A designated initializer in C++ allows you to specify values for specific members of a structure or class at the time of initialization, enhancing code clarity and precision.
struct Point {
int x;
int y;
};
Point p = {.y = 10, .x = 5}; // Designated initializer
Introduction to Designated Initializers
What Are Designated Initializers?
Designated initializers are a feature in C++ that allow developers to initialize the members of a struct or class in a more intuitive and readable manner. By explicitly stating the member names when assigning values, we gain clarity in how each member is set. Unlike traditional initialization, which can lead to confusion when the structure contains many members or when certain members are omitted, designated initializers offer a clean and straightforward syntax.
Why Use Designated Initializers?
Utilizing designated initializers can vastly improve the readability of your code. They clarify which value corresponds to which member of a struct or class, making it easier for others (and future you) to understand the intention behind the code. Furthermore, this method provides greater flexibility in code maintenance, allowing for the addition or modification of members without having to reorder the initialization values, thereby minimizing error. Designated initializers are particularly useful in scenarios where you are working with complex data structures or aggregate types.

Understanding C++ Designated Initializers
Basics of Designated Initializers in C++
In C++, the syntax for designated initializers is simple yet powerful. It uses a combination of curly braces, member names, and assigned values. While traditional constructors typically rely on the order of parameters, designated initializers allow you to specify the member's name directly, enhancing both clarity and safety.
The Syntax of Designated Initializers
To employ designated initializers, you define your struct or class and specify values using the member names. Here’s an example demonstrating this syntax:
struct Point {
int x;
int y;
};
Point p1 = {.x = 10, .y = 20};
In this example, the struct `Point` has two integer members: `x` and `y`. Using designated initializers, we can directly specify their values, making it immediately clear which value corresponds to which member.
Using Designated Initializers with Aggregate Types
Designated initializers are especially beneficial when initializing aggregate types, which are types that do not have user-defined constructors or private/public members. Here’s an example illustrating how to initialize an array of aggregates using designated initializers:
struct Rectangle {
int width;
int height;
};
Rectangle rects[2] = {
{.width = 20, .height = 30},
{.width = 40, .height = 50}
};
This practice enhances readability and reduces the risk of mistakenly assigning a value to the wrong member.
Limitations of Designated Initializers
Supported Types and Structures
It's important to note that designated initializers are limited to aggregate types. If you attempt to use them with classes that have user-defined constructors, you'll encounter difficulties. In such cases, it's advisable to revert to traditional initialization methods or constructors.
Compatibility Considerations
Another point to consider is the compatibility of designated initializers among different C++ standards and compilers. While they are widely supported in modern C++ (C++11 and later), users should verify their support in their particular development environment to avoid potential issues.

Practical Examples of Designated Initializers
Struct Initialization using Designated Initializers
Let’s delve into a more comprehensive example. Imagine you are working on a program that manipulates various shapes. Using designated initializers to manage shape properties can significantly elevate the readability of your code:
struct Circle {
double radius;
double area;
};
Circle circle = {.radius = 5.0, .area = 78.54}; // area is pre-calculated
In this code, it’s evident that `radius` and `area` are being set explicitly. This transparency reduces misunderstandings that might arise when dealing with multiple members, especially if their types are similar.
Complex Objects with Designated Initializers
Designated initializers become even more valuable when dealing with nested structs. Consider the following example where we have a struct containing another struct:
struct Color {
int red;
int green;
int blue;
};
struct Rectangle {
int width;
int height;
Color fillColor;
};
Rectangle rect = {
.width = 15,
.height = 30,
.fillColor = {.red = 255, .green = 0, .blue = 0} // Initializing nested struct
};
This clearly illustrates how designated initializers help maintain clarity, even in more complex structures, by specifying not only the main structure’s members but also the members of nested structs.
Tips for Effective Use
Best Practices for Coding with Designated Initializers
To maximize the benefits of designated initializers, adhere to best practices. Always use them in situations where clarity is paramount. Keep initialization compact when possible, but don't shy away from breaking down more complex structures into manageable pieces. This practice allows others to easily grasp your code without extensive comments.
Performance Considerations
While the performance impact of designated initializers is generally negligible, it’s essential to maintain an understanding that less straightforward syntax may lead to marginally different runtime behaviors, especially in performance-critical sections. Test the performance in such cases and use established practices as necessary.
![Understanding Unsigned Int in C++ [Quick Guide]](/_next/image?url=%2Fimages%2Fposts%2Fu%2Funsigned-int-cpp.webp&w=1080&q=75)
C++ Designated Initializers vs Other Initialization Methods
Comparison with Constructor Initialization
When comparing designated initializers to constructor initialization, each method has its inherent advantages. Designated initializers shine in terms of clarity and ease, especially in structs and aggregates. In contrast, constructor initialization provides a more controlled environment for complex logic and member validation.
Designated Initializers vs Uniform Initialization
Designated initializers offer an excellent alternative to uniform initialization methods (using `{}` syntax). While both emphasize clarity, designated initializers explicitly name members, which significantly reduces ambiguity when working with multiple members of similar types.
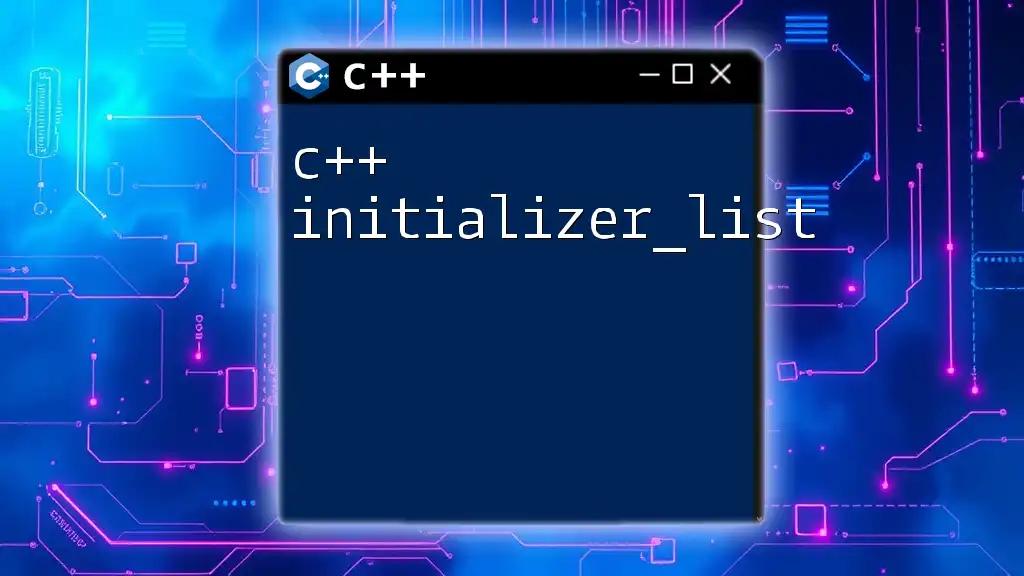
Conclusion
Designated initializers in C++ represent a powerful tool for developers seeking clarity and efficiency in their code. By adopting this method, you enhance the readability of your structures while reducing the potential for errors during initialization. With the clear syntax and flexibility in maintenance, this feature is invaluable in modern C++ programming. For further learning, consider exploring more advanced topics or engaging with the programming community to deepen your understanding.

Call to Action
Join our community for more tips and tutorials on mastering C++. Participating actively will deepen your understanding, as real-world implementations of designated initializers are explored and discussed. Try the examples in this guide, tweak them, and share your results and insights in the comments!