The `if else` statement in C++ allows you to execute different blocks of code based on whether a specified condition is true or false.
Here's an example of the syntax:
#include <iostream>
using namespace std;
int main() {
int number = 10;
if (number > 0) {
cout << "Number is positive." << endl;
} else {
cout << "Number is non-positive." << endl;
}
return 0;
}
Understanding the Basics of If Else Statements in C++
What is an If Else Statement?
An if else statement is a fundamental control structure in C++ that allows programmers to dictate the flow of execution based on specific conditions. It enables decision-making within a program, effectively directing the path that code will take based on whether a particular condition is true or false. Understanding this concept is crucial, as it forms the basis of most algorithms found in programming.
Basic Syntax of If Else Statement
The if else C++ syntax is straightforward and consists of a few key components. The basic structure is as follows:
if (condition) {
// code to execute if condition is true
} else {
// code to execute if condition is false
}
In this structure:
- The `if` keyword begins the conditional statement.
- The condition is an expression that evaluates to a boolean value—either true or false.
- The block of code within the curly braces `{}` will execute based on the evaluation of the condition. If the condition is true, the first block of code runs; otherwise, the second block executes.
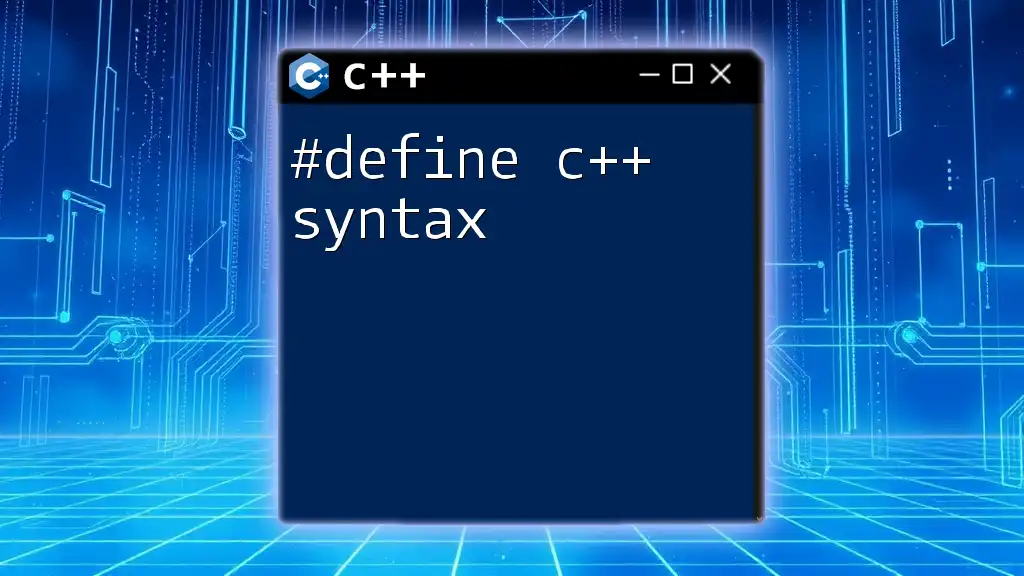
If and Else Statement in C++
Single If Statement
A single if statement executes a block of code only if the specified condition is true. For example, consider the following:
if (temperature > 30) {
cout << "It's a hot day!";
}
In this example:
- The code checks if `temperature` is greater than 30. If it is, the output will indicate a hot day.
- If the condition is false, no actions take place, and the program continues.
Compound If Else Statements
When you need to evaluate multiple conditions, compound if else statements come into play. The syntax allows for more granular decision-making:
if (score >= 90) {
cout << "Grade: A";
} else if (score >= 80) {
cout << "Grade: B";
} else {
cout << "Grade: C";
}
Here:
- The program first checks if `score` is 90 or above. If true, it prints "Grade: A".
- If the first condition fails, it checks the second condition for scores of 80 or above.
- If both conditions are false, it defaults to the `else` statement.
This approach allows for flexibility and clarity in handling multiple cases effectively.
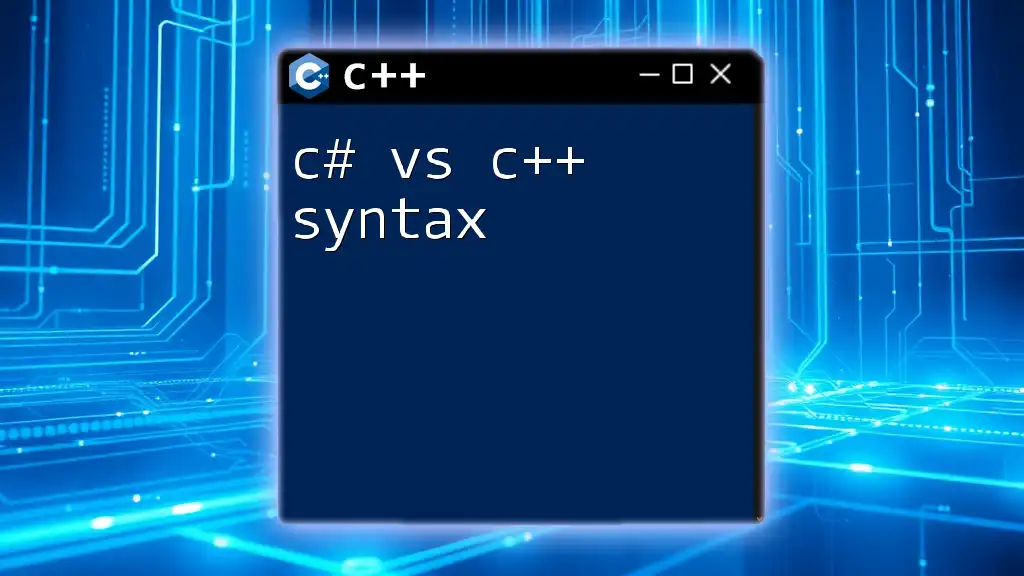
If Else Loop in C++
How If Else Statements Work with Loops
Integrating if else statements with loops enables dynamic decision-making during iteration. For example, here's how to evaluate whether numbers are even or odd within a loop:
for (int i = 1; i <= 10; i++) {
if (i % 2 == 0) {
cout << i << " is even." << endl;
} else {
cout << i << " is odd." << endl;
}
}
In the loop:
- Each iteration checks whether the loop variable `i` is divisible by 2.
- Based on the result, it categorizes `i` as either even or odd, producing output for each iteration.
This method showcases the power of if else C++ syntax when combined with loops to handle repetitive tasks and conditions seamlessly.
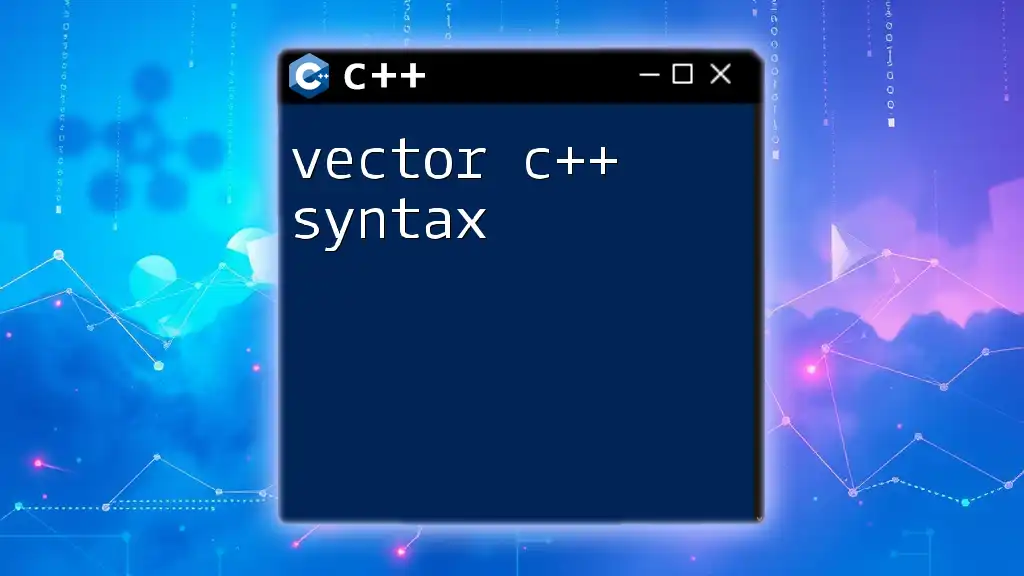
Nested If Else Statements
What are Nested If Else Statements?
Nested if else statements allow for more complex conditional logic by placing an if else structure within another. While powerful, they can lead to confusing code if overused. Here’s an example:
if (x > 0) {
cout << "X is positive." << endl;
if (x > 100) {
cout << "X is greater than 100." << endl;
}
} else {
cout << "X is non-positive." << endl;
}
In this nested example:
- The first condition checks if `x` is positive.
- If true, it checks again if `x` is greater than 100, allowing for specific messaging based on magnitude.
- If `x` is not positive, it defaults to the else statement.
While nesting can be useful, too many nested conditions may lead to less readable code, so use them judiciously.
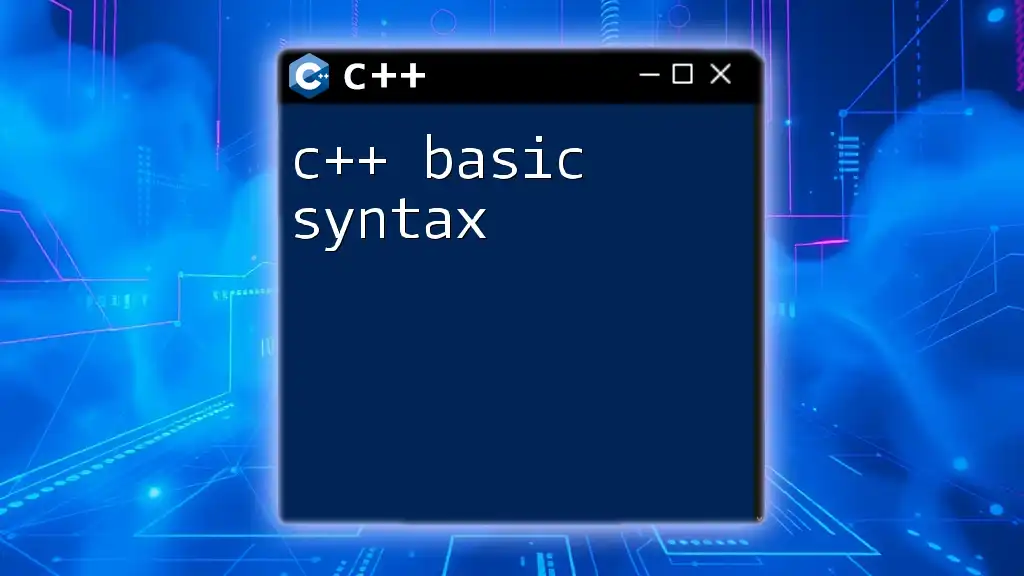
Best Practices for Using If Else Statements in C++
Writing Clear and Concise Conditions
To maintain code readability, prioritize writing conditions that are clear and convey intent. Avoid overly complex expressions within the if statement, as they can obscure the logic of your code. A simple, well-named boolean variable can often make your conditions easier to understand.
Avoiding Deep Nesting
While nesting is sometimes necessary, avoid creating deep nested structures, as they can quickly lead to code that is hard to follow. Refactor complex logic into separate functions or consider other control structures, like switch statements, for clarity and maintainability.
Using Switch Statements as Alternatives
In scenarios where a variable can have multiple discrete values to check, a switch statement may be a more appropriate choice than an if else structure. It is particularly useful when comparing the same variable against different constants.
Here is a brief example:
switch (grade) {
case 'A':
cout << "Excellent!";
break;
case 'B':
cout << "Well done!";
break;
default:
cout << "Keep trying!";
break;
}
Switch statements can enhance both clarity and performance in certain cases compared to a long chain of if else statements.
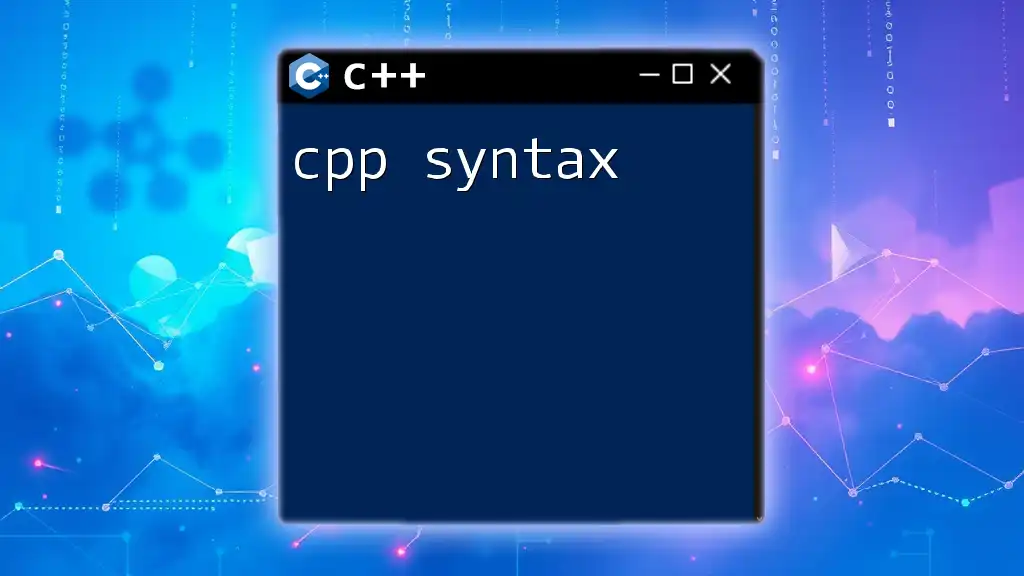
Troubleshooting Common Errors
Common Issues with If Else Statements
While using if else statements, several common mistakes may arise:
- Missing braces: In C++, omitting braces for single-body statements can lead to unexpected behavior or errors. Always use braces for clarity, even with a single statement.
- Logic errors: Always ensure the logical conditions accurately reflect the intended program flow. Debugging tools and print statements are invaluable for figuring out where logic has gone awry.
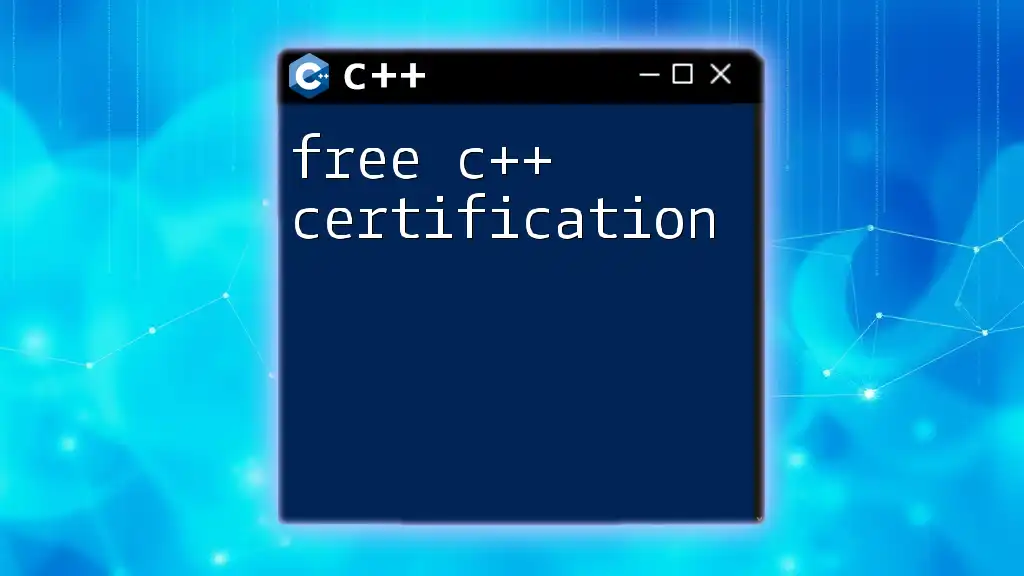
Conclusion
Understanding the if else C++ syntax is crucial for mastering control flow in your programming projects. This foundational concept allows for powerful decision-making capabilities, enabling you to create responsive, flexible applications. Practice implementing if else statements in various contexts to solidify your understanding and turn theoretical knowledge into practical skills. With continued exploration, you'll discover many ways to utilize this vital component of C++.