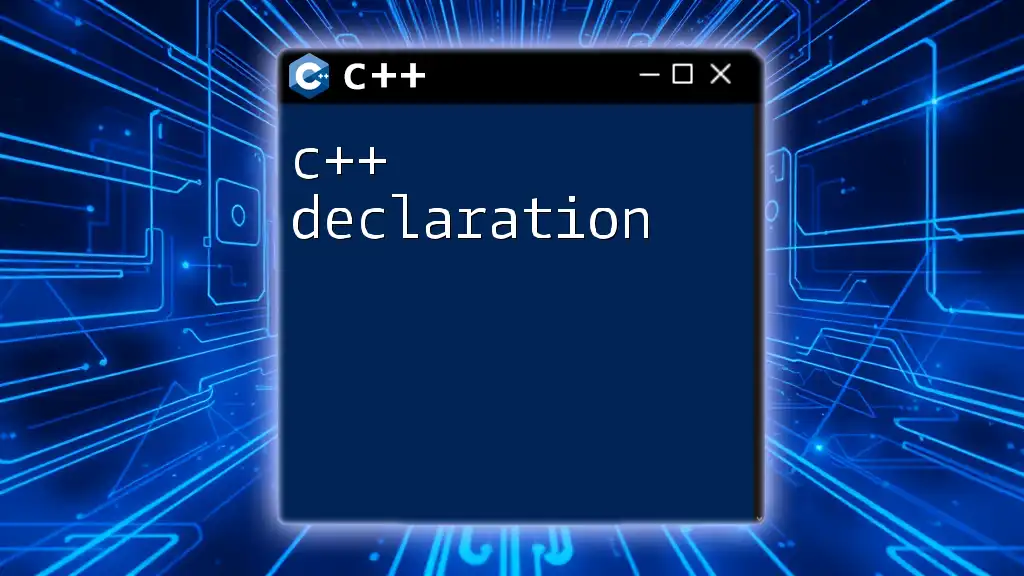
Components of Class Declaration
Member Variables
Member variables are the data attributes of the class. They hold state or properties associated with the class.
Example:
class Student {
public:
std::string name;
int age;
};
This `Student` class has two member variables: `name` and `age`, which represent the student's name and age, respectively.
Member Functions
Member functions define the behaviors of the class and can manipulate its member variables. They can be thought of as methods that operate on an object's state.
Example:
class Calculator {
public:
int add(int a, int b) {
return a + b;
}
};
In this `Calculator` class, the `add` function takes two integers and returns their sum. This example illustrates how member functions can enhance the functionality of a class.
Constructors and Destructors
Constructors are special member functions invoked when an object is instantiated. They initialize member variables, ensuring that the object starts in a valid state. Destructors, conversely, are called when an object is destroyed, allowing for cleanup and resource deallocation.
Example of constructor and destructor:
class MyClass {
public:
MyClass() {
// Constructor code
}
~MyClass() {
// Destructor code
}
};
In the above example, `MyClass` has a constructor that executes when an object of `MyClass` is created, and a destructor that executes when that object is destroyed.
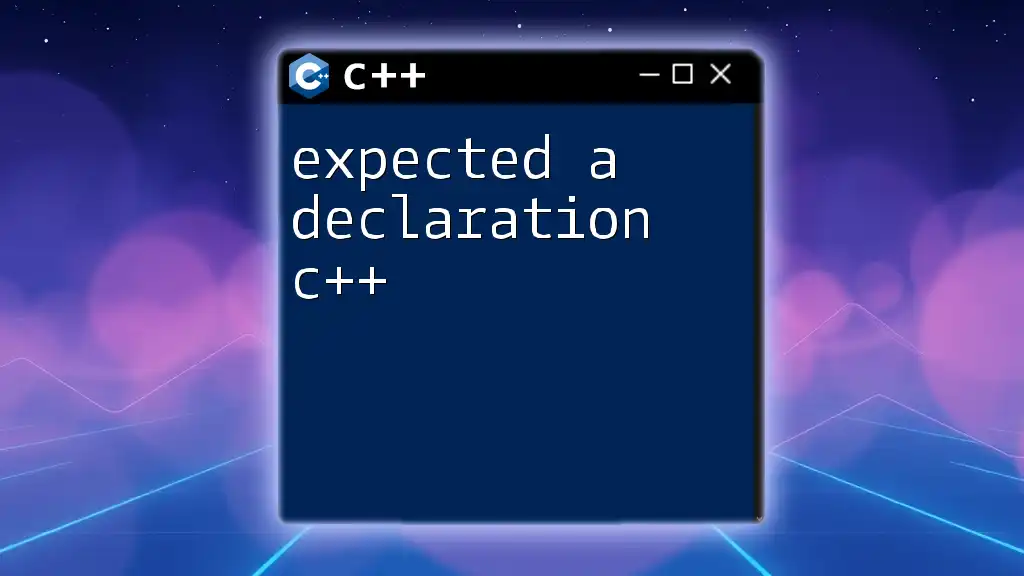
Class Declaration Best Practices
Naming Conventions
Using clear and descriptive naming conventions is essential in class declarations. Class names should generally be nouns and follow a consistent style, such as CamelCase, which enhances readability and maintains a standard throughout the codebase. For example, `Student`, `Calculator`, or `Employee`.
Use of Access Specifiers
Applying access specifiers judiciously is key to maintaining proper encapsulation. By marking member variables as private and exposing only necessary functions as public, developers can protect data integrity and control the interactions between classes.
- Public: Use for functions that should be accessible from outside.
- Private: Use for member variables to protect their integrity.
- Protected: Use for variables that should be available to derived classes.
Code Readability
Improving code readability boosts maintainability. Maintaining a consistent style, proper indentation, and adding comments where necessary can greatly enhance the understanding of the class declaration.
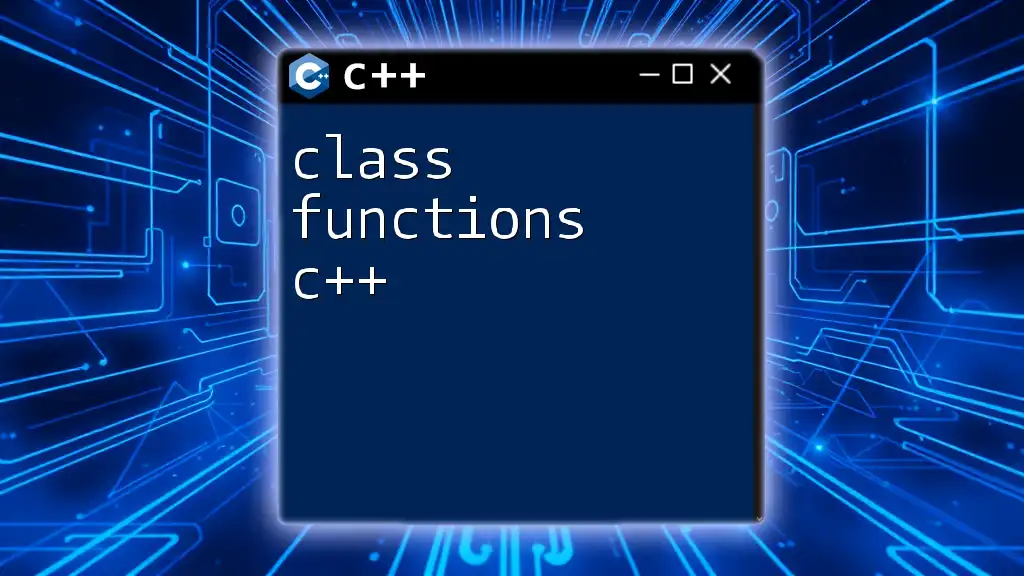
Common Mistakes in Class Declaration
Forgetting to Include Header Files
One common mistake is neglecting to include essential header files, such as `#include <iostream>`, which can lead to compilation errors. Always ensure that required headers are included at the top of your C++ files.
Misusing Access Specifiers
Developers may err by incorrectly applying access specifiers. For instance, declaring member variables public when they should be private can expose sensitive data, leading to fragile code. Consistently reviewing access can prevent such oversights.
Incorrect Syntax
Syntax errors often occur in class declarations, such as missing semicolons or mismatched brackets. Carefully verifying the structure and syntax of your class declaration can save debugging time.
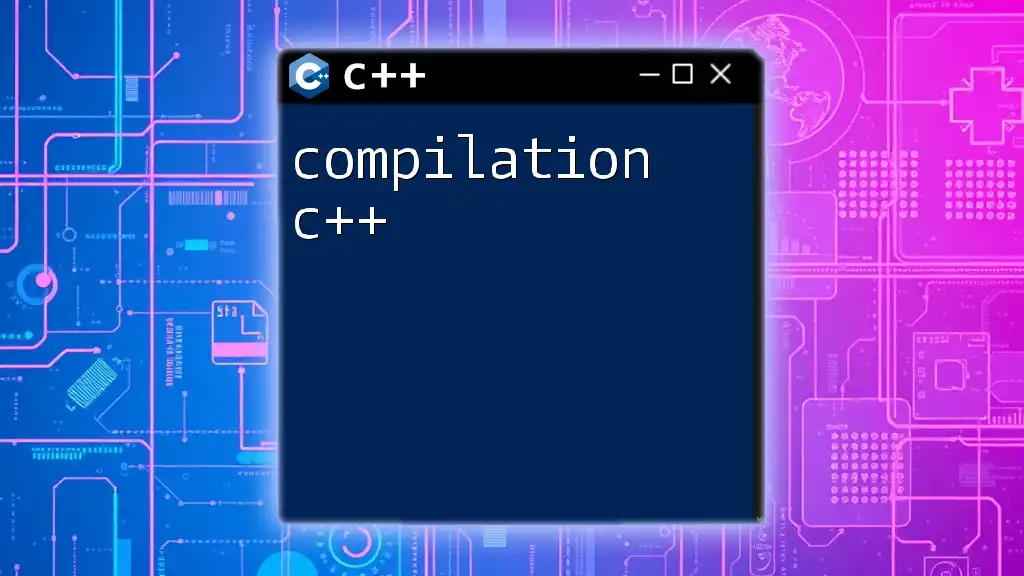
Summary
Recap of Key Points
In this guide, we've explored the intricacies of class declaration in C++, covering fundamental concepts such as syntax, components, best practices, and common pitfalls. By mastering these topics, you can establish a solid foundation for object-oriented programming in C++.
Encouragement to Practice
The best way to solidify understanding is through practice. Start by creating your own class declarations, try to implement various member functions, and utilize access specifiers effectively.
Call to Action
Stay tuned for more tutorials and resourceful content at our company as we continue to unravel the power of C++ and help you excel in mastering its complexities.