A multidimensional array in C++ is an array of arrays that enables the storage of data in a matrix format, allowing for efficient organization and manipulation of complex data structures.
#include <iostream>
int main() {
int matrix[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
for (int i = 0; i < 3; ++i) {
for (int j = 0; j < 3; ++j) {
std::cout << matrix[i][j] << " ";
}
std::cout << std::endl;
}
return 0;
}
Understanding Multidimensional Arrays
What is a Multidimensional Array?
A multidimensional array in C++ is an array that contains more than one index for each element. More commonly, you'll encounter 2D arrays, akin to matrices in mathematics, which allow for the storage of data in a tabular format. Unlike single-dimensional arrays that only extend in one direction, multidimensional arrays can extend in multiple dimensions — think of them as grids of data.
For instance, a 2D array can be visualized as a table with rows and columns. The fundamental difference between single-dimensional and multidimensional arrays is how the data is structured and accessed, highlighting the significance of indices in locating elements.
Syntax of Multidimensional Arrays in C++
The syntax for declaring a multidimensional array in C++ is relatively straightforward. The basic structure looks as follows:
datatype arrayName[size1][size2];
- datatype - This could be any valid C++ data type such as `int`, `float`, or `char`.
- arrayName - This is the identifier for your array.
- size1 and size2 - These define the number of rows and columns, respectively.
For example, to declare a 2D array named `matrix` of integers with 3 rows and 4 columns, you can write:
int matrix[3][4];
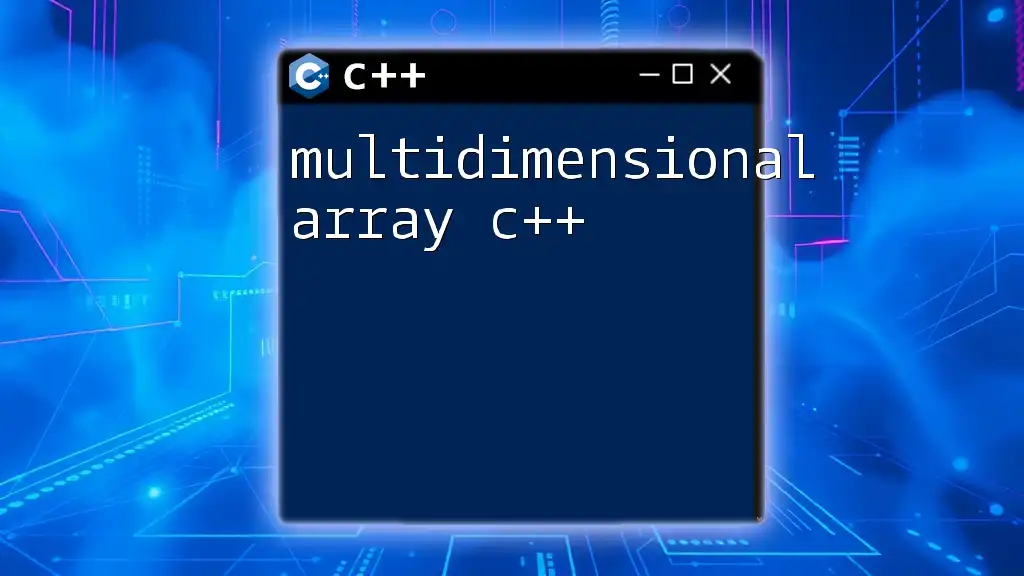
Declaring and Initializing Multidimensional Arrays
Declaration of Multidimensional Arrays
To declare a multidimensional array, simply specify its type and its dimensions. The declaration statement allocates memory for the specified dimensions, preparing the array for data storage. Consider this example:
int matrix[3][4]; // Declaration of a 3x4 integer matrix
In this declaration, the array can hold a total of 12 integers (3 rows * 4 columns).
Initialization of Multidimensional Arrays
Once declared, arrays can be initialized in various manners. The most straightforward method is static initialization, where you define initial values upon declaration. Here's how to do it:
int matrix[3][4] = { {1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12} };
In this example, each inner curly brace `{}` represents a row of the matrix, effectively filling the 2D array with specific integer values.
Dynamic Allocation of Multidimensional Arrays
Dynamic memory management provides flexibility, allowing arrays to be created at runtime. This is particularly useful when the size of the array is not known until execution. The following example demonstrates how to dynamically allocate a 2D array with pointers:
int** array = new int*[rows];
for(int i = 0; i < rows; i++)
array[i] = new int[cols];
In this snippet, we first allocate a 1D array of pointers to hold the rows. Each pointer is then allocated an array to hold the columns. It is crucial to manage memory efficiently. Consequently, when done with the array, use:
for(int i = 0; i < rows; i++)
delete[] array[i];
delete[] array;
This ensures there are no memory leaks by properly deallocating the memory once the array is no longer needed.
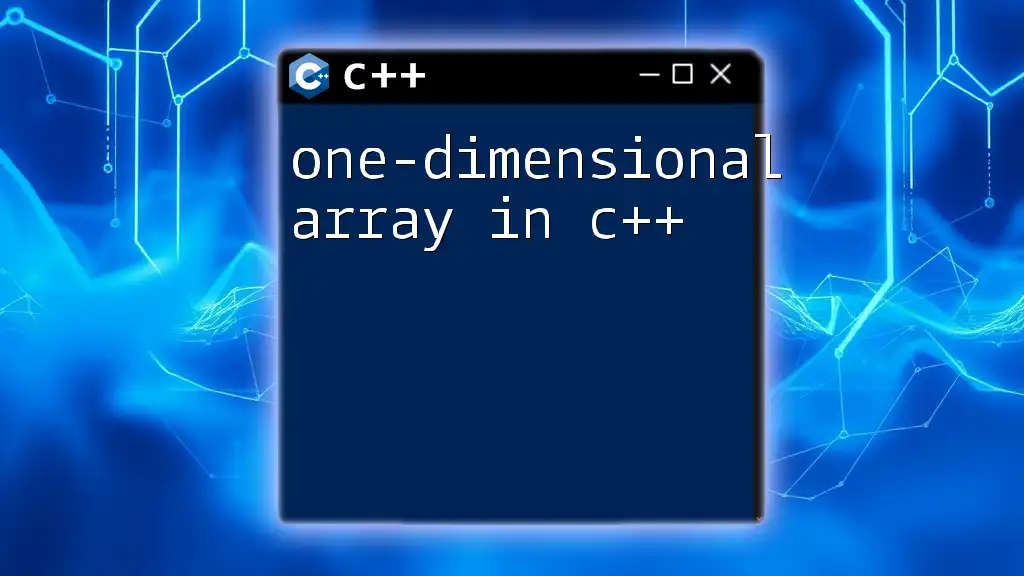
Accessing Elements in Multidimensional Arrays
Indexing in Multidimensional Arrays
In C++, multidimensional arrays utilize a zero-based indexing system. Therefore, the first element in a `2D array` can be accessed using both the row and column index, like this:
int value = matrix[1][2]; // Accessing the element at row 1, column 2
This retrieves the appropriate integer from the second row and third column (keeping in mind that indexing begins at 0).
Traversing Multidimensional Arrays
To process or display all elements within a multidimensional array, you often employ nested loops. The outer loop iterates through the rows, while the inner loop visits each column within those rows. Here's a practical example using a nested loop to display the elements of `matrix` initialized previously:
for(int i = 0; i < 3; i++) {
for(int j = 0; j < 4; j++) {
cout << matrix[i][j] << " ";
}
cout << endl;
}
This code snippet iterates over each element of the matrix and outputs it to the console, achieving a clear presentation of the 2D array’s contents.
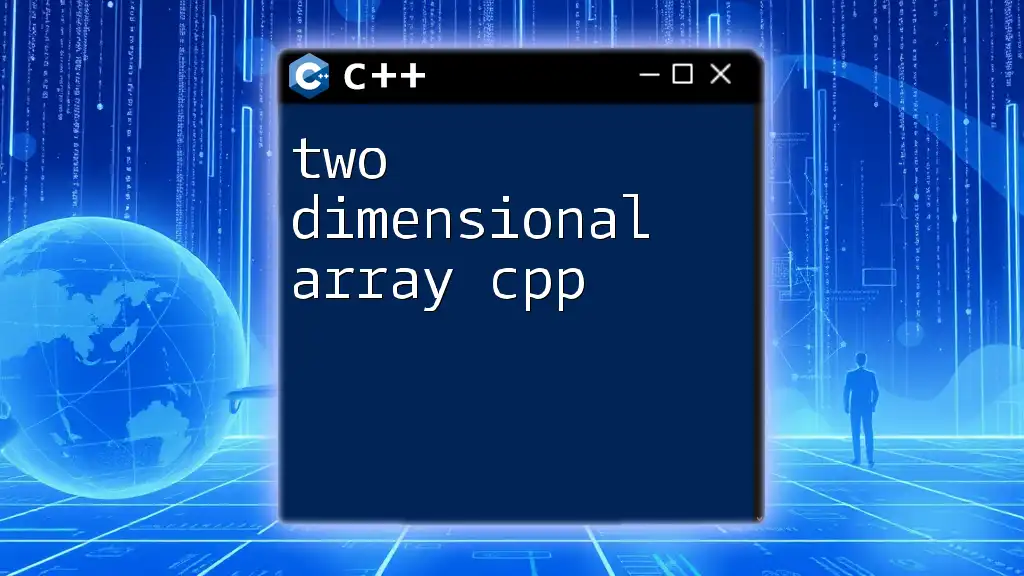
Applications of Multidimensional Arrays
Mathematical Operations
Multidimensional arrays serve essential roles in mathematical computations, particularly for operations involving matrices. For instance, consider you want to add two matrices. You start by defining them as follows:
int a[2][2] = {{1, 2}, {3, 4}};
int b[2][2] = {{5, 6}, {7, 8}};
To add matrices `a` and `b`, you can also utilize nested loops, creating a third matrix to store the results:
int result[2][2];
for(int i = 0; i < 2; i++) {
for(int j = 0; j < 2; j++) {
result[i][j] = a[i][j] + b[i][j];
}
}
This straightforward operation displays how multidimensional arrays facilitate mathematical operations efficiently.
Storing Complex Data Structures
Additionally, multidimensional arrays can store complex data structures, including, but not limited to, graphs and images. For example, a black-and-white image can be represented as a 2D array, where each pixel is either black (0) or white (1). This conceptualization allows for simplified image processing and manipulation using C++'s array structures.
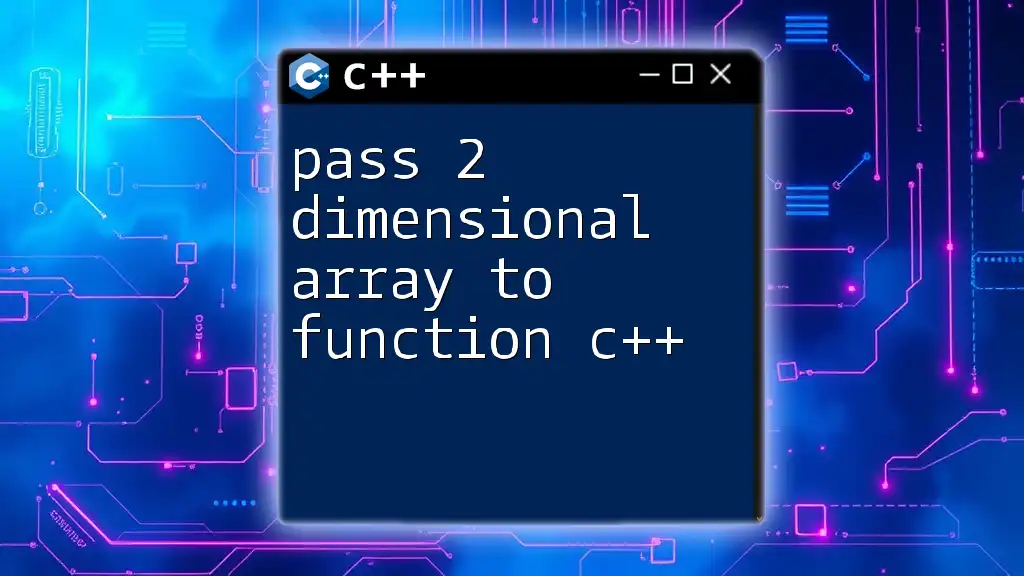
Common Pitfalls and Best Practices
Common Issues with Multidimensional Arrays
When working with multidimensional arrays, be wary of common mistakes such as misunderstanding indexing, which often leads to accessing out-of-bounds elements. Such errors can result in undefined behavior, making debugging a frustrating process.
Best Practices for Using Multidimensional Arrays
-
Use `const` for Array Sizes: To prevent accidental changes, declare array sizes as constants, enhancing code readability and maintainability.
-
Initialize All Elements if Needed: Uninitialized array elements may contain garbage values, leading to unpredictable behavior.
-
Consider Using Data Structures Like `std::vector`: If flexibility is essential, prefer using `std::vector`, which automatically handles memory management and allows dynamic resizing.
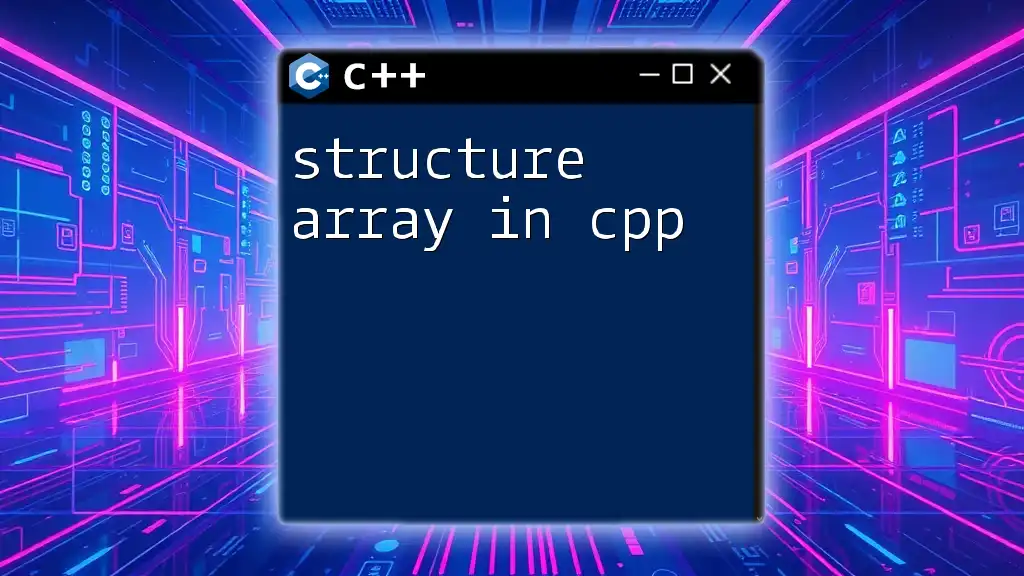
Conclusion
In summary, the multidimensional array in C++ is a powerful structure that facilitates complex data organization and manipulation across multiple dimensions. From mathematical operations to data representation, its versatility allows programmers to handle a wide array of tasks efficiently. To master this versatile tool, practice with real-world examples and explore advanced topics like 3D arrays and `STL` containers for a deeper understanding and greater programming capability.