In C++, an "assertion failed" error occurs when the `assert()` macro determines that a specified condition is false during program execution, indicating a logical flaw that needs to be addressed.
Here's a simple code snippet demonstrating its usage:
#include <cassert>
int main() {
int x = 5;
assert(x == 10); // This assertion will fail, triggering an error
return 0;
}
Understanding Assertions
What Is an Assertion?
Assertions are a critical aspect of C++ programming used to test assumptions made by the program. They act as internal self-checks within the code and help developers verify that certain conditions hold true at specific points during execution. When an assertion fails, it indicates a serious error, typically suggesting a bug in the code that needs immediate attention.
Benefits of Using Assertions
Using assertions in your code brings several significant advantages:
-
Early Error Detection: Assertions help catch logical errors during the development phase rather than after the code is deployed. This proactive measure enables developers to identify issues before they escalate.
-
Improved Code Reliability: By defining conditions that must hold true, assertions promote the creation of more robust and reliable software. When assertions are correctly implemented, they serve as a foundation for ensuring that critical invariants are maintained.
-
Ease of Maintenance: Clean, well-placed assertions can document the developer's intent and assumptions about the code, making it easier for others (or future you) to understand and maintain it.
Common Use Cases
Assertions are particularly useful in several scenarios:
-
Debugging Complex Logic: In intricate algorithms where multiple conditions need to be checked, assertions act as a safety net, allowing developers to focus on logic without needing extensive manual verification.
-
Validating Invariants in Algorithms: Wherever an invariant must be maintained—such as in data structures like linked lists or binary trees—assertions can verify the integrity of the data at various stages of processing.
-
Ensuring Preconditions and Postconditions: Assertions can check that function parameters meet necessary preconditions before execution and validate outputs against expected postconditions. This practice ensures that functions behave as intended.

The Mechanics of Assertions in C++
Syntax of Assertions
To use assertions in C++, the syntax is straightforward:
assert(condition);
This function takes a condition as its argument. If the condition evaluates to false, the assertion fails, and the program is terminated. Here is a simple example illustrating its use:
#include <cassert>
void checkValue(int value) {
assert(value > 0); // This will terminate the program if value is not greater than 0
}
In this case, if `checkValue` is called with a non-positive number, the assertion will fail, highlighting a logic error in the calling code.
How to Enable/Disable Assertions
Assertions can be enabled or disabled using the `NDEBUG` macro. By defining `NDEBUG`, you prevent assertions from being evaluated, which can be useful in production scenarios where performance is crucial.
Here's how you can enable and disable assertions:
#define NDEBUG // This will disable assertions
#include <cassert>
Conversely, if you comment out `#define NDEBUG`, assertions will be active, thus allowing their checks during testing and debugging phases.

Analyzing Assertion Failures
What Happens When an Assertion Fails?
When an assertion fails, the program ceases execution, and a diagnostic message is output to the console. This message typically offers insights into what went wrong, including the failed condition, the corresponding function, and the line number.
To illustrate this, consider the following scenario that leads to assertion failure:
#include <cassert>
#include <iostream>
void divide(int a, int b) {
assert(b != 0); // Asserting that divisor b is not zero
std::cout << a / b << std::endl;
}
int main() {
divide(10, 0); // This will trigger an assertion failure
return 0;
}
Upon executing this code, the assertion fails because the divisor `b` is zero, leading to an error message similar to:
Assertion failed: (b != 0), function divide, file main.cpp, line 4.
This message provides vital context for debugging, pinpointing where the error occurred.
Error Messages and Debugging
When you encounter assertion failures, the error messages convey essential debugging information. Understanding how to read these messages can significantly streamline the debugging process. Pay attention to:
- The condition that failed.
- The function and file in which the failure occurred.
- The specific line number, which directs you to the exact code segment in question.
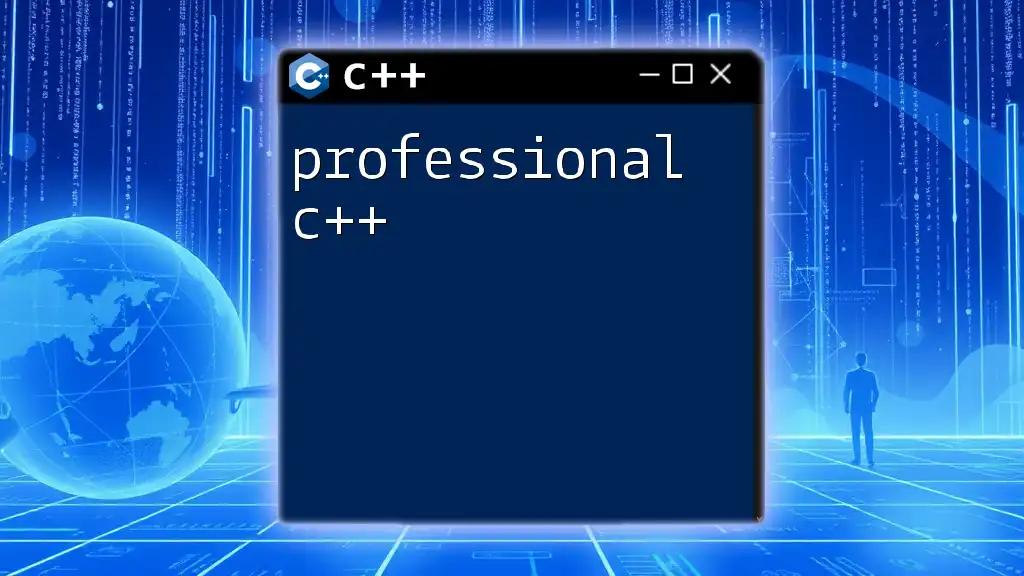
Best Practices for Using Assertions
When to Use Assertions
Assertions should be effectively employed to confirm conditions that are assumed to be true during development. However, it’s essential to recognize that assertions are not a substitute for error handling in production code. They should be used for:
- Checking logic and assumptions that should never fail unless there is a bug.
- Validating internal consistency of data structures.
Writing Clear and Informative Assertion Messages
Providing meaningful assertion messages enhances the usability of assertions. A clear, descriptive message can lead to quicker resolutions of bugs. Consider this example:
assert(value != nullptr && "Value should not be null."); // Good practice
assert(value); // Poor practice
The first example provides contextual information that can be quickly grasped by the developer, whereas the second lacks clarity and can lead to confusion.
Avoiding Common Pitfalls
Common pitfalls when using assertions include:
-
Overusing Assertions: While assertions are helpful, relying on them for flow control or regular error handling can lead to poor design. They should primarily be used for development and debugging, not for validating user input.
-
Not Using Assertions in Production Code: Compiling code with `NDEBUG` in production removes assertions. Always be cautious not to bypass essential checks meant to catch critical errors.
-
Misunderstanding the Purpose of Assertions: Assertions are intended as a debugging aid. They are not a replacement for robust error handling; use exceptions for handling runtime errors effectively.
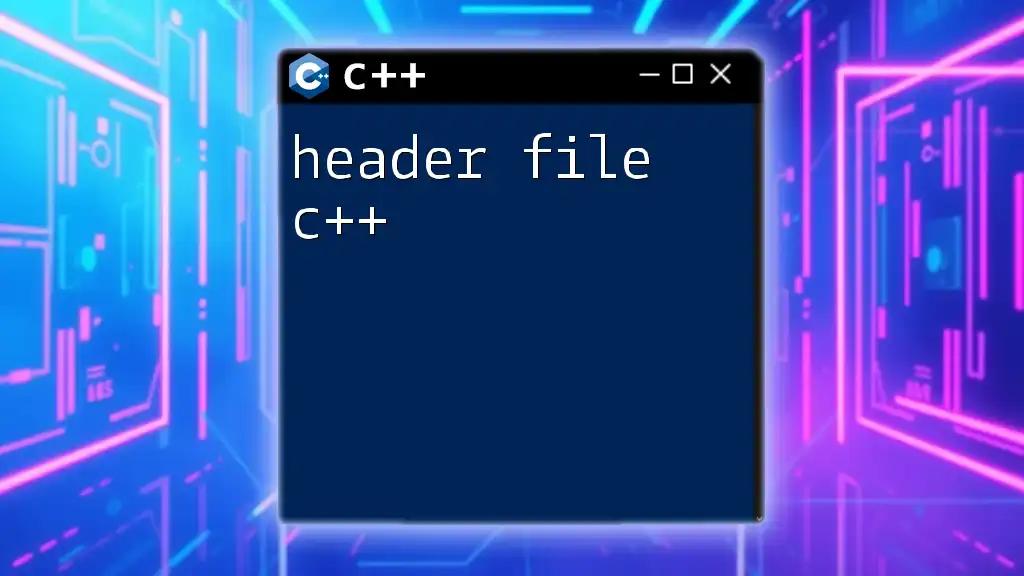
Alternatives to Assertions
Error Handling Strategies
While assertions are beneficial, they should be complemented by structured error handling. Use exceptions for conditions that can occur in production and require handling. For instance, instead of asserting that a value is non-null, it may be more fitting to throw an exception if the requirement is violated:
if (value == nullptr) {
throw std::invalid_argument("Value cannot be null.");
}
Static Analysis Tools
Static analysis tools can be powerful alternatives in catching logic errors and improving code quality without relying solely on assertions. They analyze the codebase without executing it, identifying potential pitfalls. Popular static analysis tools for C++ include:
- CPPcheck
- Clang Static Analyzer
- SonarQube
These tools can serve as an additional layer of validation beyond what assertions provide.
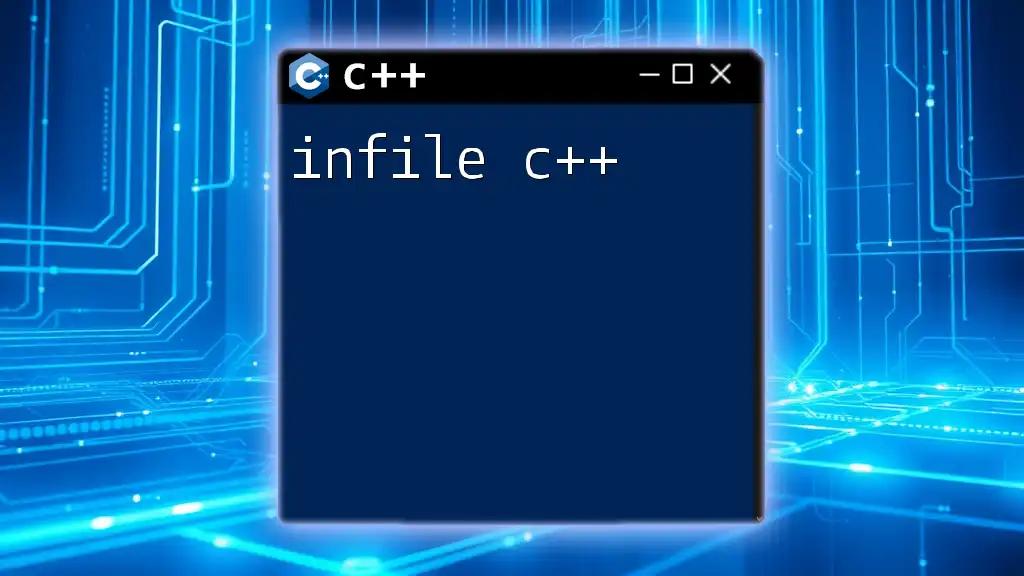
Conclusion
In summary, understanding the concept of assertion failed C++ is crucial for any developer aiming to enhance the reliability and maintainability of their code. By implementing assertions thoughtfully, you can catch errors early in the development cycle, clarify the developer's intentions, and subsequently improve overall code quality.
Utilizing best practices for writing assertions—ensuring clarity, using them judiciously, and recognizing their limitations—will surely contribute to creating more robust applications.
Finally, I encourage you to incorporate assertions into your coding practices, as they stand as a valuable tool in your C++ toolkit, paving the way for more productive and error-free development. If you’ve had experiences with assertions, please share your thoughts and insights. Let's continue learning and improving together!