An "assertion failed" error in Microsoft Visual C++ occurs when an assertion statement evaluates to false, indicating that a condition assumed to be true in the code has failed, which can lead to debugging to identify the underlying issue.
Here's a code snippet that demonstrates an assertion in C++:
#include <cassert>
int main() {
int x = 5;
assert(x == 10); // This will trigger an assertion failure because the condition is false.
return 0;
}
Understanding Assertions
What is an Assertion?
An assertion is a conditional statement used in programming to evaluate whether a condition is true. If the condition evaluates to false, an assertion failure occurs, signaling a problem in the code. The primary purpose of assertions is to catch bugs during the development phase, which allows developers to identify issues early, rather than allowing them to propagate and complicate debugging later.
Assertions differ from exceptions in that assertions are primarily used for debugging and may be removed in production code. In contrast, exceptions handle expected runtime errors without abruptly terminating the program.
How Assertions Work in C++
In C++, assertions are typically implemented using the `assert` macro, defined in the `<cassert>` header. When the `assert` function is called, it checks a specified condition. If the condition is false, it triggers an assertion failure, interrupting the program and often providing a message that includes the line number in the source code where the failure occurred. This messaging is crucial, as it points developers directly to the issue.
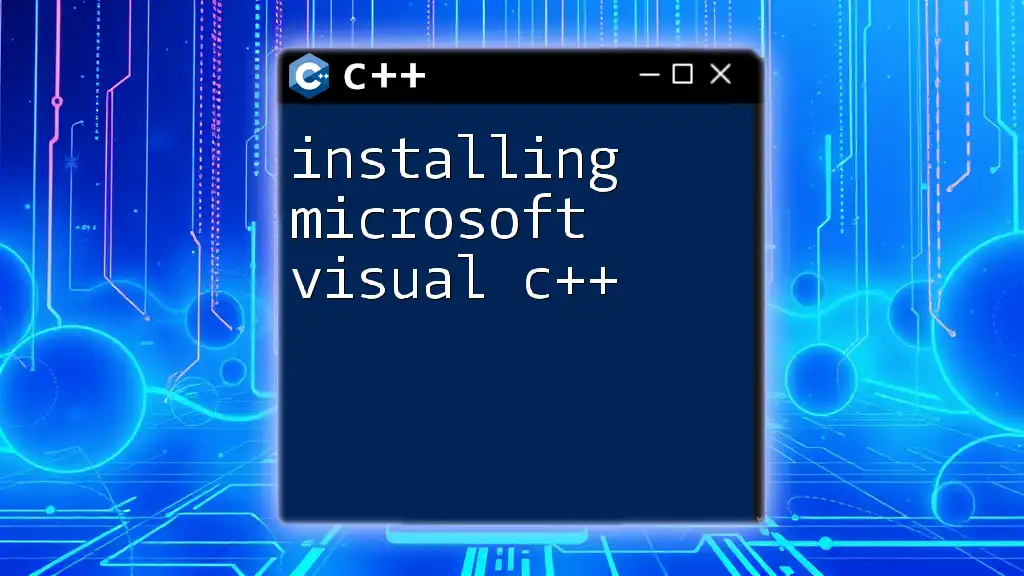
Assertion Failed in Microsoft Visual C++
What Does "Assertion Failed" Mean?
The phrase “Assertion Failed” is a diagnostic message produced by the Microsoft Visual C++ runtime when a condition specified in an assertion does not hold true. This usually indicates that there is a logical error in the code that violates the assumed conditions of the program's state.
Microsoft C++ Runtime Library Assertion Failed
The Microsoft C++ Runtime Library provides a rich set of functionalities, including memory management and error handling. An assertion failure within this library often occurs when the application encounters a situation that it cannot handle. The runtime's built-in assertions check for conditions that must be true, based on the expectations set by the code. Failing to meet these expectations leads directly to an assertion failure, thus halting program execution and aiding in debugging.
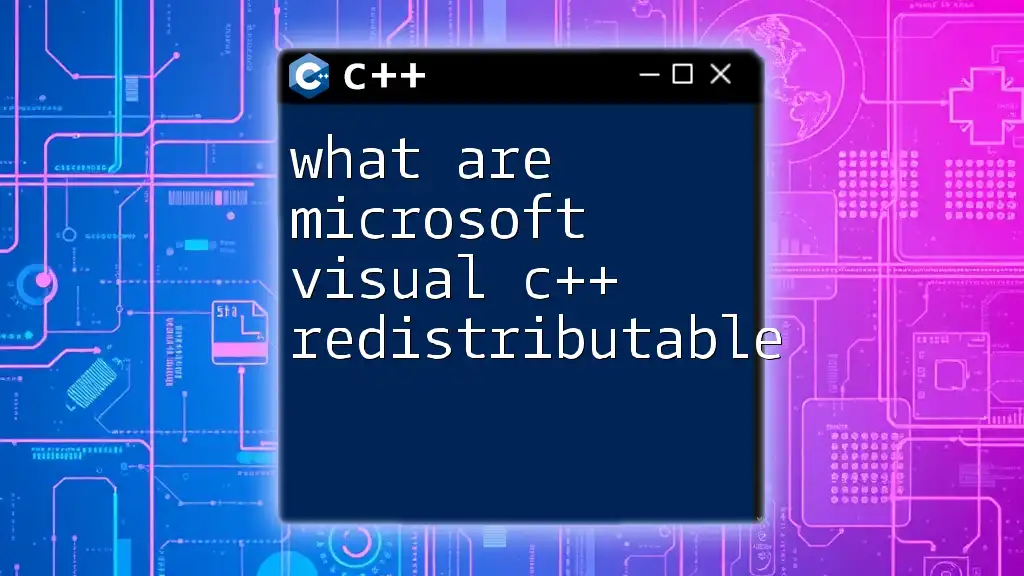
Common Causes of Assertion Failures
Uninitialized Variables
Using a variable that has not been initialized can lead to unpredictable behavior because the variable may contain garbage values. This problem is prevalent in C++, where local variables default to an indeterminate state. For instance:
#include <cassert>
void checkValue() {
int value; // Uninitialized variable
assert(value >= 0); // This assertion will fail
}
To resolve this, always initialize variables before using them:
#include <cassert>
void checkValue() {
int value = 0; // Now initialized
assert(value >= 0); // This will pass
}
Out-of-Bounds Access
Accessing elements outside of an array's bounds is another common source of assertion failures. This error can lead to crashes or data corruption. Consider the following example:
#include <cassert>
void accessArray(int* arr, int index) {
assert(index >= 0 && index < 10); // This will fail if index is out of bounds
int value = arr[index]; // If the assertion fails, this part will not execute
}
To prevent this issue, always ensure the index is within valid limits before accessing the array.
Invalid Function Parameters
Passing invalid parameters to functions can trigger assertion failures. This might occur if a function expects a pointer but receives `nullptr`. For example:
#include <cassert>
void processData(int* data) {
assert(data != nullptr); // This checks for valid pointer
// Process data...
}
To mitigate this, validate function arguments before proceeding with the logic. Always ensure that inputs meet the required criteria to avoid assertion failures.
Memory Management Issues
Improper management of dynamic memory can lead to assertion failures, particularly in complex applications that use `new` and `delete`. An example would be:
#include <cassert>
void deleteMemory(int* ptr) {
delete ptr;
delete ptr; // Attempting to delete memory that has already been freed
assert(ptr == nullptr); // This will fail
}
To avoid these issues, set pointers to `nullptr` after deleting:
#include <cassert>
void deleteMemory(int*& ptr) {
delete ptr;
ptr = nullptr; // Now we safe-guard against double deletion
}
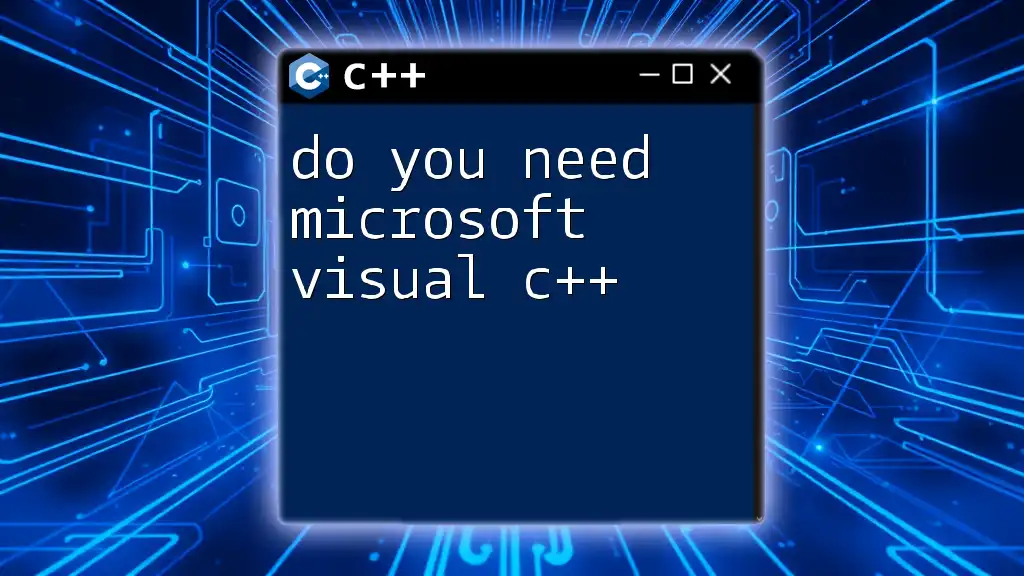
How to Debug Assertion Failures
Tools and Features in Visual C++
Microsoft Visual C++ provides robust debugging tools that can assist in diagnosing assertion failures. Utilize features such as breakpoints to pause execution at critical points, allowing you to inspect variable states and program flow through watch windows.
Step-by-Step Debugging Process
- Reproduce the Error: Ensure you can consistently trigger the assertion failure.
- Use Breakpoints: Set breakpoints at the assertion statement to inspect the variables involved.
- Check Stack Trace: Review the stack trace to determine how the program reached the failure point.
- Inspect Variable Values: Use the debugger to check the values of variables aligned with the assertion condition.
- Make Corrections: Once the issue is identified, revise the code to prevent the assertion from failing.
Example of a Failed Assertion and Debugging Walkthrough
Consider the following scenario where an assertion fails because of an out-of-bounds array access.
void fillArray(int* arr, int size) {
assert(size <= 10); // Assertion checking the size
for (int i = 0; i <= size; ++i) { // Off-by-one error
arr[i] = i;
}
}
When debugging, the assertion statement provides clues that the size parameter may be incorrectly managed. Inspecting the loop will reveal the off-by-one error, allowing you to modify it to `i < size`.
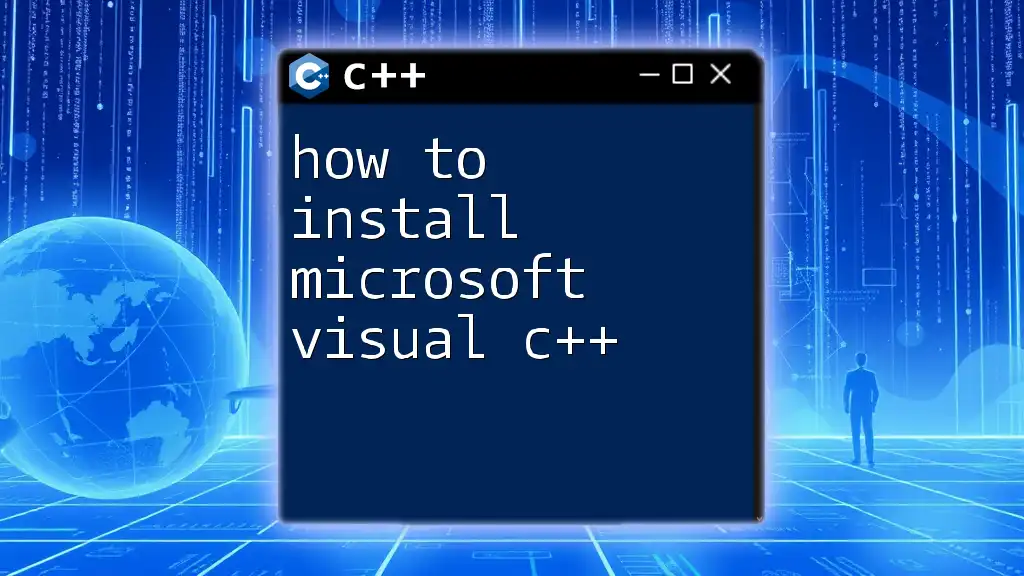
Best Practices for Using Assertions
When to Use Assertions
Assertions are most useful during the development phase when debugging is paramount. They help in validating assumptions about the code. However, avoid using assertions for handling runtime errors; instead, use exceptions for those situations.
Writing Meaningful Assertions
Crafting clear and informative assert messages can develop better understanding and maintainability. For example:
assert(userAge >= 0 && "User age cannot be negative");
This message provides immediate context about the state of the application and why the assertion failed.
Alternatives to Assertions
While assertions are helpful during the development process, they should not be relied upon for error handling in production-level code. Instead, consider using exceptions to manage known issues that can arise while running the application.
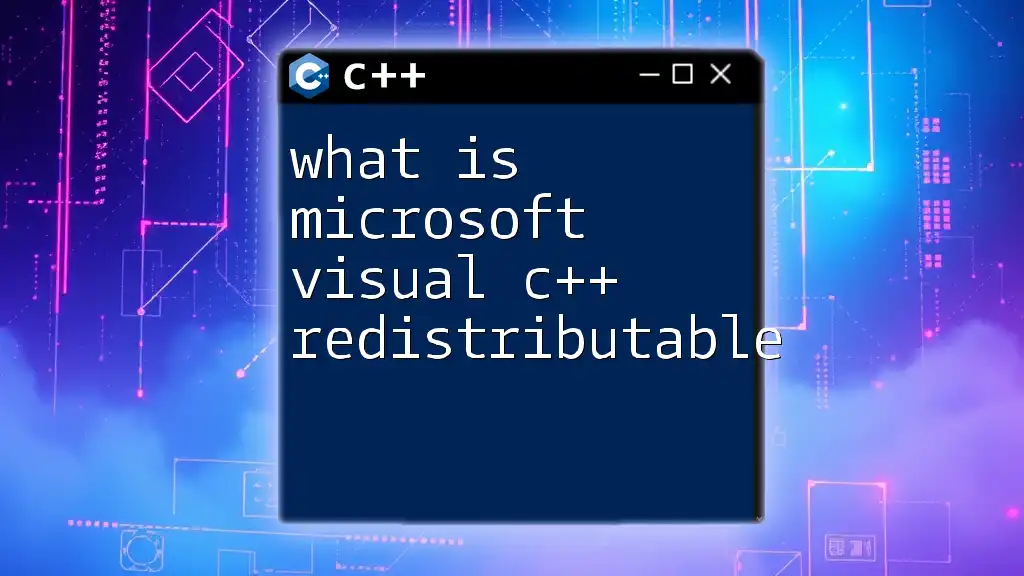
Conclusion
In summary, understanding and correctly implementing assertions in Microsoft Visual C++ can greatly enhance the process of debugging and writing reliable code. From identifying the causes of assertion failures to utilizing effective debugging techniques, embracing assertions will improve code quality and development efficiency.
Engage actively with the community and share your experiences with assertion failures, as collective knowledge is vital for overcoming common programming challenges.