To find the largest number that can be represented in C++, you can utilize the `numeric_limits` class from the `<limits>` header, which provides a portable way to get the maximum value for a specific numeric type.
Here's a code snippet demonstrating how to find the largest number for the `int` type:
#include <iostream>
#include <limits>
int main() {
std::cout << "The largest number that can be represented by an int: "
<< std::numeric_limits<int>::max() << std::endl;
return 0;
}
Understanding Basic Data Types in C++
Importance of Data Types in C++
In C++, data types play a crucial role as they define the amount of memory allocated for a variable and the operations that can be performed on it. Understanding the data types helps in optimizing performance and utilizing system resources effectively.
Overview of Numeric Data Types
C++ provides several numeric data types:
-
Integer Types:
- `int`: Typically 4 bytes, signed.
- `short`: Usually 2 bytes, signed.
- `long`: Usually 4 or 8 bytes, signed, varies with system.
- `long long`: Guaranteed to be at least 8 bytes, signed.
-
Floating Point Types:
- `float`: Single precision, usually 4 bytes.
- `double`: Double precision, usually 8 bytes.
- `long double`: Extended precision, varies by compiler implementation.
Understanding these types is essential when looking for the biggest number in cpp, as each type has its own limits.
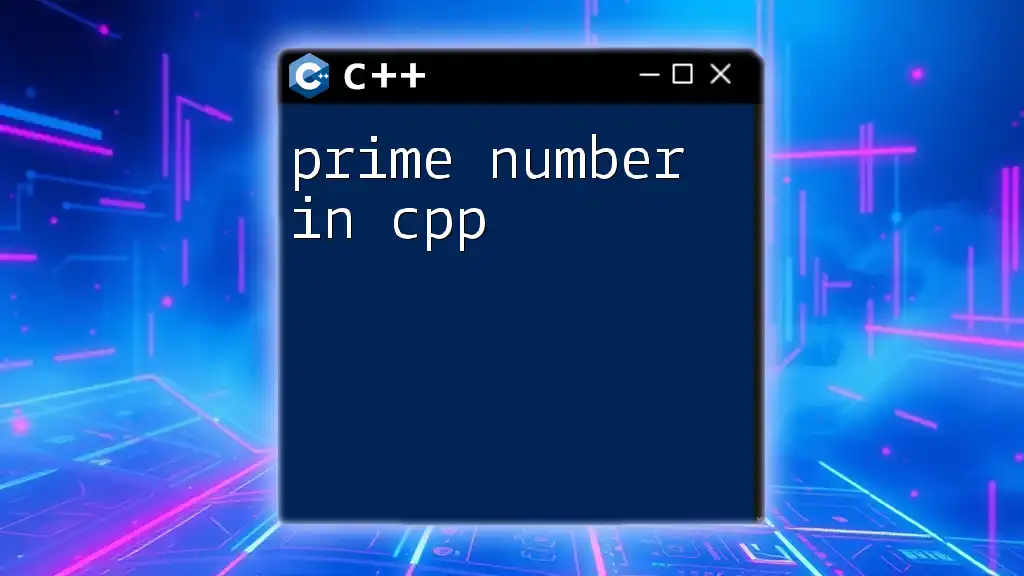
The Concept of Maximum Values in C++
What is a Maximum Value?
In programming, a maximum value refers to the highest value that can be stored within a given data type. For instance, trying to store a value larger than the maximum for an `int` could lead to overflow, resulting in undefined behavior.
Differences among Data Types
Each numeric data type has a distinct range of maximum values. For example:
- The maximum value of an `int` is generally 2,147,483,647.
- The maximum value of a `long` might be 9,223,372,036,854,775,807, depending on the architecture.
This variance highlights the need to choose the appropriate data type, particularly when working with large data sets or performing calculations that may exceed standard limits.
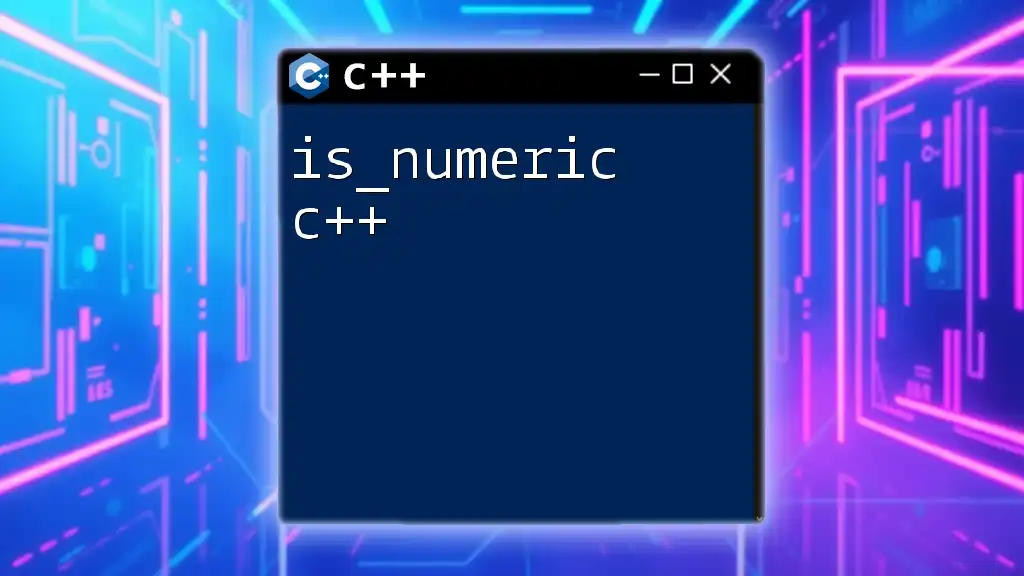
Using `<limits>` Library in C++
Introduction to `<limits>`
The `<limits>` library in C++ provides a standardized way to obtain the maximum or minimum value for built-in data types. This is essential for ensuring your code functions correctly across different systems and compilers.
Finding Maximum Values
To find the maximum values of various data types, you can use the `std::numeric_limits` template. Here’s how you can utilize it:
#include <limits>
#include <iostream>
int main() {
std::cout << "Max int: " << std::numeric_limits<int>::max() << std::endl;
std::cout << "Max float: " << std::numeric_limits<float>::max() << std::endl;
std::cout << "Max double: " << std::numeric_limits<double>::max() << std::endl;
return 0;
}
This code snippet outputs the maximum values for `int`, `float`, and `double`, showcasing the powerful utility of `<limits>`.
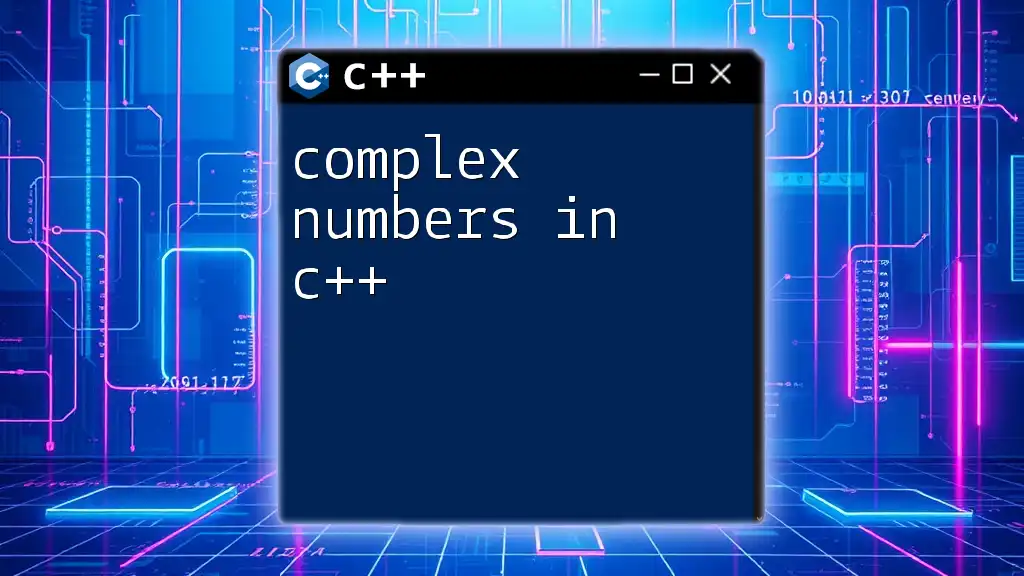
Implementing a Function to Find the Biggest Number
Creating a Function to Compare Two Numbers
In C++, you can create a simple function that accepts two parameters and returns the larger of the two. This lays the groundwork for more complex comparisons.
template <typename T>
T findMax(T a, T b) {
return (a > b) ? a : b;
}
Extending the Function to Compare Multiple Numbers
You can extend the concept to an array of values, allowing you to find the largest number from multiple inputs efficiently.
template <typename T>
T findMax(T arr[], int length) {
T maxVal = arr[0];
for (int i = 1; i < length; i++) {
if (arr[i] > maxVal) {
maxVal = arr[i];
}
}
return maxVal;
}
Example Usage of the `findMax` Function
Here’s how to utilize the `findMax` function in practice:
#include <iostream>
int main() {
int nums[] = {1, 5, 3, 9, 2};
std::cout << "Max is: " << findMax(nums, 5) << std::endl;
return 0;
}
This code will display the largest number from the array, showcasing the utility of your function.
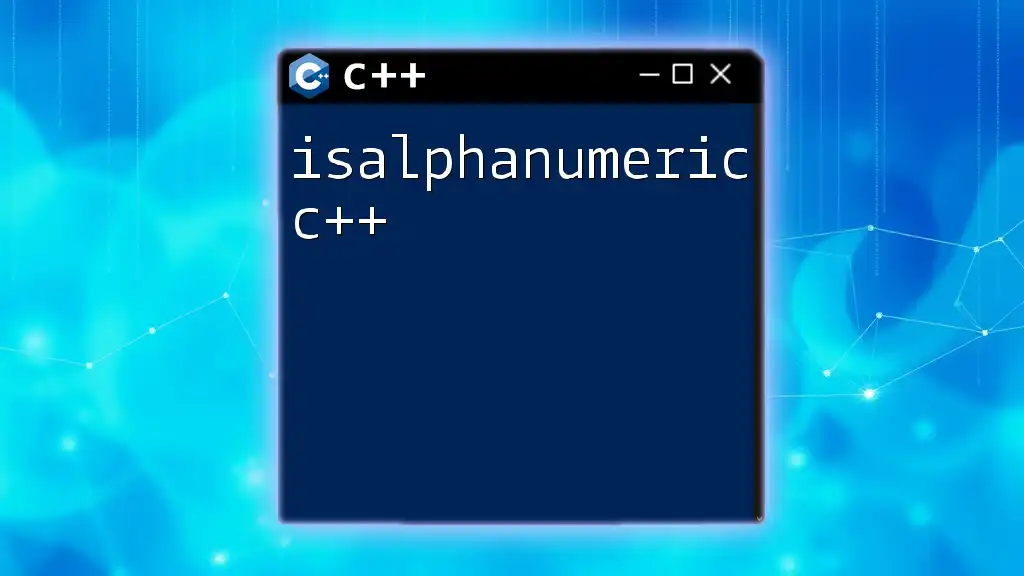
Using the Standard Template Library (STL)
Introduction to STL Algorithms
The Standard Template Library (STL) is an essential aspect of C++ programming, containing a set of template classes and functions. Among them are various algorithms that can simplify and optimize tasks, such as finding the maximum value.
The `std::max_element` Function
One of the most useful features of STL is the `std::max_element` function that directly allows you to find the largest value in a collection.
Example of Finding the Max Element in a Container
Using `std::max_element`, you can easily retrieve the maximum value from a vector:
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> numbers = {10, 50, 30, 70};
auto maxIt = std::max_element(numbers.begin(), numbers.end());
std::cout << "Max using STL: " << *maxIt << std::endl;
return 0;
}
This demonstrates the efficiency of STL in finding the biggest number in cpp without writing complex code.
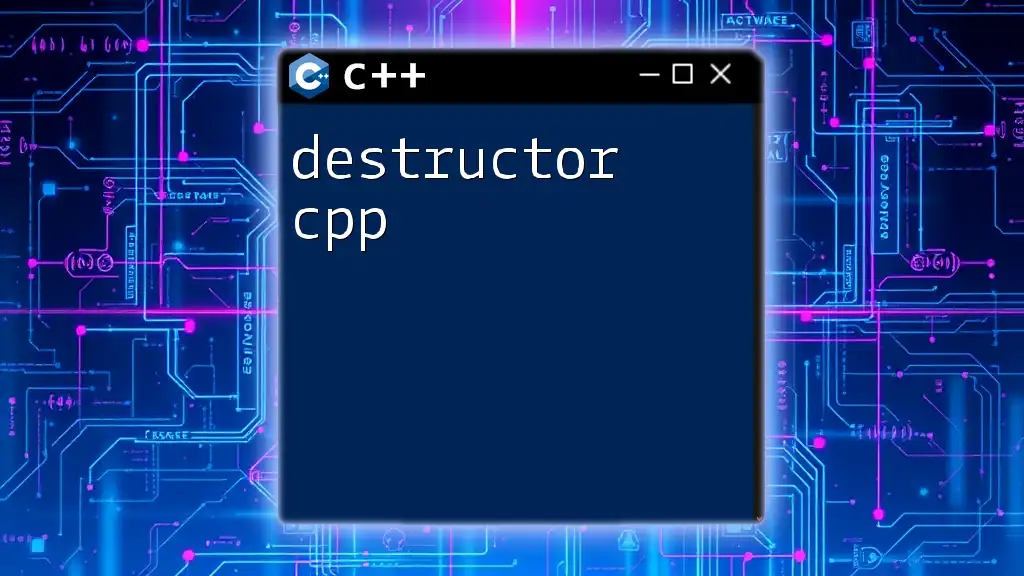
Comparing Performance Among Different Methods
Time Complexity Analysis
The performance of different approaches can vary significantly. The custom `findMax` function has a time complexity of O(n), where n is the number of elements being examined. In contrast, the STL `std::max_element` function is also O(n) but may be more optimized internally, providing better performance in larger data sets.
Pros and Cons of Each Method
- Custom Function: More control, understanding of the algorithm can be beneficial, ideally suited for tailored solutions.
- STL Algorithms: Simplifies code, likely better optimized by the compiler, generally recommended for standard tasks.
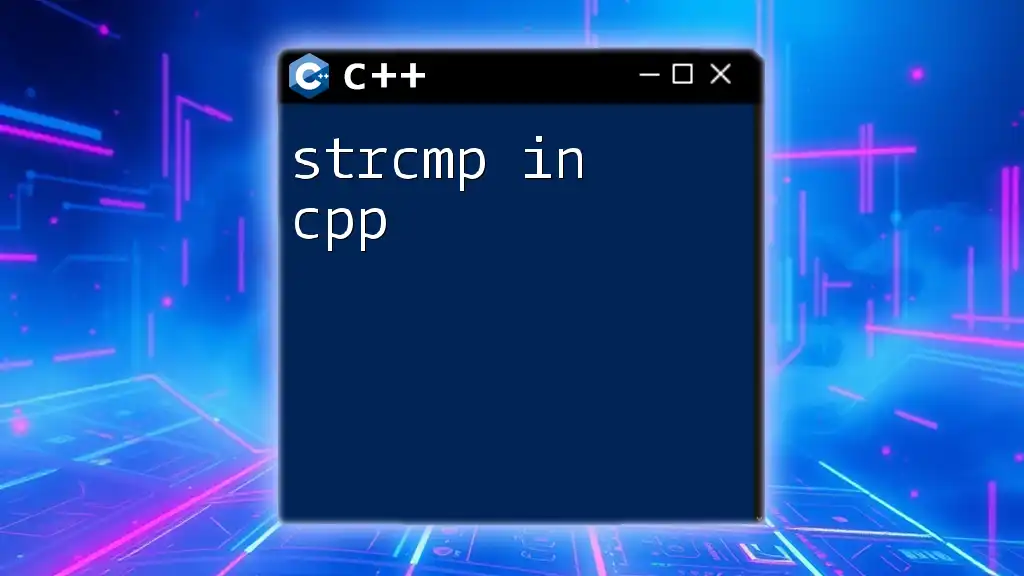
Practical Application of Finding the Biggest Number
Real-World Scenarios
Finding the maximum value has extensive applications in fields such as data analysis, gaming, and statistical modeling. For example, you might need it to determine the highest score in a game or perform statistical analysis where identifying extremes is crucial.
Importance in Algorithms
The concept of finding maximum values is integral in many algorithms, including sorting (like quicksort) and searching algorithms. Properly handling maximum values can greatly influence the correctness and efficiency of these algorithms.
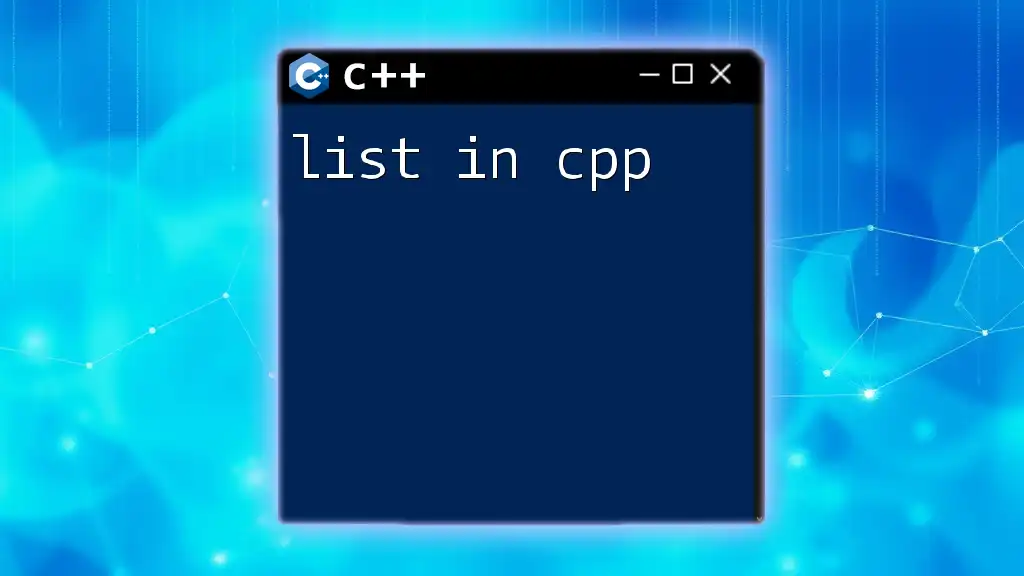
Conclusion
Summary of Key Points
Throughout this guide, we explored various methods to determine the biggest number in cpp, understanding different data types, utilizing the `<limits>` library, implementing custom functions, and leveraging STL optimally.
Encouragement for Further Exploration
This knowledge lays the foundation for advanced programming techniques in C++. From here, consider exploring how to handle negative numbers effectively or diving into algorithms for finding minimum values.
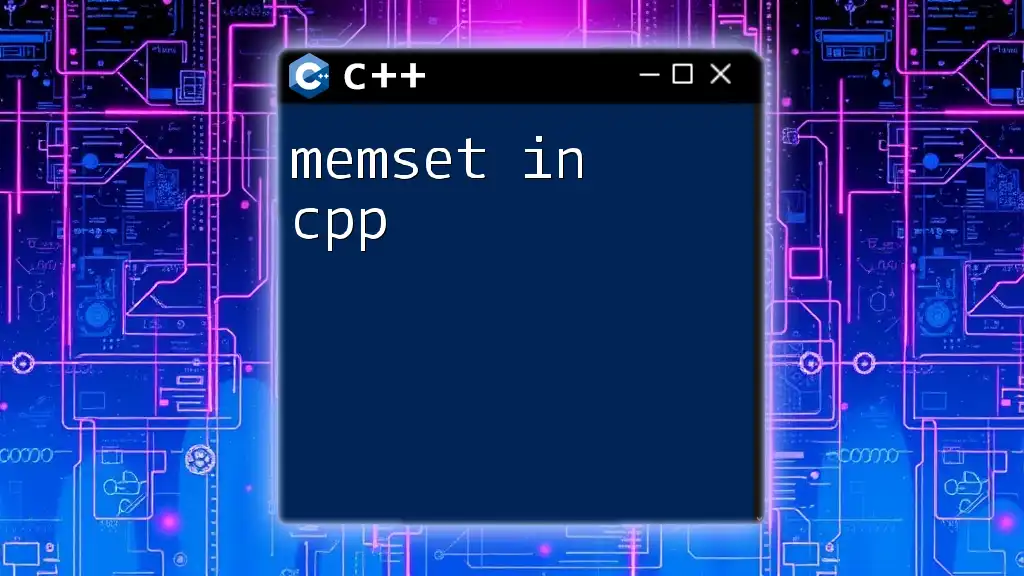
Call to Action
If you found this article helpful, consider subscribing for more insights and concise tutorials on using C++ effectively!