In C++, classes are user-defined data types that encapsulate data and functions, allowing for the creation of objects with specific attributes and behaviors.
Here's a simple example of a class in C++:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
Understanding the Basics of a Class
What is a Class?
A class in C++ is a blueprint for creating objects (instances). It encapsulates data for the object and methods to manipulate that data. Classes serve as a fundamental component of Object-Oriented Programming (OOP) in C++, allowing developers to create user-defined types that model real-world entities.
The relationship between classes and objects is similar to how a blueprint relates to a physical building. Just like a blueprint outlines the structure and features of a house, a class defines the properties and behaviors of objects created from it.
Example: Think of a class as a cookie cutter. The cookie cutter defines the shape and size of cookies that can be produced, while each cookie represents an individual object created from that class.
Anatomy of a Class
To fully understand classes in C++, it is essential to know the key components that make up a class:
- Attributes (Data Members): These are the variables that hold the state of an object.
- Methods (Member Functions): These functions define the behaviors of the object.
Consider the following code snippet that illustrates how a simple class can look in C++:
class Car {
public:
string model;
int year;
void displayDetails() {
cout << "Model: " << model << ", Year: " << year << endl;
}
};
In this example, the `Car` class has two attributes: `model` and `year`, along with a method to display the car's details.

How to Create a Class in C++
The Syntax of Class Creation in C++
Creating a class in C++ follows a straightforward syntax. The general structure begins with the keyword class, followed by the class name, and then a set of curly braces that enclose the attributes and methods. Access specifiers (`public`, `private`, and `protected`) determine the visibility of the class members.
Step-by-Step Guide: Creating a Class
To illustrate the class creation process, consider the following example of a `Student` class:
class Student {
private:
string name;
int age;
public:
void setDetails(string n, int a) {
name = n;
age = a;
}
void displayInfo() {
cout << "Name: " << name << ", Age: " << age << endl;
}
};
In this declaration:
- The attributes `name` and `age` are private, meaning they cannot be accessed directly from outside the class.
- The setDetails method allows setting the values for these private members, while the displayInfo method retrieves and displays them.
How to Use Classes in C++
Once created, using a class involves instantiating objects from it. This is a straightforward process:
Student student1;
student1.setDetails("Alice", 20);
student1.displayInfo();
In this example, an object `student1` is created from the `Student` class, and methods are called to set and display the student’s information.

Advanced Class Features in C++
Constructors and Destructors
Classes in C++ can have special member functions known as constructors and destructors.
- Constructor: A constructor initializes an object when it is created. It can be default (no parameters) or parameterized (with parameters).
- Destructor: A destructor cleans up when an object is destroyed, freeing up any resources that were allocated.
Here’s an example using a `Book` class:
class Book {
private:
string title;
public:
Book(string t) : title(t) {
cout << "Book created: " << title << endl;
}
~Book() {
cout << "Book destroyed: " << title << endl;
}
};
In this snippet, the constructor initializes the title of the book, while the destructor outputs a message when the book object is destroyed.
Inheritance in C++
Inheritance is a feature of OOP that allows a class to inherit properties and methods from another class, promoting code reuse and logical organization.
There are several types of inheritance, including single inheritance and multiple inheritance. Consider the following code demonstrating inheritance with a `Vehicle` base class and a `Bike` derived class:
class Vehicle {
public:
void display() {
cout << "This is a vehicle." << endl;
}
};
class Bike : public Vehicle {
public:
void show() {
cout << "This is a bike." << endl;
}
};
Here, `Bike` inherits from `Vehicle`, allowing it to access the `display` method while also defining its specific behavior.
Polymorphism with Classes
Polymorphism allows methods to act differently based on the object invoking them. C++ supports polymorphism through function overloading and overriding.
Overriding, which is particularly common in inheritance, lets derived classes provide specific implementations of a function defined in the base class. Here’s an example:
class Animal {
public:
virtual void sound() {
cout << "Animal sound" << endl;
}
};
class Dog : public Animal {
public:
void sound() override {
cout << "Bark" << endl;
}
};
In this case, calling the `sound` method on an instance of `Dog` will result in "Bark", demonstrating how derived classes can modify inherited behavior.
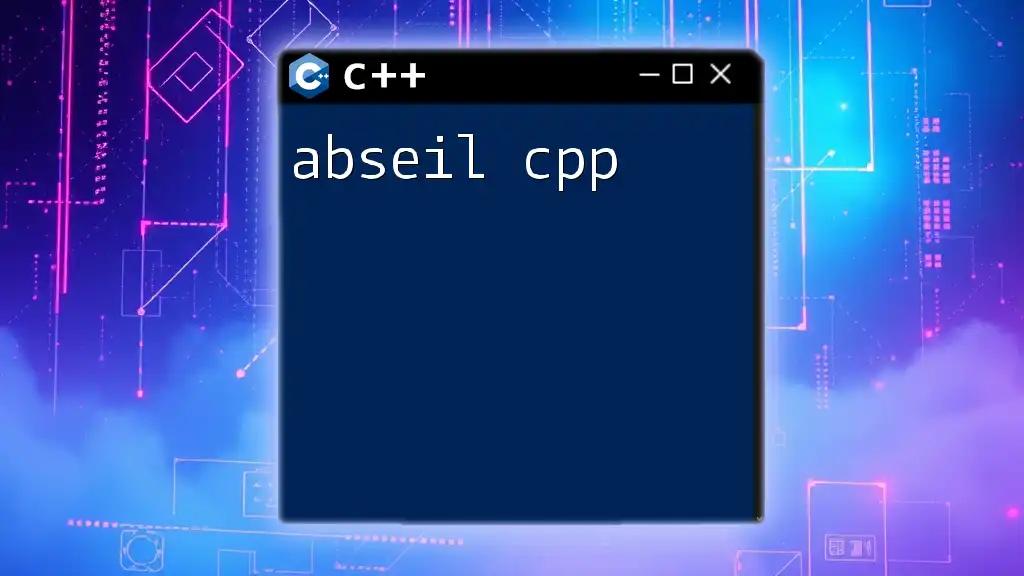
Tips for Writing Efficient Classes
Principles of Good Class Design
When designing classes in C++, follow these principles for clearer, more maintainable code:
- Encapsulation: Keep data hidden within the class, exposing only necessary interfaces through public methods.
- Cohesion: Ensure that the class focuses on a single responsibility, making it easier to understand and maintain.
Common Mistakes to Avoid
Even seasoned programmers can make mistakes when working with classes. Here are a few pitfalls to sidestep:
- Neglecting Access Modifiers: Ensure that critical attributes are kept private to maintain data integrity.
- Forgetting Constructors and Destructors: Always define the necessary constructors and destructors to manage resources effectively.
Example: Consider a class that defines attributes without proper access control or constructors, potentially leading to unexpected behaviors or resource leaks.
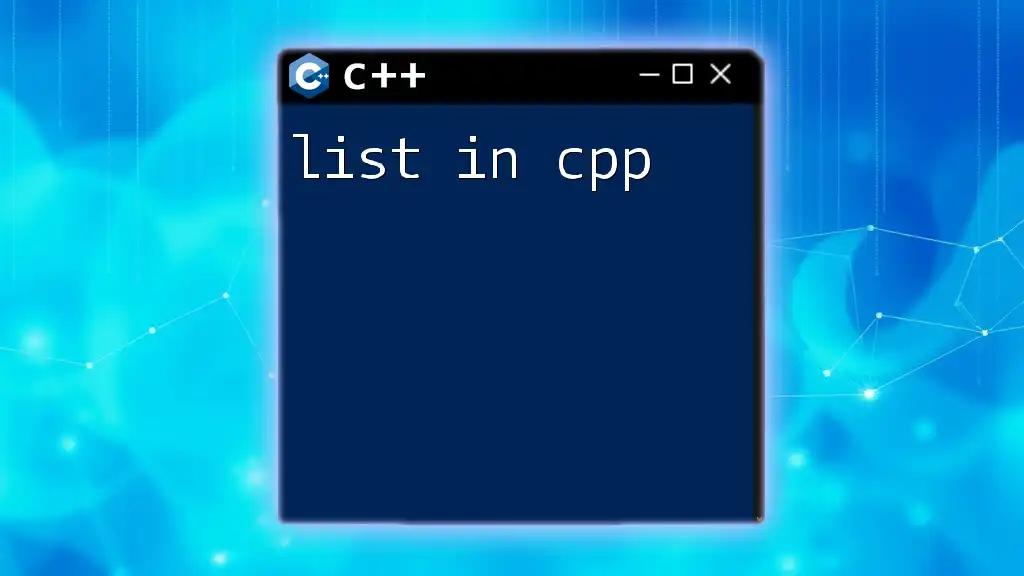
Conclusion
Classes are a foundational aspect of C++ programming and are crucial for implementing Object-Oriented principles. Understanding how to create and use classes effectively can significantly enhance your programming skills. As you continue to practice, try to experiment with different class designs and functionalities.
Call to Action
Engage with the community by sharing your experiences with classes in C++, asking questions about challenges you've faced, or providing tips based on your learning journey!