A class template in C++ allows you to create a blueprint for a class that can operate with any data type, enabling code reusability and flexibility.
Here's a simple example:
#include <iostream>
using namespace std;
template <typename T>
class Box {
public:
Box(T value) : value(value) {}
T getValue() { return value; }
private:
T value;
};
int main() {
Box<int> intBox(123);
Box<string> strBox("Hello");
cout << intBox.getValue() << endl; // Outputs: 123
cout << strBox.getValue() << endl; // Outputs: Hello
return 0;
}
What is a Class Template in C++?
A class template in C++ is a blueprint for creating classes based on types specified at compile time. This allows developers to create a single class definition that can work with any data type. The significance of class templates lies in their ability to provide code reusability and type safety, enabling developers to write more generic code with reduced redundancy.
Advantages of Using Class Templates
- Code Reusability: Templates allow you to write a class once and use it with any data type without rewriting the class for each type.
- Type Safety: Class templates are type-checked at compile time, which helps catch errors early in the development process.
- Flexibility: You can create classes that can handle different types or sizes, making your code more adaptable to future changes.
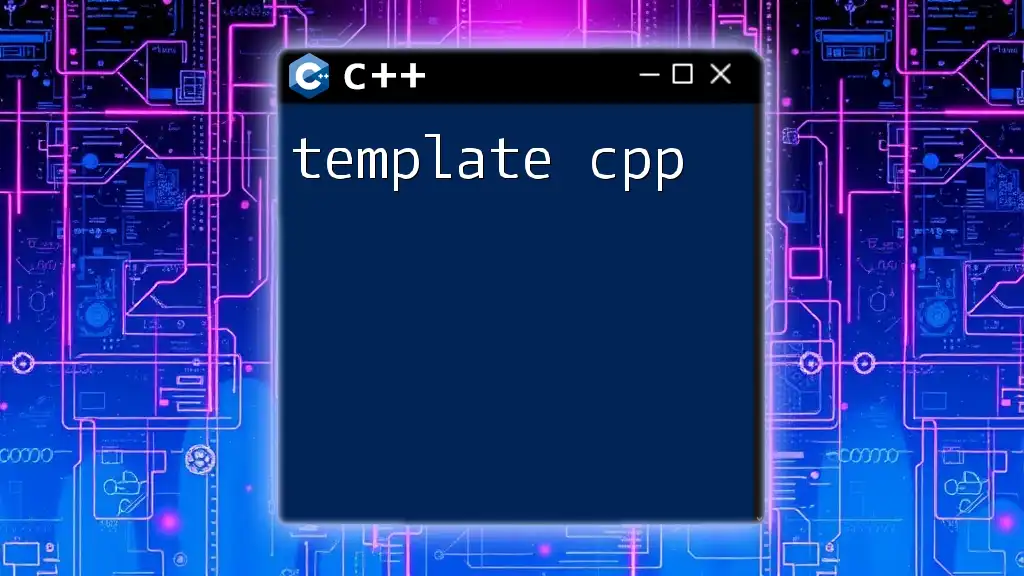
Basics of C++ Class Templates
Syntax of Class Template in C++
The syntax for defining a class template in C++ is straightforward. It begins with the `template` keyword followed by template parameters enclosed within angle brackets (`<>`). Here's the general structure:
template <typename T>
class ClassName {
// Members and methods
};
Example of Template Class in C++
Here's a simple example of a template class called Box:
template <typename T>
class Box {
public:
T value;
Box(T val) : value(val) {}
};
In this example, the class Box can store a value of any type, as specified during instantiation.
How to Instantiate a Template Class
Instantiating template classes involves specifying the type when creating an instance. Here are a couple of examples:
Box<int> intBox(123);
Box<std::string> strBox("Hello, World!");
In these examples, intBox holds an integer, while strBox holds a string.
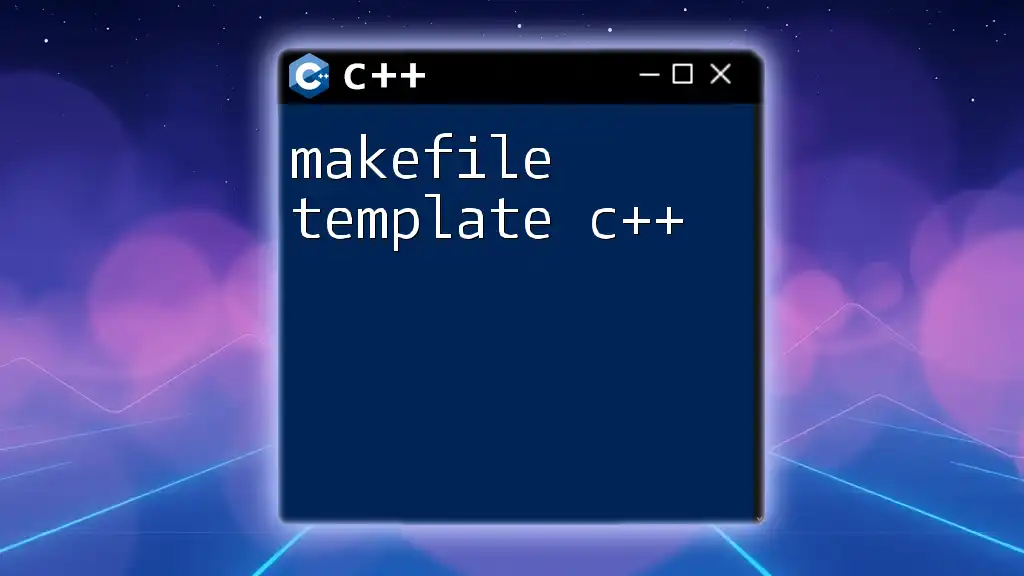
C++ Template Class Features
Default Template Parameters
C++ allows you to specify default template parameters. This feature can simplify class usage by providing a default data type if none is specified.
template <typename T = int>
class DefaultBox {
public:
T value;
DefaultBox(T val) : value(val) {}
};
In this example, if you create a DefaultBox without specifying a type, it will default to `int`.
Non-Type Template Parameters
Non-type template parameters allow you to pass constant values as template arguments, which can be particularly useful for defining classes with fixed sizes or limits.
template <typename T, int N>
class Array {
public:
T arr[N];
};
In this case, Array requires an integer N that determines the number of elements the array can hold.
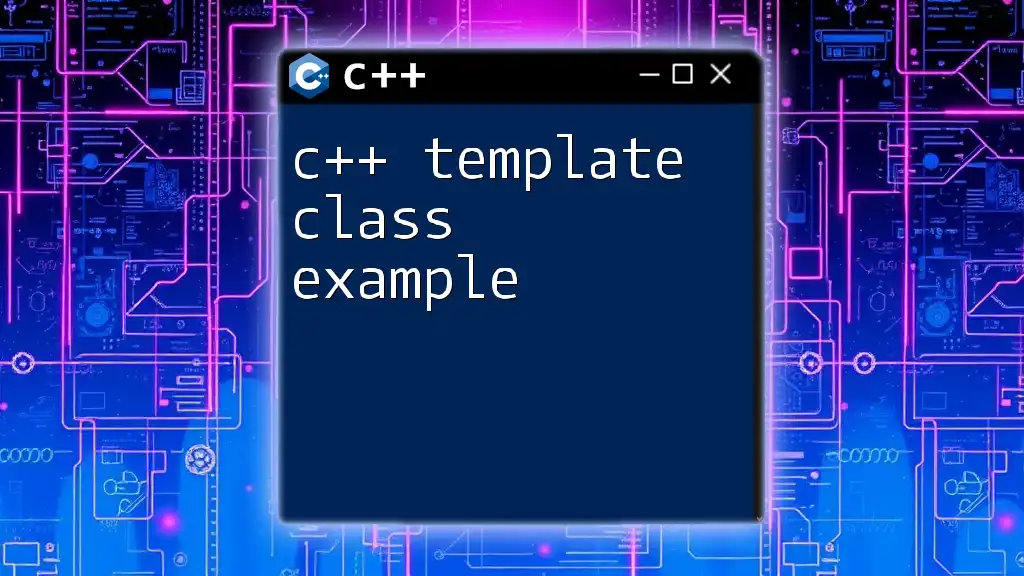
Advanced Class Template Concepts
Member Functions in Template Class
A class template can also have member functions that are templated. This not only increases flexibility but also allows the same member function to operate on different types.
template <typename T>
class Calculator {
public:
T add(T a, T b) { return a + b; }
};
Here, the add member function can add integers, floats, or any other type specified as T.
Template Specialization
Template specialization allows you to define specific implementations of a template for certain data types. This can be achieved through full specialization or partial specialization.
Full Specialization involves creating a distinct implementation for a specific data type.
template<>
class Calculator<std::string> {
public:
std::string add(std::string a, std::string b) {
return a + " " + b;
}
};
In this instance, we provide a specialist version for adding string values, differentiating it from the general implementation for other types.
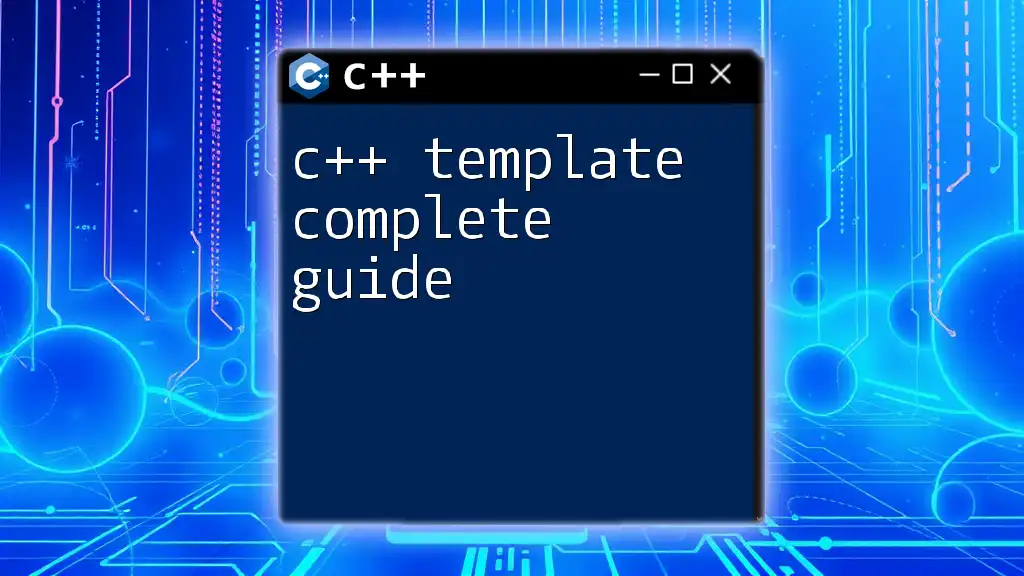
Practical Applications of C++ Template Classes
Creating Generic Data Structures
Class templates are invaluable when creating generic data structures, such as stacks or queues. These structures can manage data while maintaining type safety, which is crucial for avoiding runtime errors.
Template Classes in the Standard Template Library (STL)
The Standard Template Library (STL) is rich with templated classes that simplify various programming tasks. Examples include vector, list, and map, which provide developers with a robust set of tools to handle common data structures in a flexible and efficient manner.
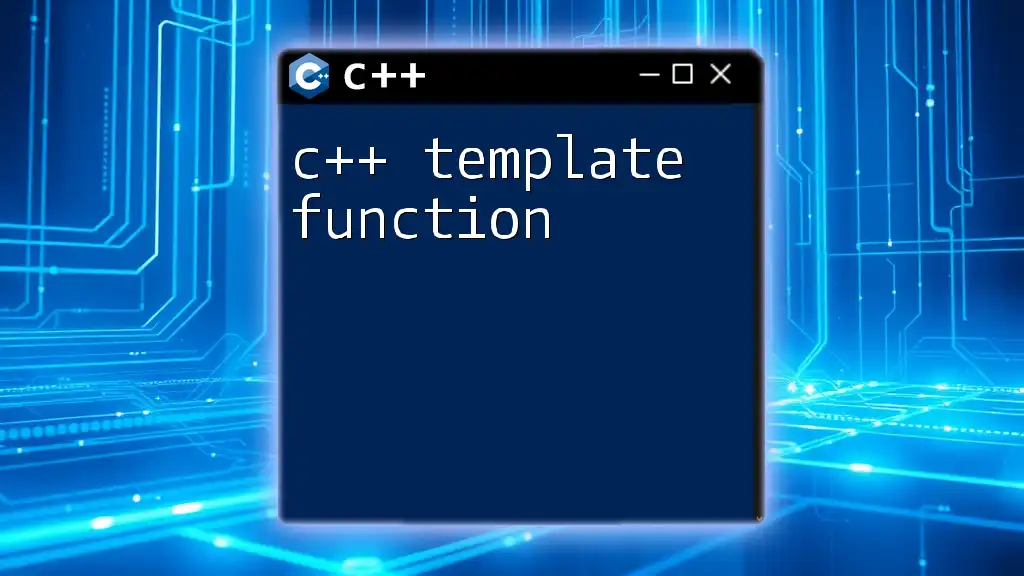
Best Practices for Using Class Templates
Naming Conventions for Template Classes
Maintaining consistent naming conventions for template classes is essential for enhancing readability and maintainability. A common practice is to use a prefix `T`, such as TBox for a templated box class, which indicates that the class is generic.
When to Use Templates vs. Inheritance
While both templates and inheritance serve to promote code reuse, they have distinct applications. Use templates when:
- You need type flexibility without changing the implementation logic.
- You are working on generic algorithms that require different data types.
Use inheritance when you have a clear hierarchical relationship among classes and want to share common behavior or attributes.
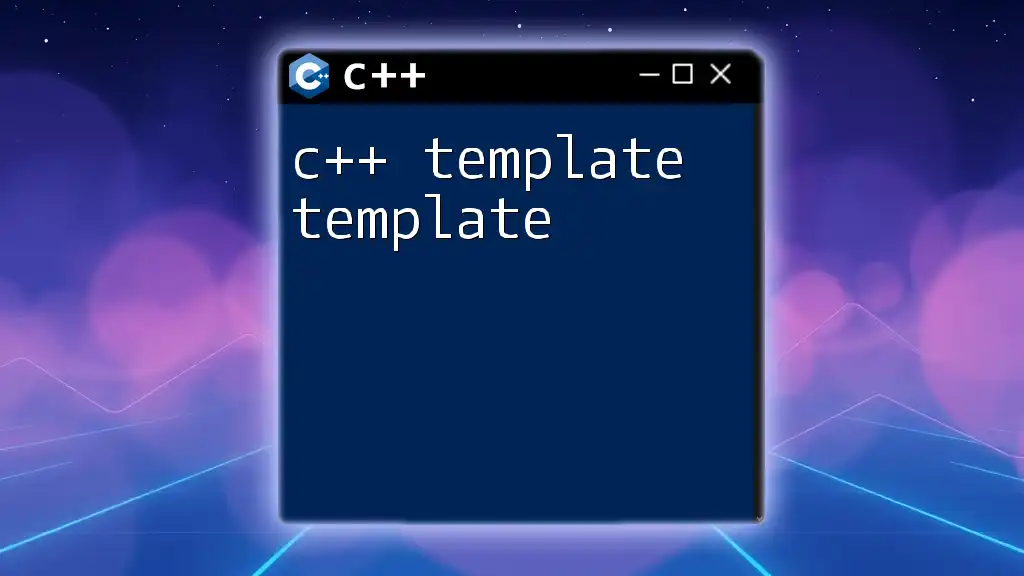
Common Pitfalls and Troubleshooting
Template Compilation Errors
Template programs can generate complex error messages, especially for novice programmers. Common pitfalls include:
- Forgetting to specify template arguments during instantiation.
- Mismatched types during function calls.
Analyzing error messages and incrementally building more complex templates can help mitigate these issues.
Performance Considerations
Using templates can lead to increased compilation time and larger binary sizes because the compiler generates separate versions of the template for each type used. Careful design and optimization are necessary for maintaining efficiency.
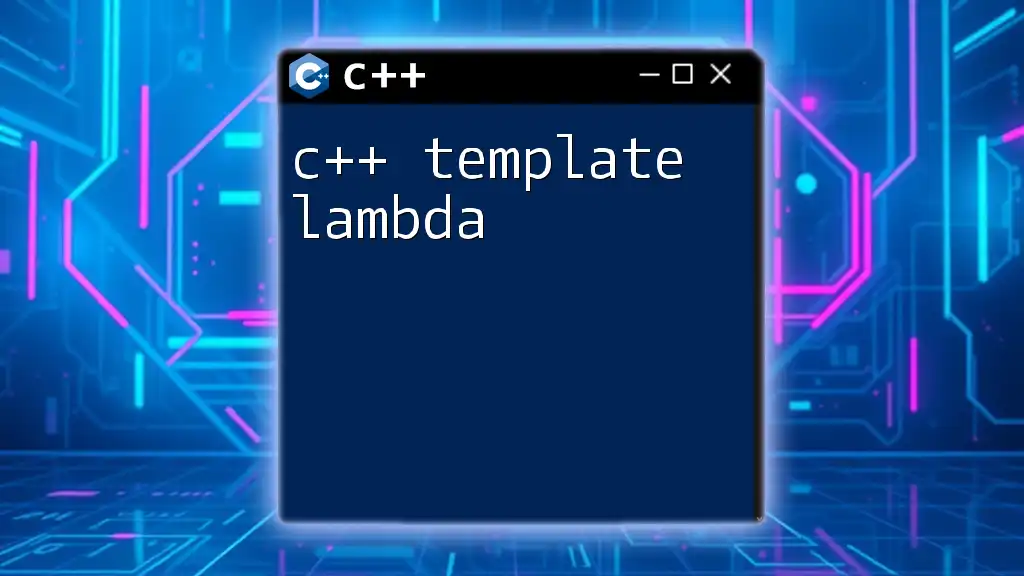
Conclusion
The concept of class template cpp provides a powerful tool for developing flexible and reusable code that can handle various data types efficiently. Understanding the basics of class templates, their syntax, advantages, and how to implement them effectively can enhance your C++ programming skills significantly. By mastering these concepts, you can take full advantage of the strengths that this feature offers in your software development projects.
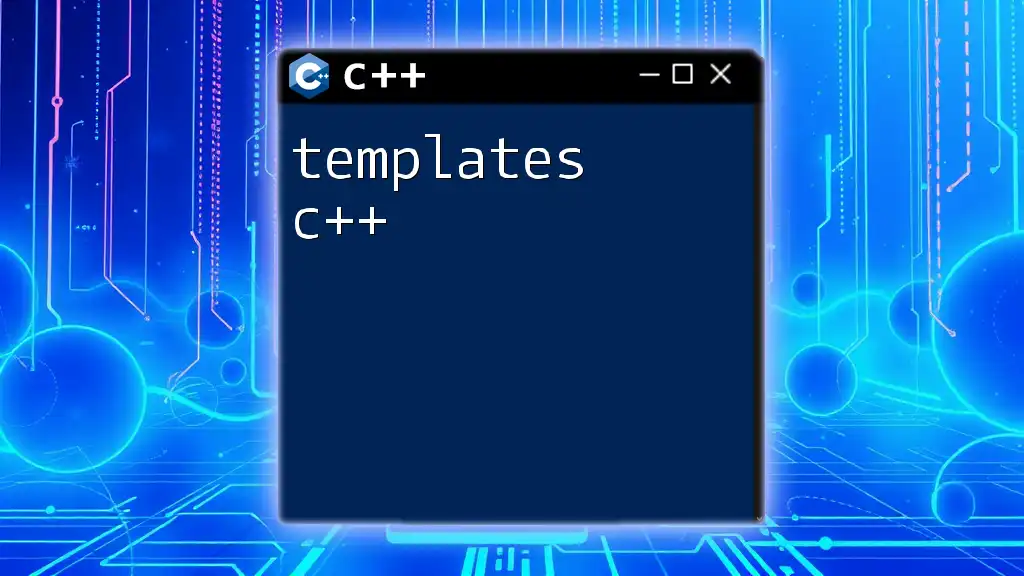
Additional Resources
For further learning, consider consulting advanced programming textbooks that focus on C++ templates, joining online forums for discussions, or exploring code repositories for examples of template class implementations. Expanding your knowledge in this area will aid in mastering class templates in C++ and elevating your programming capabilities.