In C++, the end-of-file (EOF) can be detected using the `eof()` function from the `ifstream` class, which indicates when the end of a file has been reached during input operations.
#include <iostream>
#include <fstream>
int main() {
std::ifstream file("example.txt");
if (file.is_open()) {
while (!file.eof()) {
std::string line;
std::getline(file, line);
std::cout << line << std::endl;
}
file.close();
}
return 0;
}
Understanding End of File (EOF) in C++
What is EOF?
In programming, the term End of File (EOF) refers to a special marker that signifies no more data is available to be read from a file or standard input stream. It plays a critical role in file handling and input/output processes, as it indicates to the program that it has reached the end of the data stream it is attempting to process. Without a clear mechanism to detect EOF, programs may experience errors, infinite loops, or unexpected behavior when attempting to read from an input stream.
How EOF is Represented in C++
In C++, EOF is represented by a constant defined within the standard library. When working with file streams, the `std::ifstream` class provides methods to help manage and detect EOF. Specifically, the `std::ifstream::eof()` function allows programmers to check whether the end of the file has been reached. Understanding how EOF interacts with stream states (namely, `good`, `bad`, and `fail`) is pivotal for robust file handling in C++.
- Stream States:
- `good`: Indicates that the stream is in a good state for reading.
- `bad`: Indicates that an unrecoverable error has occurred.
- `fail`: Indicates that a logical error has occurred (e.g., trying to read beyond EOF or reading incompatible data types).
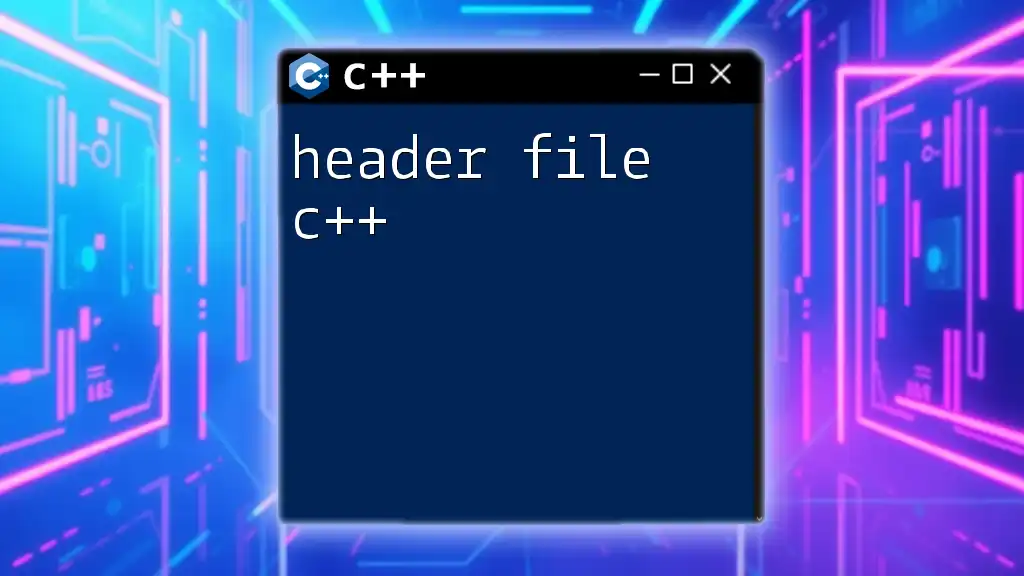
Detecting EOF in C++
Common Methods to Check for EOF
Detecting EOF in C++ can be accomplished through various methods, with the most common being a loop structure that checks for EOF during input operations.
Example: Using EOF with a While Loop
You can effectively use a while loop to detect EOF when reading from standard input using `std::getline()`. This method keeps reading until it encounters the EOF marker.
#include <iostream>
int main() {
std::string line;
while (std::getline(std::cin, line)) {
std::cout << line << std::endl;
}
return 0;
}
Best Practices
When dealing with file processing in C++, it is essential to check for EOF as part of your input validation process. This ensures that your program does not attempt to read past the end of the file, preventing potential errors.
It's also crucial to recognize common pitfalls, such as assuming EOF will trigger immediately after reading. EOF is only set after an attempted read operation has exhausted the available data, so proper checks must be in place prior to processing input.
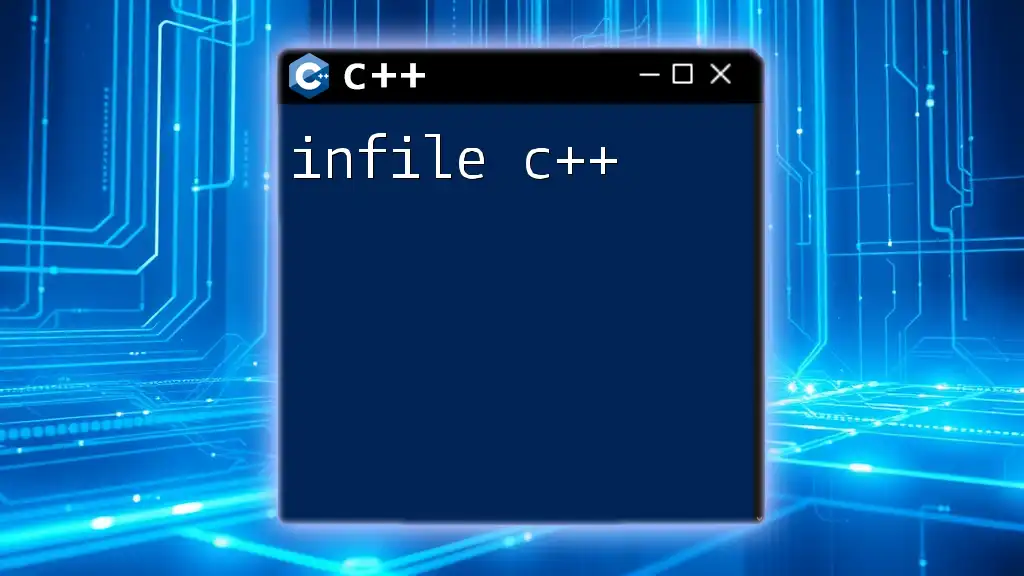
Practical Use Cases of EOF
Reading Files until EOF
One of the fundamental applications of EOF is to read an entire file until all data has been processed. By utilizing a loop and `std::getline()`, you can easily display the contents of a file line by line.
Example: Reading a File
Here’s a simple example of how to read from a file until EOF is reached:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.txt");
std::string line;
while (std::getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
return 0;
}
In this code, the `while` loop continues until all lines in `example.txt` are read. The use of `std::getline()` allows for capturing each line until EOF is encountered.
Handling Multiple Data Types
C++ also allows for efficient reading of mixed data types until EOF using the `>>` operator with file streams. This is useful when processing structured data files.
Example: Reading Different Data Types
To demonstrate this, here's an example of reading integers and strings from a file:
#include <iostream>
#include <fstream>
int main() {
std::ifstream file("data.txt");
int number;
std::string text;
while (file >> number >> text) {
std::cout << "Number: " << number << ", Text: " << text << std::endl;
}
file.close();
return 0;
}
This code will read pairs of integers and strings from `data.txt` until EOF is reached, which allows for efficient processing of structured data formats.
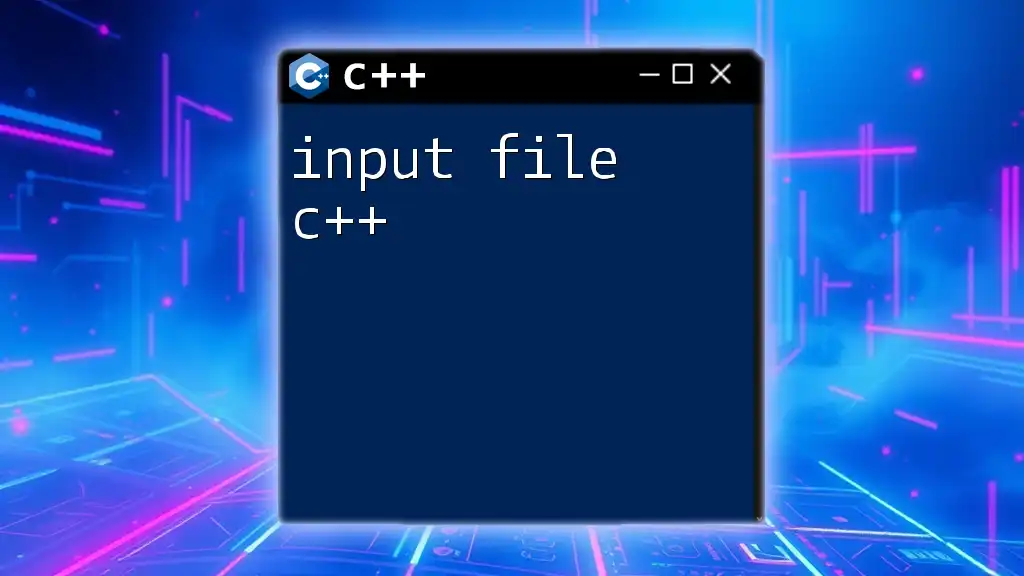
EOF in Standard Input vs. File Input
Differences Between Standard Input and File Input
When dealing with EOF, it is important to understand the differences between standard input and file input. When reading from standard input (e.g., keyboard), EOF is typically signaled by specific key combinations such as Ctrl+D on UNIX/Linux systems and Ctrl+Z on Windows. This allows users to indicate that they are finished providing input.
In contrast, when reading from a file, EOF is reached naturally as the end of the file's data is encountered. This difference affects how users interact with console applications versus file-based applications, necessitating awareness of EOF handling in both contexts.
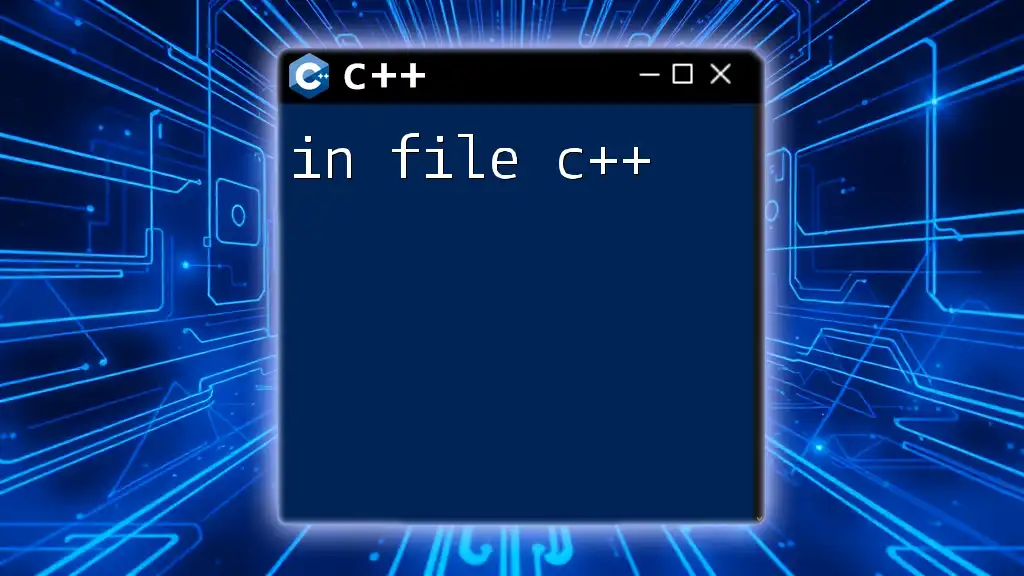
Conclusion
Essentials of EOF in C++
In summary, the concept of end of file (EOF) in C++ is critical for effective file handling and data processing. Understanding how to detect and manage EOF can prevent common programming errors and lead to robust applications.
Practicing EOF handling, such as reading lines or mixed data types until the end of the stream, is an essential skill for any C++ developer. By mastering these concepts, you will enhance your ability to create reliable and user-friendly applications.
Additional Resources
For further learning, consider checking out recommended books and online tutorials that cover C++ programming in detail. Engaging with trusted communities and forums can also provide valuable insights and support as you delve deeper into the world of C++.