Microsoft Visual C++ is an integrated development environment (IDE) that provides tools for developing applications, libraries, and components using the C++ programming language, facilitating efficient coding, debugging, and performance optimization.
Here’s a simple code snippet demonstrating how to output "Hello, World!" in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Microsoft Visual C++?
Microsoft Visual C++ (MSVC) is an integrated development environment (IDE) from Microsoft designed specifically for C and C++ development. Providing a comprehensive suite of tools for software developers, it has grown significantly since its inception, evolving through multiple versions to support modern programming standards and development practices. Today, it plays a crucial role in creating high-performance applications across various platforms, primarily Windows.
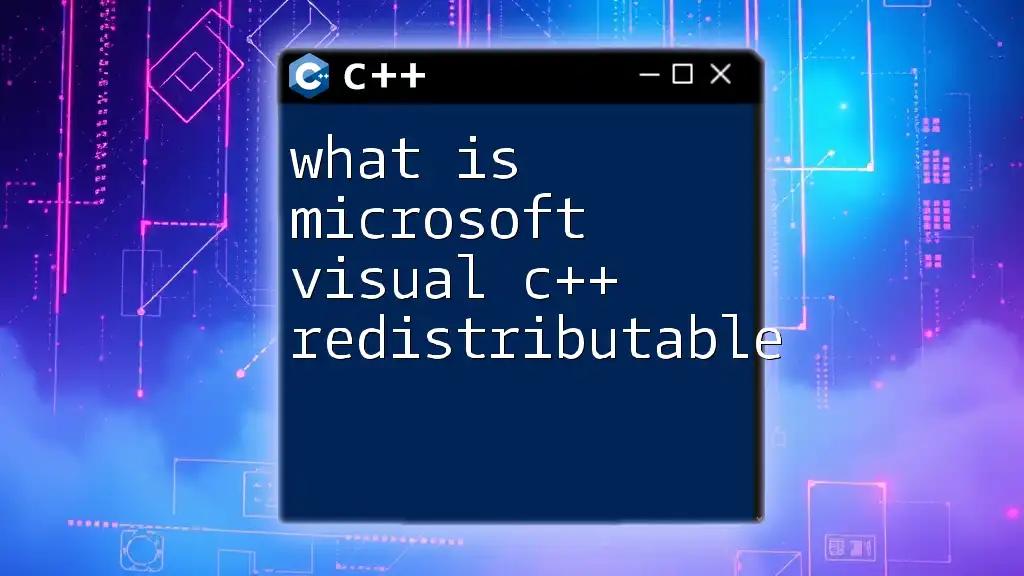
Key Features of Microsoft Visual C++
Integrated Development Environment (IDE)
The MSVC IDE is a powerful environment that includes various tools like a code editor, debugging tools, and UI design capabilities. It integrates features that streamline the coding process, allowing developers to write and manage code efficiently. The code editor offers syntax highlighting, auto-completion, and sophisticated navigation features to enhance productivity.
Compiler and Linker
At the heart of MSVC is its compiler, which translates C++ code into machine code. The compiler supports C++ language standards and various extensions that improve performance and usability. The linker plays a critical role in combining different code modules and libraries into a single executable, making it essential for building applications. This interplay between compiling and linking ensures that developers can create robust applications tailored to specific requirements.
Standard Libraries
MSVC incorporates the C++ Standard Library, providing developers with a comprehensive set of pre-written classes and functions. These libraries facilitate common tasks, such as input/output operations, data structure manipulation, and algorithms. By leveraging these resources, developers can focus on building unique features rather than reinventing the wheel.
Platform Support
MSVC is primarily designed for Windows development, making it an ideal choice for creating Windows-based applications. However, it also supports the development of applications for platforms like Windows Phone and Universal Windows Platform (UWP), as well as limited capabilities for cross-platform development through various project configurations.
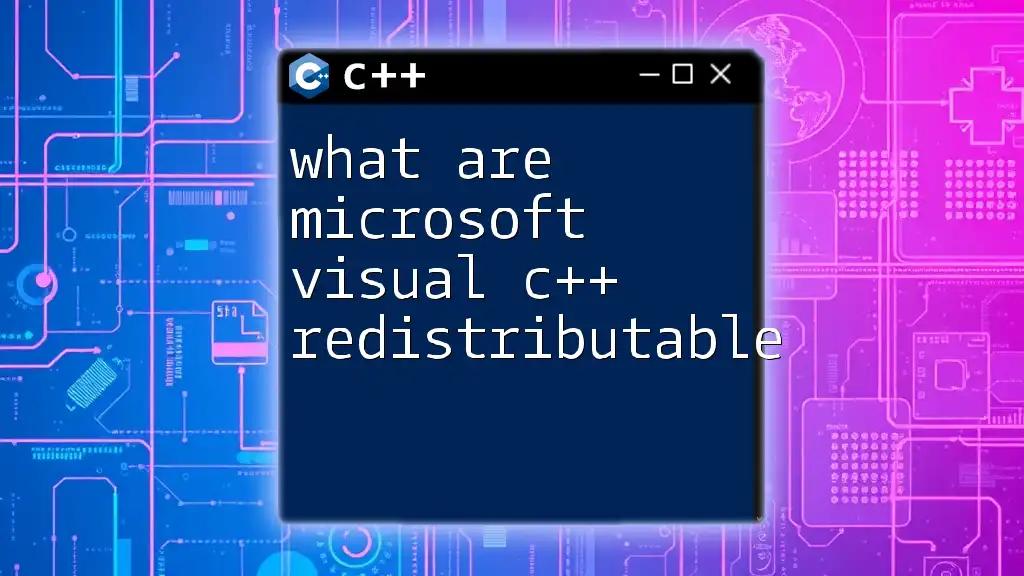
Getting Started with Microsoft Visual C++
Installation Process
To get started with MSVC, install Visual Studio, which encompasses the Visual C++ tools. The installation process involves downloading the Visual Studio installer, selecting the desired components (including the C++ workload), and following the on-screen instructions. It's essential to check system requirements to ensure compatibility for a seamless setup.
Creating a New Project
Once installed, creating a new C++ project is straightforward. Open Visual Studio, select "Create a New Project," and choose the C++ template that suits your objectives. Whether it's a Console Application, Windows Desktop Application, or a more complex setup, MSVC provides various templates that pre-configure essential project settings. Understanding these settings allows you to tailor your project for specific needs effectively.
Understanding the Workspace
The MSVC workspace consists of a solution explorer, properties panel, and editor windows. The solution explorer showcases the project's files and folder structure, permitting easy navigation. Familiarizing yourself with these components is critical for efficient coding and project management.

Writing Your First C++ Program
Code Explanation
To write a basic C++ program, start with a simple "Hello, World!" example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code snippet, `#include <iostream>` includes the necessary library for input/output operations. The `main` function serves as the entry point of the program, while `std::cout` sends the message to the console.
Compiling the Program
Compiling your program in MSVC is achievable with one click of the "Build" button. This triggers the build process, where the compiler checks for errors. If there are any syntax mistakes, MSVC will provide error messages that help identify and rectify issues. Common compilation errors may include missing semicolons or misnamed variables.
Debugging Tools
MSVC offers robust debugging features that allow developers to find and fix issues efficiently. You can set breakpoints to pause program execution and inspect variable values at runtime. For example, by clicking on the left margin next to a line of code, you can place a breakpoint. When the program hits this line, you can examine the current state of variables and control flow, enabling you to make informed decisions about necessary changes.

Advanced Features in Microsoft Visual C++
Smart Pointers and Memory Management
Modern C++ emphasizes memory management and resource safety. MSVC supports smart pointers, which automate memory management, significantly reducing memory leaks. Here’s an example with `std::shared_ptr`:
#include <memory>
void example() {
std::shared_ptr<int> ptr = std::make_shared<int>(42);
// ptr will automatically be deallocated when it goes out of scope
}
This code snippet illustrates how `shared_ptr` manages dynamically allocated memory automatically, enhancing program safety and performance.
Templates and Generic Programming
C++ templates allow developers to write flexible and reusable code. Templates can be applied to functions and classes, enabling them to operate with any data type. Here is an example of a simple template function:
template <typename T>
T add(T a, T b) {
return a + b;
}
This function can now be used with various data types, such as integers or floating-point numbers, promoting code reuse and maintainability.
Multi-threading Support
MSVC provides tools to create multi-threaded applications, which is increasingly vital in modern software to achieve better performance. Developers can create and manage threads using the `<thread>` header. Here’s a simple example of starting a thread:
#include <iostream>
#include <thread>
void threadFunction() {
std::cout << "Hello from a thread!" << std::endl;
}
int main() {
std::thread t(threadFunction);
t.join(); // Wait for the thread to finish
return 0;
}
In this example, the `threadFunction` runs in a separate thread, illustrating how multithreading can be utilized to handle concurrent tasks efficiently.
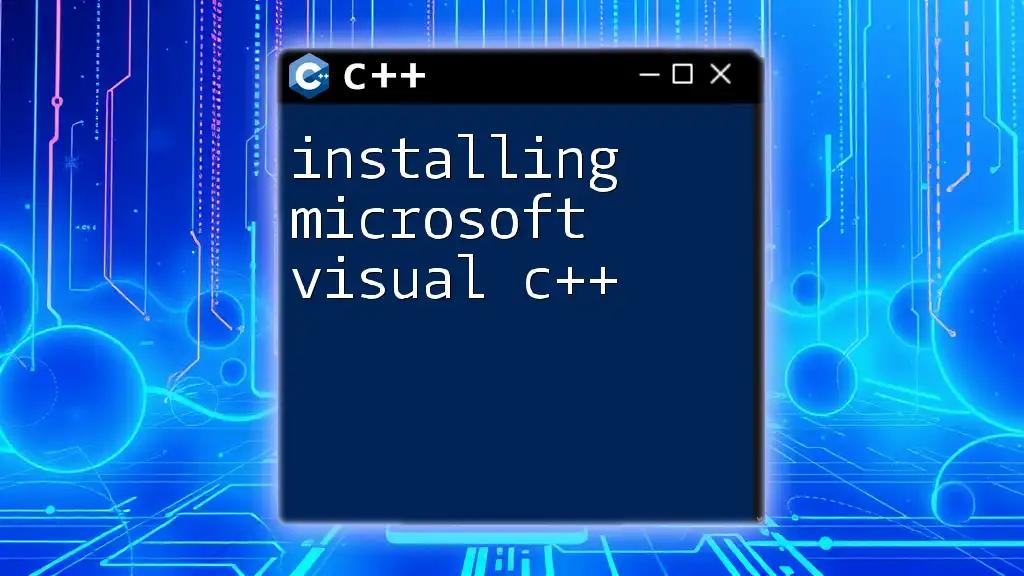
Common Use Cases for Microsoft Visual C++
Game Development
MSVC is a popular choice in game development, particularly because it seamlessly integrates with game engines like Unreal Engine and Unity. Many developers leverage MSVC's powerful debugging and optimization capabilities to produce high-performance games that require significant computational resources.
Application Development
MSVC is versatile for creating various applications, from simple console applications to complex desktop software. Its robust feature set supports the entire development lifecycle, making it easier for developers to build effective Windows applications.
Embedded Systems
In embedded programming, MSVC provides tools needed for developing firmware and software for devices with stringent resource constraints. Using C++ in embedded systems allows for higher performance and better resource management, making MSVC a solid choice for such applications.
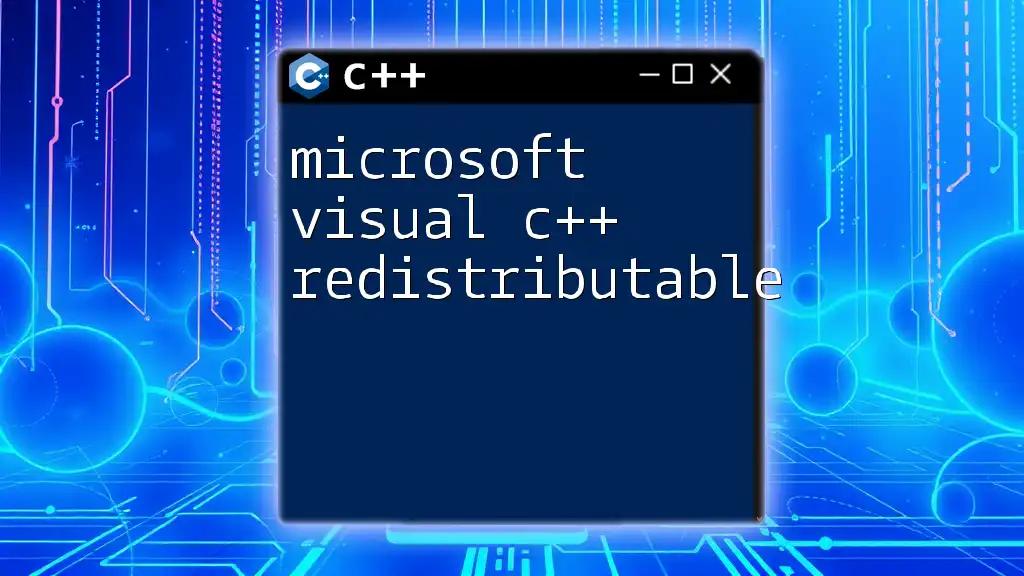
Best Practices for Using Microsoft Visual C++
Code Organization
Structuring your project logically is essential to maintaining clean and manageable code. Group related files and modules together, and utilize namespaces to avoid naming collisions. A well-organized project structure improves readability and simplifies collaboration among team members.
Effective Debugging Techniques
Effective debugging can save development time. Utilize MSVC's debugging tools to step through code systematically. Prioritize understanding error messages, inspect variable states, and utilize logging to track program behavior. These strategies can lead to more efficient problem-solving.
Performance Optimization
Optimizing C++ programs entails writing efficient code and using the right tools for analysis. MSVC provides profiling tools that help identify performance bottlenecks. Techniques such as using the correct data structures, minimizing copies, and leveraging algorithms can significantly boost application performance.
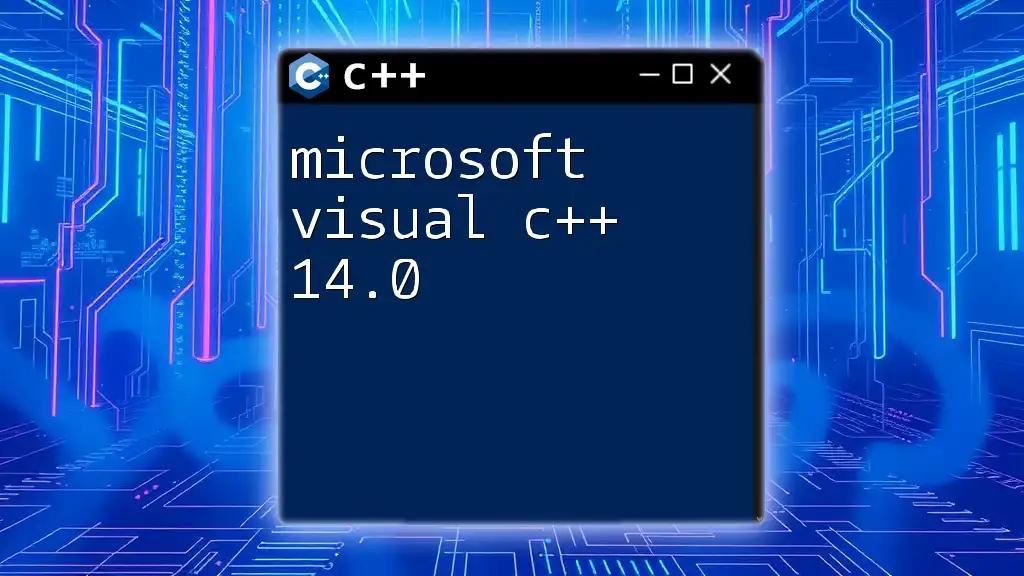
Conclusion
Understanding what Microsoft Visual C++ does is essential for developers looking to create efficient and high-performance applications. From its robust IDE and comprehensive libraries to advanced features like smart pointers and multi-threading support, MSVC provides developers with the tools necessary to excel in their craft. Embracing these capabilities while adhering to best practices can help in delivering high-quality software tailored to specific needs.
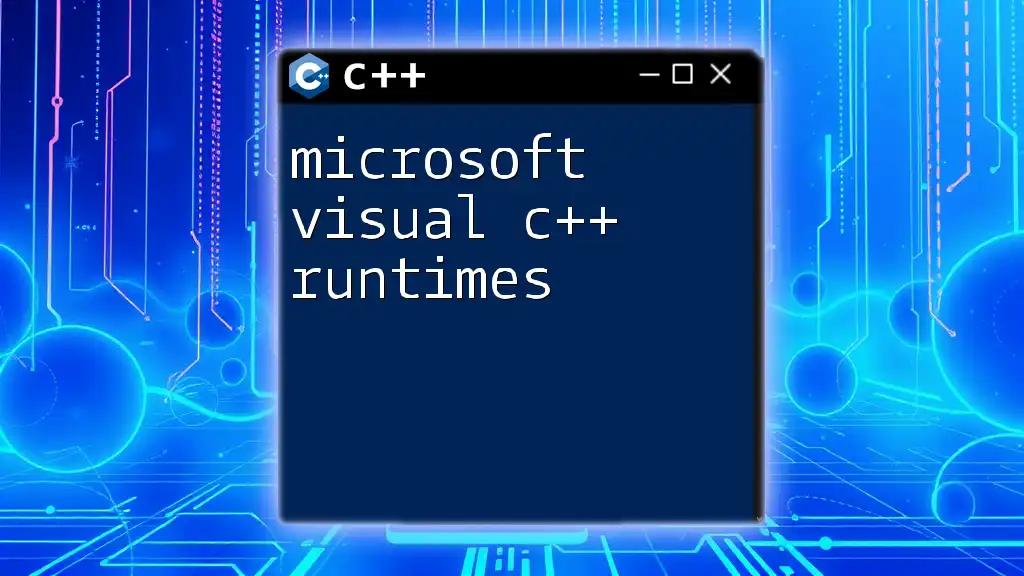
Additional Resources
For further exploration, refer to Microsoft's official documentation on Visual C++, engage with online communities for support, and consider enrolling in courses to deepen your understanding of C++ programming with MSVC.
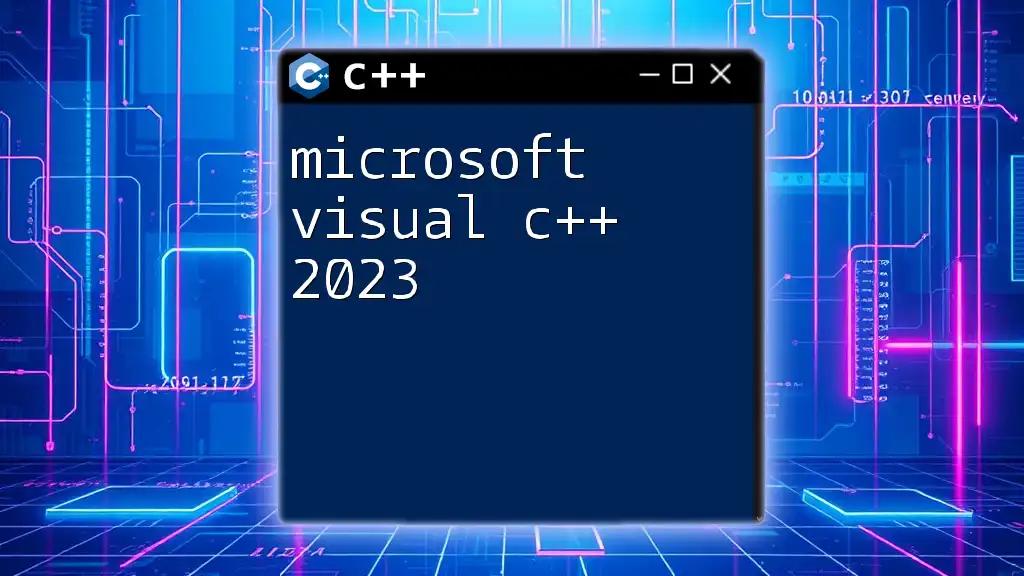
Call to Action
We encourage you to try out Microsoft Visual C++ for your next project! Share your experiences, tips, and questions in the comments below. Don’t forget to check out our services to enhance your C++ programming skills and unlock your potential.