In C++, declaring a variable involves specifying its type and name, while initializing it assigns a value; for example, to declare and initialize an integer variable named `age` with a value of `25`, you can use the following code:
int age = 25;
What are Variables in C++?
Variables are fundamental components in programming that allow developers to store, manipulate, and retrieve data. In C++, a variable serves as a named space in memory where data is kept, and its value can change throughout program execution. Understanding variables is essential for effective programming as they directly influence how data is processed.
Types of Variables
In C++, variables can be classified into different types based on their scope and lifespan:
- Local Variables: These variables are declared within a function or block and can only be accessed within that specific scope.
- Global Variables: These are declared outside any function and have a program-wide scope, making them accessible from any function.
- Static Variables: These variables retain their value between function calls, even if they are declared within a function.
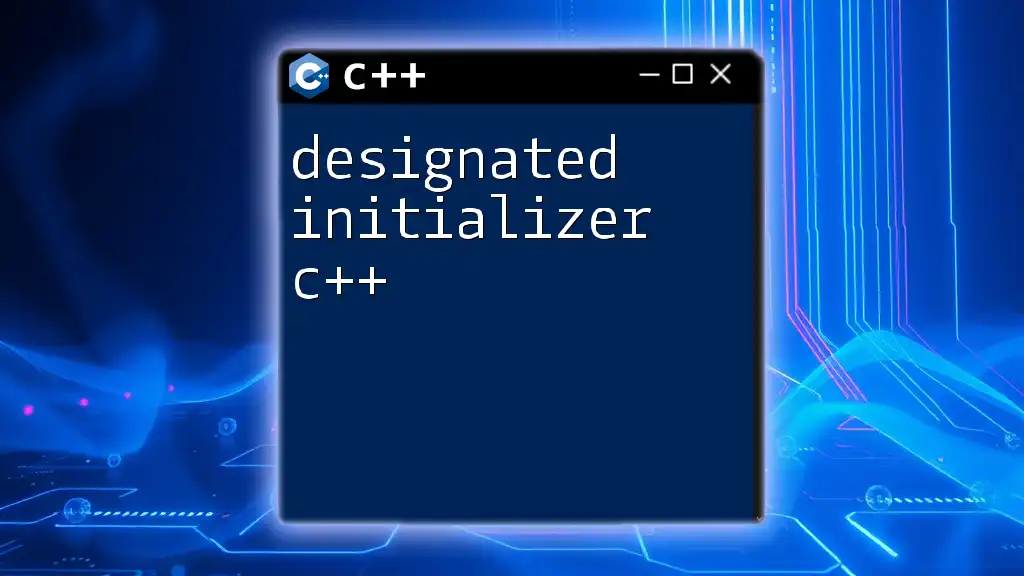
Understanding Variable Declaration
What is Variable Declaration?
Variable declaration is the process of creating a variable and specifying its data type. This step is crucial as it informs the compiler about the type of data that the variable will hold. C++ is a statically typed language, which means that all variable types must be declared before they can be utilized.
Syntax of Variable Declaration
The basic syntax for declaring a variable in C++ follows this pattern:
dataType variableName;
For example, to declare an integer variable named `number`, you would use:
int number;
Declaration without Initialization
Variables can be declared without being initialized. This means that the variable is given a name and a data type, but no value is assigned to it initially.
int age; // declared but not initialized
It's important to note that uninitialized variables can lead to undefined behavior since they may contain garbage values.
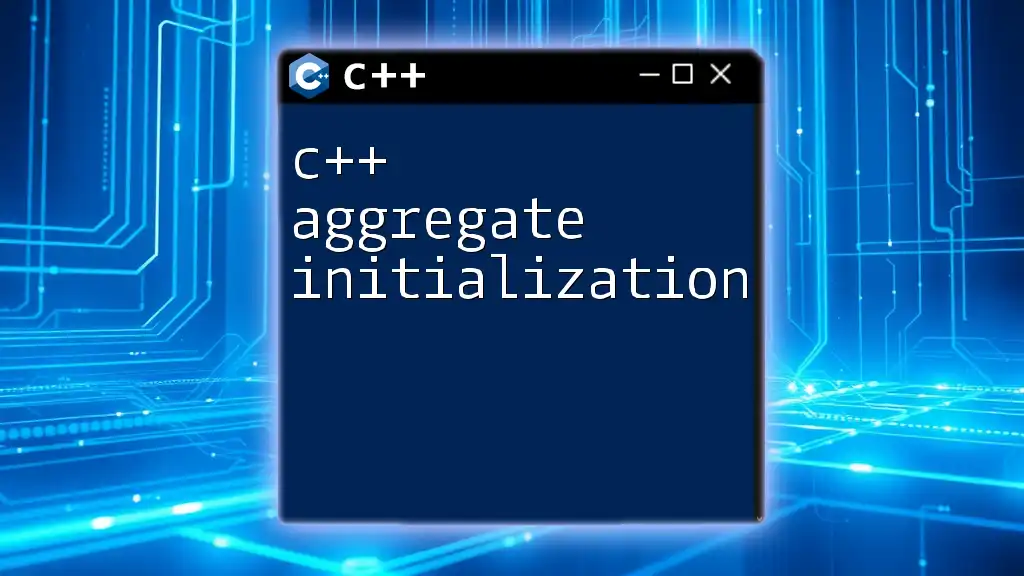
Understanding Variable Initialization
What is Variable Initialization?
Variable initialization is the act of assigning a value to a variable during its declaration or afterwards. This vital step ensures that a variable has a defined value before it is used in expressions or statements.
Syntax of Variable Initialization
To initialize a variable, you can use the following syntax:
dataType variableName = initialValue;
For example, to declare and initialize a variable named `number` with the value 10, the code would look like:
int number = 10;
Initialization at Declaration
When you initialize a variable at the time of declaration, you not only create the variable but also set its initial value. This practice is often recommended as it helps avoid mistakes associated with uninitialized variables.
float pi = 3.14f; // initialized at the time of declaration
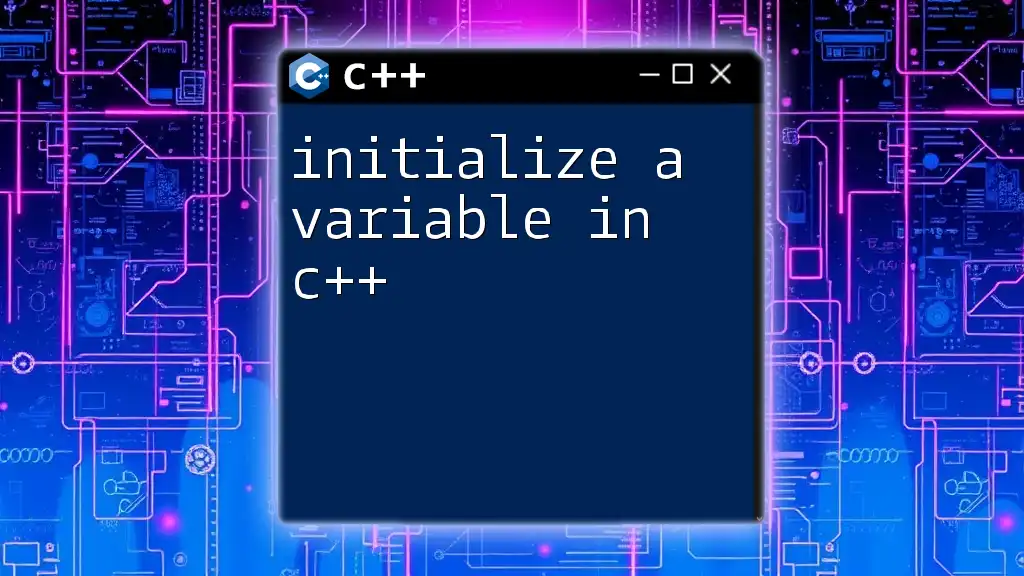
Types of Initialization in C++
Implicit Initialization
Implicit initialization occurs when a variable is declared but not explicitly assigned a value, often initializing it to zero or a default state.
int x{}; // x is initialized to 0
Explicit Initialization
Explicit initialization is when you assign a specific value during the declaration of the variable.
double y = 45.67; // explicit initialization
Copy Initialization
In copy initialization, the value of another variable can be assigned to a new variable at the time of declaration.
int z = number; // copying value of number to z
Uniform Initialization (C++11 and later)
Introduced in C++11, uniform initialization uses curly braces to prevent narrowing conversions and provide a consistent way to initialize variables.
int a{10}; // uses curly braces
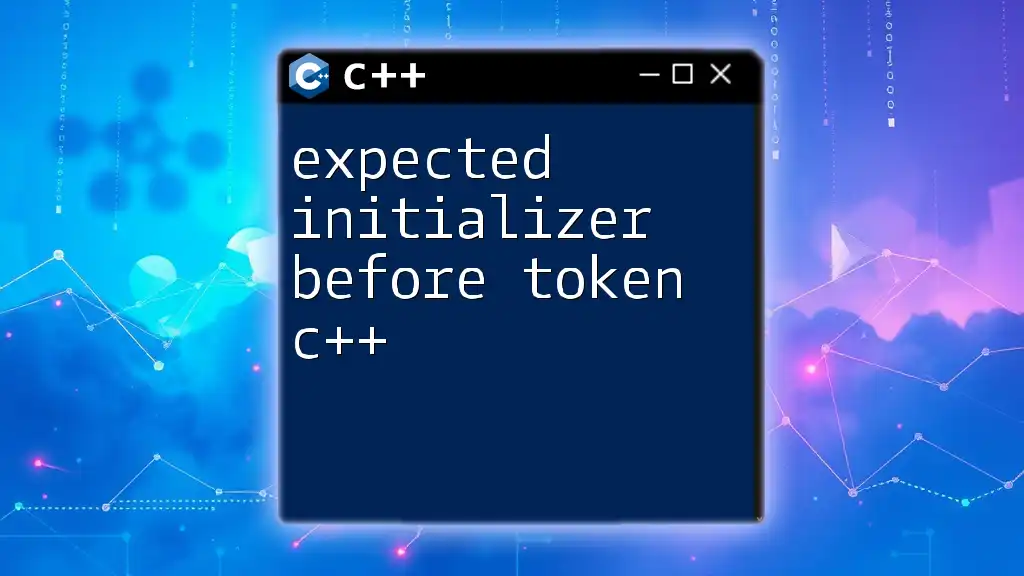
The Importance of Choosing the Right Type
Basic Data Types
In C++, a variety of basic data types can be chosen depending on the nature of the values you want to store. These include:
- int: represents integers (e.g., `int count = 5;`)
- float: for single-precision floating-point numbers (e.g., `float temperature = 36.6;`)
- double: for double-precision floating-point numbers (e.g., `double piValue = 3.14159;`)
- char: for single characters (e.g., `char grade = 'A';`)
- bool: for Boolean values (true or false).
User-Defined Data Types
C++ also allows the creation of user-defined types like structures and classes, enhancing the flexibility of variable use.
For instance, you can define a structure representing a person:
struct Person {
std::string name;
int age;
};
Person p{"John", 25}; // user-defined type initialization
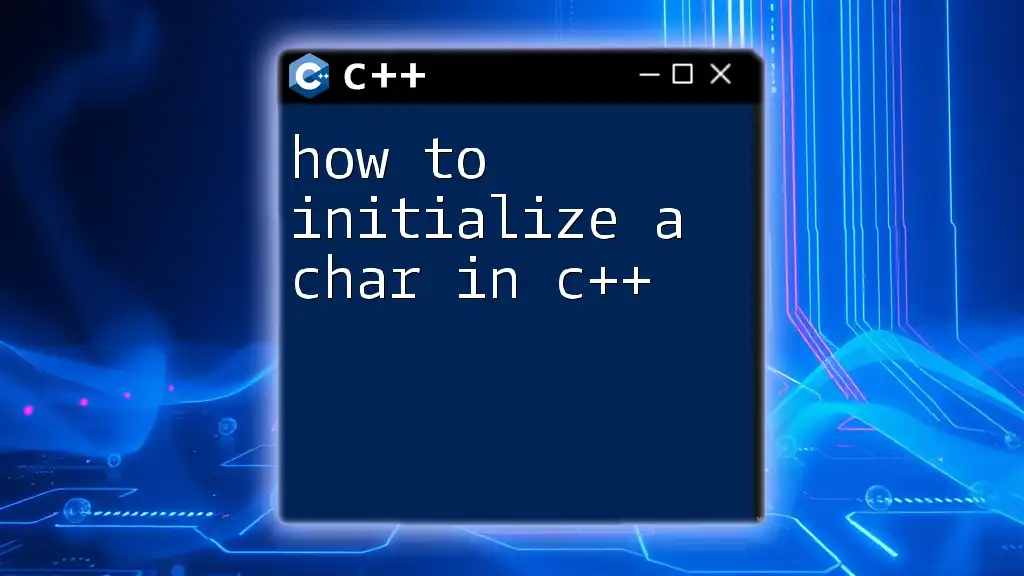
Scope and Lifetime of Variables
The Concept of Scope
The scope of a variable determines where it can be accessed within the code. Local variables are limited to the function or block in which they are declared, while global variables can be accessed from any function within the program.
Lifetime of Variables
Lifetime refers to the duration a variable can hold its state in memory. Local variables exist only while the block in which they are defined is executing, whereas global variables remain until the program terminates. Static variables, declared with the `static` keyword, maintain their value between function calls.
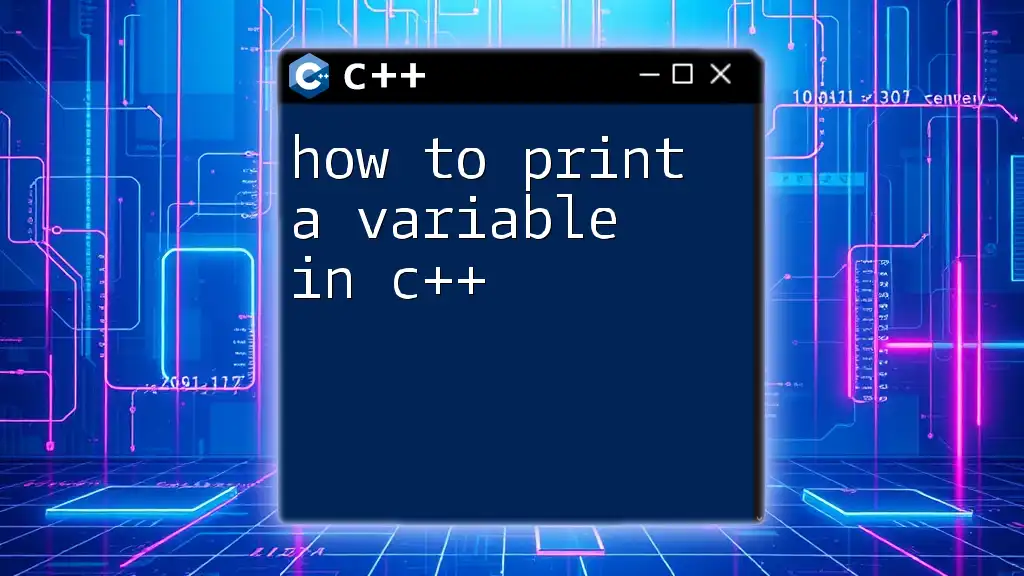
Common Mistakes in Variable Declaration and Initialization
Uninitialized Variables
One of the most common mistakes is using uninitialized variables, which can yield unpredictable results, often referred to as "undefined behavior." For instance:
int uninitialized;
std::cout << uninitialized; // may output garbage value
Type Mismatch
Another common pitfall is attempting to assign a value to a variable that does not match its data type. This results in compilation errors. For example:
int num = "string"; // causes a compilation error
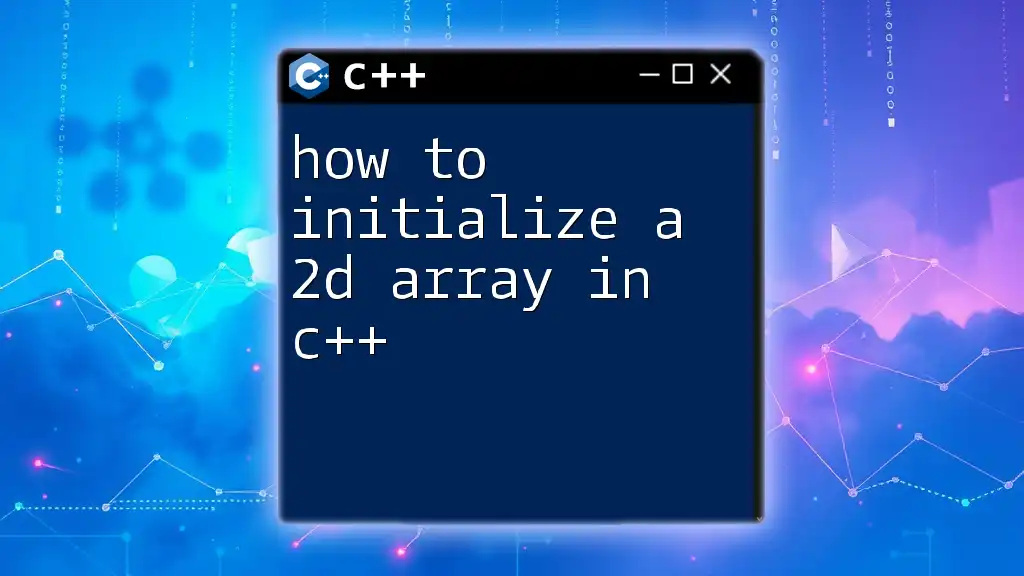
Best Practices for Variable Declaration and Initialization
Naming Conventions
When declaring variables, it’s best to use meaningful names that describe their purpose clearly. This practice enhances code readability and maintainability. For instance, instead of naming a variable `x`, consider naming it `numberOfStudents`.
Avoiding Magic Numbers
Always use named constants instead of "magic numbers" that can make code confusing. By defining constants, you enhance the clarity of your code. For example:
const int MAX_SIZE = 100; // using constants instead of magic numbers
Consistency in Declaration and Initialization
Strive for consistency in how you declare and initialize variables. Keeping a clear and structured method aids readability and reduces errors.
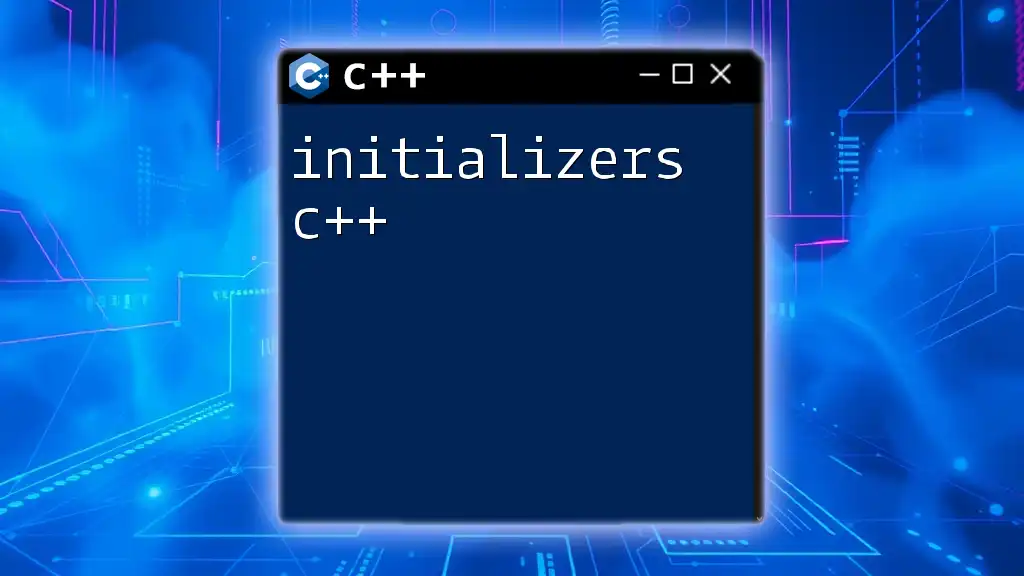
Conclusion
In summary, understanding how to effectively declare and initialize C++ variables is essential for any budding programmer. This knowledge allows for proper data storage and manipulation within a program. By practicing the principles outlined above, you will enhance your skills in C++ programming, leading to more efficient and reliable code. For continued learning, consider exploring additional resources and practice coding regularly to solidify your understanding.