In C++, you can convert a string to a number using the `std::stoi` function for integers or `std::stod` for double precision, as demonstrated in the following code snippet:
#include <string>
#include <iostream>
int main() {
std::string str = "42";
int num = std::stoi(str);
std::cout << "The number is: " << num << std::endl;
return 0;
}
Understanding the Need for Conversion
In programming, particularly in C++, there are times when we need to convert a string into a numeric representation. Knowing how to efficiently convert strings to numbers is crucial in scenarios such as calculations, user input processing, or when handling data from external sources like files or APIs. By understanding conversion from string to number in C++, you open up possibilities for more robust data manipulation and application functionality.
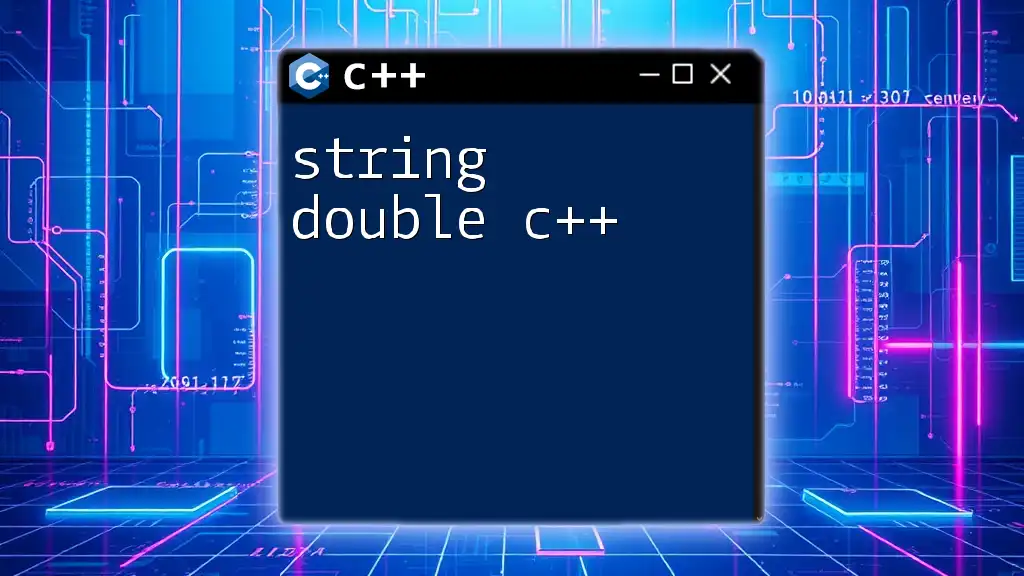
What is a String in C++?
A string in C++ can be defined as a sequence of characters. It can be represented in two primary forms:
- `std::string`: This is a part of the Standard Template Library and provides dynamic memory management, making it easier to manipulate string data without worrying about memory allocations.
- C-style strings: These are arrays of characters terminated by a null character. They can be less convenient but are important to know for legacy code and certain interfacing situations.
Understanding these representations is foundational when we delve into conversions from string to number.
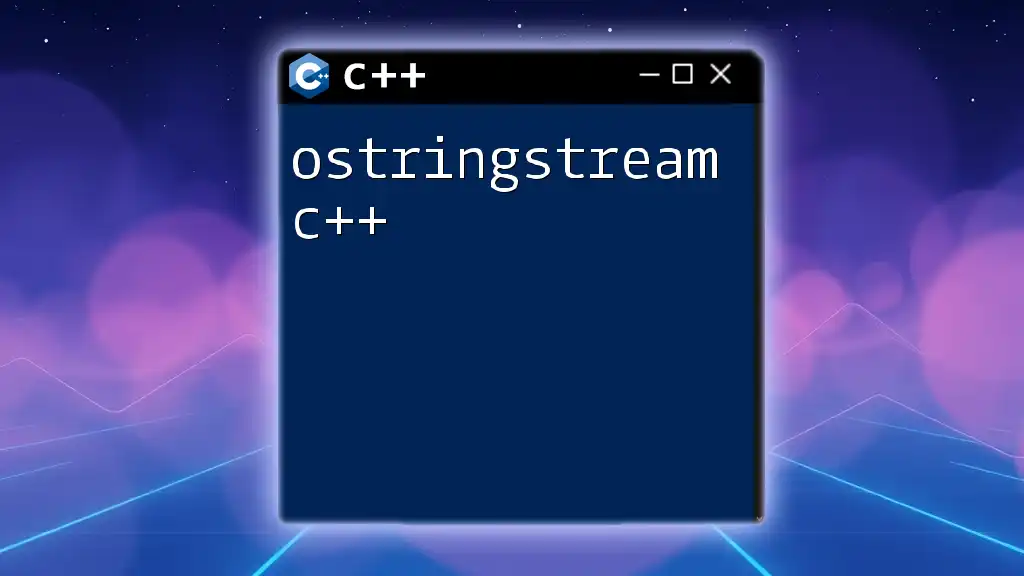
Why Convert Strings to Numbers?
Converting strings to numbers is vital for various tasks like:
- Performing arithmetic operations upon user input or file data.
- Aggregating numeric results from string-based data sources.
- Implementing functionality that requires numeric comparisons or measurements.
These operations illustrate the practical necessity of converting strings into integers (or other numeric types) in C++.
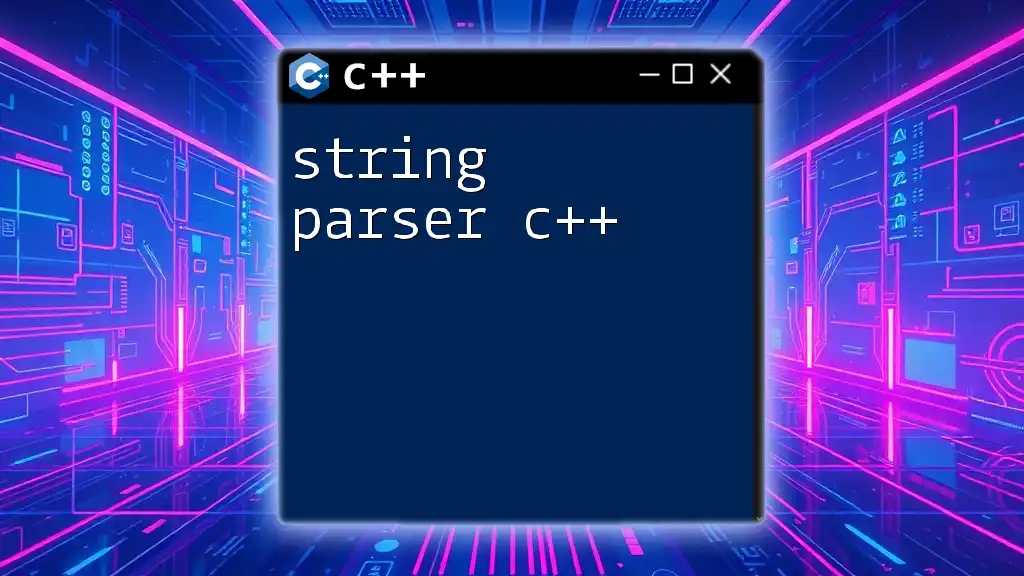
Using `std::stoi` for Conversion
Overview of `std::stoi` Function
The `std::stoi` (String to Integer) function is a straightforward and commonly used method to convert strings to integers in C++. This function not only converts a string to an integer but also provides error handling features through exceptions.
Syntax and Parameters
The function can be declared as follows:
int std::stoi(const std::string& str, std::size_t* idx = 0, int base = 10);
- `str`: The string to convert.
- `idx`: An optional pointer that will point to the character after the last character used in the conversion (if provided).
- `base`: The base for the conversion (default is base 10).
Example Code
Here’s how to use `std::stoi` effectively:
#include <iostream>
#include <string>
int main() {
std::string str = "1234";
int num = std::stoi(str);
std::cout << "Converted number: " << num << std::endl;
return 0;
}
In this example, the string `"1234"` is successfully converted to the integer `1234` and printed to the console.
Handling Exceptions with `std::stoi`
Using `std::stoi` also means you need to handle possible exceptions to ensure the code runs smoothly. The two most common exceptions are:
- `std::invalid_argument`: Raised when the input string cannot be converted to an integer.
- `std::out_of_range`: Raised when the converted integer is outside the range of representable values.
Example Code with Exception Handling
Consider the below example that demonstrates how to manage exceptions:
#include <iostream>
#include <string>
int main() {
std::string str = "abc"; // Invalid integer
try {
int num = std::stoi(str);
std::cout << "Converted number: " << num << std::endl;
} catch (std::invalid_argument& e) {
std::cout << "Invalid input: " << e.what() << std::endl;
} catch (std::out_of_range& e) {
std::cout << "Input is out of range: " << e.what() << std::endl;
}
return 0;
}
In this scenario, since `"abc"` cannot be converted to an integer, the program catches the `std::invalid_argument` exception and prints an error message.
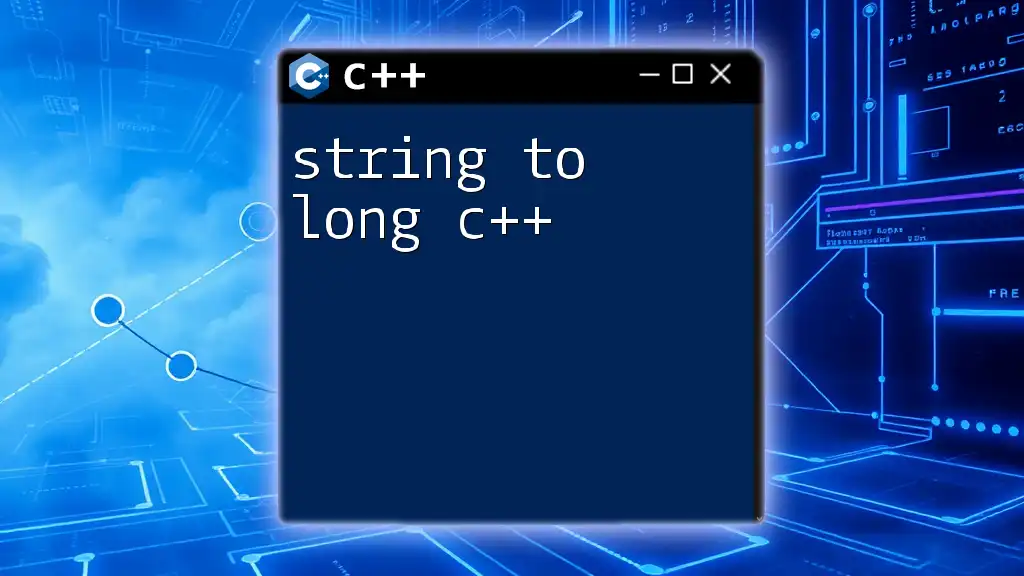
C-style Conversion with `atoi`
Overview
The `atoi` function is a C-style function that also converts a string to an integer. While easy to use, `atoi` does not handle errors in a way that throws exceptions, so it’s vital to ensure the string is valid prior to calling it.
Example Code
Here is how to use `atoi`:
#include <iostream>
#include <cstdlib>
int main() {
const char* str = "5678";
int num = std::atoi(str);
std::cout << "Converted number: " << num << std::endl;
return 0;
}
In this case, the string is successfully converted, yielding the output `5678`.
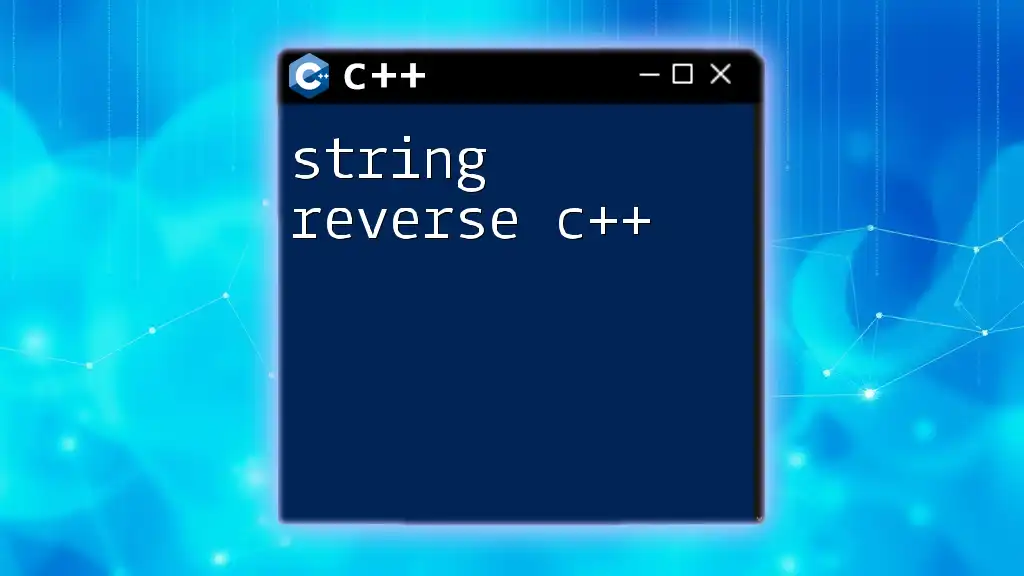
Using `stringstream` for Conversion
Overview of `stringstream`
The `stringstream` class provides another method to convert strings to numerical types. This method can be particularly helpful when you need to parse not just integers but also floats or other types.
Example Code
Here’s how to utilize `stringstream` for conversion:
#include <iostream>
#include <sstream>
#include <string>
int main() {
std::string str = "91011";
int num;
std::stringstream(str) >> num;
std::cout << "Converted number: " << num << std::endl;
return 0;
}
This approach uses the stream extraction operator `>>` to parse the integer from the string within `stringstream`.
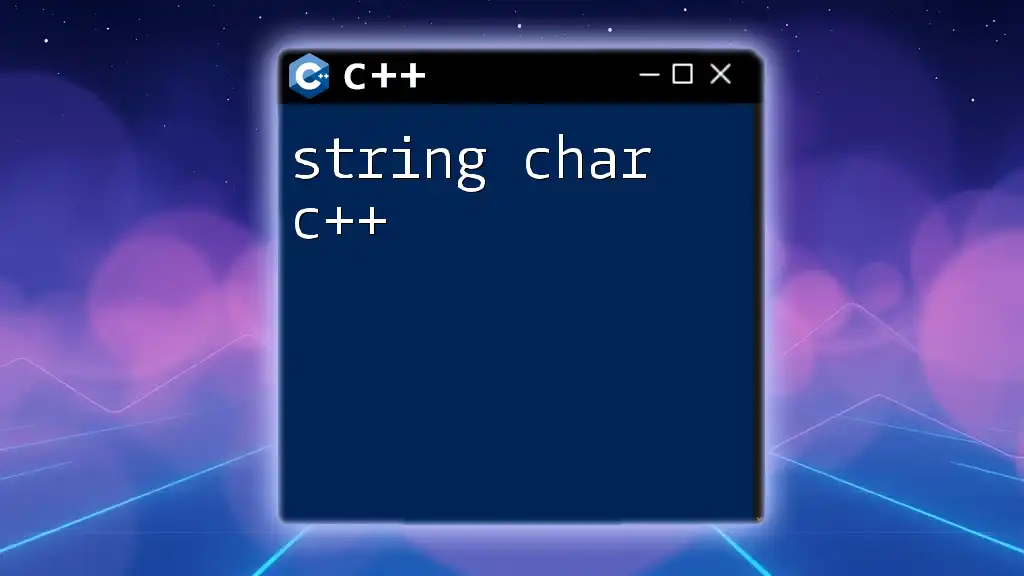
Comparing Performance of Different Methods
When considering conversion methods, it’s essential to measure their performance in different contexts. For example, `std::stoi` is typically preferred for its built-in error handling and ease of use. Conversely, `atoi` may be more suitable for performance-critical applications due to its simpler design, but without proper input validation, it might lead to incorrect behavior.
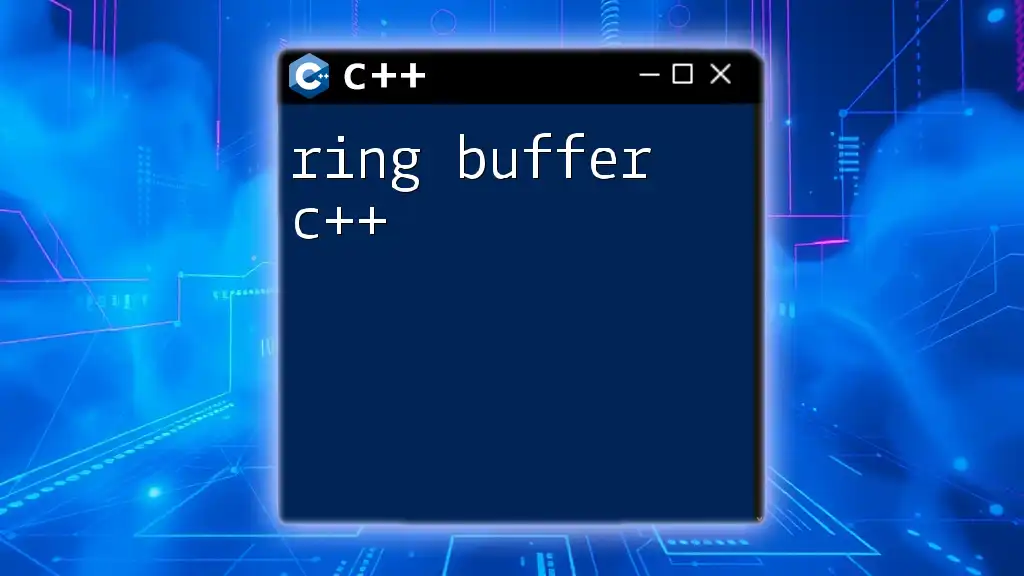
Common Pitfalls and Troubleshooting
Input Validation
Always validate your input strings before conversion. This practice ensures that only well-formed strings make it through the conversion process, reducing the risk of exceptions or undefined behavior.
Debugging Conversion Errors
When debugging conversion issues, consider employing logging or print statements to show the state of the string being converted. By doing so, you can pinpoint where invalid values may be affecting your results.
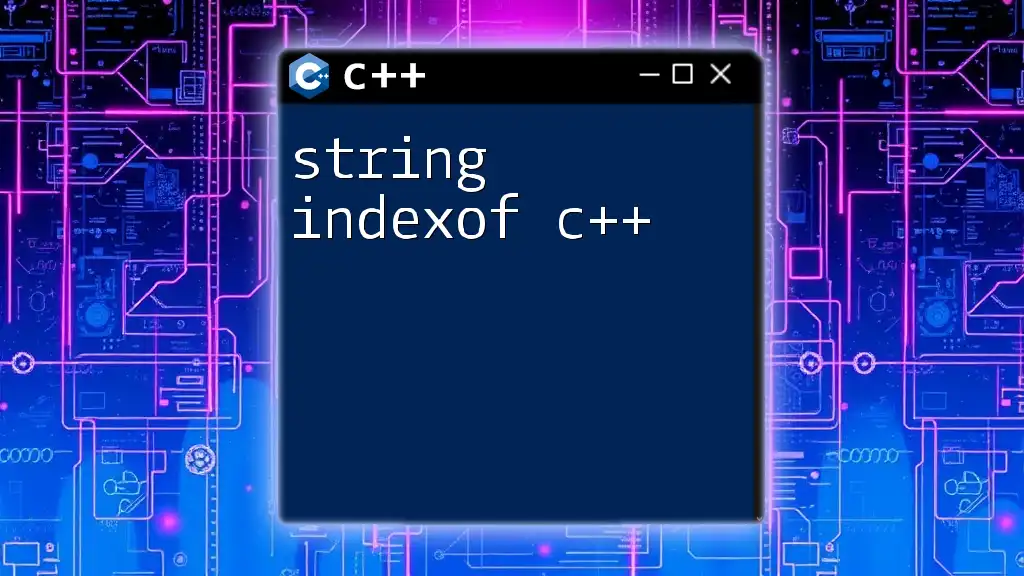
Recap of String to Number Conversion Techniques in C++
In conclusion, understanding how to convert strings to numbers in C++ is an essential skill. Utilizing functions like `std::stoi`, `atoi`, and `stringstream` allows for flexible and robust string manipulation to suit various needs within your applications. Whether you're processing user input, reading from files, or managing data APIs, mastering these conversion techniques is crucial for effective C++ development.
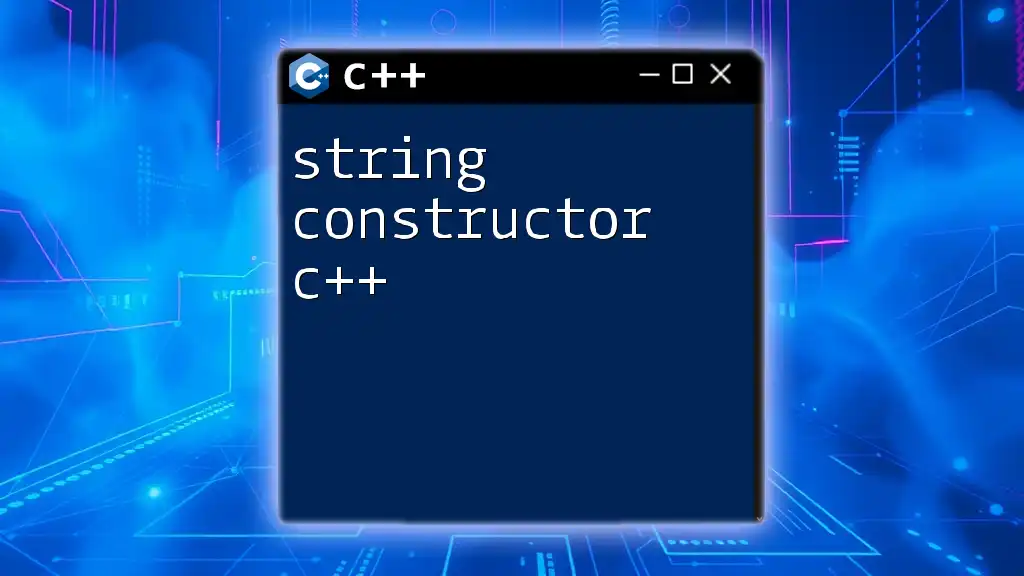
Additional Resources
For further learning on string manipulation in C++, consider referring to the official C++ documentation and resources dedicated to deepening your understanding of the Standard Template Library and string processing functions.