In C++, the `if-else` statement allows you to execute one block of code if a condition is true and another block if it's false, helping you control the flow of your program. Here's a simple example:
#include <iostream>
using namespace std;
int main() {
int number = 10;
if (number > 0) {
cout << "The number is positive." << endl;
} else {
cout << "The number is non-positive." << endl;
}
return 0;
}
Understanding Control Flow Statements
What are Control Flow Statements?
Control flow statements dictate the order in which code executes. By deciding which block of code to run based on specific conditions, these statements play a crucial role in programming logic. The most common types of flow statements are conditional statements, looping statements, and jump statements.
Categories of Control Flow Statements
- Conditional Statements: These determine the course of action based on whether a condition is true or false. Examples include "if", "else if", and "else".
- Looping Statements: These repeatedly execute a block of code as long as a specified condition is met. Examples are "for", "while", and "do-while" loops.
- Jump Statements: These can alter the flow of execution, such as "break" to exit loops prematurely or "continue" to skip the current iteration.
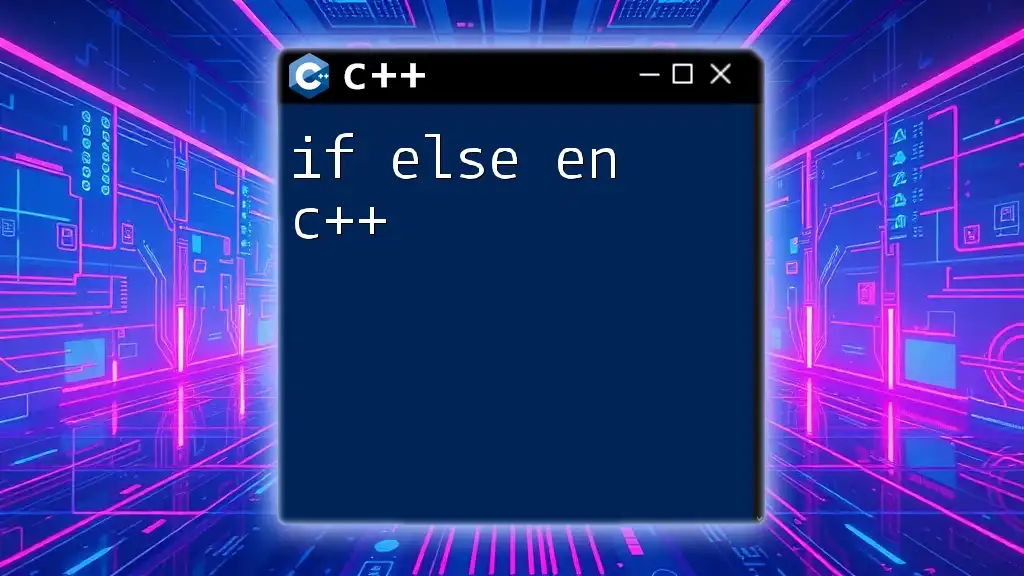
The "If" Statement in C++
Syntax of the "If" Statement
The syntax of the "if" statement is straightforward:
if (condition) {
// code to execute if condition is true
}
In this structure, `condition` is evaluated, and if it is true, the code block within the braces gets executed. If false, the program skips to the following statement outside the if block.
Example of a Simple "If" Statement
Consider the following code snippet:
int age = 18;
if (age >= 18) {
std::cout << "You are an adult." << std::endl;
}
In this example, the variable `age` is checked to see if it is greater than or equal to 18. Since the condition is true, the output will be "You are an adult." This illustrates how the "if" statement allows you to execute code conditionally.
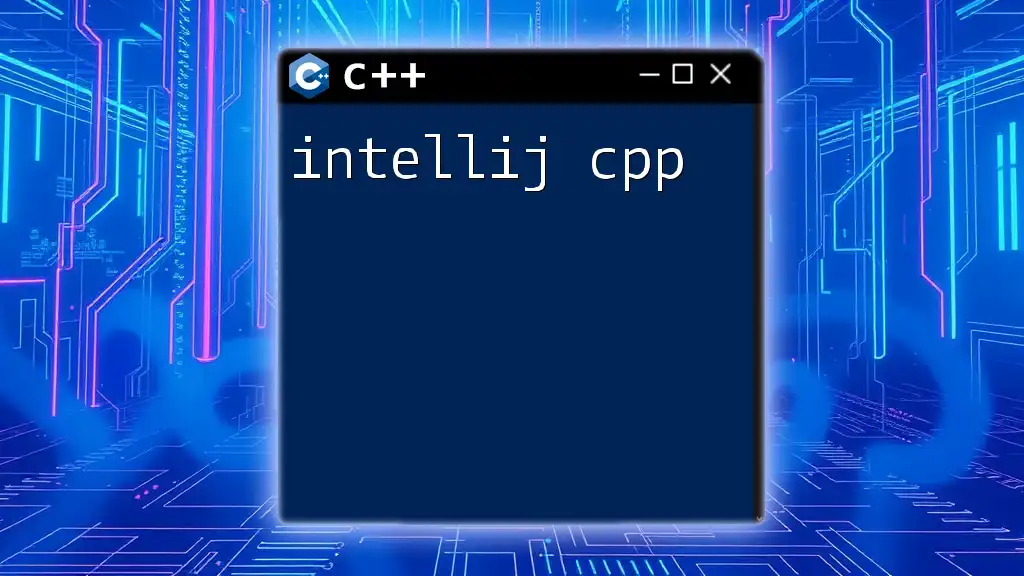
The "If Else" Statement in C++
Syntax of the "If Else" Statement
The `if else` statement allows for two possible branches of execution:
if (condition) {
// code to execute if condition is true
} else {
// code to execute if condition is false
}
This structure enables the programmer to handle alternative paths in their code.
Example of an "If Else" Statement
Here's an example demonstrating this structure:
int age = 16;
if (age >= 18) {
std::cout << "You are an adult." << std::endl;
} else {
std::cout << "You are a minor." << std::endl;
}
In this scenario, the condition checks if `age` is 18 or more. Since `age` is 16, the `else` block executes, resulting in the output, "You are a minor." This highlights the fundamental logic behind the `if else` statement, making decisions more efficient and precise.
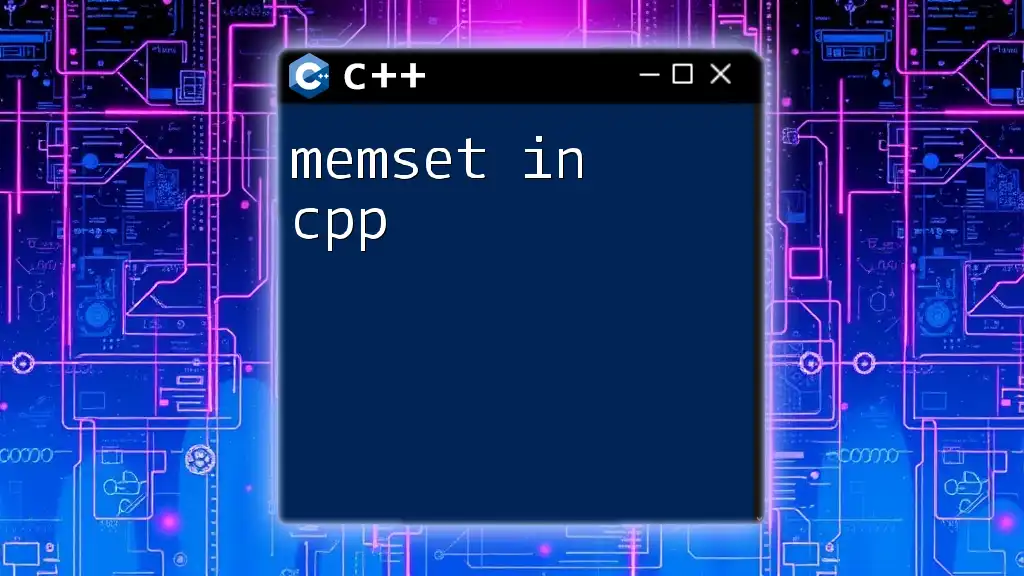
The "Else If" Ladder
Using "Else If" to Handle Multiple Conditions
When you want to evaluate multiple conditions, the `else if` construct becomes your ally. The syntax appears as follows:
if (condition1) {
// code for condition1
} else if (condition2) {
// code for condition2
} else {
// code if none of the conditions are true
}
This structure is beneficial for cases where you have more than two possible paths.
Example of an "Else If" Ladder
Consider the following example:
int score = 85;
if (score >= 90) {
std::cout << "Grade: A" << std::endl;
} else if (score >= 80) {
std::cout << "Grade: B" << std::endl;
} else if (score >= 70) {
std::cout << "Grade: C" << std::endl;
} else {
std::cout << "Grade: D" << std::endl;
}
In this snippet, the program evaluates the `score` variable against several thresholds. Since `score` is 85, the output will be "Grade: B." This example demonstrates how the `else if` ladder allows for versatile decision-making based on varying conditions.
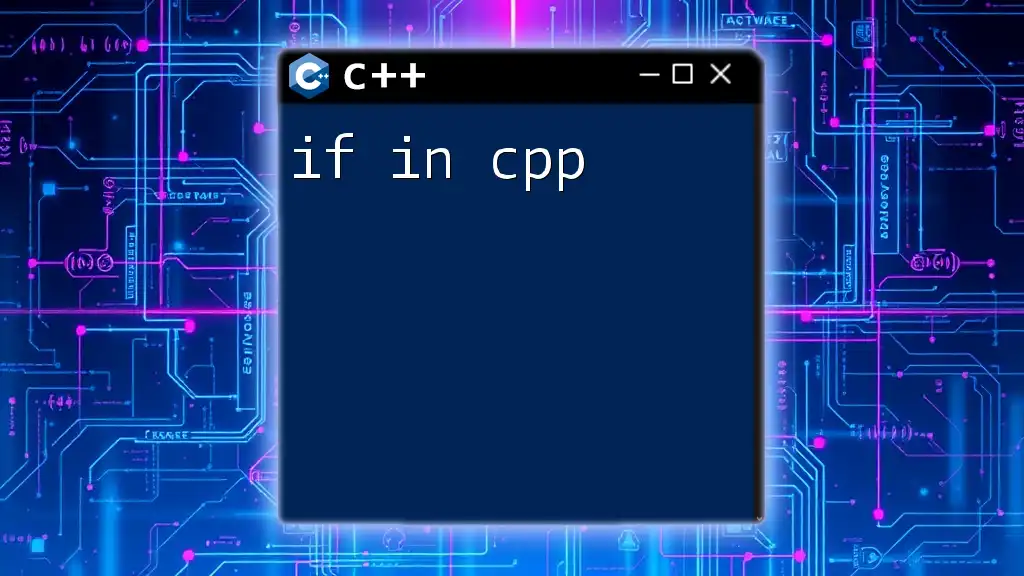
Nesting If Else Statements
What is Nesting?
Nesting occurs when an if statement is placed within another if statement. This allows for detailed checks and more granular decision-making based on multiple conditions. However, it can increase complexity and potential confusion if not managed carefully.
Example of Nested If Else Statement
int age = 20;
if (age >= 18) {
if (age >= 65) {
std::cout << "You are a senior citizen." << std::endl;
} else {
std::cout << "You are an adult." << std::endl;
}
} else {
std::cout << "You are a minor." << std::endl;
}
This code first checks if `age` is 18 or above. If true, it further checks if `age` is 65 or older before proceeding to print the corresponding output. In this case, the output will be "You are an adult." Nesting can effectively handle more specific conditions, but it’s essential to maintain readability in your code.
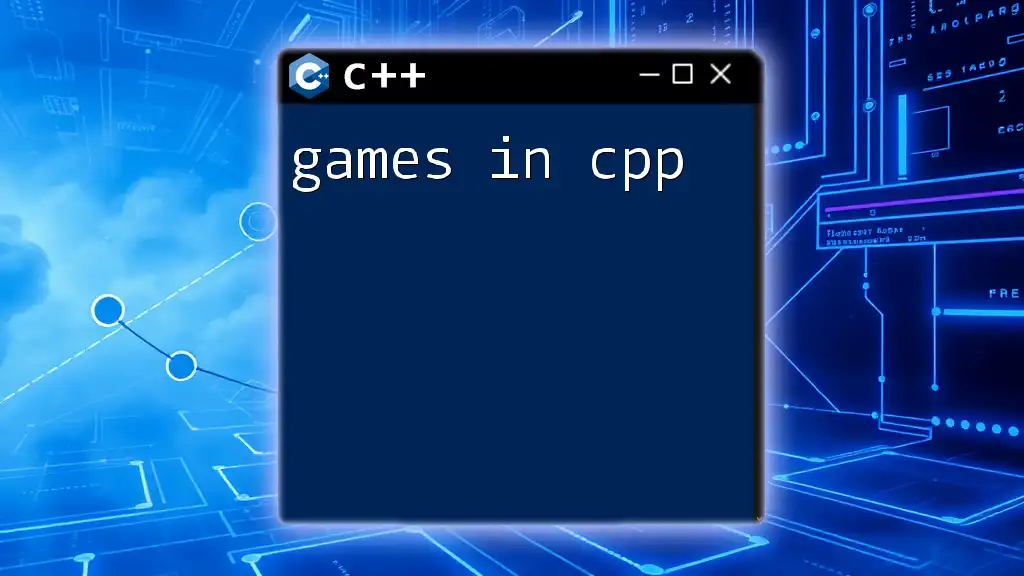
Logical Operators in If Statements
Understanding Logical Operators
Logical operators are powerful tools used to combine multiple conditions within `if` statements. The most common operators include:
- AND (&&): Evaluates to true if both conditions are true.
- OR (||): Evaluates to true if at least one of the conditions is true.
- NOT (!): Reverses the truth value of a condition.
Using Logical Operators in Conditions
Example of Combining Conditions
int age = 25;
bool isStudent = false;
if (age >= 18 && !isStudent) {
std::cout << "You are an adult and not a student." << std::endl;
}
In this example, the code checks if `age` is greater than or equal to 18 while ensuring `isStudent` is false. Since both conditions are true, the output will be "You are an adult and not a student." Logical operators enable more complex decision-making and refine the control flow of your program.
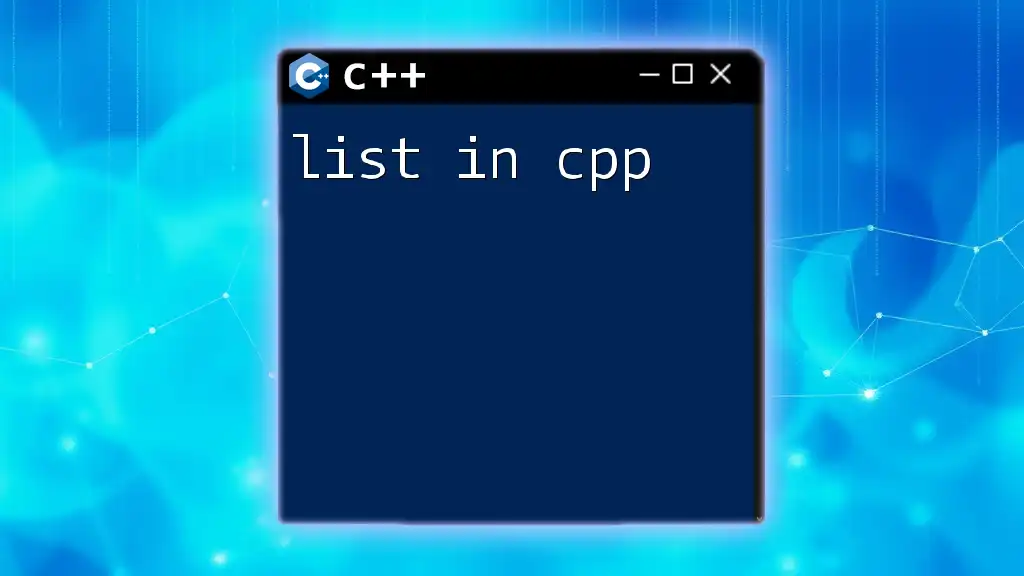
Common Mistakes with If Else Statements
Misplaced Braces
One of the most common mistakes when working with `if else` statements is improper placement of braces. This can lead to unexpected behavior or syntax errors. For example:
if (condition)
std::cout << "Hello";
std::cout << "World"; // This will always execute
In the above code, only the first `cout` is controlled by the `if`. To prevent such issues, always use braces, even for single-line statements.
Forgetting Condition Check
Logical errors can occur if you mistakenly leave out a condition or make incorrect assumptions about the values being checked. Thorough testing and debugging are key to mitigating such errors.
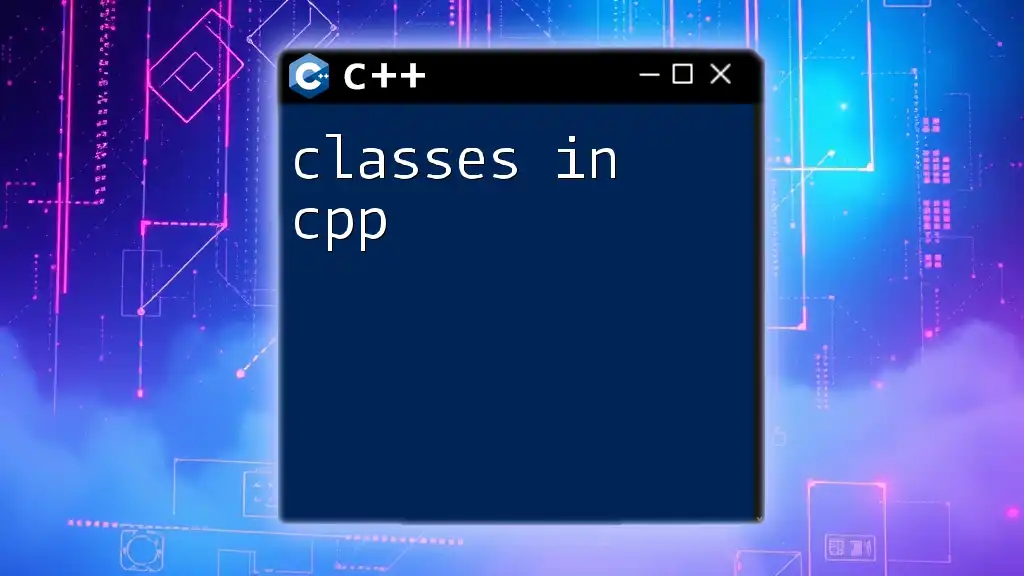
Conclusion
In summary, mastering the if else in cpp statements is essential for controlling the flow of execution within your program. With various constructs like `if`, `else if`, and nesting, you can handle complex decision-making scenarios efficiently. By leveraging logical operators, you elevate your code's capability to evaluate multiple conditions simultaneously.
The key takeaway is to practice writing your own `if else` statements, allowing you to become proficient in crafting logical constructs. Remember to explore the flexibility of nested statements and the importance of clarity in your code. Happy coding!
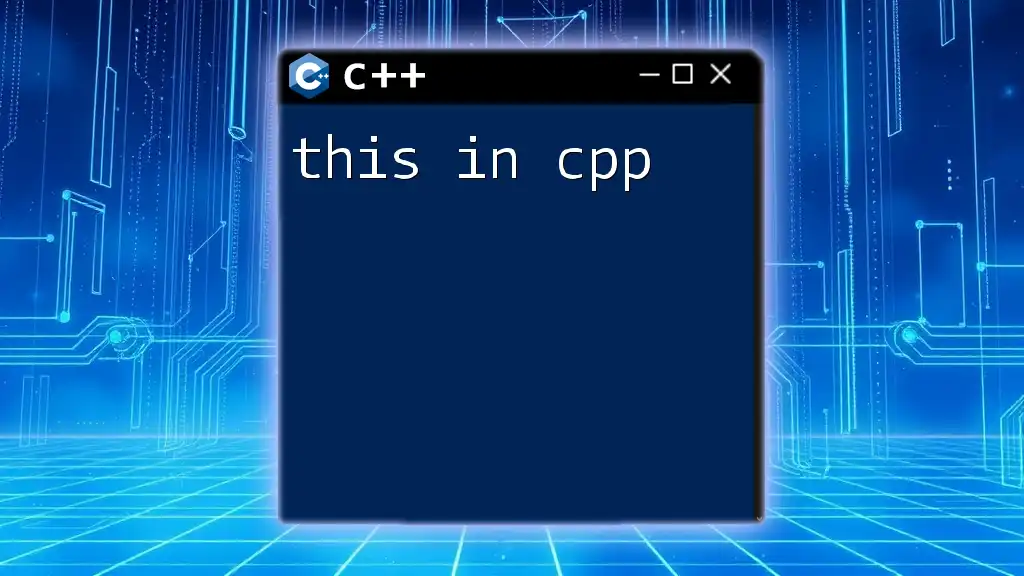
Further Learning Resources
To enhance your understanding of `if else in cpp`, consider exploring recommended books, online platforms, and coding practices. Engage in hands-on exercises to solidify your knowledge and improve your command over control flow statements in C++.
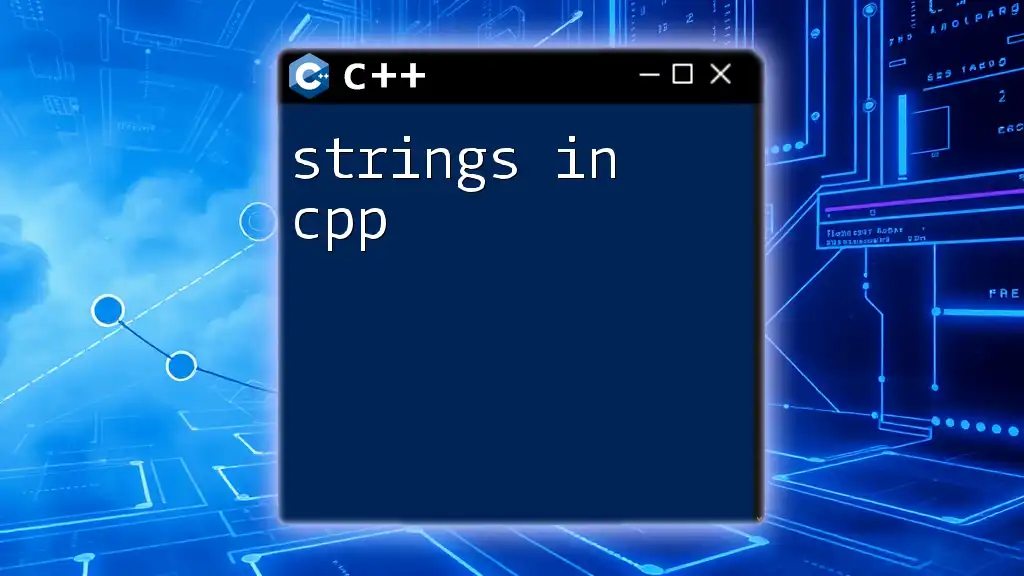
Call to Action
Now it’s your turn! Try writing your own `if else` statements and experiment with different conditions. Share your experiences or any questions you might have in the comments section below!