To initialize a 2D array in C++, you can define it with specific dimensions and assign values using braces for each row, as shown in the following code snippet:
int array[3][4] = {{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}};
What is a 2D Array?
A 2D array is essentially a collection of elements organized in a grid, represented by rows and columns. Unlike 1D arrays, which store elements in a linear format, 2D arrays allow you to represent data in a more structured way, making them particularly useful for applications such as:
- Matrices for mathematical computations
- Game boards, such as grids in chess
- Image representation, where pixels can be accessed via coordinates
Understanding how to utilize 2D arrays is critical for efficient programming in C++.
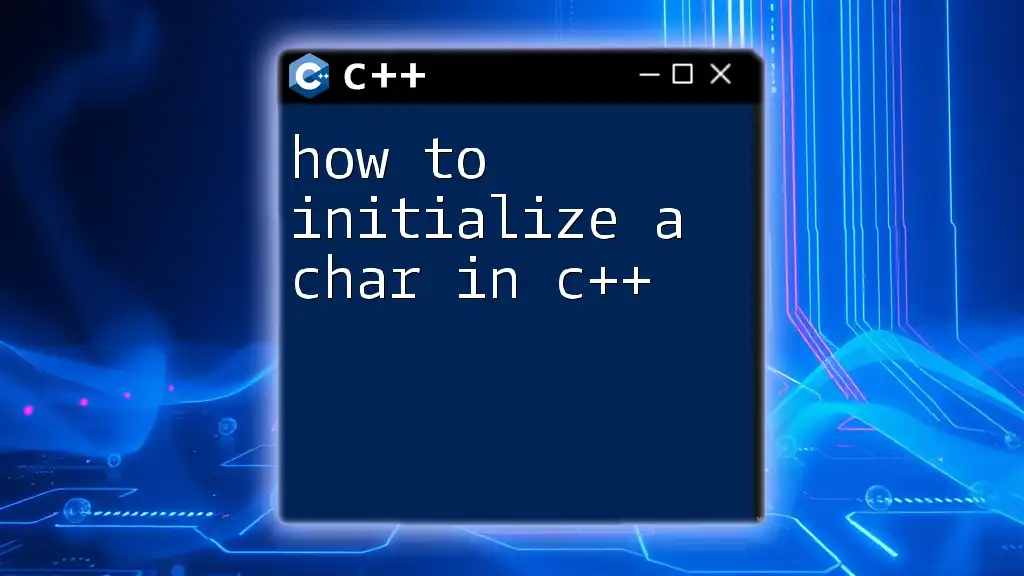
How to Declare a 2D Array in C++
Declaring a 2D array in C++ requires specifying the type and dimensions. The general syntax is as follows:
datatype arrayName[rows][columns];
For instance, to declare a 2D array of integers with 3 rows and 4 columns, you would write:
int arr[3][4];
Here, `rows` refers to the number of horizontal arrays, while `columns` refers to the size of each horizontal array. Choosing the appropriate data type is crucial since it dictates what kind of elements the array will hold.
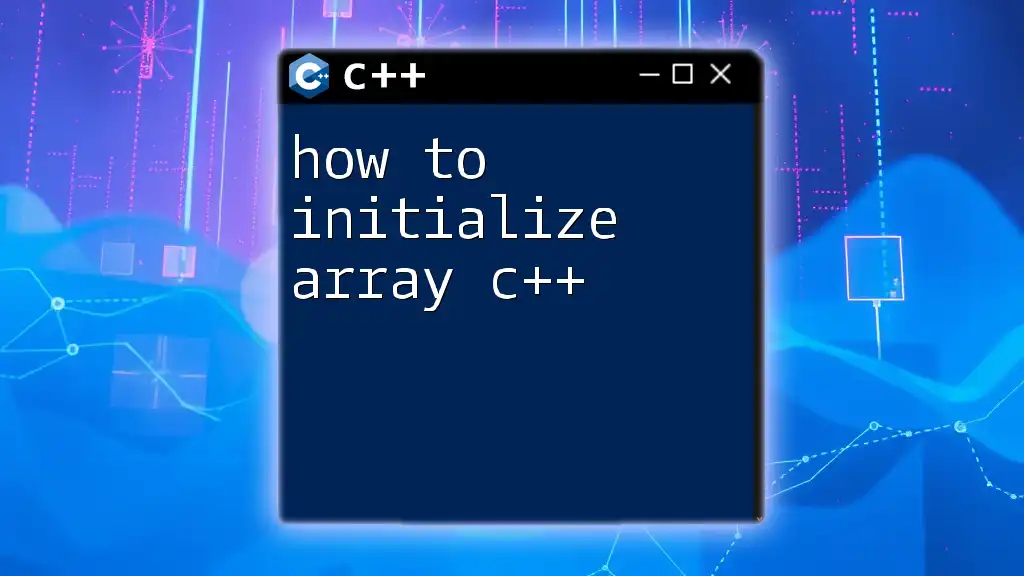
Different Methods to Initialize a 2D Array in C++
Initialize a 2D Array at the Time of Declaration
One of the easiest ways to initialize a 2D array is by doing it at the time of declaration. This approach is known as static initialization. The syntax is straightforward:
int arr[3][4] = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}
};
In this example, we've defined a 3x4 matrix initialized with specific values. This method is not only simple but also improves code readability since the values are visible at a glance.
Using Nested Loops for Initialization
For scenarios where the array size is known but the values will be calculated at runtime, you can use nested loops for dynamic initialization. Here is how you can implement it:
int arr[3][4];
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 4; j++) {
arr[i][j] = i * 4 + j + 1; // Filling with sequential numbers
}
}
This example fills the array with sequential numbers from 1 to 12. Using loops allows for initialization based on logic, making this method particularly advantageous for larger arrays or when the elements are derived from complex calculations.
Using the `std::vector` for Dynamic Initialization
For cases where the size of the array may change or is not known at compile time, you can opt for `std::vector`, which is a part of the C++ Standard Template Library (STL). This method provides flexibility and automatically handles memory management.
Here’s how you can use `std::vector` to create a 2D array:
#include <vector>
std::vector<std::vector<int>> arr(3, std::vector<int>(4));
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 4; j++) {
arr[i][j] = i * 4 + j + 1;
}
}
In this code, we define a 2D vector that acts like a dynamic array. Each sub-vector can adjust its size independently. This approach simplifies handling more complex data manipulation scenarios.
Using `std::array` for a Fixed-Size Array
Another modern C++ alternative is `std::array`, which provides the benefits of both arrays and STL functionality while maintaining fixed sizes. Here's how it's done:
#include <array>
std::array<std::array<int, 4>, 3> arr = {{
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}
}};
The `std::array` approach offers bounds checking and eliminates many issues related to memory management often faced with traditional C-style arrays. This makes it a safer option while maintaining the benefits of fixed-size arrays.
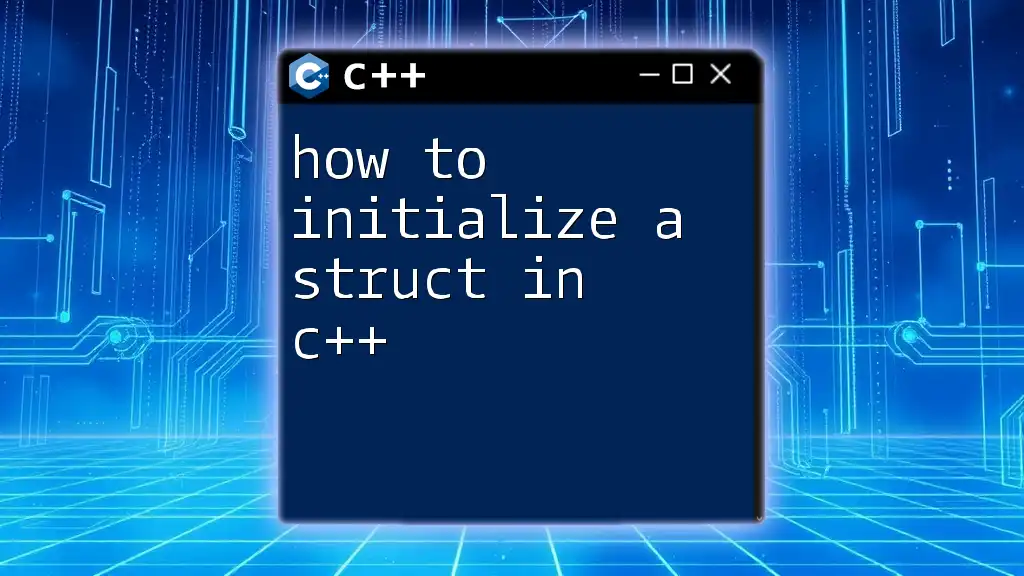
Accessing Elements of a 2D Array
Accessing elements in a 2D array is achieved through indexing, using two indices—one for the row and another for the column. The syntax looks like this:
std::cout << "Element at (1, 2): " << arr[1][2] << std::endl; // Outputs 7
It’s important to remember that arrays in C++ use zero-based indexing. This means the indices start from 0, so the first row is accessed with `arr[0]`, and the last index is always one less than the total number of rows or columns.
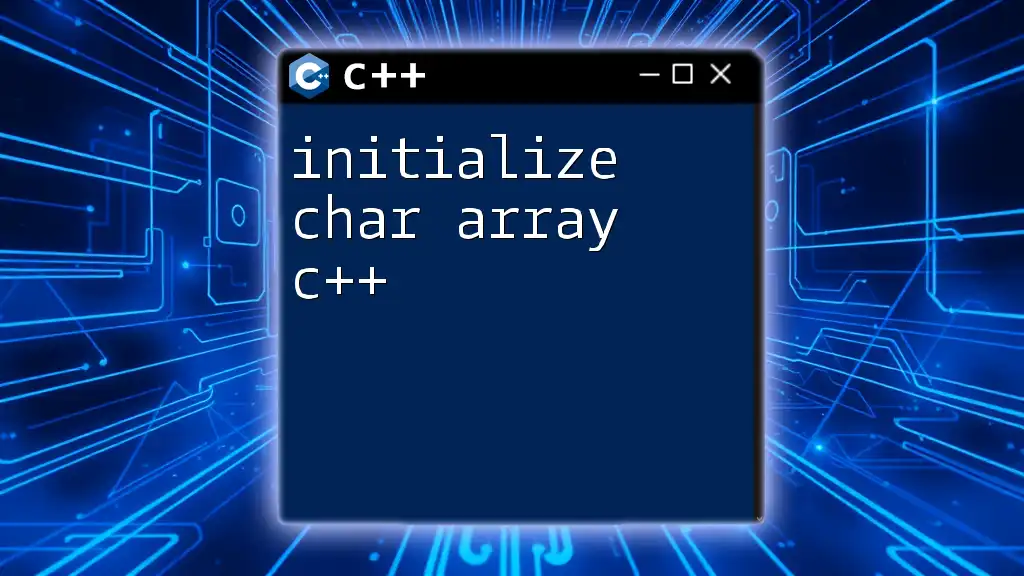
Important Considerations
When working with 2D arrays, there are a few essential considerations:
- Memory Management: Large arrays can consume significant memory. Be aware of stack vs. heap allocation; prefer dynamic arrays for larger datasets.
- Variable Sizes: C++ does not support variable-length arrays, so use structures like `std::vector` for truly dynamic needs.
- Error Handling: Always implement bounds checking to prevent access violations which can lead to undefined behavior.
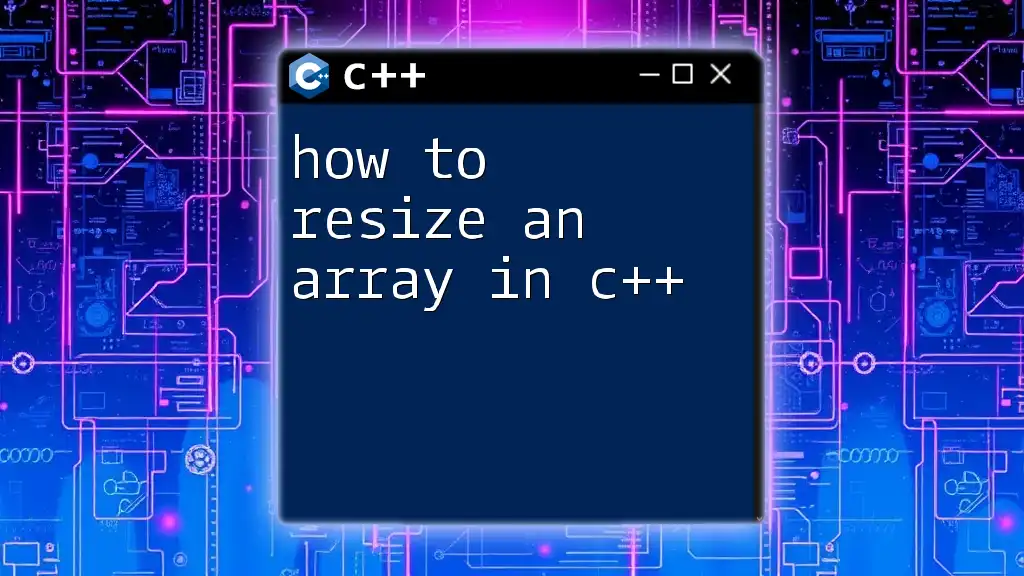
Conclusion
Knowing how to initialize a 2D array in C++ opens up a variety of programming possibilities, from scientific computations to game design. In this guide, we explored several methods to initialize 2D arrays, including static initialization at declaration, dynamic initialization using nested loops, and flexible options like `std::vector` and `std::array`. Each method has its advantages and best-use scenarios, providing you with tools to approach your programming tasks more intelligently.
Feel encouraged to practice the concepts presented here and experiment with various initialization techniques to deepen your understanding of 2D arrays in C++.