The `assert` macro in C++ is used to perform runtime checks and trigger an error message if a specified condition evaluates to false, helping developers identify logical errors during the debugging process.
#include <cassert>
int main() {
int x = 5;
assert(x > 0 && "Assertion failed: x should be greater than 0");
return 0;
}
What is an Assert in C++?
Definition of Assert
In C++, `assert` is a macro defined in the `<cassert>` header file that is primarily used to create assertions in your code. An assertion is a statement that specifies a condition that is expected to be true at a certain point in the program. If the condition evaluates to false, the program will terminate and display an error message indicating the failed assertion. This functionality makes it valuable for debugging during the development process.
Purpose of Assertions
Assertions serve a crucial role in C++ development, providing developers with a way to enforce invariants within their code. By using assertions, developers can ensure that certain conditions are met before the program proceeds. This early detection of errors allows for simpler code maintenance and reduces conflicts later in the code execution. Assertions act as a safety net, catching potential bugs before they lead to more significant issues.

How to Use Assert in C++
Basic Syntax of Assert
The syntax of the `assert` macro is straightforward. It takes a single expression as its argument. This expression is evaluated as a boolean condition. If the condition is true, the program continues execution. If it evaluates to false, the program displays an error message and stops running.
Here's a basic example illustrating how to use it:
#include <cassert>
int main() {
int x = 5;
assert(x > 0); // This assertion will pass
return 0;
}
In this example, `assert` validates that `x` is greater than zero. Since this condition is true, the program proceeds without any interruptions.
Using Assert with Message
Definition of Assert with Message
When you use assertions, adding a custom error message can significantly enhance the debugging experience. By providing an informative message, you easily communicate the reason for the assertion's failure, which can save valuable time when identifying issues in your code.
Syntax for Assert with Message
To implement an assertion with an accompanying message, you stack the condition with a logical AND operator `&&` followed by a string literal for the message. Here’s a code snippet showing how this can be done:
#include <cassert>
void checkPositive(int number) {
assert(number > 0 && "Number must be positive");
}
int main() {
checkPositive(-1); // This will trigger the assert
return 0;
}
In this code sample, the assertion checks if the `number` is positive. If it isn’t, the assertion fails and outputs the message "Number must be positive." This allows developers to pinpoint the source of the problem quickly.
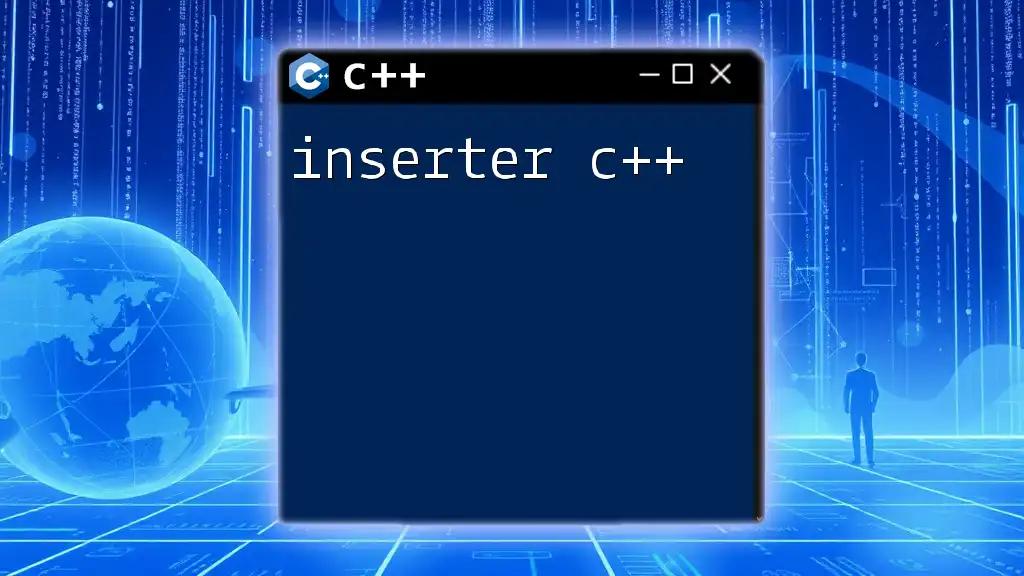
Benefits of Using Assert with Messages
Enhanced Debugging
One of the most significant advantages of utilizing assert messages is that specific messages can help identify issues promptly. Instead of just knowing an assertion failed, you get contextual information that can guide you toward the root cause of the problem. This is particularly useful in larger codebases where pinpointing errors can become quite complex.
Better Code Maintenance
By enhancing clarity within your codebase, assert messages contribute to better maintainability. If the assert fails, the message provides immediate feedback about the failed condition, reducing ambiguity. This practice proves beneficial not just for you, the original developer, but also for any future team members who may work on the project.
Attractiveness for Team Projects
Effective use of assertions with clear messages plays an essential role in team collaborations. In joint projects, clarity becomes even more critical. Assertions can help streamline communication among team members, allowing new developers to onboard quickly and understand the requirements and constraints set forth in the code.

Common Pitfalls and Best Practices
Pitfall: Overusing Assertions
While assertions are a powerful tool, overusing them can lead to cluttered code. Assertions should primarily respond to conditions that indicate crucial logical errors—conditions that should never occur. Using assertions to check for conditions that could happen during normal execution is ill-advised as it may lead to unnecessary termination of the program.
Best Practices for Assert Messages
Creating effective assert messages involves several best practices:
- Be specific and concise: Detail the exact problem without being verbose.
- Avoid jargon: Clear language ensures that corroborators and future developers comprehend the message.
- Focus on the condition: Highlight what went wrong, allowing for easy identification of the issue.
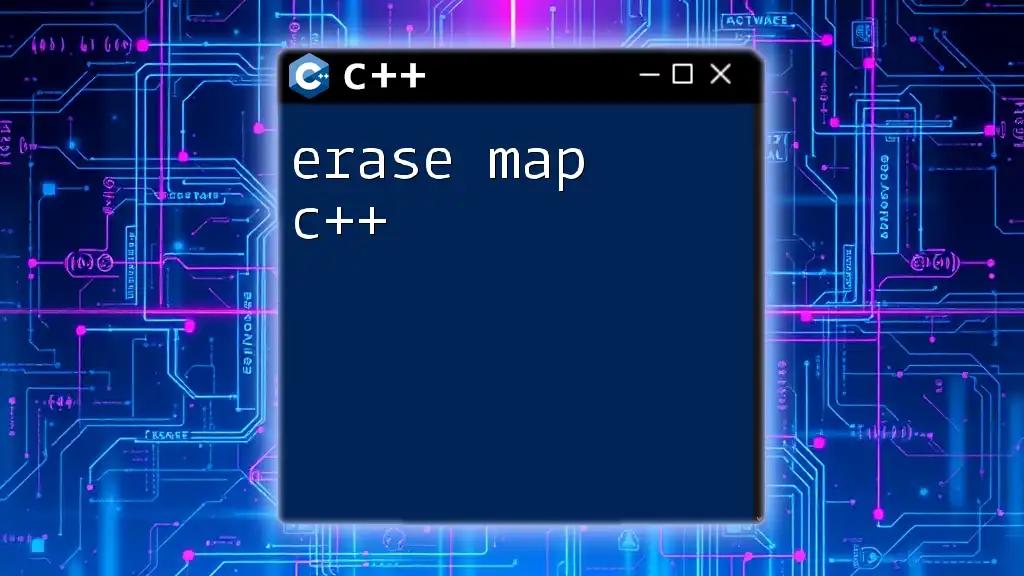
Alternatives to Assert in C++
Choosing Between Assert and Exception Handling
While `assert` is helpful, it is essential to recognize when to utilize other mechanisms like exception handling. Assertions are meant for debugging and are typically removed in production code, while exceptions are designed to indicate run-time errors.
#include <stdexcept>
void checkPositive(int number) {
if (number <= 0) {
throw std::invalid_argument("Number must be positive");
}
}
In this example, exception handling is appropriately used to handle cases where the input should be validated during normal execution, showing that assertions aren't always the right tool to use.
Other Debugging Techniques
Besides assertions and exceptions, additional debugging techniques can enhance code quality. Logging, static analysis, and various debugging tools can be employed alongside assertions, fostering a robust, multi-faceted approach to error handling and debugging.

Conclusion
In summary, assert message C++ plays a pivotal role in maintaining code integrity and enhancing the debugging process. Utilizing assertions with informative messages not only streamlines development but also contributes to better collaboration among programmers. By applying the best practices noted above, developers can transform assertions into a vital component of their coding toolkit.

Call to Action
Engage with more C++ programming topics and continue to enhance your skills. Discover additional resources and materials that strengthen your grasp on this powerful language and elevate your programming capabilities.

Additional Resources
For further learning, consider exploring:
- Official documentation on assertions in C++
- Recommended books on effective debugging techniques
- Online courses designed to improve your understanding of C++ programming and best practices
By adopting these strategies and tools, your journey in mastering C++ can be profoundly enhanced. Happy coding!