A header file in C++ is a file that typically contains function declarations, macro definitions, and class definitions, allowing for code reuse and organization across multiple source files.
Here's an example of a simple header file in C++:
// example.h
#ifndef EXAMPLE_H
#define EXAMPLE_H
void sayHello();
#endif // EXAMPLE_H
What Are Header Files?
Header files in C++ are essential components that help manage code organization and modularity. They typically have the extension `.h` or `.hpp` and contain declarations for functions, classes, and variables, allowing you to separate interface from implementation. This separation enhances code manageability and reusability.
When you include a header file in your C++ program, you are essentially informing the compiler about the definitions it needs without disclosing the actual code definitions contained within implementation files (usually `.cpp`).
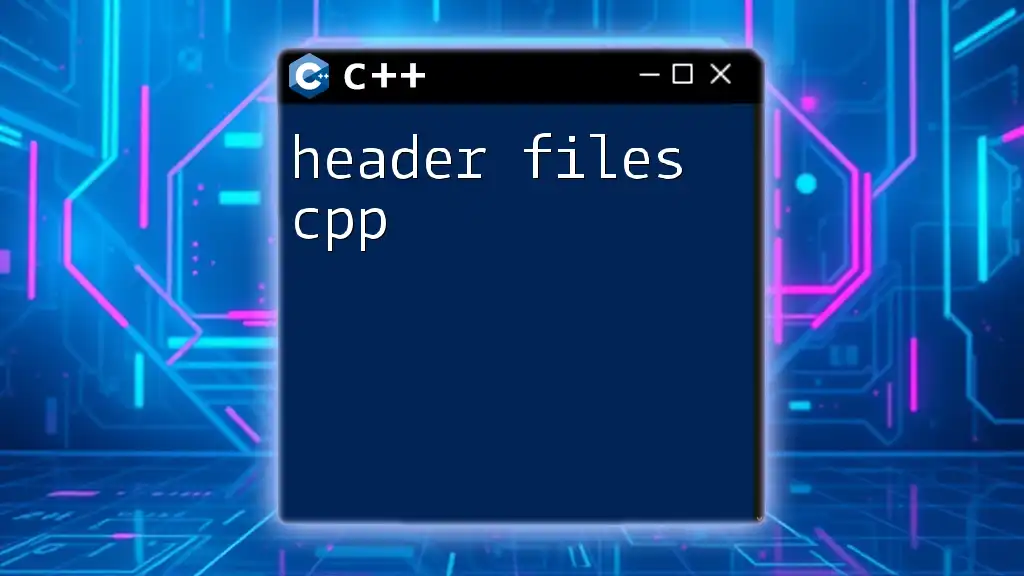
Overview of C++ Header Files
C++ header files serve as the bridge between different code modules. They declare the entities (functions, classes, etc.) that can be used in other files. Understanding the difference between header files and source files is crucial: header files declare entities while source files define them.
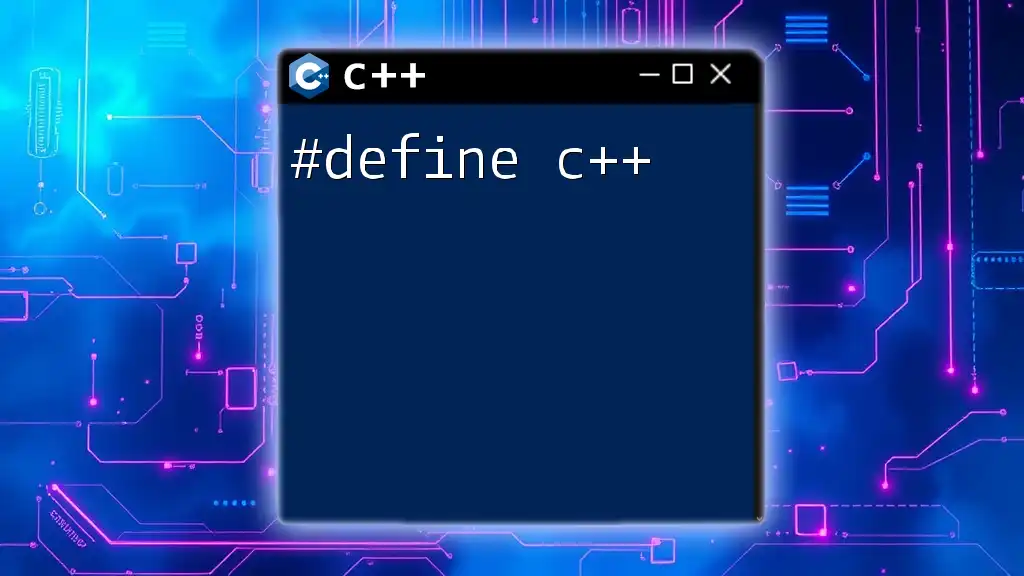
The Structure of a C++ Header File
Basic Syntax of a Header File
A C++ header file usually consists of the following key components:
- Preprocessor directives (e.g., `#ifndef`, `#define`, `#endif`)
- Declaration of functions and classes
- Extern variables
- Documentation comments
Here’s a simple example of the structure of a header file:
// myheader.h
#ifndef MYHEADER_H
#define MYHEADER_H
void myFunction();
#endif // MYHEADER_H
Include Guards
Include guards are a mechanism used to prevent multiple inclusions of the same header file, which could lead to errors and code bloat. By wrapping your header file's content with `#ifndef`, `#define`, and `#endif`, you ensure that if a header file has already been included, the compiler will ignore subsequent inclusions.
#ifndef MYHEADER_H
#define MYHEADER_H
// declarations
#endif // MYHEADER_H
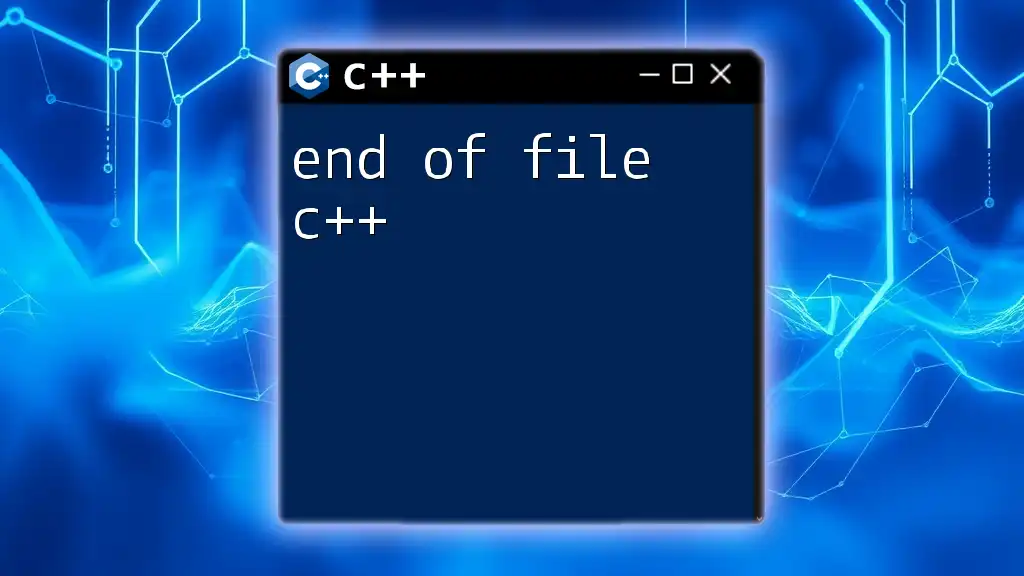
Creating a C++ Header File
Step-by-Step Guide to Writing a Header File
Creating a well-structured header file involves several steps:
-
Define the Purpose: Determine what functionality or data your header file should provide. This encompasses identifying the classes or functions to declare.
-
Structure Your Code: Organize the code logically. Group related functions and classes together, ensuring that the header file remains coherent and focused.
-
Write Necessary Prototypes: Provide function prototypes and class declarations without implementation details.
Example: Creating a Header File in C++
Here’s an example showing how to create a header file for a simple `Rectangle` class.
// shapes.h
#ifndef SHAPES_H
#define SHAPES_H
class Rectangle {
public:
Rectangle(double length, double width);
double area();
private:
double length;
double width;
};
#endif // SHAPES_H
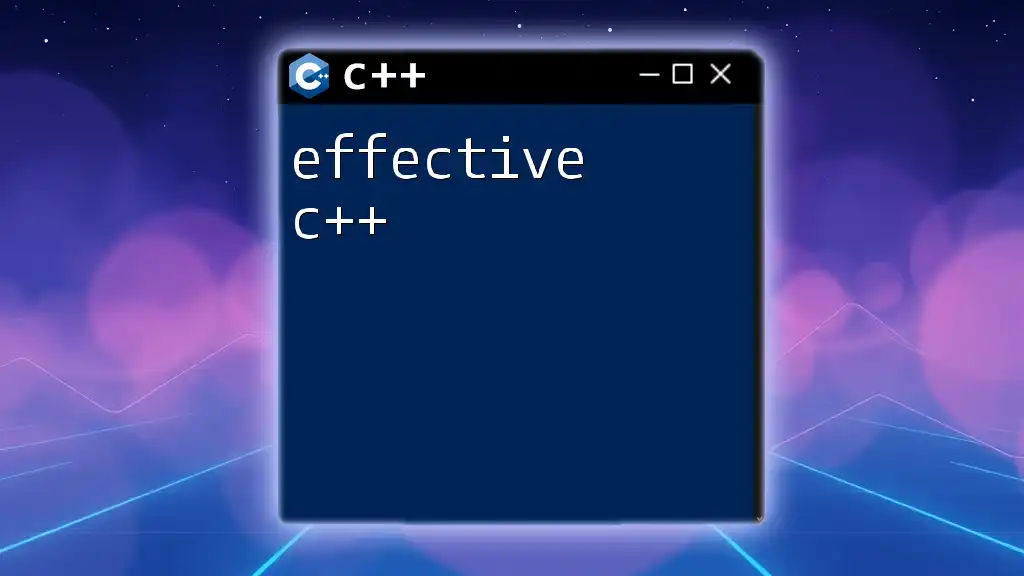
Using Header Files in C++
How to Include Header Files
To utilize a header file in a C++ source file, the `#include` directive is pivotal. Depending on the source of the header file, you can choose between angle brackets and double quotes.
- Use double quotes for user-defined header files: `#include "myheader.h"`
- Use angle brackets for system or standard library headers: `#include <iostream>`
Code Snippet: Including a Header File
#include "shapes.h" // User-defined header file
#include <iostream> // Standard library header file
The Impact of Header Files on Compilation
Header files significantly influence the C++ compilation process. Each time a header file is included, its declarations are read into the program. Thus, if you make changes to a header file, all associated `.cpp` files may need recompilation. Understanding this helps maintain efficient builds.
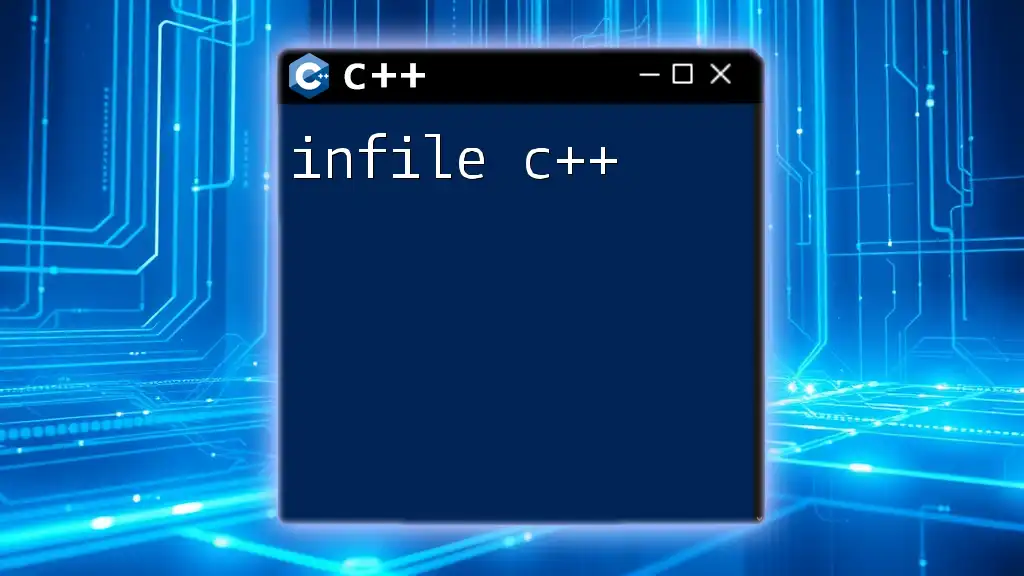
Organizing Header Files
Best Practices for Organizing Header Files in C++
When working with multiple header files within a C++ project, adhering to best practices enhances clarity and maintainability:
-
Naming Conventions: Stick to a consistent naming scheme for header files (e.g., `.h` for C-style headers and `.hpp` for C++ headers).
-
Keep Files Concise: Strive for a clear focus in each header file. Avoid cramming unnecessary declarations into a single file.
-
Separate Interface from Implementation: Organize code in a way that header files contain only declarations while the definitions reside in corresponding source files.
Real-world Example of Header File Organization
Consider a project that implements geometric shapes. You could have separate header files like `shapes.h`, `circle.h`, and `rectangle.h`, each dedicated to the specific declarations of their associated classes.
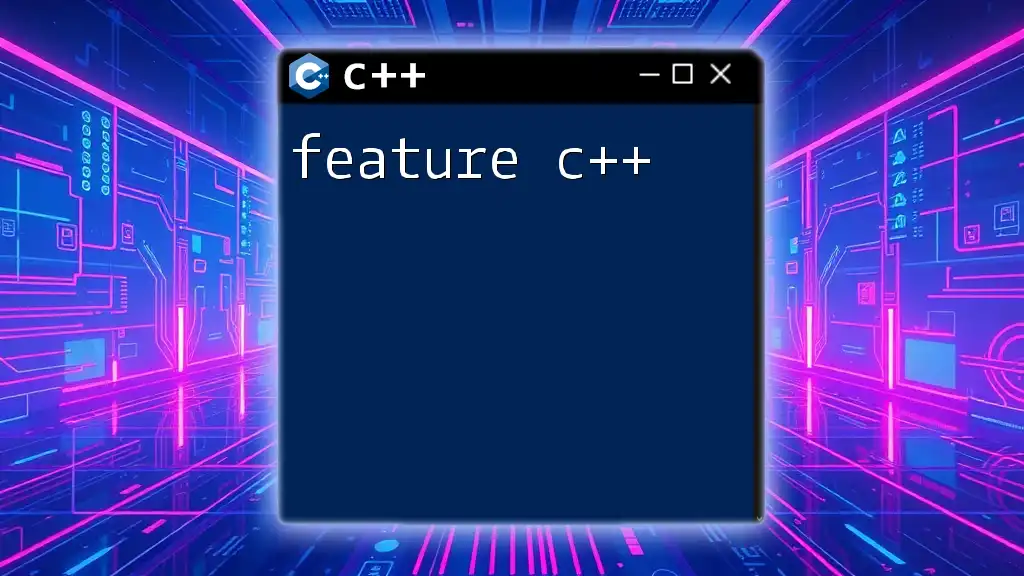
Advanced Topics in Header Files
C++ Template Header Files
Templates allow for writing generic code applicable to any data type. Template header files declare generic classes or functions, enabling versatile and reusable code design.
Example: C++ Template Header File
// mytemplate.h
#ifndef MYTEMPLATE_H
#define MYTEMPLATE_H
template <typename T>
class MyTemplate {
public:
void display(T value);
};
#endif // MYTEMPLATE_H
Header Files and Namespaces
Namespaces prevent naming collisions in larger projects. When you declare a class or function, wrap it in a namespace to avoid conflicts with other code libraries.
Code Snippet: Namespaces in a Header File
namespace MyNamespace {
void myFunction();
}
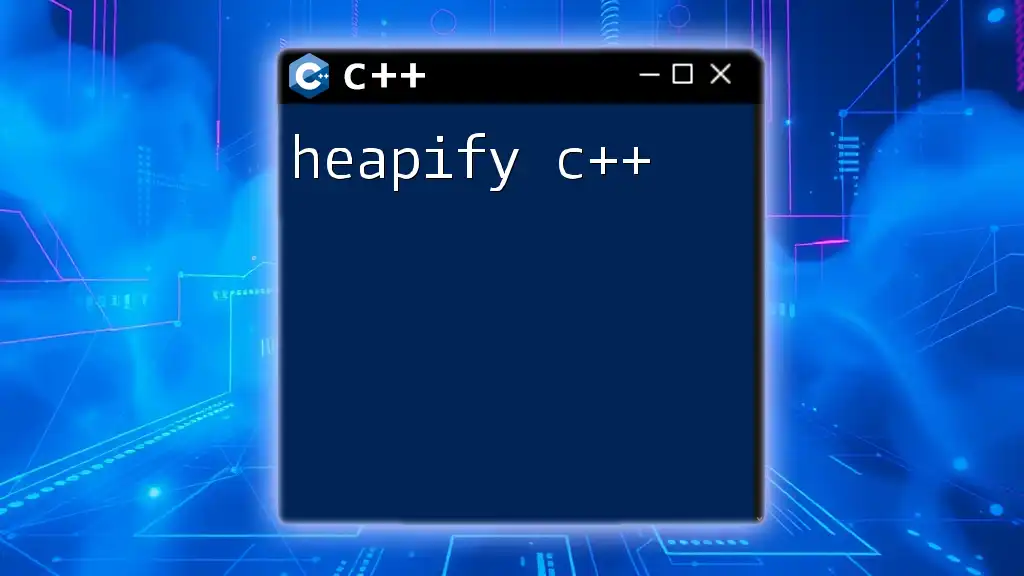
Common Mistakes with C++ Header Files
Avoiding Circular Dependencies
Circular dependencies occur when two or more header files include each other. This can lead to compilation errors and vague behavior in programs. To prevent this, utilize forward declarations to avoid unnecessary include statements.
Forward Declaration vs. Include
A forward declaration informs the compiler about a class or function without fully defining it, thus maintaining dependencies' clarity and minimizing overhead.
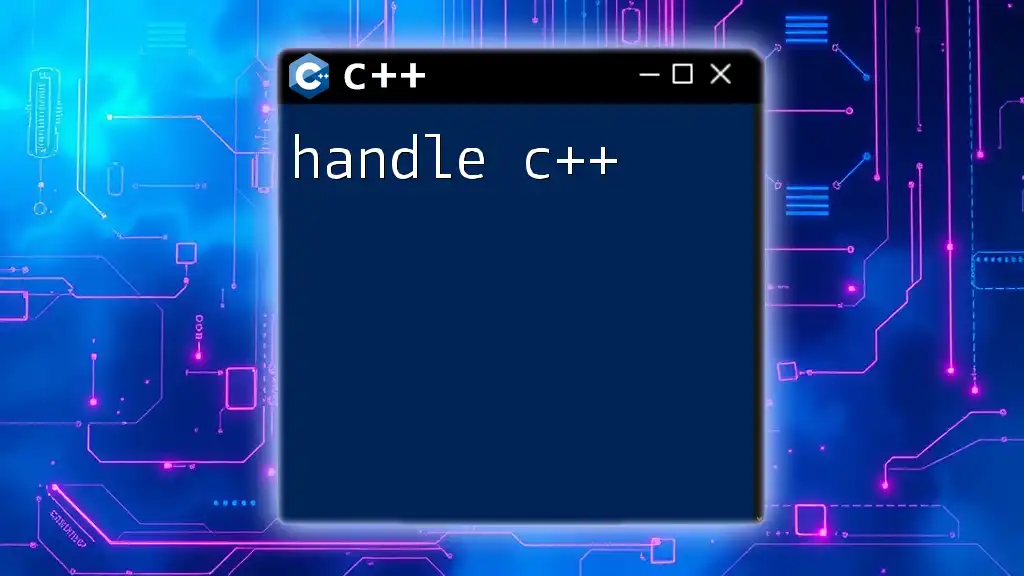
Conclusion
Header files in C++ are foundational to maintaining organized, reusable, and efficient code structures. By understanding how to create and use header files, you empower yourself to manage larger projects seamlessly and foster better programming practices. Whether you draw from individual examples or structured templates, practicing these concepts will deepen your know-how and elevate your programming skills.
With this comprehensive guide on header files, you are now equipped to implement and maintain these indispensable tools in your C++ programming endeavors. Happy coding!