The `if else` statement in C++ allows you to execute different blocks of code based on whether a specified condition evaluates to true or false.
Here's a code snippet to illustrate its use:
#include <iostream>
using namespace std;
int main() {
int number = 10;
if (number > 0) {
cout << "The number is positive." << endl;
} else {
cout << "The number is not positive." << endl;
}
return 0;
}
Understanding the Basics of Conditional Statements
Conditional statements are fundamental to programming paradigms in C++. They allow the program to make decisions based on certain conditions. The main purpose of these statements is to control the flow of execution in your code by executing specific blocks of code depending on whether conditions are true or false.
Types of Conditional Statements in C++
In C++, the primary conditional statements include if, else, and else if. Each of these plays a significant role in constructing a logical flow based on the criteria defined by the programmer.

The Syntax of If Else in C++
The syntax for the if else statement is quite straightforward. Understanding its basic structure is essential for effective programming in C++.
Here’s the basic structure:
if (condition) {
// execute this block
} else {
// execute this block
}
- if is followed by a condition enclosed in parentheses.
- If the condition evaluates to true, the block of code within the first set of curly braces is executed.
- If the condition is false, the block of code within the else section executes.
Using If and If Else Statement in C++
When to utilize if else statements can greatly impact how a program operates. They are particularly useful in situations where multiple outcomes can arise from a single decision point.
Consider the following example:
int number = 10;
if (number > 5) {
std::cout << "Number is greater than 5";
} else {
std::cout << "Number is 5 or less";
}
In this code snippet:
- The condition checks if number is greater than 5.
- Since it is true in this case, the output will be "Number is greater than 5".
This demonstrates how simple if else statements allow you to control output based on conditions.

Exploring Else If in C++
What is Else If in C++?
The else if statement provides a way to evaluate multiple conditions sequentially. This is particularly useful when you have more than two possible outcomes to consider.
C++ Else If Example
Here’s a practical example using else if:
int score = 85;
if (score >= 90) {
std::cout << "Grade: A";
} else if (score >= 80) {
std::cout << "Grade: B";
} else {
std::cout << "Grade: C";
}
In this example:
- The program first checks if score is greater than or equal to 90.
- If this condition is false, it checks if the score is 80 or above.
- If neither condition is met, the else block executes, implying a grade of C.
This hierarchical checking demonstrates how compactly multiple conditions can be managed using if, else if, and else.

Combining If Else and Else If Statements
C++ If Else If Else Structure
To construct more complex decision-making processes, you can combine multiple `if`, `else if`, and `else` statements. This structure is highly efficient in evaluating diverse scenarios.
Consider the following code snippet:
int age = 20;
if (age < 13) {
std::cout << "Child";
} else if (age < 20) {
std::cout << "Teenager";
} else {
std::cout << "Adult";
}
In this piece of code:
- The program checks the age category of the individual.
- The decision-making flows sequentially through the conditions, correctly identifying the age group as "Adult."
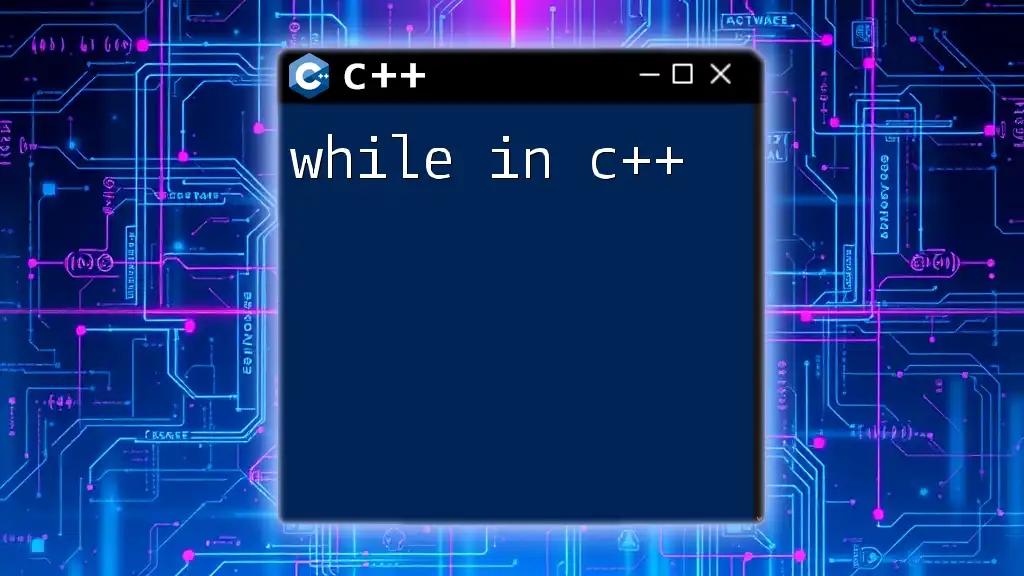
Common Mistakes While Using If Else in C++
While coding with if else statements, certain common mistakes may arise. Awareness of these pitfalls is crucial for developing debugging skills and writing cleaner code.
Mistakes to Avoid
- Missing braces: Often, beginners forget to use curly braces `{}` which can lead to errors in understanding which code belongs to which condition.
- Incorrect condition evaluation: Ensure that the conditions make logical sense and do not contradict each other.
Best Practices
- Indent your code consistently to enhance readability.
- Always use braces even for single-line conditions to avoid confusion in future modifications.
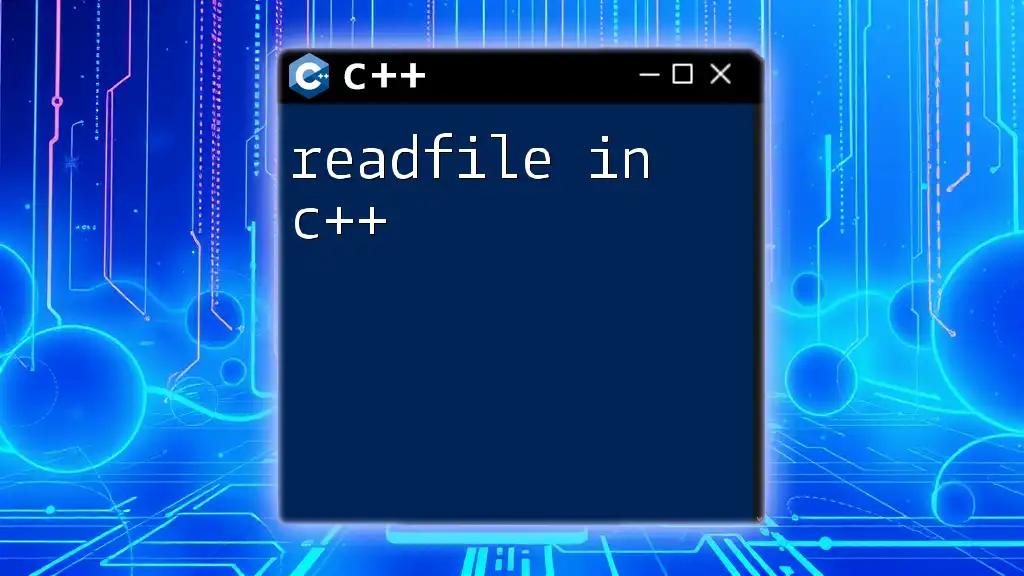
Advanced C++ If Else Usage
Nested If Statements
Nesting `if` statements is a technique where you place one `if` statement inside another. This can allow for more granular control over decision-making.
Here’s a simple demonstration:
int temperature = 30;
if (temperature > 20) {
if (temperature > 30) {
std::cout << "It's hot!";
} else {
std::cout << "It's warm.";
}
} else {
std::cout << "It's cold.";
}
In this example:
- The outer if checks if the temperature is above 20, then checks internally if it exceeds 30.
- This creates a more detailed and nuanced series of outputs based on the temperature.
Ternary Operators as an Alternative
C++ also supports the ternary operator which can simplify simple conditions. It serves as a compact shorthand for `if else` statements.
Here’s an illustration:
int number = 10;
std::cout << (number > 5 ? "Greater than 5" : "5 or less");
This single line effectively replaces what could be a longer `if else` structure, enhancing code brevity while maintaining readability.

Conclusion
In conclusion, mastering if else en C++ is essential for anyone looking to effectively control program flow in their coding projects. Understanding the syntax, usages, and common pitfalls equips developers to write more efficient and error-free programs. As with any programming concept, practice is key to becoming proficient. Consider experimenting with various conditions and scenarios to solidify your understanding and application of these essential control statements.

Additional Resources
To further deepen your understanding of if else statements, explore recommended books, online courses, and coding platforms dedicated to C++. Continuous learning and application will accelerate your coding skills and confidence in programming.