A "debug assertion failed" error in C++ typically indicates that an assertion (a condition that must be true) has evaluated to false during debugging, helping developers identify logical errors in their code.
Here's an example of how to use an assertion in C++:
#include <cassert>
void check_positive(int num) {
assert(num > 0 && "Number must be positive.");
}
int main() {
check_positive(-1); // This will trigger a debug assertion failed error
return 0;
}
Understanding Assertions in C++
What is an Assertion?
An assertion is a statement that checks if a condition is true at a specific point in your program's execution. If the condition evaluates to false, the program will terminate, typically displaying an error message. Assertions serve as a vital debugging aid, helping developers catch programming errors during development rather than allowing them to manifest in production.
The syntax of an assertion in C++ is straightforward:
assert(condition);
Using `assert` in C++
To use assertions in your C++ program, you must include the `<cassert>` header file. This grants you access to the `assert()` function, which enables you to create assertions as follows:
#include <cassert>
void checkPositive(int number) {
assert(number > 0); // Asserts that the number is positive
}
When to Use Assertions
Assertions are particularly useful for validating assumptions within your code. Ideal situations for using assertions include:
- Checking preconditions and postconditions in functions.
- Verifying invariants within classes.
- Establishing valid states in complex algorithms.
The advantages of using assertions are significant. They improve code reliability and documentation, serve as a self-check mechanism, and aid in debugging.
However, there are limitations. Assertions should not replace regular error handling since they may be disabled in production builds, leading to undefined behavior if the assertion is triggered.

The Mechanism Behind Debug Assertion Failed
Triggers of Debug Assertion Failed
A "debug assertion failed" error occurs when an assertion fails during program execution. This typically happens in scenarios such as:
- An unexpected value or state in the program.
- Logic errors that lead to invalid conditions being checked.
Understanding the error message is crucial for troubleshooting. The message often indicates where the assertion failed and can provide a useful error code or description enabling developers to trace the source of the issue.
Common Causes of the Error
Several factors can lead to a debug assertion failure:
- Invalid Conditions: If the logic you have placed in the `assert()` statement evaluates to false, it will trigger a failure. For example:
int divide(int a, int b) {
assert(b != 0); // This will fail if b is 0
return a / b;
}
-
Memory Issues: Improper memory management can lead to access violations or dereferencing of invalid pointers. This includes:
- Dangling Pointers: Where a pointer still references a memory location that has been freed.
-
Out-of-Bounds Access: Accessing an array index that is beyond its allocated range will lead to a failure.
void printArrayElements(int* arr, int size) {
assert(size > 0); // Asserts that size is greater than 0
for (int i = 0; i <= size; i++) { // Potential out-of-bounds access
printf("%d\n", arr[i]);
}
}
Understanding the Debugger's Role
When debugging C++ applications, your debugger plays a crucial role. It allows you to step through your code execution, examine the state of variables, and identify where things go wrong. Common debugging tools include GDB (GNU Debugger) and IDE-integrated debuggers. These tools can help you track down the source of a debug assertion failure by providing insight into the call stack and current program state.
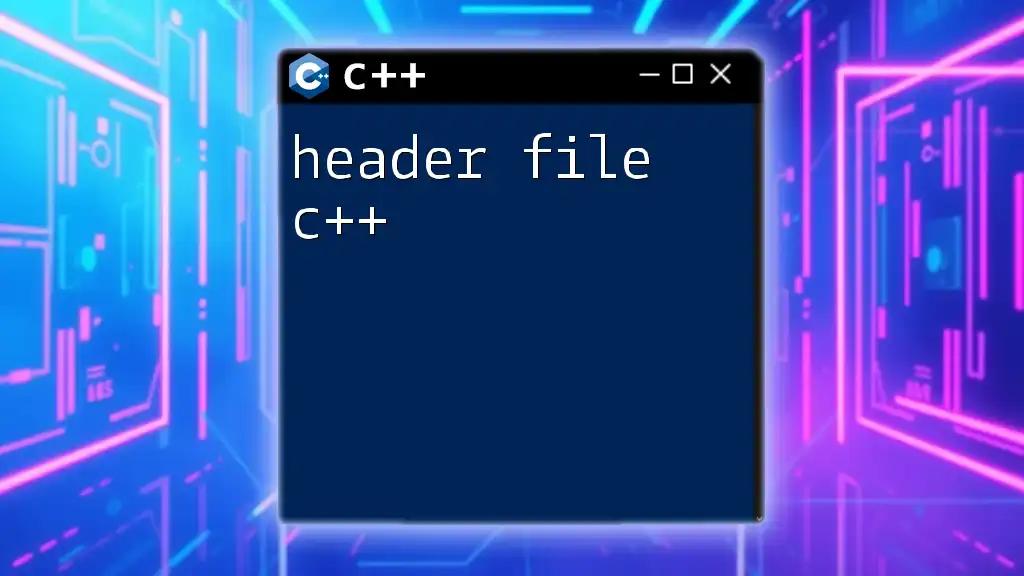
Debugging Techniques for Assertion Failures
Best Practices for Assertions
To write effective assertions, keep the following practices in mind:
- Ensure that your assertions are meaningful and clear. They should represent conditions that must hold true.
- Avoid overusing assertions, as excessive assertions can clutter the code and make it difficult to identify genuine issues.
Step-by-Step Debugging Process
When you encounter a debug assertion failure, follow these steps:
- Reproduce the Error: Ensure that you can replicate the assertion failure consistently.
- Analyze the Call Stack: Use your debugger to examine the call stack at the time of the failure. This helps you trace back to where the assertion was triggered.
- Examine Variable States: Check the values of relevant variables at the time of the assertion. This may reveal unexpected conditions leading to failure.
int main() {
int num = -5;
checkPositive(num); // This will trigger the assert
return 0;
}
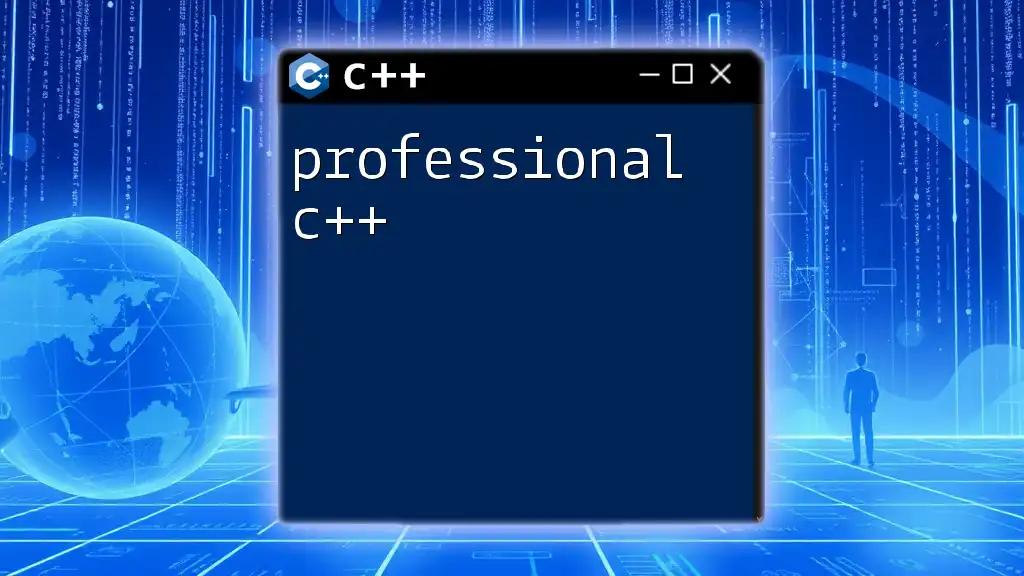
Practical Examples
Example 1: Simple Assertion Failure
Consider the following simple code that demonstrates an assertion failure:
#include <cassert>
void checkPositive(int number) {
assert(number > 0); // This will fail if number is not positive
}
int main() {
int num = -10;
checkPositive(num); // Will cause a "debug assertion failed" error
return 0;
}
When you run this code, the assertion fails because the `num` variable holds a negative value. The message typically points to the line where the assertion is defined, helping you recognize the condition that failed and correct it.
Example 2: Complex Assertion Scenario
Now let’s look at a more complex situation involving multiple assertions. Consider the following code snippet that manages an array:
#include <cassert>
void printArrayElements(int* arr, int size) {
assert(size > 0); // Ensure size is greater than 0
for (int i = 0; i <= size; i++) { // Potential off-by-one error
printf("%d\n", arr[i]);
}
}
int main() {
int arr[] = {1, 2, 3, 4};
printArrayElements(arr, 4); // This will cause a "debug assertion failed" error
return 0;
}
In this code, the assertion `assert(size > 0);` passes, but the `for` loop condition `i <= size` results in an off-by-one error. Properly indexing should use `<`. This scenario illustrates the importance of maintaining correct loop limits in conjunction with assertions to prevent unintended behaviors.

Resolving Debug Assertion Failed Issues
Strategies for Fixing Assertion Failures
To address assertion failures, consider the following strategies:
-
Review Your Logic: Revisit the logic surrounding your assertions, ensuring the conditions being checked are accurate and not overly strict.
-
Testing Boundary Conditions: Particularly in cases involving arrays or other indexed data structures, always evaluate boundary conditions to confirm they behave as intended.
-
Memory Management Considerations: Always verify that pointers reference valid memory locations and are appropriately managed to avoid "dangling pointers".
Tools and Techniques for Effective Troubleshooting
Leverage your development environment's debugging features. Most modern IDEs come equipped with robust debugging tools that allow you to:
- Set breakpoints to halt program execution at specified locations.
- Step through the code line by line to observe variable changes.
- Inspect the memory and stack frames to better understand the state of your program leading up to the failure.

Final Thoughts
The use of assertions in C++ is a powerful practice for enhancing code quality. However, it's crucial to distinguish between assertions and robust error handling. While assertions are a handy debugging tool during development, they should not replace conventional exception handling in production-level code.
By understanding and applying these principles, you'll cultivate a deeper grasp of error handling and debugging in C++. This approach not only enhances your coding skills but also contributes to the development of more reliable software.

FAQs
What is an assertion in C++? An assertion is a statement that verifies whether a condition is true in your program. If the condition is false, the program terminates with an error message.
Is it okay to use assertions in production code? While assertions are useful for debugging in development, they should be used with caution in production code as they can be disabled, leading to potential undefined behavior if the assertions trigger unexpectedly.
How can I avoid debug assertion failure in my projects? Avoid assertion failures by carefully validating your logic, ensuring correct memory management, and thoroughly testing boundary conditions in your code to capture potential errors early.

Additional Resources
Explore books and online courses focused on C++ debugging for in-depth knowledge, and participate in community forums for peer advice and shared experiences. Engaging with these resources will enhance your understanding and skills in addressing "debug assertion failed" issues effectively.