Nested if-else statements in C++ allow you to evaluate multiple conditions by placing an if-else statement within another if or else block. Here’s a simple example:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
if (number > 0) {
cout << "Positive number";
} else if (number < 0) {
cout << "Negative number";
} else {
cout << "Zero";
}
return 0;
}
What are Nested If Else Statements?
Nested if else statements are a crucial element in C++ programming that allows developers to create conditional logic capable of handling multiple scenarios. Essentially, nested if else statements provide a way to check a condition, and if that condition is true, execute a block of code; if it is false, check another condition, and so on. This nesting creates a hierarchy of decisions that can help in coding more complex logical flows.
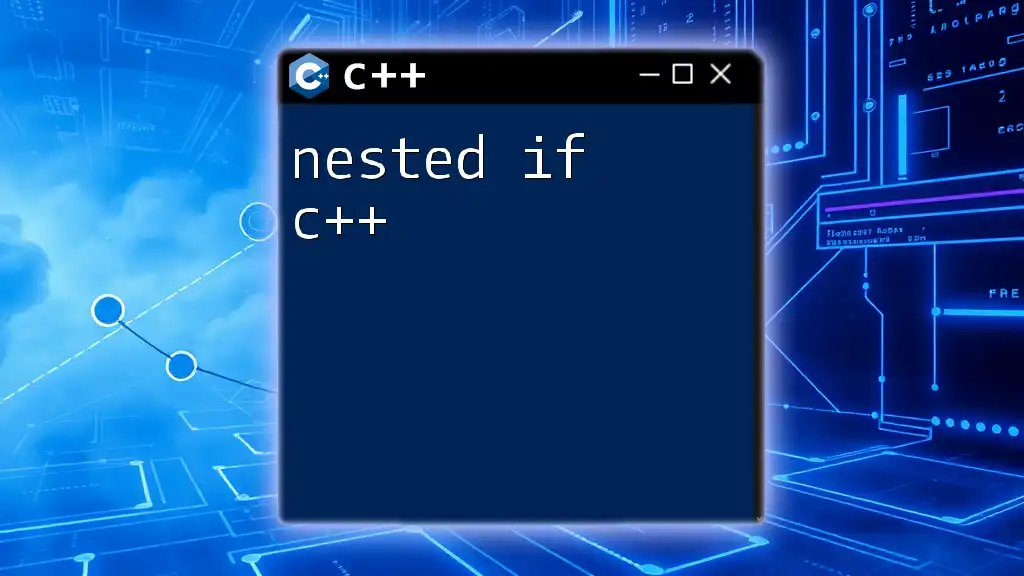
C++ If Else Statement Overview
Explanation of How If Else Statements Work
In C++, if else statements allow you to perform different actions based on whether a condition evaluates to true or false. The simplest form consists of an `if` clause followed by an optional `else` clause.
Basic Structure:
if (condition) {
// code to execute if condition is true
} else {
// code to execute if condition is false
}
This foundational structure serves as the basis for more complex nesting scenarios.
Single versus Nested If Else
While a single if else statement solves straightforward problems, nested if else statements come into play for scenarios requiring multiple conditions to be checked sequentially.
Example Illustrating the Difference: A single if else structure might look like this:
if (temperature > 30) {
cout << "It's hot outside!";
} else {
cout << "The weather is comfortable.";
}
But when you need to determine more specific temperature ranges:
if (temperature > 30) {
cout << "It's hot outside!";
} else if (temperature > 20) {
cout << "The weather is warm.";
} else {
cout << "It's cold outside!";
}
The latter is a simple nested if else structure, leading to multiple decision points.
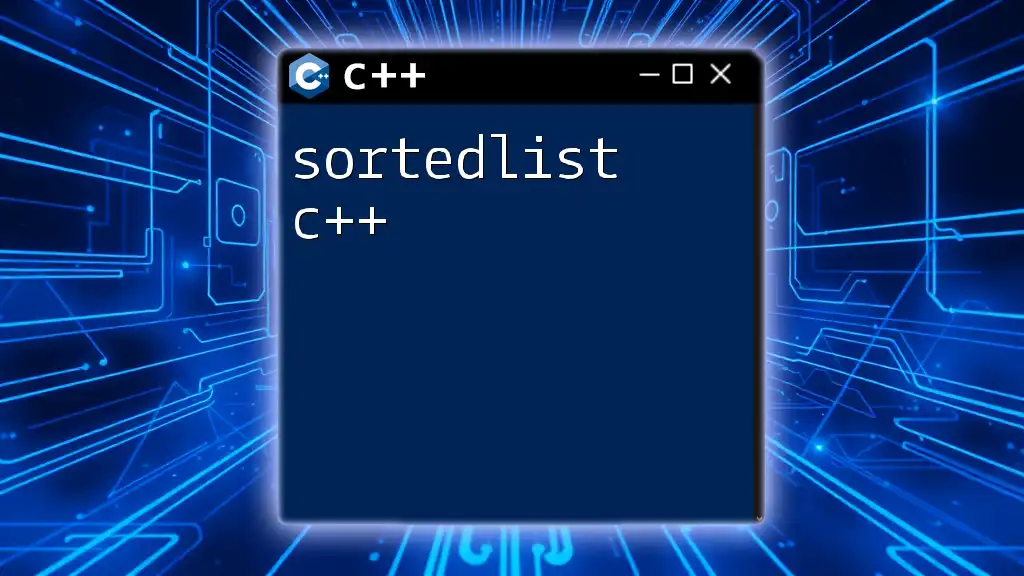
Structure of Nested If Else Statements
Syntax of Nested If Else Statements in C++
The syntax of a nested if else statement allows you to place an if else block within another if or else block. This enables complex decision-making and can be well structured for clarity.
Key Syntax:
if (condition1) {
// code block for condition1 true
if (condition2) {
// code block for condition2 true
} else {
// code block for condition2 false
}
} else {
// code block for condition1 false
}
This structure exemplifies how multiple conditions can be layered, and each level adds a layer of decision-making.
Flow of Control in Nested If Else
Understanding the flow of control is essential. When a nested if else statement executes, it starts from the outermost condition and checks each condition sequentially. If a condition is true, its corresponding block of code executes, and the subsequent inner conditions are ignored. This structure allows for organized and layered control flows.
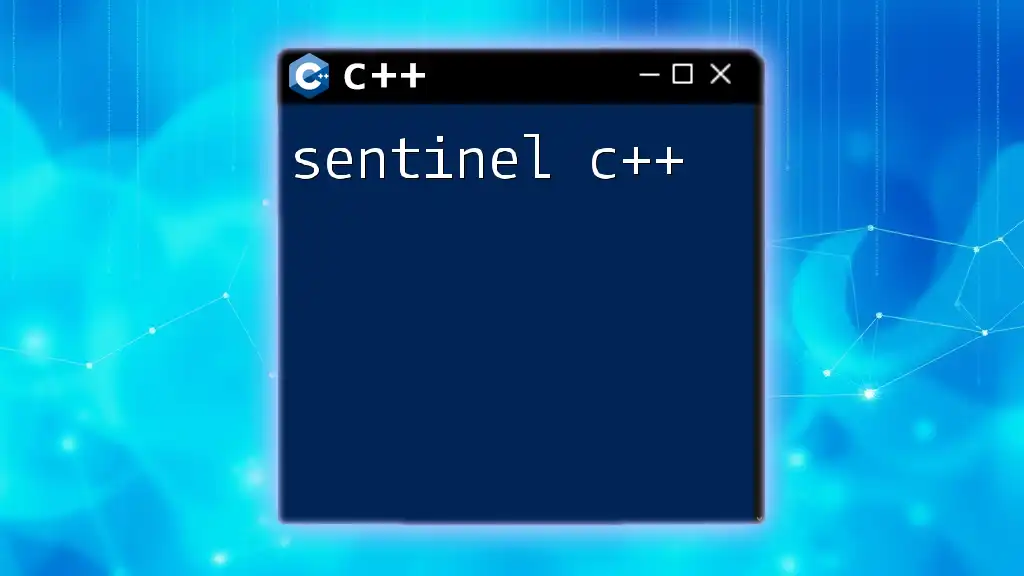
Practical Examples of Nested If Else Statements in C++
Example 1: Grading System
Let’s illustrate nested if else statements with a grading system as an example.
int score;
cout << "Enter your score: ";
cin >> score;
if (score >= 90) {
cout << "You received an A!";
} else if (score >= 80) {
cout << "You received a B!";
} else if (score >= 70) {
cout << "You received a C!";
} else if (score >= 60) {
cout << "You received a D!";
} else {
cout << "You received an F.";
}
In this scenario, we check a student’s score against multiple thresholds to assign a corresponding grade. Every `if` and `else if` acts as a series of conditions narrowing down the possible outcomes.
Example 2: Age Classification
Another practical use case is classifying age groups. Here's how it can be done:
int age;
cout << "Enter your age: ";
cin >> age;
if (age < 13) {
cout << "You are a child.";
} else if (age < 20) {
cout << "You are a teenager.";
} else if (age < 65) {
cout << "You are an adult.";
} else {
cout << "You are a senior.";
}
This example leverages a nested approach to ensure each age bracket is appropriately categorized based on the user input.
Example 3: Multi-Level Conditional Logic
Sometimes, nested if else statements are required for more complex logic, like ticket classification for events. Consider this scenario:
int age;
bool hasTicket;
cout << "Enter your age: ";
cin >> age;
cout << "Do you have a ticket? (1 for yes, 0 for no): ";
cin >> hasTicket;
if (age < 12) {
cout << "Child ticket.";
} else if (age < 65) {
if (hasTicket) {
cout << "Adult ticket.";
} else {
cout << "Adult ticket, please buy a ticket.";
}
} else {
cout << "Senior ticket.";
}
This layered decision tree checks both age and ticket possession, demonstrating how nested if else statements can effectively manage multiple criteria for branching logic.
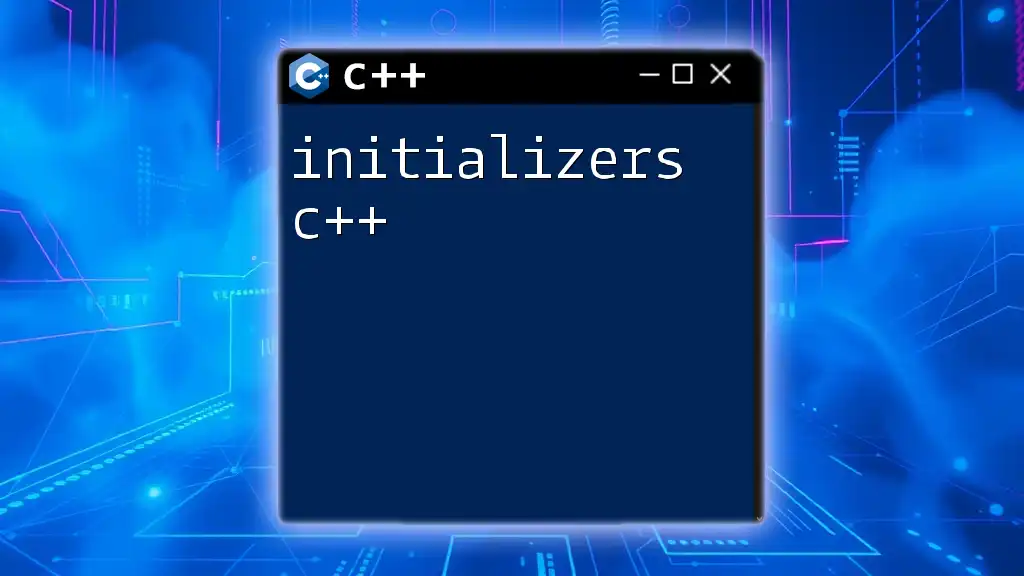
Best Practices for Using Nested If Else Statements in C++
Readability and Maintainability
When working with nested if else statements, readability is paramount. Clearly structure your code by maintaining consistent indentation levels and using meaningful variable names. This practice helps both you and future developers comprehend the logic at a glance.
Avoiding Deep Nesting
Deeply nested structures can lead to complexity and confusion, making the code harder to debug and understand. Always look for alternatives, like using switch statements or refactoring your code to use functions that handle specific conditions, thereby flattening the structure.
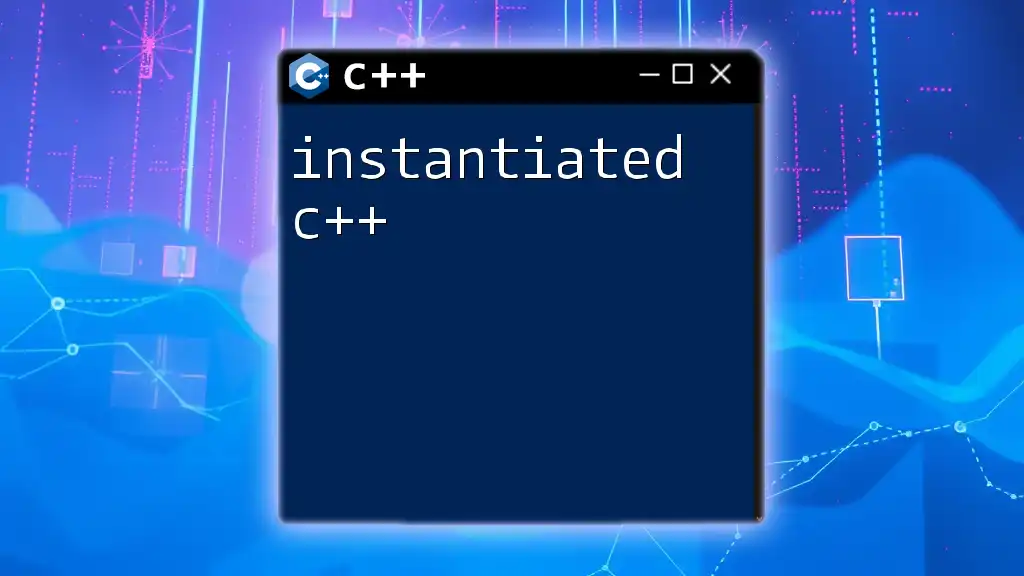
Common Mistakes to Avoid in Nested If Else Statements
Logical Errors
One common mistake when using nested if else statements involves modifications to conditions that may yield unintended results. For example:
if (x > 10) {
if (x < 5) {
// This code will never run
}
}
Always review your logic flow to ensure that conditions make sense.
Syntax Errors
Syntax errors often arise in complex nested structures due to misplaced braces or incorrect placement of else statements. Always verify the bracket placements and ensure that each if has a matching else or else if where necessary.
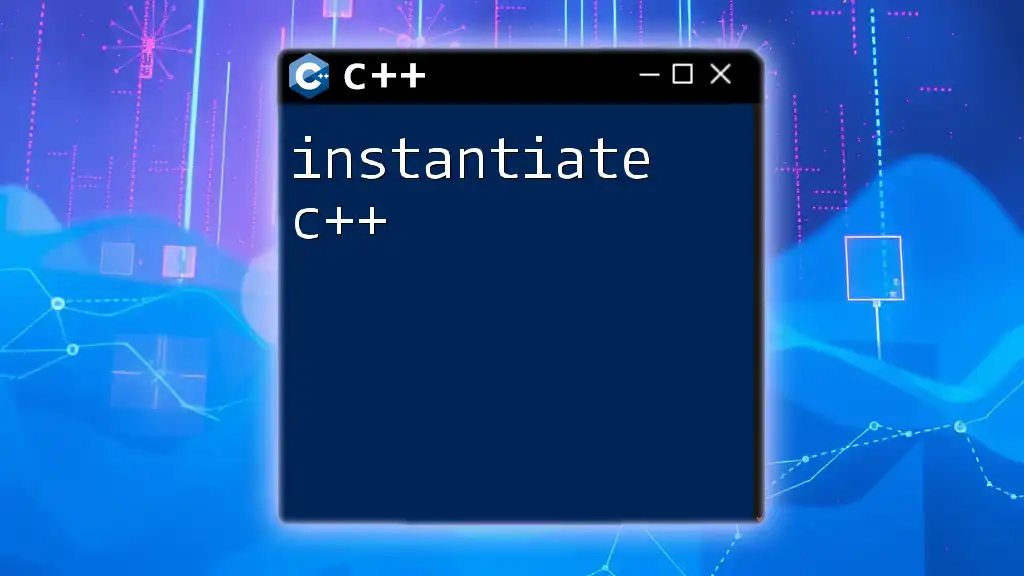
Conclusion: Mastering Nested If Else in C++
Understanding nested if else statements is essential for effective C++ programming. By mastering this concept, you can create complex decision-making structures that allow your code to handle various scenarios gracefully.
Encouragement to Practice
By actively practicing various nested if else scenarios, you can enhance your programming capabilities in C++ and improve your problem-solving skills. The more you code, the more proficient you will become, enabling you to tackle increasingly complex programming challenges with confidence.
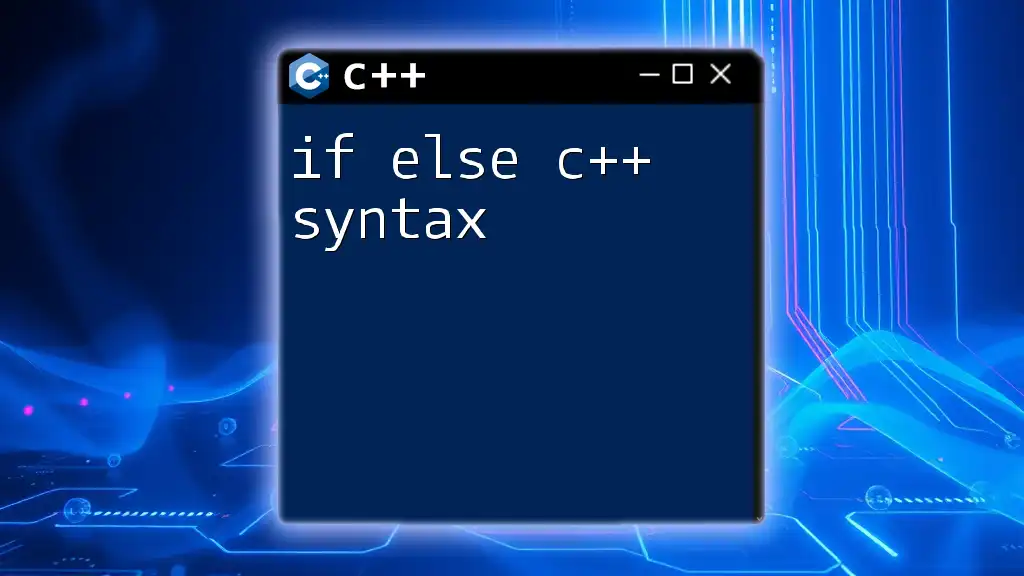
Additional Resources
For further learning, consider checking out online tutorials, programming books, and resources on C++ that delve deeper into conditional structures, including nested if else statements and their best practices. Always aim to stay informed and practice regularly to enhance your command over C++.